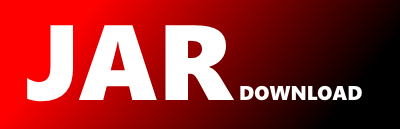
dev.vality.swag.payments.api.InvoicesApi Maven / Gradle / Ivy
package dev.vality.swag.payments.api;
import dev.vality.swag.payments.ApiClient;
import dev.vality.swag.payments.model.AccessToken;
import dev.vality.swag.payments.model.DefaultLogicError;
import dev.vality.swag.payments.model.ExternalIDConflictError;
import dev.vality.swag.payments.model.GeneralError;
import dev.vality.swag.payments.model.InlineResponse40012;
import dev.vality.swag.payments.model.InlineResponse4006;
import dev.vality.swag.payments.model.InlineResponse4007;
import dev.vality.swag.payments.model.Invoice;
import dev.vality.swag.payments.model.InvoiceAndToken;
import dev.vality.swag.payments.model.InvoiceEvent;
import dev.vality.swag.payments.model.InvoiceParams;
import dev.vality.swag.payments.model.PaymentMethod;
import dev.vality.swag.payments.model.Reason;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestClientException;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.web.util.UriComponentsBuilder;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2022-08-22T08:18:24.176Z")
@Component("dev.vality.swag.payments.api.InvoicesApi")
public class InvoicesApi {
private ApiClient apiClient;
public InvoicesApi() {
this(new ApiClient());
}
@Autowired
public InvoicesApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
*
* Создать новый инвойс.
* 201 - Инвойс создан
*
400 - Ошибочные данные для создания инвойса
*
401 - Ошибка авторизации
*
409 - Переданное значение `externalID` уже использовалось вами ранее с другими параметрами запроса
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceParams Параметры создаваемого инвойса
* @param xRequestDeadline Максимальное время обработки запроса
* @return InvoiceAndToken
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public InvoiceAndToken createInvoice(String xRequestID, InvoiceParams invoiceParams, String xRequestDeadline) throws RestClientException {
Object postBody = invoiceParams;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling createInvoice");
}
// verify the required parameter 'invoiceParams' is set
if (invoiceParams == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceParams' when calling createInvoice");
}
String path = UriComponentsBuilder.fromPath("/processing/invoices").build().toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Создать новый токен для доступа к указанному инвойсу.
* 201 - Токен для доступа к инвойсу создан.
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param xRequestDeadline Максимальное время обработки запроса
* @return AccessToken
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public AccessToken createInvoiceAccessToken(String xRequestID, String invoiceID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling createInvoiceAccessToken");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling createInvoiceAccessToken");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/access-tokens").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Погасить указанный инвойс.
* 204 - Инвойс погашен
*
400 - Ошибка погашения инвойса
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param fulfillInvoice Произвольная причина совершения операции
* @param xRequestDeadline Максимальное время обработки запроса
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public void fulfillInvoice(String xRequestID, String invoiceID, Reason fulfillInvoice, String xRequestDeadline) throws RestClientException {
Object postBody = fulfillInvoice;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling fulfillInvoice");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling fulfillInvoice");
}
// verify the required parameter 'fulfillInvoice' is set
if (fulfillInvoice == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'fulfillInvoice' when calling fulfillInvoice");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/fulfill").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить инвойс по указанному внешнему идентификатору.
* 200 - Инвойс
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param externalID Внешний идентификатор инвойса
* @param xRequestDeadline Максимальное время обработки запроса
* @return Invoice
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Invoice getInvoiceByExternalID(String xRequestID, String externalID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getInvoiceByExternalID");
}
// verify the required parameter 'externalID' is set
if (externalID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'externalID' when calling getInvoiceByExternalID");
}
String path = UriComponentsBuilder.fromPath("/processing/invoices").build().toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "externalID", externalID));
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить данные инвойса по его идентификатору.
* 200 - Данные инвойса
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param xRequestDeadline Максимальное время обработки запроса
* @return Invoice
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Invoice getInvoiceByID(String xRequestID, String invoiceID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getInvoiceByID");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling getInvoiceByID");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить историю указанного инвойса в виде набора событий.
* 200 - Набор событий
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param limit Лимит выборки
* @param xRequestDeadline Максимальное время обработки запроса
* @param eventID Идентификатор события. Все события, возникшие в системе _после_ указанного, попадут в выборку.
* @return List<InvoiceEvent>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getInvoiceEvents(String xRequestID, String invoiceID, Integer limit, String xRequestDeadline, Integer eventID) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getInvoiceEvents");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling getInvoiceEvents");
}
// verify the required parameter 'limit' is set
if (limit == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'limit' when calling getInvoiceEvents");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/events").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "limit", limit));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "eventID", eventID));
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference> returnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить доступные для инвойса методы оплаты.
* 200 - Список методов оплаты
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param xRequestDeadline Максимальное время обработки запроса
* @return List<PaymentMethod>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getInvoicePaymentMethods(String xRequestID, String invoiceID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getInvoicePaymentMethods");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling getInvoicePaymentMethods");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payment-methods").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference> returnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Перевести инвойс в состояние \"Отменен\" со статусом \"Аннулирован\".
* 204 - Инвойс аннулирован
*
400 - Ошибка отмены инвойса
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param rescindInvoice Произвольная причина совершения операции
* @param xRequestDeadline Максимальное время обработки запроса
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public void rescindInvoice(String xRequestID, String invoiceID, Reason rescindInvoice, String xRequestDeadline) throws RestClientException {
Object postBody = rescindInvoice;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling rescindInvoice");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling rescindInvoice");
}
// verify the required parameter 'rescindInvoice' is set
if (rescindInvoice == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'rescindInvoice' when calling rescindInvoice");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/rescind").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
}