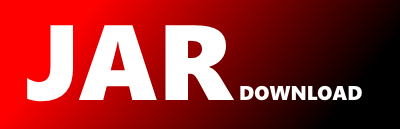
zio.aws.personalize.model.package.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zio-aws-personalize_3 Show documentation
Show all versions of zio-aws-personalize_3 Show documentation
Low-level AWS wrapper for ZIO
package zio.aws.personalize
import zio.prelude.Subtype
import zio.aws.personalize.model.primitives.{
MetricName,
CategoricalValue,
ParameterName,
ParameterValue,
Arn,
ColumnName,
DatasetType,
MetricValue,
TagKey
}
import java.time.Instant
import zio.aws.personalize.model.primitives.ContinuousMaxValue.Type
package object model {
import scala.jdk.CollectionConverters.*
object primitives {
object AccountId extends zio.prelude.Subtype[String]
type AccountId = zio.aws.personalize.model.primitives.AccountId.Type
object Arn extends zio.prelude.Subtype[String]
type Arn = zio.aws.personalize.model.primitives.Arn.Type
object AvroSchema extends zio.prelude.Subtype[String]
type AvroSchema = zio.aws.personalize.model.primitives.AvroSchema.Type
object CategoricalValue extends zio.prelude.Subtype[String]
type CategoricalValue =
zio.aws.personalize.model.primitives.CategoricalValue.Type
object ColumnName extends zio.prelude.Subtype[String]
type ColumnName = zio.aws.personalize.model.primitives.ColumnName.Type
object ContinuousMaxValue extends zio.prelude.Subtype[Double]
type ContinuousMaxValue =
zio.aws.personalize.model.primitives.ContinuousMaxValue.Type
object ContinuousMinValue extends zio.prelude.Subtype[Double]
type ContinuousMinValue =
zio.aws.personalize.model.primitives.ContinuousMinValue.Type
object DatasetType extends zio.prelude.Subtype[String]
type DatasetType = zio.aws.personalize.model.primitives.DatasetType.Type
object Date extends zio.prelude.Subtype[Instant]
type Date = zio.aws.personalize.model.primitives.Date.Type
object Description extends zio.prelude.Subtype[String]
type Description = zio.aws.personalize.model.primitives.Description.Type
object DockerURI extends zio.prelude.Subtype[String]
type DockerURI = zio.aws.personalize.model.primitives.DockerURI.Type
object EventType extends zio.prelude.Subtype[String]
type EventType = zio.aws.personalize.model.primitives.EventType.Type
object EventValueThreshold extends zio.prelude.Subtype[String]
type EventValueThreshold =
zio.aws.personalize.model.primitives.EventValueThreshold.Type
object FailureReason extends zio.prelude.Subtype[String]
type FailureReason = zio.aws.personalize.model.primitives.FailureReason.Type
object FilterExpression extends zio.prelude.Subtype[String]
type FilterExpression =
zio.aws.personalize.model.primitives.FilterExpression.Type
object HPOObjectiveType extends zio.prelude.Subtype[String]
type HPOObjectiveType =
zio.aws.personalize.model.primitives.HPOObjectiveType.Type
object HPOResource extends zio.prelude.Subtype[String]
type HPOResource = zio.aws.personalize.model.primitives.HPOResource.Type
type Integer = Int
object IntegerMaxValue extends zio.prelude.Subtype[Int]
type IntegerMaxValue =
zio.aws.personalize.model.primitives.IntegerMaxValue.Type
object IntegerMinValue extends zio.prelude.Subtype[Int]
type IntegerMinValue =
zio.aws.personalize.model.primitives.IntegerMinValue.Type
object ItemAttribute extends zio.prelude.Subtype[String]
type ItemAttribute = zio.aws.personalize.model.primitives.ItemAttribute.Type
object KmsKeyArn extends zio.prelude.Subtype[String]
type KmsKeyArn = zio.aws.personalize.model.primitives.KmsKeyArn.Type
object MaxResults extends zio.prelude.Subtype[Int]
type MaxResults = zio.aws.personalize.model.primitives.MaxResults.Type
object MetricExpression extends zio.prelude.Subtype[String]
type MetricExpression =
zio.aws.personalize.model.primitives.MetricExpression.Type
object MetricName extends zio.prelude.Subtype[String]
type MetricName = zio.aws.personalize.model.primitives.MetricName.Type
object MetricRegex extends zio.prelude.Subtype[String]
type MetricRegex = zio.aws.personalize.model.primitives.MetricRegex.Type
object MetricValue extends zio.prelude.Subtype[Double]
type MetricValue = zio.aws.personalize.model.primitives.MetricValue.Type
object Name extends zio.prelude.Subtype[String]
type Name = zio.aws.personalize.model.primitives.Name.Type
object NextToken extends zio.prelude.Subtype[String]
type NextToken = zio.aws.personalize.model.primitives.NextToken.Type
object NumBatchResults extends zio.prelude.Subtype[Int]
type NumBatchResults =
zio.aws.personalize.model.primitives.NumBatchResults.Type
object ParameterName extends zio.prelude.Subtype[String]
type ParameterName = zio.aws.personalize.model.primitives.ParameterName.Type
object ParameterValue extends zio.prelude.Subtype[String]
type ParameterValue =
zio.aws.personalize.model.primitives.ParameterValue.Type
object PerformAutoML extends zio.prelude.Subtype[Boolean]
type PerformAutoML = zio.aws.personalize.model.primitives.PerformAutoML.Type
object PerformAutoTraining extends zio.prelude.Subtype[Boolean]
type PerformAutoTraining =
zio.aws.personalize.model.primitives.PerformAutoTraining.Type
object PerformHPO extends zio.prelude.Subtype[Boolean]
type PerformHPO = zio.aws.personalize.model.primitives.PerformHPO.Type
object RecipeType extends zio.prelude.Subtype[String]
type RecipeType = zio.aws.personalize.model.primitives.RecipeType.Type
object RoleArn extends zio.prelude.Subtype[String]
type RoleArn = zio.aws.personalize.model.primitives.RoleArn.Type
object S3Location extends zio.prelude.Subtype[String]
type S3Location = zio.aws.personalize.model.primitives.S3Location.Type
object SchedulingExpression extends zio.prelude.Subtype[String]
type SchedulingExpression =
zio.aws.personalize.model.primitives.SchedulingExpression.Type
object Status extends zio.prelude.Subtype[String]
type Status = zio.aws.personalize.model.primitives.Status.Type
object TagKey extends zio.prelude.Subtype[String]
type TagKey = zio.aws.personalize.model.primitives.TagKey.Type
object TagValue extends zio.prelude.Subtype[String]
type TagValue = zio.aws.personalize.model.primitives.TagValue.Type
object TrackingId extends zio.prelude.Subtype[String]
type TrackingId = zio.aws.personalize.model.primitives.TrackingId.Type
object TrainingHours extends zio.prelude.Subtype[Double]
type TrainingHours = zio.aws.personalize.model.primitives.TrainingHours.Type
object TrainingInputMode extends zio.prelude.Subtype[String]
type TrainingInputMode =
zio.aws.personalize.model.primitives.TrainingInputMode.Type
object TransactionsPerSecond extends zio.prelude.Subtype[Int]
type TransactionsPerSecond =
zio.aws.personalize.model.primitives.TransactionsPerSecond.Type
object Tunable extends zio.prelude.Subtype[Boolean]
type Tunable = zio.aws.personalize.model.primitives.Tunable.Type
}
type ArnList = List[Arn]
type BatchInferenceJobs =
List[zio.aws.personalize.model.BatchInferenceJobSummary]
type BatchSegmentJobs = List[zio.aws.personalize.model.BatchSegmentJobSummary]
type Campaigns = List[zio.aws.personalize.model.CampaignSummary]
type CategoricalHyperParameterRanges =
List[zio.aws.personalize.model.CategoricalHyperParameterRange]
type CategoricalValues = List[CategoricalValue]
type ColumnNamesList = List[ColumnName]
type ContinuousHyperParameterRanges =
List[zio.aws.personalize.model.ContinuousHyperParameterRange]
type DataDeletionJobs = List[zio.aws.personalize.model.DataDeletionJobSummary]
type DatasetExportJobs =
List[zio.aws.personalize.model.DatasetExportJobSummary]
type DatasetGroups = List[zio.aws.personalize.model.DatasetGroupSummary]
type DatasetImportJobs =
List[zio.aws.personalize.model.DatasetImportJobSummary]
type Datasets = List[zio.aws.personalize.model.DatasetSummary]
type DefaultCategoricalHyperParameterRanges =
List[zio.aws.personalize.model.DefaultCategoricalHyperParameterRange]
type DefaultContinuousHyperParameterRanges =
List[zio.aws.personalize.model.DefaultContinuousHyperParameterRange]
type DefaultIntegerHyperParameterRanges =
List[zio.aws.personalize.model.DefaultIntegerHyperParameterRange]
type EventTrackers = List[zio.aws.personalize.model.EventTrackerSummary]
type ExcludedDatasetColumns = Map[DatasetType, Iterable[ColumnName]]
type FeatureTransformationParameters = Map[ParameterName, ParameterValue]
type FeaturizationParameters = Map[ParameterName, ParameterValue]
type Filters = List[zio.aws.personalize.model.FilterSummary]
type HyperParameters = Map[ParameterName, ParameterValue]
type IntegerHyperParameterRanges =
List[zio.aws.personalize.model.IntegerHyperParameterRange]
type MetricAttributes = List[zio.aws.personalize.model.MetricAttribute]
type MetricAttributesNamesList = List[MetricName]
type MetricAttributions =
List[zio.aws.personalize.model.MetricAttributionSummary]
type Metrics = Map[MetricName, MetricValue]
type Recipes = List[zio.aws.personalize.model.RecipeSummary]
type Recommenders = List[zio.aws.personalize.model.RecommenderSummary]
type ResourceConfig = Map[ParameterName, ParameterValue]
type Schemas = List[zio.aws.personalize.model.DatasetSchemaSummary]
type SolutionVersions = List[zio.aws.personalize.model.SolutionVersionSummary]
type Solutions = List[zio.aws.personalize.model.SolutionSummary]
type TagKeys = List[TagKey]
type Tags = List[zio.aws.personalize.model.Tag]
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy