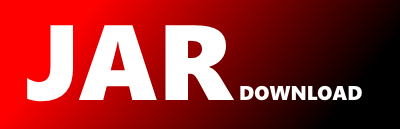
dk.grinn.keycloak.admin.GkcadmConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of keycloak-migrate-core Show documentation
Show all versions of keycloak-migrate-core Show documentation
Keycloak migrate main implementation.
package dk.grinn.keycloak.admin;
/*-
* #%L
* Keycloak : Migrate : Core
* %%
* Copyright (C) 2019 Jonas Grann & Kim Jersin
* %%
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
* #L%
*/
import dk.grinn.keycloak.migration.core.BaseJavaMigration;
import static java.util.Arrays.asList;
import static java.util.Collections.emptyList;
import java.util.List;
import javax.enterprise.context.RequestScoped;
import javax.enterprise.inject.Produces;
import javax.inject.Inject;
import javax.inject.Named;
import org.apache.commons.configuration2.CompositeConfiguration;
import org.apache.commons.configuration2.Configuration;
/**
* Bean configuration representation.
*
* @author Kim Jersin <[email protected]>
*/
@RequestScoped
public class GkcadmConfiguration extends AbstractConfiguration {
private static final String DEFAULT_SUBSET = "gkcadm";
private String location;
private Configuration rootConfig;
private CompositeConfiguration config;
private List scopes;
public GkcadmConfiguration() {
}
@Inject
public void setConfiguration(CompositeConfiguration config) {
this.rootConfig = config.subset(DEFAULT_SUBSET);
}
@Produces
@RequestScoped
@Named("location")
public String getLocation() {
if (location == null) {
throw new IllegalStateException("Location has not been set");
}
return location;
}
public void setLocation(String location) {
this.location = location;
config = new CompositeConfiguration(asList(
rootConfig.subset("location." + location),
rootConfig
));
scopes = config.getList(String.class, "scopes", emptyList());
}
public boolean containsScopeFor(BaseJavaMigration migration) {
// No scope means use always
if (migration.getScope() == null) {
return true;
}
return scopes.contains(migration.getScope());
}
public Configuration getRootConfig() {
return rootConfig;
}
public int getConnectRetry() {
return config.getInt("connect.retry", 0);
}
public boolean isUsePackageAsDefaultScope() {
return "".equals(config.getString("default.scope"));
}
@Override
protected CompositeConfiguration getConfig() {
return config;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy