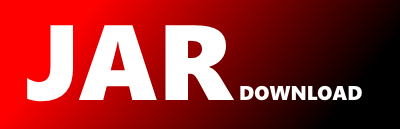
dev.vality.fraudo.FraudoPaymentVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fraudo Show documentation
Show all versions of fraudo Show documentation
Language for describing antifraud patterns
// Generated from dev.vality.fraudo/FraudoPayment.g4 by ANTLR 4.7.1
package dev.vality.fraudo;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link FraudoPaymentParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface FraudoPaymentVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#parse}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParse(FraudoPaymentParser.ParseContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpression(FraudoPaymentParser.ExpressionContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#booleanAndExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBooleanAndExpression(FraudoPaymentParser.BooleanAndExpressionContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#equalityExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEqualityExpression(FraudoPaymentParser.EqualityExpressionContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#stringExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStringExpression(FraudoPaymentParser.StringExpressionContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#relationalExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRelationalExpression(FraudoPaymentParser.RelationalExpressionContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#unaryExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnaryExpression(FraudoPaymentParser.UnaryExpressionContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#integerExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIntegerExpression(FraudoPaymentParser.IntegerExpressionContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#floatExpression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFloatExpression(FraudoPaymentParser.FloatExpressionContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#count_success}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCount_success(FraudoPaymentParser.Count_successContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#count_pending}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCount_pending(FraudoPaymentParser.Count_pendingContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#count_error}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCount_error(FraudoPaymentParser.Count_errorContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#count_chargeback}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCount_chargeback(FraudoPaymentParser.Count_chargebackContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#count_refund}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCount_refund(FraudoPaymentParser.Count_refundContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#sum_success}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSum_success(FraudoPaymentParser.Sum_successContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#sum_error}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSum_error(FraudoPaymentParser.Sum_errorContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#sum_chargeback}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSum_chargeback(FraudoPaymentParser.Sum_chargebackContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#sum_refund}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSum_refund(FraudoPaymentParser.Sum_refundContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#in}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIn(FraudoPaymentParser.InContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#is_mobile}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIs_mobile(FraudoPaymentParser.Is_mobileContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#is_recurrent}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIs_recurrent(FraudoPaymentParser.Is_recurrentContext ctx);
/**
* Visit a parse tree produced by the {@code isTrusted}
* labeled alternative in {@link FraudoPaymentParser#is_trusted}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsTrusted(FraudoPaymentParser.IsTrustedContext ctx);
/**
* Visit a parse tree produced by the {@code isTrustedTemplateName}
* labeled alternative in {@link FraudoPaymentParser#is_trusted}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsTrustedTemplateName(FraudoPaymentParser.IsTrustedTemplateNameContext ctx);
/**
* Visit a parse tree produced by the {@code isTrustedConditionsSingleList}
* labeled alternative in {@link FraudoPaymentParser#is_trusted}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsTrustedConditionsSingleList(FraudoPaymentParser.IsTrustedConditionsSingleListContext ctx);
/**
* Visit a parse tree produced by the {@code isTrustedPaymentsAndWithdrawalConditions}
* labeled alternative in {@link FraudoPaymentParser#is_trusted}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIsTrustedPaymentsAndWithdrawalConditions(FraudoPaymentParser.IsTrustedPaymentsAndWithdrawalConditionsContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#payment_conditions}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPayment_conditions(FraudoPaymentParser.Payment_conditionsContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#withdrawal_conditions}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitWithdrawal_conditions(FraudoPaymentParser.Withdrawal_conditionsContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#conditions_list}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConditions_list(FraudoPaymentParser.Conditions_listContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#trusted_token_condition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrusted_token_condition(FraudoPaymentParser.Trusted_token_conditionContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#rand}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRand(FraudoPaymentParser.RandContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#fraud_rule}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFraud_rule(FraudoPaymentParser.Fraud_ruleContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#comparator}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComparator(FraudoPaymentParser.ComparatorContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#binary}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBinary(FraudoPaymentParser.BinaryContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#bool}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBool(FraudoPaymentParser.BoolContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#amount}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAmount(FraudoPaymentParser.AmountContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#currency}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCurrency(FraudoPaymentParser.CurrencyContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#payment_system}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPayment_system(FraudoPaymentParser.Payment_systemContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#card_category}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCard_category(FraudoPaymentParser.Card_categoryContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#payer_type}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPayer_type(FraudoPaymentParser.Payer_typeContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#token_provider}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitToken_provider(FraudoPaymentParser.Token_providerContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#count}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCount(FraudoPaymentParser.CountContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#sum}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSum(FraudoPaymentParser.SumContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#unique}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitUnique(FraudoPaymentParser.UniqueContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#in_white_list}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIn_white_list(FraudoPaymentParser.In_white_listContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#in_black_list}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIn_black_list(FraudoPaymentParser.In_black_listContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#in_grey_list}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIn_grey_list(FraudoPaymentParser.In_grey_listContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#in_list}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitIn_list(FraudoPaymentParser.In_listContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#like}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLike(FraudoPaymentParser.LikeContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#country_by}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCountry_by(FraudoPaymentParser.Country_byContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#result}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitResult(FraudoPaymentParser.ResultContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#catch_result}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCatch_result(FraudoPaymentParser.Catch_resultContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#string_list}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitString_list(FraudoPaymentParser.String_listContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#time_window}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTime_window(FraudoPaymentParser.Time_windowContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#time_unit}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTime_unit(FraudoPaymentParser.Time_unitContext ctx);
/**
* Visit a parse tree produced by {@link FraudoPaymentParser#group_by}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitGroup_by(FraudoPaymentParser.Group_byContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy