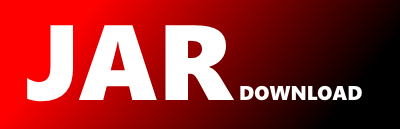
edu.berkeley.nlp.lm.phrasetable.MosesPhraseTableReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleylm Show documentation
Show all versions of berkeleylm Show documentation
An N-gram Language Model Library from UC Berkeley
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy