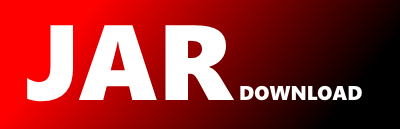
temper.std.temporal.TemporalGlobal.map Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of temper-std Show documentation
Show all versions of temper-std Show documentation
Optional support library provided with Temper
{ "version": 3, "file": "java/std/src/main/java/temper/std/temporal/TemporalGlobal.java", "sources": [ "std/temporal.temper.md" ], "sourcesContent": [ "# Temporal\n\nWe're creating an initial Date type to help with developing\nTemper's machinery to connect to existing Date types in\ntarget languages.\n\nSome facts about the Gregorian calendar.\n\n /** Indexed by the month number: 1 = January */\n let daysInMonth = [\n 0,\n /* January */ 31,\n /* February */ 28, // Special case leap days\n /* March */ 31,\n /* April */ 30,\n /* May */ 31,\n /* June */ 30,\n /* July */ 31,\n /* August */ 31,\n /* September */ 30,\n /* October */ 31,\n /* November */ 30,\n /* December */ 31,\n ];\n\n let isLeapYear(year: Int): Boolean {\n year % 4 == 0 \u0026\u0026 (year % 100 != 0 || year % 400 == 0)\n }\n\n /**\n * If the decimal representation of \\|num\\| is longer than [padding],\n * then that representation.\n * Otherwise any sign for [num] followed by the prefix of [padding]\n * that would bring the integer portion up to the length of [padding].\n *\n * ```temper\n * pad(\u00220000\u0022, 123) == \u00220123\u0022) \u0026\u0026\n * pad(\u0022000\u0022, 123) == \u0022123\u0022) \u0026\u0026\n * pad(\u002200\u0022, 123) == \u0022123\u0022) \u0026\u0026\n * pad(\u00220000\u0022, -123) == \u0022-0123\u0022) \u0026\u0026\n * pad(\u0022000\u0022, -123) == \u0022-123\u0022) \u0026\u0026\n * pad(\u002200\u0022, -123) == \u0022-123\u0022)\n * ```\n */\n let pad(padding: String, num: Int): String {\n let decimal = num.toString(10);\n var decimalCodePoints = decimal.codePoints;\n let sign: String;\n if (decimalCodePoints.read() == 45 /* - */) {\n sign = \u0022-\u0022;\n decimalCodePoints = decimalCodePoints.advance(1);\n } else {\n sign = \u0022\u0022;\n }\n let paddingCp = padding.codePoints;\n let nNeeded = paddingCp.length - decimalCodePoints.length;\n if (nNeeded \u003c= 0) {\n decimal\n } else {\n let pad = paddingCp.limit(nNeeded).toString();\n let decimalOnly = decimalCodePoints.toString();\n \u0022\u0024{sign}\u0024{pad}\u0024{decimalOnly}\u0022\n }\n }\n\nHere's just enough of a Date type to get us started.\n\n /**\n * A Date identifies a day in the proleptic Gregorian calendar.\n * It is unconnected to a time of day or a timezone.\n */\n @connected(\u0022Date\u0022)\n export class Date {\n /** The year. 1900 means 1900. */\n @connected(\u0022Date::getYear\u0022)\n public year: Int;\n /** The month of the year in [1, 12]. */\n @connected(\u0022Date::getMonth\u0022)\n public month: Int;\n /**\n * The day of the month in [1, 31]\n * additionally constrained by the length of [month].\n */\n @connected(\u0022Date::getDay\u0022)\n public day: Int;\n\n @connected(\u0022Date::constructor\u0022)\n public constructor(year: Int, month: Int, day: Int): Void | Bubble {\n if (1 \u003c= month \u0026\u0026 month \u003c= 12 \u0026\u0026\n 1 \u003c= day \u0026\u0026 (\n (month != 2 || day != 29)\n ? day \u003c= daysInMonth[month]\n : isLeapYear(year))) {\n this.year = year;\n this.month = month;\n this.day = day;\n } else {\n bubble();\n }\n }\n\n /** An ISO 8601 Date string with dashes like \u00222000-12-31\u0022. */\n @connected(\u0022Date::toString\u0022)\n public toString(): String {\n \u0022\u0024{pad(\u00220000\u0022, year)\n }-\u0024{pad(\u002200\u0022, month)\n }-\u0024{pad(\u002200\u0022, day)}\u0022\n }\n\n /**\n * The count of whole years between the two dates.\n *\n * Think of this as floor of the magnitude of a range:\n *\n * ⌊ [start, end] ⌋\n *\n * If you think of it as subtraction, you have to reverse\n * the order of arguments.\n *\n * ⌊ end - start ⌋, NOT ⌊ start - end ⌋\n *\n * \u0022Whole year\u0022 is based on month/day calculations, not\n * day-of-year. This means that there is one full year\n * between 2020-03-01 and 2021-03-01 even though, because\n * February of 2020 has 29 days, 2020-03-01 is the 61st\n * day of 2020 but 2021-03-01 is only the 60th day of\n * that year.\n */\n @connected(\u0022Date::yearsBetween\u0022)\n public static let yearsBetween(start: Date, end: Date): Int {\n let yearDelta = end.year - start.year;\n let monthDelta = end.month - start.month;\n yearDelta - (\n // If the end month/day is before the start's then we\n // don't have a full year.\n (monthDelta \u003c 0 || monthDelta == 0 \u0026\u0026 end.day \u003c start.day)\n ? 1 : 0)\n }\n\n /** Today's date in UTC */\n // TODO: take a zone\n @connected(\u0022Date::today\u0022)\n public static let today(): Date;\n };\n\nTODO: an auto-balancing Date builder.\nParse from ISO\nOther temporal values\nDay of week\n" ], "names": [ "daysInMonth", "isLeapYear", "year", "return", "t#132", "pad", "padding", "num", "t#185", "decimal", "t#181", "decimalCodePoints", "sign", "paddingCp", "nNeeded", "decimalOnly" ], "mappings": "AASI,cAsIE,CAAA,AAtIF,GAsIE,CAAA,AAtIF,QAsIE,CAAA;AAtIF,aAsIE,CAAA,AAtIF,IAsIE,CAAA,AAtIF,IAsIE,CAAA,AAtIF,oBAsIE,CAAA;AAtIF,aAsIE,CAAA,AAtIF,IAsIE,CAAA,AAtIF,IAsIE,CAAA;AAtIF,WAsIE,CAAA,AAtIF,IAsIE,CAAA,AAtIF,IAsIE,CAAA;AAtIF,YAsIE,MAAA,AAtIF,eAsIE,EAAA;AAtIF,WAsIE,AAtIF,eAsIE,EAAA,AAtIF;AAAA,KAsIE;AAtIF,gBAcC,AAdG,KAAW,AAAX,QAAW,CAAA,AAAf,CAAAA,eAcC;AAED,UAEC,AAF0B,QAAO,AAA9B,CAAAC,cAAU,CAAU,AAAH,GAAG,AAAT,CAAAC,QAAS,CAAW,EAAA;AAAR,eAAO,AAAP,CAAAC,UAAA,CACuB;AAAV,WAAU,AAAV,CAAAC,KAAU,CAAA;AAA/C,YAAAF,QAAI,AAAJ,EAAQ,AAAD,EAAC,AAAR,GAAa,AAAD,EAAC,CAAA,AAAb;AAAkB,gBAAAA,QAAI,AAAJ,EAAU,AAAH,IAAG,AAAV,GAAe,AAAD,EAAC;AAAA,gBAAAC,UAAA;AAAA,kBAAI;AAAA,gBAAAC,KAAA,EAAU,AAAV,CAAAF,QAAI,AAAJ,EAAU,AAAH,IAAG,CAAA;AAAV,gBAAAC,UAAA,EAAe,AAAf,CAAAC,KAAU,AAAV,GAAe,AAAD,EAAC;AAAA;AAAA,SAAC;AAAA,YAAAD,UAAA;AAAA;AACvD,cAAA,AAF0B,CAAAA,UAAA;AAE1B;AAiBD,UAmBC,AAnBmC,OAAM,AAAtC,CAAAE,OAAG,CAAgB,AAAN,MAAM,AAAf,CAAAC,WAAe,CAAU,AAAH,IAAG,AAAR,CAAAC,OAAQ,CAAU,EAAA;AAAP,cAAM,AAAN,CAAAJ,UAAA,CAMgB;AAA5B,4BAA4B,AAA5B,CAAAK,KAA4B,CAAA;AALlD,cAA8B,AAA1B,CAAAC,WAAO,EAAG,QAAgB,CAAA,AAAhB,QAAgB,CAAA,AAAhBF,OAAG,CAAU,GAAE,CAAC,CACY;AAAlB,4BAAkB,AAAlB,CAAAG,KAAkB,EAAA,AAAlB,KAAkB,CAAA,AAAlB,gBAAkB,CAAA,AAAlBD,WAAO,CAAW,CAAA;AAAvC,4BAAuC,AAAtC,CAAAE,qBAAiB,EAAG,CAAAD,KAAkB,CAC1B;AAAhB,cAAgB,AAAZ,CAAAE,QAAI;AACJ,YAAAD,qBAAiB,CAAA,AAAjB,IAAwB,EAAA,AAAxB,GAA8B,AAAF,GAAE,CAAU;AAC1C,YAAAC,QAAI,AAAJ,EAAU,AAAH,IAAG;AACU,YAAAJ,KAAA,EAA4B,AAA5B,CAAAG,qBAAiB,CAAC,OAAO,CAAC,CAAC,CAAC,CAAA;AAAhD,YAAAA,qBAAiB,AAAjB,EAAgD,AAA5B,CAAAH,KAA4B;AAAA,SACjD,KACC;AAAA,YAAAI,QAAI,AAAJ,EAAS,AAAF,GAAE;AAAA,SAEuB;AAAlC,4BAAkC,AAA9B,CAAAC,aAAS,EAAG,KAAkB,CAAA,AAAlB,gBAAkB,CAAA,AAAlBP,WAAO,CAAW,CACuB;AAAzD,WAAyD,AAArD,CAAAQ,WAAO,EAAG,CAAAD,aAAS,CAAA,AAAT,MAAgB,EAAA,AAAhB,EAA2C,AAAxB,CAAAF,qBAAiB,CAAA,AAAjB,MAAwB;AAAA,YACrDG,WAAO,AAAP,GAAY,AAAD,EAAC,CACd;AAAA,YAAAX,UAAA,EAAO,AAAP,CAAAM,WAAO;AAAA,cACF,EACwC;AAA7C,kBAA6C,AAAzC,CAAAJ,OAAG,EAAG,CAAAQ,aAAS,CAAC,KAAK,CAACC,WAAO,CAAC,CAAA,AAAxB,QAAmC,GACC;AAA9C,kBAA8C,AAA1C,CAAAC,eAAW,EAAG,CAAAJ,qBAAiB,CAAA,AAAjB,QAA4B;AAC9C,YAAAR,UAAA,EAA6B,AAA1B,CAAAS,QAAO,EAAG,AAAH,CAAAP,OAAM,EAAW,AAAX,CAAAU,eAAa;AAAA;AAEhC,cAAA,AAnBmC,CAAAZ,UAAA;AAmBnC,KAAA;AAtDD;AAAA,QAAAH,eAcC,AAdD,EAcC,AAdiB,KAcjB,CAAA,AAdiB,EAcjB,CAAA,AAbC,CAAC,CACe,GAAE,CACF,GAAE,CACF,GAAE,CACF,GAAE,CACF,GAAE,CACF,GAAE,CACF,GAAE,CACF,GAAE,CACF,GAAE,CACF,GAAE,CACF,GAAE,CACF,GAAE,CACnB;AAAA;AAwHC" }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy