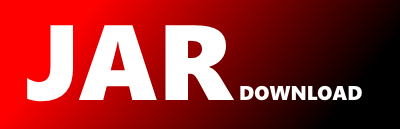
zio.aws.codeguruprofiler.model.AgentConfiguration.scala Maven / Gradle / Ivy
package zio.aws.codeguruprofiler.model
import zio.ZIO
import zio.aws.core.{AwsError, BuilderHelper}
import zio.prelude.data.Optional
import zio.aws.codeguruprofiler.model.primitives.Integer
import scala.jdk.CollectionConverters.*
final case class AgentConfiguration(
agentParameters: Optional[
Map[zio.aws.codeguruprofiler.model.AgentParameterField, String]
] = Optional.Absent,
periodInSeconds: Integer,
shouldProfile: Boolean
) {
def buildAwsValue()
: software.amazon.awssdk.services.codeguruprofiler.model.AgentConfiguration = {
import AgentConfiguration.zioAwsBuilderHelper.BuilderOps
software.amazon.awssdk.services.codeguruprofiler.model.AgentConfiguration
.builder()
.optionallyWith(
agentParameters.map(value =>
value
.map({ case (key, value) =>
key.unwrap.toString -> (value: java.lang.String)
})
.asJava
)
)(_.agentParametersWithStrings)
.periodInSeconds(periodInSeconds: java.lang.Integer)
.shouldProfile(shouldProfile: java.lang.Boolean)
.build()
}
def asReadOnly: zio.aws.codeguruprofiler.model.AgentConfiguration.ReadOnly =
zio.aws.codeguruprofiler.model.AgentConfiguration.wrap(buildAwsValue())
}
object AgentConfiguration {
private lazy val zioAwsBuilderHelper: BuilderHelper[
software.amazon.awssdk.services.codeguruprofiler.model.AgentConfiguration
] = BuilderHelper.apply
trait ReadOnly {
def asEditable: zio.aws.codeguruprofiler.model.AgentConfiguration =
zio.aws.codeguruprofiler.model.AgentConfiguration(
agentParameters.map(value => value),
periodInSeconds,
shouldProfile
)
def agentParameters: Optional[
Map[zio.aws.codeguruprofiler.model.AgentParameterField, String]
]
def periodInSeconds: Integer
def shouldProfile: Boolean
def getAgentParameters: ZIO[Any, AwsError, Map[
zio.aws.codeguruprofiler.model.AgentParameterField,
String
]] = AwsError.unwrapOptionField("agentParameters", agentParameters)
def getPeriodInSeconds: ZIO[Any, Nothing, Integer] =
ZIO.succeed(periodInSeconds)
def getShouldProfile: ZIO[Any, Nothing, Boolean] =
ZIO.succeed(shouldProfile)
}
private final class Wrapper(
impl: software.amazon.awssdk.services.codeguruprofiler.model.AgentConfiguration
) extends zio.aws.codeguruprofiler.model.AgentConfiguration.ReadOnly {
override val agentParameters: Optional[
Map[zio.aws.codeguruprofiler.model.AgentParameterField, String]
] = zio.aws.core.internal
.optionalFromNullable(impl.agentParameters())
.map(value =>
value.asScala
.map({ case (key, value) =>
zio.aws.codeguruprofiler.model.AgentParameterField
.wrap(key) -> (value: String)
})
.toMap
)
override val periodInSeconds: Integer = impl.periodInSeconds(): Integer
override val shouldProfile: Boolean = impl.shouldProfile(): Boolean
}
def wrap(
impl: software.amazon.awssdk.services.codeguruprofiler.model.AgentConfiguration
): zio.aws.codeguruprofiler.model.AgentConfiguration.ReadOnly = new Wrapper(
impl
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy