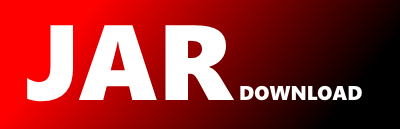
dev.vality.swag.payments.model.Invoice Maven / Gradle / Ivy
/*
* Vality Payments API
* ## Описание Vality Payments API предназначен для мерчантов, принимающих платежи из своего пользовательского интерфейса, например веб-сайта или мобильного приложения, и является единственной точкой взаимодействия с системой для проведения операций оплаты товаров и услуг. ## Детали взаимодействия При любом обращении к API в заголовке `X-Request-ID` соответствующего запроса необходимо передать его уникальный идентификатор: ``` X-Request-ID: 37d735d4-0f42-4f05-89fa-eaa478fb5aa9 ``` ### Тип содержимого и кодировка Система принимает и возвращает данные в формате JSON и кодировке UTF-8: ``` Content-Type: application/json; charset=utf-8 ``` ### Формат дат Система принимает и возвращает значения отметок времени в формате `date-time`, описанном в [RFC 3339](https://datatracker.ietf.org/doc/html/rfc3339): ``` 2017-01-01T00:00:00Z 2017-01-01T00:00:01+00:00 ``` ### Максимальное время обработки запроса При любом обращении к API в заголовке `X-Request-Deadline` соответствующего запроса можно передать параметр отсечки по времени, определяющий максимальное время ожидания завершения операции по запросу: ``` X-Request-Deadline: 10s ``` По истечении указанного времени система прекращает обработку запроса. Рекомендуется указывать значение не более одной минуты, но не менее трёх секунд. `X-Request-Deadline` может: * задаваться в формате `date-time` согласно [RFC 3339](https://datatracker.ietf.org/doc/html/rfc3339); * задаваться в относительных величинах: в миллисекундах (`150000ms`), секундах (`540s`) или минутах (`3.5m`).
*
* OpenAPI spec version: 2.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package dev.vality.swag.payments.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import dev.vality.swag.payments.model.Allocation;
import dev.vality.swag.payments.model.InvoiceBankAccount;
import dev.vality.swag.payments.model.InvoiceCart;
import dev.vality.swag.payments.model.InvoiceClientInfo;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
/**
* Invoice
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.JavaClientCodegen", date = "2022-08-22T08:18:24.176Z")
public class Invoice {
@JsonProperty("id")
private String id = null;
@JsonProperty("shopID")
private String shopID = null;
@JsonProperty("externalID")
private String externalID = null;
@JsonProperty("createdAt")
private OffsetDateTime createdAt = null;
@JsonProperty("dueDate")
private OffsetDateTime dueDate = null;
@JsonProperty("amount")
private Long amount = null;
@JsonProperty("currency")
private String currency = null;
@JsonProperty("product")
private String product = null;
@JsonProperty("description")
private String description = null;
@JsonProperty("invoiceTemplateID")
private String invoiceTemplateID = null;
@JsonProperty("cart")
private InvoiceCart cart = null;
@JsonProperty("allocation")
private Allocation allocation = null;
@JsonProperty("bankAccount")
private InvoiceBankAccount bankAccount = null;
@JsonProperty("metadata")
private Object metadata = null;
@JsonProperty("clientInfo")
private InvoiceClientInfo clientInfo = null;
/**
* Статус инвойса
*/
public enum StatusEnum {
UNPAID("unpaid"),
CANCELLED("cancelled"),
PAID("paid"),
FULFILLED("fulfilled");
private String value;
StatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("status")
private StatusEnum status = null;
@JsonProperty("reason")
private String reason = null;
public Invoice id(String id) {
this.id = id;
return this;
}
/**
* Идентификатор инвойса
* @return id
**/
@ApiModelProperty(required = true, value = "Идентификатор инвойса")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Invoice shopID(String shopID) {
this.shopID = shopID;
return this;
}
/**
* Идентификатор магазина
* @return shopID
**/
@ApiModelProperty(required = true, value = "Идентификатор магазина")
public String getShopID() {
return shopID;
}
public void setShopID(String shopID) {
this.shopID = shopID;
}
public Invoice externalID(String externalID) {
this.externalID = externalID;
return this;
}
/**
* Внешний идентификатор инвойса
* @return externalID
**/
@ApiModelProperty(value = "Внешний идентификатор инвойса")
public String getExternalID() {
return externalID;
}
public void setExternalID(String externalID) {
this.externalID = externalID;
}
public Invoice createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Дата и время создания
* @return createdAt
**/
@ApiModelProperty(required = true, value = "Дата и время создания")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public Invoice dueDate(OffsetDateTime dueDate) {
this.dueDate = dueDate;
return this;
}
/**
* Дата и время окончания действия
* @return dueDate
**/
@ApiModelProperty(required = true, value = "Дата и время окончания действия")
public OffsetDateTime getDueDate() {
return dueDate;
}
public void setDueDate(OffsetDateTime dueDate) {
this.dueDate = dueDate;
}
public Invoice amount(Long amount) {
this.amount = amount;
return this;
}
/**
* Стоимость предлагаемых товаров или услуг, в минорных денежных единицах, например в копейках в случае указания российских рублей в качестве валюты.
* minimum: 1
* @return amount
**/
@ApiModelProperty(required = true, value = "Стоимость предлагаемых товаров или услуг, в минорных денежных единицах, например в копейках в случае указания российских рублей в качестве валюты. ")
public Long getAmount() {
return amount;
}
public void setAmount(Long amount) {
this.amount = amount;
}
public Invoice currency(String currency) {
this.currency = currency;
return this;
}
/**
* Валюта, символьный код согласно [ISO 4217](http://www.iso.org/iso/home/standards/currency_codes.htm).
* @return currency
**/
@ApiModelProperty(required = true, value = "Валюта, символьный код согласно [ISO 4217](http://www.iso.org/iso/home/standards/currency_codes.htm).")
public String getCurrency() {
return currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public Invoice product(String product) {
this.product = product;
return this;
}
/**
* Наименование предлагаемых товаров или услуг
* @return product
**/
@ApiModelProperty(required = true, value = "Наименование предлагаемых товаров или услуг")
public String getProduct() {
return product;
}
public void setProduct(String product) {
this.product = product;
}
public Invoice description(String description) {
this.description = description;
return this;
}
/**
* Описание предлагаемых товаров или услуг
* @return description
**/
@ApiModelProperty(value = "Описание предлагаемых товаров или услуг")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Invoice invoiceTemplateID(String invoiceTemplateID) {
this.invoiceTemplateID = invoiceTemplateID;
return this;
}
/**
* Идентификатор шаблона (для инвойсов, созданных по шаблону).
* @return invoiceTemplateID
**/
@ApiModelProperty(value = "Идентификатор шаблона (для инвойсов, созданных по шаблону).")
public String getInvoiceTemplateID() {
return invoiceTemplateID;
}
public void setInvoiceTemplateID(String invoiceTemplateID) {
this.invoiceTemplateID = invoiceTemplateID;
}
public Invoice cart(InvoiceCart cart) {
this.cart = cart;
return this;
}
/**
* Get cart
* @return cart
**/
@ApiModelProperty(value = "")
public InvoiceCart getCart() {
return cart;
}
public void setCart(InvoiceCart cart) {
this.cart = cart;
}
public Invoice allocation(Allocation allocation) {
this.allocation = allocation;
return this;
}
/**
* Get allocation
* @return allocation
**/
@ApiModelProperty(value = "")
public Allocation getAllocation() {
return allocation;
}
public void setAllocation(Allocation allocation) {
this.allocation = allocation;
}
public Invoice bankAccount(InvoiceBankAccount bankAccount) {
this.bankAccount = bankAccount;
return this;
}
/**
* Get bankAccount
* @return bankAccount
**/
@ApiModelProperty(value = "")
public InvoiceBankAccount getBankAccount() {
return bankAccount;
}
public void setBankAccount(InvoiceBankAccount bankAccount) {
this.bankAccount = bankAccount;
}
public Invoice metadata(Object metadata) {
this.metadata = metadata;
return this;
}
/**
* Связанные с инвойсом метаданные
* @return metadata
**/
@ApiModelProperty(required = true, value = "Связанные с инвойсом метаданные")
public Object getMetadata() {
return metadata;
}
public void setMetadata(Object metadata) {
this.metadata = metadata;
}
public Invoice clientInfo(InvoiceClientInfo clientInfo) {
this.clientInfo = clientInfo;
return this;
}
/**
* Get clientInfo
* @return clientInfo
**/
@ApiModelProperty(value = "")
public InvoiceClientInfo getClientInfo() {
return clientInfo;
}
public void setClientInfo(InvoiceClientInfo clientInfo) {
this.clientInfo = clientInfo;
}
public Invoice status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Статус инвойса
* @return status
**/
@ApiModelProperty(required = true, value = "Статус инвойса")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public Invoice reason(String reason) {
this.reason = reason;
return this;
}
/**
* Причина отмены или погашения инвойса
* @return reason
**/
@ApiModelProperty(value = "Причина отмены или погашения инвойса")
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Invoice invoice = (Invoice) o;
return Objects.equals(this.id, invoice.id) &&
Objects.equals(this.shopID, invoice.shopID) &&
Objects.equals(this.externalID, invoice.externalID) &&
Objects.equals(this.createdAt, invoice.createdAt) &&
Objects.equals(this.dueDate, invoice.dueDate) &&
Objects.equals(this.amount, invoice.amount) &&
Objects.equals(this.currency, invoice.currency) &&
Objects.equals(this.product, invoice.product) &&
Objects.equals(this.description, invoice.description) &&
Objects.equals(this.invoiceTemplateID, invoice.invoiceTemplateID) &&
Objects.equals(this.cart, invoice.cart) &&
Objects.equals(this.allocation, invoice.allocation) &&
Objects.equals(this.bankAccount, invoice.bankAccount) &&
Objects.equals(this.metadata, invoice.metadata) &&
Objects.equals(this.clientInfo, invoice.clientInfo) &&
Objects.equals(this.status, invoice.status) &&
Objects.equals(this.reason, invoice.reason);
}
@Override
public int hashCode() {
return Objects.hash(id, shopID, externalID, createdAt, dueDate, amount, currency, product, description, invoiceTemplateID, cart, allocation, bankAccount, metadata, clientInfo, status, reason);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Invoice {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" shopID: ").append(toIndentedString(shopID)).append("\n");
sb.append(" externalID: ").append(toIndentedString(externalID)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" dueDate: ").append(toIndentedString(dueDate)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" product: ").append(toIndentedString(product)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" invoiceTemplateID: ").append(toIndentedString(invoiceTemplateID)).append("\n");
sb.append(" cart: ").append(toIndentedString(cart)).append("\n");
sb.append(" allocation: ").append(toIndentedString(allocation)).append("\n");
sb.append(" bankAccount: ").append(toIndentedString(bankAccount)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" clientInfo: ").append(toIndentedString(clientInfo)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" reason: ").append(toIndentedString(reason)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy