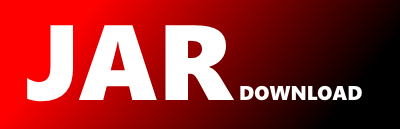
dk.cloudcreate.essentials.shared.FailFast Maven / Gradle / Ivy
/*
* Copyright 2021-2024 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package dk.cloudcreate.essentials.shared;
import java.util.*;
import static dk.cloudcreate.essentials.shared.MessageFormatter.msg;
/**
* Collection of {@link Objects#requireNonNull(Object)} replacement methods
*/
public final class FailFast {
/**
* Assert that the objectThatMustBeAnInstanceOf
is an instance of mustBeAnInstanceOf
parameter, if not then {@link IllegalArgumentException} is thrown
*
* @param objectThatMustBeAnInstanceOf the object that must be an instance of mustBeAnInstanceOf
parameter
* @param mustBeAnInstanceOf the type that the objectThatMustBeAnInstanceOf
parameter must be an instance of
* @param the type of class that the objectThatMustBeAnInstanceOf
must be an instance of
* @return the "objectThatMustBeAnInstanceOf" IF it is of the right type
*/
public static T requireMustBeInstanceOf(Object objectThatMustBeAnInstanceOf, Class mustBeAnInstanceOf) {
return requireMustBeInstanceOf(objectThatMustBeAnInstanceOf, mustBeAnInstanceOf, null);
}
/**
* Assert that the objectThatMustBeAnInstanceOf
is an instance of mustBeAnInstanceOf
parameter, if not then {@link IllegalArgumentException} is thrown
*
* @param objectThatMustBeAnInstanceOf the object that must be an instance of mustBeAnInstanceOf
parameter
* @param mustBeAnInstanceOf the type that the objectThatMustBeAnInstanceOf
parameter must be an instance of
* @param message the optional message that will become the message of the {@link IllegalArgumentException} in case the objectThatMustBeAnInstanceOf
is NOT an instance of mustBeAnInstanceOf
* @param the type of class that the objectThatMustBeAnInstanceOf
must be an instance of
* @return the "objectThatMustBeAnInstanceOf" IF it is of the right type
*/
@SuppressWarnings("unchecked")
public static T requireMustBeInstanceOf(Object objectThatMustBeAnInstanceOf, Class> mustBeAnInstanceOf, String message) {
if (objectThatMustBeAnInstanceOf == null) {
throw new IllegalArgumentException(message != null ? message : "Object was null and therefore not an instance of " + mustBeAnInstanceOf.getName());
}
if (mustBeAnInstanceOf == null) {
throw new IllegalArgumentException(message != null ? message : "Cannot verify instanceOf since the mustBeAnInstanceOf parameter is null");
}
if (!mustBeAnInstanceOf.isAssignableFrom(objectThatMustBeAnInstanceOf.getClass())) {
throw new IllegalArgumentException(message != null ? message : msg("Expected {} to be an instance of {}", objectThatMustBeAnInstanceOf.getClass().getName(), mustBeAnInstanceOf.getName()));
}
return (T) objectThatMustBeAnInstanceOf;
}
/**
* Assert that the objectThatMayNotBeNull
is NOT null. If it's null then an {@link IllegalArgumentException} is thrown
*
* @param objectThatMayNotBeNull the object that must NOT be null
* @param message the optional message that will become the message of the {@link IllegalArgumentException} in case the objectThatMayNotBeNull
is NULL
* @param the type of the objectThatMayNotBeNull
* @return the "objectThatMayNotBeNull" IF it's not null
*/
public static T requireNonNull(T objectThatMayNotBeNull, String message) {
if (objectThatMayNotBeNull == null) {
throw new IllegalArgumentException(message != null ? message : "Object was null, we expected it to be non-null");
}
return objectThatMayNotBeNull;
}
/**
* Assert that the characterStreamThatMustNotBeEmptyOrNull
is NOT null AND NOT empty/blank, otherwise a {@link IllegalArgumentException} is thrown
*
* @param characterStreamThatMustNotBeEmptyOrNull the object that must NOT be null or empty
* @param message the optional message that will become the message of the {@link IllegalArgumentException} in case the characterStreamThatMustNotBeEmptyOrNull
is NULL
* @param the type of the characterStreamThatMustNotBeEmptyOrNull
* @return the "characterStreamThatMustNotBeEmptyOrNull" IF it's not null
*/
public static T requireNonBlank(T characterStreamThatMustNotBeEmptyOrNull, String message) {
if (characterStreamThatMustNotBeEmptyOrNull == null) {
throw new IllegalArgumentException(message != null ? message : "Character sequence was null, we expected it to be non-null and not empty");
}
if (characterStreamThatMustNotBeEmptyOrNull.length() == 0) {
throw new IllegalArgumentException(message != null ? message : "Character sequence was empty, we expected it to be non empty");
}
return characterStreamThatMustNotBeEmptyOrNull;
}
/**
* Assert that the objectThatMayNotBeNull
is NOT null. If it's null then an {@link IllegalArgumentException} is thrown
* Short handle for calling {@code requireNonNull(someObjectThatMustNotBeNull, m("Message with {} placeholder", someMessageObject))} - (see {@link MessageFormatter#msg(String, Object...)})
*
* @param objectThatMayNotBeNull the object that must NOT be null
* @param message the optional message that will become the message of the {@link IllegalArgumentException} in case the objectThatMayNotBeNull
is NULL
* @param messageArguments any placeholders for the message (see {@link MessageFormatter#msg(String, Object...)})
* @param the type of the objectThatMayNotBeNull
* @return the "objectThatMayNotBeNull" IF it's not null
*/
public static T requireNonNull(T objectThatMayNotBeNull, String message, Object... messageArguments) {
if (objectThatMayNotBeNull == null) {
throw new IllegalArgumentException(message != null ? msg(message, messageArguments) : "Object was null, we expected it to be non-null");
}
return objectThatMayNotBeNull;
}
/**
* Assert that the objectThatMayNotBeNull
is NOT null. If it's null then an {@link IllegalArgumentException} is thrown
*
* @param objectThatMayNotBeNull the object that must NOT be null
* @param the type of the objectThatMayNotBeNull
* @return the "objectThatMayNotBeNull" IF it's not null
*/
public static T requireNonNull(T objectThatMayNotBeNull) {
return requireNonNull(objectThatMayNotBeNull, null);
}
/**
* Assert that the boolean value mustBeTrue
is true
. If not then an {@link IllegalArgumentException} is thrown
*
* @param mustBeTrue boolean value that we will assert MUST be TRUE
* @param message the NON optional message that will become the message of the {@link IllegalArgumentException} in case the mustBeTrue
is FALSE
*/
public static void requireTrue(boolean mustBeTrue, String message) {
requireNonNull(message, "You must provide a NON NULL message argument to requireTrue(boolean, String)");
if (!mustBeTrue) {
throw new IllegalArgumentException(message);
}
}
/**
* Assert that the boolean value mustBeTrue
is true
. If not then an {@link IllegalArgumentException} is thrown
*
* @param mustBeFalse boolean value that we will assert MUST be FALSE
* @param message the NON optional message that will become the message of the {@link IllegalArgumentException} in case the mustBeFalse
is TRUE
*/
public static void requireFalse(boolean mustBeFalse, String message) {
requireNonNull(message, "You must provide a NON NULL message argument to requireFalse(boolean, String)");
if (mustBeFalse) {
throw new IllegalArgumentException(message);
}
}
public static Object[] requireNonEmpty(Object[] items, String message) {
if (items == null || items.length == 0) {
throw new IllegalArgumentException(message);
}
return items;
}
public static List requireNonEmpty(List items, String message) {
if (items == null || items.size() == 0) {
throw new IllegalArgumentException(message);
}
return items;
}
public static Set requireNonEmpty(Set items, String message) {
if (items == null || items.size() == 0) {
throw new IllegalArgumentException(message);
}
return items;
}
public static Map requireNonEmpty(Map items, String message) {
if (items == null || items.size() == 0) {
throw new IllegalArgumentException(message);
}
return items;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy