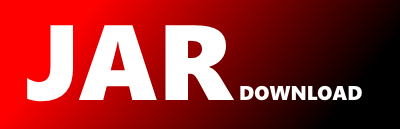
dk.grinn.keycloak.migration.entities.RealmHistory Maven / Gradle / Ivy
package dk.grinn.keycloak.migration.entities;
/*-
* #%L
* Keycloak : Migrate : Spi
* %%
* Copyright (C) 2019 Jonas Grann & Kim Jersin
* %%
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
* #L%
*/
import java.io.Serializable;
import java.util.Date;
import java.util.List;
import javax.persistence.Basic;
import javax.persistence.Column;
import javax.persistence.EmbeddedId;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.NamedQueries;
import javax.persistence.NamedQuery;
import javax.persistence.Table;
import javax.persistence.Temporal;
import javax.persistence.TemporalType;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
/**
*
* @author @author Kim Jersin <[email protected]>
*/
@Entity
@Table(name = "gkcadm_realm_history")
@NamedQueries({
@NamedQuery(name = "RealmHistory.findAll",
query = "SELECT h "
+ "FROM RealmHistory h"
),
@NamedQuery(name = "RealmHistory.countOpen",
query = "SELECT count(h) "
+ "FROM RealmHistory h "
+ "where h.historyLock.session = :session and h.script is null"
),
@NamedQuery(name = "RealmHistory.findBySessionAndKey",
query = "SELECT h "
+ "FROM RealmHistory h "
+ "where h.pk = :pk and h.historyLock.session = :session and h.script is null"
),
@NamedQuery(name = "RealmHistory.removeBySessionAndKey",
query = "DELETE "
+ "FROM RealmHistory h "
+ "where h.pk = :pk and h.script is null"
),
@NamedQuery(name = "RealmHistory.findBySessionAndVersion",
query = "SELECT h "
+ "FROM RealmHistory h "
+ "where h.version = :version and h.historyLock.session = :session"
),
@NamedQuery(name = "RealmHistory.findScriptHistoryBySession",
query = "SELECT new dk.grinn.keycloak.migration.entities.ScriptHistory("
+ " h.version, h.script, h.checksum, h.executionTime, h.installedOn, h.installedBy"
+ ") FROM RealmHistory h "
+ "where h.historyLock.session = :session and h.version is not null "
+ "order by h.pk.installedRank"
),
@NamedQuery(name = "RealmHistory.findScriptHistoryBySessionIdAndRank",
query = "SELECT new dk.grinn.keycloak.migration.entities.ScriptHistory("
+ " h.version, h.script, h.checksum, h.executionTime, h.installedOn, h.installedBy"
+ ") FROM RealmHistory h "
+ "where h.historyLock.session = :session and h.pk.id = :id and h.pk.installedRank = :installedRank and h.version is not null "
),
@NamedQuery(name = "RealmHistory.findScriptHistoryByRealmAndVersion",
query = "SELECT new dk.grinn.keycloak.migration.entities.ScriptHistory("
+ " h.version, h.script, h.checksum, h.executionTime, h.installedOn, h.installedBy"
+ ") FROM RealmHistory h "
+ "where h.historyLock.realm = :realm and h.version = :version "
),
@NamedQuery(name = "RealmHistory.findScriptHistoryByRealm",
query = "SELECT new dk.grinn.keycloak.migration.entities.ScriptHistory("
+ " h.version, h.script, h.checksum, h.executionTime, h.installedOn, h.installedBy"
+ ") FROM RealmHistory h "
+ "where h.historyLock.realm = :realm and h.version is not null "
+ "order by h.pk.installedRank"
)
})
public class RealmHistory implements Serializable {
private static final long serialVersionUID = 1L;
@EmbeddedId
protected RealmHistoryPK pk;
private String version;
@Basic(optional = false)
private String description;
@Basic(optional = false)
private String type;
private String script;
private Long checksum;
@Column(name = "execution_time")
private Integer executionTime;
@Column(name = "installed_on")
@Temporal(TemporalType.TIMESTAMP)
private Date installedOn;
@Basic(optional = false)
@Column(name = "installed_by")
private String installedBy;
@JoinColumn(name = "id", referencedColumnName = "id", insertable = false, updatable = false)
@ManyToOne(optional = false, fetch = FetchType.LAZY)
private RealmHistoryLock historyLock;
public RealmHistory() {
}
public RealmHistory(RealmHistoryPK pk) {
this.pk = pk;
}
public RealmHistory(RealmHistoryLock lock, List realms, String description, String installedBy) {
this.pk = new RealmHistoryPK(lock.getId(), lock.getCurrentRank());
this.description = description;
this.type = "REALM";
this.script = String.join(",", realms);
this.installedOn = new Date();
this.installedBy = installedBy;
}
public RealmHistory(RealmHistoryLock lock, String version, String description, String type, String installedBy) {
this(lock, version, description, type, null, installedBy);
}
public RealmHistory(RealmHistoryLock lock, String version, String description, String type, String script, String installedBy) {
this.pk = new RealmHistoryPK(lock.getId(), lock.incrementAndGetCurrentRank());
this.version = version;
this.description = description;
this.type = type;
this.script = script;
this.installedOn = new Date();
this.installedBy = installedBy;
}
public RealmHistoryPK getPk() {
return pk;
}
public void setPk(RealmHistoryPK pk) {
this.pk = pk;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getScript() {
return script;
}
public void setScript(String script) {
this.script = script;
}
public Long getChecksum() {
return checksum;
}
public void setChecksum(Long checksum) {
this.checksum = checksum;
}
public Integer getExecutionTime() {
return executionTime;
}
public void setExecutionTime(Integer executionTime) {
this.executionTime = executionTime;
}
public Date getInstalledOn() {
return installedOn;
}
public void setInstalledOn(Date installedOn) {
this.installedOn = installedOn;
}
public String getInstalledBy() {
return installedBy;
}
public void setInstalledBy(String installedBy) {
this.installedBy = installedBy;
}
public RealmHistoryLock getHistoryLock() {
return historyLock;
}
public void setHistoryLock(RealmHistoryLock historyLock) {
this.historyLock = historyLock;
}
@Override
public int hashCode() {
int hash = 0;
hash += (pk != null ? pk.hashCode() : 0);
return hash;
}
@Override
public boolean equals(Object object) {
// TODO: Warning - this method won't work in the case the id fields are not set
if (!(object instanceof RealmHistory)) {
return false;
}
RealmHistory other = (RealmHistory) object;
if ((this.pk == null && other.pk != null) || (this.pk != null && !this.pk.equals(other.pk))) {
return false;
}
return true;
}
@Override
public String toString() {
return "dk.grinn.keycloak.migration.entities.GkcadmRealmHistory[ gkcadmRealmHistoryPK=" + pk + " ]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy