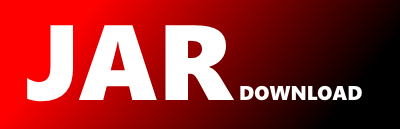
digital.nedra.commons.starter.witsml.clt.service.WitsmlGatewayService Maven / Gradle / Ivy
/*
* Copyright 2023 Nedra Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package digital.nedra.commons.starter.witsml.clt.service;
import static digital.nedra.commons.starter.witsml.clt.constant.ConstantMessages.SOAP_ACTION;
import static digital.nedra.commons.starter.witsml.clt.constant.ExceptionMessages.WITSML_RESPONSE_ERROR;
import digital.nedra.commons.starter.witsml.clt.config.WitsmlServerProperties;
import digital.nedra.commons.starter.witsml.clt.exception.WitsmlClientException;
import digital.nedra.commons.starter.witsml.clt.model.soap.WmlsGetFromStore;
import digital.nedra.commons.starter.witsml.clt.model.soap.WmlsGetFromStoreResponse;
import digital.nedra.commons.starter.witsml.clt.validator.SaxParserValidator;
import java.util.Optional;
import org.springframework.oxm.jaxb.Jaxb2Marshaller;
import org.springframework.stereotype.Service;
import org.springframework.ws.client.core.support.WebServiceGatewaySupport;
import org.springframework.ws.soap.client.core.SoapActionCallback;
import org.springframework.ws.transport.WebServiceMessageSender;
@Service
public class WitsmlGatewayService extends WebServiceGatewaySupport {
protected final WitsmlServerProperties witsmlServerProperties;
private final SaxParserValidator saxParserValidator;
private final SoapActionCallback soapActionCallback = new SoapActionCallback(SOAP_ACTION);
public WitsmlGatewayService(
WitsmlServerProperties witsmlServerProperties,
WebServiceMessageSender messageSender,
Jaxb2Marshaller marshaller,
SaxParserValidator validator) {
this.witsmlServerProperties = witsmlServerProperties;
this.saxParserValidator = validator;
setMessageSender(messageSender);
setMarshaller(marshaller);
setUnmarshaller(marshaller);
}
public WmlsGetFromStore prepareGetObject(String requestWitsmlString) {
saxParserValidator.validate(requestWitsmlString);
String objName = requestWitsmlString
.substring(1, requestWitsmlString.indexOf(" ") - 1);
var fromStore = new WmlsGetFromStore();
fromStore.setWmlTypeIn(objName);
fromStore.setQueryIn(requestWitsmlString);
return fromStore;
}
public Optional getFromStoreRawString(WmlsGetFromStore request) {
try {
WmlsGetFromStoreResponse response = marshalSendAndReceive(request);
String xmlOut = response.getXmlOut();
return Optional.ofNullable(xmlOut);
} catch (Exception e) {
throw new WitsmlClientException(WITSML_RESPONSE_ERROR + ": " + e.getMessage());
}
}
private WmlsGetFromStoreResponse marshalSendAndReceive(WmlsGetFromStore request) {
return (WmlsGetFromStoreResponse) getWebServiceTemplate().marshalSendAndReceive(
witsmlServerProperties.getUrl().toString(),
request,
soapActionCallback);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy