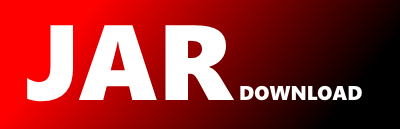
org.valkyriercp.application.ApplicationPage Maven / Gradle / Ivy
package org.valkyriercp.application;
import org.valkyriercp.factory.ControlFactory;
import java.util.List;
public interface ApplicationPage extends ControlFactory {
String getId();
ApplicationWindow getWindow();
boolean close();
boolean close(PageComponent pageComponent);
public List getPageComponents();
PageComponent getActiveComponent();
void setActiveComponent(PageComponent pageComponent);
void addPageComponentListener(PageComponentListener listener);
void removePageComponentListener(PageComponentListener listener);
/**
* Shows the {@link View} with the given id.
*
* If the {@link View} is already opened, the view will be reused.
*
* NOTE: this is NOT the same as calling this.showView(id, null)
.
*
* @param id
* the view id, cannot be empty
*
* @return the {@link View} that is shown
*/
View showView(String id);
/**
* Shows the {@link View} with the given id, and passes the input to the {@link View}, by calling
* {@link View#setInput(Object)}.
*
* If the {@link View} is already opened, the view will be reused.
*
* @param id
* the view id, cannot be empty
* @param input
* the input, can be null
*
* @return the {@link View} that is shown
*/
View showView(String id, Object input);
/**
* Returns the {@link View} with the given id. Returns null
if no {@link View} with the given id is
* shown.
*
* This method is "generified" to avoid extra casts when calling this method:
*
*
* ApplicationPage page = ...; // get a reference to the ApplicationPage
* InitialView initialView = page.getView("initialView");
*
*
* @param id
* the id, cannot be null
* @return the {@link View}, or null
*/
T getView(String id);
View showView(ViewDescriptor viewDescriptor);
View showView(ViewDescriptor viewDescriptor, Object input);
}