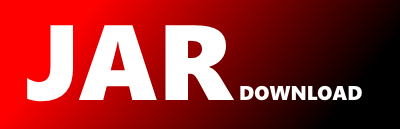
dev.vality.swag.payments.api.CustomersApi Maven / Gradle / Ivy
package dev.vality.swag.payments.api;
import dev.vality.swag.payments.ApiClient;
import dev.vality.swag.payments.model.AccessToken;
import dev.vality.swag.payments.model.Customer;
import dev.vality.swag.payments.model.CustomerAndToken;
import dev.vality.swag.payments.model.CustomerBinding;
import dev.vality.swag.payments.model.CustomerBindingParams;
import dev.vality.swag.payments.model.CustomerEvent;
import dev.vality.swag.payments.model.CustomerParams;
import dev.vality.swag.payments.model.DefaultLogicError;
import dev.vality.swag.payments.model.ExternalIDConflictError;
import dev.vality.swag.payments.model.GeneralError;
import dev.vality.swag.payments.model.InlineResponse400;
import dev.vality.swag.payments.model.InlineResponse4001;
import dev.vality.swag.payments.model.InlineResponse4002;
import dev.vality.swag.payments.model.PaymentMethod;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestClientException;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.web.util.UriComponentsBuilder;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2022-08-22T08:18:24.176Z")
@Component("dev.vality.swag.payments.api.CustomersApi")
public class CustomersApi {
private ApiClient apiClient;
public CustomersApi() {
this(new ApiClient());
}
@Autowired
public CustomersApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
*
* Запустить новую привязку к плательшику.
* 201 - Привязка запущена
*
400 - Ошибочные данные для привязки
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
*
409 - Переданное значение `externalID` уже использовалось вами ранее с другими параметрами запроса
* @param xRequestID Уникальный идентификатор запроса к системе
* @param customerID Идентификатор кастомера
* @param bindingParams Параметры создаваемой привязки
* @param xRequestDeadline Максимальное время обработки запроса
* @return CustomerBinding
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public CustomerBinding createBinding(String xRequestID, String customerID, CustomerBindingParams bindingParams, String xRequestDeadline) throws RestClientException {
Object postBody = bindingParams;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling createBinding");
}
// verify the required parameter 'customerID' is set
if (customerID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerID' when calling createBinding");
}
// verify the required parameter 'bindingParams' is set
if (bindingParams == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'bindingParams' when calling createBinding");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("customerID", customerID);
String path = UriComponentsBuilder.fromPath("/processing/customers/{customerID}/bindings").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Создать нового плательщика.
* 201 - Плательщик создан
*
400 - Ошибочные данные плательщика
*
401 - Ошибка авторизации
*
409 - Переданное значение `externalID` уже использовалось вами ранее с другими параметрами запроса
* @param xRequestID Уникальный идентификатор запроса к системе
* @param customerParams Параметры создаваемого плательщика
* @param xRequestDeadline Максимальное время обработки запроса
* @return CustomerAndToken
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public CustomerAndToken createCustomer(String xRequestID, CustomerParams customerParams, String xRequestDeadline) throws RestClientException {
Object postBody = customerParams;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling createCustomer");
}
// verify the required parameter 'customerParams' is set
if (customerParams == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerParams' when calling createCustomer");
}
String path = UriComponentsBuilder.fromPath("/processing/customers").build().toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Создать новый токен для доступа к указанному плательщику.
* 201 - Токен для доступа создан
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param customerID Идентификатор кастомера
* @param xRequestDeadline Максимальное время обработки запроса
* @return AccessToken
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public AccessToken createCustomerAccessToken(String xRequestID, String customerID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling createCustomerAccessToken");
}
// verify the required parameter 'customerID' is set
if (customerID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerID' when calling createCustomerAccessToken");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("customerID", customerID);
String path = UriComponentsBuilder.fromPath("/processing/customers/{customerID}/access-tokens").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Удалить плательщика по его идентификатору
* 204 - Плательщик удален
*
400 - Ошибка удаления плательщика
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param customerID Идентификатор кастомера
* @param xRequestDeadline Максимальное время обработки запроса
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public void deleteCustomer(String xRequestID, String customerID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling deleteCustomer");
}
// verify the required parameter 'customerID' is set
if (customerID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerID' when calling deleteCustomer");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("customerID", customerID);
String path = UriComponentsBuilder.fromPath("/processing/customers/{customerID}").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
apiClient.invokeAPI(path, HttpMethod.DELETE, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить информацию о привязке к плательщику.
* 200 - Данные привязки
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param customerID Идентификатор кастомера
* @param customerBindingID Идентификатор привязки к кастомера
* @param xRequestDeadline Максимальное время обработки запроса
* @return CustomerBinding
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public CustomerBinding getBinding(String xRequestID, String customerID, String customerBindingID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getBinding");
}
// verify the required parameter 'customerID' is set
if (customerID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerID' when calling getBinding");
}
// verify the required parameter 'customerBindingID' is set
if (customerBindingID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerBindingID' when calling getBinding");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("customerID", customerID);
uriVariables.put("customerBindingID", customerBindingID);
String path = UriComponentsBuilder.fromPath("/processing/customers/{customerID}/bindings/{customerBindingID}").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить все привязки к плательщику.
* 200 - Список привязок
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param customerID Идентификатор кастомера
* @param xRequestDeadline Максимальное время обработки запроса
* @return List<CustomerBinding>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getBindings(String xRequestID, String customerID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getBindings");
}
// verify the required parameter 'customerID' is set
if (customerID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerID' when calling getBindings");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("customerID", customerID);
String path = UriComponentsBuilder.fromPath("/processing/customers/{customerID}/bindings").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference> returnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить данные плательщика по его идентификатору.
* 200 - Данные плательщика
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param customerID Идентификатор кастомера
* @param xRequestDeadline Максимальное время обработки запроса
* @return Customer
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Customer getCustomerById(String xRequestID, String customerID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getCustomerById");
}
// verify the required parameter 'customerID' is set
if (customerID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerID' when calling getCustomerById");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("customerID", customerID);
String path = UriComponentsBuilder.fromPath("/processing/customers/{customerID}").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить историю указанного плательщика в виде набора событий.
* 200 - Набор событий
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param customerID Идентификатор кастомера
* @param limit Лимит выборки
* @param xRequestDeadline Максимальное время обработки запроса
* @param eventID Идентификатор события. Все события, возникшие в системе _после_ указанного, попадут в выборку.
* @return List<CustomerEvent>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getCustomerEvents(String xRequestID, String customerID, Integer limit, String xRequestDeadline, Integer eventID) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getCustomerEvents");
}
// verify the required parameter 'customerID' is set
if (customerID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerID' when calling getCustomerEvents");
}
// verify the required parameter 'limit' is set
if (limit == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'limit' when calling getCustomerEvents");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("customerID", customerID);
String path = UriComponentsBuilder.fromPath("/processing/customers/{customerID}/events").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "limit", limit));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "eventID", eventID));
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference> returnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить доступные для плательщика методы оплаты.
* 200 - Список методов оплаты
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param customerID Идентификатор кастомера
* @param xRequestDeadline Максимальное время обработки запроса
* @return List<PaymentMethod>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getCustomerPaymentMethods(String xRequestID, String customerID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getCustomerPaymentMethods");
}
// verify the required parameter 'customerID' is set
if (customerID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'customerID' when calling getCustomerPaymentMethods");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("customerID", customerID);
String path = UriComponentsBuilder.fromPath("/processing/customers/{customerID}/payment-methods").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference> returnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
}