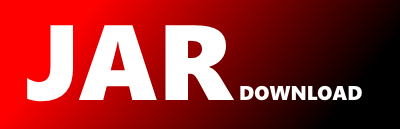
dk.grinn.keycloak.migration.boundary.SessionRessourceImpl Maven / Gradle / Ivy
package dk.grinn.keycloak.migration.boundary;
/*-
* #%L
* Keycloak : Migrate : Resource
* %%
* Copyright (C) 2019 Jonas Grann & Kim Jersin
* %%
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
* #L%
*/
import dk.grinn.keycloak.migration.entities.CreateRealmKey;
import dk.grinn.keycloak.migration.entities.Session;
import org.keycloak.connections.jpa.JpaConnectionProvider;
import org.keycloak.models.KeycloakSession;
import org.keycloak.models.RealmModel;
import javax.annotation.security.RolesAllowed;
import javax.persistence.EntityManager;
import javax.persistence.NoResultException;
import javax.ws.rs.DefaultValue;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Response;
import java.util.List;
import java.util.UUID;
import static dk.grinn.keycloak.migration.resource.RealmAccessAuthFilter.getAuthResult;
import static javax.servlet.http.HttpServletResponse.SC_NOT_FOUND;
/**
* REST end point to {@link RealmHistoryLockController}.
*
* @author @author Kim Jersin <[email protected]>
*/
@RolesAllowed("admin")
public class SessionRessourceImpl implements SessionResource {
private final KeycloakSession session;
private final RealmHistoryLockController controller;
private final RealmKeyController realmKeyController;
public SessionRessourceImpl(KeycloakSession session) {
EntityManager em = session.getProvider(JpaConnectionProvider.class).getEntityManager();
this.controller = new RealmHistoryLockController(em);
this.realmKeyController = new RealmKeyController(em);
this.session = session;
}
/**
* {@inheritDoc}
*/
@Override
public List getSessions(@QueryParam("activeOnly") @DefaultValue("false") boolean activeOnly) {
return controller.getSessions(activeOnly);
}
/**
* {@inheritDoc}
*/
@Override
public Response createSession(CreateSession args) {
UUID sessionId = controller.createSession(args.realm,
getAuthResult(session).getToken().getPreferredUsername()
);
return Response.created(
session.getContext().getUri().getAbsolutePathBuilder().path(sessionId.toString()).build()
).build();
}
/**
* {@inheritDoc}
*/
@Override
public Session getSession(UUID sessionId) {
return controller.get(sessionId).toSession();
}
/**
* {@inheritDoc}
*/
@Override
public Response releaseSession(UUID sessionId) {
return controller.releaseSession(sessionId) == 1
? Response.noContent().build()
: Response.status(SC_NOT_FOUND).build();
}
/**
* {@inheritDoc}
*/
@Override
public ScriptResource scripts(UUID sessionId) {
return new ScriptResourceImpl(session, sessionId);
}
@Override
public Response setRealmKey(UUID sessionId, CreateRealmKey createRealmKey) {
controller.get(sessionId);
RealmModel realmModel = session.realms().getRealm(createRealmKey.getRealmId());
realmKeyController.setRealmKey(realmModel, createRealmKey);
return Response.noContent().build();
}
@Override
public Response disableRsaGeneratedKey(UUID sessionId, String realmId) {
controller.get(sessionId);
RealmKeyController.disableRsaGenerated(session.realms().getRealm(realmId));
return Response.noContent().build();
}
@Override
public Response removeSession(UUID sessionId) {
try {
controller.removeSession(sessionId);
} catch (NoResultException ex) {
return Response.status(SC_NOT_FOUND).build();
}
return Response.noContent().build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy