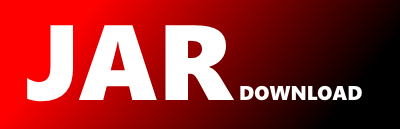
dev.vality.swag.payments.api.SearchApi Maven / Gradle / Ivy
package dev.vality.swag.payments.api;
import dev.vality.swag.payments.ApiClient;
import dev.vality.swag.payments.model.DefaultLogicError;
import dev.vality.swag.payments.model.GeneralError;
import dev.vality.swag.payments.model.InlineResponse200;
import dev.vality.swag.payments.model.InlineResponse2001;
import dev.vality.swag.payments.model.InlineResponse2002;
import dev.vality.swag.payments.model.InlineResponse2003;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestClientException;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.web.util.UriComponentsBuilder;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2022-08-22T08:18:24.176Z")
@Component("dev.vality.swag.payments.api.SearchApi")
public class SearchApi {
private ApiClient apiClient;
public SearchApi() {
this(new ApiClient());
}
@Autowired
public SearchApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
*
* Поиск инвойсов
* 200 - Найденные инвойсы
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param shopID Идентификатор магазина
* @param fromTime Начало временного отрезка
* @param toTime Конец временного отрезка
* @param limit Лимит выборки
* @param xRequestDeadline Максимальное время обработки запроса
* @param invoiceStatus Статус инвойса для поиска
* @param paymentStatus Статус платежа для поиска
* @param paymentFlow Flow платежа
* @param paymentMethod Метод оплаты
* @param paymentTerminalProvider Провайдер платежного терминала
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа
* @param payerEmail Email, указанный при оплате
* @param payerIP IP-адрес плательщика
* @param payerFingerprint Уникальный отпечаток user agent'а плательщика
* @param customerID Идентификатор плательщика
* @param bankCardTokenProvider Провайдер платежных токенов. Набор провайдеров, доступных для проведения платежей, можно узнать, вызвав соответствующую [операцию](#operation/getInvoicePaymentMethods) после создания инвойса.
* @param bankCardPaymentSystem Платежная система. Набор систем, доступных для проведения платежей, можно узнать, вызвав соответствующую [операцию](#operation/getInvoicePaymentMethods) после создания инвойса.
* @param first6 Первые 6 цифр номера карты
* @param last4 Последние цифры номера карты
* @param rrn Retrieval Reference Number
* @param paymentAmount Сумма платежа
* @param invoiceAmount Сумма инвойса
* @param continuationToken Токен, сигнализирующий о том, что в ответе передана только часть данных. Для получения следующей части нужно повторно обратиться к сервису, указав тот-же набор условий и полученый токен. Если токена нет, получена последняя часть данных.
* @return InlineResponse200
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public InlineResponse200 searchInvoices(String xRequestID, String shopID, OffsetDateTime fromTime, OffsetDateTime toTime, Integer limit, String xRequestDeadline, String invoiceStatus, String paymentStatus, String paymentFlow, String paymentMethod, String paymentTerminalProvider, String invoiceID, String paymentID, String payerEmail, String payerIP, String payerFingerprint, String customerID, String bankCardTokenProvider, String bankCardPaymentSystem, String first6, String last4, String rrn, Long paymentAmount, Long invoiceAmount, String continuationToken) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling searchInvoices");
}
// verify the required parameter 'shopID' is set
if (shopID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'shopID' when calling searchInvoices");
}
// verify the required parameter 'fromTime' is set
if (fromTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'fromTime' when calling searchInvoices");
}
// verify the required parameter 'toTime' is set
if (toTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'toTime' when calling searchInvoices");
}
// verify the required parameter 'limit' is set
if (limit == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'limit' when calling searchInvoices");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("shopID", shopID);
String path = UriComponentsBuilder.fromPath("/analytics/shops/{shopID}/invoices").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "fromTime", fromTime));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "toTime", toTime));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "limit", limit));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "invoiceStatus", invoiceStatus));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentStatus", paymentStatus));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentFlow", paymentFlow));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentMethod", paymentMethod));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentTerminalProvider", paymentTerminalProvider));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "invoiceID", invoiceID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentID", paymentID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "payerEmail", payerEmail));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "payerIP", payerIP));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "payerFingerprint", payerFingerprint));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "customerID", customerID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "bankCardTokenProvider", bankCardTokenProvider));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "bankCardPaymentSystem", bankCardPaymentSystem));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "first6", first6));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "last4", last4));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "rrn", rrn));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentAmount", paymentAmount));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "invoiceAmount", invoiceAmount));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "continuationToken", continuationToken));
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Поиск платежей
* 200 - Найденные платежи
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param shopID Идентификатор магазина
* @param fromTime Начало временного отрезка
* @param toTime Конец временного отрезка
* @param limit Лимит выборки
* @param xRequestDeadline Максимальное время обработки запроса
* @param paymentStatus Статус платежа для поиска
* @param paymentFlow Flow платежа
* @param paymentMethod Метод оплаты
* @param paymentTerminalProvider Провайдер платежного терминала
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа
* @param payerEmail Email, указанный при оплате
* @param payerIP IP-адрес плательщика
* @param payerFingerprint Уникальный отпечаток user agent'а плательщика
* @param customerID Идентификатор плательщика
* @param first6 Первые 6 цифр номера карты
* @param last4 Последние цифры номера карты
* @param rrn Retrieval Reference Number
* @param approvalCode Authorization Approval Code
* @param bankCardTokenProvider Провайдер платежных токенов. Набор провайдеров, доступных для проведения платежей, можно узнать, вызвав соответствующую [операцию](#operation/getInvoicePaymentMethods) после создания инвойса.
* @param bankCardPaymentSystem Платежная система. Набор систем, доступных для проведения платежей, можно узнать, вызвав соответствующую [операцию](#operation/getInvoicePaymentMethods) после создания инвойса.
* @param paymentAmount Сумма платежа
* @param continuationToken Токен, сигнализирующий о том, что в ответе передана только часть данных. Для получения следующей части нужно повторно обратиться к сервису, указав тот-же набор условий и полученый токен. Если токена нет, получена последняя часть данных.
* @return InlineResponse2001
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public InlineResponse2001 searchPayments(String xRequestID, String shopID, OffsetDateTime fromTime, OffsetDateTime toTime, Integer limit, String xRequestDeadline, String paymentStatus, String paymentFlow, String paymentMethod, String paymentTerminalProvider, String invoiceID, String paymentID, String payerEmail, String payerIP, String payerFingerprint, String customerID, String first6, String last4, String rrn, String approvalCode, String bankCardTokenProvider, String bankCardPaymentSystem, Long paymentAmount, String continuationToken) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling searchPayments");
}
// verify the required parameter 'shopID' is set
if (shopID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'shopID' when calling searchPayments");
}
// verify the required parameter 'fromTime' is set
if (fromTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'fromTime' when calling searchPayments");
}
// verify the required parameter 'toTime' is set
if (toTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'toTime' when calling searchPayments");
}
// verify the required parameter 'limit' is set
if (limit == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'limit' when calling searchPayments");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("shopID", shopID);
String path = UriComponentsBuilder.fromPath("/analytics/shops/{shopID}/payments").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "fromTime", fromTime));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "toTime", toTime));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "limit", limit));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentStatus", paymentStatus));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentFlow", paymentFlow));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentMethod", paymentMethod));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentTerminalProvider", paymentTerminalProvider));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "invoiceID", invoiceID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentID", paymentID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "payerEmail", payerEmail));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "payerIP", payerIP));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "payerFingerprint", payerFingerprint));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "customerID", customerID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "first6", first6));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "last4", last4));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "rrn", rrn));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "approvalCode", approvalCode));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "bankCardTokenProvider", bankCardTokenProvider));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "bankCardPaymentSystem", bankCardPaymentSystem));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentAmount", paymentAmount));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "continuationToken", continuationToken));
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Поиск выплат
* 200 - Найденные выплаты
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param shopID Идентификатор магазина
* @param fromTime Начало временного отрезка
* @param toTime Конец временного отрезка
* @param limit Лимит выборки
* @param xRequestDeadline Максимальное время обработки запроса
* @param offset Смещение выборки
* @param payoutID Идентификатор выплаты
* @param payoutToolType Тип выплаты для поиска * PayoutAccount - выплата на банковский счёт * Wallet - выплата на кошелёк * PaymentInstitutionAccount - выплата на счёт платежной организации
* @return InlineResponse2002
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public InlineResponse2002 searchPayouts(String xRequestID, String shopID, OffsetDateTime fromTime, OffsetDateTime toTime, Integer limit, String xRequestDeadline, Integer offset, String payoutID, String payoutToolType) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling searchPayouts");
}
// verify the required parameter 'shopID' is set
if (shopID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'shopID' when calling searchPayouts");
}
// verify the required parameter 'fromTime' is set
if (fromTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'fromTime' when calling searchPayouts");
}
// verify the required parameter 'toTime' is set
if (toTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'toTime' when calling searchPayouts");
}
// verify the required parameter 'limit' is set
if (limit == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'limit' when calling searchPayouts");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("shopID", shopID);
String path = UriComponentsBuilder.fromPath("/analytics/shops/{shopID}/payouts").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "fromTime", fromTime));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "toTime", toTime));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "limit", limit));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "offset", offset));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "payoutID", payoutID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "payoutToolType", payoutToolType));
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
/**
*
* Поиск возвратов
* 200 - Найденные возвраты
*
400 - Неверные данные
*
401 - Ошибка авторизации
*
404 - Заданный ресурс не найден
* @param xRequestID Уникальный идентификатор запроса к системе
* @param shopID Идентификатор магазина
* @param fromTime Начало временного отрезка
* @param toTime Конец временного отрезка
* @param limit Лимит выборки
* @param xRequestDeadline Максимальное время обработки запроса
* @param offset Смещение выборки
* @param invoiceID Идентификатор инвойса
* @param paymentID Идентификатор платежа
* @param refundID Идентификатор возврата
* @param rrn Retrieval Reference Number
* @param approvalCode Authorization Approval Code
* @param refundStatus Статус возврата
* @return InlineResponse2003
* @throws RestClientException if an error occurs while attempting to invoke the API
*/
public InlineResponse2003 searchRefunds(String xRequestID, String shopID, OffsetDateTime fromTime, OffsetDateTime toTime, Integer limit, String xRequestDeadline, Integer offset, String invoiceID, String paymentID, String refundID, String rrn, String approvalCode, String refundStatus) throws RestClientException {
Object postBody = null;
// verify the required parameter 'xRequestID' is set
if (xRequestID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'xRequestID' when calling searchRefunds");
}
// verify the required parameter 'shopID' is set
if (shopID == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'shopID' when calling searchRefunds");
}
// verify the required parameter 'fromTime' is set
if (fromTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'fromTime' when calling searchRefunds");
}
// verify the required parameter 'toTime' is set
if (toTime == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'toTime' when calling searchRefunds");
}
// verify the required parameter 'limit' is set
if (limit == null) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Missing the required parameter 'limit' when calling searchRefunds");
}
// create path and map variables
final Map uriVariables = new HashMap();
uriVariables.put("shopID", shopID);
String path = UriComponentsBuilder.fromPath("/analytics/shops/{shopID}/refunds").buildAndExpand(uriVariables).toUriString();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "fromTime", fromTime));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "toTime", toTime));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "limit", limit));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "offset", offset));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "invoiceID", invoiceID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "paymentID", paymentID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "refundID", refundID));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "rrn", rrn));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "approvalCode", approvalCode));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "refundStatus", refundStatus));
if (xRequestID != null)
headerParams.add("X-Request-ID", apiClient.parameterToString(xRequestID));
if (xRequestDeadline != null)
headerParams.add("X-Request-Deadline", apiClient.parameterToString(xRequestDeadline));
final String[] accepts = {
"application/json; charset=utf-8"
};
final List accept = apiClient.selectHeaderAccept(accepts);
final String[] contentTypes = {
"application/json; charset=utf-8"
};
final MediaType contentType = apiClient.selectHeaderContentType(contentTypes);
String[] authNames = new String[] { "bearer" };
ParameterizedTypeReference returnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI(path, HttpMethod.GET, queryParams, postBody, headerParams, formParams, accept, contentType, authNames, returnType);
}
}