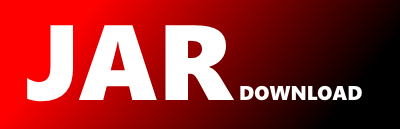
zio.aws.waf.Waf.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zio-aws-waf_2.13 Show documentation
Show all versions of zio-aws-waf_2.13 Show documentation
Low-level AWS wrapper for ZIO
package zio.aws.waf
import zio.aws.waf.model.UpdateRegexMatchSetResponse.ReadOnly
import zio.aws.waf.model.{
DeleteRateBasedRuleRequest,
GetRateBasedRuleManagedKeysRequest,
UpdateWebAclRequest,
GetRuleGroupRequest,
GetLoggingConfigurationRequest,
UpdateByteMatchSetRequest,
DeleteWebAclRequest,
UpdateRateBasedRuleRequest,
DeleteRuleGroupRequest,
GetIpSetRequest,
GetSizeConstraintSetRequest,
GetPermissionPolicyRequest,
DeleteSqlInjectionMatchSetRequest,
UpdateIpSetRequest,
GetXssMatchSetRequest,
CreateByteMatchSetRequest,
CreateXssMatchSetRequest,
CreateWebAclMigrationStackRequest,
ListRulesRequest,
GetRuleRequest,
PutPermissionPolicyRequest,
GetChangeTokenRequest,
UpdateSqlInjectionMatchSetRequest,
DeleteRegexMatchSetRequest,
ListTagsForResourceRequest,
GetRegexPatternSetRequest,
ListSizeConstraintSetsRequest,
ListRuleGroupsRequest,
PutLoggingConfigurationRequest,
ListLoggingConfigurationsRequest,
ListActivatedRulesInRuleGroupRequest,
DeleteIpSetRequest,
GetRegexMatchSetRequest,
ListRegexMatchSetsRequest,
DeletePermissionPolicyRequest,
CreateGeoMatchSetRequest,
CreateRuleRequest,
GetGeoMatchSetRequest,
GetWebAclRequest,
DeleteLoggingConfigurationRequest,
UpdateGeoMatchSetRequest,
DeleteXssMatchSetRequest,
GetSqlInjectionMatchSetRequest,
ListSubscribedRuleGroupsRequest,
DeleteRuleRequest,
ListGeoMatchSetsRequest,
GetRateBasedRuleRequest,
DeleteRegexPatternSetRequest,
CreateRegexPatternSetRequest,
GetSampledRequestsRequest,
DeleteByteMatchSetRequest,
UpdateRuleGroupRequest,
UntagResourceRequest,
CreateRuleGroupRequest,
GetByteMatchSetRequest,
CreateSqlInjectionMatchSetRequest,
CreateRateBasedRuleRequest,
DeleteSizeConstraintSetRequest,
CreateRegexMatchSetRequest,
ListXssMatchSetsRequest,
UpdateRuleRequest,
UpdateRegexMatchSetRequest,
DeleteGeoMatchSetRequest,
CreateSizeConstraintSetRequest,
GetChangeTokenStatusRequest,
ListRateBasedRulesRequest,
ListByteMatchSetsRequest,
UpdateXssMatchSetRequest,
UpdateRegexPatternSetRequest,
UpdateSizeConstraintSetRequest,
ListSqlInjectionMatchSetsRequest,
TagResourceRequest,
ListWebAcLsRequest,
CreateWebAclRequest,
ListRegexPatternSetsRequest,
ListIpSetsRequest,
CreateIpSetRequest
}
import zio.aws.core.config.AwsConfig
import software.amazon.awssdk.services.waf.{
WafAsyncClientBuilder,
WafAsyncClient
}
import software.amazon.awssdk.services.waf.model.{
GetSizeConstraintSetResponse,
GetRegexPatternSetResponse,
CreateSqlInjectionMatchSetResponse,
PutPermissionPolicyResponse,
GetChangeTokenStatusResponse,
UpdateIpSetResponse,
ListSqlInjectionMatchSetsResponse,
UpdateSqlInjectionMatchSetResponse,
GetRateBasedRuleResponse,
DeleteLoggingConfigurationResponse,
DeleteXssMatchSetResponse,
ListRulesResponse,
DeleteRuleGroupResponse,
DeleteRateBasedRuleResponse,
CreateSizeConstraintSetResponse,
UpdateRateBasedRuleResponse,
CreateRegexPatternSetResponse,
DeletePermissionPolicyResponse,
GetIpSetResponse,
ListIpSetsResponse,
ListRegexMatchSetsResponse,
CreateIpSetResponse,
DeleteWebAclResponse,
ListRateBasedRulesResponse,
UpdateSizeConstraintSetResponse,
UpdateRuleResponse,
CreateRuleGroupResponse,
GetPermissionPolicyResponse,
DeleteByteMatchSetResponse,
CreateGeoMatchSetResponse,
GetRuleResponse,
GetRateBasedRuleManagedKeysResponse,
CreateWebAclResponse,
UpdateByteMatchSetResponse,
ListSizeConstraintSetsResponse,
CreateWebAclMigrationStackResponse,
ListTagsForResourceResponse,
ListGeoMatchSetsResponse,
UntagResourceResponse,
UpdateXssMatchSetResponse,
CreateXssMatchSetResponse,
ListLoggingConfigurationsResponse,
DeleteIpSetResponse,
UpdateRegexPatternSetResponse,
ListSubscribedRuleGroupsResponse,
GetSqlInjectionMatchSetResponse,
CreateRegexMatchSetResponse,
UpdateGeoMatchSetResponse,
GetXssMatchSetResponse,
GetGeoMatchSetResponse,
GetByteMatchSetResponse,
UpdateWebAclResponse,
ListWebAcLsResponse,
GetWebAclResponse,
GetLoggingConfigurationResponse,
GetSampledRequestsResponse,
ListXssMatchSetsResponse,
GetChangeTokenResponse,
CreateRuleResponse,
ListRegexPatternSetsResponse,
UpdateRegexMatchSetResponse,
GetRuleGroupResponse,
ListActivatedRulesInRuleGroupResponse,
DeleteRegexPatternSetResponse,
CreateByteMatchSetResponse,
PutLoggingConfigurationResponse,
DeleteSizeConstraintSetResponse,
TagResourceResponse,
GetRegexMatchSetResponse,
UpdateRuleGroupResponse,
CreateRateBasedRuleResponse,
DeleteRegexMatchSetResponse,
ListRuleGroupsResponse,
DeleteRuleResponse,
DeleteSqlInjectionMatchSetResponse,
DeleteGeoMatchSetResponse,
ListByteMatchSetsResponse
}
import zio.aws.core.{AwsServiceBase, AwsError}
import zio.aws.core.aspects.{AwsCallAspect, AspectSupport}
import zio.{ZEnvironment, IO, ZIO, ZLayer, Scope}
import scala.jdk.CollectionConverters._
trait Waf extends AspectSupport[Waf] {
val api: WafAsyncClient
def listXssMatchSets(
request: ListXssMatchSetsRequest
): IO[AwsError, zio.aws.waf.model.ListXssMatchSetsResponse.ReadOnly]
def getRuleGroup(
request: GetRuleGroupRequest
): IO[AwsError, zio.aws.waf.model.GetRuleGroupResponse.ReadOnly]
def listGeoMatchSets(
request: ListGeoMatchSetsRequest
): IO[AwsError, zio.aws.waf.model.ListGeoMatchSetsResponse.ReadOnly]
def updateByteMatchSet(
request: UpdateByteMatchSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateByteMatchSetResponse.ReadOnly]
def updateRegexMatchSet(
request: UpdateRegexMatchSetRequest
): IO[AwsError, ReadOnly]
def createRegexMatchSet(
request: CreateRegexMatchSetRequest
): IO[AwsError, zio.aws.waf.model.CreateRegexMatchSetResponse.ReadOnly]
def getWebACL(
request: GetWebAclRequest
): IO[AwsError, zio.aws.waf.model.GetWebAclResponse.ReadOnly]
def getRateBasedRule(
request: GetRateBasedRuleRequest
): IO[AwsError, zio.aws.waf.model.GetRateBasedRuleResponse.ReadOnly]
def listSizeConstraintSets(
request: ListSizeConstraintSetsRequest
): IO[AwsError, zio.aws.waf.model.ListSizeConstraintSetsResponse.ReadOnly]
def listRegexMatchSets(
request: ListRegexMatchSetsRequest
): IO[AwsError, zio.aws.waf.model.ListRegexMatchSetsResponse.ReadOnly]
def getRegexMatchSet(
request: GetRegexMatchSetRequest
): IO[AwsError, zio.aws.waf.model.GetRegexMatchSetResponse.ReadOnly]
def listSubscribedRuleGroups(
request: ListSubscribedRuleGroupsRequest
): IO[AwsError, zio.aws.waf.model.ListSubscribedRuleGroupsResponse.ReadOnly]
def getSqlInjectionMatchSet(
request: GetSqlInjectionMatchSetRequest
): IO[AwsError, zio.aws.waf.model.GetSqlInjectionMatchSetResponse.ReadOnly]
def getIPSet(
request: GetIpSetRequest
): IO[AwsError, zio.aws.waf.model.GetIpSetResponse.ReadOnly]
def createRuleGroup(
request: CreateRuleGroupRequest
): IO[AwsError, zio.aws.waf.model.CreateRuleGroupResponse.ReadOnly]
def listRuleGroups(
request: ListRuleGroupsRequest
): IO[AwsError, zio.aws.waf.model.ListRuleGroupsResponse.ReadOnly]
def deleteRegexPatternSet(
request: DeleteRegexPatternSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteRegexPatternSetResponse.ReadOnly]
def updateRateBasedRule(
request: UpdateRateBasedRuleRequest
): IO[AwsError, zio.aws.waf.model.UpdateRateBasedRuleResponse.ReadOnly]
def updateSqlInjectionMatchSet(
request: UpdateSqlInjectionMatchSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateSqlInjectionMatchSetResponse.ReadOnly]
def deleteGeoMatchSet(
request: DeleteGeoMatchSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteGeoMatchSetResponse.ReadOnly]
def createIPSet(
request: CreateIpSetRequest
): IO[AwsError, zio.aws.waf.model.CreateIpSetResponse.ReadOnly]
def getRateBasedRuleManagedKeys(
request: GetRateBasedRuleManagedKeysRequest
): IO[
AwsError,
zio.aws.waf.model.GetRateBasedRuleManagedKeysResponse.ReadOnly
]
def updateGeoMatchSet(
request: UpdateGeoMatchSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateGeoMatchSetResponse.ReadOnly]
def listRegexPatternSets(
request: ListRegexPatternSetsRequest
): IO[AwsError, zio.aws.waf.model.ListRegexPatternSetsResponse.ReadOnly]
def listByteMatchSets(
request: ListByteMatchSetsRequest
): IO[AwsError, zio.aws.waf.model.ListByteMatchSetsResponse.ReadOnly]
def updateIPSet(
request: UpdateIpSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateIpSetResponse.ReadOnly]
def createRateBasedRule(
request: CreateRateBasedRuleRequest
): IO[AwsError, zio.aws.waf.model.CreateRateBasedRuleResponse.ReadOnly]
def deleteXssMatchSet(
request: DeleteXssMatchSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteXssMatchSetResponse.ReadOnly]
def getByteMatchSet(
request: GetByteMatchSetRequest
): IO[AwsError, zio.aws.waf.model.GetByteMatchSetResponse.ReadOnly]
def deleteRule(
request: DeleteRuleRequest
): IO[AwsError, zio.aws.waf.model.DeleteRuleResponse.ReadOnly]
def getSizeConstraintSet(
request: GetSizeConstraintSetRequest
): IO[AwsError, zio.aws.waf.model.GetSizeConstraintSetResponse.ReadOnly]
def createByteMatchSet(
request: CreateByteMatchSetRequest
): IO[AwsError, zio.aws.waf.model.CreateByteMatchSetResponse.ReadOnly]
def updateRule(
request: UpdateRuleRequest
): IO[AwsError, zio.aws.waf.model.UpdateRuleResponse.ReadOnly]
def getRegexPatternSet(
request: GetRegexPatternSetRequest
): IO[AwsError, zio.aws.waf.model.GetRegexPatternSetResponse.ReadOnly]
def untagResource(
request: UntagResourceRequest
): IO[AwsError, zio.aws.waf.model.UntagResourceResponse.ReadOnly]
def deleteRateBasedRule(
request: DeleteRateBasedRuleRequest
): IO[AwsError, zio.aws.waf.model.DeleteRateBasedRuleResponse.ReadOnly]
def putLoggingConfiguration(
request: PutLoggingConfigurationRequest
): IO[AwsError, zio.aws.waf.model.PutLoggingConfigurationResponse.ReadOnly]
def getXssMatchSet(
request: GetXssMatchSetRequest
): IO[AwsError, zio.aws.waf.model.GetXssMatchSetResponse.ReadOnly]
def deleteWebACL(
request: DeleteWebAclRequest
): IO[AwsError, zio.aws.waf.model.DeleteWebAclResponse.ReadOnly]
def createRegexPatternSet(
request: CreateRegexPatternSetRequest
): IO[AwsError, zio.aws.waf.model.CreateRegexPatternSetResponse.ReadOnly]
def listWebACLs(
request: ListWebAcLsRequest
): IO[AwsError, zio.aws.waf.model.ListWebAcLsResponse.ReadOnly]
def putPermissionPolicy(
request: PutPermissionPolicyRequest
): IO[AwsError, zio.aws.waf.model.PutPermissionPolicyResponse.ReadOnly]
def createXssMatchSet(
request: CreateXssMatchSetRequest
): IO[AwsError, zio.aws.waf.model.CreateXssMatchSetResponse.ReadOnly]
def getChangeTokenStatus(
request: GetChangeTokenStatusRequest
): IO[AwsError, zio.aws.waf.model.GetChangeTokenStatusResponse.ReadOnly]
def createSizeConstraintSet(
request: CreateSizeConstraintSetRequest
): IO[AwsError, zio.aws.waf.model.CreateSizeConstraintSetResponse.ReadOnly]
def getLoggingConfiguration(
request: GetLoggingConfigurationRequest
): IO[AwsError, zio.aws.waf.model.GetLoggingConfigurationResponse.ReadOnly]
def listTagsForResource(
request: ListTagsForResourceRequest
): IO[AwsError, zio.aws.waf.model.ListTagsForResourceResponse.ReadOnly]
def tagResource(
request: TagResourceRequest
): IO[AwsError, zio.aws.waf.model.TagResourceResponse.ReadOnly]
def listRules(
request: ListRulesRequest
): IO[AwsError, zio.aws.waf.model.ListRulesResponse.ReadOnly]
def listLoggingConfigurations(
request: ListLoggingConfigurationsRequest
): IO[AwsError, zio.aws.waf.model.ListLoggingConfigurationsResponse.ReadOnly]
def updateXssMatchSet(
request: UpdateXssMatchSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateXssMatchSetResponse.ReadOnly]
def deleteLoggingConfiguration(
request: DeleteLoggingConfigurationRequest
): IO[AwsError, zio.aws.waf.model.DeleteLoggingConfigurationResponse.ReadOnly]
def getGeoMatchSet(
request: GetGeoMatchSetRequest
): IO[AwsError, zio.aws.waf.model.GetGeoMatchSetResponse.ReadOnly]
def updateSizeConstraintSet(
request: UpdateSizeConstraintSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateSizeConstraintSetResponse.ReadOnly]
def listRateBasedRules(
request: ListRateBasedRulesRequest
): IO[AwsError, zio.aws.waf.model.ListRateBasedRulesResponse.ReadOnly]
def updateRuleGroup(
request: UpdateRuleGroupRequest
): IO[AwsError, zio.aws.waf.model.UpdateRuleGroupResponse.ReadOnly]
def updateRegexPatternSet(
request: UpdateRegexPatternSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateRegexPatternSetResponse.ReadOnly]
def createWebACL(
request: CreateWebAclRequest
): IO[AwsError, zio.aws.waf.model.CreateWebAclResponse.ReadOnly]
def createGeoMatchSet(
request: CreateGeoMatchSetRequest
): IO[AwsError, zio.aws.waf.model.CreateGeoMatchSetResponse.ReadOnly]
def getPermissionPolicy(
request: GetPermissionPolicyRequest
): IO[AwsError, zio.aws.waf.model.GetPermissionPolicyResponse.ReadOnly]
def updateWebACL(
request: UpdateWebAclRequest
): IO[AwsError, zio.aws.waf.model.UpdateWebAclResponse.ReadOnly]
def deleteRuleGroup(
request: DeleteRuleGroupRequest
): IO[AwsError, zio.aws.waf.model.DeleteRuleGroupResponse.ReadOnly]
def deleteByteMatchSet(
request: DeleteByteMatchSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteByteMatchSetResponse.ReadOnly]
def getChangeToken(
request: GetChangeTokenRequest
): IO[AwsError, zio.aws.waf.model.GetChangeTokenResponse.ReadOnly]
def deleteRegexMatchSet(
request: DeleteRegexMatchSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteRegexMatchSetResponse.ReadOnly]
def listIPSets(
request: ListIpSetsRequest
): IO[AwsError, zio.aws.waf.model.ListIpSetsResponse.ReadOnly]
def deleteSizeConstraintSet(
request: DeleteSizeConstraintSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteSizeConstraintSetResponse.ReadOnly]
def getSampledRequests(
request: GetSampledRequestsRequest
): IO[AwsError, zio.aws.waf.model.GetSampledRequestsResponse.ReadOnly]
def listActivatedRulesInRuleGroup(
request: ListActivatedRulesInRuleGroupRequest
): IO[
AwsError,
zio.aws.waf.model.ListActivatedRulesInRuleGroupResponse.ReadOnly
]
def deleteIPSet(
request: DeleteIpSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteIpSetResponse.ReadOnly]
def deletePermissionPolicy(
request: DeletePermissionPolicyRequest
): IO[AwsError, zio.aws.waf.model.DeletePermissionPolicyResponse.ReadOnly]
def deleteSqlInjectionMatchSet(
request: DeleteSqlInjectionMatchSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteSqlInjectionMatchSetResponse.ReadOnly]
def listSqlInjectionMatchSets(
request: ListSqlInjectionMatchSetsRequest
): IO[AwsError, zio.aws.waf.model.ListSqlInjectionMatchSetsResponse.ReadOnly]
def getRule(
request: GetRuleRequest
): IO[AwsError, zio.aws.waf.model.GetRuleResponse.ReadOnly]
def createWebACLMigrationStack(
request: CreateWebAclMigrationStackRequest
): IO[AwsError, zio.aws.waf.model.CreateWebAclMigrationStackResponse.ReadOnly]
def createSqlInjectionMatchSet(
request: CreateSqlInjectionMatchSetRequest
): IO[AwsError, zio.aws.waf.model.CreateSqlInjectionMatchSetResponse.ReadOnly]
def createRule(
request: CreateRuleRequest
): IO[AwsError, zio.aws.waf.model.CreateRuleResponse.ReadOnly]
}
object Waf {
val live: ZLayer[AwsConfig, java.lang.Throwable, Waf] = customized(identity)
def customized(
customization: WafAsyncClientBuilder => WafAsyncClientBuilder
): ZLayer[AwsConfig, java.lang.Throwable, Waf] =
ZLayer.scoped(scoped(customization))
def scoped(
customization: WafAsyncClientBuilder => WafAsyncClientBuilder
): ZIO[AwsConfig with Scope, java.lang.Throwable, Waf] = for (
awsConfig <- ZIO.service[AwsConfig]; executor <- ZIO.executor;
builder = WafAsyncClient
.builder()
.asyncConfiguration(
software.amazon.awssdk.core.client.config.ClientAsyncConfiguration
.builder()
.advancedOption(
software.amazon.awssdk.core.client.config.SdkAdvancedAsyncClientOption.FUTURE_COMPLETION_EXECUTOR,
executor.asJava
)
.build()
);
b0 <- awsConfig.configure[WafAsyncClient, WafAsyncClientBuilder](builder);
b1 <- awsConfig.configureHttpClient[WafAsyncClient, WafAsyncClientBuilder](
b0,
zio.aws.core.httpclient.ServiceHttpCapabilities(supportsHttp2 = false)
);
client <- ZIO.attempt(
customization(
b1.region(_root_.software.amazon.awssdk.regions.Region.AWS_GLOBAL)
).build()
)
) yield new WafImpl(client, AwsCallAspect.identity, ZEnvironment.empty)
private class WafImpl[R](
override val api: WafAsyncClient,
override val aspect: AwsCallAspect[R],
r: ZEnvironment[R]
) extends Waf
with AwsServiceBase[R] {
override val serviceName: String = "Waf"
override def withAspect[R1](
newAspect: AwsCallAspect[R1],
r: ZEnvironment[R1]
): WafImpl[R1] = new WafImpl(api, newAspect, r)
def listXssMatchSets(
request: ListXssMatchSetsRequest
): IO[AwsError, zio.aws.waf.model.ListXssMatchSetsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListXssMatchSetsRequest,
ListXssMatchSetsResponse
]("listXssMatchSets", api.listXssMatchSets)(request.buildAwsValue())
.map(zio.aws.waf.model.ListXssMatchSetsResponse.wrap)
.provideEnvironment(r)
def getRuleGroup(
request: GetRuleGroupRequest
): IO[AwsError, zio.aws.waf.model.GetRuleGroupResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetRuleGroupRequest,
GetRuleGroupResponse
]("getRuleGroup", api.getRuleGroup)(request.buildAwsValue())
.map(zio.aws.waf.model.GetRuleGroupResponse.wrap)
.provideEnvironment(r)
def listGeoMatchSets(
request: ListGeoMatchSetsRequest
): IO[AwsError, zio.aws.waf.model.ListGeoMatchSetsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListGeoMatchSetsRequest,
ListGeoMatchSetsResponse
]("listGeoMatchSets", api.listGeoMatchSets)(request.buildAwsValue())
.map(zio.aws.waf.model.ListGeoMatchSetsResponse.wrap)
.provideEnvironment(r)
def updateByteMatchSet(
request: UpdateByteMatchSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateByteMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateByteMatchSetRequest,
UpdateByteMatchSetResponse
]("updateByteMatchSet", api.updateByteMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.UpdateByteMatchSetResponse.wrap)
.provideEnvironment(r)
def updateRegexMatchSet(
request: UpdateRegexMatchSetRequest
): IO[AwsError, ReadOnly] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateRegexMatchSetRequest,
UpdateRegexMatchSetResponse
]("updateRegexMatchSet", api.updateRegexMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.UpdateRegexMatchSetResponse.wrap)
.provideEnvironment(r)
def createRegexMatchSet(
request: CreateRegexMatchSetRequest
): IO[AwsError, zio.aws.waf.model.CreateRegexMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateRegexMatchSetRequest,
CreateRegexMatchSetResponse
]("createRegexMatchSet", api.createRegexMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.CreateRegexMatchSetResponse.wrap)
.provideEnvironment(r)
def getWebACL(
request: GetWebAclRequest
): IO[AwsError, zio.aws.waf.model.GetWebAclResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetWebAclRequest,
GetWebAclResponse
]("getWebACL", api.getWebACL)(request.buildAwsValue())
.map(zio.aws.waf.model.GetWebAclResponse.wrap)
.provideEnvironment(r)
def getRateBasedRule(
request: GetRateBasedRuleRequest
): IO[AwsError, zio.aws.waf.model.GetRateBasedRuleResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetRateBasedRuleRequest,
GetRateBasedRuleResponse
]("getRateBasedRule", api.getRateBasedRule)(request.buildAwsValue())
.map(zio.aws.waf.model.GetRateBasedRuleResponse.wrap)
.provideEnvironment(r)
def listSizeConstraintSets(
request: ListSizeConstraintSetsRequest
): IO[AwsError, zio.aws.waf.model.ListSizeConstraintSetsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListSizeConstraintSetsRequest,
ListSizeConstraintSetsResponse
]("listSizeConstraintSets", api.listSizeConstraintSets)(
request.buildAwsValue()
).map(zio.aws.waf.model.ListSizeConstraintSetsResponse.wrap)
.provideEnvironment(r)
def listRegexMatchSets(
request: ListRegexMatchSetsRequest
): IO[AwsError, zio.aws.waf.model.ListRegexMatchSetsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListRegexMatchSetsRequest,
ListRegexMatchSetsResponse
]("listRegexMatchSets", api.listRegexMatchSets)(request.buildAwsValue())
.map(zio.aws.waf.model.ListRegexMatchSetsResponse.wrap)
.provideEnvironment(r)
def getRegexMatchSet(
request: GetRegexMatchSetRequest
): IO[AwsError, zio.aws.waf.model.GetRegexMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetRegexMatchSetRequest,
GetRegexMatchSetResponse
]("getRegexMatchSet", api.getRegexMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.GetRegexMatchSetResponse.wrap)
.provideEnvironment(r)
def listSubscribedRuleGroups(request: ListSubscribedRuleGroupsRequest): IO[
AwsError,
zio.aws.waf.model.ListSubscribedRuleGroupsResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListSubscribedRuleGroupsRequest,
ListSubscribedRuleGroupsResponse
]("listSubscribedRuleGroups", api.listSubscribedRuleGroups)(
request.buildAwsValue()
).map(zio.aws.waf.model.ListSubscribedRuleGroupsResponse.wrap)
.provideEnvironment(r)
def getSqlInjectionMatchSet(request: GetSqlInjectionMatchSetRequest): IO[
AwsError,
zio.aws.waf.model.GetSqlInjectionMatchSetResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetSqlInjectionMatchSetRequest,
GetSqlInjectionMatchSetResponse
]("getSqlInjectionMatchSet", api.getSqlInjectionMatchSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.GetSqlInjectionMatchSetResponse.wrap)
.provideEnvironment(r)
def getIPSet(
request: GetIpSetRequest
): IO[AwsError, zio.aws.waf.model.GetIpSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetIpSetRequest,
GetIpSetResponse
]("getIPSet", api.getIPSet)(request.buildAwsValue())
.map(zio.aws.waf.model.GetIpSetResponse.wrap)
.provideEnvironment(r)
def createRuleGroup(
request: CreateRuleGroupRequest
): IO[AwsError, zio.aws.waf.model.CreateRuleGroupResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateRuleGroupRequest,
CreateRuleGroupResponse
]("createRuleGroup", api.createRuleGroup)(request.buildAwsValue())
.map(zio.aws.waf.model.CreateRuleGroupResponse.wrap)
.provideEnvironment(r)
def listRuleGroups(
request: ListRuleGroupsRequest
): IO[AwsError, zio.aws.waf.model.ListRuleGroupsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListRuleGroupsRequest,
ListRuleGroupsResponse
]("listRuleGroups", api.listRuleGroups)(request.buildAwsValue())
.map(zio.aws.waf.model.ListRuleGroupsResponse.wrap)
.provideEnvironment(r)
def deleteRegexPatternSet(
request: DeleteRegexPatternSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteRegexPatternSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteRegexPatternSetRequest,
DeleteRegexPatternSetResponse
]("deleteRegexPatternSet", api.deleteRegexPatternSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.DeleteRegexPatternSetResponse.wrap)
.provideEnvironment(r)
def updateRateBasedRule(
request: UpdateRateBasedRuleRequest
): IO[AwsError, zio.aws.waf.model.UpdateRateBasedRuleResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateRateBasedRuleRequest,
UpdateRateBasedRuleResponse
]("updateRateBasedRule", api.updateRateBasedRule)(request.buildAwsValue())
.map(zio.aws.waf.model.UpdateRateBasedRuleResponse.wrap)
.provideEnvironment(r)
def updateSqlInjectionMatchSet(
request: UpdateSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.waf.model.UpdateSqlInjectionMatchSetResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateSqlInjectionMatchSetRequest,
UpdateSqlInjectionMatchSetResponse
]("updateSqlInjectionMatchSet", api.updateSqlInjectionMatchSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.UpdateSqlInjectionMatchSetResponse.wrap)
.provideEnvironment(r)
def deleteGeoMatchSet(
request: DeleteGeoMatchSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteGeoMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteGeoMatchSetRequest,
DeleteGeoMatchSetResponse
]("deleteGeoMatchSet", api.deleteGeoMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.DeleteGeoMatchSetResponse.wrap)
.provideEnvironment(r)
def createIPSet(
request: CreateIpSetRequest
): IO[AwsError, zio.aws.waf.model.CreateIpSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateIpSetRequest,
CreateIpSetResponse
]("createIPSet", api.createIPSet)(request.buildAwsValue())
.map(zio.aws.waf.model.CreateIpSetResponse.wrap)
.provideEnvironment(r)
def getRateBasedRuleManagedKeys(
request: GetRateBasedRuleManagedKeysRequest
): IO[
AwsError,
zio.aws.waf.model.GetRateBasedRuleManagedKeysResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetRateBasedRuleManagedKeysRequest,
GetRateBasedRuleManagedKeysResponse
]("getRateBasedRuleManagedKeys", api.getRateBasedRuleManagedKeys)(
request.buildAwsValue()
).map(zio.aws.waf.model.GetRateBasedRuleManagedKeysResponse.wrap)
.provideEnvironment(r)
def updateGeoMatchSet(
request: UpdateGeoMatchSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateGeoMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateGeoMatchSetRequest,
UpdateGeoMatchSetResponse
]("updateGeoMatchSet", api.updateGeoMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.UpdateGeoMatchSetResponse.wrap)
.provideEnvironment(r)
def listRegexPatternSets(
request: ListRegexPatternSetsRequest
): IO[AwsError, zio.aws.waf.model.ListRegexPatternSetsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListRegexPatternSetsRequest,
ListRegexPatternSetsResponse
]("listRegexPatternSets", api.listRegexPatternSets)(
request.buildAwsValue()
).map(zio.aws.waf.model.ListRegexPatternSetsResponse.wrap)
.provideEnvironment(r)
def listByteMatchSets(
request: ListByteMatchSetsRequest
): IO[AwsError, zio.aws.waf.model.ListByteMatchSetsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListByteMatchSetsRequest,
ListByteMatchSetsResponse
]("listByteMatchSets", api.listByteMatchSets)(request.buildAwsValue())
.map(zio.aws.waf.model.ListByteMatchSetsResponse.wrap)
.provideEnvironment(r)
def updateIPSet(
request: UpdateIpSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateIpSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateIpSetRequest,
UpdateIpSetResponse
]("updateIPSet", api.updateIPSet)(request.buildAwsValue())
.map(zio.aws.waf.model.UpdateIpSetResponse.wrap)
.provideEnvironment(r)
def createRateBasedRule(
request: CreateRateBasedRuleRequest
): IO[AwsError, zio.aws.waf.model.CreateRateBasedRuleResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateRateBasedRuleRequest,
CreateRateBasedRuleResponse
]("createRateBasedRule", api.createRateBasedRule)(request.buildAwsValue())
.map(zio.aws.waf.model.CreateRateBasedRuleResponse.wrap)
.provideEnvironment(r)
def deleteXssMatchSet(
request: DeleteXssMatchSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteXssMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteXssMatchSetRequest,
DeleteXssMatchSetResponse
]("deleteXssMatchSet", api.deleteXssMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.DeleteXssMatchSetResponse.wrap)
.provideEnvironment(r)
def getByteMatchSet(
request: GetByteMatchSetRequest
): IO[AwsError, zio.aws.waf.model.GetByteMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetByteMatchSetRequest,
GetByteMatchSetResponse
]("getByteMatchSet", api.getByteMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.GetByteMatchSetResponse.wrap)
.provideEnvironment(r)
def deleteRule(
request: DeleteRuleRequest
): IO[AwsError, zio.aws.waf.model.DeleteRuleResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteRuleRequest,
DeleteRuleResponse
]("deleteRule", api.deleteRule)(request.buildAwsValue())
.map(zio.aws.waf.model.DeleteRuleResponse.wrap)
.provideEnvironment(r)
def getSizeConstraintSet(
request: GetSizeConstraintSetRequest
): IO[AwsError, zio.aws.waf.model.GetSizeConstraintSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetSizeConstraintSetRequest,
GetSizeConstraintSetResponse
]("getSizeConstraintSet", api.getSizeConstraintSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.GetSizeConstraintSetResponse.wrap)
.provideEnvironment(r)
def createByteMatchSet(
request: CreateByteMatchSetRequest
): IO[AwsError, zio.aws.waf.model.CreateByteMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateByteMatchSetRequest,
CreateByteMatchSetResponse
]("createByteMatchSet", api.createByteMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.CreateByteMatchSetResponse.wrap)
.provideEnvironment(r)
def updateRule(
request: UpdateRuleRequest
): IO[AwsError, zio.aws.waf.model.UpdateRuleResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateRuleRequest,
UpdateRuleResponse
]("updateRule", api.updateRule)(request.buildAwsValue())
.map(zio.aws.waf.model.UpdateRuleResponse.wrap)
.provideEnvironment(r)
def getRegexPatternSet(
request: GetRegexPatternSetRequest
): IO[AwsError, zio.aws.waf.model.GetRegexPatternSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetRegexPatternSetRequest,
GetRegexPatternSetResponse
]("getRegexPatternSet", api.getRegexPatternSet)(request.buildAwsValue())
.map(zio.aws.waf.model.GetRegexPatternSetResponse.wrap)
.provideEnvironment(r)
def untagResource(
request: UntagResourceRequest
): IO[AwsError, zio.aws.waf.model.UntagResourceResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UntagResourceRequest,
UntagResourceResponse
]("untagResource", api.untagResource)(request.buildAwsValue())
.map(zio.aws.waf.model.UntagResourceResponse.wrap)
.provideEnvironment(r)
def deleteRateBasedRule(
request: DeleteRateBasedRuleRequest
): IO[AwsError, zio.aws.waf.model.DeleteRateBasedRuleResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteRateBasedRuleRequest,
DeleteRateBasedRuleResponse
]("deleteRateBasedRule", api.deleteRateBasedRule)(request.buildAwsValue())
.map(zio.aws.waf.model.DeleteRateBasedRuleResponse.wrap)
.provideEnvironment(r)
def putLoggingConfiguration(request: PutLoggingConfigurationRequest): IO[
AwsError,
zio.aws.waf.model.PutLoggingConfigurationResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.PutLoggingConfigurationRequest,
PutLoggingConfigurationResponse
]("putLoggingConfiguration", api.putLoggingConfiguration)(
request.buildAwsValue()
).map(zio.aws.waf.model.PutLoggingConfigurationResponse.wrap)
.provideEnvironment(r)
def getXssMatchSet(
request: GetXssMatchSetRequest
): IO[AwsError, zio.aws.waf.model.GetXssMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetXssMatchSetRequest,
GetXssMatchSetResponse
]("getXssMatchSet", api.getXssMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.GetXssMatchSetResponse.wrap)
.provideEnvironment(r)
def deleteWebACL(
request: DeleteWebAclRequest
): IO[AwsError, zio.aws.waf.model.DeleteWebAclResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteWebAclRequest,
DeleteWebAclResponse
]("deleteWebACL", api.deleteWebACL)(request.buildAwsValue())
.map(zio.aws.waf.model.DeleteWebAclResponse.wrap)
.provideEnvironment(r)
def createRegexPatternSet(
request: CreateRegexPatternSetRequest
): IO[AwsError, zio.aws.waf.model.CreateRegexPatternSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateRegexPatternSetRequest,
CreateRegexPatternSetResponse
]("createRegexPatternSet", api.createRegexPatternSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.CreateRegexPatternSetResponse.wrap)
.provideEnvironment(r)
def listWebACLs(
request: ListWebAcLsRequest
): IO[AwsError, zio.aws.waf.model.ListWebAcLsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListWebAcLsRequest,
ListWebAcLsResponse
]("listWebACLs", api.listWebACLs)(request.buildAwsValue())
.map(zio.aws.waf.model.ListWebAcLsResponse.wrap)
.provideEnvironment(r)
def putPermissionPolicy(
request: PutPermissionPolicyRequest
): IO[AwsError, zio.aws.waf.model.PutPermissionPolicyResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.PutPermissionPolicyRequest,
PutPermissionPolicyResponse
]("putPermissionPolicy", api.putPermissionPolicy)(request.buildAwsValue())
.map(zio.aws.waf.model.PutPermissionPolicyResponse.wrap)
.provideEnvironment(r)
def createXssMatchSet(
request: CreateXssMatchSetRequest
): IO[AwsError, zio.aws.waf.model.CreateXssMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateXssMatchSetRequest,
CreateXssMatchSetResponse
]("createXssMatchSet", api.createXssMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.CreateXssMatchSetResponse.wrap)
.provideEnvironment(r)
def getChangeTokenStatus(
request: GetChangeTokenStatusRequest
): IO[AwsError, zio.aws.waf.model.GetChangeTokenStatusResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetChangeTokenStatusRequest,
GetChangeTokenStatusResponse
]("getChangeTokenStatus", api.getChangeTokenStatus)(
request.buildAwsValue()
).map(zio.aws.waf.model.GetChangeTokenStatusResponse.wrap)
.provideEnvironment(r)
def createSizeConstraintSet(request: CreateSizeConstraintSetRequest): IO[
AwsError,
zio.aws.waf.model.CreateSizeConstraintSetResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateSizeConstraintSetRequest,
CreateSizeConstraintSetResponse
]("createSizeConstraintSet", api.createSizeConstraintSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.CreateSizeConstraintSetResponse.wrap)
.provideEnvironment(r)
def getLoggingConfiguration(request: GetLoggingConfigurationRequest): IO[
AwsError,
zio.aws.waf.model.GetLoggingConfigurationResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetLoggingConfigurationRequest,
GetLoggingConfigurationResponse
]("getLoggingConfiguration", api.getLoggingConfiguration)(
request.buildAwsValue()
).map(zio.aws.waf.model.GetLoggingConfigurationResponse.wrap)
.provideEnvironment(r)
def listTagsForResource(
request: ListTagsForResourceRequest
): IO[AwsError, zio.aws.waf.model.ListTagsForResourceResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListTagsForResourceRequest,
ListTagsForResourceResponse
]("listTagsForResource", api.listTagsForResource)(request.buildAwsValue())
.map(zio.aws.waf.model.ListTagsForResourceResponse.wrap)
.provideEnvironment(r)
def tagResource(
request: TagResourceRequest
): IO[AwsError, zio.aws.waf.model.TagResourceResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.TagResourceRequest,
TagResourceResponse
]("tagResource", api.tagResource)(request.buildAwsValue())
.map(zio.aws.waf.model.TagResourceResponse.wrap)
.provideEnvironment(r)
def listRules(
request: ListRulesRequest
): IO[AwsError, zio.aws.waf.model.ListRulesResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListRulesRequest,
ListRulesResponse
]("listRules", api.listRules)(request.buildAwsValue())
.map(zio.aws.waf.model.ListRulesResponse.wrap)
.provideEnvironment(r)
def listLoggingConfigurations(
request: ListLoggingConfigurationsRequest
): IO[
AwsError,
zio.aws.waf.model.ListLoggingConfigurationsResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListLoggingConfigurationsRequest,
ListLoggingConfigurationsResponse
]("listLoggingConfigurations", api.listLoggingConfigurations)(
request.buildAwsValue()
).map(zio.aws.waf.model.ListLoggingConfigurationsResponse.wrap)
.provideEnvironment(r)
def updateXssMatchSet(
request: UpdateXssMatchSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateXssMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateXssMatchSetRequest,
UpdateXssMatchSetResponse
]("updateXssMatchSet", api.updateXssMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.UpdateXssMatchSetResponse.wrap)
.provideEnvironment(r)
def deleteLoggingConfiguration(
request: DeleteLoggingConfigurationRequest
): IO[
AwsError,
zio.aws.waf.model.DeleteLoggingConfigurationResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteLoggingConfigurationRequest,
DeleteLoggingConfigurationResponse
]("deleteLoggingConfiguration", api.deleteLoggingConfiguration)(
request.buildAwsValue()
).map(zio.aws.waf.model.DeleteLoggingConfigurationResponse.wrap)
.provideEnvironment(r)
def getGeoMatchSet(
request: GetGeoMatchSetRequest
): IO[AwsError, zio.aws.waf.model.GetGeoMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetGeoMatchSetRequest,
GetGeoMatchSetResponse
]("getGeoMatchSet", api.getGeoMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.GetGeoMatchSetResponse.wrap)
.provideEnvironment(r)
def updateSizeConstraintSet(request: UpdateSizeConstraintSetRequest): IO[
AwsError,
zio.aws.waf.model.UpdateSizeConstraintSetResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateSizeConstraintSetRequest,
UpdateSizeConstraintSetResponse
]("updateSizeConstraintSet", api.updateSizeConstraintSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.UpdateSizeConstraintSetResponse.wrap)
.provideEnvironment(r)
def listRateBasedRules(
request: ListRateBasedRulesRequest
): IO[AwsError, zio.aws.waf.model.ListRateBasedRulesResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListRateBasedRulesRequest,
ListRateBasedRulesResponse
]("listRateBasedRules", api.listRateBasedRules)(request.buildAwsValue())
.map(zio.aws.waf.model.ListRateBasedRulesResponse.wrap)
.provideEnvironment(r)
def updateRuleGroup(
request: UpdateRuleGroupRequest
): IO[AwsError, zio.aws.waf.model.UpdateRuleGroupResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateRuleGroupRequest,
UpdateRuleGroupResponse
]("updateRuleGroup", api.updateRuleGroup)(request.buildAwsValue())
.map(zio.aws.waf.model.UpdateRuleGroupResponse.wrap)
.provideEnvironment(r)
def updateRegexPatternSet(
request: UpdateRegexPatternSetRequest
): IO[AwsError, zio.aws.waf.model.UpdateRegexPatternSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateRegexPatternSetRequest,
UpdateRegexPatternSetResponse
]("updateRegexPatternSet", api.updateRegexPatternSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.UpdateRegexPatternSetResponse.wrap)
.provideEnvironment(r)
def createWebACL(
request: CreateWebAclRequest
): IO[AwsError, zio.aws.waf.model.CreateWebAclResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateWebAclRequest,
CreateWebAclResponse
]("createWebACL", api.createWebACL)(request.buildAwsValue())
.map(zio.aws.waf.model.CreateWebAclResponse.wrap)
.provideEnvironment(r)
def createGeoMatchSet(
request: CreateGeoMatchSetRequest
): IO[AwsError, zio.aws.waf.model.CreateGeoMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateGeoMatchSetRequest,
CreateGeoMatchSetResponse
]("createGeoMatchSet", api.createGeoMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.CreateGeoMatchSetResponse.wrap)
.provideEnvironment(r)
def getPermissionPolicy(
request: GetPermissionPolicyRequest
): IO[AwsError, zio.aws.waf.model.GetPermissionPolicyResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetPermissionPolicyRequest,
GetPermissionPolicyResponse
]("getPermissionPolicy", api.getPermissionPolicy)(request.buildAwsValue())
.map(zio.aws.waf.model.GetPermissionPolicyResponse.wrap)
.provideEnvironment(r)
def updateWebACL(
request: UpdateWebAclRequest
): IO[AwsError, zio.aws.waf.model.UpdateWebAclResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.UpdateWebAclRequest,
UpdateWebAclResponse
]("updateWebACL", api.updateWebACL)(request.buildAwsValue())
.map(zio.aws.waf.model.UpdateWebAclResponse.wrap)
.provideEnvironment(r)
def deleteRuleGroup(
request: DeleteRuleGroupRequest
): IO[AwsError, zio.aws.waf.model.DeleteRuleGroupResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteRuleGroupRequest,
DeleteRuleGroupResponse
]("deleteRuleGroup", api.deleteRuleGroup)(request.buildAwsValue())
.map(zio.aws.waf.model.DeleteRuleGroupResponse.wrap)
.provideEnvironment(r)
def deleteByteMatchSet(
request: DeleteByteMatchSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteByteMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteByteMatchSetRequest,
DeleteByteMatchSetResponse
]("deleteByteMatchSet", api.deleteByteMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.DeleteByteMatchSetResponse.wrap)
.provideEnvironment(r)
def getChangeToken(
request: GetChangeTokenRequest
): IO[AwsError, zio.aws.waf.model.GetChangeTokenResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetChangeTokenRequest,
GetChangeTokenResponse
]("getChangeToken", api.getChangeToken)(request.buildAwsValue())
.map(zio.aws.waf.model.GetChangeTokenResponse.wrap)
.provideEnvironment(r)
def deleteRegexMatchSet(
request: DeleteRegexMatchSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteRegexMatchSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteRegexMatchSetRequest,
DeleteRegexMatchSetResponse
]("deleteRegexMatchSet", api.deleteRegexMatchSet)(request.buildAwsValue())
.map(zio.aws.waf.model.DeleteRegexMatchSetResponse.wrap)
.provideEnvironment(r)
def listIPSets(
request: ListIpSetsRequest
): IO[AwsError, zio.aws.waf.model.ListIpSetsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListIpSetsRequest,
ListIpSetsResponse
]("listIPSets", api.listIPSets)(request.buildAwsValue())
.map(zio.aws.waf.model.ListIpSetsResponse.wrap)
.provideEnvironment(r)
def deleteSizeConstraintSet(request: DeleteSizeConstraintSetRequest): IO[
AwsError,
zio.aws.waf.model.DeleteSizeConstraintSetResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteSizeConstraintSetRequest,
DeleteSizeConstraintSetResponse
]("deleteSizeConstraintSet", api.deleteSizeConstraintSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.DeleteSizeConstraintSetResponse.wrap)
.provideEnvironment(r)
def getSampledRequests(
request: GetSampledRequestsRequest
): IO[AwsError, zio.aws.waf.model.GetSampledRequestsResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetSampledRequestsRequest,
GetSampledRequestsResponse
]("getSampledRequests", api.getSampledRequests)(request.buildAwsValue())
.map(zio.aws.waf.model.GetSampledRequestsResponse.wrap)
.provideEnvironment(r)
def listActivatedRulesInRuleGroup(
request: ListActivatedRulesInRuleGroupRequest
): IO[
AwsError,
zio.aws.waf.model.ListActivatedRulesInRuleGroupResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListActivatedRulesInRuleGroupRequest,
ListActivatedRulesInRuleGroupResponse
]("listActivatedRulesInRuleGroup", api.listActivatedRulesInRuleGroup)(
request.buildAwsValue()
).map(zio.aws.waf.model.ListActivatedRulesInRuleGroupResponse.wrap)
.provideEnvironment(r)
def deleteIPSet(
request: DeleteIpSetRequest
): IO[AwsError, zio.aws.waf.model.DeleteIpSetResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteIpSetRequest,
DeleteIpSetResponse
]("deleteIPSet", api.deleteIPSet)(request.buildAwsValue())
.map(zio.aws.waf.model.DeleteIpSetResponse.wrap)
.provideEnvironment(r)
def deletePermissionPolicy(
request: DeletePermissionPolicyRequest
): IO[AwsError, zio.aws.waf.model.DeletePermissionPolicyResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeletePermissionPolicyRequest,
DeletePermissionPolicyResponse
]("deletePermissionPolicy", api.deletePermissionPolicy)(
request.buildAwsValue()
).map(zio.aws.waf.model.DeletePermissionPolicyResponse.wrap)
.provideEnvironment(r)
def deleteSqlInjectionMatchSet(
request: DeleteSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.waf.model.DeleteSqlInjectionMatchSetResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.DeleteSqlInjectionMatchSetRequest,
DeleteSqlInjectionMatchSetResponse
]("deleteSqlInjectionMatchSet", api.deleteSqlInjectionMatchSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.DeleteSqlInjectionMatchSetResponse.wrap)
.provideEnvironment(r)
def listSqlInjectionMatchSets(
request: ListSqlInjectionMatchSetsRequest
): IO[
AwsError,
zio.aws.waf.model.ListSqlInjectionMatchSetsResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.ListSqlInjectionMatchSetsRequest,
ListSqlInjectionMatchSetsResponse
]("listSqlInjectionMatchSets", api.listSqlInjectionMatchSets)(
request.buildAwsValue()
).map(zio.aws.waf.model.ListSqlInjectionMatchSetsResponse.wrap)
.provideEnvironment(r)
def getRule(
request: GetRuleRequest
): IO[AwsError, zio.aws.waf.model.GetRuleResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.GetRuleRequest,
GetRuleResponse
]("getRule", api.getRule)(request.buildAwsValue())
.map(zio.aws.waf.model.GetRuleResponse.wrap)
.provideEnvironment(r)
def createWebACLMigrationStack(
request: CreateWebAclMigrationStackRequest
): IO[
AwsError,
zio.aws.waf.model.CreateWebAclMigrationStackResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateWebAclMigrationStackRequest,
CreateWebAclMigrationStackResponse
]("createWebACLMigrationStack", api.createWebACLMigrationStack)(
request.buildAwsValue()
).map(zio.aws.waf.model.CreateWebAclMigrationStackResponse.wrap)
.provideEnvironment(r)
def createSqlInjectionMatchSet(
request: CreateSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.waf.model.CreateSqlInjectionMatchSetResponse.ReadOnly
] = asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateSqlInjectionMatchSetRequest,
CreateSqlInjectionMatchSetResponse
]("createSqlInjectionMatchSet", api.createSqlInjectionMatchSet)(
request.buildAwsValue()
).map(zio.aws.waf.model.CreateSqlInjectionMatchSetResponse.wrap)
.provideEnvironment(r)
def createRule(
request: CreateRuleRequest
): IO[AwsError, zio.aws.waf.model.CreateRuleResponse.ReadOnly] =
asyncRequestResponse[
software.amazon.awssdk.services.waf.model.CreateRuleRequest,
CreateRuleResponse
]("createRule", api.createRule)(request.buildAwsValue())
.map(zio.aws.waf.model.CreateRuleResponse.wrap)
.provideEnvironment(r)
}
def listXssMatchSets(request: ListXssMatchSetsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListXssMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listXssMatchSets(request))
def getRuleGroup(request: GetRuleGroupRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRuleGroup(request))
def listGeoMatchSets(request: ListGeoMatchSetsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListGeoMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listGeoMatchSets(request))
def updateByteMatchSet(request: UpdateByteMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateByteMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateByteMatchSet(request))
def updateRegexMatchSet(
request: UpdateRegexMatchSetRequest
): ZIO[zio.aws.waf.Waf, AwsError, ReadOnly] =
ZIO.serviceWithZIO(_.updateRegexMatchSet(request))
def createRegexMatchSet(request: CreateRegexMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateRegexMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRegexMatchSet(request))
def getWebACL(request: GetWebAclRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getWebACL(request))
def getRateBasedRule(request: GetRateBasedRuleRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetRateBasedRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRateBasedRule(request))
def listSizeConstraintSets(request: ListSizeConstraintSetsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListSizeConstraintSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listSizeConstraintSets(request))
def listRegexMatchSets(request: ListRegexMatchSetsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListRegexMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRegexMatchSets(request))
def getRegexMatchSet(request: GetRegexMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetRegexMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRegexMatchSet(request))
def listSubscribedRuleGroups(request: ListSubscribedRuleGroupsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListSubscribedRuleGroupsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listSubscribedRuleGroups(request))
def getSqlInjectionMatchSet(request: GetSqlInjectionMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetSqlInjectionMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getSqlInjectionMatchSet(request))
def getIPSet(request: GetIpSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetIpSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getIPSet(request))
def createRuleGroup(request: CreateRuleGroupRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRuleGroup(request))
def listRuleGroups(request: ListRuleGroupsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListRuleGroupsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRuleGroups(request))
def deleteRegexPatternSet(request: DeleteRegexPatternSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteRegexPatternSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRegexPatternSet(request))
def updateRateBasedRule(request: UpdateRateBasedRuleRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateRateBasedRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateRateBasedRule(request))
def updateSqlInjectionMatchSet(
request: UpdateSqlInjectionMatchSetRequest
): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateSqlInjectionMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateSqlInjectionMatchSet(request))
def deleteGeoMatchSet(request: DeleteGeoMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteGeoMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteGeoMatchSet(request))
def createIPSet(request: CreateIpSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateIpSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createIPSet(request))
def getRateBasedRuleManagedKeys(
request: GetRateBasedRuleManagedKeysRequest
): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetRateBasedRuleManagedKeysResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRateBasedRuleManagedKeys(request))
def updateGeoMatchSet(request: UpdateGeoMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateGeoMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateGeoMatchSet(request))
def listRegexPatternSets(request: ListRegexPatternSetsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListRegexPatternSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRegexPatternSets(request))
def listByteMatchSets(request: ListByteMatchSetsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListByteMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listByteMatchSets(request))
def updateIPSet(request: UpdateIpSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateIpSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateIPSet(request))
def createRateBasedRule(request: CreateRateBasedRuleRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateRateBasedRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRateBasedRule(request))
def deleteXssMatchSet(request: DeleteXssMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteXssMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteXssMatchSet(request))
def getByteMatchSet(request: GetByteMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetByteMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getByteMatchSet(request))
def deleteRule(request: DeleteRuleRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRule(request))
def getSizeConstraintSet(request: GetSizeConstraintSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetSizeConstraintSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getSizeConstraintSet(request))
def createByteMatchSet(request: CreateByteMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateByteMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createByteMatchSet(request))
def updateRule(request: UpdateRuleRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateRule(request))
def getRegexPatternSet(request: GetRegexPatternSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetRegexPatternSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRegexPatternSet(request))
def untagResource(request: UntagResourceRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UntagResourceResponse.ReadOnly
] = ZIO.serviceWithZIO(_.untagResource(request))
def deleteRateBasedRule(request: DeleteRateBasedRuleRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteRateBasedRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRateBasedRule(request))
def putLoggingConfiguration(request: PutLoggingConfigurationRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.PutLoggingConfigurationResponse.ReadOnly
] = ZIO.serviceWithZIO(_.putLoggingConfiguration(request))
def getXssMatchSet(request: GetXssMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetXssMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getXssMatchSet(request))
def deleteWebACL(request: DeleteWebAclRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteWebACL(request))
def createRegexPatternSet(request: CreateRegexPatternSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateRegexPatternSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRegexPatternSet(request))
def listWebACLs(request: ListWebAcLsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListWebAcLsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listWebACLs(request))
def putPermissionPolicy(request: PutPermissionPolicyRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.PutPermissionPolicyResponse.ReadOnly
] = ZIO.serviceWithZIO(_.putPermissionPolicy(request))
def createXssMatchSet(request: CreateXssMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateXssMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createXssMatchSet(request))
def getChangeTokenStatus(request: GetChangeTokenStatusRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetChangeTokenStatusResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getChangeTokenStatus(request))
def createSizeConstraintSet(request: CreateSizeConstraintSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateSizeConstraintSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createSizeConstraintSet(request))
def getLoggingConfiguration(request: GetLoggingConfigurationRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetLoggingConfigurationResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getLoggingConfiguration(request))
def listTagsForResource(request: ListTagsForResourceRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListTagsForResourceResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listTagsForResource(request))
def tagResource(request: TagResourceRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.TagResourceResponse.ReadOnly
] = ZIO.serviceWithZIO(_.tagResource(request))
def listRules(request: ListRulesRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListRulesResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRules(request))
def listLoggingConfigurations(request: ListLoggingConfigurationsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListLoggingConfigurationsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listLoggingConfigurations(request))
def updateXssMatchSet(request: UpdateXssMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateXssMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateXssMatchSet(request))
def deleteLoggingConfiguration(
request: DeleteLoggingConfigurationRequest
): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteLoggingConfigurationResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteLoggingConfiguration(request))
def getGeoMatchSet(request: GetGeoMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetGeoMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getGeoMatchSet(request))
def updateSizeConstraintSet(request: UpdateSizeConstraintSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateSizeConstraintSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateSizeConstraintSet(request))
def listRateBasedRules(request: ListRateBasedRulesRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListRateBasedRulesResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRateBasedRules(request))
def updateRuleGroup(request: UpdateRuleGroupRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateRuleGroup(request))
def updateRegexPatternSet(request: UpdateRegexPatternSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateRegexPatternSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateRegexPatternSet(request))
def createWebACL(request: CreateWebAclRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createWebACL(request))
def createGeoMatchSet(request: CreateGeoMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateGeoMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createGeoMatchSet(request))
def getPermissionPolicy(request: GetPermissionPolicyRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetPermissionPolicyResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getPermissionPolicy(request))
def updateWebACL(request: UpdateWebAclRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.UpdateWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateWebACL(request))
def deleteRuleGroup(request: DeleteRuleGroupRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRuleGroup(request))
def deleteByteMatchSet(request: DeleteByteMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteByteMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteByteMatchSet(request))
def getChangeToken(request: GetChangeTokenRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetChangeTokenResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getChangeToken(request))
def deleteRegexMatchSet(request: DeleteRegexMatchSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteRegexMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRegexMatchSet(request))
def listIPSets(request: ListIpSetsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListIpSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listIPSets(request))
def deleteSizeConstraintSet(request: DeleteSizeConstraintSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteSizeConstraintSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteSizeConstraintSet(request))
def getSampledRequests(request: GetSampledRequestsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetSampledRequestsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getSampledRequests(request))
def listActivatedRulesInRuleGroup(
request: ListActivatedRulesInRuleGroupRequest
): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListActivatedRulesInRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listActivatedRulesInRuleGroup(request))
def deleteIPSet(request: DeleteIpSetRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteIpSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteIPSet(request))
def deletePermissionPolicy(request: DeletePermissionPolicyRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeletePermissionPolicyResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deletePermissionPolicy(request))
def deleteSqlInjectionMatchSet(
request: DeleteSqlInjectionMatchSetRequest
): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.DeleteSqlInjectionMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteSqlInjectionMatchSet(request))
def listSqlInjectionMatchSets(request: ListSqlInjectionMatchSetsRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.ListSqlInjectionMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listSqlInjectionMatchSets(request))
def getRule(request: GetRuleRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.GetRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRule(request))
def createWebACLMigrationStack(
request: CreateWebAclMigrationStackRequest
): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateWebAclMigrationStackResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createWebACLMigrationStack(request))
def createSqlInjectionMatchSet(
request: CreateSqlInjectionMatchSetRequest
): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateSqlInjectionMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createSqlInjectionMatchSet(request))
def createRule(request: CreateRuleRequest): ZIO[
zio.aws.waf.Waf,
AwsError,
zio.aws.waf.model.CreateRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRule(request))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy