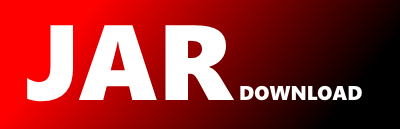
dk.apaq.crud.core.BaseCrud Maven / Gradle / Ivy
/*
* CrudContainer
* Copyright (C) 2011 by Apaq
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package dk.apaq.crud.core;
import dk.apaq.crud.Crud;
import dk.apaq.crud.CrudEvent;
import dk.apaq.crud.CrudListener;
import dk.apaq.crud.CrudNotifier;
import java.lang.ref.WeakReference;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
/**
* An abstract implementation of a Crud
instance taking care
* of CrudNotifer logic. An implementing class needs only to call the fire* methods(fx. fireOnRead)
* and this class will make sure to fire evetns on the listeners.
*/
public abstract class BaseCrud implements
Crud, CrudNotifier {
private final List> listeners = new ArrayList>();
/**
* {@inheritDoc}
*/
@Override
public void addListener(CrudListener listener) {
WeakReference refListener = new WeakReference(listener);
listeners.add(refListener);
}
/**
* {@inheritDoc}
*/
@Override
public void removeListener(CrudListener listener) {
synchronized(listeners) {
Iterator> it = listeners.iterator();
while(it.hasNext()) {
WeakReference refListener = it.next();
if(refListener.get() == listener) {
it.remove();
break;
}
}
}
}
protected List getListeners() {
List list = new ArrayList();
Iterator> it = listeners.iterator();
while(it.hasNext()) {
WeakReference refListener = it.next();
if(refListener.get() == null) {
it.remove();
} else {
list.add(refListener.get());
}
}
return Collections.unmodifiableList(list);
}
public List listIds() {
return listIds(null);
}
public List list() {
return list(null);
}
/**
* Tells listeners that an entity has been read from crud.
* @param entity The entity that was read.
*/
protected void fireOnRead(IDT id, BT entity) {
CrudEvent.WithIdAndEntity e = createEventWithIdAndEntity(id, entity);
for (CrudListener listener : getListeners()) {
listener.onEntityRead(e);
}
}
/**
* Tells listeners that an entity is about tobe read.
* @param id The id of the entity that is to be read.
*/
protected void fireOnBeforeRead(IDT id) {
CrudEvent.WithId e = createEventWithId(id);
for (CrudListener listener : getListeners()) {
listener.onBeforeEntityRead(e);
}
}
protected CrudEvent createEvent() {
return new CrudEvent(this);
}
protected CrudEvent.WithId createEventWithId(IDT id) {
return new CrudEvent.WithId(this, id);
}
protected CrudEvent.WithEntity createEventWithEntity(BT entity) {
return new CrudEvent.WithEntity(this,entity);
}
protected CrudEvent.WithIdAndEntity createEventWithIdAndEntity(IDT id, BT entity) {
return new CrudEvent.WithIdAndEntity(this, id, entity);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy