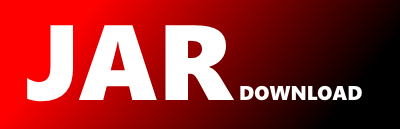
dev.vality.http.client.SyncAsyncHttpClient Maven / Gradle / Ivy
The newest version!
package dev.vality.http.client;
import dev.vality.http.client.callback.LogFutureCallback;
import dev.vality.http.client.domain.Response;
import dev.vality.http.client.exception.RemoteInvocationException;
import dev.vality.http.client.exception.UnknownClientException;
import io.micrometer.core.instrument.MeterRegistry;
import io.micrometer.core.instrument.Timer;
import lombok.Builder;
import lombok.extern.slf4j.Slf4j;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.*;
import org.apache.http.impl.nio.client.CloseableHttpAsyncClient;
import java.util.concurrent.Future;
import java.util.function.Function;
@Slf4j
@Builder
public class SyncAsyncHttpClient implements HttpClient {
private MeterRegistry registry;
private boolean isEnableMetric;
private CloseableHttpAsyncClient client;
@Override
public Response post(String methodName,
HttpPost httpPost,
Function handler,
CloseableHttpAsyncClient client) {
return httpExecution(methodName, httpPost, handler, client);
}
@Override
public Response post(String methodName, HttpPost httpPost, Function handler) {
return httpExecution(methodName, httpPost, handler, client);
}
@Override
public Response get(String methodName,
HttpGet httpGet,
Function handler,
CloseableHttpAsyncClient client) {
return httpExecution(methodName, httpGet, handler, client);
}
@Override
public Response get(String methodName, HttpGet httpGet, Function handler) {
return httpExecution(methodName, httpGet, handler, client);
}
@Override
public Response delete(String methodName,
HttpDelete httpDelete,
Function handler,
CloseableHttpAsyncClient client) {
return httpExecution(methodName, httpDelete, handler, client);
}
@Override
public Response delete(String methodName, HttpDelete httpDelete, Function handler) {
return httpExecution(methodName, httpDelete, handler, client);
}
@Override
public Response put(String methodName,
HttpPut httpPut,
Function handler,
CloseableHttpAsyncClient client) {
return httpExecution(methodName, httpPut, handler, client);
}
@Override
public Response put(String methodName, HttpPut httpPut, Function handler) {
return httpExecution(methodName, httpPut, handler, client);
}
private Response httpExecution(String methodName,
HttpRequestBase httpRequestBase,
Function handler,
CloseableHttpAsyncClient client) {
if (client == null) {
log.error("SimpleHttpClient client is unknown!");
throw new UnknownClientException();
}
try {
Timer.Sample sample = startSampleTimer();
if (!client.isRunning()) {
client.start();
}
Future execute = client.execute(httpRequestBase, new LogFutureCallback(httpRequestBase));
String methodType = httpRequestBase.getMethod();
HttpResponse httpResponse = execute.get();
finishSampleTimer(methodType, methodName, sample, httpResponse);
T result = handler.apply(httpResponse);
log.debug("HttpClient finish methodType: {} methodName: {} httpGet: {} with result: {}",
methodType, methodName, httpRequestBase, result);
return new Response<>(result);
} catch (Exception e) {
log.error("Error when httpExecution e: ", e);
throw new RemoteInvocationException(e);
}
}
private void finishSampleTimer(String methodType, String methodName, Timer.Sample sample, HttpResponse response) {
if (isEnableMetric && response != null && response.getStatusLine() != null && sample != null) {
sample.stop(registry.timer(methodType, methodName,
String.valueOf(response.getStatusLine().getStatusCode())));
}
}
private Timer.Sample startSampleTimer() {
if (isEnableMetric && registry != null) {
return Timer.start(registry);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy