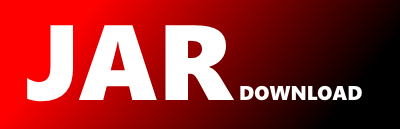
digital.nedra.commons.starter.witsml.clt.transport.TokenProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of witsml-clt-starter Show documentation
Show all versions of witsml-clt-starter Show documentation
Spring Boot starter for WITSML connection.
/*
* Copyright 2023 Nedra Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package digital.nedra.commons.starter.witsml.clt.transport;
import static digital.nedra.commons.starter.witsml.clt.constant.ConstantMessages.AUTH_URI;
import static digital.nedra.commons.starter.witsml.clt.constant.ConstantMessages.GRANTTYPE_PLACEHOLDER;
import static digital.nedra.commons.starter.witsml.clt.constant.ConstantMessages.PASSWORD_PLACEHOLDER;
import static digital.nedra.commons.starter.witsml.clt.constant.ConstantMessages.USERNAME_PLACEHOLDER;
import static digital.nedra.commons.starter.witsml.clt.constant.ExceptionMessages.AUTH_TOKEN_ERROR;
import digital.nedra.commons.starter.witsml.clt.config.WitsmlServerProperties;
import digital.nedra.commons.starter.witsml.clt.exception.WitsmlAuthorizationTokenException;
import lombok.RequiredArgsConstructor;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Component;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestTemplate;
@Component
@RequiredArgsConstructor
public class TokenProvider {
private final WitsmlServerProperties witsmlServerProperties;
private final RestTemplate restTemplate = new RestTemplate();
public String getAuthorizationToken() {
try {
MultiValueMap body = getBody();
HttpHeaders headers = getHeaders();
var httpEntity = new HttpEntity<>(body, headers);
String authUrl = witsmlServerProperties.getUrl() + AUTH_URI;
return restTemplate.postForEntity(authUrl, httpEntity, String.class).getBody();
} catch (Exception e) {
throw new WitsmlAuthorizationTokenException(AUTH_TOKEN_ERROR + ": " + e.getMessage());
}
}
private MultiValueMap getBody() {
var formData = new LinkedMultiValueMap();
formData.add(USERNAME_PLACEHOLDER, witsmlServerProperties.getUsername());
formData.add(PASSWORD_PLACEHOLDER, witsmlServerProperties.getPassword());
formData.add(GRANTTYPE_PLACEHOLDER, PASSWORD_PLACEHOLDER);
return formData;
}
private HttpHeaders getHeaders() {
var headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_FORM_URLENCODED);
return headers;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy