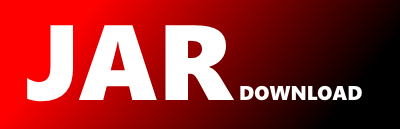
org.valkyriercp.component.PatchedJFormattedTextField Maven / Gradle / Ivy
package org.valkyriercp.component;
import javax.swing.*;
import javax.swing.text.DefaultFormatter;
import java.awt.event.ActionEvent;
import java.awt.event.KeyEvent;
import java.text.Format;
public class PatchedJFormattedTextField extends JFormattedTextField {
private static final String TOGGLE_OVERWRITE_MODE_ACTION = "toggleOverwriteModeAction";
public PatchedJFormattedTextField() {
super();
customInit();
}
public PatchedJFormattedTextField(Object value) {
super(value);
customInit();
}
public PatchedJFormattedTextField(Format format) {
super(format);
customInit();
}
public PatchedJFormattedTextField(AbstractFormatter formatter) {
super(formatter);
customInit();
}
public PatchedJFormattedTextField(AbstractFormatterFactory factory) {
super(factory);
customInit();
}
public PatchedJFormattedTextField(AbstractFormatterFactory factory, Object currentValue) {
super(factory, currentValue);
customInit();
}
private void customInit() {
setFocusLostBehavior(COMMIT);
if (getFormatter() instanceof DefaultFormatter) {
final DefaultFormatter d = (DefaultFormatter)getFormatter();
AbstractAction toggleOverwrite = new AbstractAction() {
public void actionPerformed(ActionEvent e) {
d.setOverwriteMode(!(d.getOverwriteMode()));
}
};
getInputMap().put(KeyStroke.getKeyStroke(KeyEvent.VK_INSERT, 0), TOGGLE_OVERWRITE_MODE_ACTION);
getActionMap().put(TOGGLE_OVERWRITE_MODE_ACTION, toggleOverwrite);
}
}
/**
* Overiding this method prevents the TextField from intercepting the Enter
* Key when focus is not on it. This allows default buttons to function.
* This should be removed when Swing fixes their bug.
*
* @see javax.swing.JComponent#processKeyBinding(javax.swing.KeyStroke,
* java.awt.event.KeyEvent, int, boolean)
*/
public boolean processKeyBinding(KeyStroke ks, KeyEvent e, int condition, boolean pressed) {
if (ks == KeyStroke.getKeyStroke(KeyEvent.VK_ENTER, 0) || ks == KeyStroke.getKeyStroke(KeyEvent.VK_ESCAPE, 0)) {
return false;
}
return super.processKeyBinding(ks, e, condition, pressed);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy