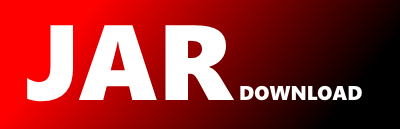
dev.vality.fraudo.FraudoPaymentParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fraudo Show documentation
Show all versions of fraudo Show documentation
Language for describing antifraud patterns
// Generated from dev.vality.fraudo/FraudoPayment.g4 by ANTLR 4.7.1
package dev.vality.fraudo;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class FraudoPaymentParser extends Parser {
static { RuntimeMetaData.checkVersion("4.7.1", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
T__0=1, T__1=2, T__2=3, T__3=4, T__4=5, T__5=6, T__6=7, T__7=8, T__8=9,
T__9=10, T__10=11, T__11=12, T__12=13, T__13=14, T__14=15, T__15=16, T__16=17,
T__17=18, T__18=19, T__19=20, T__20=21, T__21=22, T__22=23, T__23=24,
T__24=25, T__25=26, T__26=27, T__27=28, T__28=29, T__29=30, T__30=31,
T__31=32, T__32=33, T__33=34, T__34=35, T__35=36, T__36=37, T__37=38,
T__38=39, T__39=40, T__40=41, T__41=42, T__42=43, T__43=44, T__44=45,
STRING=46, DELIMITER=47, COMMENT=48, RETURN=49, CATCH_ERROR=50, RULE_BLOCK=51,
AND=52, OR=53, NOT=54, TRUE=55, FALSE=56, GT=57, GE=58, LT=59, LE=60,
EQ=61, NEQ=62, LPAREN=63, RPAREN=64, DECIMAL=65, INTEGER=66, IDENTIFIER=67,
WS=68, SCOL=69, BOOLEAN=70;
public static final int
RULE_parse = 0, RULE_expression = 1, RULE_booleanAndExpression = 2, RULE_equalityExpression = 3,
RULE_stringExpression = 4, RULE_relationalExpression = 5, RULE_unaryExpression = 6,
RULE_integerExpression = 7, RULE_floatExpression = 8, RULE_count_success = 9,
RULE_count_pending = 10, RULE_count_error = 11, RULE_count_chargeback = 12,
RULE_count_refund = 13, RULE_sum_success = 14, RULE_sum_error = 15, RULE_sum_chargeback = 16,
RULE_sum_refund = 17, RULE_in = 18, RULE_is_mobile = 19, RULE_is_recurrent = 20,
RULE_is_trusted = 21, RULE_payment_conditions = 22, RULE_withdrawal_conditions = 23,
RULE_conditions_list = 24, RULE_trusted_token_condition = 25, RULE_rand = 26,
RULE_fraud_rule = 27, RULE_comparator = 28, RULE_binary = 29, RULE_bool = 30,
RULE_amount = 31, RULE_currency = 32, RULE_payment_system = 33, RULE_card_category = 34,
RULE_payer_type = 35, RULE_token_provider = 36, RULE_count = 37, RULE_sum = 38,
RULE_unique = 39, RULE_in_white_list = 40, RULE_in_black_list = 41, RULE_in_grey_list = 42,
RULE_in_list = 43, RULE_like = 44, RULE_country_by = 45, RULE_result = 46,
RULE_catch_result = 47, RULE_string_list = 48, RULE_time_window = 49,
RULE_time_unit = 50, RULE_group_by = 51;
public static final String[] ruleNames = {
"parse", "expression", "booleanAndExpression", "equalityExpression", "stringExpression",
"relationalExpression", "unaryExpression", "integerExpression", "floatExpression",
"count_success", "count_pending", "count_error", "count_chargeback", "count_refund",
"sum_success", "sum_error", "sum_chargeback", "sum_refund", "in", "is_mobile",
"is_recurrent", "is_trusted", "payment_conditions", "withdrawal_conditions",
"conditions_list", "trusted_token_condition", "rand", "fraud_rule", "comparator",
"binary", "bool", "amount", "currency", "payment_system", "card_category",
"payer_type", "token_provider", "count", "sum", "unique", "in_white_list",
"in_black_list", "in_grey_list", "in_list", "like", "country_by", "result",
"catch_result", "string_list", "time_window", "time_unit", "group_by"
};
private static final String[] _LITERAL_NAMES = {
null, "'countSuccess'", "'countPending'", "'countError'", "'countChargeback'",
"'countRefund'", "'sumSuccess'", "'sumError'", "'sumChargeback'", "'sumRefund'",
"'in'", "'isMobile'", "'isRecurrent'", "'isTrusted'", "'paymentsConditions'",
"'withdrawalsConditions'", "'condition'", "'rand'", "':'", "'amount'",
"'currency'", "'paymentSystem'", "'cardCategory'", "'payerType'", "'tokenProvider'",
"'count'", "'sum'", "'unique'", "'inWhiteList'", "'inBlackList'", "'inGreyList'",
"'inList'", "'like'", "'countryBy'", "'accept'", "'3ds'", "'highRisk'",
"'decline'", "'notify'", "'declineAndNotify'", "'acceptAndNotify'", "'minutes'",
"'hours'", "'days'", "'calendar_months'", "'calendar_days'", null, "','",
null, "'->'", "'catch:'", "'rule:'", null, null, null, null, null, "'>'",
"'>='", "'<'", "'<='", "'='", "'!='", "'('", "')'", null, null, null,
null, "';'"
};
private static final String[] _SYMBOLIC_NAMES = {
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, null, null,
null, null, null, null, null, null, null, null, null, null, "STRING",
"DELIMITER", "COMMENT", "RETURN", "CATCH_ERROR", "RULE_BLOCK", "AND",
"OR", "NOT", "TRUE", "FALSE", "GT", "GE", "LT", "LE", "EQ", "NEQ", "LPAREN",
"RPAREN", "DECIMAL", "INTEGER", "IDENTIFIER", "WS", "SCOL", "BOOLEAN"
};
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "FraudoPayment.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public FraudoPaymentParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class ParseContext extends ParserRuleContext {
public TerminalNode EOF() { return getToken(FraudoPaymentParser.EOF, 0); }
public List fraud_rule() {
return getRuleContexts(Fraud_ruleContext.class);
}
public Fraud_ruleContext fraud_rule(int i) {
return getRuleContext(Fraud_ruleContext.class,i);
}
public ParseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parse; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitParse(this);
else return visitor.visitChildren(this);
}
}
public final ParseContext parse() throws RecognitionException {
ParseContext _localctx = new ParseContext(_ctx, getState());
enterRule(_localctx, 0, RULE_parse);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(107);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==RULE_BLOCK) {
{
{
setState(104);
fraud_rule();
}
}
setState(109);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(110);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ExpressionContext extends ParserRuleContext {
public List booleanAndExpression() {
return getRuleContexts(BooleanAndExpressionContext.class);
}
public BooleanAndExpressionContext booleanAndExpression(int i) {
return getRuleContext(BooleanAndExpressionContext.class,i);
}
public List OR() { return getTokens(FraudoPaymentParser.OR); }
public TerminalNode OR(int i) {
return getToken(FraudoPaymentParser.OR, i);
}
public ExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_expression; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitExpression(this);
else return visitor.visitChildren(this);
}
}
public final ExpressionContext expression() throws RecognitionException {
ExpressionContext _localctx = new ExpressionContext(_ctx, getState());
enterRule(_localctx, 2, RULE_expression);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(112);
booleanAndExpression();
setState(117);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,1,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(113);
match(OR);
setState(114);
booleanAndExpression();
}
}
}
setState(119);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,1,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BooleanAndExpressionContext extends ParserRuleContext {
public List equalityExpression() {
return getRuleContexts(EqualityExpressionContext.class);
}
public EqualityExpressionContext equalityExpression(int i) {
return getRuleContext(EqualityExpressionContext.class,i);
}
public List AND() { return getTokens(FraudoPaymentParser.AND); }
public TerminalNode AND(int i) {
return getToken(FraudoPaymentParser.AND, i);
}
public BooleanAndExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_booleanAndExpression; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitBooleanAndExpression(this);
else return visitor.visitChildren(this);
}
}
public final BooleanAndExpressionContext booleanAndExpression() throws RecognitionException {
BooleanAndExpressionContext _localctx = new BooleanAndExpressionContext(_ctx, getState());
enterRule(_localctx, 4, RULE_booleanAndExpression);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(120);
equalityExpression();
setState(125);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,2,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(121);
match(AND);
setState(122);
equalityExpression();
}
}
}
setState(127);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,2,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class EqualityExpressionContext extends ParserRuleContext {
public RelationalExpressionContext relationalExpression() {
return getRuleContext(RelationalExpressionContext.class,0);
}
public List stringExpression() {
return getRuleContexts(StringExpressionContext.class);
}
public StringExpressionContext stringExpression(int i) {
return getRuleContext(StringExpressionContext.class,i);
}
public TerminalNode EQ() { return getToken(FraudoPaymentParser.EQ, 0); }
public TerminalNode NEQ() { return getToken(FraudoPaymentParser.NEQ, 0); }
public TerminalNode NOT() { return getToken(FraudoPaymentParser.NOT, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public EqualityExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_equalityExpression; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitEqualityExpression(this);
else return visitor.visitChildren(this);
}
}
public final EqualityExpressionContext equalityExpression() throws RecognitionException {
EqualityExpressionContext _localctx = new EqualityExpressionContext(_ctx, getState());
enterRule(_localctx, 6, RULE_equalityExpression);
int _la;
try {
setState(139);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__0:
case T__1:
case T__2:
case T__3:
case T__4:
case T__5:
case T__6:
case T__7:
case T__8:
case T__9:
case T__10:
case T__11:
case T__12:
case T__16:
case T__18:
case T__24:
case T__25:
case T__26:
case T__27:
case T__28:
case T__29:
case T__30:
case T__31:
case DECIMAL:
case INTEGER:
enterOuterAlt(_localctx, 1);
{
setState(128);
relationalExpression();
}
break;
case T__19:
case T__20:
case T__21:
case T__32:
case STRING:
enterOuterAlt(_localctx, 2);
{
setState(129);
stringExpression();
setState(130);
_la = _input.LA(1);
if ( !(_la==EQ || _la==NEQ) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(131);
stringExpression();
}
break;
case NOT:
enterOuterAlt(_localctx, 3);
{
setState(133);
match(NOT);
setState(134);
expression();
}
break;
case LPAREN:
enterOuterAlt(_localctx, 4);
{
setState(135);
match(LPAREN);
setState(136);
expression();
setState(137);
match(RPAREN);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class StringExpressionContext extends ParserRuleContext {
public Country_byContext country_by() {
return getRuleContext(Country_byContext.class,0);
}
public CurrencyContext currency() {
return getRuleContext(CurrencyContext.class,0);
}
public Payment_systemContext payment_system() {
return getRuleContext(Payment_systemContext.class,0);
}
public Card_categoryContext card_category() {
return getRuleContext(Card_categoryContext.class,0);
}
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public StringExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_stringExpression; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitStringExpression(this);
else return visitor.visitChildren(this);
}
}
public final StringExpressionContext stringExpression() throws RecognitionException {
StringExpressionContext _localctx = new StringExpressionContext(_ctx, getState());
enterRule(_localctx, 8, RULE_stringExpression);
try {
setState(146);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__32:
enterOuterAlt(_localctx, 1);
{
setState(141);
country_by();
}
break;
case T__19:
enterOuterAlt(_localctx, 2);
{
setState(142);
currency();
}
break;
case T__20:
enterOuterAlt(_localctx, 3);
{
setState(143);
payment_system();
}
break;
case T__21:
enterOuterAlt(_localctx, 4);
{
setState(144);
card_category();
}
break;
case STRING:
enterOuterAlt(_localctx, 5);
{
setState(145);
match(STRING);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class RelationalExpressionContext extends ParserRuleContext {
public List unaryExpression() {
return getRuleContexts(UnaryExpressionContext.class);
}
public UnaryExpressionContext unaryExpression(int i) {
return getRuleContext(UnaryExpressionContext.class,i);
}
public TerminalNode LT() { return getToken(FraudoPaymentParser.LT, 0); }
public TerminalNode LE() { return getToken(FraudoPaymentParser.LE, 0); }
public TerminalNode GT() { return getToken(FraudoPaymentParser.GT, 0); }
public TerminalNode GE() { return getToken(FraudoPaymentParser.GE, 0); }
public TerminalNode EQ() { return getToken(FraudoPaymentParser.EQ, 0); }
public TerminalNode NEQ() { return getToken(FraudoPaymentParser.NEQ, 0); }
public InContext in() {
return getRuleContext(InContext.class,0);
}
public In_white_listContext in_white_list() {
return getRuleContext(In_white_listContext.class,0);
}
public In_black_listContext in_black_list() {
return getRuleContext(In_black_listContext.class,0);
}
public In_grey_listContext in_grey_list() {
return getRuleContext(In_grey_listContext.class,0);
}
public In_listContext in_list() {
return getRuleContext(In_listContext.class,0);
}
public LikeContext like() {
return getRuleContext(LikeContext.class,0);
}
public Is_mobileContext is_mobile() {
return getRuleContext(Is_mobileContext.class,0);
}
public Is_recurrentContext is_recurrent() {
return getRuleContext(Is_recurrentContext.class,0);
}
public Is_trustedContext is_trusted() {
return getRuleContext(Is_trustedContext.class,0);
}
public RelationalExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_relationalExpression; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitRelationalExpression(this);
else return visitor.visitChildren(this);
}
}
public final RelationalExpressionContext relationalExpression() throws RecognitionException {
RelationalExpressionContext _localctx = new RelationalExpressionContext(_ctx, getState());
enterRule(_localctx, 10, RULE_relationalExpression);
int _la;
try {
setState(161);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__0:
case T__1:
case T__2:
case T__3:
case T__4:
case T__5:
case T__6:
case T__7:
case T__8:
case T__16:
case T__18:
case T__24:
case T__25:
case T__26:
case DECIMAL:
case INTEGER:
enterOuterAlt(_localctx, 1);
{
setState(148);
unaryExpression();
setState(149);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << GT) | (1L << GE) | (1L << LT) | (1L << LE) | (1L << EQ) | (1L << NEQ))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(150);
unaryExpression();
}
break;
case T__9:
enterOuterAlt(_localctx, 2);
{
setState(152);
in();
}
break;
case T__27:
enterOuterAlt(_localctx, 3);
{
setState(153);
in_white_list();
}
break;
case T__28:
enterOuterAlt(_localctx, 4);
{
setState(154);
in_black_list();
}
break;
case T__29:
enterOuterAlt(_localctx, 5);
{
setState(155);
in_grey_list();
}
break;
case T__30:
enterOuterAlt(_localctx, 6);
{
setState(156);
in_list();
}
break;
case T__31:
enterOuterAlt(_localctx, 7);
{
setState(157);
like();
}
break;
case T__10:
enterOuterAlt(_localctx, 8);
{
setState(158);
is_mobile();
}
break;
case T__11:
enterOuterAlt(_localctx, 9);
{
setState(159);
is_recurrent();
}
break;
case T__12:
enterOuterAlt(_localctx, 10);
{
setState(160);
is_trusted();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class UnaryExpressionContext extends ParserRuleContext {
public IntegerExpressionContext integerExpression() {
return getRuleContext(IntegerExpressionContext.class,0);
}
public FloatExpressionContext floatExpression() {
return getRuleContext(FloatExpressionContext.class,0);
}
public UnaryExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unaryExpression; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitUnaryExpression(this);
else return visitor.visitChildren(this);
}
}
public final UnaryExpressionContext unaryExpression() throws RecognitionException {
UnaryExpressionContext _localctx = new UnaryExpressionContext(_ctx, getState());
enterRule(_localctx, 12, RULE_unaryExpression);
try {
setState(165);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__0:
case T__1:
case T__2:
case T__3:
case T__4:
case T__16:
case T__24:
case T__26:
case INTEGER:
enterOuterAlt(_localctx, 1);
{
setState(163);
integerExpression();
}
break;
case T__5:
case T__6:
case T__7:
case T__8:
case T__18:
case T__25:
case DECIMAL:
enterOuterAlt(_localctx, 2);
{
setState(164);
floatExpression();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class IntegerExpressionContext extends ParserRuleContext {
public CountContext count() {
return getRuleContext(CountContext.class,0);
}
public Count_pendingContext count_pending() {
return getRuleContext(Count_pendingContext.class,0);
}
public Count_successContext count_success() {
return getRuleContext(Count_successContext.class,0);
}
public Count_errorContext count_error() {
return getRuleContext(Count_errorContext.class,0);
}
public Count_chargebackContext count_chargeback() {
return getRuleContext(Count_chargebackContext.class,0);
}
public Count_refundContext count_refund() {
return getRuleContext(Count_refundContext.class,0);
}
public UniqueContext unique() {
return getRuleContext(UniqueContext.class,0);
}
public RandContext rand() {
return getRuleContext(RandContext.class,0);
}
public TerminalNode INTEGER() { return getToken(FraudoPaymentParser.INTEGER, 0); }
public IntegerExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_integerExpression; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIntegerExpression(this);
else return visitor.visitChildren(this);
}
}
public final IntegerExpressionContext integerExpression() throws RecognitionException {
IntegerExpressionContext _localctx = new IntegerExpressionContext(_ctx, getState());
enterRule(_localctx, 14, RULE_integerExpression);
try {
setState(176);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__24:
enterOuterAlt(_localctx, 1);
{
setState(167);
count();
}
break;
case T__1:
enterOuterAlt(_localctx, 2);
{
setState(168);
count_pending();
}
break;
case T__0:
enterOuterAlt(_localctx, 3);
{
setState(169);
count_success();
}
break;
case T__2:
enterOuterAlt(_localctx, 4);
{
setState(170);
count_error();
}
break;
case T__3:
enterOuterAlt(_localctx, 5);
{
setState(171);
count_chargeback();
}
break;
case T__4:
enterOuterAlt(_localctx, 6);
{
setState(172);
count_refund();
}
break;
case T__26:
enterOuterAlt(_localctx, 7);
{
setState(173);
unique();
}
break;
case T__16:
enterOuterAlt(_localctx, 8);
{
setState(174);
rand();
}
break;
case INTEGER:
enterOuterAlt(_localctx, 9);
{
setState(175);
match(INTEGER);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FloatExpressionContext extends ParserRuleContext {
public SumContext sum() {
return getRuleContext(SumContext.class,0);
}
public Sum_successContext sum_success() {
return getRuleContext(Sum_successContext.class,0);
}
public Sum_errorContext sum_error() {
return getRuleContext(Sum_errorContext.class,0);
}
public Sum_chargebackContext sum_chargeback() {
return getRuleContext(Sum_chargebackContext.class,0);
}
public Sum_refundContext sum_refund() {
return getRuleContext(Sum_refundContext.class,0);
}
public AmountContext amount() {
return getRuleContext(AmountContext.class,0);
}
public TerminalNode DECIMAL() { return getToken(FraudoPaymentParser.DECIMAL, 0); }
public FloatExpressionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_floatExpression; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitFloatExpression(this);
else return visitor.visitChildren(this);
}
}
public final FloatExpressionContext floatExpression() throws RecognitionException {
FloatExpressionContext _localctx = new FloatExpressionContext(_ctx, getState());
enterRule(_localctx, 16, RULE_floatExpression);
try {
setState(185);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__25:
enterOuterAlt(_localctx, 1);
{
setState(178);
sum();
}
break;
case T__5:
enterOuterAlt(_localctx, 2);
{
setState(179);
sum_success();
}
break;
case T__6:
enterOuterAlt(_localctx, 3);
{
setState(180);
sum_error();
}
break;
case T__7:
enterOuterAlt(_localctx, 4);
{
setState(181);
sum_chargeback();
}
break;
case T__8:
enterOuterAlt(_localctx, 5);
{
setState(182);
sum_refund();
}
break;
case T__18:
enterOuterAlt(_localctx, 6);
{
setState(183);
amount();
}
break;
case DECIMAL:
enterOuterAlt(_localctx, 7);
{
setState(184);
match(DECIMAL);
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Count_successContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public Count_successContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_count_success; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCount_success(this);
else return visitor.visitChildren(this);
}
}
public final Count_successContext count_success() throws RecognitionException {
Count_successContext _localctx = new Count_successContext(_ctx, getState());
enterRule(_localctx, 18, RULE_count_success);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(187);
match(T__0);
setState(188);
match(LPAREN);
setState(189);
match(STRING);
setState(190);
time_window();
setState(192);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(191);
group_by();
}
}
setState(194);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Count_pendingContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public Count_pendingContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_count_pending; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCount_pending(this);
else return visitor.visitChildren(this);
}
}
public final Count_pendingContext count_pending() throws RecognitionException {
Count_pendingContext _localctx = new Count_pendingContext(_ctx, getState());
enterRule(_localctx, 20, RULE_count_pending);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(196);
match(T__1);
setState(197);
match(LPAREN);
setState(198);
match(STRING);
setState(199);
time_window();
setState(201);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(200);
group_by();
}
}
setState(203);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Count_errorContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public List STRING() { return getTokens(FraudoPaymentParser.STRING); }
public TerminalNode STRING(int i) {
return getToken(FraudoPaymentParser.STRING, i);
}
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public TerminalNode DELIMITER() { return getToken(FraudoPaymentParser.DELIMITER, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public Count_errorContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_count_error; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCount_error(this);
else return visitor.visitChildren(this);
}
}
public final Count_errorContext count_error() throws RecognitionException {
Count_errorContext _localctx = new Count_errorContext(_ctx, getState());
enterRule(_localctx, 22, RULE_count_error);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(205);
match(T__2);
setState(206);
match(LPAREN);
setState(207);
match(STRING);
setState(208);
time_window();
setState(211);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,11,_ctx) ) {
case 1:
{
setState(209);
match(DELIMITER);
setState(210);
match(STRING);
}
break;
}
setState(214);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(213);
group_by();
}
}
setState(216);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Count_chargebackContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public Count_chargebackContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_count_chargeback; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCount_chargeback(this);
else return visitor.visitChildren(this);
}
}
public final Count_chargebackContext count_chargeback() throws RecognitionException {
Count_chargebackContext _localctx = new Count_chargebackContext(_ctx, getState());
enterRule(_localctx, 24, RULE_count_chargeback);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(218);
match(T__3);
setState(219);
match(LPAREN);
setState(220);
match(STRING);
setState(221);
time_window();
setState(223);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(222);
group_by();
}
}
setState(225);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Count_refundContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public Count_refundContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_count_refund; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCount_refund(this);
else return visitor.visitChildren(this);
}
}
public final Count_refundContext count_refund() throws RecognitionException {
Count_refundContext _localctx = new Count_refundContext(_ctx, getState());
enterRule(_localctx, 26, RULE_count_refund);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(227);
match(T__4);
setState(228);
match(LPAREN);
setState(229);
match(STRING);
setState(230);
time_window();
setState(232);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(231);
group_by();
}
}
setState(234);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Sum_successContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public Sum_successContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_sum_success; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitSum_success(this);
else return visitor.visitChildren(this);
}
}
public final Sum_successContext sum_success() throws RecognitionException {
Sum_successContext _localctx = new Sum_successContext(_ctx, getState());
enterRule(_localctx, 28, RULE_sum_success);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(236);
match(T__5);
setState(237);
match(LPAREN);
setState(238);
match(STRING);
setState(239);
time_window();
setState(241);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(240);
group_by();
}
}
setState(243);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Sum_errorContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public List STRING() { return getTokens(FraudoPaymentParser.STRING); }
public TerminalNode STRING(int i) {
return getToken(FraudoPaymentParser.STRING, i);
}
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public TerminalNode DELIMITER() { return getToken(FraudoPaymentParser.DELIMITER, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public Sum_errorContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_sum_error; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitSum_error(this);
else return visitor.visitChildren(this);
}
}
public final Sum_errorContext sum_error() throws RecognitionException {
Sum_errorContext _localctx = new Sum_errorContext(_ctx, getState());
enterRule(_localctx, 30, RULE_sum_error);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(245);
match(T__6);
setState(246);
match(LPAREN);
setState(247);
match(STRING);
setState(248);
time_window();
setState(251);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,16,_ctx) ) {
case 1:
{
setState(249);
match(DELIMITER);
setState(250);
match(STRING);
}
break;
}
setState(254);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(253);
group_by();
}
}
setState(256);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Sum_chargebackContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public Sum_chargebackContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_sum_chargeback; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitSum_chargeback(this);
else return visitor.visitChildren(this);
}
}
public final Sum_chargebackContext sum_chargeback() throws RecognitionException {
Sum_chargebackContext _localctx = new Sum_chargebackContext(_ctx, getState());
enterRule(_localctx, 32, RULE_sum_chargeback);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(258);
match(T__7);
setState(259);
match(LPAREN);
setState(260);
match(STRING);
setState(261);
time_window();
setState(263);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(262);
group_by();
}
}
setState(265);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Sum_refundContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public Sum_refundContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_sum_refund; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitSum_refund(this);
else return visitor.visitChildren(this);
}
}
public final Sum_refundContext sum_refund() throws RecognitionException {
Sum_refundContext _localctx = new Sum_refundContext(_ctx, getState());
enterRule(_localctx, 34, RULE_sum_refund);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(267);
match(T__8);
setState(268);
match(LPAREN);
setState(269);
match(STRING);
setState(270);
time_window();
setState(272);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(271);
group_by();
}
}
setState(274);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class InContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode DELIMITER() { return getToken(FraudoPaymentParser.DELIMITER, 0); }
public String_listContext string_list() {
return getRuleContext(String_listContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public StringExpressionContext stringExpression() {
return getRuleContext(StringExpressionContext.class,0);
}
public InContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_in; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIn(this);
else return visitor.visitChildren(this);
}
}
public final InContext in() throws RecognitionException {
InContext _localctx = new InContext(_ctx, getState());
enterRule(_localctx, 36, RULE_in);
try {
enterOuterAlt(_localctx, 1);
{
setState(276);
match(T__9);
setState(277);
match(LPAREN);
{
setState(278);
stringExpression();
}
setState(279);
match(DELIMITER);
setState(280);
string_list();
setState(281);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Is_mobileContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Is_mobileContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_is_mobile; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIs_mobile(this);
else return visitor.visitChildren(this);
}
}
public final Is_mobileContext is_mobile() throws RecognitionException {
Is_mobileContext _localctx = new Is_mobileContext(_ctx, getState());
enterRule(_localctx, 38, RULE_is_mobile);
try {
enterOuterAlt(_localctx, 1);
{
setState(283);
match(T__10);
setState(284);
match(LPAREN);
setState(285);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Is_recurrentContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Is_recurrentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_is_recurrent; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIs_recurrent(this);
else return visitor.visitChildren(this);
}
}
public final Is_recurrentContext is_recurrent() throws RecognitionException {
Is_recurrentContext _localctx = new Is_recurrentContext(_ctx, getState());
enterRule(_localctx, 40, RULE_is_recurrent);
try {
enterOuterAlt(_localctx, 1);
{
setState(287);
match(T__11);
setState(288);
match(LPAREN);
setState(289);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Is_trustedContext extends ParserRuleContext {
public Is_trustedContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_is_trusted; }
public Is_trustedContext() { }
public void copyFrom(Is_trustedContext ctx) {
super.copyFrom(ctx);
}
}
public static class IsTrustedConditionsSingleListContext extends Is_trustedContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Payment_conditionsContext payment_conditions() {
return getRuleContext(Payment_conditionsContext.class,0);
}
public Withdrawal_conditionsContext withdrawal_conditions() {
return getRuleContext(Withdrawal_conditionsContext.class,0);
}
public IsTrustedConditionsSingleListContext(Is_trustedContext ctx) { copyFrom(ctx); }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIsTrustedConditionsSingleList(this);
else return visitor.visitChildren(this);
}
}
public static class IsTrustedPaymentsAndWithdrawalConditionsContext extends Is_trustedContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public Payment_conditionsContext payment_conditions() {
return getRuleContext(Payment_conditionsContext.class,0);
}
public TerminalNode DELIMITER() { return getToken(FraudoPaymentParser.DELIMITER, 0); }
public Withdrawal_conditionsContext withdrawal_conditions() {
return getRuleContext(Withdrawal_conditionsContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public IsTrustedPaymentsAndWithdrawalConditionsContext(Is_trustedContext ctx) { copyFrom(ctx); }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIsTrustedPaymentsAndWithdrawalConditions(this);
else return visitor.visitChildren(this);
}
}
public static class IsTrustedTemplateNameContext extends Is_trustedContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public IsTrustedTemplateNameContext(Is_trustedContext ctx) { copyFrom(ctx); }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIsTrustedTemplateName(this);
else return visitor.visitChildren(this);
}
}
public static class IsTrustedContext extends Is_trustedContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public IsTrustedContext(Is_trustedContext ctx) { copyFrom(ctx); }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIsTrusted(this);
else return visitor.visitChildren(this);
}
}
public final Is_trustedContext is_trusted() throws RecognitionException {
Is_trustedContext _localctx = new Is_trustedContext(_ctx, getState());
enterRule(_localctx, 42, RULE_is_trusted);
try {
setState(313);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,21,_ctx) ) {
case 1:
_localctx = new IsTrustedContext(_localctx);
enterOuterAlt(_localctx, 1);
{
setState(291);
match(T__12);
setState(292);
match(LPAREN);
setState(293);
match(RPAREN);
}
break;
case 2:
_localctx = new IsTrustedTemplateNameContext(_localctx);
enterOuterAlt(_localctx, 2);
{
setState(294);
match(T__12);
setState(295);
match(LPAREN);
setState(296);
match(STRING);
setState(297);
match(RPAREN);
}
break;
case 3:
_localctx = new IsTrustedConditionsSingleListContext(_localctx);
enterOuterAlt(_localctx, 3);
{
setState(298);
match(T__12);
setState(299);
match(LPAREN);
setState(302);
_errHandler.sync(this);
switch (_input.LA(1)) {
case T__13:
{
setState(300);
payment_conditions();
}
break;
case T__14:
{
setState(301);
withdrawal_conditions();
}
break;
default:
throw new NoViableAltException(this);
}
setState(304);
match(RPAREN);
}
break;
case 4:
_localctx = new IsTrustedPaymentsAndWithdrawalConditionsContext(_localctx);
enterOuterAlt(_localctx, 4);
{
setState(306);
match(T__12);
setState(307);
match(LPAREN);
setState(308);
payment_conditions();
setState(309);
match(DELIMITER);
setState(310);
withdrawal_conditions();
setState(311);
match(RPAREN);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Payment_conditionsContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public Conditions_listContext conditions_list() {
return getRuleContext(Conditions_listContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Payment_conditionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_payment_conditions; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitPayment_conditions(this);
else return visitor.visitChildren(this);
}
}
public final Payment_conditionsContext payment_conditions() throws RecognitionException {
Payment_conditionsContext _localctx = new Payment_conditionsContext(_ctx, getState());
enterRule(_localctx, 44, RULE_payment_conditions);
try {
enterOuterAlt(_localctx, 1);
{
setState(315);
match(T__13);
setState(316);
match(LPAREN);
setState(317);
conditions_list();
setState(318);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Withdrawal_conditionsContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public Conditions_listContext conditions_list() {
return getRuleContext(Conditions_listContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Withdrawal_conditionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_withdrawal_conditions; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitWithdrawal_conditions(this);
else return visitor.visitChildren(this);
}
}
public final Withdrawal_conditionsContext withdrawal_conditions() throws RecognitionException {
Withdrawal_conditionsContext _localctx = new Withdrawal_conditionsContext(_ctx, getState());
enterRule(_localctx, 46, RULE_withdrawal_conditions);
try {
enterOuterAlt(_localctx, 1);
{
setState(320);
match(T__14);
setState(321);
match(LPAREN);
setState(322);
conditions_list();
setState(323);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Conditions_listContext extends ParserRuleContext {
public List trusted_token_condition() {
return getRuleContexts(Trusted_token_conditionContext.class);
}
public Trusted_token_conditionContext trusted_token_condition(int i) {
return getRuleContext(Trusted_token_conditionContext.class,i);
}
public List DELIMITER() { return getTokens(FraudoPaymentParser.DELIMITER); }
public TerminalNode DELIMITER(int i) {
return getToken(FraudoPaymentParser.DELIMITER, i);
}
public List WS() { return getTokens(FraudoPaymentParser.WS); }
public TerminalNode WS(int i) {
return getToken(FraudoPaymentParser.WS, i);
}
public Conditions_listContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_conditions_list; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitConditions_list(this);
else return visitor.visitChildren(this);
}
}
public final Conditions_listContext conditions_list() throws RecognitionException {
Conditions_listContext _localctx = new Conditions_listContext(_ctx, getState());
enterRule(_localctx, 48, RULE_conditions_list);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(325);
trusted_token_condition();
setState(331);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==DELIMITER || _la==WS) {
{
setState(329);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DELIMITER:
{
setState(326);
match(DELIMITER);
setState(327);
trusted_token_condition();
}
break;
case WS:
{
setState(328);
match(WS);
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(333);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Trusted_token_conditionContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public List DELIMITER() { return getTokens(FraudoPaymentParser.DELIMITER); }
public TerminalNode DELIMITER(int i) {
return getToken(FraudoPaymentParser.DELIMITER, i);
}
public List INTEGER() { return getTokens(FraudoPaymentParser.INTEGER); }
public TerminalNode INTEGER(int i) {
return getToken(FraudoPaymentParser.INTEGER, i);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Trusted_token_conditionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_trusted_token_condition; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitTrusted_token_condition(this);
else return visitor.visitChildren(this);
}
}
public final Trusted_token_conditionContext trusted_token_condition() throws RecognitionException {
Trusted_token_conditionContext _localctx = new Trusted_token_conditionContext(_ctx, getState());
enterRule(_localctx, 50, RULE_trusted_token_condition);
try {
setState(352);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,24,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(334);
match(T__15);
setState(335);
match(LPAREN);
setState(336);
match(STRING);
setState(337);
match(DELIMITER);
setState(338);
match(INTEGER);
setState(339);
match(DELIMITER);
setState(340);
match(INTEGER);
setState(341);
match(DELIMITER);
setState(342);
match(INTEGER);
setState(343);
match(RPAREN);
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(344);
match(T__15);
setState(345);
match(LPAREN);
setState(346);
match(STRING);
setState(347);
match(DELIMITER);
setState(348);
match(INTEGER);
setState(349);
match(DELIMITER);
setState(350);
match(INTEGER);
setState(351);
match(RPAREN);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class RandContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode INTEGER() { return getToken(FraudoPaymentParser.INTEGER, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public RandContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_rand; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitRand(this);
else return visitor.visitChildren(this);
}
}
public final RandContext rand() throws RecognitionException {
RandContext _localctx = new RandContext(_ctx, getState());
enterRule(_localctx, 52, RULE_rand);
try {
enterOuterAlt(_localctx, 1);
{
setState(354);
match(T__16);
setState(355);
match(LPAREN);
setState(356);
match(INTEGER);
setState(357);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Fraud_ruleContext extends ParserRuleContext {
public TerminalNode RULE_BLOCK() { return getToken(FraudoPaymentParser.RULE_BLOCK, 0); }
public ExpressionContext expression() {
return getRuleContext(ExpressionContext.class,0);
}
public TerminalNode RETURN() { return getToken(FraudoPaymentParser.RETURN, 0); }
public ResultContext result() {
return getRuleContext(ResultContext.class,0);
}
public TerminalNode SCOL() { return getToken(FraudoPaymentParser.SCOL, 0); }
public TerminalNode IDENTIFIER() { return getToken(FraudoPaymentParser.IDENTIFIER, 0); }
public TerminalNode CATCH_ERROR() { return getToken(FraudoPaymentParser.CATCH_ERROR, 0); }
public Catch_resultContext catch_result() {
return getRuleContext(Catch_resultContext.class,0);
}
public Fraud_ruleContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_fraud_rule; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitFraud_rule(this);
else return visitor.visitChildren(this);
}
}
public final Fraud_ruleContext fraud_rule() throws RecognitionException {
Fraud_ruleContext _localctx = new Fraud_ruleContext(_ctx, getState());
enterRule(_localctx, 54, RULE_fraud_rule);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(359);
match(RULE_BLOCK);
setState(362);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==IDENTIFIER) {
{
setState(360);
match(IDENTIFIER);
setState(361);
match(T__17);
}
}
setState(364);
expression();
setState(365);
match(RETURN);
setState(366);
result();
setState(369);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==CATCH_ERROR) {
{
setState(367);
match(CATCH_ERROR);
setState(368);
catch_result();
}
}
setState(371);
match(SCOL);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ComparatorContext extends ParserRuleContext {
public TerminalNode GT() { return getToken(FraudoPaymentParser.GT, 0); }
public TerminalNode GE() { return getToken(FraudoPaymentParser.GE, 0); }
public TerminalNode LT() { return getToken(FraudoPaymentParser.LT, 0); }
public TerminalNode LE() { return getToken(FraudoPaymentParser.LE, 0); }
public TerminalNode EQ() { return getToken(FraudoPaymentParser.EQ, 0); }
public ComparatorContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_comparator; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitComparator(this);
else return visitor.visitChildren(this);
}
}
public final ComparatorContext comparator() throws RecognitionException {
ComparatorContext _localctx = new ComparatorContext(_ctx, getState());
enterRule(_localctx, 56, RULE_comparator);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(373);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << GT) | (1L << GE) | (1L << LT) | (1L << LE) | (1L << EQ))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BinaryContext extends ParserRuleContext {
public TerminalNode AND() { return getToken(FraudoPaymentParser.AND, 0); }
public TerminalNode OR() { return getToken(FraudoPaymentParser.OR, 0); }
public BinaryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_binary; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitBinary(this);
else return visitor.visitChildren(this);
}
}
public final BinaryContext binary() throws RecognitionException {
BinaryContext _localctx = new BinaryContext(_ctx, getState());
enterRule(_localctx, 58, RULE_binary);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(375);
_la = _input.LA(1);
if ( !(_la==AND || _la==OR) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BoolContext extends ParserRuleContext {
public TerminalNode TRUE() { return getToken(FraudoPaymentParser.TRUE, 0); }
public TerminalNode FALSE() { return getToken(FraudoPaymentParser.FALSE, 0); }
public BoolContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_bool; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitBool(this);
else return visitor.visitChildren(this);
}
}
public final BoolContext bool() throws RecognitionException {
BoolContext _localctx = new BoolContext(_ctx, getState());
enterRule(_localctx, 60, RULE_bool);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(377);
_la = _input.LA(1);
if ( !(_la==TRUE || _la==FALSE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class AmountContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public AmountContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_amount; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitAmount(this);
else return visitor.visitChildren(this);
}
}
public final AmountContext amount() throws RecognitionException {
AmountContext _localctx = new AmountContext(_ctx, getState());
enterRule(_localctx, 62, RULE_amount);
try {
enterOuterAlt(_localctx, 1);
{
setState(379);
match(T__18);
setState(380);
match(LPAREN);
setState(381);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CurrencyContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public CurrencyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_currency; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCurrency(this);
else return visitor.visitChildren(this);
}
}
public final CurrencyContext currency() throws RecognitionException {
CurrencyContext _localctx = new CurrencyContext(_ctx, getState());
enterRule(_localctx, 64, RULE_currency);
try {
enterOuterAlt(_localctx, 1);
{
setState(383);
match(T__19);
setState(384);
match(LPAREN);
setState(385);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Payment_systemContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Payment_systemContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_payment_system; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitPayment_system(this);
else return visitor.visitChildren(this);
}
}
public final Payment_systemContext payment_system() throws RecognitionException {
Payment_systemContext _localctx = new Payment_systemContext(_ctx, getState());
enterRule(_localctx, 66, RULE_payment_system);
try {
enterOuterAlt(_localctx, 1);
{
setState(387);
match(T__20);
setState(388);
match(LPAREN);
setState(389);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Card_categoryContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Card_categoryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_card_category; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCard_category(this);
else return visitor.visitChildren(this);
}
}
public final Card_categoryContext card_category() throws RecognitionException {
Card_categoryContext _localctx = new Card_categoryContext(_ctx, getState());
enterRule(_localctx, 68, RULE_card_category);
try {
enterOuterAlt(_localctx, 1);
{
setState(391);
match(T__21);
setState(392);
match(LPAREN);
setState(393);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Payer_typeContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Payer_typeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_payer_type; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitPayer_type(this);
else return visitor.visitChildren(this);
}
}
public final Payer_typeContext payer_type() throws RecognitionException {
Payer_typeContext _localctx = new Payer_typeContext(_ctx, getState());
enterRule(_localctx, 70, RULE_payer_type);
try {
enterOuterAlt(_localctx, 1);
{
setState(395);
match(T__22);
setState(396);
match(LPAREN);
setState(397);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Token_providerContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Token_providerContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_token_provider; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitToken_provider(this);
else return visitor.visitChildren(this);
}
}
public final Token_providerContext token_provider() throws RecognitionException {
Token_providerContext _localctx = new Token_providerContext(_ctx, getState());
enterRule(_localctx, 72, RULE_token_provider);
try {
enterOuterAlt(_localctx, 1);
{
setState(399);
match(T__23);
setState(400);
match(LPAREN);
setState(401);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CountContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public CountContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_count; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCount(this);
else return visitor.visitChildren(this);
}
}
public final CountContext count() throws RecognitionException {
CountContext _localctx = new CountContext(_ctx, getState());
enterRule(_localctx, 74, RULE_count);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(403);
match(T__24);
setState(404);
match(LPAREN);
setState(405);
match(STRING);
setState(406);
time_window();
setState(408);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(407);
group_by();
}
}
setState(410);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class SumContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public SumContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_sum; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitSum(this);
else return visitor.visitChildren(this);
}
}
public final SumContext sum() throws RecognitionException {
SumContext _localctx = new SumContext(_ctx, getState());
enterRule(_localctx, 76, RULE_sum);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(412);
match(T__25);
setState(413);
match(LPAREN);
setState(414);
match(STRING);
setState(415);
time_window();
setState(417);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(416);
group_by();
}
}
setState(419);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class UniqueContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public List STRING() { return getTokens(FraudoPaymentParser.STRING); }
public TerminalNode STRING(int i) {
return getToken(FraudoPaymentParser.STRING, i);
}
public TerminalNode DELIMITER() { return getToken(FraudoPaymentParser.DELIMITER, 0); }
public Time_windowContext time_window() {
return getRuleContext(Time_windowContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Group_byContext group_by() {
return getRuleContext(Group_byContext.class,0);
}
public UniqueContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_unique; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitUnique(this);
else return visitor.visitChildren(this);
}
}
public final UniqueContext unique() throws RecognitionException {
UniqueContext _localctx = new UniqueContext(_ctx, getState());
enterRule(_localctx, 78, RULE_unique);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(421);
match(T__26);
setState(422);
match(LPAREN);
setState(423);
match(STRING);
setState(424);
match(DELIMITER);
setState(425);
match(STRING);
setState(426);
time_window();
setState(428);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(427);
group_by();
}
}
setState(430);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class In_white_listContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public String_listContext string_list() {
return getRuleContext(String_listContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public In_white_listContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_in_white_list; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIn_white_list(this);
else return visitor.visitChildren(this);
}
}
public final In_white_listContext in_white_list() throws RecognitionException {
In_white_listContext _localctx = new In_white_listContext(_ctx, getState());
enterRule(_localctx, 80, RULE_in_white_list);
try {
enterOuterAlt(_localctx, 1);
{
setState(432);
match(T__27);
setState(433);
match(LPAREN);
setState(434);
string_list();
setState(435);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class In_black_listContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public String_listContext string_list() {
return getRuleContext(String_listContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public In_black_listContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_in_black_list; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIn_black_list(this);
else return visitor.visitChildren(this);
}
}
public final In_black_listContext in_black_list() throws RecognitionException {
In_black_listContext _localctx = new In_black_listContext(_ctx, getState());
enterRule(_localctx, 82, RULE_in_black_list);
try {
enterOuterAlt(_localctx, 1);
{
setState(437);
match(T__28);
setState(438);
match(LPAREN);
setState(439);
string_list();
setState(440);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class In_grey_listContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public String_listContext string_list() {
return getRuleContext(String_listContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public In_grey_listContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_in_grey_list; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIn_grey_list(this);
else return visitor.visitChildren(this);
}
}
public final In_grey_listContext in_grey_list() throws RecognitionException {
In_grey_listContext _localctx = new In_grey_listContext(_ctx, getState());
enterRule(_localctx, 84, RULE_in_grey_list);
try {
enterOuterAlt(_localctx, 1);
{
setState(442);
match(T__29);
setState(443);
match(LPAREN);
setState(444);
string_list();
setState(445);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class In_listContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public TerminalNode DELIMITER() { return getToken(FraudoPaymentParser.DELIMITER, 0); }
public String_listContext string_list() {
return getRuleContext(String_listContext.class,0);
}
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public In_listContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_in_list; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitIn_list(this);
else return visitor.visitChildren(this);
}
}
public final In_listContext in_list() throws RecognitionException {
In_listContext _localctx = new In_listContext(_ctx, getState());
enterRule(_localctx, 86, RULE_in_list);
try {
enterOuterAlt(_localctx, 1);
{
setState(447);
match(T__30);
setState(448);
match(LPAREN);
setState(449);
match(STRING);
setState(450);
match(DELIMITER);
setState(451);
string_list();
setState(452);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class LikeContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public List STRING() { return getTokens(FraudoPaymentParser.STRING); }
public TerminalNode STRING(int i) {
return getToken(FraudoPaymentParser.STRING, i);
}
public TerminalNode DELIMITER() { return getToken(FraudoPaymentParser.DELIMITER, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public LikeContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_like; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitLike(this);
else return visitor.visitChildren(this);
}
}
public final LikeContext like() throws RecognitionException {
LikeContext _localctx = new LikeContext(_ctx, getState());
enterRule(_localctx, 88, RULE_like);
try {
enterOuterAlt(_localctx, 1);
{
setState(454);
match(T__31);
setState(455);
match(LPAREN);
setState(456);
match(STRING);
setState(457);
match(DELIMITER);
setState(458);
match(STRING);
setState(459);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Country_byContext extends ParserRuleContext {
public TerminalNode LPAREN() { return getToken(FraudoPaymentParser.LPAREN, 0); }
public TerminalNode STRING() { return getToken(FraudoPaymentParser.STRING, 0); }
public TerminalNode RPAREN() { return getToken(FraudoPaymentParser.RPAREN, 0); }
public Country_byContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_country_by; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCountry_by(this);
else return visitor.visitChildren(this);
}
}
public final Country_byContext country_by() throws RecognitionException {
Country_byContext _localctx = new Country_byContext(_ctx, getState());
enterRule(_localctx, 90, RULE_country_by);
try {
enterOuterAlt(_localctx, 1);
{
setState(461);
match(T__32);
setState(462);
match(LPAREN);
setState(463);
match(STRING);
setState(464);
match(RPAREN);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ResultContext extends ParserRuleContext {
public ResultContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_result; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitResult(this);
else return visitor.visitChildren(this);
}
}
public final ResultContext result() throws RecognitionException {
ResultContext _localctx = new ResultContext(_ctx, getState());
enterRule(_localctx, 92, RULE_result);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(466);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__33) | (1L << T__34) | (1L << T__35) | (1L << T__36) | (1L << T__37) | (1L << T__38) | (1L << T__39))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Catch_resultContext extends ParserRuleContext {
public Catch_resultContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_catch_result; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitCatch_result(this);
else return visitor.visitChildren(this);
}
}
public final Catch_resultContext catch_result() throws RecognitionException {
Catch_resultContext _localctx = new Catch_resultContext(_ctx, getState());
enterRule(_localctx, 94, RULE_catch_result);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(468);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__33) | (1L << T__34) | (1L << T__35) | (1L << T__37))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class String_listContext extends ParserRuleContext {
public List STRING() { return getTokens(FraudoPaymentParser.STRING); }
public TerminalNode STRING(int i) {
return getToken(FraudoPaymentParser.STRING, i);
}
public List DELIMITER() { return getTokens(FraudoPaymentParser.DELIMITER); }
public TerminalNode DELIMITER(int i) {
return getToken(FraudoPaymentParser.DELIMITER, i);
}
public List WS() { return getTokens(FraudoPaymentParser.WS); }
public TerminalNode WS(int i) {
return getToken(FraudoPaymentParser.WS, i);
}
public String_listContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_string_list; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitString_list(this);
else return visitor.visitChildren(this);
}
}
public final String_listContext string_list() throws RecognitionException {
String_listContext _localctx = new String_listContext(_ctx, getState());
enterRule(_localctx, 96, RULE_string_list);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(470);
match(STRING);
setState(476);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==DELIMITER || _la==WS) {
{
setState(474);
_errHandler.sync(this);
switch (_input.LA(1)) {
case DELIMITER:
{
setState(471);
match(DELIMITER);
setState(472);
match(STRING);
}
break;
case WS:
{
setState(473);
match(WS);
}
break;
default:
throw new NoViableAltException(this);
}
}
setState(478);
_errHandler.sync(this);
_la = _input.LA(1);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Time_windowContext extends ParserRuleContext {
public List DELIMITER() { return getTokens(FraudoPaymentParser.DELIMITER); }
public TerminalNode DELIMITER(int i) {
return getToken(FraudoPaymentParser.DELIMITER, i);
}
public List INTEGER() { return getTokens(FraudoPaymentParser.INTEGER); }
public TerminalNode INTEGER(int i) {
return getToken(FraudoPaymentParser.INTEGER, i);
}
public Time_unitContext time_unit() {
return getRuleContext(Time_unitContext.class,0);
}
public Time_windowContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_time_window; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitTime_window(this);
else return visitor.visitChildren(this);
}
}
public final Time_windowContext time_window() throws RecognitionException {
Time_windowContext _localctx = new Time_windowContext(_ctx, getState());
enterRule(_localctx, 98, RULE_time_window);
try {
setState(495);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,32,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(479);
match(DELIMITER);
setState(480);
match(INTEGER);
setState(481);
match(DELIMITER);
setState(482);
match(INTEGER);
setState(483);
match(DELIMITER);
setState(484);
time_unit();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(485);
match(DELIMITER);
setState(486);
match(INTEGER);
setState(487);
match(DELIMITER);
setState(488);
time_unit();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(489);
match(DELIMITER);
setState(490);
match(INTEGER);
setState(491);
match(DELIMITER);
setState(492);
match(INTEGER);
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(493);
match(DELIMITER);
setState(494);
match(INTEGER);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Time_unitContext extends ParserRuleContext {
public Time_unitContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_time_unit; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitTime_unit(this);
else return visitor.visitChildren(this);
}
}
public final Time_unitContext time_unit() throws RecognitionException {
Time_unitContext _localctx = new Time_unitContext(_ctx, getState());
enterRule(_localctx, 100, RULE_time_unit);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(497);
_la = _input.LA(1);
if ( !((((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << T__40) | (1L << T__41) | (1L << T__42) | (1L << T__43) | (1L << T__44))) != 0)) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Group_byContext extends ParserRuleContext {
public TerminalNode DELIMITER() { return getToken(FraudoPaymentParser.DELIMITER, 0); }
public String_listContext string_list() {
return getRuleContext(String_listContext.class,0);
}
public Group_byContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_group_by; }
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof FraudoPaymentVisitor ) return ((FraudoPaymentVisitor extends T>)visitor).visitGroup_by(this);
else return visitor.visitChildren(this);
}
}
public final Group_byContext group_by() throws RecognitionException {
Group_byContext _localctx = new Group_byContext(_ctx, getState());
enterRule(_localctx, 102, RULE_group_by);
try {
enterOuterAlt(_localctx, 1);
{
setState(499);
match(DELIMITER);
setState(500);
string_list();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3H\u01f9\4\2\t\2\4"+
"\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13\t"+
"\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\4\22\t\22"+
"\4\23\t\23\4\24\t\24\4\25\t\25\4\26\t\26\4\27\t\27\4\30\t\30\4\31\t\31"+
"\4\32\t\32\4\33\t\33\4\34\t\34\4\35\t\35\4\36\t\36\4\37\t\37\4 \t \4!"+
"\t!\4\"\t\"\4#\t#\4$\t$\4%\t%\4&\t&\4\'\t\'\4(\t(\4)\t)\4*\t*\4+\t+\4"+
",\t,\4-\t-\4.\t.\4/\t/\4\60\t\60\4\61\t\61\4\62\t\62\4\63\t\63\4\64\t"+
"\64\4\65\t\65\3\2\7\2l\n\2\f\2\16\2o\13\2\3\2\3\2\3\3\3\3\3\3\7\3v\n\3"+
"\f\3\16\3y\13\3\3\4\3\4\3\4\7\4~\n\4\f\4\16\4\u0081\13\4\3\5\3\5\3\5\3"+
"\5\3\5\3\5\3\5\3\5\3\5\3\5\3\5\5\5\u008e\n\5\3\6\3\6\3\6\3\6\3\6\5\6\u0095"+
"\n\6\3\7\3\7\3\7\3\7\3\7\3\7\3\7\3\7\3\7\3\7\3\7\3\7\3\7\5\7\u00a4\n\7"+
"\3\b\3\b\5\b\u00a8\n\b\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\3\t\5\t\u00b3\n"+
"\t\3\n\3\n\3\n\3\n\3\n\3\n\3\n\5\n\u00bc\n\n\3\13\3\13\3\13\3\13\3\13"+
"\5\13\u00c3\n\13\3\13\3\13\3\f\3\f\3\f\3\f\3\f\5\f\u00cc\n\f\3\f\3\f\3"+
"\r\3\r\3\r\3\r\3\r\3\r\5\r\u00d6\n\r\3\r\5\r\u00d9\n\r\3\r\3\r\3\16\3"+
"\16\3\16\3\16\3\16\5\16\u00e2\n\16\3\16\3\16\3\17\3\17\3\17\3\17\3\17"+
"\5\17\u00eb\n\17\3\17\3\17\3\20\3\20\3\20\3\20\3\20\5\20\u00f4\n\20\3"+
"\20\3\20\3\21\3\21\3\21\3\21\3\21\3\21\5\21\u00fe\n\21\3\21\5\21\u0101"+
"\n\21\3\21\3\21\3\22\3\22\3\22\3\22\3\22\5\22\u010a\n\22\3\22\3\22\3\23"+
"\3\23\3\23\3\23\3\23\5\23\u0113\n\23\3\23\3\23\3\24\3\24\3\24\3\24\3\24"+
"\3\24\3\24\3\25\3\25\3\25\3\25\3\26\3\26\3\26\3\26\3\27\3\27\3\27\3\27"+
"\3\27\3\27\3\27\3\27\3\27\3\27\3\27\5\27\u0131\n\27\3\27\3\27\3\27\3\27"+
"\3\27\3\27\3\27\3\27\3\27\5\27\u013c\n\27\3\30\3\30\3\30\3\30\3\30\3\31"+
"\3\31\3\31\3\31\3\31\3\32\3\32\3\32\3\32\7\32\u014c\n\32\f\32\16\32\u014f"+
"\13\32\3\33\3\33\3\33\3\33\3\33\3\33\3\33\3\33\3\33\3\33\3\33\3\33\3\33"+
"\3\33\3\33\3\33\3\33\3\33\5\33\u0163\n\33\3\34\3\34\3\34\3\34\3\34\3\35"+
"\3\35\3\35\5\35\u016d\n\35\3\35\3\35\3\35\3\35\3\35\5\35\u0174\n\35\3"+
"\35\3\35\3\36\3\36\3\37\3\37\3 \3 \3!\3!\3!\3!\3\"\3\"\3\"\3\"\3#\3#\3"+
"#\3#\3$\3$\3$\3$\3%\3%\3%\3%\3&\3&\3&\3&\3\'\3\'\3\'\3\'\3\'\5\'\u019b"+
"\n\'\3\'\3\'\3(\3(\3(\3(\3(\5(\u01a4\n(\3(\3(\3)\3)\3)\3)\3)\3)\3)\5)"+
"\u01af\n)\3)\3)\3*\3*\3*\3*\3*\3+\3+\3+\3+\3+\3,\3,\3,\3,\3,\3-\3-\3-"+
"\3-\3-\3-\3-\3.\3.\3.\3.\3.\3.\3.\3/\3/\3/\3/\3/\3\60\3\60\3\61\3\61\3"+
"\62\3\62\3\62\3\62\7\62\u01dd\n\62\f\62\16\62\u01e0\13\62\3\63\3\63\3"+
"\63\3\63\3\63\3\63\3\63\3\63\3\63\3\63\3\63\3\63\3\63\3\63\3\63\3\63\5"+
"\63\u01f2\n\63\3\64\3\64\3\65\3\65\3\65\3\65\2\2\66\2\4\6\b\n\f\16\20"+
"\22\24\26\30\32\34\36 \"$&(*,.\60\62\64\668:<>@BDFHJLNPRTVXZ\\^`bdfh\2"+
"\n\3\2?@\3\2;@\3\2;?\3\2\66\67\3\29:\3\2$*\4\2$&((\3\2+/\2\u0202\2m\3"+
"\2\2\2\4r\3\2\2\2\6z\3\2\2\2\b\u008d\3\2\2\2\n\u0094\3\2\2\2\f\u00a3\3"+
"\2\2\2\16\u00a7\3\2\2\2\20\u00b2\3\2\2\2\22\u00bb\3\2\2\2\24\u00bd\3\2"+
"\2\2\26\u00c6\3\2\2\2\30\u00cf\3\2\2\2\32\u00dc\3\2\2\2\34\u00e5\3\2\2"+
"\2\36\u00ee\3\2\2\2 \u00f7\3\2\2\2\"\u0104\3\2\2\2$\u010d\3\2\2\2&\u0116"+
"\3\2\2\2(\u011d\3\2\2\2*\u0121\3\2\2\2,\u013b\3\2\2\2.\u013d\3\2\2\2\60"+
"\u0142\3\2\2\2\62\u0147\3\2\2\2\64\u0162\3\2\2\2\66\u0164\3\2\2\28\u0169"+
"\3\2\2\2:\u0177\3\2\2\2<\u0179\3\2\2\2>\u017b\3\2\2\2@\u017d\3\2\2\2B"+
"\u0181\3\2\2\2D\u0185\3\2\2\2F\u0189\3\2\2\2H\u018d\3\2\2\2J\u0191\3\2"+
"\2\2L\u0195\3\2\2\2N\u019e\3\2\2\2P\u01a7\3\2\2\2R\u01b2\3\2\2\2T\u01b7"+
"\3\2\2\2V\u01bc\3\2\2\2X\u01c1\3\2\2\2Z\u01c8\3\2\2\2\\\u01cf\3\2\2\2"+
"^\u01d4\3\2\2\2`\u01d6\3\2\2\2b\u01d8\3\2\2\2d\u01f1\3\2\2\2f\u01f3\3"+
"\2\2\2h\u01f5\3\2\2\2jl\58\35\2kj\3\2\2\2lo\3\2\2\2mk\3\2\2\2mn\3\2\2"+
"\2np\3\2\2\2om\3\2\2\2pq\7\2\2\3q\3\3\2\2\2rw\5\6\4\2st\7\67\2\2tv\5\6"+
"\4\2us\3\2\2\2vy\3\2\2\2wu\3\2\2\2wx\3\2\2\2x\5\3\2\2\2yw\3\2\2\2z\177"+
"\5\b\5\2{|\7\66\2\2|~\5\b\5\2}{\3\2\2\2~\u0081\3\2\2\2\177}\3\2\2\2\177"+
"\u0080\3\2\2\2\u0080\7\3\2\2\2\u0081\177\3\2\2\2\u0082\u008e\5\f\7\2\u0083"+
"\u0084\5\n\6\2\u0084\u0085\t\2\2\2\u0085\u0086\5\n\6\2\u0086\u008e\3\2"+
"\2\2\u0087\u0088\78\2\2\u0088\u008e\5\4\3\2\u0089\u008a\7A\2\2\u008a\u008b"+
"\5\4\3\2\u008b\u008c\7B\2\2\u008c\u008e\3\2\2\2\u008d\u0082\3\2\2\2\u008d"+
"\u0083\3\2\2\2\u008d\u0087\3\2\2\2\u008d\u0089\3\2\2\2\u008e\t\3\2\2\2"+
"\u008f\u0095\5\\/\2\u0090\u0095\5B\"\2\u0091\u0095\5D#\2\u0092\u0095\5"+
"F$\2\u0093\u0095\7\60\2\2\u0094\u008f\3\2\2\2\u0094\u0090\3\2\2\2\u0094"+
"\u0091\3\2\2\2\u0094\u0092\3\2\2\2\u0094\u0093\3\2\2\2\u0095\13\3\2\2"+
"\2\u0096\u0097\5\16\b\2\u0097\u0098\t\3\2\2\u0098\u0099\5\16\b\2\u0099"+
"\u00a4\3\2\2\2\u009a\u00a4\5&\24\2\u009b\u00a4\5R*\2\u009c\u00a4\5T+\2"+
"\u009d\u00a4\5V,\2\u009e\u00a4\5X-\2\u009f\u00a4\5Z.\2\u00a0\u00a4\5("+
"\25\2\u00a1\u00a4\5*\26\2\u00a2\u00a4\5,\27\2\u00a3\u0096\3\2\2\2\u00a3"+
"\u009a\3\2\2\2\u00a3\u009b\3\2\2\2\u00a3\u009c\3\2\2\2\u00a3\u009d\3\2"+
"\2\2\u00a3\u009e\3\2\2\2\u00a3\u009f\3\2\2\2\u00a3\u00a0\3\2\2\2\u00a3"+
"\u00a1\3\2\2\2\u00a3\u00a2\3\2\2\2\u00a4\r\3\2\2\2\u00a5\u00a8\5\20\t"+
"\2\u00a6\u00a8\5\22\n\2\u00a7\u00a5\3\2\2\2\u00a7\u00a6\3\2\2\2\u00a8"+
"\17\3\2\2\2\u00a9\u00b3\5L\'\2\u00aa\u00b3\5\26\f\2\u00ab\u00b3\5\24\13"+
"\2\u00ac\u00b3\5\30\r\2\u00ad\u00b3\5\32\16\2\u00ae\u00b3\5\34\17\2\u00af"+
"\u00b3\5P)\2\u00b0\u00b3\5\66\34\2\u00b1\u00b3\7D\2\2\u00b2\u00a9\3\2"+
"\2\2\u00b2\u00aa\3\2\2\2\u00b2\u00ab\3\2\2\2\u00b2\u00ac\3\2\2\2\u00b2"+
"\u00ad\3\2\2\2\u00b2\u00ae\3\2\2\2\u00b2\u00af\3\2\2\2\u00b2\u00b0\3\2"+
"\2\2\u00b2\u00b1\3\2\2\2\u00b3\21\3\2\2\2\u00b4\u00bc\5N(\2\u00b5\u00bc"+
"\5\36\20\2\u00b6\u00bc\5 \21\2\u00b7\u00bc\5\"\22\2\u00b8\u00bc\5$\23"+
"\2\u00b9\u00bc\5@!\2\u00ba\u00bc\7C\2\2\u00bb\u00b4\3\2\2\2\u00bb\u00b5"+
"\3\2\2\2\u00bb\u00b6\3\2\2\2\u00bb\u00b7\3\2\2\2\u00bb\u00b8\3\2\2\2\u00bb"+
"\u00b9\3\2\2\2\u00bb\u00ba\3\2\2\2\u00bc\23\3\2\2\2\u00bd\u00be\7\3\2"+
"\2\u00be\u00bf\7A\2\2\u00bf\u00c0\7\60\2\2\u00c0\u00c2\5d\63\2\u00c1\u00c3"+
"\5h\65\2\u00c2\u00c1\3\2\2\2\u00c2\u00c3\3\2\2\2\u00c3\u00c4\3\2\2\2\u00c4"+
"\u00c5\7B\2\2\u00c5\25\3\2\2\2\u00c6\u00c7\7\4\2\2\u00c7\u00c8\7A\2\2"+
"\u00c8\u00c9\7\60\2\2\u00c9\u00cb\5d\63\2\u00ca\u00cc\5h\65\2\u00cb\u00ca"+
"\3\2\2\2\u00cb\u00cc\3\2\2\2\u00cc\u00cd\3\2\2\2\u00cd\u00ce\7B\2\2\u00ce"+
"\27\3\2\2\2\u00cf\u00d0\7\5\2\2\u00d0\u00d1\7A\2\2\u00d1\u00d2\7\60\2"+
"\2\u00d2\u00d5\5d\63\2\u00d3\u00d4\7\61\2\2\u00d4\u00d6\7\60\2\2\u00d5"+
"\u00d3\3\2\2\2\u00d5\u00d6\3\2\2\2\u00d6\u00d8\3\2\2\2\u00d7\u00d9\5h"+
"\65\2\u00d8\u00d7\3\2\2\2\u00d8\u00d9\3\2\2\2\u00d9\u00da\3\2\2\2\u00da"+
"\u00db\7B\2\2\u00db\31\3\2\2\2\u00dc\u00dd\7\6\2\2\u00dd\u00de\7A\2\2"+
"\u00de\u00df\7\60\2\2\u00df\u00e1\5d\63\2\u00e0\u00e2\5h\65\2\u00e1\u00e0"+
"\3\2\2\2\u00e1\u00e2\3\2\2\2\u00e2\u00e3\3\2\2\2\u00e3\u00e4\7B\2\2\u00e4"+
"\33\3\2\2\2\u00e5\u00e6\7\7\2\2\u00e6\u00e7\7A\2\2\u00e7\u00e8\7\60\2"+
"\2\u00e8\u00ea\5d\63\2\u00e9\u00eb\5h\65\2\u00ea\u00e9\3\2\2\2\u00ea\u00eb"+
"\3\2\2\2\u00eb\u00ec\3\2\2\2\u00ec\u00ed\7B\2\2\u00ed\35\3\2\2\2\u00ee"+
"\u00ef\7\b\2\2\u00ef\u00f0\7A\2\2\u00f0\u00f1\7\60\2\2\u00f1\u00f3\5d"+
"\63\2\u00f2\u00f4\5h\65\2\u00f3\u00f2\3\2\2\2\u00f3\u00f4\3\2\2\2\u00f4"+
"\u00f5\3\2\2\2\u00f5\u00f6\7B\2\2\u00f6\37\3\2\2\2\u00f7\u00f8\7\t\2\2"+
"\u00f8\u00f9\7A\2\2\u00f9\u00fa\7\60\2\2\u00fa\u00fd\5d\63\2\u00fb\u00fc"+
"\7\61\2\2\u00fc\u00fe\7\60\2\2\u00fd\u00fb\3\2\2\2\u00fd\u00fe\3\2\2\2"+
"\u00fe\u0100\3\2\2\2\u00ff\u0101\5h\65\2\u0100\u00ff\3\2\2\2\u0100\u0101"+
"\3\2\2\2\u0101\u0102\3\2\2\2\u0102\u0103\7B\2\2\u0103!\3\2\2\2\u0104\u0105"+
"\7\n\2\2\u0105\u0106\7A\2\2\u0106\u0107\7\60\2\2\u0107\u0109\5d\63\2\u0108"+
"\u010a\5h\65\2\u0109\u0108\3\2\2\2\u0109\u010a\3\2\2\2\u010a\u010b\3\2"+
"\2\2\u010b\u010c\7B\2\2\u010c#\3\2\2\2\u010d\u010e\7\13\2\2\u010e\u010f"+
"\7A\2\2\u010f\u0110\7\60\2\2\u0110\u0112\5d\63\2\u0111\u0113\5h\65\2\u0112"+
"\u0111\3\2\2\2\u0112\u0113\3\2\2\2\u0113\u0114\3\2\2\2\u0114\u0115\7B"+
"\2\2\u0115%\3\2\2\2\u0116\u0117\7\f\2\2\u0117\u0118\7A\2\2\u0118\u0119"+
"\5\n\6\2\u0119\u011a\7\61\2\2\u011a\u011b\5b\62\2\u011b\u011c\7B\2\2\u011c"+
"\'\3\2\2\2\u011d\u011e\7\r\2\2\u011e\u011f\7A\2\2\u011f\u0120\7B\2\2\u0120"+
")\3\2\2\2\u0121\u0122\7\16\2\2\u0122\u0123\7A\2\2\u0123\u0124\7B\2\2\u0124"+
"+\3\2\2\2\u0125\u0126\7\17\2\2\u0126\u0127\7A\2\2\u0127\u013c\7B\2\2\u0128"+
"\u0129\7\17\2\2\u0129\u012a\7A\2\2\u012a\u012b\7\60\2\2\u012b\u013c\7"+
"B\2\2\u012c\u012d\7\17\2\2\u012d\u0130\7A\2\2\u012e\u0131\5.\30\2\u012f"+
"\u0131\5\60\31\2\u0130\u012e\3\2\2\2\u0130\u012f\3\2\2\2\u0131\u0132\3"+
"\2\2\2\u0132\u0133\7B\2\2\u0133\u013c\3\2\2\2\u0134\u0135\7\17\2\2\u0135"+
"\u0136\7A\2\2\u0136\u0137\5.\30\2\u0137\u0138\7\61\2\2\u0138\u0139\5\60"+
"\31\2\u0139\u013a\7B\2\2\u013a\u013c\3\2\2\2\u013b\u0125\3\2\2\2\u013b"+
"\u0128\3\2\2\2\u013b\u012c\3\2\2\2\u013b\u0134\3\2\2\2\u013c-\3\2\2\2"+
"\u013d\u013e\7\20\2\2\u013e\u013f\7A\2\2\u013f\u0140\5\62\32\2\u0140\u0141"+
"\7B\2\2\u0141/\3\2\2\2\u0142\u0143\7\21\2\2\u0143\u0144\7A\2\2\u0144\u0145"+
"\5\62\32\2\u0145\u0146\7B\2\2\u0146\61\3\2\2\2\u0147\u014d\5\64\33\2\u0148"+
"\u0149\7\61\2\2\u0149\u014c\5\64\33\2\u014a\u014c\7F\2\2\u014b\u0148\3"+
"\2\2\2\u014b\u014a\3\2\2\2\u014c\u014f\3\2\2\2\u014d\u014b\3\2\2\2\u014d"+
"\u014e\3\2\2\2\u014e\63\3\2\2\2\u014f\u014d\3\2\2\2\u0150\u0151\7\22\2"+
"\2\u0151\u0152\7A\2\2\u0152\u0153\7\60\2\2\u0153\u0154\7\61\2\2\u0154"+
"\u0155\7D\2\2\u0155\u0156\7\61\2\2\u0156\u0157\7D\2\2\u0157\u0158\7\61"+
"\2\2\u0158\u0159\7D\2\2\u0159\u0163\7B\2\2\u015a\u015b\7\22\2\2\u015b"+
"\u015c\7A\2\2\u015c\u015d\7\60\2\2\u015d\u015e\7\61\2\2\u015e\u015f\7"+
"D\2\2\u015f\u0160\7\61\2\2\u0160\u0161\7D\2\2\u0161\u0163\7B\2\2\u0162"+
"\u0150\3\2\2\2\u0162\u015a\3\2\2\2\u0163\65\3\2\2\2\u0164\u0165\7\23\2"+
"\2\u0165\u0166\7A\2\2\u0166\u0167\7D\2\2\u0167\u0168\7B\2\2\u0168\67\3"+
"\2\2\2\u0169\u016c\7\65\2\2\u016a\u016b\7E\2\2\u016b\u016d\7\24\2\2\u016c"+
"\u016a\3\2\2\2\u016c\u016d\3\2\2\2\u016d\u016e\3\2\2\2\u016e\u016f\5\4"+
"\3\2\u016f\u0170\7\63\2\2\u0170\u0173\5^\60\2\u0171\u0172\7\64\2\2\u0172"+
"\u0174\5`\61\2\u0173\u0171\3\2\2\2\u0173\u0174\3\2\2\2\u0174\u0175\3\2"+
"\2\2\u0175\u0176\7G\2\2\u01769\3\2\2\2\u0177\u0178\t\4\2\2\u0178;\3\2"+
"\2\2\u0179\u017a\t\5\2\2\u017a=\3\2\2\2\u017b\u017c\t\6\2\2\u017c?\3\2"+
"\2\2\u017d\u017e\7\25\2\2\u017e\u017f\7A\2\2\u017f\u0180\7B\2\2\u0180"+
"A\3\2\2\2\u0181\u0182\7\26\2\2\u0182\u0183\7A\2\2\u0183\u0184\7B\2\2\u0184"+
"C\3\2\2\2\u0185\u0186\7\27\2\2\u0186\u0187\7A\2\2\u0187\u0188\7B\2\2\u0188"+
"E\3\2\2\2\u0189\u018a\7\30\2\2\u018a\u018b\7A\2\2\u018b\u018c\7B\2\2\u018c"+
"G\3\2\2\2\u018d\u018e\7\31\2\2\u018e\u018f\7A\2\2\u018f\u0190\7B\2\2\u0190"+
"I\3\2\2\2\u0191\u0192\7\32\2\2\u0192\u0193\7A\2\2\u0193\u0194\7B\2\2\u0194"+
"K\3\2\2\2\u0195\u0196\7\33\2\2\u0196\u0197\7A\2\2\u0197\u0198\7\60\2\2"+
"\u0198\u019a\5d\63\2\u0199\u019b\5h\65\2\u019a\u0199\3\2\2\2\u019a\u019b"+
"\3\2\2\2\u019b\u019c\3\2\2\2\u019c\u019d\7B\2\2\u019dM\3\2\2\2\u019e\u019f"+
"\7\34\2\2\u019f\u01a0\7A\2\2\u01a0\u01a1\7\60\2\2\u01a1\u01a3\5d\63\2"+
"\u01a2\u01a4\5h\65\2\u01a3\u01a2\3\2\2\2\u01a3\u01a4\3\2\2\2\u01a4\u01a5"+
"\3\2\2\2\u01a5\u01a6\7B\2\2\u01a6O\3\2\2\2\u01a7\u01a8\7\35\2\2\u01a8"+
"\u01a9\7A\2\2\u01a9\u01aa\7\60\2\2\u01aa\u01ab\7\61\2\2\u01ab\u01ac\7"+
"\60\2\2\u01ac\u01ae\5d\63\2\u01ad\u01af\5h\65\2\u01ae\u01ad\3\2\2\2\u01ae"+
"\u01af\3\2\2\2\u01af\u01b0\3\2\2\2\u01b0\u01b1\7B\2\2\u01b1Q\3\2\2\2\u01b2"+
"\u01b3\7\36\2\2\u01b3\u01b4\7A\2\2\u01b4\u01b5\5b\62\2\u01b5\u01b6\7B"+
"\2\2\u01b6S\3\2\2\2\u01b7\u01b8\7\37\2\2\u01b8\u01b9\7A\2\2\u01b9\u01ba"+
"\5b\62\2\u01ba\u01bb\7B\2\2\u01bbU\3\2\2\2\u01bc\u01bd\7 \2\2\u01bd\u01be"+
"\7A\2\2\u01be\u01bf\5b\62\2\u01bf\u01c0\7B\2\2\u01c0W\3\2\2\2\u01c1\u01c2"+
"\7!\2\2\u01c2\u01c3\7A\2\2\u01c3\u01c4\7\60\2\2\u01c4\u01c5\7\61\2\2\u01c5"+
"\u01c6\5b\62\2\u01c6\u01c7\7B\2\2\u01c7Y\3\2\2\2\u01c8\u01c9\7\"\2\2\u01c9"+
"\u01ca\7A\2\2\u01ca\u01cb\7\60\2\2\u01cb\u01cc\7\61\2\2\u01cc\u01cd\7"+
"\60\2\2\u01cd\u01ce\7B\2\2\u01ce[\3\2\2\2\u01cf\u01d0\7#\2\2\u01d0\u01d1"+
"\7A\2\2\u01d1\u01d2\7\60\2\2\u01d2\u01d3\7B\2\2\u01d3]\3\2\2\2\u01d4\u01d5"+
"\t\7\2\2\u01d5_\3\2\2\2\u01d6\u01d7\t\b\2\2\u01d7a\3\2\2\2\u01d8\u01de"+
"\7\60\2\2\u01d9\u01da\7\61\2\2\u01da\u01dd\7\60\2\2\u01db\u01dd\7F\2\2"+
"\u01dc\u01d9\3\2\2\2\u01dc\u01db\3\2\2\2\u01dd\u01e0\3\2\2\2\u01de\u01dc"+
"\3\2\2\2\u01de\u01df\3\2\2\2\u01dfc\3\2\2\2\u01e0\u01de\3\2\2\2\u01e1"+
"\u01e2\7\61\2\2\u01e2\u01e3\7D\2\2\u01e3\u01e4\7\61\2\2\u01e4\u01e5\7"+
"D\2\2\u01e5\u01e6\7\61\2\2\u01e6\u01f2\5f\64\2\u01e7\u01e8\7\61\2\2\u01e8"+
"\u01e9\7D\2\2\u01e9\u01ea\7\61\2\2\u01ea\u01f2\5f\64\2\u01eb\u01ec\7\61"+
"\2\2\u01ec\u01ed\7D\2\2\u01ed\u01ee\7\61\2\2\u01ee\u01f2\7D\2\2\u01ef"+
"\u01f0\7\61\2\2\u01f0\u01f2\7D\2\2\u01f1\u01e1\3\2\2\2\u01f1\u01e7\3\2"+
"\2\2\u01f1\u01eb\3\2\2\2\u01f1\u01ef\3\2\2\2\u01f2e\3\2\2\2\u01f3\u01f4"+
"\t\t\2\2\u01f4g\3\2\2\2\u01f5\u01f6\7\61\2\2\u01f6\u01f7\5b\62\2\u01f7"+
"i\3\2\2\2#mw\177\u008d\u0094\u00a3\u00a7\u00b2\u00bb\u00c2\u00cb\u00d5"+
"\u00d8\u00e1\u00ea\u00f3\u00fd\u0100\u0109\u0112\u0130\u013b\u014b\u014d"+
"\u0162\u016c\u0173\u019a\u01a3\u01ae\u01dc\u01de\u01f1";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy