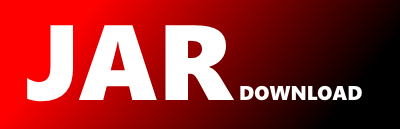
ec.gob.senescyt.sniese.commons.services.ServicioReportesPdf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sniese-commons Show documentation
Show all versions of sniese-commons Show documentation
Librería que contiene clases de uso comun para sniese hechos en dropwizard
package ec.gob.senescyt.sniese.commons.services;
import ec.gob.senescyt.sniese.commons.core.Reporte;
import ec.gob.senescyt.sniese.commons.exceptions.ReporteException;
import fr.opensagres.xdocreport.converter.ConverterTypeTo;
import fr.opensagres.xdocreport.converter.Options;
import fr.opensagres.xdocreport.core.XDocReportException;
import fr.opensagres.xdocreport.document.IXDocReport;
import fr.opensagres.xdocreport.document.registry.XDocReportRegistry;
import fr.opensagres.xdocreport.template.TemplateEngineKind;
import javax.validation.Validation;
import javax.validation.Validator;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
public class ServicioReportesPdf implements ServicioReportes {
private static final String MENSAJE_ERROR_CONVERTIR_PDF = "Error al convertir reporte a PDF";
private static final String MENSAJE_ERROR_ELIMINAR_ARCHIVO_TEMPORAL = "Error al eliminar archivo temporal";
private final XDocReportRegistry registry;
private String plantilla;
private Validator validator;
private IXDocReport report;
public ServicioReportesPdf(XDocReportRegistry registry, String plantilla) throws IOException, XDocReportException {
this.registry = registry;
this.plantilla = plantilla;
this.validator = Validation.buildDefaultValidatorFactory().getValidator();
this.report = registry.loadReport(adquirirPlantilla(plantilla), TemplateEngineKind.Velocity);
}
@Override
public InputStream generarReporte(Reporte reporte) {
validar(reporte);
InputStream inputStream;
FileOutputStream outputStream;
File file = new File(reporte.getNombre());
try {
outputStream = new FileOutputStream(file);
report.convert(reporte.getDatos(), Options.getTo(ConverterTypeTo.PDF), outputStream);
inputStream = new FileInputStream(file);
eliminarArchivoTemporal(file);
outputStream.close();
} catch (XDocReportException | IOException e) {
eliminarArchivoTemporal(file);
throw new ReporteException(MENSAJE_ERROR_CONVERTIR_PDF, e);
}
return inputStream;
}
public XDocReportRegistry getRegistry() {
return registry;
}
public String getPlantilla() {
return plantilla;
}
private void validar(Reporte reporte) {
validator.validate(reporte).stream().forEach(v -> {
throw new ReporteException(v.getMessage());
});
}
private InputStream adquirirPlantilla(String plantilla) throws FileNotFoundException {
return getClass().getResourceAsStream(plantilla);
}
private void eliminarArchivoTemporal(File file) {
if(file.exists() && !file.delete()){
throw new ReporteException(MENSAJE_ERROR_ELIMINAR_ARCHIVO_TEMPORAL);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy