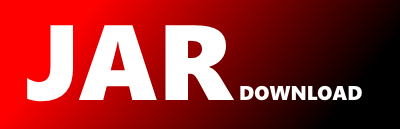
dk.clanie.test.logging.CapturedLoggingEvents Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2008, Claus Nielsen, [email protected]
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along
* with this program; if not, write to the Free Software Foundation, Inc.,
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
*/
package dk.clanie.test.logging;
import static dk.clanie.collections.CollectionFactory.newArrayList;
import java.util.Collections;
import java.util.List;
import org.hamcrest.Matcher;
import ch.qos.logback.classic.Level;
import ch.qos.logback.classic.spi.ILoggingEvent;
import ch.qos.logback.classic.spi.ThrowableProxy;
/**
* Immutable collection of LoggingEvents captured by {@link LogCapturingTestTemplate}.
*
* @author Claus Nielsen
*/
public class CapturedLoggingEvents {
final List events;
CapturedLoggingEvents(List events) {
this.events = Collections.unmodifiableList(events);
}
/**
* Returns a new CapturedLoggingEvents instance containing the subset of
* LoggingEvents which satisfies the supplied Matcher.
*
* @param matcher
* @return CapturedLoggingEvents
*/
public CapturedLoggingEvents filter(Matcher matcher) {
List matchingEvents = newArrayList();
for (ILoggingEvent event : events) {
if (matcher.matches(event))
matchingEvents.add(event);
}
return new CapturedLoggingEvents(matchingEvents);
}
/**
* Gets all LoggingEvents.
*
* @return List<LoggingEvent>
*/
public List getEvents() {
return events;
}
/**
* Gets all LoggingEvents which satisfies the supplied Matcher.
*
* @param matcher
* @return List<LoggingEvent>
*/
public List getEvents(Matcher matcher) {
return filter(matcher).getEvents();
}
/**
* Gets messages from all LoggingEvents.
*
* @return List<String>
*/
public List getMessages() {
List messages = newArrayList();
for (ILoggingEvent event : events) {
messages.add(event.getMessage());
}
return messages;
}
/**
* Gets messages from all LoggingEvents which satisfies the supplied Matcher.
*
* @param matcher
* @return List<String>
*/
public List getMessages(Matcher matcher) {
return filter(matcher).getMessages();
}
/**
* Gets Thowables from all LoggingEvents.
*
* Note that not all LoggingEvents have Throwables and therefore the
* returned Collection may be less than the number of LoggingEvents.
*
* @return List<Throwable>
*/
public List getThrowables() {
List throwables = newArrayList();
for (ILoggingEvent event : events) {
ThrowableProxy throwableProxy = (ThrowableProxy) event.getThrowableProxy();
if (throwableProxy != null)
throwables.add(throwableProxy.getThrowable());
}
return throwables;
}
/**
* Gets Thowables from all LoggingEvents which satisfies the supplied Matcher.
*
* @param matcher
* @return List<Throwable>
*/
public List getThrowables(Matcher matcher) {
return filter(matcher).getThrowables();
}
/**
* Gets Levels from all LoggingEvents.
*
* @return List<Level>
*/
public List getLevels() {
List levels = newArrayList();
for (ILoggingEvent event : events) {
levels.add(event.getLevel());
}
return levels;
}
/**
* Gets Levels from all LoggingEvents which satisfies the supplied Matcher.
*
* @param matcher
* @return List<Level>
*/
public List getLevels(Matcher matcher) {
return filter(matcher).getLevels();
}
/**
* Gets the number of LoggingEvents.
*
* @return the number of LoggingEvents.
*/
public Integer getSize() {
return events.size();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy