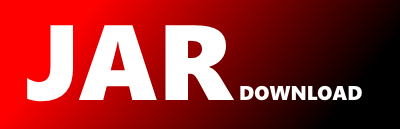
zio.aws.wafregional.WafRegional.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zio-aws-wafregional_2.13 Show documentation
Show all versions of zio-aws-wafregional_2.13 Show documentation
Low-level AWS wrapper for ZIO
package zio.aws.wafregional
import zio.aws.wafregional.model.CreateSizeConstraintSetResponse.ReadOnly
import zio.aws.core.config.AwsConfig
import software.amazon.awssdk.services.waf.model.{
ListResourcesForWebAclResponse,
DeleteRateBasedRuleRequest,
GetSizeConstraintSetResponse,
GetRateBasedRuleManagedKeysRequest,
UpdateWebAclRequest,
GetRuleGroupRequest,
GetLoggingConfigurationRequest,
UpdateByteMatchSetRequest,
DisassociateWebAclResponse,
GetRegexPatternSetResponse,
AssociateWebAclRequest,
GetWebAclForResourceResponse,
CreateSqlInjectionMatchSetResponse,
PutPermissionPolicyResponse,
GetChangeTokenStatusResponse,
DeleteWebAclRequest,
UpdateRateBasedRuleRequest,
DeleteRuleGroupRequest,
GetIpSetRequest,
GetSizeConstraintSetRequest,
UpdateIpSetResponse,
ListSqlInjectionMatchSetsResponse,
GetPermissionPolicyRequest,
DeleteSqlInjectionMatchSetRequest,
UpdateSqlInjectionMatchSetResponse,
UpdateIpSetRequest,
GetRateBasedRuleResponse,
DeleteLoggingConfigurationResponse,
GetXssMatchSetRequest,
DeleteXssMatchSetResponse,
ListRulesResponse,
DeleteRuleGroupResponse,
CreateByteMatchSetRequest,
CreateXssMatchSetRequest,
CreateWebAclMigrationStackRequest,
DeleteRateBasedRuleResponse,
ListRulesRequest,
CreateSizeConstraintSetResponse,
GetRuleRequest,
UpdateRateBasedRuleResponse,
PutPermissionPolicyRequest,
CreateRegexPatternSetResponse,
DeletePermissionPolicyResponse,
GetIpSetResponse,
ListIpSetsResponse,
ListRegexMatchSetsResponse,
GetChangeTokenRequest,
UpdateSqlInjectionMatchSetRequest,
DeleteRegexMatchSetRequest,
CreateIpSetResponse,
DeleteWebAclResponse,
ListRateBasedRulesResponse,
UpdateSizeConstraintSetResponse,
UpdateRuleResponse,
ListTagsForResourceRequest,
GetRegexPatternSetRequest,
CreateRuleGroupResponse,
ListSizeConstraintSetsRequest,
ListRuleGroupsRequest,
PutLoggingConfigurationRequest,
GetPermissionPolicyResponse,
DeleteByteMatchSetResponse,
ListLoggingConfigurationsRequest,
CreateGeoMatchSetResponse,
GetRuleResponse,
GetRateBasedRuleManagedKeysResponse,
ListActivatedRulesInRuleGroupRequest,
DeleteIpSetRequest,
CreateWebAclResponse,
UpdateByteMatchSetResponse,
GetRegexMatchSetRequest,
ListSizeConstraintSetsResponse,
ListRegexMatchSetsRequest,
DeletePermissionPolicyRequest,
CreateWebAclMigrationStackResponse,
CreateGeoMatchSetRequest,
ListTagsForResourceResponse,
ListGeoMatchSetsResponse,
UntagResourceResponse,
UpdateXssMatchSetResponse,
CreateRuleRequest,
CreateXssMatchSetResponse,
ListLoggingConfigurationsResponse,
GetGeoMatchSetRequest,
DeleteIpSetResponse,
GetWebAclRequest,
DeleteLoggingConfigurationRequest,
UpdateGeoMatchSetRequest,
DeleteXssMatchSetRequest,
AssociateWebAclResponse,
UpdateRegexPatternSetResponse,
ListSubscribedRuleGroupsResponse,
GetSqlInjectionMatchSetRequest,
GetSqlInjectionMatchSetResponse,
ListSubscribedRuleGroupsRequest,
DeleteRuleRequest,
ListGeoMatchSetsRequest,
GetRateBasedRuleRequest,
CreateRegexMatchSetResponse,
DisassociateWebAclRequest,
UpdateGeoMatchSetResponse,
GetXssMatchSetResponse,
GetGeoMatchSetResponse,
DeleteRegexPatternSetRequest,
GetByteMatchSetResponse,
CreateRegexPatternSetRequest,
GetSampledRequestsRequest,
DeleteByteMatchSetRequest,
UpdateWebAclResponse,
UpdateRuleGroupRequest,
ListResourcesForWebAclRequest,
UntagResourceRequest,
CreateRuleGroupRequest,
GetByteMatchSetRequest,
CreateSqlInjectionMatchSetRequest,
ListWebAcLsResponse,
CreateRateBasedRuleRequest,
GetWebAclResponse,
GetLoggingConfigurationResponse,
DeleteSizeConstraintSetRequest,
GetSampledRequestsResponse,
CreateRegexMatchSetRequest,
ListXssMatchSetsResponse,
GetChangeTokenResponse,
ListXssMatchSetsRequest,
CreateRuleResponse,
ListRegexPatternSetsResponse,
UpdateRuleRequest,
UpdateRegexMatchSetResponse,
GetWebAclForResourceRequest,
GetRuleGroupResponse,
ListActivatedRulesInRuleGroupResponse,
UpdateRegexMatchSetRequest,
DeleteRegexPatternSetResponse,
CreateByteMatchSetResponse,
DeleteGeoMatchSetRequest,
PutLoggingConfigurationResponse,
DeleteSizeConstraintSetResponse,
TagResourceResponse,
CreateSizeConstraintSetRequest,
GetChangeTokenStatusRequest,
GetRegexMatchSetResponse,
UpdateRuleGroupResponse,
ListRateBasedRulesRequest,
CreateRateBasedRuleResponse,
ListByteMatchSetsRequest,
DeleteRegexMatchSetResponse,
ListRuleGroupsResponse,
DeleteRuleResponse,
UpdateXssMatchSetRequest,
UpdateRegexPatternSetRequest,
UpdateSizeConstraintSetRequest,
DeleteSqlInjectionMatchSetResponse,
ListSqlInjectionMatchSetsRequest,
TagResourceRequest,
DeleteGeoMatchSetResponse,
ListWebAcLsRequest,
CreateWebAclRequest,
ListRegexPatternSetsRequest,
ListByteMatchSetsResponse,
ListIpSetsRequest,
CreateIpSetRequest
}
import zio.aws.core.{AwsServiceBase, AwsError}
import zio.aws.core.aspects.{AwsCallAspect, AspectSupport}
import zio.{ZEnvironment, IO, ZIO, ZLayer, Scope}
import software.amazon.awssdk.services.waf.regional.{
WafRegionalAsyncClientBuilder,
WafRegionalAsyncClient
}
import scala.jdk.CollectionConverters._
trait WafRegional extends AspectSupport[WafRegional] {
val api: WafRegionalAsyncClient
def listXssMatchSets(
request: zio.aws.wafregional.model.ListXssMatchSetsRequest
): IO[AwsError, zio.aws.wafregional.model.ListXssMatchSetsResponse.ReadOnly]
def getRuleGroup(
request: zio.aws.wafregional.model.GetRuleGroupRequest
): IO[AwsError, zio.aws.wafregional.model.GetRuleGroupResponse.ReadOnly]
def listGeoMatchSets(
request: zio.aws.wafregional.model.ListGeoMatchSetsRequest
): IO[AwsError, zio.aws.wafregional.model.ListGeoMatchSetsResponse.ReadOnly]
def updateByteMatchSet(
request: zio.aws.wafregional.model.UpdateByteMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateByteMatchSetResponse.ReadOnly]
def updateRegexMatchSet(
request: zio.aws.wafregional.model.UpdateRegexMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateRegexMatchSetResponse.ReadOnly
]
def createRegexMatchSet(
request: zio.aws.wafregional.model.CreateRegexMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateRegexMatchSetResponse.ReadOnly
]
def getWebACL(
request: zio.aws.wafregional.model.GetWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.GetWebAclResponse.ReadOnly]
def getRateBasedRule(
request: zio.aws.wafregional.model.GetRateBasedRuleRequest
): IO[AwsError, zio.aws.wafregional.model.GetRateBasedRuleResponse.ReadOnly]
def listSizeConstraintSets(
request: zio.aws.wafregional.model.ListSizeConstraintSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListSizeConstraintSetsResponse.ReadOnly
]
def listRegexMatchSets(
request: zio.aws.wafregional.model.ListRegexMatchSetsRequest
): IO[AwsError, zio.aws.wafregional.model.ListRegexMatchSetsResponse.ReadOnly]
def getRegexMatchSet(
request: zio.aws.wafregional.model.GetRegexMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.GetRegexMatchSetResponse.ReadOnly]
def listSubscribedRuleGroups(
request: zio.aws.wafregional.model.ListSubscribedRuleGroupsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListSubscribedRuleGroupsResponse.ReadOnly
]
def getSqlInjectionMatchSet(
request: zio.aws.wafregional.model.GetSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetSqlInjectionMatchSetResponse.ReadOnly
]
def getIPSet(
request: zio.aws.wafregional.model.GetIpSetRequest
): IO[AwsError, zio.aws.wafregional.model.GetIpSetResponse.ReadOnly]
def createRuleGroup(
request: zio.aws.wafregional.model.CreateRuleGroupRequest
): IO[AwsError, zio.aws.wafregional.model.CreateRuleGroupResponse.ReadOnly]
def listRuleGroups(
request: zio.aws.wafregional.model.ListRuleGroupsRequest
): IO[AwsError, zio.aws.wafregional.model.ListRuleGroupsResponse.ReadOnly]
def deleteRegexPatternSet(
request: zio.aws.wafregional.model.DeleteRegexPatternSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteRegexPatternSetResponse.ReadOnly
]
def updateRateBasedRule(
request: zio.aws.wafregional.model.UpdateRateBasedRuleRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateRateBasedRuleResponse.ReadOnly
]
def disassociateWebACL(
request: zio.aws.wafregional.model.DisassociateWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.DisassociateWebAclResponse.ReadOnly]
def listResourcesForWebACL(
request: zio.aws.wafregional.model.ListResourcesForWebAclRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListResourcesForWebAclResponse.ReadOnly
]
def updateSqlInjectionMatchSet(
request: zio.aws.wafregional.model.UpdateSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateSqlInjectionMatchSetResponse.ReadOnly
]
def deleteGeoMatchSet(
request: zio.aws.wafregional.model.DeleteGeoMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteGeoMatchSetResponse.ReadOnly]
def createIPSet(
request: zio.aws.wafregional.model.CreateIpSetRequest
): IO[AwsError, zio.aws.wafregional.model.CreateIpSetResponse.ReadOnly]
def getWebACLForResource(
request: zio.aws.wafregional.model.GetWebAclForResourceRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetWebAclForResourceResponse.ReadOnly
]
def getRateBasedRuleManagedKeys(
request: zio.aws.wafregional.model.GetRateBasedRuleManagedKeysRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetRateBasedRuleManagedKeysResponse.ReadOnly
]
def updateGeoMatchSet(
request: zio.aws.wafregional.model.UpdateGeoMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateGeoMatchSetResponse.ReadOnly]
def listRegexPatternSets(
request: zio.aws.wafregional.model.ListRegexPatternSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListRegexPatternSetsResponse.ReadOnly
]
def listByteMatchSets(
request: zio.aws.wafregional.model.ListByteMatchSetsRequest
): IO[AwsError, zio.aws.wafregional.model.ListByteMatchSetsResponse.ReadOnly]
def updateIPSet(
request: zio.aws.wafregional.model.UpdateIpSetRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateIpSetResponse.ReadOnly]
def createRateBasedRule(
request: zio.aws.wafregional.model.CreateRateBasedRuleRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateRateBasedRuleResponse.ReadOnly
]
def deleteXssMatchSet(
request: zio.aws.wafregional.model.DeleteXssMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteXssMatchSetResponse.ReadOnly]
def getByteMatchSet(
request: zio.aws.wafregional.model.GetByteMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.GetByteMatchSetResponse.ReadOnly]
def deleteRule(
request: zio.aws.wafregional.model.DeleteRuleRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteRuleResponse.ReadOnly]
def getSizeConstraintSet(
request: zio.aws.wafregional.model.GetSizeConstraintSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetSizeConstraintSetResponse.ReadOnly
]
def createByteMatchSet(
request: zio.aws.wafregional.model.CreateByteMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.CreateByteMatchSetResponse.ReadOnly]
def updateRule(
request: zio.aws.wafregional.model.UpdateRuleRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateRuleResponse.ReadOnly]
def associateWebACL(
request: zio.aws.wafregional.model.AssociateWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.AssociateWebAclResponse.ReadOnly]
def getRegexPatternSet(
request: zio.aws.wafregional.model.GetRegexPatternSetRequest
): IO[AwsError, zio.aws.wafregional.model.GetRegexPatternSetResponse.ReadOnly]
def untagResource(
request: zio.aws.wafregional.model.UntagResourceRequest
): IO[AwsError, zio.aws.wafregional.model.UntagResourceResponse.ReadOnly]
def deleteRateBasedRule(
request: zio.aws.wafregional.model.DeleteRateBasedRuleRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteRateBasedRuleResponse.ReadOnly
]
def putLoggingConfiguration(
request: zio.aws.wafregional.model.PutLoggingConfigurationRequest
): IO[
AwsError,
zio.aws.wafregional.model.PutLoggingConfigurationResponse.ReadOnly
]
def getXssMatchSet(
request: zio.aws.wafregional.model.GetXssMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.GetXssMatchSetResponse.ReadOnly]
def deleteWebACL(
request: zio.aws.wafregional.model.DeleteWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteWebAclResponse.ReadOnly]
def createRegexPatternSet(
request: zio.aws.wafregional.model.CreateRegexPatternSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateRegexPatternSetResponse.ReadOnly
]
def listWebACLs(
request: zio.aws.wafregional.model.ListWebAcLsRequest
): IO[AwsError, zio.aws.wafregional.model.ListWebAcLsResponse.ReadOnly]
def putPermissionPolicy(
request: zio.aws.wafregional.model.PutPermissionPolicyRequest
): IO[
AwsError,
zio.aws.wafregional.model.PutPermissionPolicyResponse.ReadOnly
]
def createXssMatchSet(
request: zio.aws.wafregional.model.CreateXssMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.CreateXssMatchSetResponse.ReadOnly]
def getChangeTokenStatus(
request: zio.aws.wafregional.model.GetChangeTokenStatusRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetChangeTokenStatusResponse.ReadOnly
]
def createSizeConstraintSet(
request: zio.aws.wafregional.model.CreateSizeConstraintSetRequest
): IO[AwsError, ReadOnly]
def getLoggingConfiguration(
request: zio.aws.wafregional.model.GetLoggingConfigurationRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetLoggingConfigurationResponse.ReadOnly
]
def listTagsForResource(
request: zio.aws.wafregional.model.ListTagsForResourceRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListTagsForResourceResponse.ReadOnly
]
def tagResource(
request: zio.aws.wafregional.model.TagResourceRequest
): IO[AwsError, zio.aws.wafregional.model.TagResourceResponse.ReadOnly]
def listRules(
request: zio.aws.wafregional.model.ListRulesRequest
): IO[AwsError, zio.aws.wafregional.model.ListRulesResponse.ReadOnly]
def listLoggingConfigurations(
request: zio.aws.wafregional.model.ListLoggingConfigurationsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListLoggingConfigurationsResponse.ReadOnly
]
def updateXssMatchSet(
request: zio.aws.wafregional.model.UpdateXssMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateXssMatchSetResponse.ReadOnly]
def deleteLoggingConfiguration(
request: zio.aws.wafregional.model.DeleteLoggingConfigurationRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteLoggingConfigurationResponse.ReadOnly
]
def getGeoMatchSet(
request: zio.aws.wafregional.model.GetGeoMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.GetGeoMatchSetResponse.ReadOnly]
def updateSizeConstraintSet(
request: zio.aws.wafregional.model.UpdateSizeConstraintSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateSizeConstraintSetResponse.ReadOnly
]
def listRateBasedRules(
request: zio.aws.wafregional.model.ListRateBasedRulesRequest
): IO[AwsError, zio.aws.wafregional.model.ListRateBasedRulesResponse.ReadOnly]
def updateRuleGroup(
request: zio.aws.wafregional.model.UpdateRuleGroupRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateRuleGroupResponse.ReadOnly]
def updateRegexPatternSet(
request: zio.aws.wafregional.model.UpdateRegexPatternSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateRegexPatternSetResponse.ReadOnly
]
def createWebACL(
request: zio.aws.wafregional.model.CreateWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.CreateWebAclResponse.ReadOnly]
def createGeoMatchSet(
request: zio.aws.wafregional.model.CreateGeoMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.CreateGeoMatchSetResponse.ReadOnly]
def getPermissionPolicy(
request: zio.aws.wafregional.model.GetPermissionPolicyRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetPermissionPolicyResponse.ReadOnly
]
def updateWebACL(
request: zio.aws.wafregional.model.UpdateWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateWebAclResponse.ReadOnly]
def deleteRuleGroup(
request: zio.aws.wafregional.model.DeleteRuleGroupRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteRuleGroupResponse.ReadOnly]
def deleteByteMatchSet(
request: zio.aws.wafregional.model.DeleteByteMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteByteMatchSetResponse.ReadOnly]
def getChangeToken(
request: zio.aws.wafregional.model.GetChangeTokenRequest
): IO[AwsError, zio.aws.wafregional.model.GetChangeTokenResponse.ReadOnly]
def deleteRegexMatchSet(
request: zio.aws.wafregional.model.DeleteRegexMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteRegexMatchSetResponse.ReadOnly
]
def listIPSets(
request: zio.aws.wafregional.model.ListIpSetsRequest
): IO[AwsError, zio.aws.wafregional.model.ListIpSetsResponse.ReadOnly]
def deleteSizeConstraintSet(
request: zio.aws.wafregional.model.DeleteSizeConstraintSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteSizeConstraintSetResponse.ReadOnly
]
def getSampledRequests(
request: zio.aws.wafregional.model.GetSampledRequestsRequest
): IO[AwsError, zio.aws.wafregional.model.GetSampledRequestsResponse.ReadOnly]
def listActivatedRulesInRuleGroup(
request: zio.aws.wafregional.model.ListActivatedRulesInRuleGroupRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListActivatedRulesInRuleGroupResponse.ReadOnly
]
def deleteIPSet(
request: zio.aws.wafregional.model.DeleteIpSetRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteIpSetResponse.ReadOnly]
def deletePermissionPolicy(
request: zio.aws.wafregional.model.DeletePermissionPolicyRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeletePermissionPolicyResponse.ReadOnly
]
def deleteSqlInjectionMatchSet(
request: zio.aws.wafregional.model.DeleteSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteSqlInjectionMatchSetResponse.ReadOnly
]
def listSqlInjectionMatchSets(
request: zio.aws.wafregional.model.ListSqlInjectionMatchSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListSqlInjectionMatchSetsResponse.ReadOnly
]
def getRule(
request: zio.aws.wafregional.model.GetRuleRequest
): IO[AwsError, zio.aws.wafregional.model.GetRuleResponse.ReadOnly]
def createWebACLMigrationStack(
request: zio.aws.wafregional.model.CreateWebAclMigrationStackRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateWebAclMigrationStackResponse.ReadOnly
]
def createSqlInjectionMatchSet(
request: zio.aws.wafregional.model.CreateSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateSqlInjectionMatchSetResponse.ReadOnly
]
def createRule(
request: zio.aws.wafregional.model.CreateRuleRequest
): IO[AwsError, zio.aws.wafregional.model.CreateRuleResponse.ReadOnly]
}
object WafRegional {
val live: ZLayer[AwsConfig, java.lang.Throwable, WafRegional] = customized(
identity
)
def customized(
customization: WafRegionalAsyncClientBuilder => WafRegionalAsyncClientBuilder
): ZLayer[AwsConfig, java.lang.Throwable, WafRegional] =
ZLayer.scoped(scoped(customization))
def scoped(
customization: WafRegionalAsyncClientBuilder => WafRegionalAsyncClientBuilder
): ZIO[AwsConfig with Scope, java.lang.Throwable, WafRegional] = for (
awsConfig <- ZIO.service[AwsConfig]; executor <- ZIO.executor;
builder = WafRegionalAsyncClient
.builder()
.asyncConfiguration(
software.amazon.awssdk.core.client.config.ClientAsyncConfiguration
.builder()
.advancedOption(
software.amazon.awssdk.core.client.config.SdkAdvancedAsyncClientOption.FUTURE_COMPLETION_EXECUTOR,
executor.asJava
)
.build()
);
b0 <- awsConfig
.configure[WafRegionalAsyncClient, WafRegionalAsyncClientBuilder](
builder
);
b1 <- awsConfig.configureHttpClient[
WafRegionalAsyncClient,
WafRegionalAsyncClientBuilder
](
b0,
zio.aws.core.httpclient.ServiceHttpCapabilities(supportsHttp2 = false)
);
client <- ZIO.attempt(
customization(
b1.region(_root_.software.amazon.awssdk.regions.Region.AWS_GLOBAL)
).build()
)
)
yield new WafRegionalImpl(
client,
AwsCallAspect.identity,
ZEnvironment.empty
)
private class WafRegionalImpl[R](
override val api: WafRegionalAsyncClient,
override val aspect: AwsCallAspect[R],
r: ZEnvironment[R]
) extends WafRegional
with AwsServiceBase[R] {
override val serviceName: String = "WafRegional"
override def withAspect[R1](
newAspect: AwsCallAspect[R1],
r: ZEnvironment[R1]
): WafRegionalImpl[R1] = new WafRegionalImpl(api, newAspect, r)
def listXssMatchSets(
request: zio.aws.wafregional.model.ListXssMatchSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListXssMatchSetsResponse.ReadOnly
] = asyncRequestResponse[ListXssMatchSetsRequest, ListXssMatchSetsResponse](
"listXssMatchSets",
api.listXssMatchSets
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListXssMatchSetsResponse.wrap)
.provideEnvironment(r)
def getRuleGroup(
request: zio.aws.wafregional.model.GetRuleGroupRequest
): IO[AwsError, zio.aws.wafregional.model.GetRuleGroupResponse.ReadOnly] =
asyncRequestResponse[GetRuleGroupRequest, GetRuleGroupResponse](
"getRuleGroup",
api.getRuleGroup
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetRuleGroupResponse.wrap)
.provideEnvironment(r)
def listGeoMatchSets(
request: zio.aws.wafregional.model.ListGeoMatchSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListGeoMatchSetsResponse.ReadOnly
] = asyncRequestResponse[ListGeoMatchSetsRequest, ListGeoMatchSetsResponse](
"listGeoMatchSets",
api.listGeoMatchSets
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListGeoMatchSetsResponse.wrap)
.provideEnvironment(r)
def updateByteMatchSet(
request: zio.aws.wafregional.model.UpdateByteMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateByteMatchSetResponse.ReadOnly
] = asyncRequestResponse[
UpdateByteMatchSetRequest,
UpdateByteMatchSetResponse
]("updateByteMatchSet", api.updateByteMatchSet)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UpdateByteMatchSetResponse.wrap)
.provideEnvironment(r)
def updateRegexMatchSet(
request: zio.aws.wafregional.model.UpdateRegexMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateRegexMatchSetResponse.ReadOnly
] = asyncRequestResponse[
UpdateRegexMatchSetRequest,
UpdateRegexMatchSetResponse
]("updateRegexMatchSet", api.updateRegexMatchSet)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UpdateRegexMatchSetResponse.wrap)
.provideEnvironment(r)
def createRegexMatchSet(
request: zio.aws.wafregional.model.CreateRegexMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateRegexMatchSetResponse.ReadOnly
] = asyncRequestResponse[
CreateRegexMatchSetRequest,
CreateRegexMatchSetResponse
]("createRegexMatchSet", api.createRegexMatchSet)(request.buildAwsValue())
.map(zio.aws.wafregional.model.CreateRegexMatchSetResponse.wrap)
.provideEnvironment(r)
def getWebACL(
request: zio.aws.wafregional.model.GetWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.GetWebAclResponse.ReadOnly] =
asyncRequestResponse[GetWebAclRequest, GetWebAclResponse](
"getWebACL",
api.getWebACL
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetWebAclResponse.wrap)
.provideEnvironment(r)
def getRateBasedRule(
request: zio.aws.wafregional.model.GetRateBasedRuleRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetRateBasedRuleResponse.ReadOnly
] = asyncRequestResponse[GetRateBasedRuleRequest, GetRateBasedRuleResponse](
"getRateBasedRule",
api.getRateBasedRule
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetRateBasedRuleResponse.wrap)
.provideEnvironment(r)
def listSizeConstraintSets(
request: zio.aws.wafregional.model.ListSizeConstraintSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListSizeConstraintSetsResponse.ReadOnly
] = asyncRequestResponse[
ListSizeConstraintSetsRequest,
ListSizeConstraintSetsResponse
]("listSizeConstraintSets", api.listSizeConstraintSets)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.ListSizeConstraintSetsResponse.wrap)
.provideEnvironment(r)
def listRegexMatchSets(
request: zio.aws.wafregional.model.ListRegexMatchSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListRegexMatchSetsResponse.ReadOnly
] = asyncRequestResponse[
ListRegexMatchSetsRequest,
ListRegexMatchSetsResponse
]("listRegexMatchSets", api.listRegexMatchSets)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListRegexMatchSetsResponse.wrap)
.provideEnvironment(r)
def getRegexMatchSet(
request: zio.aws.wafregional.model.GetRegexMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetRegexMatchSetResponse.ReadOnly
] = asyncRequestResponse[GetRegexMatchSetRequest, GetRegexMatchSetResponse](
"getRegexMatchSet",
api.getRegexMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetRegexMatchSetResponse.wrap)
.provideEnvironment(r)
def listSubscribedRuleGroups(
request: zio.aws.wafregional.model.ListSubscribedRuleGroupsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListSubscribedRuleGroupsResponse.ReadOnly
] = asyncRequestResponse[
ListSubscribedRuleGroupsRequest,
ListSubscribedRuleGroupsResponse
]("listSubscribedRuleGroups", api.listSubscribedRuleGroups)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.ListSubscribedRuleGroupsResponse.wrap)
.provideEnvironment(r)
def getSqlInjectionMatchSet(
request: zio.aws.wafregional.model.GetSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetSqlInjectionMatchSetResponse.ReadOnly
] = asyncRequestResponse[
GetSqlInjectionMatchSetRequest,
GetSqlInjectionMatchSetResponse
]("getSqlInjectionMatchSet", api.getSqlInjectionMatchSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.GetSqlInjectionMatchSetResponse.wrap)
.provideEnvironment(r)
def getIPSet(
request: zio.aws.wafregional.model.GetIpSetRequest
): IO[AwsError, zio.aws.wafregional.model.GetIpSetResponse.ReadOnly] =
asyncRequestResponse[GetIpSetRequest, GetIpSetResponse](
"getIPSet",
api.getIPSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetIpSetResponse.wrap)
.provideEnvironment(r)
def createRuleGroup(
request: zio.aws.wafregional.model.CreateRuleGroupRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateRuleGroupResponse.ReadOnly
] = asyncRequestResponse[CreateRuleGroupRequest, CreateRuleGroupResponse](
"createRuleGroup",
api.createRuleGroup
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.CreateRuleGroupResponse.wrap)
.provideEnvironment(r)
def listRuleGroups(
request: zio.aws.wafregional.model.ListRuleGroupsRequest
): IO[AwsError, zio.aws.wafregional.model.ListRuleGroupsResponse.ReadOnly] =
asyncRequestResponse[ListRuleGroupsRequest, ListRuleGroupsResponse](
"listRuleGroups",
api.listRuleGroups
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListRuleGroupsResponse.wrap)
.provideEnvironment(r)
def deleteRegexPatternSet(
request: zio.aws.wafregional.model.DeleteRegexPatternSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteRegexPatternSetResponse.ReadOnly
] = asyncRequestResponse[
DeleteRegexPatternSetRequest,
DeleteRegexPatternSetResponse
]("deleteRegexPatternSet", api.deleteRegexPatternSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.DeleteRegexPatternSetResponse.wrap)
.provideEnvironment(r)
def updateRateBasedRule(
request: zio.aws.wafregional.model.UpdateRateBasedRuleRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateRateBasedRuleResponse.ReadOnly
] = asyncRequestResponse[
UpdateRateBasedRuleRequest,
UpdateRateBasedRuleResponse
]("updateRateBasedRule", api.updateRateBasedRule)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UpdateRateBasedRuleResponse.wrap)
.provideEnvironment(r)
def disassociateWebACL(
request: zio.aws.wafregional.model.DisassociateWebAclRequest
): IO[
AwsError,
zio.aws.wafregional.model.DisassociateWebAclResponse.ReadOnly
] = asyncRequestResponse[
DisassociateWebAclRequest,
DisassociateWebAclResponse
]("disassociateWebACL", api.disassociateWebACL)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DisassociateWebAclResponse.wrap)
.provideEnvironment(r)
def listResourcesForWebACL(
request: zio.aws.wafregional.model.ListResourcesForWebAclRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListResourcesForWebAclResponse.ReadOnly
] = asyncRequestResponse[
ListResourcesForWebAclRequest,
ListResourcesForWebAclResponse
]("listResourcesForWebACL", api.listResourcesForWebACL)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.ListResourcesForWebAclResponse.wrap)
.provideEnvironment(r)
def updateSqlInjectionMatchSet(
request: zio.aws.wafregional.model.UpdateSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateSqlInjectionMatchSetResponse.ReadOnly
] = asyncRequestResponse[
UpdateSqlInjectionMatchSetRequest,
UpdateSqlInjectionMatchSetResponse
]("updateSqlInjectionMatchSet", api.updateSqlInjectionMatchSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.UpdateSqlInjectionMatchSetResponse.wrap)
.provideEnvironment(r)
def deleteGeoMatchSet(
request: zio.aws.wafregional.model.DeleteGeoMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteGeoMatchSetResponse.ReadOnly
] =
asyncRequestResponse[DeleteGeoMatchSetRequest, DeleteGeoMatchSetResponse](
"deleteGeoMatchSet",
api.deleteGeoMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DeleteGeoMatchSetResponse.wrap)
.provideEnvironment(r)
def createIPSet(
request: zio.aws.wafregional.model.CreateIpSetRequest
): IO[AwsError, zio.aws.wafregional.model.CreateIpSetResponse.ReadOnly] =
asyncRequestResponse[CreateIpSetRequest, CreateIpSetResponse](
"createIPSet",
api.createIPSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.CreateIpSetResponse.wrap)
.provideEnvironment(r)
def getWebACLForResource(
request: zio.aws.wafregional.model.GetWebAclForResourceRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetWebAclForResourceResponse.ReadOnly
] = asyncRequestResponse[
GetWebAclForResourceRequest,
GetWebAclForResourceResponse
]("getWebACLForResource", api.getWebACLForResource)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetWebAclForResourceResponse.wrap)
.provideEnvironment(r)
def getRateBasedRuleManagedKeys(
request: zio.aws.wafregional.model.GetRateBasedRuleManagedKeysRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetRateBasedRuleManagedKeysResponse.ReadOnly
] = asyncRequestResponse[
GetRateBasedRuleManagedKeysRequest,
GetRateBasedRuleManagedKeysResponse
]("getRateBasedRuleManagedKeys", api.getRateBasedRuleManagedKeys)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.GetRateBasedRuleManagedKeysResponse.wrap)
.provideEnvironment(r)
def updateGeoMatchSet(
request: zio.aws.wafregional.model.UpdateGeoMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateGeoMatchSetResponse.ReadOnly
] =
asyncRequestResponse[UpdateGeoMatchSetRequest, UpdateGeoMatchSetResponse](
"updateGeoMatchSet",
api.updateGeoMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UpdateGeoMatchSetResponse.wrap)
.provideEnvironment(r)
def listRegexPatternSets(
request: zio.aws.wafregional.model.ListRegexPatternSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListRegexPatternSetsResponse.ReadOnly
] = asyncRequestResponse[
ListRegexPatternSetsRequest,
ListRegexPatternSetsResponse
]("listRegexPatternSets", api.listRegexPatternSets)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListRegexPatternSetsResponse.wrap)
.provideEnvironment(r)
def listByteMatchSets(
request: zio.aws.wafregional.model.ListByteMatchSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListByteMatchSetsResponse.ReadOnly
] =
asyncRequestResponse[ListByteMatchSetsRequest, ListByteMatchSetsResponse](
"listByteMatchSets",
api.listByteMatchSets
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListByteMatchSetsResponse.wrap)
.provideEnvironment(r)
def updateIPSet(
request: zio.aws.wafregional.model.UpdateIpSetRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateIpSetResponse.ReadOnly] =
asyncRequestResponse[UpdateIpSetRequest, UpdateIpSetResponse](
"updateIPSet",
api.updateIPSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UpdateIpSetResponse.wrap)
.provideEnvironment(r)
def createRateBasedRule(
request: zio.aws.wafregional.model.CreateRateBasedRuleRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateRateBasedRuleResponse.ReadOnly
] = asyncRequestResponse[
CreateRateBasedRuleRequest,
CreateRateBasedRuleResponse
]("createRateBasedRule", api.createRateBasedRule)(request.buildAwsValue())
.map(zio.aws.wafregional.model.CreateRateBasedRuleResponse.wrap)
.provideEnvironment(r)
def deleteXssMatchSet(
request: zio.aws.wafregional.model.DeleteXssMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteXssMatchSetResponse.ReadOnly
] =
asyncRequestResponse[DeleteXssMatchSetRequest, DeleteXssMatchSetResponse](
"deleteXssMatchSet",
api.deleteXssMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DeleteXssMatchSetResponse.wrap)
.provideEnvironment(r)
def getByteMatchSet(
request: zio.aws.wafregional.model.GetByteMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetByteMatchSetResponse.ReadOnly
] = asyncRequestResponse[GetByteMatchSetRequest, GetByteMatchSetResponse](
"getByteMatchSet",
api.getByteMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetByteMatchSetResponse.wrap)
.provideEnvironment(r)
def deleteRule(
request: zio.aws.wafregional.model.DeleteRuleRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteRuleResponse.ReadOnly] =
asyncRequestResponse[DeleteRuleRequest, DeleteRuleResponse](
"deleteRule",
api.deleteRule
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DeleteRuleResponse.wrap)
.provideEnvironment(r)
def getSizeConstraintSet(
request: zio.aws.wafregional.model.GetSizeConstraintSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetSizeConstraintSetResponse.ReadOnly
] = asyncRequestResponse[
GetSizeConstraintSetRequest,
GetSizeConstraintSetResponse
]("getSizeConstraintSet", api.getSizeConstraintSet)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetSizeConstraintSetResponse.wrap)
.provideEnvironment(r)
def createByteMatchSet(
request: zio.aws.wafregional.model.CreateByteMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateByteMatchSetResponse.ReadOnly
] = asyncRequestResponse[
CreateByteMatchSetRequest,
CreateByteMatchSetResponse
]("createByteMatchSet", api.createByteMatchSet)(request.buildAwsValue())
.map(zio.aws.wafregional.model.CreateByteMatchSetResponse.wrap)
.provideEnvironment(r)
def updateRule(
request: zio.aws.wafregional.model.UpdateRuleRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateRuleResponse.ReadOnly] =
asyncRequestResponse[UpdateRuleRequest, UpdateRuleResponse](
"updateRule",
api.updateRule
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UpdateRuleResponse.wrap)
.provideEnvironment(r)
def associateWebACL(
request: zio.aws.wafregional.model.AssociateWebAclRequest
): IO[
AwsError,
zio.aws.wafregional.model.AssociateWebAclResponse.ReadOnly
] = asyncRequestResponse[AssociateWebAclRequest, AssociateWebAclResponse](
"associateWebACL",
api.associateWebACL
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.AssociateWebAclResponse.wrap)
.provideEnvironment(r)
def getRegexPatternSet(
request: zio.aws.wafregional.model.GetRegexPatternSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetRegexPatternSetResponse.ReadOnly
] = asyncRequestResponse[
GetRegexPatternSetRequest,
GetRegexPatternSetResponse
]("getRegexPatternSet", api.getRegexPatternSet)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetRegexPatternSetResponse.wrap)
.provideEnvironment(r)
def untagResource(
request: zio.aws.wafregional.model.UntagResourceRequest
): IO[AwsError, zio.aws.wafregional.model.UntagResourceResponse.ReadOnly] =
asyncRequestResponse[UntagResourceRequest, UntagResourceResponse](
"untagResource",
api.untagResource
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UntagResourceResponse.wrap)
.provideEnvironment(r)
def deleteRateBasedRule(
request: zio.aws.wafregional.model.DeleteRateBasedRuleRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteRateBasedRuleResponse.ReadOnly
] = asyncRequestResponse[
DeleteRateBasedRuleRequest,
DeleteRateBasedRuleResponse
]("deleteRateBasedRule", api.deleteRateBasedRule)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DeleteRateBasedRuleResponse.wrap)
.provideEnvironment(r)
def putLoggingConfiguration(
request: zio.aws.wafregional.model.PutLoggingConfigurationRequest
): IO[
AwsError,
zio.aws.wafregional.model.PutLoggingConfigurationResponse.ReadOnly
] = asyncRequestResponse[
PutLoggingConfigurationRequest,
PutLoggingConfigurationResponse
]("putLoggingConfiguration", api.putLoggingConfiguration)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.PutLoggingConfigurationResponse.wrap)
.provideEnvironment(r)
def getXssMatchSet(
request: zio.aws.wafregional.model.GetXssMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.GetXssMatchSetResponse.ReadOnly] =
asyncRequestResponse[GetXssMatchSetRequest, GetXssMatchSetResponse](
"getXssMatchSet",
api.getXssMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetXssMatchSetResponse.wrap)
.provideEnvironment(r)
def deleteWebACL(
request: zio.aws.wafregional.model.DeleteWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteWebAclResponse.ReadOnly] =
asyncRequestResponse[DeleteWebAclRequest, DeleteWebAclResponse](
"deleteWebACL",
api.deleteWebACL
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DeleteWebAclResponse.wrap)
.provideEnvironment(r)
def createRegexPatternSet(
request: zio.aws.wafregional.model.CreateRegexPatternSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateRegexPatternSetResponse.ReadOnly
] = asyncRequestResponse[
CreateRegexPatternSetRequest,
CreateRegexPatternSetResponse
]("createRegexPatternSet", api.createRegexPatternSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.CreateRegexPatternSetResponse.wrap)
.provideEnvironment(r)
def listWebACLs(
request: zio.aws.wafregional.model.ListWebAcLsRequest
): IO[AwsError, zio.aws.wafregional.model.ListWebAcLsResponse.ReadOnly] =
asyncRequestResponse[ListWebAcLsRequest, ListWebAcLsResponse](
"listWebACLs",
api.listWebACLs
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListWebAcLsResponse.wrap)
.provideEnvironment(r)
def putPermissionPolicy(
request: zio.aws.wafregional.model.PutPermissionPolicyRequest
): IO[
AwsError,
zio.aws.wafregional.model.PutPermissionPolicyResponse.ReadOnly
] = asyncRequestResponse[
PutPermissionPolicyRequest,
PutPermissionPolicyResponse
]("putPermissionPolicy", api.putPermissionPolicy)(request.buildAwsValue())
.map(zio.aws.wafregional.model.PutPermissionPolicyResponse.wrap)
.provideEnvironment(r)
def createXssMatchSet(
request: zio.aws.wafregional.model.CreateXssMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateXssMatchSetResponse.ReadOnly
] =
asyncRequestResponse[CreateXssMatchSetRequest, CreateXssMatchSetResponse](
"createXssMatchSet",
api.createXssMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.CreateXssMatchSetResponse.wrap)
.provideEnvironment(r)
def getChangeTokenStatus(
request: zio.aws.wafregional.model.GetChangeTokenStatusRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetChangeTokenStatusResponse.ReadOnly
] = asyncRequestResponse[
GetChangeTokenStatusRequest,
GetChangeTokenStatusResponse
]("getChangeTokenStatus", api.getChangeTokenStatus)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetChangeTokenStatusResponse.wrap)
.provideEnvironment(r)
def createSizeConstraintSet(
request: zio.aws.wafregional.model.CreateSizeConstraintSetRequest
): IO[AwsError, ReadOnly] = asyncRequestResponse[
CreateSizeConstraintSetRequest,
CreateSizeConstraintSetResponse
]("createSizeConstraintSet", api.createSizeConstraintSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.CreateSizeConstraintSetResponse.wrap)
.provideEnvironment(r)
def getLoggingConfiguration(
request: zio.aws.wafregional.model.GetLoggingConfigurationRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetLoggingConfigurationResponse.ReadOnly
] = asyncRequestResponse[
GetLoggingConfigurationRequest,
GetLoggingConfigurationResponse
]("getLoggingConfiguration", api.getLoggingConfiguration)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.GetLoggingConfigurationResponse.wrap)
.provideEnvironment(r)
def listTagsForResource(
request: zio.aws.wafregional.model.ListTagsForResourceRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListTagsForResourceResponse.ReadOnly
] = asyncRequestResponse[
ListTagsForResourceRequest,
ListTagsForResourceResponse
]("listTagsForResource", api.listTagsForResource)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListTagsForResourceResponse.wrap)
.provideEnvironment(r)
def tagResource(
request: zio.aws.wafregional.model.TagResourceRequest
): IO[AwsError, zio.aws.wafregional.model.TagResourceResponse.ReadOnly] =
asyncRequestResponse[TagResourceRequest, TagResourceResponse](
"tagResource",
api.tagResource
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.TagResourceResponse.wrap)
.provideEnvironment(r)
def listRules(
request: zio.aws.wafregional.model.ListRulesRequest
): IO[AwsError, zio.aws.wafregional.model.ListRulesResponse.ReadOnly] =
asyncRequestResponse[ListRulesRequest, ListRulesResponse](
"listRules",
api.listRules
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListRulesResponse.wrap)
.provideEnvironment(r)
def listLoggingConfigurations(
request: zio.aws.wafregional.model.ListLoggingConfigurationsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListLoggingConfigurationsResponse.ReadOnly
] = asyncRequestResponse[
ListLoggingConfigurationsRequest,
ListLoggingConfigurationsResponse
]("listLoggingConfigurations", api.listLoggingConfigurations)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.ListLoggingConfigurationsResponse.wrap)
.provideEnvironment(r)
def updateXssMatchSet(
request: zio.aws.wafregional.model.UpdateXssMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateXssMatchSetResponse.ReadOnly
] =
asyncRequestResponse[UpdateXssMatchSetRequest, UpdateXssMatchSetResponse](
"updateXssMatchSet",
api.updateXssMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UpdateXssMatchSetResponse.wrap)
.provideEnvironment(r)
def deleteLoggingConfiguration(
request: zio.aws.wafregional.model.DeleteLoggingConfigurationRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteLoggingConfigurationResponse.ReadOnly
] = asyncRequestResponse[
DeleteLoggingConfigurationRequest,
DeleteLoggingConfigurationResponse
]("deleteLoggingConfiguration", api.deleteLoggingConfiguration)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.DeleteLoggingConfigurationResponse.wrap)
.provideEnvironment(r)
def getGeoMatchSet(
request: zio.aws.wafregional.model.GetGeoMatchSetRequest
): IO[AwsError, zio.aws.wafregional.model.GetGeoMatchSetResponse.ReadOnly] =
asyncRequestResponse[GetGeoMatchSetRequest, GetGeoMatchSetResponse](
"getGeoMatchSet",
api.getGeoMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetGeoMatchSetResponse.wrap)
.provideEnvironment(r)
def updateSizeConstraintSet(
request: zio.aws.wafregional.model.UpdateSizeConstraintSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateSizeConstraintSetResponse.ReadOnly
] = asyncRequestResponse[
UpdateSizeConstraintSetRequest,
UpdateSizeConstraintSetResponse
]("updateSizeConstraintSet", api.updateSizeConstraintSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.UpdateSizeConstraintSetResponse.wrap)
.provideEnvironment(r)
def listRateBasedRules(
request: zio.aws.wafregional.model.ListRateBasedRulesRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListRateBasedRulesResponse.ReadOnly
] = asyncRequestResponse[
ListRateBasedRulesRequest,
ListRateBasedRulesResponse
]("listRateBasedRules", api.listRateBasedRules)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListRateBasedRulesResponse.wrap)
.provideEnvironment(r)
def updateRuleGroup(
request: zio.aws.wafregional.model.UpdateRuleGroupRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateRuleGroupResponse.ReadOnly
] = asyncRequestResponse[UpdateRuleGroupRequest, UpdateRuleGroupResponse](
"updateRuleGroup",
api.updateRuleGroup
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UpdateRuleGroupResponse.wrap)
.provideEnvironment(r)
def updateRegexPatternSet(
request: zio.aws.wafregional.model.UpdateRegexPatternSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.UpdateRegexPatternSetResponse.ReadOnly
] = asyncRequestResponse[
UpdateRegexPatternSetRequest,
UpdateRegexPatternSetResponse
]("updateRegexPatternSet", api.updateRegexPatternSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.UpdateRegexPatternSetResponse.wrap)
.provideEnvironment(r)
def createWebACL(
request: zio.aws.wafregional.model.CreateWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.CreateWebAclResponse.ReadOnly] =
asyncRequestResponse[CreateWebAclRequest, CreateWebAclResponse](
"createWebACL",
api.createWebACL
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.CreateWebAclResponse.wrap)
.provideEnvironment(r)
def createGeoMatchSet(
request: zio.aws.wafregional.model.CreateGeoMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateGeoMatchSetResponse.ReadOnly
] =
asyncRequestResponse[CreateGeoMatchSetRequest, CreateGeoMatchSetResponse](
"createGeoMatchSet",
api.createGeoMatchSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.CreateGeoMatchSetResponse.wrap)
.provideEnvironment(r)
def getPermissionPolicy(
request: zio.aws.wafregional.model.GetPermissionPolicyRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetPermissionPolicyResponse.ReadOnly
] = asyncRequestResponse[
GetPermissionPolicyRequest,
GetPermissionPolicyResponse
]("getPermissionPolicy", api.getPermissionPolicy)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetPermissionPolicyResponse.wrap)
.provideEnvironment(r)
def updateWebACL(
request: zio.aws.wafregional.model.UpdateWebAclRequest
): IO[AwsError, zio.aws.wafregional.model.UpdateWebAclResponse.ReadOnly] =
asyncRequestResponse[UpdateWebAclRequest, UpdateWebAclResponse](
"updateWebACL",
api.updateWebACL
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.UpdateWebAclResponse.wrap)
.provideEnvironment(r)
def deleteRuleGroup(
request: zio.aws.wafregional.model.DeleteRuleGroupRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteRuleGroupResponse.ReadOnly
] = asyncRequestResponse[DeleteRuleGroupRequest, DeleteRuleGroupResponse](
"deleteRuleGroup",
api.deleteRuleGroup
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DeleteRuleGroupResponse.wrap)
.provideEnvironment(r)
def deleteByteMatchSet(
request: zio.aws.wafregional.model.DeleteByteMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteByteMatchSetResponse.ReadOnly
] = asyncRequestResponse[
DeleteByteMatchSetRequest,
DeleteByteMatchSetResponse
]("deleteByteMatchSet", api.deleteByteMatchSet)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DeleteByteMatchSetResponse.wrap)
.provideEnvironment(r)
def getChangeToken(
request: zio.aws.wafregional.model.GetChangeTokenRequest
): IO[AwsError, zio.aws.wafregional.model.GetChangeTokenResponse.ReadOnly] =
asyncRequestResponse[GetChangeTokenRequest, GetChangeTokenResponse](
"getChangeToken",
api.getChangeToken
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetChangeTokenResponse.wrap)
.provideEnvironment(r)
def deleteRegexMatchSet(
request: zio.aws.wafregional.model.DeleteRegexMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteRegexMatchSetResponse.ReadOnly
] = asyncRequestResponse[
DeleteRegexMatchSetRequest,
DeleteRegexMatchSetResponse
]("deleteRegexMatchSet", api.deleteRegexMatchSet)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DeleteRegexMatchSetResponse.wrap)
.provideEnvironment(r)
def listIPSets(
request: zio.aws.wafregional.model.ListIpSetsRequest
): IO[AwsError, zio.aws.wafregional.model.ListIpSetsResponse.ReadOnly] =
asyncRequestResponse[ListIpSetsRequest, ListIpSetsResponse](
"listIPSets",
api.listIPSets
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.ListIpSetsResponse.wrap)
.provideEnvironment(r)
def deleteSizeConstraintSet(
request: zio.aws.wafregional.model.DeleteSizeConstraintSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteSizeConstraintSetResponse.ReadOnly
] = asyncRequestResponse[
DeleteSizeConstraintSetRequest,
DeleteSizeConstraintSetResponse
]("deleteSizeConstraintSet", api.deleteSizeConstraintSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.DeleteSizeConstraintSetResponse.wrap)
.provideEnvironment(r)
def getSampledRequests(
request: zio.aws.wafregional.model.GetSampledRequestsRequest
): IO[
AwsError,
zio.aws.wafregional.model.GetSampledRequestsResponse.ReadOnly
] = asyncRequestResponse[
GetSampledRequestsRequest,
GetSampledRequestsResponse
]("getSampledRequests", api.getSampledRequests)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetSampledRequestsResponse.wrap)
.provideEnvironment(r)
def listActivatedRulesInRuleGroup(
request: zio.aws.wafregional.model.ListActivatedRulesInRuleGroupRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListActivatedRulesInRuleGroupResponse.ReadOnly
] = asyncRequestResponse[
ListActivatedRulesInRuleGroupRequest,
ListActivatedRulesInRuleGroupResponse
]("listActivatedRulesInRuleGroup", api.listActivatedRulesInRuleGroup)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.ListActivatedRulesInRuleGroupResponse.wrap)
.provideEnvironment(r)
def deleteIPSet(
request: zio.aws.wafregional.model.DeleteIpSetRequest
): IO[AwsError, zio.aws.wafregional.model.DeleteIpSetResponse.ReadOnly] =
asyncRequestResponse[DeleteIpSetRequest, DeleteIpSetResponse](
"deleteIPSet",
api.deleteIPSet
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.DeleteIpSetResponse.wrap)
.provideEnvironment(r)
def deletePermissionPolicy(
request: zio.aws.wafregional.model.DeletePermissionPolicyRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeletePermissionPolicyResponse.ReadOnly
] = asyncRequestResponse[
DeletePermissionPolicyRequest,
DeletePermissionPolicyResponse
]("deletePermissionPolicy", api.deletePermissionPolicy)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.DeletePermissionPolicyResponse.wrap)
.provideEnvironment(r)
def deleteSqlInjectionMatchSet(
request: zio.aws.wafregional.model.DeleteSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.DeleteSqlInjectionMatchSetResponse.ReadOnly
] = asyncRequestResponse[
DeleteSqlInjectionMatchSetRequest,
DeleteSqlInjectionMatchSetResponse
]("deleteSqlInjectionMatchSet", api.deleteSqlInjectionMatchSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.DeleteSqlInjectionMatchSetResponse.wrap)
.provideEnvironment(r)
def listSqlInjectionMatchSets(
request: zio.aws.wafregional.model.ListSqlInjectionMatchSetsRequest
): IO[
AwsError,
zio.aws.wafregional.model.ListSqlInjectionMatchSetsResponse.ReadOnly
] = asyncRequestResponse[
ListSqlInjectionMatchSetsRequest,
ListSqlInjectionMatchSetsResponse
]("listSqlInjectionMatchSets", api.listSqlInjectionMatchSets)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.ListSqlInjectionMatchSetsResponse.wrap)
.provideEnvironment(r)
def getRule(
request: zio.aws.wafregional.model.GetRuleRequest
): IO[AwsError, zio.aws.wafregional.model.GetRuleResponse.ReadOnly] =
asyncRequestResponse[GetRuleRequest, GetRuleResponse](
"getRule",
api.getRule
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.GetRuleResponse.wrap)
.provideEnvironment(r)
def createWebACLMigrationStack(
request: zio.aws.wafregional.model.CreateWebAclMigrationStackRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateWebAclMigrationStackResponse.ReadOnly
] = asyncRequestResponse[
CreateWebAclMigrationStackRequest,
CreateWebAclMigrationStackResponse
]("createWebACLMigrationStack", api.createWebACLMigrationStack)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.CreateWebAclMigrationStackResponse.wrap)
.provideEnvironment(r)
def createSqlInjectionMatchSet(
request: zio.aws.wafregional.model.CreateSqlInjectionMatchSetRequest
): IO[
AwsError,
zio.aws.wafregional.model.CreateSqlInjectionMatchSetResponse.ReadOnly
] = asyncRequestResponse[
CreateSqlInjectionMatchSetRequest,
CreateSqlInjectionMatchSetResponse
]("createSqlInjectionMatchSet", api.createSqlInjectionMatchSet)(
request.buildAwsValue()
).map(zio.aws.wafregional.model.CreateSqlInjectionMatchSetResponse.wrap)
.provideEnvironment(r)
def createRule(
request: zio.aws.wafregional.model.CreateRuleRequest
): IO[AwsError, zio.aws.wafregional.model.CreateRuleResponse.ReadOnly] =
asyncRequestResponse[CreateRuleRequest, CreateRuleResponse](
"createRule",
api.createRule
)(request.buildAwsValue())
.map(zio.aws.wafregional.model.CreateRuleResponse.wrap)
.provideEnvironment(r)
}
def listXssMatchSets(
request: zio.aws.wafregional.model.ListXssMatchSetsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListXssMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listXssMatchSets(request))
def getRuleGroup(request: zio.aws.wafregional.model.GetRuleGroupRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRuleGroup(request))
def listGeoMatchSets(
request: zio.aws.wafregional.model.ListGeoMatchSetsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListGeoMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listGeoMatchSets(request))
def updateByteMatchSet(
request: zio.aws.wafregional.model.UpdateByteMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateByteMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateByteMatchSet(request))
def updateRegexMatchSet(
request: zio.aws.wafregional.model.UpdateRegexMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateRegexMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateRegexMatchSet(request))
def createRegexMatchSet(
request: zio.aws.wafregional.model.CreateRegexMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateRegexMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRegexMatchSet(request))
def getWebACL(request: zio.aws.wafregional.model.GetWebAclRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getWebACL(request))
def getRateBasedRule(
request: zio.aws.wafregional.model.GetRateBasedRuleRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetRateBasedRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRateBasedRule(request))
def listSizeConstraintSets(
request: zio.aws.wafregional.model.ListSizeConstraintSetsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListSizeConstraintSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listSizeConstraintSets(request))
def listRegexMatchSets(
request: zio.aws.wafregional.model.ListRegexMatchSetsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListRegexMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRegexMatchSets(request))
def getRegexMatchSet(
request: zio.aws.wafregional.model.GetRegexMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetRegexMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRegexMatchSet(request))
def listSubscribedRuleGroups(
request: zio.aws.wafregional.model.ListSubscribedRuleGroupsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListSubscribedRuleGroupsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listSubscribedRuleGroups(request))
def getSqlInjectionMatchSet(
request: zio.aws.wafregional.model.GetSqlInjectionMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetSqlInjectionMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getSqlInjectionMatchSet(request))
def getIPSet(request: zio.aws.wafregional.model.GetIpSetRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetIpSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getIPSet(request))
def createRuleGroup(
request: zio.aws.wafregional.model.CreateRuleGroupRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRuleGroup(request))
def listRuleGroups(
request: zio.aws.wafregional.model.ListRuleGroupsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListRuleGroupsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRuleGroups(request))
def deleteRegexPatternSet(
request: zio.aws.wafregional.model.DeleteRegexPatternSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteRegexPatternSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRegexPatternSet(request))
def updateRateBasedRule(
request: zio.aws.wafregional.model.UpdateRateBasedRuleRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateRateBasedRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateRateBasedRule(request))
def disassociateWebACL(
request: zio.aws.wafregional.model.DisassociateWebAclRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DisassociateWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.disassociateWebACL(request))
def listResourcesForWebACL(
request: zio.aws.wafregional.model.ListResourcesForWebAclRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListResourcesForWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listResourcesForWebACL(request))
def updateSqlInjectionMatchSet(
request: zio.aws.wafregional.model.UpdateSqlInjectionMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateSqlInjectionMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateSqlInjectionMatchSet(request))
def deleteGeoMatchSet(
request: zio.aws.wafregional.model.DeleteGeoMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteGeoMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteGeoMatchSet(request))
def createIPSet(request: zio.aws.wafregional.model.CreateIpSetRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateIpSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createIPSet(request))
def getWebACLForResource(
request: zio.aws.wafregional.model.GetWebAclForResourceRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetWebAclForResourceResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getWebACLForResource(request))
def getRateBasedRuleManagedKeys(
request: zio.aws.wafregional.model.GetRateBasedRuleManagedKeysRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetRateBasedRuleManagedKeysResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRateBasedRuleManagedKeys(request))
def updateGeoMatchSet(
request: zio.aws.wafregional.model.UpdateGeoMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateGeoMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateGeoMatchSet(request))
def listRegexPatternSets(
request: zio.aws.wafregional.model.ListRegexPatternSetsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListRegexPatternSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRegexPatternSets(request))
def listByteMatchSets(
request: zio.aws.wafregional.model.ListByteMatchSetsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListByteMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listByteMatchSets(request))
def updateIPSet(request: zio.aws.wafregional.model.UpdateIpSetRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateIpSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateIPSet(request))
def createRateBasedRule(
request: zio.aws.wafregional.model.CreateRateBasedRuleRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateRateBasedRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRateBasedRule(request))
def deleteXssMatchSet(
request: zio.aws.wafregional.model.DeleteXssMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteXssMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteXssMatchSet(request))
def getByteMatchSet(
request: zio.aws.wafregional.model.GetByteMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetByteMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getByteMatchSet(request))
def deleteRule(request: zio.aws.wafregional.model.DeleteRuleRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRule(request))
def getSizeConstraintSet(
request: zio.aws.wafregional.model.GetSizeConstraintSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetSizeConstraintSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getSizeConstraintSet(request))
def createByteMatchSet(
request: zio.aws.wafregional.model.CreateByteMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateByteMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createByteMatchSet(request))
def updateRule(request: zio.aws.wafregional.model.UpdateRuleRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateRule(request))
def associateWebACL(
request: zio.aws.wafregional.model.AssociateWebAclRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.AssociateWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.associateWebACL(request))
def getRegexPatternSet(
request: zio.aws.wafregional.model.GetRegexPatternSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetRegexPatternSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRegexPatternSet(request))
def untagResource(
request: zio.aws.wafregional.model.UntagResourceRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UntagResourceResponse.ReadOnly
] = ZIO.serviceWithZIO(_.untagResource(request))
def deleteRateBasedRule(
request: zio.aws.wafregional.model.DeleteRateBasedRuleRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteRateBasedRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRateBasedRule(request))
def putLoggingConfiguration(
request: zio.aws.wafregional.model.PutLoggingConfigurationRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.PutLoggingConfigurationResponse.ReadOnly
] = ZIO.serviceWithZIO(_.putLoggingConfiguration(request))
def getXssMatchSet(
request: zio.aws.wafregional.model.GetXssMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetXssMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getXssMatchSet(request))
def deleteWebACL(request: zio.aws.wafregional.model.DeleteWebAclRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteWebACL(request))
def createRegexPatternSet(
request: zio.aws.wafregional.model.CreateRegexPatternSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateRegexPatternSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRegexPatternSet(request))
def listWebACLs(request: zio.aws.wafregional.model.ListWebAcLsRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListWebAcLsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listWebACLs(request))
def putPermissionPolicy(
request: zio.aws.wafregional.model.PutPermissionPolicyRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.PutPermissionPolicyResponse.ReadOnly
] = ZIO.serviceWithZIO(_.putPermissionPolicy(request))
def createXssMatchSet(
request: zio.aws.wafregional.model.CreateXssMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateXssMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createXssMatchSet(request))
def getChangeTokenStatus(
request: zio.aws.wafregional.model.GetChangeTokenStatusRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetChangeTokenStatusResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getChangeTokenStatus(request))
def createSizeConstraintSet(
request: zio.aws.wafregional.model.CreateSizeConstraintSetRequest
): ZIO[zio.aws.wafregional.WafRegional, AwsError, ReadOnly] =
ZIO.serviceWithZIO(_.createSizeConstraintSet(request))
def getLoggingConfiguration(
request: zio.aws.wafregional.model.GetLoggingConfigurationRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetLoggingConfigurationResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getLoggingConfiguration(request))
def listTagsForResource(
request: zio.aws.wafregional.model.ListTagsForResourceRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListTagsForResourceResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listTagsForResource(request))
def tagResource(request: zio.aws.wafregional.model.TagResourceRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.TagResourceResponse.ReadOnly
] = ZIO.serviceWithZIO(_.tagResource(request))
def listRules(request: zio.aws.wafregional.model.ListRulesRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListRulesResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRules(request))
def listLoggingConfigurations(
request: zio.aws.wafregional.model.ListLoggingConfigurationsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListLoggingConfigurationsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listLoggingConfigurations(request))
def updateXssMatchSet(
request: zio.aws.wafregional.model.UpdateXssMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateXssMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateXssMatchSet(request))
def deleteLoggingConfiguration(
request: zio.aws.wafregional.model.DeleteLoggingConfigurationRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteLoggingConfigurationResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteLoggingConfiguration(request))
def getGeoMatchSet(
request: zio.aws.wafregional.model.GetGeoMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetGeoMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getGeoMatchSet(request))
def updateSizeConstraintSet(
request: zio.aws.wafregional.model.UpdateSizeConstraintSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateSizeConstraintSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateSizeConstraintSet(request))
def listRateBasedRules(
request: zio.aws.wafregional.model.ListRateBasedRulesRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListRateBasedRulesResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listRateBasedRules(request))
def updateRuleGroup(
request: zio.aws.wafregional.model.UpdateRuleGroupRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateRuleGroup(request))
def updateRegexPatternSet(
request: zio.aws.wafregional.model.UpdateRegexPatternSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateRegexPatternSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateRegexPatternSet(request))
def createWebACL(request: zio.aws.wafregional.model.CreateWebAclRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createWebACL(request))
def createGeoMatchSet(
request: zio.aws.wafregional.model.CreateGeoMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateGeoMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createGeoMatchSet(request))
def getPermissionPolicy(
request: zio.aws.wafregional.model.GetPermissionPolicyRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetPermissionPolicyResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getPermissionPolicy(request))
def updateWebACL(request: zio.aws.wafregional.model.UpdateWebAclRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.UpdateWebAclResponse.ReadOnly
] = ZIO.serviceWithZIO(_.updateWebACL(request))
def deleteRuleGroup(
request: zio.aws.wafregional.model.DeleteRuleGroupRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRuleGroup(request))
def deleteByteMatchSet(
request: zio.aws.wafregional.model.DeleteByteMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteByteMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteByteMatchSet(request))
def getChangeToken(
request: zio.aws.wafregional.model.GetChangeTokenRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetChangeTokenResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getChangeToken(request))
def deleteRegexMatchSet(
request: zio.aws.wafregional.model.DeleteRegexMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteRegexMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteRegexMatchSet(request))
def listIPSets(request: zio.aws.wafregional.model.ListIpSetsRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListIpSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listIPSets(request))
def deleteSizeConstraintSet(
request: zio.aws.wafregional.model.DeleteSizeConstraintSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteSizeConstraintSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteSizeConstraintSet(request))
def getSampledRequests(
request: zio.aws.wafregional.model.GetSampledRequestsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetSampledRequestsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getSampledRequests(request))
def listActivatedRulesInRuleGroup(
request: zio.aws.wafregional.model.ListActivatedRulesInRuleGroupRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListActivatedRulesInRuleGroupResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listActivatedRulesInRuleGroup(request))
def deleteIPSet(request: zio.aws.wafregional.model.DeleteIpSetRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteIpSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteIPSet(request))
def deletePermissionPolicy(
request: zio.aws.wafregional.model.DeletePermissionPolicyRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeletePermissionPolicyResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deletePermissionPolicy(request))
def deleteSqlInjectionMatchSet(
request: zio.aws.wafregional.model.DeleteSqlInjectionMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.DeleteSqlInjectionMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.deleteSqlInjectionMatchSet(request))
def listSqlInjectionMatchSets(
request: zio.aws.wafregional.model.ListSqlInjectionMatchSetsRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.ListSqlInjectionMatchSetsResponse.ReadOnly
] = ZIO.serviceWithZIO(_.listSqlInjectionMatchSets(request))
def getRule(request: zio.aws.wafregional.model.GetRuleRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.GetRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.getRule(request))
def createWebACLMigrationStack(
request: zio.aws.wafregional.model.CreateWebAclMigrationStackRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateWebAclMigrationStackResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createWebACLMigrationStack(request))
def createSqlInjectionMatchSet(
request: zio.aws.wafregional.model.CreateSqlInjectionMatchSetRequest
): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateSqlInjectionMatchSetResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createSqlInjectionMatchSet(request))
def createRule(request: zio.aws.wafregional.model.CreateRuleRequest): ZIO[
zio.aws.wafregional.WafRegional,
AwsError,
zio.aws.wafregional.model.CreateRuleResponse.ReadOnly
] = ZIO.serviceWithZIO(_.createRule(request))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy