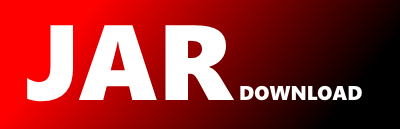
edu.amherst.acdc.exts.ore.OreRouter Maven / Gradle / Ivy
/*
* Copyright Amherst College
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package edu.amherst.acdc.exts.ore;
import static edu.amherst.acdc.exts.ore.OreHeaders.ORE_ACCEPT;
import static edu.amherst.acdc.exts.ore.OreHeaders.ORE_SUBJECT;
import static java.util.Optional.ofNullable;
import static org.apache.camel.Exchange.CONTENT_TYPE;
import static org.apache.camel.Exchange.HTTP_METHOD;
import static org.apache.camel.Exchange.HTTP_RESPONSE_CODE;
import static org.apache.camel.Exchange.HTTP_URI;
import java.util.Optional;
import org.apache.camel.builder.RouteBuilder;
import org.apache.jena.riot.Lang;
import org.apache.jena.riot.RDFLanguages;
/**
* A content router for handling ORE extension requests.
*
* @author Aaron Coburn
*/
public class OreRouter extends RouteBuilder {
private static final String DEFAULT_CONTENT_TYPE = "text/turtle";
private static final String HTTP_QUERY_CONTEXT = "context";
private static final String FCREPO_URI = "CamelFcrepoUri";
private static final String FCREPO_BASE_URL = "CamelFcrepoBaseUrl";
/**
* Configure the message route workflow.
*/
public void configure() throws Exception {
from("jetty:http://{{rest.host}}:{{rest.port}}{{rest.prefix}}?" +
"sendServerVersion=false&optionsEnabled=true&httpMethodRestrict=GET,OPTIONS")
.routeId("OreRouter")
.removeHeader("User-Agent")
.process(e -> e.getIn().setHeader(FCREPO_URI,
e.getIn().getHeader(HTTP_QUERY_CONTEXT,
e.getIn().getHeader("Apix-Ldp-Resource"))))
.setHeader(FCREPO_BASE_URL).simple("{{fcrepo.baseUrl}}")
.choice()
.when(header(HTTP_METHOD).isEqualTo("OPTIONS"))
.setHeader(CONTENT_TYPE).constant("text/turtle")
.setHeader("Allow").constant("GET,OPTIONS")
.to("language:simple:resource:classpath:options.ttl")
.when(header(HTTP_QUERY_CONTEXT).startsWith(header(FCREPO_BASE_URL)))
.to("direct:get");
from("direct:get").routeId("OreGet")
.setHeader(ORE_ACCEPT, header("Accept"))
.log("Building ORE Object ${body}")
.setBody().header(FCREPO_URI)
.to("seda:recurse?timeout={{ore.timeout}}")
.removeHeader("breadcrumbId")
.process(exchange -> {
final String contentType = exchange.getIn().getHeader(ORE_ACCEPT, String.class);
final Optional rdfLang = ofNullable(contentType).map(RDFLanguages::contentTypeToLang)
.map(Lang::getName);
exchange.getIn().setHeader(CONTENT_TYPE, rdfLang.isPresent() ? contentType : DEFAULT_CONTENT_TYPE);
})
.to("direct:serialize");
from("seda:recurse?concurrentConsumers={{ore.concurrency}}")
.routeId("OreBuildRecursive")
.setHeader(FCREPO_URI, body())
.to("direct:getResource")
.filter(header(HTTP_RESPONSE_CODE).isEqualTo(200))
.log("Getting related resources for ${headers[CamelFcrepoUri]}")
.to("direct:parse")
.setHeader(ORE_SUBJECT).header(FCREPO_URI)
.to("direct:members")
.split(body(), new ModelAggregator())
.to("seda:recurse?timeout={{ore.timeout}}");
from("direct:getResource").routeId("OreResource")
.removeHeader("breadcrumbId")
.removeHeader("Accept")
.removeHeaders("CamelHttp*")
.setHeader("Accept").header(ORE_ACCEPT)
.setHeader(HTTP_URI).header(FCREPO_URI)
.to("http4://localhost?username={{fcrepo.authUsername}}"
+ "&password={{fcrepo.authPassword}}&throwExceptionOnFailure=false");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy