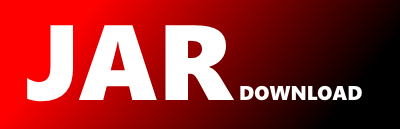
chisel.lib.fifo.BubbleFifo.scala Maven / Gradle / Ivy
The newest version!
// Author: Martin Schoeberl ([email protected])
// License: this code is released into the public domain, see README.md and http://unlicense.org/
package chisel.lib.fifo
import chisel3._
/**
* A simple bubble FIFO.
* Maximum throughput is one word every two clock cycles.
*/
class BubbleFifo[T <: Data](gen: T, depth: Int) extends Fifo(gen: T, depth: Int) {
private class Buffer() extends Module {
val io = IO(new FifoIO(gen))
val fullReg = RegInit(false.B)
val dataReg = Reg(gen)
when(fullReg) {
when(io.deq.ready) {
fullReg := false.B
}
}.otherwise {
when(io.enq.valid) {
fullReg := true.B
dataReg := io.enq.bits
}
}
io.enq.ready := !fullReg
io.deq.valid := fullReg
io.deq.bits := dataReg
}
private val buffers = Array.fill(depth) { Module(new Buffer()) }
for (i <- 0 until depth - 1) {
buffers(i + 1).io.enq <> buffers(i).io.deq
}
io.enq <> buffers(0).io.enq
io.deq <> buffers(depth - 1).io.deq
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy