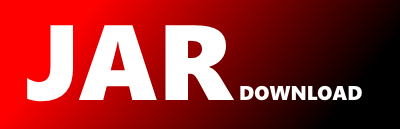
zio.aws.lexruntimev2.model.ActiveContext.scala Maven / Gradle / Ivy
package zio.aws.lexruntimev2.model
import zio.aws.lexruntimev2.model.primitives.{
Text,
ParameterName,
ActiveContextName
}
import zio.ZIO
import zio.aws.core.BuilderHelper
import scala.jdk.CollectionConverters.*
final case class ActiveContext(
name: ActiveContextName,
timeToLive: zio.aws.lexruntimev2.model.ActiveContextTimeToLive,
contextAttributes: Map[ParameterName, Text]
) {
def buildAwsValue()
: software.amazon.awssdk.services.lexruntimev2.model.ActiveContext = {
import ActiveContext.zioAwsBuilderHelper.BuilderOps
software.amazon.awssdk.services.lexruntimev2.model.ActiveContext
.builder()
.name(ActiveContextName.unwrap(name): java.lang.String)
.timeToLive(timeToLive.buildAwsValue())
.contextAttributes(
contextAttributes
.map({ case (key, value) =>
(ParameterName.unwrap(key): java.lang.String) -> (Text
.unwrap(value): java.lang.String)
})
.asJava
)
.build()
}
def asReadOnly: zio.aws.lexruntimev2.model.ActiveContext.ReadOnly =
zio.aws.lexruntimev2.model.ActiveContext.wrap(buildAwsValue())
}
object ActiveContext {
private lazy val zioAwsBuilderHelper: BuilderHelper[
software.amazon.awssdk.services.lexruntimev2.model.ActiveContext
] = BuilderHelper.apply
trait ReadOnly {
def asEditable: zio.aws.lexruntimev2.model.ActiveContext =
zio.aws.lexruntimev2.model
.ActiveContext(name, timeToLive.asEditable, contextAttributes)
def name: ActiveContextName
def timeToLive: zio.aws.lexruntimev2.model.ActiveContextTimeToLive.ReadOnly
def contextAttributes: Map[ParameterName, Text]
def getName: ZIO[Any, Nothing, ActiveContextName] = ZIO.succeed(name)
def getTimeToLive: ZIO[
Any,
Nothing,
zio.aws.lexruntimev2.model.ActiveContextTimeToLive.ReadOnly
] = ZIO.succeed(timeToLive)
def getContextAttributes: ZIO[Any, Nothing, Map[ParameterName, Text]] =
ZIO.succeed(contextAttributes)
}
private final class Wrapper(
impl: software.amazon.awssdk.services.lexruntimev2.model.ActiveContext
) extends zio.aws.lexruntimev2.model.ActiveContext.ReadOnly {
override val name: ActiveContextName =
zio.aws.lexruntimev2.model.primitives.ActiveContextName(impl.name())
override val timeToLive
: zio.aws.lexruntimev2.model.ActiveContextTimeToLive.ReadOnly =
zio.aws.lexruntimev2.model.ActiveContextTimeToLive.wrap(impl.timeToLive())
override val contextAttributes: Map[ParameterName, Text] = impl
.contextAttributes()
.asScala
.map({ case (key, value) =>
zio.aws.lexruntimev2.model.primitives.ParameterName(
key
) -> zio.aws.lexruntimev2.model.primitives.Text(value)
})
.toMap
}
def wrap(
impl: software.amazon.awssdk.services.lexruntimev2.model.ActiveContext
): zio.aws.lexruntimev2.model.ActiveContext.ReadOnly = new Wrapper(impl)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy