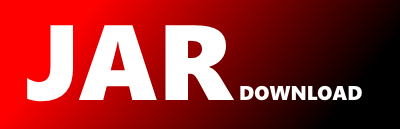
zio.aws.codecatalyst.model.EventLogEntry.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zio-aws-codecatalyst_3 Show documentation
Show all versions of zio-aws-codecatalyst_3 Show documentation
Low-level AWS wrapper for ZIO
The newest version!
package zio.aws.codecatalyst.model
import java.time.Instant
import zio.prelude.data.Optional
import zio.aws.core.{AwsError, BuilderHelper}
import zio.ZIO
import zio.aws.codecatalyst.model.primitives.SyntheticTimestamp_date_time
import scala.jdk.CollectionConverters.*
final case class EventLogEntry(
id: String,
eventName: String,
eventType: String,
eventCategory: String,
eventSource: String,
eventTime: SyntheticTimestamp_date_time,
operationType: zio.aws.codecatalyst.model.OperationType,
userIdentity: zio.aws.codecatalyst.model.UserIdentity,
projectInformation: Optional[
zio.aws.codecatalyst.model.ProjectInformation
] = Optional.Absent,
requestId: Optional[String] = Optional.Absent,
requestPayload: Optional[zio.aws.codecatalyst.model.EventPayload] =
Optional.Absent,
responsePayload: Optional[zio.aws.codecatalyst.model.EventPayload] =
Optional.Absent,
errorCode: Optional[String] = Optional.Absent,
sourceIpAddress: Optional[String] = Optional.Absent,
userAgent: Optional[String] = Optional.Absent
) {
def buildAwsValue()
: software.amazon.awssdk.services.codecatalyst.model.EventLogEntry = {
import EventLogEntry.zioAwsBuilderHelper.BuilderOps
software.amazon.awssdk.services.codecatalyst.model.EventLogEntry
.builder()
.id(id: java.lang.String)
.eventName(eventName: java.lang.String)
.eventType(eventType: java.lang.String)
.eventCategory(eventCategory: java.lang.String)
.eventSource(eventSource: java.lang.String)
.eventTime(SyntheticTimestamp_date_time.unwrap(eventTime): Instant)
.operationType(operationType.unwrap)
.userIdentity(userIdentity.buildAwsValue())
.optionallyWith(projectInformation.map(value => value.buildAwsValue()))(
_.projectInformation
)
.optionallyWith(requestId.map(value => value: java.lang.String))(
_.requestId
)
.optionallyWith(requestPayload.map(value => value.buildAwsValue()))(
_.requestPayload
)
.optionallyWith(responsePayload.map(value => value.buildAwsValue()))(
_.responsePayload
)
.optionallyWith(errorCode.map(value => value: java.lang.String))(
_.errorCode
)
.optionallyWith(sourceIpAddress.map(value => value: java.lang.String))(
_.sourceIpAddress
)
.optionallyWith(userAgent.map(value => value: java.lang.String))(
_.userAgent
)
.build()
}
def asReadOnly: zio.aws.codecatalyst.model.EventLogEntry.ReadOnly =
zio.aws.codecatalyst.model.EventLogEntry.wrap(buildAwsValue())
}
object EventLogEntry {
private lazy val zioAwsBuilderHelper: BuilderHelper[
software.amazon.awssdk.services.codecatalyst.model.EventLogEntry
] = BuilderHelper.apply
trait ReadOnly {
def asEditable: zio.aws.codecatalyst.model.EventLogEntry =
zio.aws.codecatalyst.model.EventLogEntry(
id,
eventName,
eventType,
eventCategory,
eventSource,
eventTime,
operationType,
userIdentity.asEditable,
projectInformation.map(value => value.asEditable),
requestId.map(value => value),
requestPayload.map(value => value.asEditable),
responsePayload.map(value => value.asEditable),
errorCode.map(value => value),
sourceIpAddress.map(value => value),
userAgent.map(value => value)
)
def id: String
def eventName: String
def eventType: String
def eventCategory: String
def eventSource: String
def eventTime: SyntheticTimestamp_date_time
def operationType: zio.aws.codecatalyst.model.OperationType
def userIdentity: zio.aws.codecatalyst.model.UserIdentity.ReadOnly
def projectInformation
: Optional[zio.aws.codecatalyst.model.ProjectInformation.ReadOnly]
def requestId: Optional[String]
def requestPayload
: Optional[zio.aws.codecatalyst.model.EventPayload.ReadOnly]
def responsePayload
: Optional[zio.aws.codecatalyst.model.EventPayload.ReadOnly]
def errorCode: Optional[String]
def sourceIpAddress: Optional[String]
def userAgent: Optional[String]
def getId: ZIO[Any, Nothing, String] = ZIO.succeed(id)
def getEventName: ZIO[Any, Nothing, String] = ZIO.succeed(eventName)
def getEventType: ZIO[Any, Nothing, String] = ZIO.succeed(eventType)
def getEventCategory: ZIO[Any, Nothing, String] = ZIO.succeed(eventCategory)
def getEventSource: ZIO[Any, Nothing, String] = ZIO.succeed(eventSource)
def getEventTime: ZIO[Any, Nothing, SyntheticTimestamp_date_time] =
ZIO.succeed(eventTime)
def getOperationType
: ZIO[Any, Nothing, zio.aws.codecatalyst.model.OperationType] =
ZIO.succeed(operationType)
def getUserIdentity
: ZIO[Any, Nothing, zio.aws.codecatalyst.model.UserIdentity.ReadOnly] =
ZIO.succeed(userIdentity)
def getProjectInformation: ZIO[
Any,
AwsError,
zio.aws.codecatalyst.model.ProjectInformation.ReadOnly
] = AwsError.unwrapOptionField("projectInformation", projectInformation)
def getRequestId: ZIO[Any, AwsError, String] =
AwsError.unwrapOptionField("requestId", requestId)
def getRequestPayload
: ZIO[Any, AwsError, zio.aws.codecatalyst.model.EventPayload.ReadOnly] =
AwsError.unwrapOptionField("requestPayload", requestPayload)
def getResponsePayload
: ZIO[Any, AwsError, zio.aws.codecatalyst.model.EventPayload.ReadOnly] =
AwsError.unwrapOptionField("responsePayload", responsePayload)
def getErrorCode: ZIO[Any, AwsError, String] =
AwsError.unwrapOptionField("errorCode", errorCode)
def getSourceIpAddress: ZIO[Any, AwsError, String] =
AwsError.unwrapOptionField("sourceIpAddress", sourceIpAddress)
def getUserAgent: ZIO[Any, AwsError, String] =
AwsError.unwrapOptionField("userAgent", userAgent)
}
private final class Wrapper(
impl: software.amazon.awssdk.services.codecatalyst.model.EventLogEntry
) extends zio.aws.codecatalyst.model.EventLogEntry.ReadOnly {
override val id: String = impl.id(): String
override val eventName: String = impl.eventName(): String
override val eventType: String = impl.eventType(): String
override val eventCategory: String = impl.eventCategory(): String
override val eventSource: String = impl.eventSource(): String
override val eventTime: SyntheticTimestamp_date_time =
zio.aws.codecatalyst.model.primitives
.SyntheticTimestamp_date_time(impl.eventTime())
override val operationType: zio.aws.codecatalyst.model.OperationType =
zio.aws.codecatalyst.model.OperationType.wrap(impl.operationType())
override val userIdentity
: zio.aws.codecatalyst.model.UserIdentity.ReadOnly =
zio.aws.codecatalyst.model.UserIdentity.wrap(impl.userIdentity())
override val projectInformation
: Optional[zio.aws.codecatalyst.model.ProjectInformation.ReadOnly] =
zio.aws.core.internal
.optionalFromNullable(impl.projectInformation())
.map(value => zio.aws.codecatalyst.model.ProjectInformation.wrap(value))
override val requestId: Optional[String] = zio.aws.core.internal
.optionalFromNullable(impl.requestId())
.map(value => value: String)
override val requestPayload
: Optional[zio.aws.codecatalyst.model.EventPayload.ReadOnly] =
zio.aws.core.internal
.optionalFromNullable(impl.requestPayload())
.map(value => zio.aws.codecatalyst.model.EventPayload.wrap(value))
override val responsePayload
: Optional[zio.aws.codecatalyst.model.EventPayload.ReadOnly] =
zio.aws.core.internal
.optionalFromNullable(impl.responsePayload())
.map(value => zio.aws.codecatalyst.model.EventPayload.wrap(value))
override val errorCode: Optional[String] = zio.aws.core.internal
.optionalFromNullable(impl.errorCode())
.map(value => value: String)
override val sourceIpAddress: Optional[String] = zio.aws.core.internal
.optionalFromNullable(impl.sourceIpAddress())
.map(value => value: String)
override val userAgent: Optional[String] = zio.aws.core.internal
.optionalFromNullable(impl.userAgent())
.map(value => value: String)
}
def wrap(
impl: software.amazon.awssdk.services.codecatalyst.model.EventLogEntry
): zio.aws.codecatalyst.model.EventLogEntry.ReadOnly = new Wrapper(impl)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy