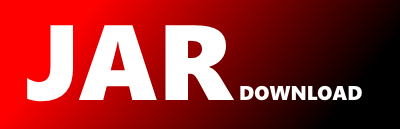
zio.aws.lexruntimev2.LexRuntimeV2.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zio-aws-lexruntimev2_2.13 Show documentation
Show all versions of zio-aws-lexruntimev2_2.13 Show documentation
Low-level AWS wrapper for ZIO
package zio.aws.lexruntimev2
import zio.aws.lexruntimev2.model.RecognizeTextResponse.ReadOnly
import zio.aws.core.config.AwsConfig
import software.amazon.awssdk.core.async.SdkPublisher
import software.amazon.awssdk.services.lexruntimev2.{
LexRuntimeV2AsyncClientBuilder,
LexRuntimeV2AsyncClient
}
import zio.aws.core.{AwsServiceBase, StreamingOutputResult, AwsError}
import zio.aws.core.aspects.{AwsCallAspect, AspectSupport}
import zio.aws.lexruntimev2.model.{
RecognizeUtteranceRequest,
ConfigurationEvent,
RecognizeTextRequest,
DeleteSessionRequest,
GetSessionRequest,
StartConversationRequest,
PutSessionRequest
}
import zio.{ZEnvironment, Task, IO, ZIO, ZLayer, Scope}
import software.amazon.awssdk.awscore.eventstream.EventStreamResponseHandler
import zio.stream.ZStream
import software.amazon.awssdk.services.lexruntimev2.model.{
RecognizeUtteranceResponse,
PlaybackInterruptionEvent,
RecognizeTextResponse,
StartConversationResponseEventStream,
StartConversationResponse,
DeleteSessionResponse,
StartConversationResponseHandler,
GetSessionResponse,
StartConversationRequestEventStream,
PutSessionResponse
}
import org.reactivestreams.Publisher
import scala.jdk.CollectionConverters._
trait LexRuntimeV2 extends AspectSupport[LexRuntimeV2] {
val api: LexRuntimeV2AsyncClient
def startConversation(
request: StartConversationRequest,
input: ZStream[Any, AwsError, ConfigurationEvent]
): ZStream[
Any,
AwsError,
zio.aws.lexruntimev2.model.PlaybackInterruptionEvent.ReadOnly
]
def recognizeUtterance(
request: RecognizeUtteranceRequest,
body: ZStream[Any, AwsError, Byte]
): IO[AwsError, StreamingOutputResult[
Any,
zio.aws.lexruntimev2.model.RecognizeUtteranceResponse.ReadOnly,
Byte
]]
def deleteSession(
request: DeleteSessionRequest
): IO[AwsError, zio.aws.lexruntimev2.model.DeleteSessionResponse.ReadOnly]
def recognizeText(request: RecognizeTextRequest): IO[AwsError, ReadOnly]
def putSession(
request: PutSessionRequest
): IO[AwsError, StreamingOutputResult[
Any,
zio.aws.lexruntimev2.model.PutSessionResponse.ReadOnly,
Byte
]]
def getSession(
request: GetSessionRequest
): IO[AwsError, zio.aws.lexruntimev2.model.GetSessionResponse.ReadOnly]
}
object LexRuntimeV2 {
val live: ZLayer[AwsConfig, java.lang.Throwable, LexRuntimeV2] = customized(
identity
)
def customized(
customization: LexRuntimeV2AsyncClientBuilder => LexRuntimeV2AsyncClientBuilder
): ZLayer[AwsConfig, java.lang.Throwable, LexRuntimeV2] =
ZLayer.scoped(scoped(customization))
def scoped(
customization: LexRuntimeV2AsyncClientBuilder => LexRuntimeV2AsyncClientBuilder
): ZIO[AwsConfig with Scope, java.lang.Throwable, LexRuntimeV2] = for (
awsConfig <- ZIO.service[AwsConfig]; executor <- ZIO.executor;
builder = LexRuntimeV2AsyncClient
.builder()
.asyncConfiguration(
software.amazon.awssdk.core.client.config.ClientAsyncConfiguration
.builder()
.advancedOption(
software.amazon.awssdk.core.client.config.SdkAdvancedAsyncClientOption.FUTURE_COMPLETION_EXECUTOR,
executor.asJava
)
.build()
);
b0 <- awsConfig
.configure[LexRuntimeV2AsyncClient, LexRuntimeV2AsyncClientBuilder](
builder
);
b1 <- awsConfig.configureHttpClient[
LexRuntimeV2AsyncClient,
LexRuntimeV2AsyncClientBuilder
](
b0,
zio.aws.core.httpclient.ServiceHttpCapabilities(supportsHttp2 = false)
); client <- ZIO.attempt(customization(b1).build())
)
yield new LexRuntimeV2Impl(
client,
AwsCallAspect.identity,
ZEnvironment.empty
)
private class LexRuntimeV2Impl[R](
override val api: LexRuntimeV2AsyncClient,
override val aspect: AwsCallAspect[R],
r: ZEnvironment[R]
) extends LexRuntimeV2
with AwsServiceBase[R] {
override val serviceName: String = "LexRuntimeV2"
override def withAspect[R1](
newAspect: AwsCallAspect[R1],
r: ZEnvironment[R1]
): LexRuntimeV2Impl[R1] = new LexRuntimeV2Impl(api, newAspect, r)
def startConversation(
request: StartConversationRequest,
input: ZStream[Any, AwsError, ConfigurationEvent]
): ZStream[
Any,
AwsError,
zio.aws.lexruntimev2.model.PlaybackInterruptionEvent.ReadOnly
] = asyncRequestEventInputOutputStream[
software.amazon.awssdk.services.lexruntimev2.model.StartConversationRequest,
StartConversationResponse,
StartConversationRequestEventStream,
StartConversationResponseHandler,
StartConversationResponseEventStream,
PlaybackInterruptionEvent
](
"StartConversation",
(
request: software.amazon.awssdk.services.lexruntimev2.model.StartConversationRequest,
input: Publisher[StartConversationRequestEventStream],
handler: StartConversationResponseHandler
) => api.startConversation(request, input, handler),
(impl: EventStreamResponseHandler[
StartConversationResponse,
StartConversationResponseEventStream
]) =>
new StartConversationResponseHandler {
override def responseReceived(
response: StartConversationResponse
): Unit = impl.responseReceived(response)
override def onEventStream(
publisher: SdkPublisher[StartConversationResponseEventStream]
): Unit = impl.onEventStream(publisher)
override def exceptionOccurred(throwable: java.lang.Throwable): Unit =
impl.exceptionOccurred(throwable)
override def complete(): Unit = impl.complete()
}
)(request.buildAwsValue(), input.map(_.buildAwsValue()))
.map(item =>
zio.aws.lexruntimev2.model.PlaybackInterruptionEvent.wrap(item)
)
.provideEnvironment(r)
def recognizeUtterance(
request: RecognizeUtteranceRequest,
body: ZStream[Any, AwsError, Byte]
): IO[AwsError, StreamingOutputResult[
Any,
zio.aws.lexruntimev2.model.RecognizeUtteranceResponse.ReadOnly,
Byte
]] = asyncRequestInputOutputStream[
software.amazon.awssdk.services.lexruntimev2.model.RecognizeUtteranceRequest,
RecognizeUtteranceResponse
](
"recognizeUtterance",
api.recognizeUtterance[Task[
StreamingOutputResult[R, RecognizeUtteranceResponse, Byte]
]],
AwsServiceBase.noContentLength[
software.amazon.awssdk.services.lexruntimev2.model.RecognizeUtteranceRequest
]
)(request.buildAwsValue(), body)
.map(
_.mapResponse(
zio.aws.lexruntimev2.model.RecognizeUtteranceResponse.wrap
).provideEnvironment(r)
)
.provideEnvironment(r)
def deleteSession(
request: DeleteSessionRequest
): IO[AwsError, zio.aws.lexruntimev2.model.DeleteSessionResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.lexruntimev2.model.DeleteSessionRequest,
DeleteSessionResponse
]("deleteSession", api.deleteSession)(request.buildAwsValue())
.map(zio.aws.lexruntimev2.model.DeleteSessionResponse.wrap)
.provideEnvironment(r)
def recognizeText(request: RecognizeTextRequest): IO[AwsError, ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.lexruntimev2.model.RecognizeTextRequest,
RecognizeTextResponse
]("recognizeText", api.recognizeText)(request.buildAwsValue())
.map(zio.aws.lexruntimev2.model.RecognizeTextResponse.wrap)
.provideEnvironment(r)
def putSession(
request: PutSessionRequest
): IO[AwsError, StreamingOutputResult[
Any,
zio.aws.lexruntimev2.model.PutSessionResponse.ReadOnly,
Byte
]] = asyncRequestOutputStream[
software.amazon.awssdk.services.lexruntimev2.model.PutSessionRequest,
PutSessionResponse
](
"putSession",
api.putSession[Task[StreamingOutputResult[R, PutSessionResponse, Byte]]]
)(request.buildAwsValue())
.map(
_.mapResponse(zio.aws.lexruntimev2.model.PutSessionResponse.wrap)
.provideEnvironment(r)
)
.provideEnvironment(r)
def getSession(
request: GetSessionRequest
): IO[AwsError, zio.aws.lexruntimev2.model.GetSessionResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.lexruntimev2.model.GetSessionRequest,
GetSessionResponse
]("getSession", api.getSession)(request.buildAwsValue())
.map(zio.aws.lexruntimev2.model.GetSessionResponse.wrap)
.provideEnvironment(r)
}
def startConversation(
request: StartConversationRequest,
input: ZStream[Any, AwsError, ConfigurationEvent]
): ZStream[
zio.aws.lexruntimev2.LexRuntimeV2,
AwsError,
zio.aws.lexruntimev2.model.PlaybackInterruptionEvent.ReadOnly
] = ZStream.serviceWithStream(_.startConversation(request, input))
def recognizeUtterance(
request: RecognizeUtteranceRequest,
body: ZStream[Any, AwsError, Byte]
): ZIO[zio.aws.lexruntimev2.LexRuntimeV2, AwsError, StreamingOutputResult[
Any,
zio.aws.lexruntimev2.model.RecognizeUtteranceResponse.ReadOnly,
Byte
]] = ZIO.serviceWithZIO(_.recognizeUtterance(request, body))
def deleteSession(request: DeleteSessionRequest): ZIO[
zio.aws.lexruntimev2.LexRuntimeV2,
AwsError,
zio.aws.lexruntimev2.model.DeleteSessionResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteSession(request))
def recognizeText(
request: RecognizeTextRequest
): ZIO[zio.aws.lexruntimev2.LexRuntimeV2, AwsError, ReadOnly] =
ZIO.serviceWithZIO(_.recognizeText(request))
def putSession(
request: PutSessionRequest
): ZIO[zio.aws.lexruntimev2.LexRuntimeV2, AwsError, StreamingOutputResult[
Any,
zio.aws.lexruntimev2.model.PutSessionResponse.ReadOnly,
Byte
]] = ZIO.serviceWithZIO(_.putSession(request))
def getSession(request: GetSessionRequest): ZIO[
zio.aws.lexruntimev2.LexRuntimeV2,
AwsError,
zio.aws.lexruntimev2.model.GetSessionResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getSession(request))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy