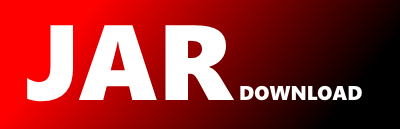
dev.the_fireplace.grandeconomy.command.commands.WalletCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of GrandEconomy Show documentation
Show all versions of GrandEconomy Show documentation
A server-side economy mod/api for Minecraft.
package dev.the_fireplace.grandeconomy.command.commands;
import com.mojang.brigadier.Command;
import com.mojang.brigadier.CommandDispatcher;
import com.mojang.brigadier.arguments.DoubleArgumentType;
import com.mojang.brigadier.builder.ArgumentBuilder;
import com.mojang.brigadier.builder.LiteralArgumentBuilder;
import com.mojang.brigadier.context.CommandContext;
import com.mojang.brigadier.exceptions.CommandSyntaxException;
import com.mojang.brigadier.tree.CommandNode;
import dev.the_fireplace.grandeconomy.GrandEconomy;
import dev.the_fireplace.grandeconomy.command.GeCommand;
import net.minecraft.class_1657;
import net.minecraft.class_2168;
import net.minecraft.class_2170;
import net.minecraft.class_2186;
import net.minecraft.class_3222;
public final class WalletCommand extends GeCommand {
@Override
public CommandNode register(CommandDispatcher commandDispatcher) {
LiteralArgumentBuilder walletCommand = class_2170.method_9247("wallet")
.requires(requirements::manageGameSettings);
walletCommand.then(class_2170.method_9247("balance")
.then(class_2170.method_9244("player", class_2186.method_9305())
.executes((command) -> {
class_3222 targetPlayer = class_2186.method_9315(command, "player");
return runBalanceCommand(command, targetPlayer);
})
)
);
walletCommand.then(class_2170.method_9247("set")
.then(class_2170.method_9244("player", class_2186.method_9305())
.then(class_2170.method_9244("amount", DoubleArgumentType.doubleArg(0))
.executes(this::runSetCommand)
)
)
);
addAliased(walletCommand, new String[]{"give", "add"},
class_2170.method_9244("player", class_2186.method_9305())
.then(class_2170.method_9244("amount", DoubleArgumentType.doubleArg(0))
.executes(this::runGiveCommand)
)
);
walletCommand.then(class_2170.method_9247("take")
.then(class_2170.method_9244("target", class_2186.method_9305())
.then(class_2170.method_9244("amount", DoubleArgumentType.doubleArg(0))
.executes(this::runTakeCommand)
)
)
);
return commandDispatcher.register(walletCommand);
}
private void addAliased(ArgumentBuilder baseCommand, String[] aliases, ArgumentBuilder remainingArgs) {
for (String alias: aliases) {
baseCommand.then(class_2170.method_9247(alias)
.then(remainingArgs)
);
}
}
private int runSetCommand(CommandContext command) throws CommandSyntaxException {
class_1657 targetPlayer = class_2186.method_9315(command, "player");
double amount = command.getArgument("amount", Double.class);
if (amount < 0) {
return feedbackSender.throwFailure(command, "commands.grandeconomy.wallet.negative", targetPlayer.method_5476());
}
currencyAPI.setBalance(targetPlayer.method_5667(), amount, true);
command.getSource().method_9226(GrandEconomy.getTranslator().getTextForTarget(command.getSource(), "commands.grandeconomy.wallet.set", targetPlayer.method_5476(), currencyAPI.formatCurrency(amount)), false);
return Command.SINGLE_SUCCESS;
}
private int runBalanceCommand(CommandContext command, class_3222 targetPlayer) {
feedbackSender.basic(command, "commands.grandeconomy.wallet.balance", targetPlayer.method_5476(), currencyAPI.getFormattedBalance(targetPlayer.method_5667(), true));
return Command.SINGLE_SUCCESS;
}
private int runGiveCommand(CommandContext command) throws CommandSyntaxException {
class_1657 targetPlayer = class_2186.method_9315(command, "player");
double amount = command.getArgument("amount", Double.class);
if (currencyAPI.getBalance(targetPlayer.method_5667(), true) + amount < 0) {
return feedbackSender.throwFailure(command, "commands.grandeconomy.wallet.negative", targetPlayer.method_5476());
}
currencyAPI.addToBalance(targetPlayer.method_5667(), amount, true);
feedbackSender.basic(command, "commands.grandeconomy.wallet.given", currencyAPI.formatCurrency(amount), targetPlayer.method_5476());
return Command.SINGLE_SUCCESS;
}
private int runTakeCommand(CommandContext command) throws CommandSyntaxException {
class_1657 targetPlayer = class_2186.method_9315(command, "target");
double amount = command.getArgument("amount", Double.class);
if (currencyAPI.getBalance(targetPlayer.method_5667(), true) - amount < 0) {
return feedbackSender.throwFailure(command, "commands.grandeconomy.wallet.negative", targetPlayer.method_5476());
}
currencyAPI.takeFromBalance(targetPlayer.method_5667(), amount, true);
feedbackSender.basic(command, "commands.grandeconomy.wallet.taken", currencyAPI.formatCurrency(amount), targetPlayer.method_5476());
return Command.SINGLE_SUCCESS;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy