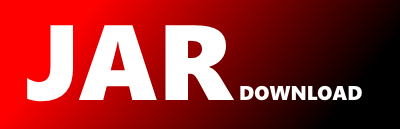
dev.vality.swag.payments.api.PaymentsApi Maven / Gradle / Ivy
package dev.vality.swag.payments.api;
import dev.vality.swag.payments.ApiClient;
import dev.vality.swag.payments.model.CaptureParams;
import dev.vality.swag.payments.model.Chargeback;
import dev.vality.swag.payments.model.DefaultLogicError;
import dev.vality.swag.payments.model.ExternalIDConflictError;
import dev.vality.swag.payments.model.GeneralError;
import dev.vality.swag.payments.model.InlineResponse40010;
import dev.vality.swag.payments.model.InlineResponse40011;
import dev.vality.swag.payments.model.InlineResponse4008;
import dev.vality.swag.payments.model.InlineResponse4009;
import dev.vality.swag.payments.model.Payment;
import dev.vality.swag.payments.model.PaymentParams;
import dev.vality.swag.payments.model.Reason;
import dev.vality.swag.payments.model.Refund;
import dev.vality.swag.payments.model.RefundParams;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestClientException;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.web.util.UriComponentsBuilder;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2022-08-22T08:18:24.176Z")
@Component("dev.vality.swag.payments.api.PaymentsApi")
public class PaymentsApi {
private ApiClient apiClient;
public PaymentsApi() {
this(new ApiClient());
}
@Autowired
public PaymentsApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
*
* Отменить указанный платеж.
* 202 - Запрос на отмену платежа принят
*
400 - Ошибка отмены платежа
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа в рамках инвойса
* @param cancelPayment Произвольная причина совершения операции
* @param xRequestDeadline Максимальное время обработки запроса
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public void cancelPayment(String xRequestID, String invoiceID, String paymentID, Reason cancelPayment, String xRequestDeadline) throws RestClientException {
Object postBody = cancelPayment;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling cancelPayment");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling cancelPayment");
}
// verify the required parameter 'paymentID' is set
if (paymentID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'paymentID' when calling cancelPayment");
}
// verify the required parameter 'cancelPayment' is set
if (cancelPayment == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'cancelPayment' when calling cancelPayment");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
uriVariables.put("paymentID", paymentID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments/{paymentID}/cancel").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Подтвердить указанный платеж. В случае передачи суммы подтверждения, меньшей, чем оригинальная, оставшаяся часть платежа будет возвращена.(см. [Варианты проведения оплаты](#tag/Payments))
* 202 - Запрос на подтверждение платежа принят
*
400 - Ошибка подтверждения платежа
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа в рамках инвойса
* @param capturePayment Параметры подтверждения платежа
* @param xRequestDeadline Максимальное время обработки запроса
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public void capturePayment(String xRequestID, String invoiceID, String paymentID, CaptureParams capturePayment, String xRequestDeadline) throws RestClientException {
Object postBody = capturePayment;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling capturePayment");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling capturePayment");
}
// verify the required parameter 'paymentID' is set
if (paymentID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'paymentID' when calling capturePayment");
}
// verify the required parameter 'capturePayment' is set
if (capturePayment == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'capturePayment' when calling capturePayment");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
uriVariables.put("paymentID", paymentID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments/{paymentID}/capture").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Создать новый платеж по указанному инвойсу.
* 201 - Платёж создан
*
400 - Ошибочные данные для запуска платежа
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
*
409 - Переданное значение `externalID` уже использовалось вами ранее с другими параметрами запроса
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param paymentParams Параметры создаваемого платежа
* @param xRequestDeadline Максимальное время обработки запроса
* @return Payment
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Payment createPayment(String xRequestID, String invoiceID, PaymentParams paymentParams, String xRequestDeadline) throws RestClientException {
Object postBody = paymentParams;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling createPayment");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling createPayment");
}
// verify the required parameter 'paymentParams' is set
if (paymentParams == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'paymentParams' when calling createPayment");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Создать возврат указанного платежа
* 201 - Возврат создан
*
400 - Ошибочные данные для возврата
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
*
409 - Переданное значение `externalID` уже использовалось вами ранее с другими параметрами запроса
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа в рамках инвойса
* @param refundParams Параметры создаваемого возврата платежа
* @param xRequestDeadline Максимальное время обработки запроса
* @return Refund
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Refund createRefund(String xRequestID, String invoiceID, String paymentID, RefundParams refundParams, String xRequestDeadline) throws RestClientException {
Object postBody = refundParams;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling createRefund");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling createRefund");
}
// verify the required parameter 'paymentID' is set
if (paymentID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'paymentID' when calling createRefund");
}
// verify the required parameter 'refundParams' is set
if (refundParams == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'refundParams' when calling createRefund");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
uriVariables.put("paymentID", paymentID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments/{paymentID}/refunds").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.POST, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить данные определённого чарджбэка для указанного платежа.
* 200 - Данные чарджбэка
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа в рамках инвойса
* @param chargebackID Идентификатор возвратного платежа в рамках платежа
* @param xRequestDeadline Максимальное время обработки запроса
* @return Chargeback
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Chargeback getChargebackByID(String xRequestID, String invoiceID, String paymentID, String chargebackID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getChargebackByID");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling getChargebackByID");
}
// verify the required parameter 'paymentID' is set
if (paymentID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'paymentID' when calling getChargebackByID");
}
// verify the required parameter 'chargebackID' is set
if (chargebackID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'chargebackID' when calling getChargebackByID");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
uriVariables.put("paymentID", paymentID);
uriVariables.put("chargebackID", chargebackID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments/{paymentID}/chargebacks/{chargebackID}").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить все чарджбэки по указанному платежу.
* 200 - Данные чарджбэков по платежу
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа в рамках инвойса
* @param xRequestDeadline Максимальное время обработки запроса
* @return List<Chargeback>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getChargebacks(String xRequestID, String invoiceID, String paymentID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getChargebacks");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling getChargebacks");
}
// verify the required parameter 'paymentID' is set
if (paymentID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'paymentID' when calling getChargebacks");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
uriVariables.put("paymentID", paymentID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments/{paymentID}/chargebacks").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference> returnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить платёж по указанному внешнему идентификатору.
* 200 - Платёж
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param externalID Внешний идентификатор платежа
* @param xRequestDeadline Максимальное время обработки запроса
* @return Payment
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Payment getPaymentByExternalID(String xRequestID, String externalID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getPaymentByExternalID");
}
// verify the required parameter 'externalID' is set
if (externalID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'externalID' when calling getPaymentByExternalID");
}
String path = UriComponentsBuilder.fromPath("/processing/payments").build().toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "externalID", externalID));
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить данные платежа по указанному инвойсу.
* 200 - Данные платежа
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа в рамках инвойса
* @param xRequestDeadline Максимальное время обработки запроса
* @return Payment
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Payment getPaymentByID(String xRequestID, String invoiceID, String paymentID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getPaymentByID");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling getPaymentByID");
}
// verify the required parameter 'paymentID' is set
if (paymentID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'paymentID' when calling getPaymentByID");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
uriVariables.put("paymentID", paymentID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments/{paymentID}").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить все платежи по указанному инвойсу.
* 200 - Данные платежей по инвойсу
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param xRequestDeadline Максимальное время обработки запроса
* @return List<Payment>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getPayments(String xRequestID, String invoiceID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getPayments");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling getPayments");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference> returnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить возврат по указанному внешнему идентификатору.
* 200 - Данные возврата
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param externalID Внешний идентификатор возврата
* @param xRequestDeadline Максимальное время обработки запроса
* @return Refund
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Refund getRefundByExternalID(String xRequestID, String externalID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getRefundByExternalID");
}
// verify the required parameter 'externalID' is set
if (externalID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'externalID' when calling getRefundByExternalID");
}
String path = UriComponentsBuilder.fromPath("/processing/refunds").build().toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "externalID", externalID));
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить данные определённого возврата указанного платежа.
* 200 - Данные возврата
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа в рамках инвойса
* @param refundID Идентификатор возврата в рамках платежа
* @param xRequestDeadline Максимальное время обработки запроса
* @return Refund
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public Refund getRefundByID(String xRequestID, String invoiceID, String paymentID, String refundID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getRefundByID");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling getRefundByID");
}
// verify the required parameter 'paymentID' is set
if (paymentID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'paymentID' when calling getRefundByID");
}
// verify the required parameter 'refundID' is set
if (refundID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'refundID' when calling getRefundByID");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
uriVariables.put("paymentID", paymentID);
uriVariables.put("refundID", refundID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments/{paymentID}/refunds/{refundID}").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Получить все возвраты указанного платежа.
* 200 - Данные возвратов по платежу
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа в рамках инвойса
* @param xRequestDeadline Максимальное время обработки запроса
* @return List<Refund>
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public List getRefunds(String xRequestID, String invoiceID, String paymentID, String xRequestDeadline) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling getRefunds");
}
// verify the required parameter 'invoiceID' is set
if (invoiceID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'invoiceID' when calling getRefunds");
}
// verify the required parameter 'paymentID' is set
if (paymentID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'paymentID' when calling getRefunds");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("invoiceID", invoiceID);
uriVariables.put("paymentID", paymentID);
String path = UriComponentsBuilder.fromPath("/processing/invoices/{invoiceID}/payments/{paymentID}/refunds").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference> returnType = new ParameterizedTypeReference>() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
}