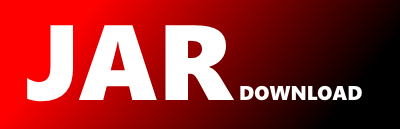
dev.vality.damsel.analytics.AnalyticsServiceSrv Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analytics-proto Show documentation
Show all versions of analytics-proto Show documentation
Generates jar artifact containing compiled thrift classes based on generated thrift IDL files
/**
* Autogenerated by Thrift Compiler (0.14.2)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package dev.vality.damsel.analytics;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@javax.annotation.Generated(value = "Autogenerated by Thrift Compiler (0.14.2)", date = "2022-11-14")
public class AnalyticsServiceSrv {
/**
* Сервис для работы с аналитикой
*
*/
public interface Iface {
/**
* Получение распределения использования платежных инструментов для ЛК.
*
*
* @param request
*/
public PaymentToolDistributionResponse getPaymentsToolDistribution(FilterRequest request) throws org.apache.thrift.TException;
/**
* Получение списка оборотов с группировкой по валютам для ЛК.
*
*
* @param request
*/
public AmountResponse getPaymentsAmount(FilterRequest request) throws org.apache.thrift.TException;
/**
* Получение списка зачислений с группировкой по валютам для ЛК.
*
*
* @param request
*/
public AmountResponse getCreditingsAmount(FilterRequest request) throws org.apache.thrift.TException;
/**
* Получение среднего размера платежа с группировкой по валютам для ЛК.
*
*
* @param request
*/
public AmountResponse getAveragePayment(FilterRequest request) throws org.apache.thrift.TException;
/**
* Получение колличества платежей с группировкой по валютам для ЛК.
*
*
* @param request
*/
public CountResponse getPaymentsCount(FilterRequest request) throws org.apache.thrift.TException;
/**
* Получение распределения ошибок по причине ошибки для ЛК.
*
*
* @param request
*/
public ErrorDistributionsResponse getPaymentsErrorDistribution(FilterRequest request) throws org.apache.thrift.TException;
/**
* Получение распределения ошибок c подошибками для ЛК.
*
*
* @param request
*/
public SubErrorDistributionsResponse getPaymentsSubErrorDistribution(FilterRequest request) throws org.apache.thrift.TException;
/**
* Получение списка оборотов с группировкой по валютам и разделенные по временным интервалам для ЛК.
*
*
* @param request
*/
public SplitAmountResponse getPaymentsSplitAmount(SplitFilterRequest request) throws org.apache.thrift.TException;
/**
* Получение колличества платежей с группировкой по валютам и статусам, разделенные по временным интервалам для ЛК.
*
*
* @param request
*/
public SplitCountResponse getPaymentsSplitCount(SplitFilterRequest request) throws org.apache.thrift.TException;
/**
* Получение списка возвратов с группировкой по валютам для ЛК.
*
*
* @param request
*/
public AmountResponse getRefundsAmount(FilterRequest request) throws org.apache.thrift.TException;
/**
* Получение балансов с группировкой по валютам для ЛК.
*
*
* @param merchant_filter
*/
public AmountResponse getCurrentBalances(MerchantFilter merchant_filter) throws org.apache.thrift.TException;
/**
* Получение текущего баланса с группировкой по магазинам
*
*
* @param merchant_filter
*/
public ShopAmountResponse getCurrentShopBalances(MerchantFilter merchant_filter) throws org.apache.thrift.TException;
}
public interface AsyncIface {
public void getPaymentsToolDistribution(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getPaymentsAmount(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getCreditingsAmount(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getAveragePayment(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getPaymentsCount(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getPaymentsErrorDistribution(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getPaymentsSubErrorDistribution(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getPaymentsSplitAmount(SplitFilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getPaymentsSplitCount(SplitFilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getRefundsAmount(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getCurrentBalances(MerchantFilter merchant_filter, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
public void getCurrentShopBalances(MerchantFilter merchant_filter, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException;
}
public static class Client extends org.apache.thrift.TServiceClient implements Iface {
public static class Factory implements org.apache.thrift.TServiceClientFactory {
public Factory() {}
public Client getClient(org.apache.thrift.protocol.TProtocol prot) {
return new Client(prot);
}
public Client getClient(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
return new Client(iprot, oprot);
}
}
public Client(org.apache.thrift.protocol.TProtocol prot)
{
super(prot, prot);
}
public Client(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TProtocol oprot) {
super(iprot, oprot);
}
public PaymentToolDistributionResponse getPaymentsToolDistribution(FilterRequest request) throws org.apache.thrift.TException
{
sendGetPaymentsToolDistribution(request);
return recvGetPaymentsToolDistribution();
}
public void sendGetPaymentsToolDistribution(FilterRequest request) throws org.apache.thrift.TException
{
GetPaymentsToolDistribution_args args = new GetPaymentsToolDistribution_args();
args.setRequest(request);
sendBase("GetPaymentsToolDistribution", args);
}
public PaymentToolDistributionResponse recvGetPaymentsToolDistribution() throws org.apache.thrift.TException
{
GetPaymentsToolDistribution_result result = new GetPaymentsToolDistribution_result();
receiveBase(result, "GetPaymentsToolDistribution");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetPaymentsToolDistribution failed: unknown result");
}
public AmountResponse getPaymentsAmount(FilterRequest request) throws org.apache.thrift.TException
{
sendGetPaymentsAmount(request);
return recvGetPaymentsAmount();
}
public void sendGetPaymentsAmount(FilterRequest request) throws org.apache.thrift.TException
{
GetPaymentsAmount_args args = new GetPaymentsAmount_args();
args.setRequest(request);
sendBase("GetPaymentsAmount", args);
}
public AmountResponse recvGetPaymentsAmount() throws org.apache.thrift.TException
{
GetPaymentsAmount_result result = new GetPaymentsAmount_result();
receiveBase(result, "GetPaymentsAmount");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetPaymentsAmount failed: unknown result");
}
public AmountResponse getCreditingsAmount(FilterRequest request) throws org.apache.thrift.TException
{
sendGetCreditingsAmount(request);
return recvGetCreditingsAmount();
}
public void sendGetCreditingsAmount(FilterRequest request) throws org.apache.thrift.TException
{
GetCreditingsAmount_args args = new GetCreditingsAmount_args();
args.setRequest(request);
sendBase("GetCreditingsAmount", args);
}
public AmountResponse recvGetCreditingsAmount() throws org.apache.thrift.TException
{
GetCreditingsAmount_result result = new GetCreditingsAmount_result();
receiveBase(result, "GetCreditingsAmount");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetCreditingsAmount failed: unknown result");
}
public AmountResponse getAveragePayment(FilterRequest request) throws org.apache.thrift.TException
{
sendGetAveragePayment(request);
return recvGetAveragePayment();
}
public void sendGetAveragePayment(FilterRequest request) throws org.apache.thrift.TException
{
GetAveragePayment_args args = new GetAveragePayment_args();
args.setRequest(request);
sendBase("GetAveragePayment", args);
}
public AmountResponse recvGetAveragePayment() throws org.apache.thrift.TException
{
GetAveragePayment_result result = new GetAveragePayment_result();
receiveBase(result, "GetAveragePayment");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetAveragePayment failed: unknown result");
}
public CountResponse getPaymentsCount(FilterRequest request) throws org.apache.thrift.TException
{
sendGetPaymentsCount(request);
return recvGetPaymentsCount();
}
public void sendGetPaymentsCount(FilterRequest request) throws org.apache.thrift.TException
{
GetPaymentsCount_args args = new GetPaymentsCount_args();
args.setRequest(request);
sendBase("GetPaymentsCount", args);
}
public CountResponse recvGetPaymentsCount() throws org.apache.thrift.TException
{
GetPaymentsCount_result result = new GetPaymentsCount_result();
receiveBase(result, "GetPaymentsCount");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetPaymentsCount failed: unknown result");
}
public ErrorDistributionsResponse getPaymentsErrorDistribution(FilterRequest request) throws org.apache.thrift.TException
{
sendGetPaymentsErrorDistribution(request);
return recvGetPaymentsErrorDistribution();
}
public void sendGetPaymentsErrorDistribution(FilterRequest request) throws org.apache.thrift.TException
{
GetPaymentsErrorDistribution_args args = new GetPaymentsErrorDistribution_args();
args.setRequest(request);
sendBase("GetPaymentsErrorDistribution", args);
}
public ErrorDistributionsResponse recvGetPaymentsErrorDistribution() throws org.apache.thrift.TException
{
GetPaymentsErrorDistribution_result result = new GetPaymentsErrorDistribution_result();
receiveBase(result, "GetPaymentsErrorDistribution");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetPaymentsErrorDistribution failed: unknown result");
}
public SubErrorDistributionsResponse getPaymentsSubErrorDistribution(FilterRequest request) throws org.apache.thrift.TException
{
sendGetPaymentsSubErrorDistribution(request);
return recvGetPaymentsSubErrorDistribution();
}
public void sendGetPaymentsSubErrorDistribution(FilterRequest request) throws org.apache.thrift.TException
{
GetPaymentsSubErrorDistribution_args args = new GetPaymentsSubErrorDistribution_args();
args.setRequest(request);
sendBase("GetPaymentsSubErrorDistribution", args);
}
public SubErrorDistributionsResponse recvGetPaymentsSubErrorDistribution() throws org.apache.thrift.TException
{
GetPaymentsSubErrorDistribution_result result = new GetPaymentsSubErrorDistribution_result();
receiveBase(result, "GetPaymentsSubErrorDistribution");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetPaymentsSubErrorDistribution failed: unknown result");
}
public SplitAmountResponse getPaymentsSplitAmount(SplitFilterRequest request) throws org.apache.thrift.TException
{
sendGetPaymentsSplitAmount(request);
return recvGetPaymentsSplitAmount();
}
public void sendGetPaymentsSplitAmount(SplitFilterRequest request) throws org.apache.thrift.TException
{
GetPaymentsSplitAmount_args args = new GetPaymentsSplitAmount_args();
args.setRequest(request);
sendBase("GetPaymentsSplitAmount", args);
}
public SplitAmountResponse recvGetPaymentsSplitAmount() throws org.apache.thrift.TException
{
GetPaymentsSplitAmount_result result = new GetPaymentsSplitAmount_result();
receiveBase(result, "GetPaymentsSplitAmount");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetPaymentsSplitAmount failed: unknown result");
}
public SplitCountResponse getPaymentsSplitCount(SplitFilterRequest request) throws org.apache.thrift.TException
{
sendGetPaymentsSplitCount(request);
return recvGetPaymentsSplitCount();
}
public void sendGetPaymentsSplitCount(SplitFilterRequest request) throws org.apache.thrift.TException
{
GetPaymentsSplitCount_args args = new GetPaymentsSplitCount_args();
args.setRequest(request);
sendBase("GetPaymentsSplitCount", args);
}
public SplitCountResponse recvGetPaymentsSplitCount() throws org.apache.thrift.TException
{
GetPaymentsSplitCount_result result = new GetPaymentsSplitCount_result();
receiveBase(result, "GetPaymentsSplitCount");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetPaymentsSplitCount failed: unknown result");
}
public AmountResponse getRefundsAmount(FilterRequest request) throws org.apache.thrift.TException
{
sendGetRefundsAmount(request);
return recvGetRefundsAmount();
}
public void sendGetRefundsAmount(FilterRequest request) throws org.apache.thrift.TException
{
GetRefundsAmount_args args = new GetRefundsAmount_args();
args.setRequest(request);
sendBase("GetRefundsAmount", args);
}
public AmountResponse recvGetRefundsAmount() throws org.apache.thrift.TException
{
GetRefundsAmount_result result = new GetRefundsAmount_result();
receiveBase(result, "GetRefundsAmount");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetRefundsAmount failed: unknown result");
}
public AmountResponse getCurrentBalances(MerchantFilter merchant_filter) throws org.apache.thrift.TException
{
sendGetCurrentBalances(merchant_filter);
return recvGetCurrentBalances();
}
public void sendGetCurrentBalances(MerchantFilter merchant_filter) throws org.apache.thrift.TException
{
GetCurrentBalances_args args = new GetCurrentBalances_args();
args.setMerchantFilter(merchant_filter);
sendBase("GetCurrentBalances", args);
}
public AmountResponse recvGetCurrentBalances() throws org.apache.thrift.TException
{
GetCurrentBalances_result result = new GetCurrentBalances_result();
receiveBase(result, "GetCurrentBalances");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetCurrentBalances failed: unknown result");
}
public ShopAmountResponse getCurrentShopBalances(MerchantFilter merchant_filter) throws org.apache.thrift.TException
{
sendGetCurrentShopBalances(merchant_filter);
return recvGetCurrentShopBalances();
}
public void sendGetCurrentShopBalances(MerchantFilter merchant_filter) throws org.apache.thrift.TException
{
GetCurrentShopBalances_args args = new GetCurrentShopBalances_args();
args.setMerchantFilter(merchant_filter);
sendBase("GetCurrentShopBalances", args);
}
public ShopAmountResponse recvGetCurrentShopBalances() throws org.apache.thrift.TException
{
GetCurrentShopBalances_result result = new GetCurrentShopBalances_result();
receiveBase(result, "GetCurrentShopBalances");
if (result.isSetSuccess()) {
return result.success;
}
throw new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.MISSING_RESULT, "GetCurrentShopBalances failed: unknown result");
}
}
public static class AsyncClient extends org.apache.thrift.async.TAsyncClient implements AsyncIface {
public static class Factory implements org.apache.thrift.async.TAsyncClientFactory {
private org.apache.thrift.async.TAsyncClientManager clientManager;
private org.apache.thrift.protocol.TProtocolFactory protocolFactory;
public Factory(org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.protocol.TProtocolFactory protocolFactory) {
this.clientManager = clientManager;
this.protocolFactory = protocolFactory;
}
public AsyncClient getAsyncClient(org.apache.thrift.transport.TNonblockingTransport transport) {
return new AsyncClient(protocolFactory, clientManager, transport);
}
}
public AsyncClient(org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.async.TAsyncClientManager clientManager, org.apache.thrift.transport.TNonblockingTransport transport) {
super(protocolFactory, clientManager, transport);
}
public void getPaymentsToolDistribution(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetPaymentsToolDistribution_call method_call = new GetPaymentsToolDistribution_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetPaymentsToolDistribution_call extends org.apache.thrift.async.TAsyncMethodCall {
private FilterRequest request;
public GetPaymentsToolDistribution_call(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetPaymentsToolDistribution", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetPaymentsToolDistribution_args args = new GetPaymentsToolDistribution_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public PaymentToolDistributionResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetPaymentsToolDistribution();
}
}
public void getPaymentsAmount(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetPaymentsAmount_call method_call = new GetPaymentsAmount_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetPaymentsAmount_call extends org.apache.thrift.async.TAsyncMethodCall {
private FilterRequest request;
public GetPaymentsAmount_call(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetPaymentsAmount", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetPaymentsAmount_args args = new GetPaymentsAmount_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public AmountResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetPaymentsAmount();
}
}
public void getCreditingsAmount(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetCreditingsAmount_call method_call = new GetCreditingsAmount_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetCreditingsAmount_call extends org.apache.thrift.async.TAsyncMethodCall {
private FilterRequest request;
public GetCreditingsAmount_call(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetCreditingsAmount", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetCreditingsAmount_args args = new GetCreditingsAmount_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public AmountResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetCreditingsAmount();
}
}
public void getAveragePayment(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetAveragePayment_call method_call = new GetAveragePayment_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetAveragePayment_call extends org.apache.thrift.async.TAsyncMethodCall {
private FilterRequest request;
public GetAveragePayment_call(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetAveragePayment", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetAveragePayment_args args = new GetAveragePayment_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public AmountResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetAveragePayment();
}
}
public void getPaymentsCount(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetPaymentsCount_call method_call = new GetPaymentsCount_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetPaymentsCount_call extends org.apache.thrift.async.TAsyncMethodCall {
private FilterRequest request;
public GetPaymentsCount_call(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetPaymentsCount", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetPaymentsCount_args args = new GetPaymentsCount_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public CountResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetPaymentsCount();
}
}
public void getPaymentsErrorDistribution(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetPaymentsErrorDistribution_call method_call = new GetPaymentsErrorDistribution_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetPaymentsErrorDistribution_call extends org.apache.thrift.async.TAsyncMethodCall {
private FilterRequest request;
public GetPaymentsErrorDistribution_call(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetPaymentsErrorDistribution", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetPaymentsErrorDistribution_args args = new GetPaymentsErrorDistribution_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public ErrorDistributionsResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetPaymentsErrorDistribution();
}
}
public void getPaymentsSubErrorDistribution(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetPaymentsSubErrorDistribution_call method_call = new GetPaymentsSubErrorDistribution_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetPaymentsSubErrorDistribution_call extends org.apache.thrift.async.TAsyncMethodCall {
private FilterRequest request;
public GetPaymentsSubErrorDistribution_call(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetPaymentsSubErrorDistribution", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetPaymentsSubErrorDistribution_args args = new GetPaymentsSubErrorDistribution_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public SubErrorDistributionsResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetPaymentsSubErrorDistribution();
}
}
public void getPaymentsSplitAmount(SplitFilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetPaymentsSplitAmount_call method_call = new GetPaymentsSplitAmount_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetPaymentsSplitAmount_call extends org.apache.thrift.async.TAsyncMethodCall {
private SplitFilterRequest request;
public GetPaymentsSplitAmount_call(SplitFilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetPaymentsSplitAmount", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetPaymentsSplitAmount_args args = new GetPaymentsSplitAmount_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public SplitAmountResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetPaymentsSplitAmount();
}
}
public void getPaymentsSplitCount(SplitFilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetPaymentsSplitCount_call method_call = new GetPaymentsSplitCount_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetPaymentsSplitCount_call extends org.apache.thrift.async.TAsyncMethodCall {
private SplitFilterRequest request;
public GetPaymentsSplitCount_call(SplitFilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetPaymentsSplitCount", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetPaymentsSplitCount_args args = new GetPaymentsSplitCount_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public SplitCountResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetPaymentsSplitCount();
}
}
public void getRefundsAmount(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetRefundsAmount_call method_call = new GetRefundsAmount_call(request, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetRefundsAmount_call extends org.apache.thrift.async.TAsyncMethodCall {
private FilterRequest request;
public GetRefundsAmount_call(FilterRequest request, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.request = request;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetRefundsAmount", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetRefundsAmount_args args = new GetRefundsAmount_args();
args.setRequest(request);
args.write(prot);
prot.writeMessageEnd();
}
public AmountResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetRefundsAmount();
}
}
public void getCurrentBalances(MerchantFilter merchant_filter, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetCurrentBalances_call method_call = new GetCurrentBalances_call(merchant_filter, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetCurrentBalances_call extends org.apache.thrift.async.TAsyncMethodCall {
private MerchantFilter merchant_filter;
public GetCurrentBalances_call(MerchantFilter merchant_filter, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.merchant_filter = merchant_filter;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetCurrentBalances", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetCurrentBalances_args args = new GetCurrentBalances_args();
args.setMerchantFilter(merchant_filter);
args.write(prot);
prot.writeMessageEnd();
}
public AmountResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetCurrentBalances();
}
}
public void getCurrentShopBalances(MerchantFilter merchant_filter, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
checkReady();
GetCurrentShopBalances_call method_call = new GetCurrentShopBalances_call(merchant_filter, resultHandler, this, ___protocolFactory, ___transport);
this.___currentMethod = method_call;
___manager.call(method_call);
}
public static class GetCurrentShopBalances_call extends org.apache.thrift.async.TAsyncMethodCall {
private MerchantFilter merchant_filter;
public GetCurrentShopBalances_call(MerchantFilter merchant_filter, org.apache.thrift.async.AsyncMethodCallback resultHandler, org.apache.thrift.async.TAsyncClient client, org.apache.thrift.protocol.TProtocolFactory protocolFactory, org.apache.thrift.transport.TNonblockingTransport transport) throws org.apache.thrift.TException {
super(client, protocolFactory, transport, resultHandler, false);
this.merchant_filter = merchant_filter;
}
public void write_args(org.apache.thrift.protocol.TProtocol prot) throws org.apache.thrift.TException {
prot.writeMessageBegin(new org.apache.thrift.protocol.TMessage("GetCurrentShopBalances", org.apache.thrift.protocol.TMessageType.CALL, 0));
GetCurrentShopBalances_args args = new GetCurrentShopBalances_args();
args.setMerchantFilter(merchant_filter);
args.write(prot);
prot.writeMessageEnd();
}
public ShopAmountResponse getResult() throws org.apache.thrift.TException {
if (getState() != org.apache.thrift.async.TAsyncMethodCall.State.RESPONSE_READ) {
throw new java.lang.IllegalStateException("Method call not finished!");
}
org.apache.thrift.transport.TMemoryInputTransport memoryTransport = new org.apache.thrift.transport.TMemoryInputTransport(getFrameBuffer().array());
org.apache.thrift.protocol.TProtocol prot = client.getProtocolFactory().getProtocol(memoryTransport);
return (new Client(prot)).recvGetCurrentShopBalances();
}
}
}
public static class Processor extends org.apache.thrift.TBaseProcessor implements org.apache.thrift.TProcessor {
private static final org.slf4j.Logger _LOGGER = org.slf4j.LoggerFactory.getLogger(Processor.class.getName());
public Processor(I iface) {
super(iface, getProcessMap(new java.util.HashMap>()));
}
protected Processor(I iface, java.util.Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static java.util.Map> getProcessMap(java.util.Map> processMap) {
processMap.put("GetPaymentsToolDistribution", new GetPaymentsToolDistribution());
processMap.put("GetPaymentsAmount", new GetPaymentsAmount());
processMap.put("GetCreditingsAmount", new GetCreditingsAmount());
processMap.put("GetAveragePayment", new GetAveragePayment());
processMap.put("GetPaymentsCount", new GetPaymentsCount());
processMap.put("GetPaymentsErrorDistribution", new GetPaymentsErrorDistribution());
processMap.put("GetPaymentsSubErrorDistribution", new GetPaymentsSubErrorDistribution());
processMap.put("GetPaymentsSplitAmount", new GetPaymentsSplitAmount());
processMap.put("GetPaymentsSplitCount", new GetPaymentsSplitCount());
processMap.put("GetRefundsAmount", new GetRefundsAmount());
processMap.put("GetCurrentBalances", new GetCurrentBalances());
processMap.put("GetCurrentShopBalances", new GetCurrentShopBalances());
return processMap;
}
public static class GetPaymentsToolDistribution extends org.apache.thrift.ProcessFunction {
public GetPaymentsToolDistribution() {
super("GetPaymentsToolDistribution");
}
public GetPaymentsToolDistribution_args getEmptyArgsInstance() {
return new GetPaymentsToolDistribution_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetPaymentsToolDistribution_result getResult(I iface, GetPaymentsToolDistribution_args args) throws org.apache.thrift.TException {
GetPaymentsToolDistribution_result result = new GetPaymentsToolDistribution_result();
result.success = iface.getPaymentsToolDistribution(args.request);
return result;
}
}
public static class GetPaymentsAmount extends org.apache.thrift.ProcessFunction {
public GetPaymentsAmount() {
super("GetPaymentsAmount");
}
public GetPaymentsAmount_args getEmptyArgsInstance() {
return new GetPaymentsAmount_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetPaymentsAmount_result getResult(I iface, GetPaymentsAmount_args args) throws org.apache.thrift.TException {
GetPaymentsAmount_result result = new GetPaymentsAmount_result();
result.success = iface.getPaymentsAmount(args.request);
return result;
}
}
public static class GetCreditingsAmount extends org.apache.thrift.ProcessFunction {
public GetCreditingsAmount() {
super("GetCreditingsAmount");
}
public GetCreditingsAmount_args getEmptyArgsInstance() {
return new GetCreditingsAmount_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetCreditingsAmount_result getResult(I iface, GetCreditingsAmount_args args) throws org.apache.thrift.TException {
GetCreditingsAmount_result result = new GetCreditingsAmount_result();
result.success = iface.getCreditingsAmount(args.request);
return result;
}
}
public static class GetAveragePayment extends org.apache.thrift.ProcessFunction {
public GetAveragePayment() {
super("GetAveragePayment");
}
public GetAveragePayment_args getEmptyArgsInstance() {
return new GetAveragePayment_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetAveragePayment_result getResult(I iface, GetAveragePayment_args args) throws org.apache.thrift.TException {
GetAveragePayment_result result = new GetAveragePayment_result();
result.success = iface.getAveragePayment(args.request);
return result;
}
}
public static class GetPaymentsCount extends org.apache.thrift.ProcessFunction {
public GetPaymentsCount() {
super("GetPaymentsCount");
}
public GetPaymentsCount_args getEmptyArgsInstance() {
return new GetPaymentsCount_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetPaymentsCount_result getResult(I iface, GetPaymentsCount_args args) throws org.apache.thrift.TException {
GetPaymentsCount_result result = new GetPaymentsCount_result();
result.success = iface.getPaymentsCount(args.request);
return result;
}
}
public static class GetPaymentsErrorDistribution extends org.apache.thrift.ProcessFunction {
public GetPaymentsErrorDistribution() {
super("GetPaymentsErrorDistribution");
}
public GetPaymentsErrorDistribution_args getEmptyArgsInstance() {
return new GetPaymentsErrorDistribution_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetPaymentsErrorDistribution_result getResult(I iface, GetPaymentsErrorDistribution_args args) throws org.apache.thrift.TException {
GetPaymentsErrorDistribution_result result = new GetPaymentsErrorDistribution_result();
result.success = iface.getPaymentsErrorDistribution(args.request);
return result;
}
}
public static class GetPaymentsSubErrorDistribution extends org.apache.thrift.ProcessFunction {
public GetPaymentsSubErrorDistribution() {
super("GetPaymentsSubErrorDistribution");
}
public GetPaymentsSubErrorDistribution_args getEmptyArgsInstance() {
return new GetPaymentsSubErrorDistribution_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetPaymentsSubErrorDistribution_result getResult(I iface, GetPaymentsSubErrorDistribution_args args) throws org.apache.thrift.TException {
GetPaymentsSubErrorDistribution_result result = new GetPaymentsSubErrorDistribution_result();
result.success = iface.getPaymentsSubErrorDistribution(args.request);
return result;
}
}
public static class GetPaymentsSplitAmount extends org.apache.thrift.ProcessFunction {
public GetPaymentsSplitAmount() {
super("GetPaymentsSplitAmount");
}
public GetPaymentsSplitAmount_args getEmptyArgsInstance() {
return new GetPaymentsSplitAmount_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetPaymentsSplitAmount_result getResult(I iface, GetPaymentsSplitAmount_args args) throws org.apache.thrift.TException {
GetPaymentsSplitAmount_result result = new GetPaymentsSplitAmount_result();
result.success = iface.getPaymentsSplitAmount(args.request);
return result;
}
}
public static class GetPaymentsSplitCount extends org.apache.thrift.ProcessFunction {
public GetPaymentsSplitCount() {
super("GetPaymentsSplitCount");
}
public GetPaymentsSplitCount_args getEmptyArgsInstance() {
return new GetPaymentsSplitCount_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetPaymentsSplitCount_result getResult(I iface, GetPaymentsSplitCount_args args) throws org.apache.thrift.TException {
GetPaymentsSplitCount_result result = new GetPaymentsSplitCount_result();
result.success = iface.getPaymentsSplitCount(args.request);
return result;
}
}
public static class GetRefundsAmount extends org.apache.thrift.ProcessFunction {
public GetRefundsAmount() {
super("GetRefundsAmount");
}
public GetRefundsAmount_args getEmptyArgsInstance() {
return new GetRefundsAmount_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetRefundsAmount_result getResult(I iface, GetRefundsAmount_args args) throws org.apache.thrift.TException {
GetRefundsAmount_result result = new GetRefundsAmount_result();
result.success = iface.getRefundsAmount(args.request);
return result;
}
}
public static class GetCurrentBalances extends org.apache.thrift.ProcessFunction {
public GetCurrentBalances() {
super("GetCurrentBalances");
}
public GetCurrentBalances_args getEmptyArgsInstance() {
return new GetCurrentBalances_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetCurrentBalances_result getResult(I iface, GetCurrentBalances_args args) throws org.apache.thrift.TException {
GetCurrentBalances_result result = new GetCurrentBalances_result();
result.success = iface.getCurrentBalances(args.merchant_filter);
return result;
}
}
public static class GetCurrentShopBalances extends org.apache.thrift.ProcessFunction {
public GetCurrentShopBalances() {
super("GetCurrentShopBalances");
}
public GetCurrentShopBalances_args getEmptyArgsInstance() {
return new GetCurrentShopBalances_args();
}
protected boolean isOneway() {
return false;
}
@Override
protected boolean rethrowUnhandledExceptions() {
return false;
}
public GetCurrentShopBalances_result getResult(I iface, GetCurrentShopBalances_args args) throws org.apache.thrift.TException {
GetCurrentShopBalances_result result = new GetCurrentShopBalances_result();
result.success = iface.getCurrentShopBalances(args.merchant_filter);
return result;
}
}
}
public static class AsyncProcessor extends org.apache.thrift.TBaseAsyncProcessor {
private static final org.slf4j.Logger _LOGGER = org.slf4j.LoggerFactory.getLogger(AsyncProcessor.class.getName());
public AsyncProcessor(I iface) {
super(iface, getProcessMap(new java.util.HashMap>()));
}
protected AsyncProcessor(I iface, java.util.Map> processMap) {
super(iface, getProcessMap(processMap));
}
private static java.util.Map> getProcessMap(java.util.Map> processMap) {
processMap.put("GetPaymentsToolDistribution", new GetPaymentsToolDistribution());
processMap.put("GetPaymentsAmount", new GetPaymentsAmount());
processMap.put("GetCreditingsAmount", new GetCreditingsAmount());
processMap.put("GetAveragePayment", new GetAveragePayment());
processMap.put("GetPaymentsCount", new GetPaymentsCount());
processMap.put("GetPaymentsErrorDistribution", new GetPaymentsErrorDistribution());
processMap.put("GetPaymentsSubErrorDistribution", new GetPaymentsSubErrorDistribution());
processMap.put("GetPaymentsSplitAmount", new GetPaymentsSplitAmount());
processMap.put("GetPaymentsSplitCount", new GetPaymentsSplitCount());
processMap.put("GetRefundsAmount", new GetRefundsAmount());
processMap.put("GetCurrentBalances", new GetCurrentBalances());
processMap.put("GetCurrentShopBalances", new GetCurrentShopBalances());
return processMap;
}
public static class GetPaymentsToolDistribution extends org.apache.thrift.AsyncProcessFunction {
public GetPaymentsToolDistribution() {
super("GetPaymentsToolDistribution");
}
public GetPaymentsToolDistribution_args getEmptyArgsInstance() {
return new GetPaymentsToolDistribution_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.PaymentToolDistributionResponse o) {
GetPaymentsToolDistribution_result result = new GetPaymentsToolDistribution_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetPaymentsToolDistribution_result result = new GetPaymentsToolDistribution_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetPaymentsToolDistribution_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getPaymentsToolDistribution(args.request,resultHandler);
}
}
public static class GetPaymentsAmount extends org.apache.thrift.AsyncProcessFunction {
public GetPaymentsAmount() {
super("GetPaymentsAmount");
}
public GetPaymentsAmount_args getEmptyArgsInstance() {
return new GetPaymentsAmount_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.AmountResponse o) {
GetPaymentsAmount_result result = new GetPaymentsAmount_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetPaymentsAmount_result result = new GetPaymentsAmount_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetPaymentsAmount_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getPaymentsAmount(args.request,resultHandler);
}
}
public static class GetCreditingsAmount extends org.apache.thrift.AsyncProcessFunction {
public GetCreditingsAmount() {
super("GetCreditingsAmount");
}
public GetCreditingsAmount_args getEmptyArgsInstance() {
return new GetCreditingsAmount_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.AmountResponse o) {
GetCreditingsAmount_result result = new GetCreditingsAmount_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetCreditingsAmount_result result = new GetCreditingsAmount_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetCreditingsAmount_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getCreditingsAmount(args.request,resultHandler);
}
}
public static class GetAveragePayment extends org.apache.thrift.AsyncProcessFunction {
public GetAveragePayment() {
super("GetAveragePayment");
}
public GetAveragePayment_args getEmptyArgsInstance() {
return new GetAveragePayment_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.AmountResponse o) {
GetAveragePayment_result result = new GetAveragePayment_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetAveragePayment_result result = new GetAveragePayment_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetAveragePayment_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getAveragePayment(args.request,resultHandler);
}
}
public static class GetPaymentsCount extends org.apache.thrift.AsyncProcessFunction {
public GetPaymentsCount() {
super("GetPaymentsCount");
}
public GetPaymentsCount_args getEmptyArgsInstance() {
return new GetPaymentsCount_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.CountResponse o) {
GetPaymentsCount_result result = new GetPaymentsCount_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetPaymentsCount_result result = new GetPaymentsCount_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetPaymentsCount_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getPaymentsCount(args.request,resultHandler);
}
}
public static class GetPaymentsErrorDistribution extends org.apache.thrift.AsyncProcessFunction {
public GetPaymentsErrorDistribution() {
super("GetPaymentsErrorDistribution");
}
public GetPaymentsErrorDistribution_args getEmptyArgsInstance() {
return new GetPaymentsErrorDistribution_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.ErrorDistributionsResponse o) {
GetPaymentsErrorDistribution_result result = new GetPaymentsErrorDistribution_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetPaymentsErrorDistribution_result result = new GetPaymentsErrorDistribution_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetPaymentsErrorDistribution_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getPaymentsErrorDistribution(args.request,resultHandler);
}
}
public static class GetPaymentsSubErrorDistribution extends org.apache.thrift.AsyncProcessFunction {
public GetPaymentsSubErrorDistribution() {
super("GetPaymentsSubErrorDistribution");
}
public GetPaymentsSubErrorDistribution_args getEmptyArgsInstance() {
return new GetPaymentsSubErrorDistribution_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.SubErrorDistributionsResponse o) {
GetPaymentsSubErrorDistribution_result result = new GetPaymentsSubErrorDistribution_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetPaymentsSubErrorDistribution_result result = new GetPaymentsSubErrorDistribution_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetPaymentsSubErrorDistribution_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getPaymentsSubErrorDistribution(args.request,resultHandler);
}
}
public static class GetPaymentsSplitAmount extends org.apache.thrift.AsyncProcessFunction {
public GetPaymentsSplitAmount() {
super("GetPaymentsSplitAmount");
}
public GetPaymentsSplitAmount_args getEmptyArgsInstance() {
return new GetPaymentsSplitAmount_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.SplitAmountResponse o) {
GetPaymentsSplitAmount_result result = new GetPaymentsSplitAmount_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetPaymentsSplitAmount_result result = new GetPaymentsSplitAmount_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetPaymentsSplitAmount_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getPaymentsSplitAmount(args.request,resultHandler);
}
}
public static class GetPaymentsSplitCount extends org.apache.thrift.AsyncProcessFunction {
public GetPaymentsSplitCount() {
super("GetPaymentsSplitCount");
}
public GetPaymentsSplitCount_args getEmptyArgsInstance() {
return new GetPaymentsSplitCount_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.SplitCountResponse o) {
GetPaymentsSplitCount_result result = new GetPaymentsSplitCount_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetPaymentsSplitCount_result result = new GetPaymentsSplitCount_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetPaymentsSplitCount_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getPaymentsSplitCount(args.request,resultHandler);
}
}
public static class GetRefundsAmount extends org.apache.thrift.AsyncProcessFunction {
public GetRefundsAmount() {
super("GetRefundsAmount");
}
public GetRefundsAmount_args getEmptyArgsInstance() {
return new GetRefundsAmount_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.AmountResponse o) {
GetRefundsAmount_result result = new GetRefundsAmount_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetRefundsAmount_result result = new GetRefundsAmount_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetRefundsAmount_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getRefundsAmount(args.request,resultHandler);
}
}
public static class GetCurrentBalances extends org.apache.thrift.AsyncProcessFunction {
public GetCurrentBalances() {
super("GetCurrentBalances");
}
public GetCurrentBalances_args getEmptyArgsInstance() {
return new GetCurrentBalances_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.AmountResponse o) {
GetCurrentBalances_result result = new GetCurrentBalances_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetCurrentBalances_result result = new GetCurrentBalances_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetCurrentBalances_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getCurrentBalances(args.merchant_filter,resultHandler);
}
}
public static class GetCurrentShopBalances extends org.apache.thrift.AsyncProcessFunction {
public GetCurrentShopBalances() {
super("GetCurrentShopBalances");
}
public GetCurrentShopBalances_args getEmptyArgsInstance() {
return new GetCurrentShopBalances_args();
}
public org.apache.thrift.async.AsyncMethodCallback getResultHandler(final org.apache.thrift.server.AbstractNonblockingServer.AsyncFrameBuffer fb, final int seqid) {
final org.apache.thrift.AsyncProcessFunction fcall = this;
return new org.apache.thrift.async.AsyncMethodCallback() {
public void onComplete(dev.vality.damsel.analytics.ShopAmountResponse o) {
GetCurrentShopBalances_result result = new GetCurrentShopBalances_result();
result.success = o;
try {
fcall.sendResponse(fb, result, org.apache.thrift.protocol.TMessageType.REPLY,seqid);
} catch (org.apache.thrift.transport.TTransportException e) {
_LOGGER.error("TTransportException writing to internal frame buffer", e);
fb.close();
} catch (java.lang.Exception e) {
_LOGGER.error("Exception writing to internal frame buffer", e);
onError(e);
}
}
public void onError(java.lang.Exception e) {
byte msgType = org.apache.thrift.protocol.TMessageType.REPLY;
org.apache.thrift.TSerializable msg;
GetCurrentShopBalances_result result = new GetCurrentShopBalances_result();
if (e instanceof org.apache.thrift.transport.TTransportException) {
_LOGGER.error("TTransportException inside handler", e);
fb.close();
return;
} else if (e instanceof org.apache.thrift.TApplicationException) {
_LOGGER.error("TApplicationException inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = (org.apache.thrift.TApplicationException)e;
} else {
_LOGGER.error("Exception inside handler", e);
msgType = org.apache.thrift.protocol.TMessageType.EXCEPTION;
msg = new org.apache.thrift.TApplicationException(org.apache.thrift.TApplicationException.INTERNAL_ERROR, e.getMessage());
}
try {
fcall.sendResponse(fb,msg,msgType,seqid);
} catch (java.lang.Exception ex) {
_LOGGER.error("Exception writing to internal frame buffer", ex);
fb.close();
}
}
};
}
protected boolean isOneway() {
return false;
}
public void start(I iface, GetCurrentShopBalances_args args, org.apache.thrift.async.AsyncMethodCallback resultHandler) throws org.apache.thrift.TException {
iface.getCurrentShopBalances(args.merchant_filter,resultHandler);
}
}
}
public static class GetPaymentsToolDistribution_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsToolDistribution_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsToolDistribution_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsToolDistribution_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable FilterRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, FilterRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsToolDistribution_args.class, metaDataMap);
}
public GetPaymentsToolDistribution_args() {
}
public GetPaymentsToolDistribution_args(
FilterRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsToolDistribution_args(GetPaymentsToolDistribution_args other) {
if (other.isSetRequest()) {
this.request = new FilterRequest(other.request);
}
}
public GetPaymentsToolDistribution_args deepCopy() {
return new GetPaymentsToolDistribution_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public FilterRequest getRequest() {
return this.request;
}
public GetPaymentsToolDistribution_args setRequest(@org.apache.thrift.annotation.Nullable FilterRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((FilterRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsToolDistribution_args)
return this.equals((GetPaymentsToolDistribution_args)that);
return false;
}
public boolean equals(GetPaymentsToolDistribution_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsToolDistribution_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsToolDistribution_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsToolDistribution_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsToolDistribution_argsStandardScheme getScheme() {
return new GetPaymentsToolDistribution_argsStandardScheme();
}
}
private static class GetPaymentsToolDistribution_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsToolDistribution_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsToolDistribution_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsToolDistribution_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsToolDistribution_argsTupleScheme getScheme() {
return new GetPaymentsToolDistribution_argsTupleScheme();
}
}
private static class GetPaymentsToolDistribution_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsToolDistribution_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsToolDistribution_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsToolDistribution_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsToolDistribution_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsToolDistribution_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsToolDistribution_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable PaymentToolDistributionResponse success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, PaymentToolDistributionResponse.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsToolDistribution_result.class, metaDataMap);
}
public GetPaymentsToolDistribution_result() {
}
public GetPaymentsToolDistribution_result(
PaymentToolDistributionResponse success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsToolDistribution_result(GetPaymentsToolDistribution_result other) {
if (other.isSetSuccess()) {
this.success = new PaymentToolDistributionResponse(other.success);
}
}
public GetPaymentsToolDistribution_result deepCopy() {
return new GetPaymentsToolDistribution_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public PaymentToolDistributionResponse getSuccess() {
return this.success;
}
public GetPaymentsToolDistribution_result setSuccess(@org.apache.thrift.annotation.Nullable PaymentToolDistributionResponse success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((PaymentToolDistributionResponse)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsToolDistribution_result)
return this.equals((GetPaymentsToolDistribution_result)that);
return false;
}
public boolean equals(GetPaymentsToolDistribution_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsToolDistribution_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsToolDistribution_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsToolDistribution_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsToolDistribution_resultStandardScheme getScheme() {
return new GetPaymentsToolDistribution_resultStandardScheme();
}
}
private static class GetPaymentsToolDistribution_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsToolDistribution_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new PaymentToolDistributionResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsToolDistribution_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsToolDistribution_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsToolDistribution_resultTupleScheme getScheme() {
return new GetPaymentsToolDistribution_resultTupleScheme();
}
}
private static class GetPaymentsToolDistribution_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsToolDistribution_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsToolDistribution_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new PaymentToolDistributionResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsAmount_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsAmount_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsAmount_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsAmount_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable FilterRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, FilterRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsAmount_args.class, metaDataMap);
}
public GetPaymentsAmount_args() {
}
public GetPaymentsAmount_args(
FilterRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsAmount_args(GetPaymentsAmount_args other) {
if (other.isSetRequest()) {
this.request = new FilterRequest(other.request);
}
}
public GetPaymentsAmount_args deepCopy() {
return new GetPaymentsAmount_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public FilterRequest getRequest() {
return this.request;
}
public GetPaymentsAmount_args setRequest(@org.apache.thrift.annotation.Nullable FilterRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((FilterRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsAmount_args)
return this.equals((GetPaymentsAmount_args)that);
return false;
}
public boolean equals(GetPaymentsAmount_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsAmount_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsAmount_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsAmount_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsAmount_argsStandardScheme getScheme() {
return new GetPaymentsAmount_argsStandardScheme();
}
}
private static class GetPaymentsAmount_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsAmount_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsAmount_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsAmount_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsAmount_argsTupleScheme getScheme() {
return new GetPaymentsAmount_argsTupleScheme();
}
}
private static class GetPaymentsAmount_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsAmount_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsAmount_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsAmount_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsAmount_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsAmount_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsAmount_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable AmountResponse success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, AmountResponse.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsAmount_result.class, metaDataMap);
}
public GetPaymentsAmount_result() {
}
public GetPaymentsAmount_result(
AmountResponse success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsAmount_result(GetPaymentsAmount_result other) {
if (other.isSetSuccess()) {
this.success = new AmountResponse(other.success);
}
}
public GetPaymentsAmount_result deepCopy() {
return new GetPaymentsAmount_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public AmountResponse getSuccess() {
return this.success;
}
public GetPaymentsAmount_result setSuccess(@org.apache.thrift.annotation.Nullable AmountResponse success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((AmountResponse)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsAmount_result)
return this.equals((GetPaymentsAmount_result)that);
return false;
}
public boolean equals(GetPaymentsAmount_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsAmount_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsAmount_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsAmount_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsAmount_resultStandardScheme getScheme() {
return new GetPaymentsAmount_resultStandardScheme();
}
}
private static class GetPaymentsAmount_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsAmount_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new AmountResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsAmount_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsAmount_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsAmount_resultTupleScheme getScheme() {
return new GetPaymentsAmount_resultTupleScheme();
}
}
private static class GetPaymentsAmount_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsAmount_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsAmount_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new AmountResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetCreditingsAmount_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetCreditingsAmount_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetCreditingsAmount_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetCreditingsAmount_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable FilterRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, FilterRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetCreditingsAmount_args.class, metaDataMap);
}
public GetCreditingsAmount_args() {
}
public GetCreditingsAmount_args(
FilterRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public GetCreditingsAmount_args(GetCreditingsAmount_args other) {
if (other.isSetRequest()) {
this.request = new FilterRequest(other.request);
}
}
public GetCreditingsAmount_args deepCopy() {
return new GetCreditingsAmount_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public FilterRequest getRequest() {
return this.request;
}
public GetCreditingsAmount_args setRequest(@org.apache.thrift.annotation.Nullable FilterRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((FilterRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetCreditingsAmount_args)
return this.equals((GetCreditingsAmount_args)that);
return false;
}
public boolean equals(GetCreditingsAmount_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(GetCreditingsAmount_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetCreditingsAmount_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetCreditingsAmount_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetCreditingsAmount_argsStandardScheme getScheme() {
return new GetCreditingsAmount_argsStandardScheme();
}
}
private static class GetCreditingsAmount_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetCreditingsAmount_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetCreditingsAmount_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetCreditingsAmount_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetCreditingsAmount_argsTupleScheme getScheme() {
return new GetCreditingsAmount_argsTupleScheme();
}
}
private static class GetCreditingsAmount_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetCreditingsAmount_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetCreditingsAmount_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetCreditingsAmount_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetCreditingsAmount_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetCreditingsAmount_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetCreditingsAmount_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable AmountResponse success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, AmountResponse.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetCreditingsAmount_result.class, metaDataMap);
}
public GetCreditingsAmount_result() {
}
public GetCreditingsAmount_result(
AmountResponse success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public GetCreditingsAmount_result(GetCreditingsAmount_result other) {
if (other.isSetSuccess()) {
this.success = new AmountResponse(other.success);
}
}
public GetCreditingsAmount_result deepCopy() {
return new GetCreditingsAmount_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public AmountResponse getSuccess() {
return this.success;
}
public GetCreditingsAmount_result setSuccess(@org.apache.thrift.annotation.Nullable AmountResponse success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((AmountResponse)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetCreditingsAmount_result)
return this.equals((GetCreditingsAmount_result)that);
return false;
}
public boolean equals(GetCreditingsAmount_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(GetCreditingsAmount_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetCreditingsAmount_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetCreditingsAmount_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetCreditingsAmount_resultStandardScheme getScheme() {
return new GetCreditingsAmount_resultStandardScheme();
}
}
private static class GetCreditingsAmount_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetCreditingsAmount_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new AmountResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetCreditingsAmount_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetCreditingsAmount_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetCreditingsAmount_resultTupleScheme getScheme() {
return new GetCreditingsAmount_resultTupleScheme();
}
}
private static class GetCreditingsAmount_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetCreditingsAmount_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetCreditingsAmount_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new AmountResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetAveragePayment_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetAveragePayment_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetAveragePayment_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetAveragePayment_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable FilterRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, FilterRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetAveragePayment_args.class, metaDataMap);
}
public GetAveragePayment_args() {
}
public GetAveragePayment_args(
FilterRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public GetAveragePayment_args(GetAveragePayment_args other) {
if (other.isSetRequest()) {
this.request = new FilterRequest(other.request);
}
}
public GetAveragePayment_args deepCopy() {
return new GetAveragePayment_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public FilterRequest getRequest() {
return this.request;
}
public GetAveragePayment_args setRequest(@org.apache.thrift.annotation.Nullable FilterRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((FilterRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetAveragePayment_args)
return this.equals((GetAveragePayment_args)that);
return false;
}
public boolean equals(GetAveragePayment_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(GetAveragePayment_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetAveragePayment_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetAveragePayment_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetAveragePayment_argsStandardScheme getScheme() {
return new GetAveragePayment_argsStandardScheme();
}
}
private static class GetAveragePayment_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetAveragePayment_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetAveragePayment_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetAveragePayment_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetAveragePayment_argsTupleScheme getScheme() {
return new GetAveragePayment_argsTupleScheme();
}
}
private static class GetAveragePayment_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetAveragePayment_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetAveragePayment_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetAveragePayment_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetAveragePayment_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetAveragePayment_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetAveragePayment_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable AmountResponse success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, AmountResponse.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetAveragePayment_result.class, metaDataMap);
}
public GetAveragePayment_result() {
}
public GetAveragePayment_result(
AmountResponse success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public GetAveragePayment_result(GetAveragePayment_result other) {
if (other.isSetSuccess()) {
this.success = new AmountResponse(other.success);
}
}
public GetAveragePayment_result deepCopy() {
return new GetAveragePayment_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public AmountResponse getSuccess() {
return this.success;
}
public GetAveragePayment_result setSuccess(@org.apache.thrift.annotation.Nullable AmountResponse success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((AmountResponse)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetAveragePayment_result)
return this.equals((GetAveragePayment_result)that);
return false;
}
public boolean equals(GetAveragePayment_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(GetAveragePayment_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetAveragePayment_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetAveragePayment_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetAveragePayment_resultStandardScheme getScheme() {
return new GetAveragePayment_resultStandardScheme();
}
}
private static class GetAveragePayment_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetAveragePayment_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new AmountResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetAveragePayment_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetAveragePayment_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetAveragePayment_resultTupleScheme getScheme() {
return new GetAveragePayment_resultTupleScheme();
}
}
private static class GetAveragePayment_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetAveragePayment_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetAveragePayment_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new AmountResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsCount_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsCount_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsCount_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsCount_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable FilterRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, FilterRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsCount_args.class, metaDataMap);
}
public GetPaymentsCount_args() {
}
public GetPaymentsCount_args(
FilterRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsCount_args(GetPaymentsCount_args other) {
if (other.isSetRequest()) {
this.request = new FilterRequest(other.request);
}
}
public GetPaymentsCount_args deepCopy() {
return new GetPaymentsCount_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public FilterRequest getRequest() {
return this.request;
}
public GetPaymentsCount_args setRequest(@org.apache.thrift.annotation.Nullable FilterRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((FilterRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsCount_args)
return this.equals((GetPaymentsCount_args)that);
return false;
}
public boolean equals(GetPaymentsCount_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsCount_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsCount_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsCount_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsCount_argsStandardScheme getScheme() {
return new GetPaymentsCount_argsStandardScheme();
}
}
private static class GetPaymentsCount_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsCount_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsCount_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsCount_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsCount_argsTupleScheme getScheme() {
return new GetPaymentsCount_argsTupleScheme();
}
}
private static class GetPaymentsCount_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsCount_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsCount_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsCount_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsCount_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsCount_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsCount_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable CountResponse success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, CountResponse.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsCount_result.class, metaDataMap);
}
public GetPaymentsCount_result() {
}
public GetPaymentsCount_result(
CountResponse success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsCount_result(GetPaymentsCount_result other) {
if (other.isSetSuccess()) {
this.success = new CountResponse(other.success);
}
}
public GetPaymentsCount_result deepCopy() {
return new GetPaymentsCount_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public CountResponse getSuccess() {
return this.success;
}
public GetPaymentsCount_result setSuccess(@org.apache.thrift.annotation.Nullable CountResponse success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((CountResponse)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsCount_result)
return this.equals((GetPaymentsCount_result)that);
return false;
}
public boolean equals(GetPaymentsCount_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsCount_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsCount_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsCount_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsCount_resultStandardScheme getScheme() {
return new GetPaymentsCount_resultStandardScheme();
}
}
private static class GetPaymentsCount_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsCount_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new CountResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsCount_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsCount_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsCount_resultTupleScheme getScheme() {
return new GetPaymentsCount_resultTupleScheme();
}
}
private static class GetPaymentsCount_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsCount_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsCount_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new CountResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsErrorDistribution_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsErrorDistribution_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsErrorDistribution_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsErrorDistribution_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable FilterRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, FilterRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsErrorDistribution_args.class, metaDataMap);
}
public GetPaymentsErrorDistribution_args() {
}
public GetPaymentsErrorDistribution_args(
FilterRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsErrorDistribution_args(GetPaymentsErrorDistribution_args other) {
if (other.isSetRequest()) {
this.request = new FilterRequest(other.request);
}
}
public GetPaymentsErrorDistribution_args deepCopy() {
return new GetPaymentsErrorDistribution_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public FilterRequest getRequest() {
return this.request;
}
public GetPaymentsErrorDistribution_args setRequest(@org.apache.thrift.annotation.Nullable FilterRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((FilterRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsErrorDistribution_args)
return this.equals((GetPaymentsErrorDistribution_args)that);
return false;
}
public boolean equals(GetPaymentsErrorDistribution_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsErrorDistribution_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsErrorDistribution_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsErrorDistribution_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsErrorDistribution_argsStandardScheme getScheme() {
return new GetPaymentsErrorDistribution_argsStandardScheme();
}
}
private static class GetPaymentsErrorDistribution_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsErrorDistribution_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsErrorDistribution_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsErrorDistribution_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsErrorDistribution_argsTupleScheme getScheme() {
return new GetPaymentsErrorDistribution_argsTupleScheme();
}
}
private static class GetPaymentsErrorDistribution_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsErrorDistribution_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsErrorDistribution_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsErrorDistribution_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsErrorDistribution_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsErrorDistribution_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsErrorDistribution_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable ErrorDistributionsResponse success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, ErrorDistributionsResponse.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsErrorDistribution_result.class, metaDataMap);
}
public GetPaymentsErrorDistribution_result() {
}
public GetPaymentsErrorDistribution_result(
ErrorDistributionsResponse success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsErrorDistribution_result(GetPaymentsErrorDistribution_result other) {
if (other.isSetSuccess()) {
this.success = new ErrorDistributionsResponse(other.success);
}
}
public GetPaymentsErrorDistribution_result deepCopy() {
return new GetPaymentsErrorDistribution_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public ErrorDistributionsResponse getSuccess() {
return this.success;
}
public GetPaymentsErrorDistribution_result setSuccess(@org.apache.thrift.annotation.Nullable ErrorDistributionsResponse success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((ErrorDistributionsResponse)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsErrorDistribution_result)
return this.equals((GetPaymentsErrorDistribution_result)that);
return false;
}
public boolean equals(GetPaymentsErrorDistribution_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsErrorDistribution_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsErrorDistribution_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsErrorDistribution_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsErrorDistribution_resultStandardScheme getScheme() {
return new GetPaymentsErrorDistribution_resultStandardScheme();
}
}
private static class GetPaymentsErrorDistribution_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsErrorDistribution_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new ErrorDistributionsResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsErrorDistribution_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsErrorDistribution_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsErrorDistribution_resultTupleScheme getScheme() {
return new GetPaymentsErrorDistribution_resultTupleScheme();
}
}
private static class GetPaymentsErrorDistribution_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsErrorDistribution_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsErrorDistribution_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new ErrorDistributionsResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsSubErrorDistribution_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsSubErrorDistribution_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsSubErrorDistribution_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsSubErrorDistribution_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable FilterRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, FilterRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsSubErrorDistribution_args.class, metaDataMap);
}
public GetPaymentsSubErrorDistribution_args() {
}
public GetPaymentsSubErrorDistribution_args(
FilterRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsSubErrorDistribution_args(GetPaymentsSubErrorDistribution_args other) {
if (other.isSetRequest()) {
this.request = new FilterRequest(other.request);
}
}
public GetPaymentsSubErrorDistribution_args deepCopy() {
return new GetPaymentsSubErrorDistribution_args(this);
}
@Override
public void clear() {
this.request = null;
}
@org.apache.thrift.annotation.Nullable
public FilterRequest getRequest() {
return this.request;
}
public GetPaymentsSubErrorDistribution_args setRequest(@org.apache.thrift.annotation.Nullable FilterRequest request) {
this.request = request;
return this;
}
public void unsetRequest() {
this.request = null;
}
/** Returns true if field request is set (has been assigned a value) and false otherwise */
public boolean isSetRequest() {
return this.request != null;
}
public void setRequestIsSet(boolean value) {
if (!value) {
this.request = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case REQUEST:
if (value == null) {
unsetRequest();
} else {
setRequest((FilterRequest)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case REQUEST:
return getRequest();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case REQUEST:
return isSetRequest();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsSubErrorDistribution_args)
return this.equals((GetPaymentsSubErrorDistribution_args)that);
return false;
}
public boolean equals(GetPaymentsSubErrorDistribution_args that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_request = true && this.isSetRequest();
boolean that_present_request = true && that.isSetRequest();
if (this_present_request || that_present_request) {
if (!(this_present_request && that_present_request))
return false;
if (!this.request.equals(that.request))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetRequest()) ? 131071 : 524287);
if (isSetRequest())
hashCode = hashCode * 8191 + request.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsSubErrorDistribution_args other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetRequest(), other.isSetRequest());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetRequest()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.request, other.request);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsSubErrorDistribution_args(");
boolean first = true;
sb.append("request:");
if (this.request == null) {
sb.append("null");
} else {
sb.append(this.request);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (request != null) {
request.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsSubErrorDistribution_argsStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsSubErrorDistribution_argsStandardScheme getScheme() {
return new GetPaymentsSubErrorDistribution_argsStandardScheme();
}
}
private static class GetPaymentsSubErrorDistribution_argsStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsSubErrorDistribution_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // REQUEST
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsSubErrorDistribution_args struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.request != null) {
oprot.writeFieldBegin(REQUEST_FIELD_DESC);
struct.request.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsSubErrorDistribution_argsTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsSubErrorDistribution_argsTupleScheme getScheme() {
return new GetPaymentsSubErrorDistribution_argsTupleScheme();
}
}
private static class GetPaymentsSubErrorDistribution_argsTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsSubErrorDistribution_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetRequest()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetRequest()) {
struct.request.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsSubErrorDistribution_args struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.request = new FilterRequest();
struct.request.read(iprot);
struct.setRequestIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsSubErrorDistribution_result implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsSubErrorDistribution_result");
private static final org.apache.thrift.protocol.TField SUCCESS_FIELD_DESC = new org.apache.thrift.protocol.TField("success", org.apache.thrift.protocol.TType.STRUCT, (short)0);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsSubErrorDistribution_resultStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsSubErrorDistribution_resultTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable SubErrorDistributionsResponse success; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
SUCCESS((short)0, "success");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 0: // SUCCESS
return SUCCESS;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.SUCCESS, new org.apache.thrift.meta_data.FieldMetaData("success", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, SubErrorDistributionsResponse.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsSubErrorDistribution_result.class, metaDataMap);
}
public GetPaymentsSubErrorDistribution_result() {
}
public GetPaymentsSubErrorDistribution_result(
SubErrorDistributionsResponse success)
{
this();
this.success = success;
}
/**
* Performs a deep copy on other.
*/
public GetPaymentsSubErrorDistribution_result(GetPaymentsSubErrorDistribution_result other) {
if (other.isSetSuccess()) {
this.success = new SubErrorDistributionsResponse(other.success);
}
}
public GetPaymentsSubErrorDistribution_result deepCopy() {
return new GetPaymentsSubErrorDistribution_result(this);
}
@Override
public void clear() {
this.success = null;
}
@org.apache.thrift.annotation.Nullable
public SubErrorDistributionsResponse getSuccess() {
return this.success;
}
public GetPaymentsSubErrorDistribution_result setSuccess(@org.apache.thrift.annotation.Nullable SubErrorDistributionsResponse success) {
this.success = success;
return this;
}
public void unsetSuccess() {
this.success = null;
}
/** Returns true if field success is set (has been assigned a value) and false otherwise */
public boolean isSetSuccess() {
return this.success != null;
}
public void setSuccessIsSet(boolean value) {
if (!value) {
this.success = null;
}
}
public void setFieldValue(_Fields field, @org.apache.thrift.annotation.Nullable java.lang.Object value) {
switch (field) {
case SUCCESS:
if (value == null) {
unsetSuccess();
} else {
setSuccess((SubErrorDistributionsResponse)value);
}
break;
}
}
@org.apache.thrift.annotation.Nullable
public java.lang.Object getFieldValue(_Fields field) {
switch (field) {
case SUCCESS:
return getSuccess();
}
throw new java.lang.IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new java.lang.IllegalArgumentException();
}
switch (field) {
case SUCCESS:
return isSetSuccess();
}
throw new java.lang.IllegalStateException();
}
@Override
public boolean equals(java.lang.Object that) {
if (that instanceof GetPaymentsSubErrorDistribution_result)
return this.equals((GetPaymentsSubErrorDistribution_result)that);
return false;
}
public boolean equals(GetPaymentsSubErrorDistribution_result that) {
if (that == null)
return false;
if (this == that)
return true;
boolean this_present_success = true && this.isSetSuccess();
boolean that_present_success = true && that.isSetSuccess();
if (this_present_success || that_present_success) {
if (!(this_present_success && that_present_success))
return false;
if (!this.success.equals(that.success))
return false;
}
return true;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = hashCode * 8191 + ((isSetSuccess()) ? 131071 : 524287);
if (isSetSuccess())
hashCode = hashCode * 8191 + success.hashCode();
return hashCode;
}
@Override
public int compareTo(GetPaymentsSubErrorDistribution_result other) {
if (!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = java.lang.Boolean.compare(isSetSuccess(), other.isSetSuccess());
if (lastComparison != 0) {
return lastComparison;
}
if (isSetSuccess()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.success, other.success);
if (lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
@org.apache.thrift.annotation.Nullable
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public _Fields[] getFields() {
return _Fields.values();
}
public java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> getFieldMetaData() {
return metaDataMap;
}
public void read(org.apache.thrift.protocol.TProtocol iprot) throws org.apache.thrift.TException {
scheme(iprot).read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot) throws org.apache.thrift.TException {
scheme(oprot).write(oprot, this);
}
@Override
public java.lang.String toString() {
java.lang.StringBuilder sb = new java.lang.StringBuilder("GetPaymentsSubErrorDistribution_result(");
boolean first = true;
sb.append("success:");
if (this.success == null) {
sb.append("null");
} else {
sb.append(this.success);
}
first = false;
sb.append(")");
return sb.toString();
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
// check for sub-struct validity
if (success != null) {
success.validate();
}
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, java.lang.ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class GetPaymentsSubErrorDistribution_resultStandardSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsSubErrorDistribution_resultStandardScheme getScheme() {
return new GetPaymentsSubErrorDistribution_resultStandardScheme();
}
}
private static class GetPaymentsSubErrorDistribution_resultStandardScheme extends org.apache.thrift.scheme.StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot, GetPaymentsSubErrorDistribution_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true)
{
schemeField = iprot.readFieldBegin();
if (schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 0: // SUCCESS
if (schemeField.type == org.apache.thrift.protocol.TType.STRUCT) {
struct.success = new SubErrorDistributionsResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot, GetPaymentsSubErrorDistribution_result struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if (struct.success != null) {
oprot.writeFieldBegin(SUCCESS_FIELD_DESC);
struct.success.write(oprot);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class GetPaymentsSubErrorDistribution_resultTupleSchemeFactory implements org.apache.thrift.scheme.SchemeFactory {
public GetPaymentsSubErrorDistribution_resultTupleScheme getScheme() {
return new GetPaymentsSubErrorDistribution_resultTupleScheme();
}
}
private static class GetPaymentsSubErrorDistribution_resultTupleScheme extends org.apache.thrift.scheme.TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot, GetPaymentsSubErrorDistribution_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol oprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet optionals = new java.util.BitSet();
if (struct.isSetSuccess()) {
optionals.set(0);
}
oprot.writeBitSet(optionals, 1);
if (struct.isSetSuccess()) {
struct.success.write(oprot);
}
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot, GetPaymentsSubErrorDistribution_result struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TTupleProtocol iprot = (org.apache.thrift.protocol.TTupleProtocol) prot;
java.util.BitSet incoming = iprot.readBitSet(1);
if (incoming.get(0)) {
struct.success = new SubErrorDistributionsResponse();
struct.success.read(iprot);
struct.setSuccessIsSet(true);
}
}
}
private static S scheme(org.apache.thrift.protocol.TProtocol proto) {
return (org.apache.thrift.scheme.StandardScheme.class.equals(proto.getScheme()) ? STANDARD_SCHEME_FACTORY : TUPLE_SCHEME_FACTORY).getScheme();
}
}
public static class GetPaymentsSplitAmount_args implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("GetPaymentsSplitAmount_args");
private static final org.apache.thrift.protocol.TField REQUEST_FIELD_DESC = new org.apache.thrift.protocol.TField("request", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.scheme.SchemeFactory STANDARD_SCHEME_FACTORY = new GetPaymentsSplitAmount_argsStandardSchemeFactory();
private static final org.apache.thrift.scheme.SchemeFactory TUPLE_SCHEME_FACTORY = new GetPaymentsSplitAmount_argsTupleSchemeFactory();
public @org.apache.thrift.annotation.Nullable SplitFilterRequest request; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
REQUEST((short)1, "request");
private static final java.util.Map byName = new java.util.HashMap();
static {
for (_Fields field : java.util.EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // REQUEST
return REQUEST;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new java.lang.IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
@org.apache.thrift.annotation.Nullable
public static _Fields findByName(java.lang.String name) {
return byName.get(name);
}
private final short _thriftId;
private final java.lang.String _fieldName;
_Fields(short thriftId, java.lang.String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public java.lang.String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
java.util.Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new java.util.EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.REQUEST, new org.apache.thrift.meta_data.FieldMetaData("request", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, SplitFilterRequest.class)));
metaDataMap = java.util.Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(GetPaymentsSplitAmount_args.class, metaDataMap);
}
public GetPaymentsSplitAmount_args() {
}
public GetPaymentsSplitAmount_args(
SplitFilterRequest request)
{
this();
this.request = request;
}
/**
* Performs a deep copy on