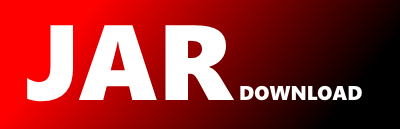
temper.std.regex.RegexFormatter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of temper-std Show documentation
Show all versions of temper-std Show documentation
Optional support library provided with Temper
package temper.std.regex;
import temper.core.Core;
import java.util.Objects;
import temper.core.Nullable;
import temper.core.Core.CodePointStringSlice;
import java.util.List;
import static temper.std.regex.RegexGlobal.Word;
import static temper.std.regex.RegexGlobal.Digit;
import static temper.std.regex.RegexGlobal.Space;
import java.util.function.Function;
import java.util.ArrayList;
import static temper.std.regex.RegexGlobal.Dot;
import static temper.std.regex.RegexGlobal.End;
import static temper.std.regex.RegexGlobal.Begin;
import static temper.std.regex.RegexGlobal.WordBoundary;
import static temper.std.regex.RegexGlobal.regexRefs__117;
final class RegexFormatter {
final List out;
public String format(Regex regex__229) {
this.pushRegex(regex__229);
List t_1141 = this.out;
Function fn__1138 = x__231 -> {
return x__231;
};
return Core.listJoinObj(t_1141, "", fn__1138);
}
void pushRegex(Regex regex__233) {
boolean t_744;
Capture t_745;
boolean t_748;
CodePoints t_749;
boolean t_752;
CodeRange t_753;
boolean t_756;
CodeSet t_757;
boolean t_760;
Or t_761;
boolean t_764;
Repeat t_765;
boolean t_768;
Sequence t_769;
try {
Core.cast(Capture.class, regex__233);
t_744 = true;
} catch (RuntimeException ignored$1) {
t_744 = false;
}
s__1258_1259: {
if (t_744) {
try {
t_745 = Core.cast(Capture.class, regex__233);
} catch (RuntimeException ignored$2) {
break s__1258_1259;
}
this.pushCapture(t_745);
} else {
try {
Core.cast(CodePoints.class, regex__233);
t_748 = true;
} catch (RuntimeException ignored$3) {
t_748 = false;
}
if (t_748) {
try {
t_749 = Core.cast(CodePoints.class, regex__233);
} catch (RuntimeException ignored$4) {
break s__1258_1259;
}
this.pushCodePoints(t_749, false);
} else {
try {
Core.cast(CodeRange.class, regex__233);
t_752 = true;
} catch (RuntimeException ignored$5) {
t_752 = false;
}
if (t_752) {
try {
t_753 = Core.cast(CodeRange.class, regex__233);
} catch (RuntimeException ignored$6) {
break s__1258_1259;
}
this.pushCodeRange(t_753);
} else {
try {
Core.cast(CodeSet.class, regex__233);
t_756 = true;
} catch (RuntimeException ignored$7) {
t_756 = false;
}
if (t_756) {
try {
t_757 = Core.cast(CodeSet.class, regex__233);
} catch (RuntimeException ignored$8) {
break s__1258_1259;
}
this.pushCodeSet(t_757);
} else {
try {
Core.cast(Or.class, regex__233);
t_760 = true;
} catch (RuntimeException ignored$9) {
t_760 = false;
}
if (t_760) {
try {
t_761 = Core.cast(Or.class, regex__233);
} catch (RuntimeException ignored$10) {
break s__1258_1259;
}
this.pushOr(t_761);
} else {
try {
Core.cast(Repeat.class, regex__233);
t_764 = true;
} catch (RuntimeException ignored$11) {
t_764 = false;
}
if (t_764) {
try {
t_765 = Core.cast(Repeat.class, regex__233);
} catch (RuntimeException ignored$12) {
break s__1258_1259;
}
this.pushRepeat(t_765);
} else {
try {
Core.cast(Sequence.class, regex__233);
t_768 = true;
} catch (RuntimeException ignored$13) {
t_768 = false;
}
if (t_768) {
try {
t_769 = Core.cast(Sequence.class, regex__233);
} catch (RuntimeException ignored$14) {
break s__1258_1259;
}
this.pushSequence(t_769);
} else if (Objects.equals(regex__233, Begin)) {
try {
Core.listAdd(this.out, "^");
} catch (RuntimeException ignored$15) {
break s__1258_1259;
}
} else if (Objects.equals(regex__233, Dot)) {
try {
Core.listAdd(this.out, ".");
} catch (RuntimeException ignored$16) {
break s__1258_1259;
}
} else if (Objects.equals(regex__233, End)) {
try {
Core.listAdd(this.out, "$");
} catch (RuntimeException ignored$17) {
break s__1258_1259;
}
} else if (Objects.equals(regex__233, WordBoundary)) {
try {
Core.listAdd(this.out, "\\b");
} catch (RuntimeException ignored$18) {
break s__1258_1259;
}
} else if (Objects.equals(regex__233, Digit)) {
try {
Core.listAdd(this.out, "\\d");
} catch (RuntimeException ignored$19) {
break s__1258_1259;
}
} else if (Objects.equals(regex__233, Space)) {
try {
Core.listAdd(this.out, "\\s");
} catch (RuntimeException ignored$20) {
break s__1258_1259;
}
} else if (Objects.equals(regex__233, Word)) {
try {
Core.listAdd(this.out, "\\w");
} catch (RuntimeException ignored$21) {
break s__1258_1259;
}
} else {
/* null: Literal expression statement */
}
}
}
}
}
}
}
return;
}
throw Core.bubble();
}
void pushCapture(Capture capture__236) {
String t_1125;
Regex t_1126;
List t_739;
{
Core.listAdd(this.out, "(");
t_739 = this.out;
t_1125 = capture__236.getName();
this.pushCaptureName(t_739, t_1125);
t_1126 = capture__236.getItem();
this.pushRegex(t_1126);
Core.listAdd(this.out, ")");
}
}
void pushCaptureName(List out__239, String name__240) {
Core.listAdd(out__239, "?<" + name__240 + ">");
}
void pushCode(int code__243, boolean insideCodeSet__244) {
temper.std.regex.Core.regexFormatterPushCodeTo(this, this.out, code__243, insideCodeSet__244);
}
void pushCodePoints(CodePoints codePoints__252, boolean insideCodeSet__253) {
int t_1114;
CodePointStringSlice t_1115;
CodePointStringSlice t_1119 = Core.stringCodePoints(codePoints__252.getValue());
CodePointStringSlice slice__255 = t_1119;
while (true) {
if (!slice__255.isEmpty()) {
t_1114 = slice__255.read();
this.pushCode(t_1114, insideCodeSet__253);
t_1115 = slice__255.advance(1);
slice__255 = t_1115;
} else {
break;
}
}
}
void pushCodeRange(CodeRange codeRange__257) {
Core.listAdd(this.out, "[");
this.pushCodeRangeUnwrapped(codeRange__257);
Core.listAdd(this.out, "]");
}
void pushCodeRangeUnwrapped(CodeRange codeRange__260) {
int t_1109;
int t_1107 = codeRange__260.getMin();
this.pushCode(t_1107, true);
{
Core.listAdd(this.out, "-");
t_1109 = codeRange__260.getMax();
this.pushCode(t_1109, true);
}
}
void pushCodeSet(CodeSet codeSet__263) {
int t_1103;
boolean t_717;
CodeSet t_718;
CodePart t_723;
Regex adjusted__265 = this.adjustCodeSet(codeSet__263, regexRefs__117);
try {
Core.cast(CodeSet.class, adjusted__265);
t_717 = true;
} catch (RuntimeException ignored$22) {
t_717 = false;
}
s__1263_1265: {
if (t_717) {
s__1264_1266: {
try {
t_718 = Core.cast(CodeSet.class, adjusted__265);
Core.listAdd(this.out, "[");
} catch (RuntimeException ignored$23) {
break s__1264_1266;
}
if (t_718.isNegated()) {
try {
Core.listAdd(this.out, "^");
} catch (RuntimeException ignored$24) {
break s__1264_1266;
}
} else {
/* null: Literal expression statement */
}
int i__266 = 0;
while (true) {
t_1103 = t_718.getItems().size();
if (i__266 < t_1103) {
try {
t_723 = Core.listGet(t_718.getItems(), i__266);
} catch (RuntimeException ignored$25) {
break s__1264_1266;
}
this.pushCodeSetItem(t_723);
i__266 = i__266 + 1;
} else {
break;
}
}
try {
Core.listAdd(this.out, "]");
break s__1263_1265;
} catch (RuntimeException ignored$26) {
}
}
throw Core.bubble();
}
this.pushRegex(adjusted__265);
}
}
Regex adjustCodeSet(CodeSet codeSet__268, RegexRefs regexRefs__269) {
return codeSet__268;
}
void pushCodeSetItem(CodePart codePart__272) {
boolean t_704;
CodePoints t_705;
boolean t_708;
CodeRange t_709;
boolean t_712;
SpecialSet t_713;
try {
Core.cast(CodePoints.class, codePart__272);
t_704 = true;
} catch (RuntimeException ignored$27) {
t_704 = false;
}
s__1267_1268: {
if (t_704) {
try {
t_705 = Core.cast(CodePoints.class, codePart__272);
} catch (RuntimeException ignored$28) {
break s__1267_1268;
}
this.pushCodePoints(t_705, true);
} else {
try {
Core.cast(CodeRange.class, codePart__272);
t_708 = true;
} catch (RuntimeException ignored$29) {
t_708 = false;
}
if (t_708) {
try {
t_709 = Core.cast(CodeRange.class, codePart__272);
} catch (RuntimeException ignored$30) {
break s__1267_1268;
}
this.pushCodeRangeUnwrapped(t_709);
} else {
try {
Core.cast(SpecialSet.class, codePart__272);
t_712 = true;
} catch (RuntimeException ignored$31) {
t_712 = false;
}
if (t_712) {
try {
t_713 = Core.cast(SpecialSet.class, codePart__272);
} catch (RuntimeException ignored$32) {
break s__1267_1268;
}
this.pushRegex(t_713);
} else {
/* null: Literal expression statement */
}
}
}
return;
}
throw Core.bubble();
}
void pushOr(Or or__275) {
int t_1087;
Regex t_696;
Regex t_701;
s__1269_1271: if (!or__275.getItems().isEmpty()) {
s__1270_1272: {
try {
Core.listAdd(this.out, "(?:");
t_696 = Core.listGet(or__275.getItems(), 0);
} catch (RuntimeException ignored$33) {
break s__1270_1272;
}
this.pushRegex(t_696);
int i__277 = 1;
while (true) {
t_1087 = or__275.getItems().size();
if (i__277 < t_1087) {
try {
Core.listAdd(this.out, "|");
t_701 = Core.listGet(or__275.getItems(), i__277);
} catch (RuntimeException ignored$34) {
break;
}
this.pushRegex(t_701);
i__277 = i__277 + 1;
} else {
try {
Core.listAdd(this.out, ")");
} catch (RuntimeException ignored$35) {
break s__1270_1272;
}
break s__1269_1271;
}
}
}
throw Core.bubble();
}
}
void pushRepeat(Repeat repeat__279) {
Regex t_1077;
boolean t_683;
boolean t_684;
boolean t_685;
int t_688;
List t_690;
s__1273_1274: {
int min__281;
@Nullable Integer max__282;
try {
Core.listAdd(this.out, "(?:");
t_1077 = repeat__279.getItem();
this.pushRegex(t_1077);
Core.listAdd(this.out, ")");
min__281 = repeat__279.getMin();
max__282 = repeat__279.getMax();
} catch (RuntimeException ignored$36) {
break s__1273_1274;
}
if (min__281 == 0) {
t_683 = Core.boxedEq(max__282, 1);
} else {
t_683 = false;
}
if (t_683) {
try {
Core.listAdd(this.out, "?");
} catch (RuntimeException ignored$37) {
break s__1273_1274;
}
} else {
if (min__281 == 0) {
t_684 = Objects.equals(max__282, null);
} else {
t_684 = false;
}
if (t_684) {
try {
Core.listAdd(this.out, "*");
} catch (RuntimeException ignored$38) {
break s__1273_1274;
}
} else {
if (min__281 == 1) {
t_685 = Objects.equals(max__282, null);
} else {
t_685 = false;
}
if (t_685) {
try {
Core.listAdd(this.out, "+");
} catch (RuntimeException ignored$39) {
break s__1273_1274;
}
} else {
try {
Core.listAdd(this.out, "{" + Integer.toString(min__281));
} catch (RuntimeException ignored$40) {
break s__1273_1274;
}
if (!Core.boxedEqRev(min__281, max__282)) {
try {
Core.listAdd(this.out, ",");
} catch (RuntimeException ignored$41) {
break s__1273_1274;
}
if (!Objects.equals(max__282, null)) {
t_690 = this.out;
try {
t_688 = Core.cast(Integer.class, max__282);
Core.listAdd(t_690, Integer.toString(t_688));
} catch (RuntimeException ignored$42) {
break s__1273_1274;
}
} else {
/* null: Literal expression statement */
}
} else {
/* null: Literal expression statement */
}
try {
Core.listAdd(this.out, "}");
} catch (RuntimeException ignored$43) {
break s__1273_1274;
}
}
}
}
if (repeat__279.isReluctant()) {
try {
Core.listAdd(this.out, "?");
} catch (RuntimeException ignored$44) {
break s__1273_1274;
}
} else {
/* null: Literal expression statement */
}
return;
}
throw Core.bubble();
}
void pushSequence(Sequence sequence__284) {
int t_1075;
Regex t_677;
int i__286 = 0;
s__1276_1277: {
while (true) {
t_1075 = sequence__284.getItems().size();
if (i__286 < t_1075) {
try {
t_677 = Core.listGet(sequence__284.getItems(), i__286);
} catch (RuntimeException ignored$45) {
break;
}
this.pushRegex(t_677);
i__286 = i__286 + 1;
} else {
break s__1276_1277;
}
}
throw Core.bubble();
}
}
public @Nullable Integer maxCode(CodePart codePart__288) {
@Nullable Integer return__116;
CodePointStringSlice t_1053;
CodePointStringSlice t_1055;
@Nullable Integer t_1060;
@Nullable Integer t_1063;
@Nullable Integer t_1066;
@Nullable Integer t_1069;
boolean t_650;
CodePoints t_651;
boolean t_663;
CodeRange t_664;
try {
Core.cast(CodePoints.class, codePart__288);
t_650 = true;
} catch (RuntimeException ignored$46) {
t_650 = false;
}
s__1278_1279: {
if (t_650) {
try {
t_651 = Core.cast(CodePoints.class, codePart__288);
} catch (RuntimeException ignored$47) {
break s__1278_1279;
}
String value__290 = t_651.getValue();
if (value__290.isEmpty()) {
return__116 = null;
} else {
int max__291 = 0;
t_1053 = Core.stringCodePoints(value__290);
CodePointStringSlice slice__292 = t_1053;
while (true) {
if (!slice__292.isEmpty()) {
int next__293 = slice__292.read();
if (next__293 > max__291) {
max__291 = next__293;
} else {
/* null: Literal expression statement */
}
t_1055 = slice__292.advance(1);
slice__292 = t_1055;
} else {
break;
}
}
return__116 = max__291;
}
} else {
try {
Core.cast(CodeRange.class, codePart__288);
t_663 = true;
} catch (RuntimeException ignored$48) {
t_663 = false;
}
if (t_663) {
try {
t_664 = Core.cast(CodeRange.class, codePart__288);
t_1060 = t_664.getMax();
return__116 = t_1060;
} catch (RuntimeException ignored$49) {
break s__1278_1279;
}
} else if (Objects.equals(codePart__288, Digit)) {
t_1063 = Core.stringCodePoints("9").read();
try {
return__116 = t_1063;
} catch (RuntimeException ignored$50) {
break s__1278_1279;
}
} else if (Objects.equals(codePart__288, Space)) {
t_1066 = Core.stringCodePoints(" ").read();
try {
return__116 = t_1066;
} catch (RuntimeException ignored$51) {
break s__1278_1279;
}
} else if (Objects.equals(codePart__288, Word)) {
t_1069 = Core.stringCodePoints("z").read();
try {
return__116 = t_1069;
} catch (RuntimeException ignored$52) {
break s__1278_1279;
}
} else {
return__116 = null;
}
}
return return__116;
}
throw Core.bubble();
}
public RegexFormatter(@Nullable List out__295) {
List t_1049;
if (out__295 == null) {
t_1049 = new ArrayList<>();
out__295 = t_1049;
}
this.out = out__295;
}
public RegexFormatter() {
this(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy