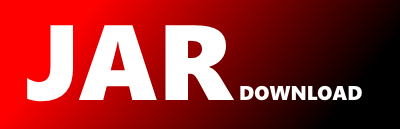
edu.berkeley.nlp.PCFGLA.BinaryCounterTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.PCFGLA;
import java.io.Serializable;
import java.util.Map;
import java.util.Set;
import edu.berkeley.nlp.util.ArrayUtil;
import edu.berkeley.nlp.util.Counter;
import edu.berkeley.nlp.util.MapFactory;
import edu.berkeley.nlp.util.MapFactory.HashMapFactory;
public class BinaryCounterTable implements Serializable {
/**
* Based on Counter.
*
* A map from objects to doubles. Includes convenience methods for getting,
* setting, and incrementing element counts. Objects not in the counter will
* return a count of zero. The counter is backed by a HashMap (unless specified
* otherwise with the MapFactory constructor).
*
* @author Slav Petrov
*/
private static final long serialVersionUID = 1L;
Map entries;
short[] numSubStates;
BinaryRule searchKey;
/**
* The elements in the counter.
*
* @return set of keys
*/
public Set keySet() {
return entries.keySet();
}
/**
* The number of entries in the counter (not the total count -- use totalCount() instead).
*/
public int size() {
return entries.size();
}
/**
* True if there are no entries in the counter (false does not mean totalCount > 0)
*/
public boolean isEmpty() {
return size() == 0;
}
/**
* Returns whether the counter contains the given key. Note that this is the
* way to distinguish keys which are in the counter with count zero, and those
* which are not in the counter (and will therefore return count zero from
* getCount().
*
* @param key
* @return whether the counter contains the key
*/
public boolean containsKey(BinaryRule key) {
return entries.containsKey(key);
}
/**
* Get the count of the element, or zero if the element is not in the
* counter. Can return null!
*
* @param key
* @return
*/
public double[][][] getCount(BinaryRule key) {
double[][][] value = entries.get(key);
return value;
}
public double[][][] getCount(short pState, short lState, short rState) {
searchKey.setNodes(pState,lState,rState);
double[][][] value = entries.get(searchKey);
return value;
}
/**
* Set the count for the given key, clobbering any previous count.
*
* @param key
* @param count
*/
public void setCount(BinaryRule key, double[][][] counts) {
entries.put(key, counts);
}
/**
* Increment a key's count by the given amount.
* Assumes for efficiency that the arrays have the same size.
*
* @param key
* @param increment
*/
public void incrementCount(BinaryRule key, double[][][] increment) {
double[][][] current = getCount(key);
if (current==null) {
setCount(key,increment);
return;
}
for (int i=0; i(), numSubStates);
}
public BinaryCounterTable(MapFactory mf, short[] numSubStates) {
entries = mf.buildMap();
searchKey = new BinaryRule((short)0,(short)0,(short)0);
this.numSubStates = numSubStates;
}
public static void main(String[] args) {
Counter counter = new Counter();
System.out.println(counter);
counter.incrementCount("planets", 7);
System.out.println(counter);
counter.incrementCount("planets", 1);
System.out.println(counter);
counter.setCount("suns", 1);
System.out.println(counter);
counter.setCount("aliens", 0);
System.out.println(counter);
System.out.println(counter.toString(2));
System.out.println("Total: " + counter.totalCount());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy