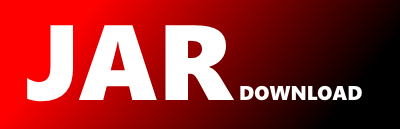
dk.cloudcreate.essentials.components.eventsourced.aggregates.decider.Decider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eventsourced-aggregates Show documentation
Show all versions of eventsourced-aggregates Show documentation
This library focuses on providing different flavors of eventsourced aggregrates
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package dk.cloudcreate.essentials.components.eventsourced.aggregates.decider;
import static dk.cloudcreate.essentials.shared.FailFast.requireNonNull;
/**
* Variant of the event sourced Decider pattern, which supports building an Aggregate STATE
based on previous EVENT
's that relate to the
* aggregate instance, and which can handle COMMAND
's, whose side effect is either an ERROR
or a List of EVENT
's (can be an empty list)
*
* @param The type of Commands that the {@link #handle(Object, Object)} can process
* @param The type of Events that can be returned by {@link #handle(Object, Object)} and applied in the {@link #applyEvent(Object, Object)}
* @param The type of Error that can be returned by the {@link #handle(Object, Object)} method
* @param The type of Aggregate State that this {@link Decider} works with in {@link #handle(Object, Object)}, {@link #initialState()}, {@link #applyEvent(Object, Object)} and {@link #isFinal(Object)}
*/
public interface Decider extends Handler, StateEvolver, InitialStateProvider, IsStateFinalResolver {
/**
* Factory method for creating a {@link Decider} instance from instances of the various {@link Decider} related interfaces
*
* @param handler A {@link Decider} related interface that is responsible for handling COMMAND
(s)
* @param initialStateProvider A {@link Decider} related interface, which provides the Initial STATE
for a given aggregate.
* @param stateEvolver A {@link Decider} related interface, which can apply EVENT
<(s) to an aggregate/i>'s STATE
instance
* @param stateIsStateFinalResolver A {@link Decider} related interface that resolves if the aggregate's state is final and no more changes can occur, i.e. no more events can be persisted
* @param The type of Commands that the {@link #handle(Object, Object)} can process
* @param The type of Events that can be returned by {@link #handle(Object, Object)} and applied in the {@link #applyEvent(Object, Object)}
* @param The type of Error that can be returned by the {@link #handle(Object, Object)} method
* @param The type of Aggregate State that this {@link Decider} works with in {@link #handle(Object, Object)}, {@link #initialState()}, {@link #applyEvent(Object, Object)} and {@link #isFinal(Object)}
* @return a {@link Decider} instance that delegates to the {@link Decider} related instances provided as parameters
*/
static Decider decider(Handler handler,
InitialStateProvider initialStateProvider,
StateEvolver stateEvolver,
IsStateFinalResolver stateIsStateFinalResolver) {
requireNonNull(handler, "No handler provided");
requireNonNull(initialStateProvider, "No initialStateProvider provided");
requireNonNull(stateEvolver, "No stateEvolver provided");
requireNonNull(stateIsStateFinalResolver, "No stateIsStateFinalResolver provided");
return new Decider<>() {
@Override
public STATE applyEvent(EVENT event, STATE state) {
return stateEvolver.applyEvent(event, state);
}
@Override
public STATE initialState() {
return initialStateProvider.initialState();
}
@Override
public HandlerResult handle(COMMAND cmd, STATE state) {
return handler.handle(cmd, state);
}
@Override
public boolean isFinal(STATE state) {
return stateIsStateFinalResolver.isFinal(state);
}
};
}
/**
* Return true from this method IF the aggregate's state is final and no more changes can occur, i.e. no more events can be persisted (i.e. {@link Handler#handle(Object, Object)} will return an ERROR
)
* Note: This method is called isTerminal
in the decider pattern
* See {@link IsStateFinalResolver#isFinal(Object)} for more details.
* The default implementation in the {@link Decider} always return false
*
* @param state the current STATE
of the aggregate
* @return true IF the aggregate's state is final, otherwise false
*/
@Override
default boolean isFinal(STATE state) {
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy