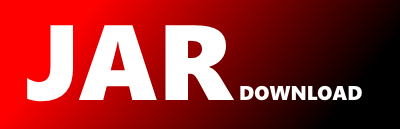
zio.aws.lexruntimev2.model.ImageResponseCard.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zio-aws-lexruntimev2_2.13 Show documentation
Show all versions of zio-aws-lexruntimev2_2.13 Show documentation
Low-level AWS wrapper for ZIO
The newest version!
package zio.aws.lexruntimev2.model
import zio.aws.lexruntimev2.model.primitives.{AttachmentUrl, AttachmentTitle}
import zio.ZIO
import zio.aws.core.{AwsError, BuilderHelper}
import zio.prelude.data.Optional
import scala.jdk.CollectionConverters._
final case class ImageResponseCard(
title: AttachmentTitle,
subtitle: Optional[AttachmentTitle] = Optional.Absent,
imageUrl: Optional[AttachmentUrl] = Optional.Absent,
buttons: Optional[Iterable[zio.aws.lexruntimev2.model.Button]] =
Optional.Absent
) {
def buildAwsValue()
: software.amazon.awssdk.services.lexruntimev2.model.ImageResponseCard = {
import ImageResponseCard.zioAwsBuilderHelper.BuilderOps
software.amazon.awssdk.services.lexruntimev2.model.ImageResponseCard
.builder()
.title(AttachmentTitle.unwrap(title): java.lang.String)
.optionallyWith(
subtitle.map(value => AttachmentTitle.unwrap(value): java.lang.String)
)(_.subtitle)
.optionallyWith(
imageUrl.map(value => AttachmentUrl.unwrap(value): java.lang.String)
)(_.imageUrl)
.optionallyWith(
buttons.map(value =>
value.map { item =>
item.buildAwsValue()
}.asJavaCollection
)
)(_.buttons)
.build()
}
def asReadOnly: zio.aws.lexruntimev2.model.ImageResponseCard.ReadOnly =
zio.aws.lexruntimev2.model.ImageResponseCard.wrap(buildAwsValue())
}
object ImageResponseCard {
private lazy val zioAwsBuilderHelper: BuilderHelper[
software.amazon.awssdk.services.lexruntimev2.model.ImageResponseCard
] = BuilderHelper.apply
trait ReadOnly {
def asEditable: zio.aws.lexruntimev2.model.ImageResponseCard =
zio.aws.lexruntimev2.model.ImageResponseCard(
title,
subtitle.map(value => value),
imageUrl.map(value => value),
buttons.map(value =>
value.map { item =>
item.asEditable
}
)
)
def title: AttachmentTitle
def subtitle: Optional[AttachmentTitle]
def imageUrl: Optional[AttachmentUrl]
def buttons: Optional[List[zio.aws.lexruntimev2.model.Button.ReadOnly]]
def getTitle: ZIO[Any, Nothing, AttachmentTitle] = ZIO.succeed(title)
def getSubtitle: ZIO[Any, AwsError, AttachmentTitle] =
AwsError.unwrapOptionField("subtitle", subtitle)
def getImageUrl: ZIO[Any, AwsError, AttachmentUrl] =
AwsError.unwrapOptionField("imageUrl", imageUrl)
def getButtons
: ZIO[Any, AwsError, List[zio.aws.lexruntimev2.model.Button.ReadOnly]] =
AwsError.unwrapOptionField("buttons", buttons)
}
private final class Wrapper(
impl: software.amazon.awssdk.services.lexruntimev2.model.ImageResponseCard
) extends zio.aws.lexruntimev2.model.ImageResponseCard.ReadOnly {
override val title: AttachmentTitle =
zio.aws.lexruntimev2.model.primitives.AttachmentTitle(impl.title())
override val subtitle: Optional[AttachmentTitle] = zio.aws.core.internal
.optionalFromNullable(impl.subtitle())
.map(value =>
zio.aws.lexruntimev2.model.primitives.AttachmentTitle(value)
)
override val imageUrl: Optional[AttachmentUrl] = zio.aws.core.internal
.optionalFromNullable(impl.imageUrl())
.map(value => zio.aws.lexruntimev2.model.primitives.AttachmentUrl(value))
override val buttons
: Optional[List[zio.aws.lexruntimev2.model.Button.ReadOnly]] =
zio.aws.core.internal
.optionalFromNullable(impl.buttons())
.map(value =>
value.asScala.map { item =>
zio.aws.lexruntimev2.model.Button.wrap(item)
}.toList
)
}
def wrap(
impl: software.amazon.awssdk.services.lexruntimev2.model.ImageResponseCard
): zio.aws.lexruntimev2.model.ImageResponseCard.ReadOnly = new Wrapper(impl)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy