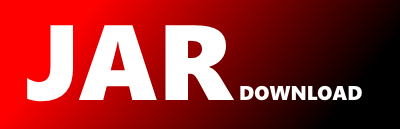
dk.eobjects.metamodel.CompositeDataContextStrategy Maven / Gradle / Ivy
The newest version!
package dk.eobjects.metamodel;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import dk.eobjects.metamodel.data.DataSet;
import dk.eobjects.metamodel.query.FromItem;
import dk.eobjects.metamodel.query.Query;
import dk.eobjects.metamodel.schema.Column;
import dk.eobjects.metamodel.schema.CompositeSchema;
import dk.eobjects.metamodel.schema.Schema;
import dk.eobjects.metamodel.schema.Table;
public class CompositeDataContextStrategy implements IDataContextStrategy {
private final Log _log = LogFactory.getLog(getClass());
private Map _compositeSchemas = new HashMap();
private DataContext[] _delegates;
public CompositeDataContextStrategy(DataContext... delegates) {
if (delegates == null) {
throw new IllegalArgumentException("delegates cannot be null");
}
_delegates = delegates;
}
public CompositeDataContextStrategy(Collection delegates) {
if (delegates == null) {
throw new IllegalArgumentException("delegates cannot be null");
}
_delegates = delegates.toArray(new DataContext[delegates.size()]);
}
public DataSet executeQuery(Query query) throws MetaModelException {
Set dataContexts = new HashSet();
List items = query.getFromClause().getItems();
for (FromItem item : items) {
List tableFromItems = MetaModelHelper
.getTableFromItems(item);
for (FromItem fromItem : tableFromItems) {
Table table = fromItem.getTable();
DataContext dc = getDataContext(table);
dataContexts.add(dc);
}
}
if (dataContexts.isEmpty()) {
throw new MetaModelException(
"No suiting delegate DataContext to execute query: "
+ query);
} else if (dataContexts.size() == 1) {
return dataContexts.iterator().next().executeQuery(query);
} else {
return new QueryPostprocessDataContextStrategy() {
@Override
public DataSet materializeMainSchemaTable(Table table,
Column[] columns, int maxRows) {
DataContext dc = getDataContext(table);
Query q = new Query().select(columns).from(table);
if (maxRows >= 0) {
q.setMaxRows(maxRows);
}
return dc.executeQuery(q);
}
@Override
protected String getMainSchemaName() throws MetaModelException {
throw new UnsupportedOperationException(
"Use CompositeDataContextStrategy for exploring the schema");
}
@Override
protected Schema getMainSchema() throws MetaModelException {
throw new UnsupportedOperationException(
"Use CompositeDataContextStrategy for exploring the schema");
}
}.executeQuery(query);
}
}
private DataContext getDataContext(Table table) {
if (table != null) {
Schema schema = table.getSchema();
if (schema != null) {
for (DataContext dc : _delegates) {
Schema dcSchema = dc.getSchemaByName(schema.getName());
if (dcSchema == schema) {
if (_log.isDebugEnabled()) {
_log.debug("DataContext for " + table
+ " resolved to: " + dcSchema);
}
return dc;
}
}
}
}
_log.warn("Couldn't resolve DataContext for " + table);
return null;
}
public String getDefaultSchemaName() throws MetaModelException {
for (DataContext dc : _delegates) {
Schema schema = dc.getDefaultSchema();
if (schema != null) {
return schema.getName();
}
}
return null;
}
public Schema getSchemaByName(String name) throws MetaModelException {
CompositeSchema compositeSchema = _compositeSchemas.get(name);
if (compositeSchema != null) {
return compositeSchema;
}
List matchingSchemas = new LinkedList();
for (DataContext dc : _delegates) {
Schema schema = dc.getSchemaByName(name);
if (schema != null) {
matchingSchemas.add(schema);
}
}
if (matchingSchemas.size() == 1) {
return matchingSchemas.iterator().next();
}
if (matchingSchemas.size() > 1) {
if (_log.isInfoEnabled()) {
_log.info("Name-clash detected for Schema '" + name
+ "'. Creating CompositeSchema.");
}
compositeSchema = new CompositeSchema(name, matchingSchemas);
_compositeSchemas.put(name, compositeSchema);
return compositeSchema;
}
return null;
}
public String[] getSchemaNames() throws MetaModelException {
Set set = new HashSet();
for (DataContext dc : _delegates) {
String[] schemaNames = dc.getSchemaNames();
for (String name : schemaNames) {
set.add(name);
}
}
String[] result = set.toArray(new String[set.size()]);
Arrays.sort(result);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy