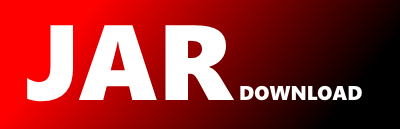
ec.gob.senescyt.sniese.commons.tests.AbstractIntegrationTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sniese-commons-test Show documentation
Show all versions of sniese-commons-test Show documentation
Librería que contiene clases de uso comun para microservicios hechos en dropwizard
package ec.gob.senescyt.sniese.commons.tests;
import com.google.common.io.Resources;
import com.sun.jersey.api.client.Client;
import com.sun.jersey.api.client.ClientResponse;
import com.sun.jersey.api.client.WebResource;
import ec.gob.senescyt.sniese.commons.applications.DecoradorAplicacion;
import ec.gob.senescyt.sniese.commons.configurations.ConfiguracionSnieseBase;
import ec.gob.senescyt.sniese.commons.core.EntidadBase;
import io.dropwizard.testing.junit.DropwizardAppRule;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.context.internal.ManagedSessionContext;
import org.junit.After;
import org.junit.Before;
import org.junit.Rule;
import javax.ws.rs.core.HttpHeaders;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.MultivaluedMap;
import java.io.File;
import java.net.URISyntaxException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static org.apache.commons.lang.RandomStringUtils.randomAlphabetic;
public abstract class AbstractIntegrationTest> {
private final DropwizardAppRule rule;
protected static final String CONFIGURACION = "test-integracion.yml";
protected SessionFactory sessionFactory;
protected Session session;
protected final Client client = new Client();
protected String defaultSchema;
protected Map headersDefecto;
public AbstractIntegrationTest(Class applicationClass) {
rule = new DropwizardAppRule<>(applicationClass, resourceFilePath(CONFIGURACION));
}
@Rule
public DropwizardAppRule getRule() {
return rule;
}
protected static String resourceFilePath(String resourceClassPathLocation) {
try {
return new File(Resources.getResource(resourceClassPathLocation).toURI()).getAbsolutePath();
} catch (URISyntaxException e) {
return null;
}
}
@Before
public void setUp() throws Exception {
if (getRule().getConfiguration().getConfiguracionPersistente().getDatabase() != null) {
defaultSchema = getRule().getConfiguration().getConfiguracionPersistente().getDefaultSchema();
sessionFactory = getSessionFactory();
session = sessionFactory.openSession();
ManagedSessionContext.bind(session);
}
headersDefecto = new HashMap<>(1);
headersDefecto.put("Authorization", randomAlphabetic(10));
}
protected abstract SessionFactory getSessionFactory();
@After
public void tearDown() {
ManagedSessionContext.unbind(sessionFactory);
}
protected ClientResponse hacerPost(final String recurso, EntidadBase entidad) {
return construirRecursoWeb(recurso, null, headersDefecto).post(ClientResponse.class, entidad);
}
protected ClientResponse hacerPost(final String recurso, Object entidad) {
return construirRecursoWeb(recurso, null, headersDefecto).post(ClientResponse.class, entidad);
}
protected ClientResponse hacerPut(final String recurso, EntidadBase entidad) {
return construirRecursoWeb(recurso, null, headersDefecto).put(ClientResponse.class, entidad);
}
protected ClientResponse hacerPut(final String rescurso, List entidades){
return construirRecursoWeb(rescurso, null, headersDefecto).put(ClientResponse.class, entidades);
}
protected ClientResponse hacerDelete(final String recurso) {
return construirRecursoWeb(recurso, null, headersDefecto).delete(ClientResponse.class);
}
protected ClientResponse hacerGet(String recurso, MultivaluedMap parametros) {
return construirRecursoWeb(recurso, parametros, headersDefecto)
.get(ClientResponse.class);
}
protected ClientResponse hacerGetConHeaders(String recurso, Map headers) {
WebResource.Builder builder = construirRecursoWeb(recurso, null, headers);
return builder.get(ClientResponse.class);
}
protected ClientResponse hacerGet(String recurso) {
return construirRecursoWeb(recurso, null, headersDefecto).get(ClientResponse.class);
}
private WebResource.Builder construirRecursoWeb(String recurso, MultivaluedMap parametros, Map headers) {
WebResource resource = client.resource(getURL(recurso));
if (parametros != null) {
resource = resource.queryParams(parametros);
}
WebResource.Builder builder = resource.header(HttpHeaders.CONTENT_TYPE, MediaType.APPLICATION_JSON);
headers.forEach((llave, valor) -> builder.header(llave, valor));
return builder;
}
protected String getURL(String recurso) {
T configuration = getRule().getConfiguration();
return String.format("https://localhost:%s/%s", configuration.getPuertoHttps(), recurso);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy