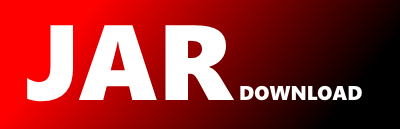
dev.vality.http.client.AsyncHttpClientImpl Maven / Gradle / Ivy
The newest version!
package dev.vality.http.client;
import dev.vality.http.client.exception.RemoteInvocationException;
import dev.vality.http.client.exception.UnknownClientException;
import io.micrometer.core.instrument.MeterRegistry;
import io.micrometer.core.instrument.Timer;
import lombok.Builder;
import lombok.extern.slf4j.Slf4j;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.*;
import org.apache.http.concurrent.FutureCallback;
import org.apache.http.impl.nio.client.CloseableHttpAsyncClient;
import java.util.concurrent.Future;
@Slf4j
@Builder
public class AsyncHttpClientImpl implements AsyncHttpClient {
private MeterRegistry registry;
private boolean isEnableMetric;
private CloseableHttpAsyncClient client;
@Override
public Future post(String methodName,
HttpPost httpPost,
FutureCallback callback,
CloseableHttpAsyncClient client) {
return httpExecution(methodName, httpPost, callback, client);
}
@Override
public Future post(String methodName,
HttpPost httpPost,
FutureCallback callback) {
return httpExecution(methodName, httpPost, callback, client);
}
@Override
public Future get(String methodName,
HttpGet httpGet,
FutureCallback callback,
CloseableHttpAsyncClient client) {
return httpExecution(methodName, httpGet, callback, client);
}
@Override
public Future get(String methodName,
HttpGet httpGet,
FutureCallback callback) {
return httpExecution(methodName, httpGet, callback, client);
}
@Override
public Future delete(String methodName,
HttpDelete httpDelete,
FutureCallback callback,
CloseableHttpAsyncClient client) {
return httpExecution(methodName, httpDelete, callback, client);
}
@Override
public Future delete(String methodName,
HttpDelete httpDelete,
FutureCallback callback) {
return httpExecution(methodName, httpDelete, callback, client);
}
@Override
public Future put(String methodName,
HttpPut httpPut,
FutureCallback callback,
CloseableHttpAsyncClient client) {
return httpExecution(methodName, httpPut, callback, client);
}
@Override
public Future put(String methodName,
HttpPut httpPut,
FutureCallback callback) {
return httpExecution(methodName, httpPut, callback, client);
}
private Future httpExecution(String methodName,
HttpRequestBase httpRequestBase,
FutureCallback callback,
CloseableHttpAsyncClient client) {
if (client == null) {
log.error("CloseableHttpAsyncClient client is unknown!");
throw new UnknownClientException();
}
try {
Timer.Sample sample = startSampleTimer();
if (!client.isRunning()) {
client.start();
}
Future execute = client.execute(httpRequestBase, callback);
String methodType = httpRequestBase.getMethod();
finishSampleTimer(methodType, methodName, sample);
log.debug("HttpClient finish methodType: {} methodName: {} httpGet: {} ",
methodType, methodName, httpRequestBase);
return execute;
} catch (Exception e) {
log.error("Error when httpExecution e: ", e);
throw new RemoteInvocationException(e);
}
}
private void finishSampleTimer(String methodType, String methodName, Timer.Sample sample) {
if (isEnableMetric && sample != null) {
sample.stop(registry.timer(methodType, methodName));
}
}
private Timer.Sample startSampleTimer() {
if (isEnableMetric && registry != null) {
return Timer.start(registry);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy