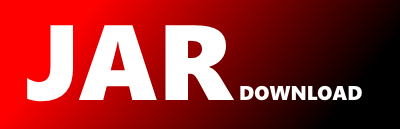
edu.berkeley.cs.jqf.plugin.ReproGoal Maven / Gradle / Ivy
Show all versions of jqf-maven-plugin Show documentation
/*
* Copyright (c) 2017-2018 The Regents of the University of California
*
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are
* met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package edu.berkeley.cs.jqf.plugin;
import java.io.File;
import java.io.IOException;
import java.io.PrintStream;
import java.io.PrintWriter;
import java.net.MalformedURLException;
import java.util.List;
import java.util.Set;
import java.util.SortedSet;
import java.util.TreeSet;
import edu.berkeley.cs.jqf.fuzz.junit.GuidedFuzzing;
import edu.berkeley.cs.jqf.fuzz.repro.ReproGuidance;
import edu.berkeley.cs.jqf.instrument.InstrumentingClassLoader;
import org.apache.maven.artifact.DependencyResolutionRequiredException;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugin.logging.Log;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.plugins.annotations.ResolutionScope;
import org.apache.maven.project.MavenProject;
import org.junit.runner.Result;
/**
* Maven plugin for replaying a test case produced by JQF.
*
* @author Rohan Padhye
*/
@Mojo(name="repro",
requiresDependencyResolution=ResolutionScope.TEST)
public class ReproGoal extends AbstractMojo {
@Parameter(defaultValue="${project}", required=true, readonly=true)
MavenProject project;
@Parameter(defaultValue="${project.build.directory}", readonly=true)
private File target;
/**
* The fully-qualified name of the test class containing methods
* to fuzz.
*
* This class will be loaded using the Maven project's test
* classpath. It must be annotated with {@code @RunWith(JQF.class)}
*/
@Parameter(property="class", required=true)
private String testClassName;
/**
* The name of the method to fuzz.
*
* This method must be annotated with {@code @Fuzz}, and take
* one or more arguments (with optional junit-quickcheck
* annotations) whose values will be fuzzed by JQF.
*
* If more than one method of this name exists in the
* test class or if the method is not declared
* {@code public void}, then the fuzzer will not launch.
*/
@Parameter(property="method", required=true)
private String testMethod;
/**
* Input file to reproduce.
*
* These files will typically be taken from the test corpus
* ("queue") directory or the failures ("crashes") directory
* generated by JQF in a previous fuzzing run, for the same
* test class and method.
*
*/
@Parameter(property="input", required=true)
private String input;
/**
* Output file to dump coverage info.
*
* This is an optional parameter. If set, the value is the name
* of a file where JQF will dump code coverage information for
* the test inputs being replayed.
*/
@Parameter(property="logCoverage")
private String logCoverage;
/**
* Comma-separated list of FQN prefixes to exclude from
* coverage instrumentation.
*
* This property is only useful if {@link #logCoverage} is
* set. The semantics are similar to the similarly named
* property in the goal jqf:fuzz.
*/
@Parameter(property="excludes")
private String excludes;
/**
* Comma-separated list of FQN prefixes to forcibly include,
* even if they match an exclude.
*
* Typically, these will be a longer prefix than a prefix
* in the excludes clauses.
*
* This property is only useful if {@link #logCoverage} is
* set. The semantics are similar to the similarly named
* property in the goal jqf:fuzz.
*/
@Parameter(property="includes")
private String includes;
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
ClassLoader loader;
ReproGuidance guidance;
Log log = getLog();
PrintStream out = System.out; // TODO: Re-route to logger from super.getLog()
Result result;
// Configure classes to instrument
if (excludes != null) {
System.setProperty("janala.excludes", excludes);
}
if (includes != null) {
System.setProperty("janala.includes", includes);
}
try {
List classpathElements = project.getTestClasspathElements();
loader = new InstrumentingClassLoader(
classpathElements.toArray(new String[0]),
getClass().getClassLoader());
} catch (DependencyResolutionRequiredException|MalformedURLException e) {
throw new MojoExecutionException("Could not get project classpath", e);
}
File inputFile = new File(input);
if (!inputFile.exists() || !inputFile.canRead()) {
throw new MojoExecutionException("Cannot find or open file " + input);
}
// If a coverage dump file was provided, enable logging via system property
if (logCoverage != null) {
System.setProperty("jqf.repro.logUniqueBranches", "true");
}
guidance = new ReproGuidance(inputFile, null);
try {
result = GuidedFuzzing.run(testClassName, testMethod, loader, guidance, out);
} catch (ClassNotFoundException e) {
throw new MojoExecutionException("Could not load test class", e);
} catch (IllegalArgumentException e) {
throw new MojoExecutionException("Bad request", e);
} catch (RuntimeException e) {
throw new MojoExecutionException("Internal error", e);
}
// If a coverage dump file was provided, then dump coverage
if (logCoverage != null) {
Set coverageSet = guidance.getBranchesCovered();
assert (coverageSet != null); // Should not happen if we set the system property above
SortedSet sortedCoverage = new TreeSet<>(coverageSet);
try (PrintWriter covOut = new PrintWriter(new File(logCoverage))) {
for (String b : sortedCoverage) {
covOut.println(b);
}
} catch (IOException e) {
log.error("Could not dump coverage info.", e);
}
}
if (!result.wasSuccessful()) {
throw new MojoFailureException("Test case produces a failure.");
}
}
}