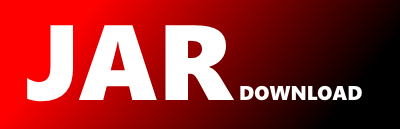
edu.berkeley.nlp.io.PTBLexer Maven / Gradle / Ivy
Show all versions of berkeleyparser Show documentation
/* The following code was generated by JFlex 1.4.1 on 10/12/07 4:49 PM */
package edu.berkeley.nlp.io;
import java.io.Reader;
//import edu.stanford.nlp.ling.AbstractMapLabel;
//import edu.stanford.nlp.ling.FeatureLabel;
/** Provides a tokenizer or lexer that does a pretty good job at
* deterministically tokenizing according to Penn Treebank conventions.
* The class is a scanner generated by
* JFlex 1.4.1 from the specification file
* PTBLexer.flex
. As well as copying what is in the Treebank,
* it now contains some extensions to deal with modern text and encoding
* issues, such as recognizing URLs and common Windows CP1252 characters.
*
* Implementation note: The scanner is caseless, but note if adding
* or changing regexps that caseless does not expand inside character
* classes. From the manual: "The %caseless option does not change the
* matched text and does not effect character classes. So [a] still only
* matches the character a and not A, too." Note that some character
* classes still deliberately don't have both cases, so the scanner's
* operation isn't completely case-independent, though it mostly is.
*
* @author Tim Grow
* @author Christopher Manning
* @author Jenny Finkel
*/
public class PTBLexer {
/** This character denotes the end of file */
public static final int YYEOF = -1;
/** initial size of the lookahead buffer */
private static final int ZZ_BUFFERSIZE = 16384;
/** lexical states */
public static final int YYINITIAL = 0;
/**
* Translates characters to character classes
*/
private static final String ZZ_CMAP_PACKED =
"\1\143\10\0\1\33\1\35\1\0\1\111\1\34\22\0\1\65\1\3"+
"\1\134\1\26\1\70\1\75\1\5\1\104\1\63\1\64\1\132\1\62"+
"\1\76\1\60\1\77\1\2\1\110\1\27\3\107\1\135\1\107\1\140"+
"\2\107\1\61\1\13\1\1\1\136\1\4\1\112\1\116\1\41\1\121"+
"\1\52\1\40\1\56\1\117\1\54\1\43\1\55\1\102\1\105\1\46"+
"\1\37\1\36\1\53\1\44\1\51\1\50\1\42\1\45\1\47\1\57"+
"\1\113\1\100\1\125\1\123\1\127\1\66\1\130\1\136\1\73\1\115"+
"\1\10\1\122\1\23\1\7\1\31\1\120\1\25\1\12\1\30\1\103"+
"\1\106\1\17\1\6\1\72\1\24\1\15\1\22\1\21\1\11\1\16"+
"\1\20\1\32\1\114\1\101\1\126\1\124\1\141\1\137\1\142\1\136"+
"\1\0\1\67\4\0\1\131\13\0\1\133\1\74\2\133\1\0\2\14"+
"\12\0\2\67\30\0\3\67\1\0\100\71\u1f13\0\2\14\3\0\1\133"+
"\1\74\2\0\2\133\10\0\1\131\205\0\1\67\udf53\0";
/**
* Translates characters to character classes
*/
private static final char [] ZZ_CMAP = zzUnpackCMap(ZZ_CMAP_PACKED);
/**
* Translates DFA states to action switch labels.
*/
private static final int [] ZZ_ACTION = zzUnpackAction();
private static final String ZZ_ACTION_PACKED_0 =
"\1\0\1\1\1\2\1\3\1\4\1\5\1\4\5\6"+
"\1\7\11\6\2\4\3\6\1\10\2\11\22\6\1\12"+
"\2\4\1\13\1\14\1\15\2\6\1\4\1\16\2\4"+
"\2\6\1\16\2\6\1\4\1\1\2\6\1\16\1\4"+
"\4\6\1\2\1\5\1\17\1\20\1\16\1\21\1\14"+
"\1\22\1\23\1\10\24\0\6\6\1\4\2\6\1\4"+
"\1\6\2\0\1\6\4\0\1\4\2\0\1\6\1\0"+
"\2\6\1\0\1\4\7\6\2\0\16\6\2\4\1\6"+
"\1\0\2\6\1\0\1\4\2\0\3\6\2\0\3\6"+
"\1\0\1\4\7\0\3\6\1\0\6\6\1\0\1\4"+
"\1\6\3\0\11\6\1\0\11\6\1\0\17\6\1\0"+
"\2\6\1\0\3\6\2\0\6\6\1\0\1\4\1\12"+
"\4\0\1\20\5\0\1\16\6\0\1\4\1\16\3\0"+
"\6\6\1\4\1\16\6\0\1\4\1\16\4\6\1\4"+
"\24\6\1\0\1\24\20\0\1\25\3\0\2\4\5\6"+
"\3\0\2\4\1\0\3\4\5\0\1\4\4\0\1\4"+
"\6\0\1\6\1\4\4\0\1\4\3\0\3\6\2\0"+
"\12\6\2\0\5\6\1\4\1\0\1\4\2\0\1\4"+
"\2\0\1\6\1\25\3\4\1\0\1\4\2\0\3\26"+
"\2\6\2\15\2\0\1\26\1\0\5\6\1\0\1\26"+
"\4\6\2\26\12\6\1\0\1\26\5\6\1\0\1\26"+
"\3\0\1\6\5\0\1\4\2\0\1\4\3\0\3\15"+
"\6\0\1\17\4\6\6\27\2\6\1\4\4\6\6\0"+
"\1\7\27\0\3\6\1\0\2\30\5\4\1\0\2\4"+
"\2\0\2\4\10\0\1\6\3\4\1\0\1\6\1\0"+
"\1\4\3\0\2\6\1\4\1\6\1\4\1\0\4\6"+
"\1\0\1\4\6\0\1\25\2\0\3\4\2\0\1\4"+
"\3\0\6\26\1\0\1\26\3\6\1\26\2\6\1\4"+
"\1\0\3\6\1\26\4\6\1\0\1\26\1\0\1\26"+
"\4\0\1\20\1\0\1\4\3\0\1\17\2\6\1\4"+
"\3\0\1\14\1\0\2\6\1\0\1\4\1\0\1\31"+
"\12\0\2\25\5\0\2\32\1\0\1\4\1\0\3\4"+
"\7\0\1\6\5\0\2\6\3\0\2\6\1\0\1\4"+
"\4\0\1\25\1\0\1\4\1\25\1\0\1\25\4\0"+
"\11\26\1\6\1\0\1\26\2\6\1\26\2\6\1\0"+
"\2\26\2\0\1\4\4\0\2\17\4\0\1\4\6\0"+
"\2\6\4\0\1\16\1\6\1\10\1\4\11\0\1\4"+
"\1\0\6\4\1\0\1\6\10\0\1\33\1\4\4\0"+
"\4\4\1\25\3\0\7\26\1\6\2\26\1\33\1\0"+
"\2\26\4\0\1\20\16\0\1\16\5\0\1\4\2\0"+
"\2\25\1\0\1\6\1\0\3\4\2\0\3\4\3\0"+
"\4\4\1\26\1\0\2\26\1\0\2\26\1\4\1\0"+
"\1\17\3\0\2\4\1\0\1\4\1\0\1\4\10\0"+
"\2\4\3\0\2\4\2\0\2\26\2\0\2\4\6\0"+
"\1\25\5\0\2\26\1\0\2\4\1\0\1\4\13\0"+
"\2\26\1\0\2\4\13\0\2\26\1\0\1\4\7\0";
private static int [] zzUnpackAction() {
int [] result = new int[967];
int offset = 0;
offset = zzUnpackAction(ZZ_ACTION_PACKED_0, offset, result);
return result;
}
private static int zzUnpackAction(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/**
* Translates a state to a row index in the transition table
*/
private static final int [] ZZ_ROWMAP = zzUnpackRowMap();
private static final String ZZ_ROWMAP_PACKED_0 =
"\0\0\0\144\0\310\0\u012c\0\u012c\0\u012c\0\u0190\0\u01f4"+
"\0\u0258\0\u02bc\0\u0320\0\u0384\0\144\0\u03e8\0\u044c\0\u04b0"+
"\0\u0514\0\u0578\0\u05dc\0\u0640\0\u06a4\0\u0708\0\u076c\0\u07d0"+
"\0\u0834\0\u0898\0\u08fc\0\u0960\0\u09c4\0\u012c\0\u0a28\0\u0a8c"+
"\0\u0af0\0\u0b54\0\u0bb8\0\u0c1c\0\u0c80\0\u0ce4\0\u0d48\0\u0dac"+
"\0\u0e10\0\u0e74\0\u0ed8\0\u0f3c\0\u0fa0\0\u1004\0\u1068\0\u10cc"+
"\0\u1130\0\u1194\0\u11f8\0\u125c\0\u12c0\0\144\0\u1324\0\u1388"+
"\0\u13ec\0\u1450\0\144\0\u14b4\0\u1518\0\u157c\0\u15e0\0\u1644"+
"\0\u16a8\0\u170c\0\u012c\0\u1770\0\u17d4\0\u1838\0\u189c\0\u1900"+
"\0\u1964\0\u19c8\0\u1a2c\0\144\0\144\0\144\0\u1a90\0\u1af4"+
"\0\u1b58\0\u012c\0\u012c\0\u012c\0\144\0\144\0\u1bbc\0\u1c20"+
"\0\u1c84\0\u1ce8\0\u1d4c\0\u1db0\0\u1e14\0\u1e78\0\u1edc\0\u1f40"+
"\0\u1fa4\0\u2008\0\u206c\0\u20d0\0\u2134\0\u2198\0\u21fc\0\u2260"+
"\0\u22c4\0\u2328\0\u238c\0\u23f0\0\u2454\0\u24b8\0\u251c\0\u2580"+
"\0\u25e4\0\u2648\0\u26ac\0\u2710\0\u2774\0\u27d8\0\u283c\0\u28a0"+
"\0\u2904\0\u2968\0\u29cc\0\u2a30\0\u2a94\0\u2af8\0\u2b5c\0\u2bc0"+
"\0\u2c24\0\u2c88\0\u2cec\0\u2d50\0\u2db4\0\u2e18\0\u2e7c\0\u2ee0"+
"\0\u2f44\0\u2fa8\0\u300c\0\u3070\0\u30d4\0\u3138\0\u319c\0\u3200"+
"\0\u3264\0\u32c8\0\u332c\0\u3390\0\u33f4\0\u3458\0\u34bc\0\u3520"+
"\0\u3584\0\u35e8\0\u364c\0\u36b0\0\u3714\0\u3778\0\u37dc\0\u3840"+
"\0\u38a4\0\u3908\0\u396c\0\u39d0\0\u3a34\0\u3a98\0\u3afc\0\u3b60"+
"\0\u3bc4\0\u3c28\0\u3c8c\0\u3cf0\0\u3d54\0\u3db8\0\u3e1c\0\u3e80"+
"\0\u3ee4\0\u3f48\0\u3fac\0\u4010\0\u4074\0\u40d8\0\u413c\0\u41a0"+
"\0\u4204\0\u4268\0\u42cc\0\u4330\0\u4394\0\u43f8\0\u445c\0\u44c0"+
"\0\u4524\0\u2260\0\u4588\0\u45ec\0\u4650\0\u46b4\0\u4718\0\u477c"+
"\0\u47e0\0\u4844\0\u48a8\0\u490c\0\u4970\0\u49d4\0\u4a38\0\u4a9c"+
"\0\u4b00\0\u4b64\0\u4bc8\0\u4c2c\0\u4c90\0\u4cf4\0\u4d58\0\u4dbc"+
"\0\u4e20\0\u4e84\0\u4ee8\0\u4f4c\0\u4fb0\0\u5014\0\u5078\0\u50dc"+
"\0\u5140\0\u51a4\0\u5208\0\u526c\0\u52d0\0\u5334\0\u5398\0\u53fc"+
"\0\u5460\0\u54c4\0\u5528\0\u558c\0\u55f0\0\u5654\0\u56b8\0\u571c"+
"\0\u5780\0\u57e4\0\u5848\0\u58ac\0\u5910\0\u5974\0\u59d8\0\u5a3c"+
"\0\u5aa0\0\u5b04\0\u5b68\0\u1194\0\u5bcc\0\u5c30\0\u5c94\0\u5cf8"+
"\0\u5d5c\0\u5dc0\0\u5e24\0\u5e88\0\u5eec\0\u5f50\0\u5fb4\0\u6018"+
"\0\u607c\0\u60e0\0\u6144\0\u61a8\0\u620c\0\144\0\u6270\0\u62d4"+
"\0\u6338\0\u639c\0\u6400\0\u6464\0\u64c8\0\u652c\0\u6590\0\u3070"+
"\0\u65f4\0\u6658\0\u66bc\0\u6720\0\u6784\0\u67e8\0\u684c\0\u68b0"+
"\0\u012c\0\u6914\0\u6978\0\u69dc\0\u6a40\0\u6aa4\0\u6b08\0\u6b6c"+
"\0\u6bd0\0\u6c34\0\u6c98\0\u6cfc\0\u6d60\0\u6dc4\0\u6e28\0\u6e8c"+
"\0\u6ef0\0\u6f54\0\u6fb8\0\u701c\0\u7080\0\u70e4\0\u7148\0\u71ac"+
"\0\u7210\0\u7274\0\u72d8\0\u012c\0\u733c\0\u73a0\0\u7404\0\u7468"+
"\0\u74cc\0\u7530\0\u7594\0\u75f8\0\u765c\0\u76c0\0\u7724\0\u7788"+
"\0\u77ec\0\u7850\0\u78b4\0\u7918\0\u797c\0\u79e0\0\u7a44\0\u7aa8"+
"\0\u7b0c\0\u7aa8\0\u7b70\0\u7bd4\0\u7c38\0\u7c9c\0\u7d00\0\u7d64"+
"\0\u7dc8\0\u7e2c\0\u7e90\0\u7ef4\0\u7f58\0\u7fbc\0\u8020\0\u2198"+
"\0\u8084\0\u80e8\0\u814c\0\u81b0\0\u8214\0\u2260\0\u8278\0\u82dc"+
"\0\u8340\0\u83a4\0\u29cc\0\u8408\0\u846c\0\u84d0\0\u8534\0\u8598"+
"\0\u85fc\0\u8660\0\u86c4\0\u86c4\0\u8728\0\u878c\0\u87f0\0\u87f0"+
"\0\u8854\0\u88b8\0\u891c\0\u8980\0\u89e4\0\u8a48\0\u8aac\0\u8b10"+
"\0\u8b74\0\u8bd8\0\u8c3c\0\u8ca0\0\u8d04\0\u8d68\0\u8dcc\0\u8e30"+
"\0\u8e94\0\u8ef8\0\u8f5c\0\u8fc0\0\u9024\0\u9088\0\u90ec\0\u9150"+
"\0\u91b4\0\u9218\0\u927c\0\u92e0\0\u9344\0\u93a8\0\u2904\0\u940c"+
"\0\u9470\0\u94d4\0\u9538\0\u959c\0\u9600\0\u9664\0\u96c8\0\u972c"+
"\0\u9790\0\u97f4\0\u9858\0\u98bc\0\u9920\0\u9984\0\u99e8\0\u2198"+
"\0\u2260\0\u9a4c\0\u9ab0\0\u9b14\0\u9b78\0\u9bdc\0\u9c40\0\u9ca4"+
"\0\u9d08\0\u9d6c\0\u9dd0\0\u9e34\0\u9e98\0\u9efc\0\u9f60\0\u9fc4"+
"\0\ua028\0\ua08c\0\ua0f0\0\ua154\0\ua1b8\0\ua21c\0\ua280\0\ua2e4"+
"\0\ua348\0\ua3ac\0\ua410\0\ua474\0\ua4d8\0\ua53c\0\ua5a0\0\ua604"+
"\0\ua668\0\ua6cc\0\ua730\0\ua794\0\ua7f8\0\ua85c\0\ua8c0\0\ua924"+
"\0\ua988\0\ua9ec\0\uaa50\0\uaab4\0\uab18\0\uab7c\0\144\0\uabe0"+
"\0\uac44\0\u012c\0\uaca8\0\uad0c\0\uad70\0\144\0\u012c\0\u1bbc"+
"\0\uadd4\0\uae38\0\uae9c\0\uaf00\0\uaf64\0\uafc8\0\ub02c\0\ub090"+
"\0\ub0f4\0\ub158\0\ub1bc\0\u012c\0\ub220\0\ub284\0\ub2e8\0\ub34c"+
"\0\ub3b0\0\ub414\0\ub478\0\ub4dc\0\ub540\0\ub5a4\0\ub608\0\ub66c"+
"\0\ub6d0\0\ub734\0\ub798\0\ub7fc\0\ub860\0\ub8c4\0\u012c\0\ub928"+
"\0\ub98c\0\ub9f0\0\uba54\0\ubab8\0\ubb1c\0\ubb80\0\ubbe4\0\ubc48"+
"\0\ubcac\0\ubd10\0\ubd74\0\ubdd8\0\ube3c\0\ubea0\0\ubf04\0\ubf68"+
"\0\ubfcc\0\uc030\0\uc094\0\uc0f8\0\uc15c\0\uc1c0\0\uc224\0\uc288"+
"\0\uc2ec\0\uc350\0\u2198\0\u2260\0\u21fc\0\uc3b4\0\uc418\0\u3fac"+
"\0\u4010\0\uc47c\0\u40d8\0\uc4e0\0\uc544\0\uc5a8\0\uae9c\0\uc60c"+
"\0\uc670\0\uc6d4\0\uc738\0\uc79c\0\uc800\0\uc864\0\uc8c8\0\uc92c"+
"\0\uc990\0\uc9f4\0\uca58\0\u2af8\0\ucabc\0\ucb20\0\ucb84\0\u2198"+
"\0\ucbe8\0\ucc4c\0\uccb0\0\ucd14\0\ucd78\0\u2774\0\ucddc\0\uce40"+
"\0\ucea4\0\ucf08\0\ucf6c\0\ucfd0\0\ud034\0\ud098\0\ud0fc\0\ud160"+
"\0\ud1c4\0\ud228\0\ud28c\0\ud2f0\0\ud354\0\ud3b8\0\ud41c\0\ud480"+
"\0\ud4e4\0\ud548\0\ud5ac\0\ud610\0\ud674\0\ud6d8\0\ud73c\0\ud7a0"+
"\0\ud804\0\ud868\0\ud8cc\0\ud930\0\ud994\0\ud9f8\0\uda5c\0\udac0"+
"\0\udb24\0\udb88\0\udbec\0\udc50\0\udcb4\0\udd18\0\udd7c\0\u9858"+
"\0\udde0\0\ude44\0\udea8\0\udf0c\0\udf70\0\udfd4\0\ue038\0\ue09c"+
"\0\ue100\0\ue164\0\ue1c8\0\ue22c\0\ue290\0\ue2f4\0\ue358\0\ue3bc"+
"\0\ue420\0\ue484\0\ue4e8\0\ue54c\0\ue5b0\0\ue614\0\ue678\0\ue6dc"+
"\0\ue740\0\ue7a4\0\ue808\0\ue86c\0\ue8d0\0\ue934\0\u2580\0\ue998"+
"\0\ue9fc\0\uea60\0\ueac4\0\ueb28\0\ueb8c\0\u012c\0\uebf0\0\uec54"+
"\0\uecb8\0\ued1c\0\ued80\0\uede4\0\uee48\0\ueeac\0\uef10\0\uef74"+
"\0\uefd8\0\uf03c\0\uf0a0\0\uf104\0\uf168\0\uf1cc\0\uf230\0\uc1c0"+
"\0\u012c\0\uf294\0\uf2f8\0\uf35c\0\uf3c0\0\u814c\0\uf424\0\uf488"+
"\0\uf4ec\0\uf550\0\uf5b4\0\uf618\0\uf67c\0\uf6e0\0\uf744\0\uf7a8"+
"\0\uf80c\0\uf870\0\uf8d4\0\uf938\0\uf99c\0\ufa00\0\ufa64\0\ufac8"+
"\0\ufb2c\0\ufb90\0\ufbf4\0\ufc58\0\u8214\0\ufcbc\0\ufd20\0\ufd84"+
"\0\ufde8\0\ufe4c\0\ufeb0\0\uff14\0\uff78\0\uffdc\0\ud610\1\100"+
"\1\244\1\u0108\1\u016c\1\u01d0\1\u0234\1\u0298\1\u02fc\1\u0360"+
"\1\u03c4\1\u0428\1\u048c\1\u04f0\1\u0554\1\u05b8\1\u061c\1\u0680"+
"\1\u06e4\1\u0748\1\u07ac\1\u0810\1\u0874\1\u08d8\1\u093c\1\u09a0"+
"\1\u0a04\1\u0a68\1\u0acc\1\u0b30\1\u0b94\1\u0bf8\1\u0c5c\0\u012c"+
"\1\u0cc0\1\u0d24\1\u0d88\1\u0dec\1\u0d88\1\u0e50\1\u0eb4\1\u0f18"+
"\1\u0f7c\1\u0fe0\1\u1044\1\u10a8\1\u110c\1\u1170\1\u11d4\1\u1238"+
"\1\u129c\1\u1300\1\u1364\0\u012c\1\u13c8\1\u142c\1\u1490\1\u14f4"+
"\1\u1558\1\u15bc\1\u1620\1\u1684\1\u16e8\1\u174c\1\u17b0\1\u1814"+
"\1\u1878\1\u18dc\1\u1940\1\u19a4\1\u1a08\1\u1a6c\1\u1ad0\1\u1b34"+
"\1\u1b98\1\u1bfc\1\u1c60\1\u1cc4\1\u1d28\1\u1d8c\1\u1df0\1\u1e54"+
"\0\u2328\1\u1eb8\1\u1f1c\1\u1f80\1\u1fe4\1\u2048\1\u20ac\1\u2110"+
"\0\uffdc\1\u2174\0\uffdc\1\u21d8\1\u223c\1\u22a0\1\u2304\1\u2368"+
"\1\u23cc\1\u2430\1\u2494\1\u24f8\1\u255c\1\u25c0\1\u2624\1\u2688"+
"\0\u43f8\1\u26ec\1\u2750\1\u27b4\1\u2818\1\u287c\1\u28e0\1\u2944"+
"\0\144\1\u29a8\1\u2a0c\1\u2a70\1\u2ad4\1\u2b38\1\u2b9c\1\u2c00"+
"\1\u2c64\1\u2cc8\1\u2d2c\1\u2d90\1\u2df4\1\u2e58\1\u2ebc\0\ub2e8"+
"\1\u2f20\1\u2f84\1\u2fe8\1\u304c\1\u30b0\1\u3114\1\u3178\1\u31dc"+
"\1\u3240\1\u32a4\1\u3308\1\u336c\1\u33d0\1\u3434\1\u3498\1\u34fc"+
"\0\u36b0\1\u3560\0\u7dc8\0\u7e2c\0\u87f0\1\u35c4\1\u3628\1\u368c"+
"\1\u36f0\1\u3754\1\u37b8\1\u1814\1\u381c\1\u3880\1\u38e4\1\u3948"+
"\1\u39ac\1\u3a10\1\u3a74\0\u86c4\1\u3ad8\1\u3b3c\1\u3ba0\1\u3c04"+
"\1\u3c68\1\u3ccc\1\u0fe0\1\u3d30\1\u1044\1\u3d94\1\u2ebc\0\ub220"+
"\0\ub34c\0\ub3b0\1\u3df8\1\u3e5c\1\u3ec0\1\u3f24\1\u3f88\0\u8534"+
"\0\u8598\1\u3fec\1\u4050\1\u40b4\0\uefd8\0\uffdc\1\u4118\1\u417c"+
"\1\u41e0\1\u4244\1\u42a8\0\ue6dc\1\u430c\1\u4370\1\u43d4\1\u4438"+
"\1\u449c\1\u4500\1\u4564\1\u45c8\1\u3ec0\1\u462c\1\u4690\1\u46f4"+
"\1\u4758\1\u47bc\1\u4820\1\u4884\1\u48e8\1\u494c\1\u49b0\1\u4a14"+
"\1\u4a78\1\u4adc\1\u4b40\1\u4ba4\1\u4c08\1\u4c6c\1\u4cd0\1\u4d34"+
"\1\u4d98\1\u4dfc\1\u4e60\1\u4ec4\1\u4f28\1\u4f8c\1\u4ff0\1\u5054"+
"\1\u50b8\1\u511c\1\u5180\1\u51e4\1\u5248\1\u52ac\1\u5310\1\u5374"+
"\1\u53d8\1\u543c\1\u54a0\1\u5504\1\u5568\1\u55cc\1\u5630\1\u5694"+
"\1\u56f8\1\u575c\1\u57c0\1\u5824\1\u5888\1\u58ec\1\u5950";
private static int [] zzUnpackRowMap() {
int [] result = new int[967];
int offset = 0;
offset = zzUnpackRowMap(ZZ_ROWMAP_PACKED_0, offset, result);
return result;
}
private static int zzUnpackRowMap(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int high = packed.charAt(i++) << 16;
result[j++] = high | packed.charAt(i++);
}
return j;
}
/**
* The transition table of the DFA
*/
private static final int [] ZZ_TRANS = zzUnpackTrans();
private static final String ZZ_TRANS_PACKED_0 =
"\1\2\1\3\1\4\1\5\1\6\1\7\1\10\1\11"+
"\1\12\1\13\1\14\1\5\1\15\1\16\1\17\1\20"+
"\1\21\1\22\1\23\1\24\1\25\1\26\1\27\1\30"+
"\1\31\1\32\1\33\1\34\1\35\1\36\1\37\1\40"+
"\1\41\1\42\1\43\1\44\1\45\1\46\1\47\1\50"+
"\1\51\1\52\1\53\1\54\1\55\1\56\1\57\1\60"+
"\1\61\1\62\1\63\1\64\1\6\1\34\1\65\1\66"+
"\1\5\1\67\1\70\1\71\1\72\1\73\1\62\1\74"+
"\1\52\1\23\1\75\1\76\1\77\1\100\1\101\1\30"+
"\1\102\1\103\1\5\1\104\1\105\1\106\1\107\1\110"+
"\1\111\1\112\1\113\1\52\1\23\1\52\1\23\1\114"+
"\1\115\1\116\1\117\1\120\1\121\1\30\1\73\1\122"+
"\1\30\1\123\1\124\1\125\1\126\4\0\6\126\1\0"+
"\13\126\1\0\3\126\3\0\22\126\2\0\1\126\3\0"+
"\2\126\1\0\5\126\1\0\1\127\4\126\1\0\2\126"+
"\4\0\2\126\1\0\16\126\2\0\1\126\4\0\1\126"+
"\2\0\1\130\1\131\2\0\5\131\2\0\11\131\2\0"+
"\3\131\3\0\22\131\12\0\1\131\5\0\4\131\1\0"+
"\2\131\4\0\2\131\2\0\10\131\161\0\1\126\4\0"+
"\1\126\1\132\1\126\1\133\1\126\1\134\1\0\2\126"+
"\1\135\1\136\1\137\1\126\1\140\1\141\1\142\1\134"+
"\1\143\1\0\2\144\1\126\3\0\1\145\1\132\1\126"+
"\1\133\1\126\1\134\1\126\1\135\1\136\1\137\1\126"+
"\1\140\1\141\1\142\1\134\2\144\1\126\2\0\1\126"+
"\3\0\2\126\1\0\1\126\1\145\3\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\1\126\1\146\1\0\1\147\1\150\1\0"+
"\1\151\1\152\1\153\1\154\1\155\1\152\1\150\1\146"+
"\1\152\1\155\2\152\1\156\2\152\1\157\1\152\1\146"+
"\1\160\1\161\1\162\1\152\1\163\2\0\1\164\1\152"+
"\1\153\1\154\1\155\2\152\1\155\2\152\1\156\2\152"+
"\1\157\1\152\1\161\1\162\1\152\1\165\1\150\1\146"+
"\2\0\1\163\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\174\4\152\1\175\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\2\177\6\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\200\5\152\1\150\1\146\3\152"+
"\1\201\1\156\4\152\1\146\1\160\1\152\1\202\1\152"+
"\1\163\2\0\1\164\10\152\1\201\1\156\5\152\1\202"+
"\1\152\1\165\1\150\1\146\2\0\1\163\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\203\1\172\1\173\1\174"+
"\4\152\1\204\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\151\1\152"+
"\1\205\1\152\1\206\1\152\1\150\1\146\1\207\1\152"+
"\1\210\1\177\1\211\4\152\1\146\1\160\2\152\1\212"+
"\1\163\2\0\1\213\1\152\1\205\1\152\1\206\1\152"+
"\1\207\1\152\1\210\1\177\1\211\6\152\1\212\1\165"+
"\1\150\1\146\2\0\1\163\1\166\1\146\1\150\1\167"+
"\1\213\1\170\1\171\1\172\1\173\1\174\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\215\4\152\1\216\1\150"+
"\1\146\1\152\1\155\2\152\1\153\2\152\1\217\1\220"+
"\1\146\1\160\1\221\1\222\1\152\1\163\2\0\1\164"+
"\4\152\1\216\1\152\1\155\2\152\1\153\2\152\1\217"+
"\1\220\1\221\1\222\1\152\1\165\1\150\1\146\2\0"+
"\1\163\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\174\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\1\152\1\223\7\152"+
"\1\146\1\160\3\152\1\163\2\0\1\164\6\152\1\223"+
"\12\152\1\165\1\150\1\146\2\0\1\163\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\174"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\151\2\152"+
"\1\153\1\152\1\153\1\150\1\146\1\152\1\224\1\225"+
"\1\152\1\226\4\152\1\146\1\160\2\152\1\220\1\163"+
"\2\0\1\164\2\152\1\153\1\152\1\153\1\152\1\224"+
"\1\225\1\152\1\226\6\152\1\220\1\165\1\150\1\146"+
"\2\0\1\163\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\174\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\4\152\1\227\1\150\1\146\3\152"+
"\1\230\1\231\4\152\1\146\1\160\1\152\1\232\1\152"+
"\1\163\2\0\1\164\4\152\1\227\3\152\1\230\1\231"+
"\5\152\1\232\1\152\1\165\1\150\1\146\2\0\1\163"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\174\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\200\2\152\1\153\2\152\1\150\1\146\1\152\1\233"+
"\7\152\1\146\1\160\3\152\1\163\2\0\1\164\2\152"+
"\1\153\3\152\1\233\12\152\1\165\1\150\1\146\2\0"+
"\1\163\1\166\1\146\1\150\1\167\1\164\1\170\1\203"+
"\1\172\1\173\1\174\4\152\1\234\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\1\163\2\0\1\164\21\152\1\165\1\150\1\146"+
"\2\0\1\163\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\235\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\11\152\1\146"+
"\1\160\1\152\1\236\1\152\1\163\2\0\1\164\17\152"+
"\1\236\1\152\1\165\1\150\1\146\2\0\1\163\1\166"+
"\1\146\1\150\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\174\4\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\1\163"+
"\2\0\1\164\21\152\1\165\1\150\1\146\2\0\1\163"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\174\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\237\2\152\1\240\2\152\1\150\1\146\7\152\1\241"+
"\1\152\1\146\1\160\1\212\2\152\1\163\2\0\1\164"+
"\2\152\1\240\11\152\1\241\1\152\1\212\2\152\1\165"+
"\1\150\1\146\2\0\1\163\1\166\1\146\1\150\1\167"+
"\1\164\1\170\1\242\1\172\1\173\1\243\4\152\1\244"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\245\5\152\1\150\1\146"+
"\2\152\1\246\1\152\1\247\1\152\1\250\2\152\1\146"+
"\1\160\3\152\1\163\2\0\1\164\7\152\1\246\1\152"+
"\1\247\1\152\1\250\5\152\1\165\1\150\1\146\2\0"+
"\1\163\1\166\1\146\1\150\1\167\1\164\1\170\1\251"+
"\1\172\1\173\1\174\4\152\1\252\2\253\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\2\152\1\153\2\152\1\150\1\146\7\152"+
"\1\254\1\152\1\146\1\160\1\152\1\255\1\152\1\163"+
"\2\0\1\164\2\152\1\153\11\152\1\254\2\152\1\255"+
"\1\152\1\165\1\150\1\146\2\0\1\163\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\174"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\1\146\1\126\4\0\6\126\1\0\12\126\1\27"+
"\1\0\3\126\3\0\22\126\2\0\1\126\3\0\2\126"+
"\1\0\5\126\1\0\1\127\4\126\1\0\2\126\4\0"+
"\2\126\1\0\16\126\2\0\1\126\4\0\1\126\1\150"+
"\1\0\1\256\1\150\1\0\1\150\5\160\2\150\11\160"+
"\1\150\1\257\3\160\3\0\22\160\1\260\1\261\1\150"+
"\2\0\1\262\1\263\3\150\1\160\1\264\1\150\1\173"+
"\2\265\4\160\1\150\2\160\2\257\1\0\1\150\2\160"+
"\1\150\1\266\10\160\5\150\1\0\1\257\1\150\1\0"+
"\1\257\3\150\1\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\2\152\1\267\6\152\1\146\1\160"+
"\3\152\1\163\2\0\1\270\7\152\1\267\11\152\1\165"+
"\1\150\1\146\2\0\1\163\1\166\1\146\1\150\1\167"+
"\1\270\1\170\1\171\1\172\1\173\1\174\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\1\152\1\225\1\152\1\271\5\152\1\146\1\160\3\152"+
"\1\163\2\0\1\164\6\152\1\225\1\152\1\271\10\152"+
"\1\165\1\150\1\146\2\0\1\163\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\174\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\2\152\1\153"+
"\1\155\1\152\1\150\1\146\1\152\1\153\7\152\1\146"+
"\1\160\3\152\1\163\2\0\1\164\2\152\1\153\1\155"+
"\2\152\1\153\12\152\1\165\1\150\1\146\2\0\1\163"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\174\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\1\146\33\0\1\34\31\0\1\34"+
"\113\0\1\36\106\0\1\146\1\0\1\147\1\150\1\0"+
"\1\272\5\152\1\150\1\146\7\152\1\273\1\152\1\146"+
"\1\160\1\152\1\274\1\152\1\163\2\0\1\275\14\276"+
"\1\277\2\276\1\300\1\276\1\165\1\150\1\301\2\0"+
"\1\163\1\166\1\146\1\302\1\167\1\303\1\170\1\304"+
"\1\172\1\173\1\174\1\276\1\152\1\276\1\152\1\305"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\1\152\1\153"+
"\1\154\1\155\1\152\1\150\1\146\1\152\1\155\2\152"+
"\1\156\2\152\1\157\1\152\1\146\1\160\1\161\1\162"+
"\1\152\1\163\2\0\1\307\1\276\1\310\1\311\1\312"+
"\2\276\1\312\2\276\1\313\2\276\1\314\1\276\1\315"+
"\1\316\1\276\1\165\1\150\1\301\2\0\1\163\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\174\1\276\1\152\1\276\1\152\1\175\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\317"+
"\1\177\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\320\5\152\1\150\1\146\3\152"+
"\1\201\1\156\4\152\1\146\1\160\1\152\1\202\1\152"+
"\1\163\2\0\1\307\10\276\1\321\1\313\5\276\1\322"+
"\1\276\1\165\1\150\1\301\2\0\1\163\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\203\1\172\1\173\1\174"+
"\1\276\1\152\1\276\1\152\1\204\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\1\152\1\205\1\152\1\206\1\152"+
"\1\150\1\146\1\207\1\152\1\210\1\177\1\211\4\152"+
"\1\146\1\160\2\152\1\212\1\163\2\0\1\323\1\276"+
"\1\324\1\276\1\325\1\276\1\326\1\276\1\327\1\317"+
"\1\330\6\276\1\331\1\165\1\150\1\301\2\0\1\163"+
"\1\166\1\146\1\302\1\167\1\213\1\170\1\171\1\172"+
"\1\173\1\174\1\276\1\152\1\276\1\152\1\214\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\332\4\152\1\216\1\150"+
"\1\146\1\152\1\155\2\152\1\153\2\152\1\217\1\220"+
"\1\146\1\160\1\221\1\222\1\152\1\163\2\0\1\307"+
"\4\276\1\333\1\276\1\312\2\276\1\310\2\276\1\334"+
"\1\335\1\336\1\337\1\276\1\165\1\150\1\301\2\0"+
"\1\163\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\174\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\1\152\1\223\7\152\1\146\1\160\3\152\1\163"+
"\2\0\1\307\6\276\1\340\12\276\1\165\1\150\1\301"+
"\2\0\1\163\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\174\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\2\152"+
"\1\153\1\152\1\153\1\150\1\146\1\152\1\224\1\225"+
"\1\152\1\226\4\152\1\146\1\160\2\152\1\220\1\163"+
"\2\0\1\307\2\276\1\310\1\276\1\310\1\276\1\341"+
"\1\342\1\276\1\343\6\276\1\335\1\165\1\150\1\301"+
"\2\0\1\163\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\174\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\4\152"+
"\1\227\1\150\1\146\3\152\1\230\1\231\4\152\1\146"+
"\1\160\1\152\1\232\1\152\1\163\2\0\1\307\4\276"+
"\1\344\3\276\1\345\1\346\5\276\1\347\1\276\1\165"+
"\1\150\1\301\2\0\1\163\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\174\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\320\2\152\1\153\2\152\1\150\1\146\1\152\1\233"+
"\7\152\1\146\1\160\3\152\1\163\2\0\1\307\2\276"+
"\1\310\3\276\1\350\12\276\1\165\1\150\1\301\2\0"+
"\1\163\1\166\1\146\1\302\1\167\1\164\1\170\1\203"+
"\1\172\1\173\1\174\1\276\1\152\1\276\1\152\1\234"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\1\163\2\0\1\307"+
"\21\276\1\165\1\150\1\301\2\0\1\163\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\235"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\11\152\1\146"+
"\1\160\1\152\1\236\1\152\1\163\2\0\1\307\17\276"+
"\1\351\1\276\1\165\1\150\1\301\2\0\1\163\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\174\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\1\163\2\0\1\307\21\276\1\165"+
"\1\150\1\301\2\0\1\163\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\174\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\352\2\152\1\240\2\152\1\150\1\146\7\152\1\241"+
"\1\152\1\146\1\160\1\212\2\152\1\163\2\0\1\307"+
"\2\276\1\353\11\276\1\354\1\276\1\331\2\276\1\165"+
"\1\150\1\301\2\0\1\163\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\242\1\172\1\173\1\243\1\276\1\152"+
"\1\276\1\152\1\244\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\355\5\152\1\150\1\146\2\152\1\246\1\152\1\247"+
"\1\152\1\250\2\152\1\146\1\160\3\152\1\163\2\0"+
"\1\307\7\276\1\356\1\276\1\357\1\276\1\360\5\276"+
"\1\165\1\150\1\301\2\0\1\163\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\361\1\172\1\173\1\174\1\276"+
"\1\152\1\276\1\152\1\362\1\363\1\253\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\306\2\152\1\153\2\152\1\150\1\146\7\152"+
"\1\254\1\152\1\146\1\160\1\152\1\255\1\152\1\163"+
"\2\0\1\307\2\276\1\310\11\276\1\364\2\276\1\365"+
"\1\276\1\165\1\150\1\301\2\0\1\163\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\174"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\2\152\1\267"+
"\6\152\1\146\1\160\3\152\1\163\2\0\1\366\7\276"+
"\1\367\11\276\1\165\1\150\1\301\2\0\1\163\1\166"+
"\1\146\1\302\1\167\1\270\1\170\1\171\1\172\1\173"+
"\1\174\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\1\152"+
"\1\225\1\152\1\271\5\152\1\146\1\160\3\152\1\163"+
"\2\0\1\307\6\276\1\342\1\276\1\370\10\276\1\165"+
"\1\150\1\301\2\0\1\163\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\174\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\2\152\1\153\1\155\1\152\1\150\1\146\1\152"+
"\1\153\7\152\1\146\1\160\3\152\1\163\2\0\1\307"+
"\2\276\1\310\1\312\2\276\1\310\12\276\1\165\1\150"+
"\1\301\2\0\1\163\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\174\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\1\146\17\0\1\371\1\0\1\371\5\0"+
"\1\372\16\0\1\371\1\0\1\371\7\0\1\373\1\374"+
"\14\0\2\374\7\0\2\372\24\0\1\372\2\0\1\372"+
"\32\0\1\372\57\0\2\372\24\0\1\372\2\0\1\372"+
"\3\0\1\126\4\0\6\126\1\0\13\126\1\372\3\126"+
"\3\0\22\126\1\0\1\374\1\126\3\0\2\126\1\0"+
"\5\126\1\374\1\375\4\126\1\0\2\126\2\372\2\0"+
"\2\126\1\0\16\126\1\0\1\372\1\126\1\0\1\372"+
"\2\0\1\126\27\0\1\376\31\0\1\377\14\0\2\377"+
"\7\0\2\376\24\0\1\376\2\0\1\376\3\0\1\126"+
"\4\0\6\126\1\0\13\126\1\0\3\126\3\0\22\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\14\126\1\u0100"+
"\1\126\2\0\1\126\4\0\2\126\4\0\1\u0101\5\67"+
"\1\0\1\126\11\67\1\126\1\0\3\67\3\0\22\67"+
"\2\0\1\126\3\0\2\126\1\0\2\67\1\126\1\u0102"+
"\1\126\1\0\1\u0103\4\67\1\u0104\2\67\4\0\2\67"+
"\1\0\1\126\10\67\5\126\2\0\1\126\4\0\1\126"+
"\1\146\1\0\1\147\1\150\1\0\1\u0105\5\152\1\150"+
"\1\146\7\152\1\273\1\152\1\146\1\160\1\152\1\274"+
"\1\152\1\163\2\0\1\303\14\152\1\273\2\152\1\274"+
"\1\152\1\165\1\150\1\146\2\0\1\163\1\166\1\146"+
"\1\150\1\167\1\303\1\170\1\304\1\172\1\173\1\174"+
"\4\152\1\305\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\1\146\1\126\4\0\6\126\1\0\13\126\1\0"+
"\3\126\3\0\22\126\2\0\1\126\3\0\2\126\1\0"+
"\2\126\1\71\2\126\1\0\1\127\4\126\1\0\2\126"+
"\4\0\2\126\1\0\16\126\2\0\1\126\4\0\2\126"+
"\4\0\1\126\2\u0106\1\126\1\u0106\1\126\1\0\2\126"+
"\1\u0107\1\u0108\1\126\1\u0109\1\126\1\u010a\3\126\1\u010b"+
"\1\126\1\u010c\1\u0109\3\0\1\u010d\2\u0106\1\126\1\u0106"+
"\2\126\1\u0107\1\u0108\1\126\1\u0109\1\126\1\u010a\3\126"+
"\1\u010c\1\u0109\2\0\1\126\3\0\2\126\1\0\1\126"+
"\1\u010d\1\126\1\u010e\1\126\1\0\1\127\4\126\1\0"+
"\2\126\1\u010f\1\u010b\2\0\2\126\1\0\15\126\1\u010e"+
"\1\0\1\u010f\1\126\1\0\1\u010f\2\0\1\126\27\0"+
"\1\372\35\0\1\u0110\11\0\1\u0111\7\0\2\372\24\0"+
"\1\372\2\0\1\372\3\0\1\146\1\0\1\147\1\150"+
"\1\0\1\306\2\152\1\u0112\2\152\1\150\1\146\3\152"+
"\1\u0113\1\153\2\152\1\u0114\1\152\1\146\1\160\3\152"+
"\1\163\2\0\1\307\2\276\1\u0115\5\276\1\u0116\1\310"+
"\2\276\1\u0117\4\276\1\165\1\150\1\301\2\0\1\163"+
"\1\166\1\146\1\302\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\174\1\276\1\152\1\276\1\152\1\u0118\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\2\152\1\u0112\2\152"+
"\1\150\1\146\3\152\1\u0113\1\153\2\152\1\u0114\1\152"+
"\1\146\1\160\3\152\1\163\2\0\1\164\2\152\1\u0112"+
"\5\152\1\u0113\1\153\2\152\1\u0114\4\152\1\165\1\150"+
"\1\146\2\0\1\163\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\174\4\152\1\u0118\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\1\146\6\0"+
"\2\u0119\1\u011a\1\u0119\1\u011a\2\0\1\u011a\1\u011b\1\u011c"+
"\1\u011a\1\u011d\1\u011a\1\u011e\2\u011a\1\0\1\u010b\1\u011a"+
"\1\u011f\1\u011d\3\0\1\u0120\2\u0119\1\u011a\1\u0119\2\u011a"+
"\1\u011b\1\u011c\1\u011a\1\u011d\1\u011a\1\u011e\3\u011a\1\u011f"+
"\1\u011d\12\0\1\u0120\5\0\4\u011a\1\u0121\2\u011a\1\u010f"+
"\1\u010b\2\0\2\u011a\2\0\10\u011a\6\0\1\u010f\2\0"+
"\1\u010f\3\0\1\146\1\0\1\147\1\150\1\0\1\306"+
"\2\152\1\u0122\2\152\1\150\1\146\7\152\1\u0123\1\152"+
"\1\146\1\160\3\152\1\163\2\0\1\307\2\276\1\u0124"+
"\11\276\1\u0125\4\276\1\165\1\150\1\301\2\0\1\163"+
"\1\166\1\146\1\302\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\174\1\276\1\152\1\276\1\152\1\214\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\310\1\153"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\2\152\1\u0122\2\152"+
"\1\150\1\146\7\152\1\u0123\1\152\1\146\1\160\3\152"+
"\1\163\2\0\1\164\2\152\1\u0122\11\152\1\u0123\4\152"+
"\1\165\1\150\1\146\2\0\1\163\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\174\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\6\152\2\153\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\1\146\1\150\1\0\1\256\1\150\1\0\1\150"+
"\5\160\2\150\11\160\1\150\1\257\3\160\3\0\22\160"+
"\1\260\1\261\1\150\2\0\1\262\1\263\3\150\1\160"+
"\1\264\1\150\1\173\2\265\2\u0126\2\160\1\150\2\160"+
"\2\257\1\0\1\150\2\160\1\150\1\266\10\160\5\150"+
"\1\0\1\257\1\150\1\0\1\257\3\150\1\146\1\0"+
"\1\147\1\150\1\0\1\306\2\152\1\u0127\2\152\1\150"+
"\1\146\11\152\1\146\1\160\1\u0128\1\u0129\1\152\1\163"+
"\2\0\1\307\2\276\1\u012a\13\276\1\u012b\1\u012c\1\276"+
"\1\165\1\150\1\301\2\0\1\163\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\174\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\u012d\1\u012e\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\u012f\1\u0130\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\2\152\1\u0127\2\152\1\150\1\146\11\152"+
"\1\146\1\160\1\u0128\1\u0129\1\152\1\163\2\0\1\164"+
"\2\152\1\u0127\13\152\1\u0128\1\u0129\1\152\1\165\1\150"+
"\1\146\2\0\1\163\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\174\4\152\1\214\2\152"+
"\2\160\1\0\1\150\2\u012e\1\150\1\176\6\152\2\u0130"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\1\146"+
"\115\0\1\u0121\26\0\1\126\4\0\6\126\1\0\13\126"+
"\1\0\3\126\3\0\22\126\2\0\1\126\3\0\2\126"+
"\1\0\5\126\1\0\1\127\4\126\1\0\2\126\4\0"+
"\2\126\1\0\1\107\15\126\2\0\1\126\4\0\1\126"+
"\1\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\1\152\1\153\1\u0131\1\152\1\u0132\4\152\1\146"+
"\1\160\1\152\1\u0133\1\152\1\163\2\0\1\307\6\276"+
"\1\310\1\u0134\1\276\1\u0135\5\276\1\u0136\1\276\1\165"+
"\1\150\1\301\2\0\1\163\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\174\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\1\152\1\153\1\u0131\1\152"+
"\1\u0132\4\152\1\146\1\160\1\152\1\u0133\1\152\1\163"+
"\2\0\1\164\6\152\1\153\1\u0131\1\152\1\u0132\5\152"+
"\1\u0133\1\152\1\165\1\150\1\146\2\0\1\163\1\166"+
"\1\146\1\150\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\174\4\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\2\152\1\u0137\1\152\1\u0129\1\150\1\146\4\152\1\u0138"+
"\4\152\1\146\1\160\3\152\1\163\2\0\1\307\2\276"+
"\1\u0139\1\276\1\u012c\4\276\1\u013a\7\276\1\165\1\150"+
"\1\301\2\0\1\163\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\174\1\276\1\152\1\276"+
"\1\152\1\u013b\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\2\152\1\u0137\1\152\1\u0129\1\150\1\146\4\152\1\u0138"+
"\4\152\1\146\1\160\3\152\1\163\2\0\1\164\2\152"+
"\1\u0137\1\152\1\u0129\4\152\1\u0138\7\152\1\165\1\150"+
"\1\146\2\0\1\163\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\174\4\152\1\u013b\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\1\146\1\126"+
"\4\0\6\126\1\0\13\126\1\0\3\126\3\0\22\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\14\126\1\117"+
"\1\126\2\0\1\126\4\0\2\126\4\0\6\126\1\0"+
"\13\126\1\0\3\126\3\0\22\126\2\0\1\126\3\0"+
"\2\126\1\0\3\126\1\u010e\1\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\15\126\1\u010e\2\0"+
"\1\126\4\0\1\126\6\0\5\u013c\2\0\11\u013c\1\0"+
"\4\u013c\3\0\22\u013c\10\0\1\u013c\1\0\1\u013c\5\0"+
"\4\u013c\1\0\4\u013c\2\0\2\u013c\2\0\10\u013c\6\0"+
"\1\u013c\2\0\1\u013c\3\0\1\126\4\0\6\126\1\0"+
"\7\126\1\u013d\1\u013e\2\126\1\0\1\126\1\u013f\1\126"+
"\3\0\1\u0140\13\126\1\u013d\1\u013e\2\126\1\u013f\1\126"+
"\2\0\1\126\3\0\2\126\1\0\1\126\1\u0140\3\126"+
"\2\0\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\1\126\3\0\1\131\2\0\5\131"+
"\2\0\11\131\2\0\3\131\3\0\22\131\12\0\1\131"+
"\5\0\4\131\1\0\2\131\4\0\2\131\2\0\10\131"+
"\15\0\4\131\1\5\137\131\1\126\4\0\2\126\1\u0141"+
"\3\126\1\0\13\126\1\0\3\126\3\0\2\126\1\u0141"+
"\17\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\1\126\1\u0142\1\126"+
"\1\u0143\2\126\1\0\1\126\1\u0144\2\126\1\u0145\4\126"+
"\1\u0146\1\126\1\0\3\126\3\0\1\126\1\u0142\1\126"+
"\1\u0143\2\126\1\u0144\2\126\1\u0145\4\126\1\u0146\3\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\2\126\4\0\6\126\1\0\2\126\1\u0147"+
"\10\126\1\0\3\126\3\0\7\126\1\u0147\12\126\2\0"+
"\1\126\3\0\2\126\1\0\5\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\2\126\4\0\6\126\1\0\3\126\1\u0147\7\126"+
"\1\0\3\126\3\0\10\126\1\u0147\11\126\2\0\1\126"+
"\3\0\2\126\1\0\5\126\1\0\1\127\4\126\1\0"+
"\2\126\4\0\2\126\1\0\16\126\2\0\1\126\4\0"+
"\2\126\4\0\6\126\1\0\2\126\1\u0147\2\126\1\u0147"+
"\5\126\1\0\3\126\3\0\7\126\1\u0147\2\126\1\u0147"+
"\7\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\3\126\1\u0143\2\126"+
"\1\0\4\126\1\u0145\1\u0147\3\126\1\u0146\1\126\1\0"+
"\3\126\3\0\3\126\1\u0143\5\126\1\u0145\1\u0147\3\126"+
"\1\u0146\3\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\6\126\1\0"+
"\3\126\1\u0147\1\u0148\1\u0147\1\126\1\u0147\3\126\1\0"+
"\3\126\3\0\10\126\1\u0147\1\u0148\1\u0147\1\126\1\u0147"+
"\5\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\2\126\1\u0149\3\126"+
"\1\0\13\126\1\0\3\126\3\0\2\126\1\u0149\17\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\2\126\4\0\2\126\1\u0149\1\u0143\2\126"+
"\1\0\4\126\1\u0145\4\126\1\u0146\1\126\1\0\3\126"+
"\3\0\2\126\1\u0149\1\u0143\5\126\1\u0145\4\126\1\u0146"+
"\3\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\6\126\1\0\13\126"+
"\1\u014a\3\126\3\0\22\126\2\0\1\126\3\0\2\126"+
"\1\0\5\126\1\0\1\127\4\126\1\0\2\126\2\u014a"+
"\2\0\2\126\1\0\16\126\1\0\1\u014a\1\126\1\0"+
"\1\u014a\2\0\2\126\4\0\3\126\1\u0143\2\126\1\0"+
"\4\126\1\u0145\4\126\1\u0146\1\126\1\0\3\126\3\0"+
"\3\126\1\u0143\5\126\1\u0145\4\126\1\u0146\3\126\2\0"+
"\1\126\3\0\2\126\1\0\5\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\2\126\4\0\6\126\1\0\13\126\1\0\3\126"+
"\3\0\22\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\3\126\2\u014b\11\126\2\0\1\126\4\0\1\126\1\146"+
"\1\0\2\150\1\0\6\146\1\150\13\146\1\150\3\146"+
"\3\0\22\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\1\146\1\150\1\0\2\150\1\0\1\150\5\u014d"+
"\2\150\11\u014d\1\150\4\u014d\3\0\22\u014d\3\150\3\0"+
"\4\150\1\u014d\5\150\4\u014d\1\150\4\u014d\1\0\1\150"+
"\2\u014d\1\150\1\266\10\u014d\5\150\1\0\1\u014d\1\150"+
"\1\0\1\u014d\4\150\1\0\2\150\1\0\26\150\3\0"+
"\25\150\3\0\23\150\1\0\4\150\1\266\15\150\1\0"+
"\2\150\1\0\4\150\1\146\1\0\2\150\1\0\3\146"+
"\1\u014e\2\146\1\150\4\146\1\u014f\3\146\1\u014f\2\146"+
"\1\150\2\u014f\1\146\3\0\3\146\1\u014e\5\146\1\u014f"+
"\3\146\1\u014f\1\146\2\u014f\1\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\3\0\1\164\21\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\164\21\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0151\4\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\3\152\1\u0128\1\152\1\150\1\146\4\152\1\153\4\152"+
"\1\146\1\160\3\152\3\0\1\164\3\152\1\u0128\5\152"+
"\1\153\7\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\2\152\2\155\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\1\163"+
"\2\0\1\164\21\152\1\165\1\150\1\146\2\0\1\163"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0152\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\3\152\1\155\1\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\1\163\2\0\1\164\3\152\1\155\15\152"+
"\1\165\1\150\1\146\2\0\1\163\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0152\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\5\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\u0153\21\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\u0153\1\170\1\171\1\172\1\173\1\u0151\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\1\146"+
"\1\150\1\0\1\147\1\150\1\0\1\150\5\160\2\150"+
"\11\160\1\150\4\160\3\0\22\160\1\165\2\150\3\0"+
"\1\263\3\150\1\160\1\264\1\150\3\173\4\160\1\150"+
"\4\160\1\0\1\150\2\160\1\150\1\266\10\160\5\150"+
"\1\0\1\160\1\150\1\0\1\160\3\150\1\146\1\0"+
"\1\147\1\150\1\0\1\151\3\152\1\u0154\1\152\1\150"+
"\1\146\6\152\1\u0155\2\152\1\146\1\160\3\152\3\0"+
"\1\u0156\3\152\1\u0154\7\152\1\u0155\5\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\u0156\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\3\152\1\u0157\1\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\164\3\152\1\u0157"+
"\15\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\1\146\33\0\1\163\31\0\1\163\56\0\1\146\1\0"+
"\1\147\1\150\1\0\1\u0158\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\303\21\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\303\1\170"+
"\1\u0159\1\172\1\173\1\u0150\4\152\1\u015a\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\1\146\1\150\1\0"+
"\2\150\1\0\1\150\5\u015b\2\150\11\u015b\1\150\1\u015c"+
"\3\u015b\3\0\22\u015b\3\150\3\0\4\150\1\u015b\5\150"+
"\4\u015b\1\150\2\u015b\2\u015c\1\0\1\150\2\u015b\1\150"+
"\1\266\10\u015b\5\150\1\0\1\u015c\1\150\1\0\1\u015c"+
"\3\150\1\146\1\0\1\147\1\150\1\0\6\146\1\150"+
"\13\146\1\150\3\146\3\0\22\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\1\151\5\167\1\150\1\146\11\167\1\146\1\150\3\167"+
"\3\0\22\167\2\150\1\146\3\0\2\146\1\150\2\167"+
"\1\146\1\171\1\146\1\150\1\u015d\4\167\1\214\2\167"+
"\2\150\1\0\1\150\2\167\1\150\1\176\10\167\5\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\2\150"+
"\1\0\1\146\5\u015e\1\150\1\146\11\u015e\1\146\1\u015f"+
"\3\u015e\3\0\22\u015e\2\150\1\146\3\0\2\146\1\150"+
"\1\146\1\u015e\3\146\1\150\1\u014c\4\u015e\1\150\2\u015e"+
"\2\u015f\1\0\1\150\2\u015e\1\150\1\176\10\u015e\5\146"+
"\1\0\1\u015f\1\146\1\0\1\u015f\2\150\2\146\1\0"+
"\2\150\1\0\1\146\2\u0160\1\146\1\u0160\1\146\1\150"+
"\3\146\1\u0161\1\146\1\u0162\5\146\1\150\2\146\1\u0162"+
"\3\0\1\146\2\u0160\1\146\1\u0160\3\146\1\u0161\1\146"+
"\1\u0162\6\146\1\u0162\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\1\146\5\172"+
"\1\150\1\146\11\172\1\146\1\173\3\172\3\0\22\172"+
"\1\u0163\1\150\1\146\3\0\2\146\1\150\1\146\1\172"+
"\2\146\1\172\1\173\1\u0164\4\172\1\150\2\172\2\173"+
"\1\0\1\150\2\172\1\150\1\176\10\172\5\146\1\0"+
"\1\173\1\146\1\0\1\173\2\150\1\146\1\150\1\0"+
"\2\150\1\0\1\150\5\173\2\150\11\173\1\150\4\173"+
"\3\0\22\173\1\u0163\2\150\3\0\4\150\1\173\2\150"+
"\7\173\1\150\4\173\1\0\1\150\2\173\1\150\1\266"+
"\10\173\5\150\1\0\1\173\1\150\1\0\1\173\3\150"+
"\1\146\1\0\2\150\1\0\1\146\5\u0165\1\u0166\1\146"+
"\6\u0165\1\u0167\1\u0168\1\u0165\1\146\1\173\1\u0165\1\u0169"+
"\1\u0165\3\0\1\u016a\13\u0165\1\u0167\1\u0168\2\u0165\1\u0169"+
"\1\u0165\1\u0163\1\u0166\1\146\3\0\2\146\1\150\1\146"+
"\1\u016a\2\146\1\172\1\u016b\1\173\4\u0165\1\150\2\u0165"+
"\2\173\1\0\1\150\2\u0165\1\150\1\176\10\u0165\5\146"+
"\1\0\1\173\1\146\1\0\1\173\2\150\1\146\1\150"+
"\1\0\2\150\1\0\1\150\2\u0166\1\150\1\u0166\5\150"+
"\1\u016c\1\150\1\u016d\10\150\1\u016d\3\0\1\150\2\u0166"+
"\1\150\1\u0166\3\150\1\u016c\1\150\1\u016d\6\150\1\u016d"+
"\3\150\3\0\23\150\1\0\4\150\1\266\2\150\2\u016e"+
"\11\150\1\0\2\150\1\0\4\150\1\u016f\1\0\2\u0170"+
"\1\0\6\u016f\1\u0170\13\u016f\1\u0170\3\u016f\3\0\22\u016f"+
"\2\u0170\1\u016f\3\0\2\u016f\1\u0170\5\u016f\1\u0170\1\u014c"+
"\4\u016f\1\u0170\2\u016f\2\u0170\1\0\1\u0170\2\u016f\1\u0170"+
"\16\u016f\1\0\1\u0170\1\u016f\1\0\3\u0170\1\u016f\1\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\10\152\1\153\1\146\1\160\3\152\3\0\1\164\15\152"+
"\1\153\3\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\2\150\1\0\3\146\1\u0171\2\146"+
"\1\150\4\146\1\u014f\3\146\1\u014f\2\146\1\150\2\u014f"+
"\1\146\3\0\3\146\1\u0171\5\146\1\u014f\3\146\1\u014f"+
"\1\146\2\u014f\1\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\u0172\21\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\u0172\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\151\5\152"+
"\1\150\1\146\1\250\1\152\1\153\3\152\1\153\2\152"+
"\1\146\1\160\3\152\3\0\1\164\5\152\1\250\1\152"+
"\1\153\3\152\1\153\5\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\2\150\1\0\1\146"+
"\2\u0173\1\u0174\1\u0173\1\u0174\1\150\1\146\2\u0174\1\u0175"+
"\1\u0174\1\u0176\4\u0174\1\146\1\u0177\2\u0174\1\u0176\3\0"+
"\1\u0174\2\u0173\1\u0174\1\u0173\3\u0174\1\u0175\1\u0174\1\u0176"+
"\6\u0174\1\u0176\2\150\1\146\3\0\2\146\1\150\1\146"+
"\1\u0174\3\146\1\150\1\u014c\4\u0174\1\150\2\u0174\2\u0177"+
"\1\0\1\150\2\u0174\1\150\1\176\10\u0174\5\146\1\0"+
"\1\u0177\1\146\1\0\1\u0177\2\150\1\146\1\150\1\0"+
"\2\150\1\0\1\150\2\u0178\1\u0179\1\u0178\1\u0177\2\150"+
"\2\u0177\1\u017a\1\u0177\1\u017b\4\u0177\1\150\3\u0177\1\u017b"+
"\3\0\1\u0177\2\u0178\1\u0179\1\u0178\3\u0177\1\u017a\1\u0177"+
"\1\u017b\6\u0177\1\u017b\3\150\3\0\4\150\1\u0177\5\150"+
"\4\u0177\1\150\4\u0177\1\0\1\150\2\u0177\1\150\1\266"+
"\10\u0177\5\150\1\0\1\u0177\1\150\1\0\1\u0177\3\150"+
"\1\146\1\0\1\147\1\150\1\0\1\151\1\155\4\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\164"+
"\1\155\20\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\151\3\152"+
"\1\u0122\1\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\164\3\152\1\u0122\15\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\4\152\1\153\4\152"+
"\1\146\1\160\3\152\3\0\1\164\11\152\1\153\7\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\2\152\1\153\2\152"+
"\1\150\1\146\11\152\1\146\1\160\1\152\1\u017c\1\152"+
"\3\0\1\164\2\152\1\153\14\152\1\u017c\1\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\151\5\152\1\150\1\146\11\152"+
"\1\146\1\160\1\u017d\2\152\3\0\1\164\16\152\1\u017d"+
"\2\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\153\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\5\152\1\150"+
"\1\146\11\152\1\146\1\160\1\152\1\155\1\152\3\0"+
"\1\164\17\152\1\155\1\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\u0158\5\152\1\150\1\146\1\152\1\u017e\7\152\1\146"+
"\1\160\3\152\3\0\1\303\6\152\1\u017e\12\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\303"+
"\1\170\1\u0159\1\172\1\173\1\u0150\4\152\1\u015a\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\1\146\1\150"+
"\1\0\2\150\1\0\1\150\2\u0166\1\150\1\u0166\5\150"+
"\1\u016c\1\150\1\u016d\10\150\1\u016d\3\0\1\150\2\u0166"+
"\1\150\1\u0166\3\150\1\u016c\1\150\1\u016d\6\150\1\u016d"+
"\3\150\3\0\23\150\1\0\4\150\1\266\15\150\1\0"+
"\2\150\1\0\4\150\1\146\1\0\2\150\1\0\3\146"+
"\1\u014e\2\146\1\150\1\146\1\u017f\1\146\1\u0180\1\u014f"+
"\3\146\1\u014f\2\146\1\150\2\u014f\1\146\3\0\3\146"+
"\1\u014e\2\146\1\u017f\1\146\1\u0180\1\u014f\3\146\1\u014f"+
"\1\146\2\u014f\1\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\11\152\1\146\1\160\1\u0181\2\152"+
"\3\0\1\164\16\152\1\u0181\2\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\1\u0182\4\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\164\1\u0182\20\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\1\152\1\155"+
"\7\152\1\146\1\160\3\152\3\0\1\164\6\152\1\155"+
"\12\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\5\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\u0183\21\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\u0183\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\1\u0184\10\152\1\146\1\160\3\152\3\0\1\u0185\5\152"+
"\1\u0184\13\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\u0185\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\151\5\152"+
"\1\150\1\146\1\152\1\u0186\7\152\1\146\1\160\3\152"+
"\3\0\1\164\6\152\1\u0186\12\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\3\0\1\164\21\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\6\152\2\153\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\6\152\1\153\2\152"+
"\1\146\1\160\3\152\3\0\1\164\13\152\1\153\5\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\7\152\1\u0187\1\152\1\146\1\160\3\152\3\0\1\164"+
"\14\152\1\u0187\4\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\3\152\1\u0188\5\152\1\146\1\160"+
"\3\152\3\0\1\164\10\152\1\u0188\10\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\11\152\1\146"+
"\1\160\1\152\1\u0189\1\152\3\0\1\164\17\152\1\u0189"+
"\1\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\5\152\1\150"+
"\1\146\11\152\1\146\1\160\1\152\1\u018a\1\152\3\0"+
"\1\164\17\152\1\u018a\1\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\u0156\21\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\u0156\1\170\1\171\1\172\1\173"+
"\1\u0150\2\153\2\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\1\152\1\153\3\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\1\163\2\0\1\164\1\152\1\153\17\152"+
"\1\165\1\150\1\146\2\0\1\163\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0152\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\1\146\1\150\1\0\2\150\1\0\1\150\2\u0178\1\u0177"+
"\1\u0178\1\u0177\2\150\2\u0177\1\u017a\1\u0177\1\u017b\4\u0177"+
"\1\150\3\u0177\1\u017b\3\0\1\u0177\2\u0178\1\u0177\1\u0178"+
"\3\u0177\1\u017a\1\u0177\1\u017b\6\u0177\1\u017b\3\150\3\0"+
"\4\150\1\u0177\5\150\4\u0177\1\150\4\u0177\1\0\1\150"+
"\2\u0177\1\150\1\266\10\u0177\5\150\1\0\1\u0177\1\150"+
"\1\0\1\u0177\3\150\1\146\1\0\2\150\1\0\1\146"+
"\3\u0165\1\u018b\1\u0165\1\u0166\1\146\6\u0165\1\u0167\1\u0168"+
"\1\u0165\1\146\1\173\1\u0165\1\u0169\1\u0165\3\0\1\u016a"+
"\3\u0165\1\u018b\7\u0165\1\u0167\1\u0168\2\u0165\1\u0169\1\u0165"+
"\1\u0163\1\u0166\1\146\3\0\2\146\1\150\1\146\1\u016a"+
"\2\146\1\172\1\u016b\1\173\4\u0165\1\150\2\u0165\2\173"+
"\1\0\1\150\2\u0165\1\150\1\176\10\u0165\5\146\1\0"+
"\1\173\1\146\1\0\1\173\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\1\156\10\152"+
"\1\146\1\160\2\152\1\155\3\0\1\164\5\152\1\156"+
"\12\152\1\155\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\2\150\1\0\3\146\1\u018c\2\146"+
"\1\150\4\146\1\u014f\3\146\1\u014f\2\146\1\150\2\u014f"+
"\1\146\3\0\3\146\1\u018c\5\146\1\u014f\3\146\1\u014f"+
"\1\146\2\u014f\1\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\1\220\1\152\1\u018d\6\152\1\146"+
"\1\160\3\152\3\0\1\u018e\5\152\1\220\1\152\1\u018d"+
"\11\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\u018e\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\3\152\1\153"+
"\1\152\1\150\1\146\2\152\1\u018f\1\152\1\u0190\4\152"+
"\1\146\1\160\3\152\3\0\1\u0191\3\152\1\153\3\152"+
"\1\u018f\1\152\1\u0190\7\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\u0191\1\170\1\171\1\172"+
"\1\173\1\u0151\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\2\150\1\0\1\146"+
"\1\u0192\1\u0160\1\146\1\u0160\1\146\1\150\3\146\1\u0161"+
"\1\146\1\u0162\5\146\1\150\2\146\1\u0162\3\0\1\146"+
"\1\u0192\1\u0160\1\146\1\u0160\3\146\1\u0161\1\146\1\u0162"+
"\6\146\1\u0162\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\1\146\1\u0165\1\u0193"+
"\3\u0165\1\u0166\1\146\6\u0165\1\u0167\1\u0168\1\u0165\1\146"+
"\1\173\1\u0165\1\u0169\1\u0165\3\0\1\u016a\1\u0165\1\u0193"+
"\11\u0165\1\u0167\1\u0168\2\u0165\1\u0169\1\u0165\1\u0163\1\u0166"+
"\1\146\3\0\2\146\1\150\1\146\1\u016a\2\146\1\172"+
"\1\u016b\1\173\4\u0165\1\150\2\u0165\2\173\1\0\1\150"+
"\2\u0165\1\150\1\176\10\u0165\5\146\1\0\1\173\1\146"+
"\1\0\1\173\2\150\1\146\1\150\1\0\2\150\1\0"+
"\1\150\1\u0194\1\u0166\1\150\1\u0166\5\150\1\u016c\1\150"+
"\1\u016d\10\150\1\u016d\3\0\1\150\1\u0194\1\u0166\1\150"+
"\1\u0166\3\150\1\u016c\1\150\1\u016d\6\150\1\u016d\3\150"+
"\3\0\23\150\1\0\4\150\1\266\15\150\1\0\2\150"+
"\1\0\4\150\1\146\1\0\2\150\1\0\3\146\1\u0195"+
"\2\146\1\150\4\146\1\u014f\3\146\1\u014f\2\146\1\150"+
"\2\u014f\1\146\3\0\3\146\1\u0195\5\146\1\u014f\3\146"+
"\1\u014f\1\146\2\u014f\1\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\1\147\1\150\1\0"+
"\1\u0196\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\164\21\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\164\1\170\1\u0197\1\172\1\173"+
"\1\u0150\4\152\1\u0118\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\11\152\1\146\1\160\1\152\1\153"+
"\1\152\3\0\1\164\17\152\1\153\1\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\1\152\1\153"+
"\7\152\1\146\1\160\3\152\3\0\1\164\6\152\1\153"+
"\12\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\2\150\1\0\1\146\2\u0160\1\146\1\u0160"+
"\1\146\1\150\3\146\1\u0161\1\146\1\u0162\1\146\1\u0198"+
"\3\146\1\150\2\146\1\u0162\3\0\1\146\2\u0160\1\146"+
"\1\u0160\3\146\1\u0161\1\146\1\u0162\1\146\1\u0198\4\146"+
"\1\u0162\2\150\1\146\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150\2\146"+
"\1\150\1\176\15\146\1\0\1\150\1\146\1\0\3\150"+
"\1\146\1\150\1\0\2\150\1\0\1\150\2\u0166\1\150"+
"\1\u0166\5\150\1\u016c\1\150\1\u016d\1\150\1\u0199\6\150"+
"\1\u016d\3\0\1\150\2\u0166\1\150\1\u0166\3\150\1\u016c"+
"\1\150\1\u016d\1\150\1\u0199\4\150\1\u016d\3\150\3\0"+
"\23\150\1\0\4\150\1\266\15\150\1\0\2\150\1\0"+
"\4\150\1\146\1\0\1\147\1\150\1\0\1\151\5\152"+
"\1\150\1\146\2\152\1\u0131\6\152\1\146\1\160\3\152"+
"\3\0\1\164\7\152\1\u0131\11\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\11\152\1\146\1\160"+
"\2\152\1\156\3\0\1\164\20\152\1\156\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\u019a\21\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\u019a\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\1\146\1\150\1\0\2\150"+
"\1\0\1\150\5\u014d\2\150\11\u014d\1\150\1\u019b\3\u014d"+
"\3\0\22\u014d\3\150\3\0\4\150\1\u014d\5\150\4\u014d"+
"\1\150\2\u014d\2\u019b\1\0\1\150\2\u014d\1\150\1\266"+
"\10\u014d\5\150\1\0\1\u019b\1\150\1\0\1\u019b\4\150"+
"\1\0\1\256\1\150\1\0\1\150\5\160\2\150\11\160"+
"\1\150\1\u019c\3\160\3\0\22\160\1\260\1\261\1\150"+
"\2\0\1\262\1\263\3\150\1\160\1\264\1\150\1\173"+
"\2\265\4\160\1\150\2\160\2\u019c\1\0\1\150\2\160"+
"\1\150\1\266\10\160\5\150\1\0\1\u019c\1\150\1\0"+
"\1\u019c\4\150\1\0\2\150\1\0\1\150\5\u015b\2\150"+
"\11\u015b\1\150\1\u019d\3\u015b\3\0\22\u015b\3\150\3\0"+
"\4\150\1\u015b\5\150\4\u015b\1\150\2\u015b\2\u019d\1\0"+
"\1\150\2\u015b\1\150\1\266\10\u015b\5\150\1\0\1\u019d"+
"\1\150\1\0\1\u019d\4\150\1\0\2\150\1\0\22\150"+
"\1\u019e\3\150\3\0\25\150\3\0\21\150\2\u019e\1\0"+
"\4\150\1\266\15\150\1\0\1\u019e\1\150\1\0\1\u019e"+
"\3\150\27\0\1\u019f\57\0\2\u019f\24\0\1\u019f\2\0"+
"\1\u019f\3\0\1\150\1\0\1\147\1\150\1\0\26\150"+
"\3\0\25\150\3\0\23\150\1\0\4\150\1\266\15\150"+
"\1\0\2\150\1\0\5\150\1\0\2\150\1\0\1\150"+
"\5\u015f\2\150\11\u015f\1\150\4\u015f\3\0\22\u015f\3\150"+
"\3\0\4\150\1\u015f\5\150\4\u015f\1\150\4\u015f\1\0"+
"\1\150\2\u015f\1\150\1\266\10\u015f\5\150\1\0\1\u015f"+
"\1\150\1\0\1\u015f\4\150\1\0\2\150\1\0\1\150"+
"\5\173\2\150\11\173\1\150\1\u01a0\3\173\3\0\22\173"+
"\1\u0163\2\150\3\0\4\150\1\173\2\150\7\173\1\150"+
"\2\173\2\u01a0\1\0\1\150\2\173\1\150\1\266\10\173"+
"\5\150\1\0\1\u01a0\1\150\1\0\1\u01a0\3\150\1\u0170"+
"\1\0\2\u0170\1\0\26\u0170\3\0\25\u0170\3\0\11\u0170"+
"\1\150\11\u0170\1\0\22\u0170\1\0\2\u0170\1\0\4\u0170"+
"\1\146\1\0\1\147\1\150\1\0\1\151\5\152\1\150"+
"\1\146\2\152\1\153\6\152\1\146\1\160\3\152\3\0"+
"\1\164\7\152\1\153\11\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\u0158\1\152\1\153\3\152\1\150\1\146\6\152\1\153"+
"\2\152\1\146\1\160\3\152\3\0\1\303\1\152\1\153"+
"\11\152\1\153\5\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\303\1\170\1\u0159\1\172\1\173"+
"\1\u0150\4\152\1\u015a\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\164\21\152\1\u01a1\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\2\150\1\0\3\146\1\u01a2\2\146"+
"\1\150\4\146\1\u014f\3\146\1\u014f\2\146\1\150\2\u014f"+
"\1\146\3\0\3\u01a3\1\u01a4\5\u01a3\1\u01a5\3\u01a3\1\u01a5"+
"\1\u01a3\2\u01a5\1\u01a3\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\1\147\1\150\1\0\1\151\3\152\1\153\1\152\1\150"+
"\1\146\11\152\1\146\1\160\2\152\1\153\3\0\1\u01a6"+
"\3\152\1\153\14\152\1\153\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\u01a6\1\170\1\171\1\172"+
"\1\173\1\u0151\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\11\152\1\146\1\160\2\152"+
"\1\153\3\0\1\164\20\152\1\153\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\2\152\2\153\4\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\275\21\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\303\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\307"+
"\21\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\306\3\152\1\153\1\152\1\150\1\146\11\152"+
"\1\146\1\160\2\152\1\153\3\0\1\u01a7\3\276\1\310"+
"\14\276\1\310\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\u01a6\1\170\1\171\1\172\1\173\1\u0151"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\11\152\1\146"+
"\1\160\2\152\1\153\3\0\1\307\20\276\1\310\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\310\1\153\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\2\150\1\0\6\146\1\150"+
"\13\146\1\150\3\146\3\0\22\u01a3\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3"+
"\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3"+
"\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\5\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\303\21\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\303\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\2\150\1\0\1\146\2\u0160\1\146\1\u0160\1\146"+
"\1\150\2\146\1\u01a8\1\u0161\1\146\1\u0162\5\146\1\150"+
"\2\146\1\u0162\3\0\1\146\2\u0160\1\146\1\u0160\2\146"+
"\1\u01a8\1\u0161\1\146\1\u0162\6\146\1\u0162\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\1\146\1\150\1\0"+
"\2\150\1\0\1\150\2\u0166\1\150\1\u0166\4\150\1\u01a9"+
"\1\u016c\1\150\1\u016d\10\150\1\u016d\3\0\1\150\2\u0166"+
"\1\150\1\u0166\2\150\1\u01a9\1\u016c\1\150\1\u016d\6\150"+
"\1\u016d\3\150\3\0\17\150\2\u01aa\2\150\1\0\4\150"+
"\1\266\15\150\1\0\2\150\1\0\4\150\1\146\1\0"+
"\2\150\1\0\3\146\1\u01ab\2\146\1\150\4\146\1\u014f"+
"\3\146\1\u014f\2\146\1\150\2\u014f\1\146\3\0\3\u01a3"+
"\1\u01ac\5\u01a3\1\u01a5\3\u01a3\1\u01a5\1\u01a3\2\u01a5\1\u01a3"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\1\147\1\150\1\0"+
"\1\u01ad\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\275\21\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\303\1\170\1\u0159\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\u015a\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\307\21\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0151\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\3\152"+
"\1\u0128\1\152\1\150\1\146\4\152\1\153\4\152\1\146"+
"\1\160\3\152\3\0\1\307\3\276\1\u012b\5\276\1\310"+
"\7\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\312\1\155\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\306\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\1\163\2\0\1\307\21\276\1\165\1\150\1\301"+
"\2\0\1\163\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0152\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\3\152"+
"\1\155\1\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\1\163\2\0\1\307\3\276\1\312\15\276\1\165\1\150"+
"\1\301\2\0\1\163\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0152\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\u01ae\21\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\u0153\1\170\1\171\1\172\1\173\1\u0151"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\3\152\1\u0154\1\152\1\150\1\146"+
"\6\152\1\u0155\2\152\1\146\1\160\3\152\3\0\1\u01af"+
"\3\276\1\u01b0\7\276\1\u01b1\5\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\u0156\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\3\152\1\u0157"+
"\1\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\307\3\276\1\u01b2\15\276\1\165\1\150\1\301\3\0"+
"\1\166\1\146\1\302\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\306\5\152\1\150\1\146"+
"\10\152\1\153\1\146\1\160\3\152\3\0\1\307\15\276"+
"\1\310\3\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\2\150"+
"\1\0\3\146\1\u01b3\2\146\1\150\4\146\1\u014f\3\146"+
"\1\u014f\2\146\1\150\2\u014f\1\146\3\0\3\u01a3\1\u01b4"+
"\5\u01a3\1\u01a5\3\u01a3\1\u01a5\1\u01a3\2\u01a5\1\u01a3\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3"+
"\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0"+
"\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\u01b5\21\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\u0172\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\1\250\1\152"+
"\1\153\3\152\1\153\2\152\1\146\1\160\3\152\3\0"+
"\1\307\5\276\1\360\1\276\1\310\3\276\1\310\5\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\u01ad\5\152\1\150\1\146\1\152\1\u017e\7\152\1\146"+
"\1\160\3\152\3\0\1\275\6\276\1\u01b6\12\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\303"+
"\1\170\1\u0159\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\u015a\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\1\155\4\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\307\1\312\20\276\1\165\1\150\1\301\3\0"+
"\1\166\1\146\1\302\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\306\3\152\1\u0122\1\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\307"+
"\3\276\1\u0124\15\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\4\152"+
"\1\153\4\152\1\146\1\160\3\152\3\0\1\307\11\276"+
"\1\310\7\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\2\152\1\153\2\152\1\150\1\146"+
"\11\152\1\146\1\160\1\152\1\u017c\1\152\3\0\1\307"+
"\2\276\1\310\14\276\1\u01b7\1\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\11\152\1\146\1\160\1\u017d\2\152\3\0\1\307"+
"\16\276\1\u01b8\2\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\310\1\153"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\11\152"+
"\1\146\1\160\1\152\1\155\1\152\3\0\1\307\17\276"+
"\1\312\1\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\2\150"+
"\1\0\3\146\1\u01ab\2\146\1\150\1\146\1\u017f\1\146"+
"\1\u0180\1\u014f\3\146\1\u014f\2\146\1\150\2\u014f\1\146"+
"\3\0\3\u01a3\1\u01ac\2\u01a3\1\u01b9\1\u01a3\1\u01ba\1\u01a5"+
"\3\u01a3\1\u01a5\1\u01a3\2\u01a5\1\u01a3\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3"+
"\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3"+
"\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\11\152\1\146\1\160\1\u0181\2\152\3\0\1\307"+
"\16\276\1\u01bb\2\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\1\u0182\4\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\307\1\u01bc\20\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\5\152\1\150\1\146\1\152\1\155\7\152\1\146"+
"\1\160\3\152\3\0\1\307\6\276\1\312\12\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\u01bd\21\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\u0183\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\1\u0184\10\152"+
"\1\146\1\160\3\152\3\0\1\u01be\5\276\1\u01bf\13\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\u0185\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\5\152\1\150\1\146\1\152\1\u0186\7\152\1\146"+
"\1\160\3\152\3\0\1\307\6\276\1\u01c0\12\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\307\21\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\310\1\153\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\6\152\1\153"+
"\2\152\1\146\1\160\3\152\3\0\1\307\13\276\1\310"+
"\5\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\306\5\152\1\150\1\146\7\152\1\u0187\1\152"+
"\1\146\1\160\3\152\3\0\1\307\14\276\1\u01c1\4\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\5\152\1\150\1\146\3\152\1\u0188\5\152\1\146"+
"\1\160\3\152\3\0\1\307\10\276\1\u01c2\10\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\11\152\1\146\1\160\1\152\1\u0189"+
"\1\152\3\0\1\307\17\276\1\u01c3\1\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\11\152\1\146\1\160\1\152\1\u018a\1\152"+
"\3\0\1\307\17\276\1\u01c4\1\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\u01af\21\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\u0156\1\170\1\171\1\172\1\173\1\u0150\1\310\1\153"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\1\152\1\153\3\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\1\163\2\0\1\307\1\276\1\310\17\276"+
"\1\165\1\150\1\301\2\0\1\163\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0152\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\306\5\152\1\150\1\146\1\156\10\152\1\146"+
"\1\160\2\152\1\155\3\0\1\307\5\276\1\313\12\276"+
"\1\312\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\2\150\1\0"+
"\3\146\1\u01c5\2\146\1\150\4\146\1\u014f\3\146\1\u014f"+
"\2\146\1\150\2\u014f\1\146\3\0\3\u01a3\1\u01c6\5\u01a3"+
"\1\u01a5\3\u01a3\1\u01a5\1\u01a3\2\u01a5\1\u01a3\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\1\220\1\152\1\u018d\6\152\1\146\1\160"+
"\3\152\3\0\1\u01c7\5\276\1\335\1\276\1\u01c8\11\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\u018e\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\3\152\1\153\1\152\1\150\1\146\2\152\1\u018f"+
"\1\152\1\u0190\4\152\1\146\1\160\3\152\3\0\1\u01c9"+
"\3\276\1\310\3\276\1\u01ca\1\276\1\u01cb\7\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\u0191"+
"\1\170\1\171\1\172\1\173\1\u0151\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\2\150\1\0\3\146\1\u01cc"+
"\2\146\1\150\4\146\1\u014f\3\146\1\u014f\2\146\1\150"+
"\2\u014f\1\146\3\0\3\u01a3\1\u01cd\5\u01a3\1\u01a5\3\u01a3"+
"\1\u01a5\1\u01a3\2\u01a5\1\u01a3\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146"+
"\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146"+
"\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\1\147\1\150\1\0\1\u01ce\5\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\307\21\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\u0197\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\u0118\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\11\152\1\146\1\160\1\152\1\153"+
"\1\152\3\0\1\307\17\276\1\310\1\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\1\152\1\153\7\152\1\146\1\160\3\152"+
"\3\0\1\307\6\276\1\310\12\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\2\150\1\0\1\146\2\u0173\1\u0174\1\u0173"+
"\1\u0174\1\150\1\146\2\u0174\1\u0175\1\u0174\1\u0176\1\u0174"+
"\1\u01cf\2\u0174\1\146\1\u0177\2\u0174\1\u0176\3\0\1\u0174"+
"\2\u0173\1\u0174\1\u0173\3\u0174\1\u0175\1\u0174\1\u0176\1\u0174"+
"\1\u01cf\4\u0174\1\u0176\2\150\1\146\3\0\2\146\1\150"+
"\1\146\1\u0174\3\146\1\150\1\u014c\4\u0174\1\150\2\u0174"+
"\2\u0177\1\0\1\150\2\u0174\1\150\1\176\10\u0174\5\146"+
"\1\0\1\u0177\1\146\1\0\1\u0177\2\150\1\146\1\150"+
"\1\0\2\150\1\0\1\150\2\u0178\1\u0177\1\u0178\1\u0177"+
"\2\150\2\u0177\1\u017a\1\u0177\1\u017b\1\u0177\1\u01d0\2\u0177"+
"\1\150\3\u0177\1\u017b\3\0\1\u0177\2\u0178\1\u0177\1\u0178"+
"\3\u0177\1\u017a\1\u0177\1\u017b\1\u0177\1\u01d0\4\u0177\1\u017b"+
"\3\150\3\0\4\150\1\u0177\5\150\4\u0177\1\150\4\u0177"+
"\1\0\1\150\2\u0177\1\150\1\266\10\u0177\5\150\1\0"+
"\1\u0177\1\150\1\0\1\u0177\3\150\1\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\2\152\1\u0131"+
"\6\152\1\146\1\160\3\152\3\0\1\307\7\276\1\u0134"+
"\11\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\306\5\152\1\150\1\146\11\152\1\146\1\160"+
"\2\152\1\156\3\0\1\307\20\276\1\313\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\u01d1"+
"\21\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\u019a\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\u01ad\1\152\1\153\3\152\1\150\1\146\6\152"+
"\1\153\2\152\1\146\1\160\3\152\3\0\1\275\1\276"+
"\1\310\11\276\1\310\5\276\1\165\1\150\1\301\3\0"+
"\1\166\1\146\1\302\1\167\1\303\1\170\1\u0159\1\172"+
"\1\173\1\u0150\1\276\1\152\1\276\1\152\1\u015a\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\306\5\152\1\150\1\146"+
"\2\152\1\153\6\152\1\146\1\160\3\152\3\0\1\307"+
"\7\276\1\310\11\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\307\21\276\1\u01a1\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\1\146\11\0\1\u01d2\7\0\1\u01d2\1\0\1\u01d2"+
"\16\0\1\u01d2\5\0\1\u01d2\1\0\1\u01d2\120\0\1\372"+
"\31\0\1\374\14\0\2\374\7\0\2\372\24\0\1\372"+
"\2\0\1\372\63\0\1\373\63\0\1\126\4\0\6\126"+
"\1\0\7\126\1\u013d\1\u013e\2\126\1\372\1\126\1\u013f"+
"\1\126\3\0\1\u0140\13\126\1\u013d\1\u013e\2\126\1\u013f"+
"\1\126\2\0\1\126\3\0\2\126\1\0\1\126\1\u0140"+
"\3\126\2\0\4\126\1\0\2\126\2\372\2\0\2\126"+
"\1\0\16\126\1\0\1\372\1\126\1\0\1\372\2\0"+
"\1\126\27\0\1\u01d3\31\0\1\377\2\0\1\5\11\0"+
"\2\377\7\0\2\u01d3\24\0\1\u01d3\2\0\1\u01d3\32\0"+
"\1\u01d4\57\0\2\u01d4\24\0\1\u01d4\2\0\1\u01d4\3\0"+
"\1\126\4\0\6\126\1\0\13\126\1\0\3\126\3\0"+
"\22\126\2\0\1\126\3\0\1\u01d5\1\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\3\126\1\u01d6"+
"\2\126\1\0\4\126\1\144\3\126\1\144\2\126\1\0"+
"\2\144\1\126\3\0\3\126\1\u01d6\5\126\1\144\3\126"+
"\1\144\1\126\2\144\1\126\2\0\1\126\3\0\2\126"+
"\1\0\5\126\1\0\1\127\4\126\1\0\2\126\4\0"+
"\2\126\1\0\16\126\2\0\1\126\4\0\2\126\4\0"+
"\1\126\2\u01d7\1\126\1\u01d7\1\126\1\0\3\126\1\u01d8"+
"\1\126\1\u01d9\5\126\1\0\2\126\1\u01d9\3\0\1\126"+
"\2\u01d7\1\126\1\u01d7\3\126\1\u01d8\1\126\1\u01d9\6\126"+
"\1\u01d9\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\6\126\1\u01da\7\126"+
"\1\u013d\1\u013e\2\126\1\0\1\126\1\u013f\1\126\3\0"+
"\1\u0140\13\126\1\u013d\1\u013e\2\126\1\u013f\1\126\1\0"+
"\1\u01da\1\126\3\0\2\126\1\0\1\126\1\u0140\3\126"+
"\1\u01da\1\0\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\1\126\6\0\2\u01da\1\0"+
"\1\u01da\5\0\1\u01db\1\0\1\u01dc\10\0\1\u01dc\4\0"+
"\2\u01da\1\0\1\u01da\3\0\1\u01db\1\0\1\u01dc\6\0"+
"\1\u01dc\64\0\1\146\1\0\2\150\1\0\3\146\1\u01dd"+
"\2\146\1\150\4\146\1\u014f\3\146\1\u014f\2\146\1\150"+
"\2\u014f\1\146\3\0\3\146\1\u01dd\5\146\1\u014f\3\146"+
"\1\u014f\1\146\2\u014f\1\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\1\146\1\u01de\4\u01df\1\u01de\5\126"+
"\1\u01df\1\u01de\11\126\1\u01de\1\u01df\3\126\3\u01df\22\126"+
"\2\u01df\1\u01de\3\u01df\2\u01de\1\u01df\1\u01de\1\126\3\u01de"+
"\1\u01df\1\u01e0\4\126\1\u01df\2\126\4\u01df\2\126\1\u01df"+
"\1\u01de\10\126\5\u01de\2\u01df\1\u01de\4\u01df\1\u01de\1\126"+
"\4\0\6\126\1\0\13\126\1\0\1\u01e1\2\126\3\0"+
"\17\126\1\u01e1\2\126\2\0\1\126\3\0\2\126\1\0"+
"\5\126\1\0\1\127\4\126\1\0\2\126\4\0\2\126"+
"\1\0\16\126\2\0\1\126\4\0\2\126\4\0\6\126"+
"\1\0\3\126\1\u0106\7\126\1\0\3\126\3\0\10\126"+
"\1\u0106\11\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\6\126\1\0"+
"\13\126\1\0\1\126\1\u0106\1\126\3\0\20\126\1\u0106"+
"\1\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\3\126\1\u01e2\2\126"+
"\1\0\13\126\1\0\3\126\3\0\3\126\1\u01e2\16\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\1\126\27\0\1\u01e3\57\0\2\u01e3\24\0"+
"\1\u01e3\2\0\1\u01e3\3\0\1\126\4\0\1\126\1\73"+
"\4\126\1\0\13\126\1\0\3\126\3\0\1\126\1\73"+
"\20\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\1\u01e4\5\126\1\0"+
"\13\126\1\0\3\126\3\0\22\126\2\0\1\126\3\0"+
"\2\126\1\0\3\126\1\73\1\126\1\0\1\127\4\126"+
"\1\5\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\1\126\27\0\1\u01e3\57\0\1\u01e3\1\u01e5\24\0"+
"\1\u01e3\2\0\1\u01e3\102\0\1\u01e6\143\0\1\u01e7\44\0"+
"\1\146\1\0\1\147\1\150\1\0\1\151\5\152\1\150"+
"\1\146\1\u01e8\10\152\1\146\1\160\3\152\3\0\1\u0153"+
"\5\152\1\u01e8\13\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\u0153\1\170\1\171\1\172\1\173"+
"\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\2\152\1\153\6\152\1\146\1\160"+
"\3\152\3\0\1\u0153\7\152\1\153\11\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\u0153\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\3\152\1\u01e9\1\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\164\3\152\1\u01e9"+
"\15\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\1\u01e8\10\152\1\146\1\160\3\152\3\0\1\u01ae"+
"\5\276\1\u01ea\13\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\u0153\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\2\152"+
"\1\153\6\152\1\146\1\160\3\152\3\0\1\u01ae\7\276"+
"\1\310\11\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\u0153\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\3\152\1\u01e9\1\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\307\3\276\1\u01eb"+
"\15\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\1\146\6\u01df\5\u01ec\2\u01df"+
"\11\u01ec\2\u01df\3\u01ec\3\u01df\22\u01ec\12\u01df\1\u01ec\5\u01df"+
"\4\u01ec\1\u01df\2\u01ec\4\u01df\2\u01ec\2\u01df\10\u01ec\15\u01df"+
"\35\u01ec\1\0\136\u01ec\1\u01ed\4\u01ec\1\0\17\u01ec\1\u01ed"+
"\35\u01ec\2\u01ee\46\u01ec\1\u01ef\15\u01ec\1\0\10\u01ec\1\u01ef"+
"\126\u01ec\1\u01ef\3\u01ec\1\0\20\u01ec\1\u01ef\75\u01ec\1\u01f0"+
"\24\u01ec\1\0\3\u01ec\1\u01f0\110\u01ec\1\5\26\u01ec\1\0"+
"\1\u01ec\1\5\111\u01ec\1\u01f1\27\u01ec\1\0\36\u01ec\1\5"+
"\7\u01ec\1\5\37\u01ec\1\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\u0153\21\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\u0153\1\170\1\171\1\172\1\173"+
"\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\4\152\1\u01f2\4\152\1\146\1\160"+
"\3\152\3\0\1\164\11\152\1\u01f2\7\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\u01ae\21\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\u0153\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\4\152\1\u01f2\4\152\1\146\1\160\3\152\3\0"+
"\1\307\11\276\1\u01f3\7\276\1\165\1\150\1\301\3\0"+
"\1\166\1\146\1\302\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\1\146"+
"\1\150\1\0\1\147\1\150\1\0\1\150\5\160\2\150"+
"\11\160\1\150\4\160\3\0\22\160\1\165\2\150\3\0"+
"\1\263\3\150\1\160\1\264\1\150\3\173\4\160\1\150"+
"\4\160\1\0\1\150\2\160\1\150\1\266\10\160\5\150"+
"\1\0\1\160\1\150\1\0\1\u01f4\3\150\1\146\1\0"+
"\1\147\1\150\1\0\1\151\3\152\1\u0155\1\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\164\3\152"+
"\1\u0155\15\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\151\3\152"+
"\1\153\1\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\164\3\152\1\153\15\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\1\152\1\153\3\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\164\1\152\1\153\17\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\306\3\152\1\u0155\1\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\307"+
"\3\276\1\u01b1\15\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\3\152\1\153\1\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\307\3\276"+
"\1\310\15\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\1\152\1\153\3\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\307\1\276\1\310"+
"\17\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\306\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\3\0\1\307\21\276\1\165\1\150\1\301\3\0"+
"\1\166\1\146\1\302\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276"+
"\1\152\2\160\1\0\1\150\1\u01f5\1\u01f6\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\164\21\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152"+
"\2\160\1\0\1\150\2\u01f6\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\7\152"+
"\1\153\1\152\1\146\1\160\3\152\3\0\1\307\14\276"+
"\1\310\4\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\7\152\1\153"+
"\1\152\1\146\1\160\3\152\3\0\1\164\14\152\1\153"+
"\4\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\2\152\1\153"+
"\2\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\164\2\152\1\153\16\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\11\152\1\146\1\160\1\153"+
"\2\152\3\0\1\164\16\152\1\153\2\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\164\21\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\2\152\2\153\4\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\2\152\1\153\2\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\307\2\276"+
"\1\310\16\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\11\152\1\146"+
"\1\160\1\153\2\152\3\0\1\307\16\276\1\310\2\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\307\21\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\310\1\153\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\151\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\u01f7\21\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\u01f7\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\7\152\1\u0128"+
"\1\152\1\146\1\160\3\152\3\0\1\164\14\152\1\u0128"+
"\4\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\u01f8\21\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\u01f7\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\5\152\1\150\1\146\7\152\1\u0128\1\152\1\146"+
"\1\160\3\152\3\0\1\307\14\276\1\u012b\4\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\1\146\1\150\1\0\2\150\1\0\1\150"+
"\2\u0166\1\150\1\u0166\5\150\1\u016c\1\150\1\u016d\3\150"+
"\1\u01f9\4\150\1\u016d\3\0\1\150\2\u0166\1\150\1\u0166"+
"\3\150\1\u016c\1\150\1\u016d\3\150\1\u01f9\2\150\1\u016d"+
"\3\150\3\0\23\150\1\0\4\150\1\266\15\150\1\0"+
"\2\150\1\0\4\150\1\126\4\0\6\126\1\0\10\126"+
"\1\u01fa\2\126\1\0\3\126\3\0\15\126\1\u01fa\4\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\2\126\4\0\6\126\1\0\5\126\1\u01fb"+
"\5\126\1\0\3\126\3\0\12\126\1\u01fb\7\126\2\0"+
"\1\126\3\0\2\126\1\0\5\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\2\126\4\0\2\126\1\u01fc\3\126\1\0\13\126"+
"\1\0\3\126\3\0\2\126\1\u01fc\17\126\2\0\1\126"+
"\3\0\2\126\1\0\5\126\1\0\1\127\4\126\1\0"+
"\2\126\4\0\2\126\1\0\16\126\2\0\1\126\4\0"+
"\2\126\4\0\6\126\1\0\13\126\1\0\1\126\1\u01fd"+
"\1\126\3\0\20\126\1\u01fd\1\126\2\0\1\126\3\0"+
"\2\126\1\0\5\126\1\0\1\127\4\126\1\0\2\126"+
"\4\0\2\126\1\0\16\126\2\0\1\126\4\0\2\126"+
"\4\0\3\126\1\u01fe\2\126\1\u01ff\13\126\1\0\3\126"+
"\3\0\3\126\1\u01fe\16\126\2\0\1\126\3\0\2\126"+
"\1\0\5\126\1\0\1\127\4\126\1\0\2\126\4\0"+
"\2\126\1\0\16\126\2\0\1\126\4\0\2\126\4\0"+
"\6\126\1\0\1\126\1\u0200\11\126\1\0\3\126\3\0"+
"\6\126\1\u0200\13\126\2\0\1\126\3\0\2\126\1\0"+
"\5\126\1\0\1\127\4\126\1\0\2\126\4\0\2\126"+
"\1\0\16\126\2\0\1\126\4\0\2\126\4\0\6\126"+
"\1\0\7\126\1\u0201\3\126\1\0\3\126\3\0\14\126"+
"\1\u0201\5\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\6\126\1\0"+
"\10\126\1\u0202\2\126\1\0\3\126\3\0\15\126\1\u0202"+
"\4\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\1\126\1\u0203\4\126"+
"\1\0\13\126\1\0\3\126\3\0\1\126\1\u0203\20\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\2\126\4\0\6\126\1\0\5\126\1\u0204"+
"\5\126\1\0\3\126\3\0\12\126\1\u0204\7\126\2\0"+
"\1\126\3\0\2\126\1\0\5\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\2\126\4\0\6\126\1\5\13\126\1\0\3\126"+
"\3\0\22\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\6\126\1\0"+
"\10\126\1\u0205\2\126\1\0\3\126\3\0\15\126\1\u0205"+
"\4\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\6\126\1\0\6\126"+
"\1\u0147\4\126\1\0\3\126\3\0\13\126\1\u0147\6\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\1\126\13\0\1\5\13\0\1\u014a\57\0"+
"\2\u014a\24\0\1\u014a\2\0\1\u014a\3\0\1\126\4\0"+
"\4\126\1\u0206\1\126\1\0\13\126\1\0\3\126\3\0"+
"\4\126\1\u0206\15\126\2\0\1\126\3\0\2\126\1\0"+
"\5\126\1\0\1\127\4\126\1\0\2\126\4\0\2\126"+
"\1\0\16\126\2\0\1\126\4\0\1\126\1\146\1\0"+
"\2\150\1\0\6\146\1\150\7\146\1\u0207\1\u0208\2\146"+
"\1\150\1\146\1\u0209\1\146\3\0\1\u020a\13\146\1\u0207"+
"\1\u0208\2\146\1\u0209\1\146\2\150\1\146\3\0\2\146"+
"\1\150\1\146\1\u020a\3\146\2\150\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\1\146\1\150\1\0\1\u020b"+
"\1\150\1\0\1\150\5\u014d\2\150\11\u014d\1\150\4\u014d"+
"\3\0\22\u014d\1\u020c\2\150\3\0\1\u020d\3\150\1\u014d"+
"\5\150\4\u014d\1\150\4\u014d\1\0\1\150\2\u014d\1\150"+
"\1\266\10\u014d\5\150\1\0\1\u014d\1\150\1\0\1\u014d"+
"\3\150\1\146\1\0\2\150\1\0\3\146\1\u020e\2\146"+
"\1\150\1\146\1\u020f\2\146\1\u0210\4\146\1\u0211\1\146"+
"\1\150\3\146\3\0\3\146\1\u020e\2\146\1\u020f\2\146"+
"\1\u0210\4\146\1\u0211\3\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\3\146"+
"\1\u020e\2\146\1\150\4\146\1\u0210\4\146\1\u0211\1\146"+
"\1\150\3\146\3\0\3\146\1\u020e\5\146\1\u0210\4\146"+
"\1\u0211\3\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\1\146\5\172\1\u0166"+
"\1\146\6\172\1\u0212\1\u0213\1\172\1\146\1\173\1\172"+
"\1\u0214\1\172\3\0\1\u0215\13\172\1\u0212\1\u0213\2\172"+
"\1\u0214\1\172\1\u0163\1\u0166\1\146\3\0\2\146\1\150"+
"\1\146\1\u0215\2\146\1\172\1\u016b\1\173\4\172\1\150"+
"\2\172\2\173\1\0\1\150\2\172\1\150\1\176\10\172"+
"\5\146\1\0\1\173\1\146\1\0\1\173\2\150\2\146"+
"\1\0\2\150\1\0\1\146\5\172\1\u0166\1\146\6\172"+
"\1\u0212\1\u0213\1\172\1\146\1\173\1\172\1\u0214\1\172"+
"\1\u0216\1\0\1\u0216\1\u0215\13\172\1\u0212\1\u0213\2\172"+
"\1\u0214\1\172\1\u0163\1\u0166\1\146\2\0\1\u0216\2\146"+
"\1\150\1\146\1\u0215\2\146\1\172\1\u016b\1\173\4\172"+
"\1\150\2\172\2\173\1\0\1\150\2\172\1\150\1\176"+
"\10\172\5\146\1\0\1\173\1\146\1\0\1\173\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\u0158\5\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\303\21\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\303\1\170\1\u0159\1\172\1\173\1\u0151\4\152\1\u015a"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\3\152\1\u0217\1\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\164"+
"\3\152\1\u0217\15\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\4\152\1\153\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\164\4\152\1\153\14\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\u0158\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\3\0\1\u0218\21\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\u0218\1\170\1\u0159\1\172"+
"\1\173\1\u0150\4\152\1\u015a\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\3\152\1\u0219\1\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\164\3\152\1\u0219\15\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\2\150\1\0\3\146\1\u021a\2\146\1\150\4\146\1\u014f"+
"\3\146\1\u014f\2\146\1\150\2\u014f\1\146\3\0\3\146"+
"\1\u021a\5\146\1\u014f\3\146\1\u014f\1\146\2\u014f\1\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\2\150\1\0\1\146\2\u0160\1\146\1\u0160\1\146"+
"\1\150\2\146\1\u021b\1\u0161\1\146\1\u0162\5\146\1\150"+
"\2\146\1\u0162\3\0\1\146\2\u0160\1\146\1\u0160\2\146"+
"\1\u021b\1\u0161\1\146\1\u0162\6\146\1\u0162\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\1\146\1\150\1\0"+
"\2\150\1\0\1\150\2\u0166\1\150\1\u0166\4\150\1\u021c"+
"\1\u016c\1\150\1\u016d\10\150\1\u016d\3\0\1\150\2\u0166"+
"\1\150\1\u0166\2\150\1\u021c\1\u016c\1\150\1\u016d\6\150"+
"\1\u016d\3\150\3\0\23\150\1\0\4\150\1\266\15\150"+
"\1\0\2\150\1\0\4\150\1\u0166\1\u01da\1\u021d\1\u0166"+
"\1\u01da\1\u0166\5\u021e\2\u0166\11\u021e\1\u0166\1\u015c\3\u021e"+
"\3\u01da\22\u021e\1\u021f\1\u0166\1\150\3\u01da\1\u0220\3\u0166"+
"\1\u021e\1\u0221\3\u0166\1\u0222\4\u021e\1\u0166\2\u021e\2\u015c"+
"\1\u01da\1\u0166\2\u021e\1\u0166\1\u0223\10\u021e\5\u0166\1\u01da"+
"\1\u015c\1\u0166\1\u01da\1\u015c\4\u0166\1\u01da\2\u0166\1\u01da"+
"\1\u0166\5\u015c\2\u0166\11\u015c\1\u0166\4\u015c\3\u01da\22\u015c"+
"\1\u0224\1\u0166\1\150\3\u01da\4\u0166\1\u015c\1\u0221\3\u0166"+
"\1\150\4\u015c\1\u0166\4\u015c\1\u01da\1\u0166\2\u015c\1\u0166"+
"\1\u0223\10\u015c\5\u0166\1\u01da\1\u015c\1\u0166\1\u01da\1\u015c"+
"\3\u0166\1\146\1\0\2\150\1\0\6\146\1\u0166\7\146"+
"\1\u0207\1\u0208\2\146\1\150\1\146\1\u0209\1\146\3\0"+
"\1\u020a\13\146\1\u0207\1\u0208\2\146\1\u0209\1\146\1\150"+
"\1\u0166\1\146\3\0\2\146\1\150\1\146\1\u020a\3\146"+
"\1\u0166\1\150\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\1\146\5\u015e\1\150"+
"\1\146\11\u015e\1\146\1\u015f\3\u015e\3\0\22\u015e\1\264"+
"\1\150\1\146\3\0\2\146\1\150\1\146\1\u015e\1\170"+
"\2\146\1\150\1\u014c\4\u015e\1\150\2\u015e\2\u015f\1\0"+
"\1\150\2\u015e\1\150\1\176\10\u015e\5\146\1\0\1\u015f"+
"\1\146\1\0\1\u015f\2\150\1\146\1\150\1\0\2\150"+
"\1\0\1\150\5\u015f\2\150\11\u015f\1\150\4\u015f\3\0"+
"\22\u015f\1\264\2\150\3\0\4\150\1\u015f\1\264\4\150"+
"\4\u015f\1\150\4\u015f\1\0\1\150\2\u015f\1\150\1\266"+
"\10\u015f\5\150\1\0\1\u015f\1\150\1\0\1\u015f\3\150"+
"\1\146\1\0\2\150\1\0\6\146\1\150\3\146\1\u0160"+
"\7\146\1\150\3\146\3\0\10\146\1\u0160\11\146\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146"+
"\1\150\2\146\2\150\1\0\1\150\2\146\1\150\1\176"+
"\15\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\6\146\1\150\13\146\1\150\1\146\1\u0160"+
"\1\146\3\0\20\146\1\u0160\1\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\1\146\1\150\1\0\2\150"+
"\1\0\1\150\5\u0225\2\150\11\u0225\1\150\1\u0226\3\u0225"+
"\3\0\22\u0225\3\150\3\0\4\150\1\u0225\5\150\4\u0225"+
"\1\150\2\u0225\2\u0226\1\0\1\150\2\u0225\1\150\1\266"+
"\10\u0225\5\150\1\0\1\u0226\1\150\1\0\1\u0226\3\150"+
"\1\146\1\0\2\150\1\0\1\146\5\172\1\150\1\146"+
"\6\172\1\u0212\1\u0213\1\172\1\146\1\173\1\172\1\u0214"+
"\1\172\3\0\1\u0215\13\172\1\u0212\1\u0213\2\172\1\u0214"+
"\1\172\1\u0163\1\150\1\146\3\0\2\146\1\150\1\146"+
"\1\u0215\2\146\1\172\2\173\4\172\1\150\2\172\2\173"+
"\1\0\1\150\2\172\1\150\1\176\10\172\5\146\1\0"+
"\1\173\1\146\1\0\1\173\2\150\2\146\1\0\2\150"+
"\1\0\1\146\5\172\1\150\1\146\11\172\1\146\1\173"+
"\3\172\3\u0227\22\172\1\u0163\1\150\1\146\2\0\1\u0227"+
"\2\146\1\150\1\146\1\172\2\146\1\172\1\173\1\u0228"+
"\4\172\1\150\2\172\2\173\1\0\1\150\2\172\1\150"+
"\1\176\10\172\5\146\1\0\1\173\1\146\1\0\1\173"+
"\2\150\2\146\1\0\2\150\1\0\1\146\5\172\1\150"+
"\1\146\7\172\1\u0229\1\172\1\146\1\173\3\172\3\u0227"+
"\15\172\1\u0229\4\172\1\u0163\1\150\1\146\2\0\1\u0227"+
"\2\146\1\150\1\146\1\172\2\146\1\172\1\173\1\u0228"+
"\4\172\1\150\2\172\2\173\1\0\1\150\2\172\1\150"+
"\1\176\10\172\5\146\1\0\1\173\1\146\1\0\1\173"+
"\2\150\2\146\1\0\2\150\1\0\1\146\5\172\1\150"+
"\1\146\4\172\1\u022a\4\172\1\146\1\173\3\172\3\u0227"+
"\12\172\1\u022a\7\172\1\u0163\1\150\1\146\2\0\1\u0227"+
"\2\146\1\150\1\146\1\172\2\146\1\172\1\173\1\u0228"+
"\4\172\1\150\2\172\2\173\1\0\1\150\2\172\1\150"+
"\1\176\10\172\5\146\1\0\1\173\1\146\1\0\1\173"+
"\2\150\2\146\1\0\2\150\1\0\1\146\1\172\1\u022b"+
"\3\172\1\150\1\146\11\172\1\146\1\173\3\172\3\u0227"+
"\2\172\1\u022b\17\172\1\u0163\1\150\1\146\2\0\1\u0227"+
"\2\146\1\150\1\146\1\172\2\146\1\172\1\173\1\u0228"+
"\4\172\1\150\2\172\2\173\1\0\1\150\2\172\1\150"+
"\1\176\10\172\5\146\1\0\1\173\1\146\1\0\1\173"+
"\2\150\2\146\1\0\2\150\1\0\1\146\5\172\1\150"+
"\1\146\11\172\1\146\1\173\1\172\1\u022c\1\172\3\u0227"+
"\20\172\1\u022c\1\172\1\u0163\1\150\1\146\2\0\1\u0227"+
"\2\146\1\150\1\146\1\172\2\146\1\172\1\173\1\u0228"+
"\4\172\1\150\2\172\2\173\1\0\1\150\2\172\1\150"+
"\1\176\10\172\5\146\1\0\1\173\1\146\1\0\1\173"+
"\2\150\1\146\1\150\1\0\2\150\1\0\12\150\1\u0166"+
"\13\150\3\0\10\150\1\u0166\14\150\3\0\23\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\5\150\1\0"+
"\2\150\1\0\24\150\1\u0166\1\150\3\0\20\150\1\u0166"+
"\4\150\3\0\23\150\1\0\4\150\1\266\15\150\1\0"+
"\2\150\1\0\5\150\1\0\2\150\1\0\17\150\1\u022d"+
"\6\150\3\0\15\150\1\u022d\7\150\3\0\23\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\4\150\1\u016f"+
"\1\0\2\u0170\1\0\6\u016f\1\u0170\13\u016f\1\u0170\3\u016f"+
"\3\0\22\u016f\2\u0170\1\u016f\3\0\2\u016f\1\u0170\5\u016f"+
"\1\u0170\1\u022e\4\u016f\1\u0170\2\u016f\2\u0170\1\0\1\u0170"+
"\2\u016f\1\u0170\16\u016f\1\0\1\u0170\1\u016f\1\0\3\u0170"+
"\1\u016f\1\u0170\1\0\2\u0170\1\0\26\u0170\3\0\25\u0170"+
"\3\0\11\u0170\1\u022f\11\u0170\1\0\22\u0170\1\0\2\u0170"+
"\1\0\4\u0170\1\146\1\0\2\150\1\0\3\146\1\u020e"+
"\2\146\1\150\1\146\1\u0230\2\146\1\u0210\4\146\1\u0211"+
"\1\146\1\150\3\146\3\0\3\146\1\u020e\2\146\1\u0230"+
"\2\146\1\u0210\4\146\1\u0211\3\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\1\147\1\150"+
"\1\0\1\u0158\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\3\0\1\303\21\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\303\1\170\1\u0159\1\172"+
"\1\173\1\u0150\4\152\1\u015a\2\u0231\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\1\146\1\u0160\1\u01da\2\u0166\1\u01da"+
"\1\u0160\5\u0174\1\u0166\1\u0160\11\u0174\1\u0160\1\u0177\3\u0174"+
"\3\u01da\22\u0174\1\u0232\1\u0166\1\146\3\u01da\2\u0160\1\u0166"+
"\1\u0160\1\u0174\1\u0233\2\u0160\1\u0166\1\u014c\4\u0174\1\u0166"+
"\2\u0174\2\u0177\1\u01da\1\u0166\2\u0174\1\u0166\1\u0234\10\u0174"+
"\5\u0160\1\u01da\1\u0177\1\u0160\1\u01da\1\u0177\2\u0166\2\u0160"+
"\1\u01da\2\u0166\1\u01da\1\u0160\5\u0174\1\u0166\1\u0160\2\u0174"+
"\1\u0173\6\u0174\1\u0160\1\u0177\3\u0174\3\u01da\10\u0174\1\u0173"+
"\11\u0174\1\u0232\1\u0166\1\146\3\u01da\2\u0160\1\u0166\1\u0160"+
"\1\u0174\1\u0233\2\u0160\1\u0166\1\u014c\4\u0174\1\u0166\2\u0174"+
"\2\u0177\1\u01da\1\u0166\2\u0174\1\u0166\1\u0234\10\u0174\5\u0160"+
"\1\u01da\1\u0177\1\u0160\1\u01da\1\u0177\2\u0166\2\u0160\1\u01da"+
"\2\u0166\1\u01da\1\u0160\5\u0174\1\u0166\1\u0160\11\u0174\1\u0160"+
"\1\u0177\1\u0174\1\u0173\1\u0174\3\u01da\20\u0174\1\u0173\1\u0174"+
"\1\u0232\1\u0166\1\146\3\u01da\2\u0160\1\u0166\1\u0160\1\u0174"+
"\1\u0233\2\u0160\1\u0166\1\u014c\4\u0174\1\u0166\2\u0174\2\u0177"+
"\1\u01da\1\u0166\2\u0174\1\u0166\1\u0234\10\u0174\5\u0160\1\u01da"+
"\1\u0177\1\u0160\1\u01da\1\u0177\2\u0166\1\u0160\1\u0166\1\u01da"+
"\2\u0166\1\u01da\1\u0166\5\u0177\2\u0166\11\u0177\1\u0166\4\u0177"+
"\3\u01da\22\u0177\1\u0232\1\u0166\1\150\3\u01da\4\u0166\1\u0177"+
"\1\u0232\3\u0166\1\150\4\u0177\1\u0166\4\u0177\1\u01da\1\u0166"+
"\2\u0177\1\u0166\1\u0223\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166"+
"\1\u01da\1\u0177\4\u0166\1\u01da\2\u0166\1\u01da\1\u0166\1\u0235"+
"\4\u0177\2\u0166\11\u0177\1\u0166\4\u0177\3\u01da\1\u0177\1\u0235"+
"\20\u0177\1\u0232\1\u0166\1\150\3\u01da\4\u0166\1\u0177\1\u0232"+
"\3\u0166\1\150\4\u0177\1\u0166\4\u0177\1\u01da\1\u0166\2\u0177"+
"\1\u0166\1\u0223\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166\1\u01da"+
"\1\u0177\4\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u0177\2\u0166"+
"\2\u0177\1\u0178\6\u0177\1\u0166\4\u0177\3\u01da\10\u0177\1\u0178"+
"\11\u0177\1\u0232\1\u0166\1\150\3\u01da\4\u0166\1\u0177\1\u0232"+
"\3\u0166\1\150\4\u0177\1\u0166\4\u0177\1\u01da\1\u0166\2\u0177"+
"\1\u0166\1\u0223\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166\1\u01da"+
"\1\u0177\4\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u0177\2\u0166"+
"\11\u0177\1\u0166\2\u0177\1\u0178\1\u0177\3\u01da\20\u0177\1\u0178"+
"\1\u0177\1\u0232\1\u0166\1\150\3\u01da\4\u0166\1\u0177\1\u0232"+
"\3\u0166\1\150\4\u0177\1\u0166\4\u0177\1\u01da\1\u0166\2\u0177"+
"\1\u0166\1\u0223\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166\1\u01da"+
"\1\u0177\3\u0166\1\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\164\21\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\2\155\2\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\164\21\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\4\152\2\153\2\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\11\152\1\146\1\160\1\u0236"+
"\2\152\3\0\1\164\16\152\1\u0236\2\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\2\150"+
"\1\0\6\146\1\150\13\146\1\150\3\146\3\0\22\146"+
"\1\u0237\1\150\1\146\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150\2\146"+
"\1\150\1\176\15\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\2\150\1\0\4\146\1\u0238\1\146\1\150"+
"\13\146\1\150\3\146\3\0\4\146\1\u0238\15\146\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146"+
"\1\150\2\146\2\150\1\0\1\150\2\146\1\150\1\176"+
"\15\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\1\147\1\150\1\0\1\u0239\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\164\21\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\u023a\1\172\1\173\1\u0150\4\152\1\u023b\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\11\152\1\146"+
"\1\160\1\152\1\u023c\1\152\3\0\1\164\17\152\1\u023c"+
"\1\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\u0158\5\152\1\150"+
"\1\146\7\152\1\271\1\152\1\146\1\160\3\152\3\0"+
"\1\303\14\152\1\271\4\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\303\1\170\1\u0159\1\172"+
"\1\173\1\u0150\4\152\1\u015a\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\1\152\1\153\7\152\1\146"+
"\1\160\3\152\3\0\1\164\6\152\1\153\12\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0151\4\152\1\214\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\u0158\3\152\1\155\1\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\1\163\2\0\1\303"+
"\3\152\1\155\15\152\1\165\1\150\1\146\2\0\1\163"+
"\1\166\1\146\1\150\1\167\1\303\1\170\1\u0159\1\172"+
"\1\173\1\u0152\4\152\1\u015a\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\1\u023d\10\152\1\146\1\160"+
"\3\152\3\0\1\164\5\152\1\u023d\13\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\1\153\10\152"+
"\1\146\1\160\3\152\3\0\1\164\5\152\1\153\13\152"+
"\1\u023e\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\2\156"+
"\6\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\5\152\1\150"+
"\1\146\4\152\1\u0128\4\152\1\146\1\160\3\152\3\0"+
"\1\164\11\152\1\u0128\7\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0151\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\3\152\1\153\1\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\164\3\152\1\153\15\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0151\4\152\1\214\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\151\2\152\1\u023f\2\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\164\2\152"+
"\1\u023f\16\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\2\150\1\0\1\146\5\172\1\150"+
"\1\146\11\172\1\146\1\173\3\172\3\u0227\22\172\1\u0163"+
"\1\150\1\146\2\0\1\u0227\2\146\1\150\1\146\1\172"+
"\2\146\1\172\1\173\1\u0240\4\172\1\150\2\172\2\173"+
"\1\0\1\150\2\172\1\150\1\176\10\172\5\146\1\0"+
"\1\173\1\146\1\0\1\173\2\150\2\146\1\0\2\150"+
"\1\0\3\146\1\u020e\2\146\1\150\1\146\1\u0241\2\146"+
"\1\u0210\4\146\1\u0211\1\146\1\150\3\146\3\0\3\146"+
"\1\u020e\2\146\1\u0241\2\146\1\u0210\4\146\1\u0211\3\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\11\152\1\146\1\160\1\u0242\2\152\3\0\1\164\16\152"+
"\1\u0242\2\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\u0158\2\152"+
"\1\u0243\2\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\u0244\2\152\1\u0243\16\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\u0244\1\170\1\u0159"+
"\1\172\1\173\1\u0150\4\152\1\u015a\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\7\152\1\153\1\152"+
"\1\146\1\160\3\152\1\163\2\0\1\164\14\152\1\153"+
"\4\152\1\165\1\150\1\146\2\0\1\163\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0152"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\151\5\152"+
"\1\150\1\146\1\153\10\152\1\146\1\160\3\152\3\0"+
"\1\164\5\152\1\153\13\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\u0158\5\152\1\150\1\146\1\152\1\u0245\7\152\1\146"+
"\1\160\3\152\3\0\1\u0218\6\152\1\u0245\12\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\u0218"+
"\1\170\1\u0159\1\172\1\173\1\u0150\4\152\1\u015a\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\2\150\1\0\6\146\1\150\10\146\1\u0246\2\146\1\150"+
"\3\146\3\0\15\146\1\u0246\4\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\1\146\5\172\1\150\1\146\11\172\1\146\1\173\3\172"+
"\3\u0227\22\172\1\u0163\1\150\1\146\2\0\1\u0227\2\146"+
"\1\150\1\146\1\172\2\146\1\172\1\173\1\u0247\4\172"+
"\1\150\2\172\2\173\1\0\1\150\2\172\1\150\1\176"+
"\10\172\5\146\1\0\1\173\1\146\1\0\1\173\2\150"+
"\1\146\1\150\1\0\2\150\1\0\17\150\1\u0248\6\150"+
"\3\0\15\150\1\u0248\7\150\3\0\23\150\1\0\4\150"+
"\1\266\15\150\1\0\2\150\1\0\4\150\1\146\1\0"+
"\2\150\1\0\3\146\1\u020e\2\146\1\150\1\146\1\u0249"+
"\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146\3\0"+
"\3\146\1\u020e\2\146\1\u0249\2\146\1\u0210\4\146\1\u0211"+
"\3\146\2\150\1\146\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150\2\146"+
"\1\150\1\176\15\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\2\150\1\0\3\146\1\u024a\2\146\1\150"+
"\4\146\1\u014f\3\146\1\u014f\2\146\1\150\2\u014f\1\146"+
"\3\0\3\146\1\u024a\5\146\1\u014f\3\146\1\u014f\1\146"+
"\2\u014f\1\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\6\146\1\150\3\146"+
"\1\u024b\7\146\1\150\3\146\3\0\10\146\1\u024b\11\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\1\146"+
"\1\150\1\0\2\150\1\0\12\150\1\u024c\13\150\3\0"+
"\10\150\1\u024c\14\150\3\0\23\150\1\0\4\150\1\266"+
"\15\150\1\0\2\150\1\0\4\150\1\146\1\0\1\147"+
"\1\150\1\0\1\u0158\5\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\1\163\2\0\1\303\21\152\1\165\1\150"+
"\1\146\2\0\1\163\1\166\1\146\1\150\1\167\1\303"+
"\1\170\1\u0159\1\172\1\173\1\u0152\4\152\1\u015a\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\1\146\1\150"+
"\1\0\1\u024d\1\150\1\0\1\150\5\u014d\2\150\11\u014d"+
"\1\150\1\u024e\3\u014d\3\0\22\u014d\1\u024f\2\150\3\0"+
"\1\u020d\3\150\1\u014d\5\150\4\u014d\1\150\2\u014d\2\u024e"+
"\1\0\1\150\2\u014d\1\150\1\266\10\u014d\5\150\1\0"+
"\1\u024e\1\150\1\0\1\u024e\4\150\1\0\1\147\1\150"+
"\1\0\1\150\5\160\2\150\11\160\1\150\1\u019c\3\160"+
"\3\0\22\160\1\u0250\1\261\1\150\2\0\1\262\1\263"+
"\3\150\1\160\1\264\1\150\1\173\2\265\4\160\1\150"+
"\2\160\2\u019c\1\0\1\150\2\160\1\150\1\266\10\160"+
"\5\150\1\0\1\u019c\1\150\1\0\1\u019c\3\150\1\u0166"+
"\1\u01da\1\u0251\1\u0166\1\u01da\1\u0166\5\u015c\2\u0166\11\u015c"+
"\1\u0166\1\u0252\3\u015c\3\u01da\22\u015c\1\u0224\1\u0166\1\150"+
"\3\u01da\1\u0253\3\u0166\1\u015c\1\u0221\3\u0166\1\150\4\u015c"+
"\1\u0166\2\u015c\2\u0252\1\u01da\1\u0166\2\u015c\1\u0166\1\u0223"+
"\10\u015c\5\u0166\1\u01da\1\u0252\1\u0166\1\u01da\1\u0252\3\u0166"+
"\1\150\1\0\2\150\1\0\22\150\1\u019e\3\150\3\0"+
"\23\150\1\261\1\150\3\0\10\150\2\261\7\150\2\u019e"+
"\1\0\4\150\1\266\15\150\1\0\1\u019e\1\150\1\0"+
"\1\u019e\3\150\2\0\1\u0254\24\0\1\u019f\36\0\1\u0255"+
"\20\0\2\u019f\24\0\1\u019f\2\0\1\u019f\3\0\1\150"+
"\1\0\2\150\1\0\1\150\5\173\2\150\11\173\1\150"+
"\1\u01a0\3\173\3\0\22\173\1\u0163\1\261\1\150\3\0"+
"\4\150\1\173\2\150\1\173\2\265\4\173\1\150\2\173"+
"\2\u01a0\1\0\1\150\2\173\1\150\1\266\10\173\5\150"+
"\1\0\1\u01a0\1\150\1\0\1\u01a0\4\150\1\0\2\150"+
"\1\0\1\150\5\u015b\2\150\3\u015b\1\u0256\5\u015b\1\150"+
"\1\u015c\3\u015b\3\0\11\u015b\1\u0256\10\u015b\3\150\3\0"+
"\4\150\1\u015b\5\150\4\u015b\1\150\2\u015b\2\u015c\1\0"+
"\1\150\2\u015b\1\150\1\266\10\u015b\5\150\1\0\1\u015c"+
"\1\150\1\0\1\u015c\3\150\1\146\1\0\2\150\1\0"+
"\1\146\1\u0257\1\146\1\u020e\2\146\1\150\1\146\1\u0258"+
"\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146\3\0"+
"\1\146\1\u0257\1\146\1\u020e\2\146\1\u0258\2\146\1\u0210"+
"\4\146\1\u0211\3\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259\5\146"+
"\1\150\13\146\1\150\3\146\3\0\22\u01a3\2\150\1\301"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\1\u0259\1\u0257\1\146"+
"\1\u020e\2\146\1\150\1\146\1\u0258\2\146\1\u0210\4\146"+
"\1\u0211\1\146\1\150\3\146\3\0\1\u01a3\1\u025a\1\u01a3"+
"\1\u025b\2\u01a3\1\u025c\2\u01a3\1\u025d\4\u01a3\1\u025e\3\u01a3"+
"\2\150\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\2\146\1\u020e\2\146\1\150\4\146\1\u0210\4\146\1\u0211"+
"\1\146\1\150\3\146\3\0\3\u01a3\1\u025b\5\u01a3\1\u025d"+
"\4\u01a3\1\u025e\3\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\1\147\1\150\1\0\1\u0158\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\303\21\152\1\u01a1\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\303\1\170"+
"\1\u0159\1\172\1\173\1\u0150\4\152\1\u015a\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\u01ad\5\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\275\21\276\1\u01a1\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\303\1\170\1\u0159"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\u015a"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\1\146\1\150\1\0\2\150\1\0\17\150\1\302\6\150"+
"\3\0\15\150\1\302\7\150\3\0\23\150\1\0\4\150"+
"\1\266\15\150\1\0\2\150\1\0\4\150\1\146\1\0"+
"\2\150\1\0\1\146\1\u0257\1\146\1\u020e\2\146\1\150"+
"\1\146\1\u020f\2\146\1\u0210\4\146\1\u0211\1\146\1\150"+
"\3\146\3\0\1\146\1\u0257\1\146\1\u020e\2\146\1\u020f"+
"\2\146\1\u0210\4\146\1\u0211\3\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\1\u0259\1\u0257\1\146\1\u020e\2\146\1\150\1\146\1\u020f"+
"\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146\3\0"+
"\1\u01a3\1\u025a\1\u01a3\1\u025b\2\u01a3\1\u025f\2\u01a3\1\u025d"+
"\4\u01a3\1\u025e\3\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\3\146\1\u0260\2\146\1\150\4\146\1\u014f"+
"\3\146\1\u014f\2\146\1\150\2\u014f\1\146\3\0\3\u01a3"+
"\1\u0261\5\u01a3\1\u01a5\3\u01a3\1\u01a5\1\u01a3\2\u01a5\1\u01a3"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\1\147\1\150\1\0"+
"\1\u01ad\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\275\21\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\303\1\170\1\u0159\1\172\1\173"+
"\1\u0151\1\276\1\152\1\276\1\152\1\u015a\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\u01ad\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\u0262\21\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\u0218\1\170"+
"\1\u0159\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\u015a\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\3\152"+
"\1\u0217\1\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\307\3\276\1\u0263\15\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\4\152\1\153"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\307"+
"\4\276\1\310\14\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\3\152\1\u0219\1\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\307\3\276"+
"\1\u0264\15\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\2\150"+
"\1\0\1\146\1\u0257\1\146\1\u020e\2\146\1\150\1\146"+
"\1\u0230\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146"+
"\3\0\1\146\1\u0257\1\146\1\u020e\2\146\1\u0230\2\146"+
"\1\u0210\4\146\1\u0211\3\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\1\u0257\1\146\1\u020e\2\146\1\150\1\146\1\u0230\2\146"+
"\1\u0210\4\146\1\u0211\1\146\1\150\3\146\3\0\1\u01a3"+
"\1\u025a\1\u01a3\1\u025b\2\u01a3\1\u0265\2\u01a3\1\u025d\4\u01a3"+
"\1\u025e\3\u01a3\2\150\1\301\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3"+
"\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\1\147"+
"\1\150\1\0\1\u01ad\5\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\275\21\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\303\1\170\1\u0159"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\u015a"+
"\1\u0266\1\u0231\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\11\152\1\146\1\160\1\u0236\2\152\3\0\1\307"+
"\16\276\1\u0267\2\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\307\21\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\1\312\1\155\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\307"+
"\21\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\310\1\153\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\2\150\1\0"+
"\1\u0259\5\146\1\150\13\146\1\150\3\146\3\0\22\u01a3"+
"\1\u0237\1\150\1\301\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146"+
"\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\1\u0259\3\146\1\u0238\1\146\1\150\13\146\1\150\3\146"+
"\3\0\4\u01a3\1\u0268\15\u01a3\2\150\1\301\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146"+
"\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146"+
"\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\1\147\1\150\1\0\1\u0269\5\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\307\21\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\u023a\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\u023b\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\11\152\1\146\1\160\1\152\1\u023c"+
"\1\152\3\0\1\307\17\276\1\u026a\1\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\u01ad\5\152"+
"\1\150\1\146\7\152\1\271\1\152\1\146\1\160\3\152"+
"\3\0\1\275\14\276\1\370\4\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\303\1\170\1\u0159"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\u015a"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\u01ad\3\152\1\155"+
"\1\152\1\150\1\146\11\152\1\146\1\160\3\152\1\163"+
"\2\0\1\275\3\276\1\312\15\276\1\165\1\150\1\301"+
"\2\0\1\163\1\166\1\146\1\302\1\167\1\303\1\170"+
"\1\u0159\1\172\1\173\1\u0152\1\276\1\152\1\276\1\152"+
"\1\u015a\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\1\152\1\153\7\152\1\146\1\160\3\152"+
"\3\0\1\307\6\276\1\310\12\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0151\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\1\u023d\10\152\1\146\1\160\3\152\3\0\1\307"+
"\5\276\1\u026b\13\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\1\153"+
"\10\152\1\146\1\160\3\152\3\0\1\307\5\276\1\310"+
"\13\276\1\u023e\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\313\1\156\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\306\5\152\1\150\1\146\4\152\1\u0128\4\152"+
"\1\146\1\160\3\152\3\0\1\307\11\276\1\u012b\7\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0151\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\3\152\1\153\1\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\307\3\276\1\310\15\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0151\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\2\152\1\u023f\2\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\3\0\1\307\2\276\1\u026c\16\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\2\150\1\0\1\146\1\u0257\1\146"+
"\1\u020e\2\146\1\150\1\146\1\u0241\2\146\1\u0210\4\146"+
"\1\u0211\1\146\1\150\3\146\3\0\1\146\1\u0257\1\146"+
"\1\u020e\2\146\1\u0241\2\146\1\u0210\4\146\1\u0211\3\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\2\150\1\0\1\u0259\1\u0257\1\146\1\u020e\2\146"+
"\1\150\1\146\1\u0241\2\146\1\u0210\4\146\1\u0211\1\146"+
"\1\150\3\146\3\0\1\u01a3\1\u025a\1\u01a3\1\u025b\2\u01a3"+
"\1\u026d\2\u01a3\1\u025d\4\u01a3\1\u025e\3\u01a3\2\150\1\301"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\1\147\1\150\1\0\1\u01ad\2\152"+
"\1\u0243\2\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\u026e\2\276\1\u026f\16\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\u0244\1\170\1\u0159"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\u015a"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\11\152\1\146\1\160\1\u0242\2\152\3\0\1\307"+
"\16\276\1\u0270\2\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\u01ad\5\152\1\150\1\146\1\152"+
"\1\u0245\7\152\1\146\1\160\3\152\3\0\1\u0262\6\276"+
"\1\u0271\12\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\u0218\1\170\1\u0159\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\u015a\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\7\152\1\153"+
"\1\152\1\146\1\160\3\152\1\163\2\0\1\307\14\276"+
"\1\310\4\276\1\165\1\150\1\301\2\0\1\163\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0152\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\1\153"+
"\10\152\1\146\1\160\3\152\3\0\1\307\5\276\1\310"+
"\13\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\2\150\1\0"+
"\1\146\1\u0257\1\146\1\u020e\2\146\1\150\1\146\1\u0272"+
"\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146\3\0"+
"\1\146\1\u0257\1\146\1\u020e\2\146\1\u0272\2\146\1\u0210"+
"\4\146\1\u0211\3\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259\1\u0257"+
"\1\146\1\u020e\2\146\1\150\1\146\1\u0272\2\146\1\u0210"+
"\4\146\1\u0211\1\146\1\150\3\146\3\0\1\u01a3\1\u025a"+
"\1\u01a3\1\u025b\2\u01a3\1\u0273\2\u01a3\1\u025d\4\u01a3\1\u025e"+
"\3\u01a3\2\150\1\301\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146"+
"\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\3\146\1\u0274\2\146\1\150\4\146\1\u014f\3\146\1\u014f"+
"\2\146\1\150\2\u014f\1\146\3\0\3\u01a3\1\u0275\5\u01a3"+
"\1\u01a5\3\u01a3\1\u01a5\1\u01a3\2\u01a5\1\u01a3\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\1\146\1\u0160\1\u01da\2\u0166\1\u01da\1\u0160\5\u0174"+
"\1\u0166\1\u0160\2\u0174\1\u0276\6\u0174\1\u0160\1\u0177\3\u0174"+
"\3\u01da\10\u0174\1\u0276\11\u0174\1\u0232\1\u0166\1\146\3\u01da"+
"\2\u0160\1\u0166\1\u0160\1\u0174\1\u0233\2\u0160\1\u0166\1\u014c"+
"\4\u0174\1\u0166\2\u0174\2\u0177\1\u01da\1\u0166\2\u0174\1\u0166"+
"\1\u0234\10\u0174\5\u0160\1\u01da\1\u0177\1\u0160\1\u01da\1\u0177"+
"\2\u0166\1\u0160\1\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u0177"+
"\2\u0166\2\u0177\1\u0277\6\u0177\1\u0166\4\u0177\3\u01da\10\u0177"+
"\1\u0277\11\u0177\1\u0232\1\u0166\1\150\3\u01da\4\u0166\1\u0177"+
"\1\u0232\3\u0166\1\150\4\u0177\1\u0166\4\u0177\1\u01da\1\u0166"+
"\2\u0177\1\u0166\1\u0223\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166"+
"\1\u01da\1\u0177\3\u0166\1\146\1\0\1\147\1\150\1\0"+
"\1\u01ad\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\1\163\2\0\1\275\21\276\1\165\1\150\1\301\2\0"+
"\1\163\1\166\1\146\1\302\1\167\1\303\1\170\1\u0159"+
"\1\172\1\173\1\u0152\1\276\1\152\1\276\1\152\1\u015a"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\1\146\121\0\2\u0278\50\0\1\u0279\31\0\1\377\2\0"+
"\1\5\11\0\2\377\7\0\2\u0279\24\0\1\u0279\2\0"+
"\1\u0279\32\0\1\u01d4\31\0\1\377\2\0\1\5\11\0"+
"\2\377\7\0\2\u01d4\24\0\1\u01d4\2\0\1\u01d4\3\0"+
"\1\126\4\0\6\126\1\0\13\126\1\0\3\126\3\0"+
"\22\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\14\126"+
"\1\u027a\1\126\2\0\1\126\4\0\2\126\4\0\3\126"+
"\1\u0143\2\126\1\0\1\126\1\u027b\2\126\1\u0145\4\126"+
"\1\u0146\1\126\1\0\3\126\3\0\3\126\1\u0143\2\126"+
"\1\u027b\2\126\1\u0145\4\126\1\u0146\3\126\2\0\1\126"+
"\3\0\2\126\1\0\5\126\1\0\1\127\4\126\1\0"+
"\2\126\4\0\2\126\1\0\16\126\2\0\1\126\4\0"+
"\2\126\4\0\6\126\1\0\3\126\1\u01d7\7\126\1\0"+
"\3\126\3\0\10\126\1\u01d7\11\126\2\0\1\126\3\0"+
"\2\126\1\0\5\126\1\0\1\127\4\126\1\0\2\126"+
"\4\0\2\126\1\0\16\126\2\0\1\126\4\0\2\126"+
"\4\0\6\126\1\0\13\126\1\0\1\126\1\u01d7\1\126"+
"\3\0\20\126\1\u01d7\1\126\2\0\1\126\3\0\2\126"+
"\1\0\5\126\1\0\1\127\4\126\1\0\2\126\4\0"+
"\2\126\1\0\16\126\2\0\1\126\4\0\1\126\17\0"+
"\1\u01da\26\0\1\u01da\126\0\1\u01da\24\0\1\u01da\65\0"+
"\1\146\1\0\2\150\1\0\3\146\1\u020e\2\146\1\150"+
"\1\146\1\u0258\2\146\1\u0210\4\146\1\u0211\1\146\1\150"+
"\3\146\3\0\3\146\1\u020e\2\146\1\u0258\2\146\1\u0210"+
"\4\146\1\u0211\3\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\1\146\1\126\4\0\6\126\1\0\3\126"+
"\1\u027c\7\126\1\0\3\126\3\0\10\126\1\u027c\11\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\2\126\4\0\6\126\1\0\4\126\1\u027d"+
"\6\126\1\0\3\126\3\0\11\126\1\u027d\10\126\2\0"+
"\1\126\3\0\2\126\1\0\5\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\1\126\33\0\3\u0227\27\0\1\u0227\56\0\1\126"+
"\4\0\3\126\1\u027e\2\126\1\0\13\126\1\0\3\126"+
"\3\0\3\126\1\u027e\16\126\2\0\1\126\3\0\2\126"+
"\1\0\5\126\1\0\1\127\4\126\1\0\2\126\4\0"+
"\2\126\1\0\16\126\2\0\1\126\4\0\1\126\11\0"+
"\1\5\21\0\3\u0227\4\0\1\5\22\0\1\u0227\143\0"+
"\1\u027f\155\0\1\u0280\44\0\1\146\1\0\1\147\1\150"+
"\1\0\1\151\2\152\1\u0281\2\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\164\2\152\1\u0281\16\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\164\21\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0152\4\152\1\214\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\2\152\1\u0281\2\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\307\2\276"+
"\1\u0282\16\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\5\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\307\21\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0152\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\1\146\17\0\1\u0283\26\0\1\u0283\105\0\1\u0284\30\0"+
"\1\u0284\102\0\6\u01df\5\0\2\u01df\11\0\2\u01df\3\0"+
"\3\u01df\22\0\12\u01df\1\0\5\u01df\4\0\1\u01df\2\0"+
"\4\u01df\2\0\2\u01df\10\0\15\u01df\20\0\1\u0285\26\0"+
"\1\u0285\104\0\1\u0286\30\0\1\u0286\102\0\1\146\1\0"+
"\1\147\1\150\1\0\1\151\5\152\1\150\1\146\11\152"+
"\1\146\1\160\1\152\1\u01e8\1\152\3\0\1\164\17\152"+
"\1\u01e8\1\152\1\165\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\11\152\1\146\1\160\1\152\1\u01e8\1\152"+
"\3\0\1\307\17\276\1\u01ea\1\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\1\146\1\150\1\0\1\147\1\150\1\0\1\150\5\160"+
"\2\150\11\160\1\150\4\160\3\0\22\160\1\165\2\150"+
"\3\0\1\263\3\150\1\160\1\264\1\150\3\173\4\160"+
"\1\150\4\160\1\0\1\150\2\160\1\150\1\266\2\u0287"+
"\6\160\5\150\1\0\1\160\1\150\1\0\1\160\3\150"+
"\1\146\1\0\1\147\1\150\1\0\1\306\5\152\1\150"+
"\1\146\11\152\1\146\1\160\3\152\3\0\1\307\21\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0288\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\164\21\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0288\4\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\u0158"+
"\5\152\1\150\1\146\6\152\1\u0289\2\152\1\146\1\160"+
"\3\152\3\0\1\303\13\152\1\u0289\5\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\303\1\170"+
"\1\u0159\1\172\1\173\1\u0150\4\152\1\u015a\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\u01ad\5\152\1\150\1\146\6\152\1\u0289"+
"\2\152\1\146\1\160\3\152\3\0\1\275\13\276\1\u028a"+
"\5\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\303\1\170\1\u0159\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\u015a\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\1\146\1\150\1\0\2\150"+
"\1\0\17\150\1\u028b\6\150\3\0\15\150\1\u028b\7\150"+
"\3\0\23\150\1\0\4\150\1\266\15\150\1\0\2\150"+
"\1\0\4\150\1\126\4\0\1\126\1\u028c\4\126\1\0"+
"\13\126\1\0\3\126\3\0\1\126\1\u028c\20\126\2\0"+
"\1\126\3\0\2\126\1\0\5\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\2\126\4\0\6\126\1\0\11\126\1\u028c\1\126"+
"\1\0\3\126\3\0\16\126\1\u028c\3\126\2\0\1\126"+
"\3\0\2\126\1\0\5\126\1\0\1\127\4\126\1\0"+
"\2\126\4\0\2\126\1\0\16\126\2\0\1\126\4\0"+
"\2\126\4\0\6\126\1\0\4\126\1\u028c\6\126\1\0"+
"\3\126\3\0\11\126\1\u028c\10\126\2\0\1\126\3\0"+
"\2\126\1\0\5\126\1\0\1\127\4\126\1\0\2\126"+
"\4\0\2\126\1\0\16\126\2\0\1\126\4\0\2\126"+
"\4\0\6\126\1\0\2\126\1\u028c\10\126\1\0\3\126"+
"\3\0\7\126\1\u028c\12\126\2\0\1\126\3\0\2\126"+
"\1\0\5\126\1\0\1\127\4\126\1\0\2\126\4\0"+
"\2\126\1\0\16\126\2\0\1\126\4\0\2\126\4\0"+
"\4\126\1\u028d\1\126\1\0\13\126\1\0\3\126\3\0"+
"\4\126\1\u028d\15\126\2\0\1\126\3\0\2\126\1\0"+
"\5\126\1\0\1\127\4\126\1\0\2\126\4\0\2\126"+
"\1\0\16\126\2\0\1\126\4\0\2\126\4\0\6\126"+
"\1\u028e\13\126\1\0\3\126\3\0\22\126\2\0\1\126"+
"\3\0\2\126\1\0\5\126\1\0\1\127\4\126\1\0"+
"\2\126\4\0\2\126\1\0\16\126\2\0\1\126\4\0"+
"\2\126\4\0\6\126\1\0\4\126\1\u028f\6\126\1\0"+
"\3\126\3\0\11\126\1\u028f\10\126\2\0\1\126\3\0"+
"\2\126\1\0\5\126\1\0\1\127\4\126\1\0\2\126"+
"\4\0\2\126\1\0\16\126\2\0\1\126\4\0\2\126"+
"\4\0\4\126\1\u0290\1\126\1\0\13\126\1\0\3\126"+
"\3\0\4\126\1\u0290\15\126\2\0\1\126\3\0\2\126"+
"\1\0\5\126\1\0\1\127\4\126\1\0\2\126\4\0"+
"\2\126\1\0\16\126\2\0\1\126\4\0\2\126\4\0"+
"\6\126\1\0\3\126\1\u0291\7\126\1\0\3\126\3\0"+
"\10\126\1\u0291\11\126\2\0\1\126\3\0\2\126\1\0"+
"\5\126\1\0\1\127\4\126\1\0\2\126\4\0\2\126"+
"\1\0\16\126\2\0\1\126\4\0\2\126\4\0\3\126"+
"\1\u0292\2\126\1\0\13\126\1\0\3\126\3\0\3\126"+
"\1\u0292\16\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\6\126\1\0"+
"\2\126\1\u0293\10\126\1\0\3\126\3\0\7\126\1\u0293"+
"\12\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\6\126\1\0\1\126"+
"\1\u0294\11\126\1\0\3\126\3\0\6\126\1\u0294\13\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\1\126\1\146\1\0\2\150\1\0\6\146"+
"\1\150\10\146\1\u0295\2\146\1\150\3\146\3\0\15\146"+
"\1\u0295\4\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\6\146\1\150\5\146"+
"\1\u0296\5\146\1\150\3\146\3\0\12\146\1\u0296\7\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\2\150\1\0\2\146\1\u0297\3\146\1\150\13\146"+
"\1\150\3\146\3\0\2\146\1\u0297\17\146\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\2\150"+
"\1\0\6\146\1\150\13\146\1\150\1\146\1\u0298\1\146"+
"\3\0\20\146\1\u0298\1\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\1\146\1\150\1\0\2\150\1\0"+
"\1\150\5\u0299\2\150\11\u0299\1\150\4\u0299\3\0\22\u0299"+
"\3\150\3\0\4\150\1\u0299\5\150\4\u0299\1\150\4\u0299"+
"\1\0\1\150\2\u0299\1\150\1\266\10\u0299\5\150\1\0"+
"\1\u0299\1\150\1\0\1\u0299\4\150\1\0\2\150\1\0"+
"\1\150\5\u029a\2\150\11\u029a\2\150\3\u029a\3\0\22\u029a"+
"\3\150\3\0\4\150\1\u029a\5\150\4\u029a\1\150\2\u029a"+
"\2\150\1\0\1\150\2\u029a\1\150\1\266\10\u029a\5\150"+
"\1\0\2\150\1\0\5\150\1\0\1\u020b\1\150\1\0"+
"\26\150\3\0\25\150\3\0\23\150\1\0\4\150\1\266"+
"\15\150\1\0\2\150\1\0\4\150\1\146\1\0\2\150"+
"\1\0\6\146\1\150\7\146\1\u029b\3\146\1\150\3\146"+
"\3\0\14\146\1\u029b\5\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\6\146"+
"\1\150\10\146\1\u029c\2\146\1\150\3\146\3\0\15\146"+
"\1\u029c\4\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\1\146\1\u029d\4\146"+
"\1\150\13\146\1\150\3\146\3\0\1\146\1\u029d\20\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\2\150\1\0\6\146\1\150\5\146\1\u029e\5\146"+
"\1\150\3\146\3\0\12\146\1\u029e\7\146\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\2\150"+
"\1\0\1\146\5\172\1\150\1\146\7\172\1\u0229\1\172"+
"\1\146\1\173\3\172\3\0\15\172\1\u0229\4\172\1\u0163"+
"\1\150\1\146\3\0\2\146\1\150\1\146\1\172\2\146"+
"\1\172\1\173\1\u0164\4\172\1\150\2\172\2\173\1\0"+
"\1\150\2\172\1\150\1\176\10\172\5\146\1\0\1\173"+
"\1\146\1\0\1\173\2\150\2\146\1\0\2\150\1\0"+
"\1\146\5\172\1\150\1\146\4\172\1\u022a\4\172\1\146"+
"\1\173\3\172\3\0\12\172\1\u022a\7\172\1\u0163\1\150"+
"\1\146\3\0\2\146\1\150\1\146\1\172\2\146\1\172"+
"\1\173\1\u0164\4\172\1\150\2\172\2\173\1\0\1\150"+
"\2\172\1\150\1\176\10\172\5\146\1\0\1\173\1\146"+
"\1\0\1\173\2\150\2\146\1\0\2\150\1\0\1\146"+
"\1\172\1\u022b\3\172\1\150\1\146\11\172\1\146\1\173"+
"\3\172\3\0\2\172\1\u022b\17\172\1\u0163\1\150\1\146"+
"\3\0\2\146\1\150\1\146\1\172\2\146\1\172\1\173"+
"\1\u0164\4\172\1\150\2\172\2\173\1\0\1\150\2\172"+
"\1\150\1\176\10\172\5\146\1\0\1\173\1\146\1\0"+
"\1\173\2\150\2\146\1\0\2\150\1\0\1\146\5\172"+
"\1\150\1\146\11\172\1\146\1\173\1\172\1\u022c\1\172"+
"\3\0\20\172\1\u022c\1\172\1\u0163\1\150\1\146\3\0"+
"\2\146\1\150\1\146\1\172\2\146\1\172\1\173\1\u0164"+
"\4\172\1\150\2\172\2\173\1\0\1\150\2\172\1\150"+
"\1\176\10\172\5\146\1\0\1\173\1\146\1\0\1\173"+
"\2\150\1\146\1\0\1\u029f\31\0\1\u02a0\1\0\1\u02a0"+
"\22\u02a1\5\0\1\u02a0\12\0\1\u02a1\1\0\1\u02a1\2\0"+
"\1\u02a1\5\0\1\u02a1\3\0\1\u02a1\1\0\1\u02a1\1\0"+
"\1\u02a1\1\0\1\u02a1\16\0\1\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\1\163\2\0\1\164\21\152\1\165\1\150\1\146"+
"\2\0\1\163\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0151\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\303\21\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\303\1\170\1\171"+
"\1\172\1\173\1\u0151\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\4\152\1\u023f\4\152"+
"\1\146\1\160\3\152\3\0\1\164\11\152\1\u023f\7\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\2\150\1\0\3\146\1\u020e\2\146\1\150\1\146"+
"\1\u02a2\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146"+
"\3\0\3\146\1\u020e\2\146\1\u02a2\2\146\1\u0210\4\146"+
"\1\u0211\3\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\1\146\1\u0166\1\u01da\1\u021d\1\u0166\1\u01da\1\u0166"+
"\5\u021e\2\u0166\11\u021e\1\u0166\1\u015c\3\u021e\3\u01da\22\u021e"+
"\1\u021f\1\u0166\1\150\3\u01da\1\u0220\3\u0166\1\u021e\1\u0221"+
"\3\u0166\1\150\4\u021e\1\u0166\2\u021e\2\u015c\1\u01da\1\u0166"+
"\2\u021e\1\u0166\1\u0223\10\u021e\5\u0166\1\u01da\1\u015c\1\u0166"+
"\1\u01da\1\u015c\3\u0166\1\150\1\0\2\150\1\0\1\150"+
"\5\u02a3\2\150\11\u02a3\1\150\1\u015c\3\u02a3\3\0\22\u02a3"+
"\3\150\3\0\4\150\1\u02a3\5\150\4\u02a3\1\150\2\u02a3"+
"\2\u015c\1\0\1\150\2\u02a3\1\150\1\266\10\u02a3\5\150"+
"\1\0\1\u015c\1\150\1\0\1\u015c\4\150\1\0\2\150"+
"\1\0\1\150\5\u02a4\2\150\11\u02a4\2\150\3\u02a4\3\0"+
"\22\u02a4\3\150\3\0\4\150\1\u02a4\5\150\4\u02a4\1\150"+
"\2\u02a4\2\150\1\0\1\150\2\u02a4\1\150\1\266\10\u02a4"+
"\5\150\1\0\2\150\1\0\5\150\1\0\2\150\1\0"+
"\1\150\5\u02a5\2\150\11\u02a5\1\150\1\u015c\3\u02a5\3\0"+
"\22\u02a5\3\150\3\0\4\150\1\u02a5\5\150\4\u02a5\1\150"+
"\2\u02a5\2\u015c\1\0\1\150\2\u02a5\1\150\1\266\10\u02a5"+
"\5\150\1\0\1\u015c\1\150\1\0\1\u015c\3\150\1\u0166"+
"\1\u01da\2\u0166\1\u01da\1\u0166\5\u0226\2\u0166\11\u0226\1\u0166"+
"\4\u0226\3\u01da\22\u0226\1\u02a6\1\u0166\1\150\3\u01da\4\u0166"+
"\1\u0226\4\u0166\1\u0222\4\u0226\1\u0166\4\u0226\1\u01da\1\u0166"+
"\2\u0226\1\u0166\1\u0223\10\u0226\5\u0166\1\u01da\1\u0226\1\u0166"+
"\1\u01da\1\u0226\4\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u0226"+
"\2\u0166\11\u0226\1\u0166\4\u0226\3\u01da\22\u0226\1\u02a6\1\u0166"+
"\1\150\3\u01da\4\u0166\1\u0226\4\u0166\1\150\4\u0226\1\u0166"+
"\4\u0226\1\u01da\1\u0166\2\u0226\1\u0166\1\u0223\10\u0226\5\u0166"+
"\1\u01da\1\u0226\1\u0166\1\u01da\1\u0226\3\u0166\1\146\1\0"+
"\2\150\1\0\1\146\5\u0165\1\150\1\146\6\u0165\1\u0167"+
"\1\u0168\1\u0165\1\146\1\173\1\u0165\1\u0169\1\u0165\3\0"+
"\1\u016a\13\u0165\1\u0167\1\u0168\2\u0165\1\u0169\1\u0165\1\u0163"+
"\1\150\1\146\3\0\2\146\1\150\1\146\1\u016a\2\146"+
"\1\172\2\173\4\u0165\1\150\2\u0165\2\173\1\0\1\150"+
"\2\u0165\1\150\1\176\10\u0165\5\146\1\0\1\173\1\146"+
"\1\0\1\173\2\150\2\146\1\0\2\150\1\0\1\146"+
"\1\u02a7\4\172\1\150\1\146\11\172\1\146\1\173\3\172"+
"\3\0\1\172\1\u02a7\20\172\1\u0163\1\150\1\146\3\0"+
"\2\146\1\150\1\146\1\172\2\146\1\172\1\173\1\u0164"+
"\4\172\1\150\2\172\2\173\1\0\1\150\2\172\1\150"+
"\1\176\10\172\5\146\1\0\1\173\1\146\1\0\1\173"+
"\2\150\2\146\1\0\2\150\1\0\1\146\5\172\1\150"+
"\1\146\10\172\1\u02a7\1\146\1\173\3\172\3\0\16\172"+
"\1\u02a7\3\172\1\u0163\1\150\1\146\3\0\2\146\1\150"+
"\1\146\1\172\2\146\1\172\1\173\1\u0164\4\172\1\150"+
"\2\172\2\173\1\0\1\150\2\172\1\150\1\176\10\172"+
"\5\146\1\0\1\173\1\146\1\0\1\173\2\150\2\146"+
"\1\0\2\150\1\0\1\146\5\172\1\150\1\146\3\172"+
"\1\u02a7\5\172\1\146\1\173\3\172\3\0\11\172\1\u02a7"+
"\10\172\1\u0163\1\150\1\146\3\0\2\146\1\150\1\146"+
"\1\172\2\146\1\172\1\173\1\u0164\4\172\1\150\2\172"+
"\2\173\1\0\1\150\2\172\1\150\1\176\10\172\5\146"+
"\1\0\1\173\1\146\1\0\1\173\2\150\2\146\1\0"+
"\2\150\1\0\1\146\5\172\1\150\1\146\1\172\1\u02a7"+
"\7\172\1\146\1\173\3\172\3\0\7\172\1\u02a7\12\172"+
"\1\u0163\1\150\1\146\3\0\2\146\1\150\1\146\1\172"+
"\2\146\1\172\1\173\1\u0164\4\172\1\150\2\172\2\173"+
"\1\0\1\150\2\172\1\150\1\176\10\172\5\146\1\0"+
"\1\173\1\146\1\0\1\173\2\150\1\146\1\150\1\0"+
"\2\150\1\0\26\150\3\0\25\150\3\0\23\150\1\0"+
"\1\150\2\302\1\150\1\266\15\150\1\0\2\150\1\0"+
"\4\150\1\u016f\1\0\2\u0170\1\0\1\u016f\5\u02a8\1\u0170"+
"\1\u016f\6\u02a8\1\u02a9\1\u02aa\1\u02a8\1\u016f\1\u0170\1\u02a8"+
"\1\u02ab\1\u02a8\3\0\1\u02ac\13\u02a8\1\u02a9\1\u02aa\2\u02a8"+
"\1\u02ab\1\u02a8\2\u0170\1\u016f\3\0\2\u016f\1\u0170\1\u016f"+
"\1\u02ac\3\u016f\1\u0170\1\150\4\u02a8\1\u0170\2\u02a8\2\u0170"+
"\1\0\1\u0170\2\u02a8\1\u0170\1\u016f\10\u02a8\5\u016f\1\0"+
"\1\u0170\1\u016f\1\0\3\u0170\1\u016f\1\u0170\1\0\2\u0170"+
"\1\0\1\u0170\5\u02ad\2\u0170\11\u02ad\2\u0170\3\u02ad\3\0"+
"\22\u02ad\3\u0170\3\0\4\u0170\1\u02ad\4\u0170\1\150\4\u02ad"+
"\1\u0170\2\u02ad\2\u0170\1\0\1\u0170\2\u02ad\2\u0170\10\u02ad"+
"\5\u0170\1\0\2\u0170\1\0\4\u0170\1\146\1\0\2\150"+
"\1\0\6\146\1\150\10\146\1\u02ae\2\146\1\150\3\146"+
"\3\0\15\146\1\u02ae\4\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\5\152\1\150\1\146\11\152\1\146\1\160\1\u02af"+
"\2\152\3\0\1\164\16\152\1\u02af\2\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\1\146\1\150\1\0"+
"\2\150\1\0\1\150\5\u0177\2\150\11\u0177\1\150\4\u0177"+
"\3\0\22\u0177\3\150\3\0\4\150\1\u0177\5\150\4\u0177"+
"\1\150\4\u0177\1\0\1\150\2\u0177\1\150\1\266\10\u0177"+
"\5\150\1\0\1\u0177\1\150\1\0\1\u0177\3\150\1\146"+
"\1\0\2\150\1\0\1\146\5\u0174\1\150\1\146\11\u0174"+
"\1\146\1\u0177\3\u0174\3\0\22\u0174\2\150\1\146\3\0"+
"\2\146\1\150\1\146\1\u0174\3\146\1\150\1\u014c\4\u0174"+
"\1\150\2\u0174\2\u0177\1\0\1\150\2\u0174\1\150\1\176"+
"\10\u0174\5\146\1\0\1\u0177\1\146\1\0\1\u0177\2\150"+
"\1\146\1\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u0177\2\u0166"+
"\11\u0177\1\u0166\1\u0177\1\u02b0\2\u0177\3\u01da\17\u0177\1\u02b0"+
"\2\u0177\1\u0232\1\u0166\1\150\3\u01da\4\u0166\1\u0177\1\u0232"+
"\3\u0166\1\150\4\u0177\1\u0166\4\u0177\1\u01da\1\u0166\2\u0177"+
"\1\u0166\1\u0223\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166\1\u01da"+
"\1\u0177\3\u0166\1\146\1\0\1\147\1\150\1\0\1\151"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\164\21\152\1\u023e\1\150\1\146\3\0\1\166\1\146"+
"\1\150\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\4\152\1\214\2\152\2\160\1\0\1\150\2\152\1\150"+
"\1\176\10\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\1\146\1\150\1\0\2\150\1\0\26\150\3\0"+
"\25\150\3\0\23\150\1\0\4\150\1\266\15\150\1\0"+
"\1\u02b1\1\150\1\0\4\150\1\146\1\0\2\150\1\0"+
"\3\146\1\u02b2\2\146\1\150\4\146\1\u014f\3\146\1\u014f"+
"\2\146\1\150\2\u014f\1\146\3\0\3\146\1\u02b2\5\146"+
"\1\u014f\3\146\1\u014f\1\146\2\u014f\1\146\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\2\150"+
"\1\0\1\146\2\u0160\1\146\1\u0160\1\146\1\150\3\146"+
"\1\u0161\1\146\1\u0162\5\146\1\150\1\u02b3\1\146\1\u0162"+
"\3\0\1\146\2\u0160\1\146\1\u0160\3\146\1\u0161\1\146"+
"\1\u0162\4\146\1\u02b3\1\146\1\u0162\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\1\146\1\150\1\0\2\150"+
"\1\0\1\150\2\u0166\1\150\1\u0166\5\150\1\u016c\1\150"+
"\1\u016d\6\150\1\u02b4\1\150\1\u016d\3\0\1\150\2\u0166"+
"\1\150\1\u0166\3\150\1\u016c\1\150\1\u016d\4\150\1\u02b4"+
"\1\150\1\u016d\3\150\3\0\23\150\1\0\4\150\1\266"+
"\15\150\1\0\2\150\1\0\4\150\1\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\1\152\1\u02b5"+
"\7\152\1\146\1\160\3\152\3\0\1\164\6\152\1\u02b5"+
"\12\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\151\3\152\1\u02b6"+
"\1\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\164\3\152\1\u02b6\15\152\1\165\1\u02b7\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\151\3\152\1\155\1\152\1\150\1\146\11\152\1\146"+
"\1\160\3\152\3\0\1\164\3\152\1\155\15\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\2\150\1\0\1\146\5\u0165\1\150\1\146\6\u0165\1\u0167"+
"\1\u0168\1\u0165\1\146\1\173\1\u0165\1\u0169\1\u0165\3\0"+
"\1\u016a\13\u0165\1\u0167\1\u0168\2\u0165\1\u0169\1\u0165\1\u02b8"+
"\1\150\1\146\3\0\2\146\1\150\1\146\1\u016a\2\146"+
"\1\172\2\173\4\u0165\1\150\2\u0165\2\173\1\0\1\150"+
"\2\u0165\1\150\1\176\10\u0165\5\146\1\0\1\173\1\146"+
"\1\0\1\173\2\150\2\146\1\0\2\150\1\0\6\146"+
"\1\150\10\146\1\u02b9\2\146\1\150\3\146\3\0\15\146"+
"\1\u02b9\4\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\1\147\1\150\1\0\1\151\5\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\164"+
"\21\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\2\153\6\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\151\1\152"+
"\1\u02ba\3\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\164\1\152\1\u02ba\17\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\7\152\1\u02bb\1\152"+
"\1\146\1\160\3\152\3\0\1\303\14\152\1\u02bb\4\152"+
"\1\165\1\150\1\146\3\0\1\166\1\146\1\150\1\167"+
"\1\303\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214"+
"\2\152\2\160\1\0\1\150\2\152\1\150\1\176\10\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\164\21\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\4\152\1\u02bc\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\2\150\1\0\6\146\1\150\13\146\1\150\3\146\3\0"+
"\1\u0238\21\146\2\150\1\146\3\0\2\146\1\150\1\146"+
"\1\u0238\3\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\146"+
"\3\u0165\1\u02bd\1\u0165\1\150\1\146\6\u0165\1\u0167\1\u0168"+
"\1\u0165\1\146\1\173\1\u0165\1\u0169\1\u0165\3\0\1\u016a"+
"\3\u0165\1\u02bd\7\u0165\1\u0167\1\u0168\2\u0165\1\u0169\1\u0165"+
"\1\u0163\1\150\1\146\3\0\2\146\1\150\1\146\1\u016a"+
"\2\146\1\172\2\173\4\u0165\1\150\2\u0165\2\173\1\0"+
"\1\150\2\u0165\1\150\1\176\10\u0165\5\146\1\0\1\173"+
"\1\146\1\0\1\173\2\150\1\146\1\150\1\0\2\150"+
"\1\0\26\150\3\0\1\302\24\150\3\0\4\150\1\302"+
"\16\150\1\0\4\150\1\266\15\150\1\0\2\150\1\0"+
"\4\150\1\146\1\0\2\150\1\0\6\146\1\150\10\146"+
"\1\u02be\2\146\1\150\3\146\3\0\15\146\1\u02be\4\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\2\150\1\0\3\146\1\u020e\2\146\1\150\1\146"+
"\1\u02bf\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146"+
"\3\0\3\146\1\u020e\2\146\1\u02bf\2\146\1\u0210\4\146"+
"\1\u0211\3\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\6\146\1\150\10\146"+
"\1\u02c0\2\146\1\150\3\146\3\0\15\146\1\u02c0\4\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\1\146"+
"\1\150\1\0\2\150\1\0\17\150\1\u02c1\6\150\3\0"+
"\15\150\1\u02c1\7\150\3\0\23\150\1\0\4\150\1\266"+
"\15\150\1\0\2\150\1\0\5\150\1\0\2\150\1\0"+
"\1\150\5\u0299\2\150\11\u0299\1\150\1\u02c2\3\u0299\3\0"+
"\22\u0299\3\150\3\0\4\150\1\u0299\5\150\4\u0299\1\150"+
"\2\u0299\2\u02c2\1\0\1\150\2\u0299\1\150\1\266\10\u0299"+
"\5\150\1\0\1\u02c2\1\150\1\0\1\u02c2\4\150\1\0"+
"\1\u024d\1\150\1\0\1\150\5\u014d\2\150\11\u014d\1\150"+
"\4\u014d\3\0\22\u014d\1\u024f\2\150\3\0\1\u020d\3\150"+
"\1\u014d\5\150\4\u014d\1\150\4\u014d\1\0\1\150\2\u014d"+
"\1\150\1\266\10\u014d\5\150\1\0\1\u014d\1\150\1\0"+
"\1\u014d\4\150\1\0\2\150\1\0\1\150\5\u029a\2\150"+
"\11\u029a\1\150\1\u02c3\3\u029a\3\0\22\u029a\3\150\3\0"+
"\4\150\1\u029a\5\150\4\u029a\1\150\2\u029a\2\u02c3\1\0"+
"\1\150\2\u029a\1\150\1\266\10\u029a\5\150\1\0\1\u02c3"+
"\1\150\1\0\1\u02c3\4\150\1\0\2\150\1\0\1\150"+
"\5\u015b\2\150\11\u015b\1\150\1\u02c4\3\u015b\3\0\22\u015b"+
"\3\150\3\0\4\150\1\u015b\5\150\4\u015b\1\150\2\u015b"+
"\2\u02c4\1\0\1\150\2\u015b\1\150\1\266\10\u015b\5\150"+
"\1\0\1\u02c4\1\150\1\0\1\u02c4\4\150\1\0\2\150"+
"\1\0\22\150\1\u02c5\3\150\3\0\25\150\3\0\21\150"+
"\2\u02c5\1\0\4\150\1\266\15\150\1\0\1\u02c5\1\150"+
"\1\0\1\u02c5\3\150\1\u0166\1\u01da\1\u0251\1\u0166\1\u01da"+
"\1\u0166\5\u015c\2\u0166\11\u015c\1\u0166\1\u02c4\3\u015c\3\u01da"+
"\22\u015c\1\u0224\1\u0166\1\150\3\u01da\1\u0253\3\u0166\1\u015c"+
"\1\u0221\3\u0166\1\150\4\u015c\1\u0166\2\u015c\2\u02c4\1\u01da"+
"\1\u0166\2\u015c\1\u0166\1\u0223\10\u015c\5\u0166\1\u01da\1\u02c4"+
"\1\u0166\1\u01da\1\u02c4\3\u0166\1\150\1\0\1\u02c6\1\150"+
"\1\0\26\150\3\0\25\150\3\0\23\150\1\0\4\150"+
"\1\266\15\150\1\0\2\150\1\0\4\150\27\0\1\u02c7"+
"\57\0\2\u02c7\24\0\1\u02c7\2\0\1\u02c7\5\0\1\u0254"+
"\141\0\1\u0166\1\u01da\1\u021d\1\u0166\1\u01da\1\u0166\5\u021e"+
"\2\u0166\11\u021e\1\u0166\1\u015c\3\u021e\3\u01da\22\u021e\1\u021f"+
"\1\u0166\1\150\3\u01da\1\u0220\3\u0166\1\u021e\1\u0221\3\u0166"+
"\1\u02c8\4\u021e\1\u0166\2\u021e\2\u015c\1\u01da\1\u0166\2\u021e"+
"\1\u0166\1\u0223\10\u021e\5\u0166\1\u01da\1\u015c\1\u0166\1\u01da"+
"\1\u015c\3\u0166\1\146\1\0\2\150\1\0\6\146\1\150"+
"\1\146\1\u02c9\11\146\1\150\3\146\3\0\6\146\1\u02c9"+
"\13\146\2\150\1\146\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150\2\146"+
"\1\150\1\176\15\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\2\150\1\0\6\146\1\150\10\146\1\u02ca"+
"\2\146\1\150\3\146\3\0\15\146\1\u02ca\4\146\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146"+
"\1\150\2\146\2\150\1\0\1\150\2\146\1\150\1\176"+
"\15\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\3\146\1\u02cb\2\146\1\150\13\146\1\150"+
"\3\146\3\0\3\u01a3\1\u02cc\16\u01a3\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3"+
"\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3"+
"\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\2\150\1\0\1\u0259\5\146\1\150\1\146"+
"\1\u02c9\11\146\1\150\3\146\3\0\6\u01a3\1\u02cd\13\u01a3"+
"\2\150\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\5\146\1\150\7\146\1\u029b\3\146\1\150\3\146\3\0"+
"\14\u01a3\1\u02ce\5\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\1\u0259\5\146\1\150\10\146\1\u02ca\2\146"+
"\1\150\3\146\3\0\15\u01a3\1\u02cf\4\u01a3\2\150\1\301"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\1\u0259\1\u029d\4\146"+
"\1\150\13\146\1\150\3\146\3\0\1\u01a3\1\u02d0\20\u01a3"+
"\2\150\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\5\146\1\150\5\146\1\u029e\5\146\1\150\3\146\3\0"+
"\12\u01a3\1\u02d1\7\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\1\u0259\5\146\1\150\10\146\1\u029c\2\146"+
"\1\150\3\146\3\0\15\u01a3\1\u02d2\4\u01a3\2\150\1\301"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\1\146\1\u0257\1\146"+
"\1\u020e\2\146\1\150\1\146\1\u02a2\2\146\1\u0210\4\146"+
"\1\u0211\1\146\1\150\3\146\3\0\1\146\1\u0257\1\146"+
"\1\u020e\2\146\1\u02a2\2\146\1\u0210\4\146\1\u0211\3\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\2\150\1\0\1\u0259\1\u0257\1\146\1\u020e\2\146"+
"\1\150\1\146\1\u02a2\2\146\1\u0210\4\146\1\u0211\1\146"+
"\1\150\3\146\3\0\1\u01a3\1\u025a\1\u01a3\1\u025b\2\u01a3"+
"\1\u02d3\2\u01a3\1\u025d\4\u01a3\1\u025e\3\u01a3\2\150\1\301"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\275"+
"\21\276\1\165\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\303\1\170\1\171\1\172\1\173\1\u0151\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\306\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\1\163\2\0\1\307\21\276\1\165\1\150\1\301"+
"\2\0\1\163\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0151\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\4\152\1\u023f\4\152\1\146\1\160\3\152"+
"\3\0\1\307\11\276\1\u026c\7\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\2\150\1\0\1\u0259\5\146\1\150\10\146"+
"\1\u02ae\2\146\1\150\3\146\3\0\15\u01a3\1\u02d4\4\u01a3"+
"\2\150\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\5\152\1\150\1\146\11\152\1\146\1\160\1\u02af"+
"\2\152\3\0\1\307\16\276\1\u02d5\2\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\307"+
"\21\276\1\u023e\1\150\1\301\3\0\1\166\1\146\1\302"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276"+
"\1\152\1\276\1\152\1\214\1\276\1\152\2\160\1\0"+
"\1\150\1\276\1\152\1\150\1\176\1\276\1\152\1\276"+
"\1\152\1\276\1\152\1\276\1\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\2\150\1\0"+
"\3\146\1\u02d6\2\146\1\150\4\146\1\u014f\3\146\1\u014f"+
"\2\146\1\150\2\u014f\1\146\3\0\3\u01a3\1\u02d7\5\u01a3"+
"\1\u01a5\3\u01a3\1\u01a5\1\u01a3\2\u01a5\1\u01a3\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\1\152\1\u02b5\7\152\1\146\1\160\3\152"+
"\3\0\1\307\6\276\1\u02d8\12\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\3\152\1\u02b6"+
"\1\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\307\3\276\1\u02d9\15\276\1\165\1\u02b7\1\301\3\0"+
"\1\166\1\146\1\302\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\306\3\152\1\155\1\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\307"+
"\3\276\1\312\15\276\1\165\1\150\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\2\150\1\0\1\u0259\5\146\1\150\10\146\1\u02b9\2\146"+
"\1\150\3\146\3\0\15\u01a3\1\u02da\4\u01a3\2\150\1\301"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\7\152\1\u02bb\1\152\1\146\1\160\3\152"+
"\3\0\1\275\14\276\1\u02db\4\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\303\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\1\152\1\u02ba"+
"\3\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\307\1\276\1\u02dc\17\276\1\165\1\150\1\301\3\0"+
"\1\166\1\146\1\302\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\1\147\1\150\1\0\1\306\5\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\307\21\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\310\1\153\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\307\21\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\u02bc\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\2\150"+
"\1\0\6\146\1\150\10\146\1\u02dd\2\146\1\150\3\146"+
"\3\0\15\146\1\u02dd\4\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\5\146\1\150\10\146\1\u02dd\2\146\1\150\3\146\3\0"+
"\15\u01a3\1\u02de\4\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\1\146\1\u0257\1\146\1\u020e\2\146\1\150"+
"\1\146\1\u02bf\2\146\1\u0210\4\146\1\u0211\1\146\1\150"+
"\3\146\3\0\1\146\1\u0257\1\146\1\u020e\2\146\1\u02bf"+
"\2\146\1\u0210\4\146\1\u0211\3\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\1\u0259\1\u0257\1\146\1\u020e\2\146\1\150\1\146\1\u02bf"+
"\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146\3\0"+
"\1\u01a3\1\u025a\1\u01a3\1\u025b\2\u01a3\1\u02df\2\u01a3\1\u025d"+
"\4\u01a3\1\u025e\3\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\1\146\1\u0160"+
"\1\u01da\2\u0166\1\u01da\1\u0160\5\u0174\1\u0166\1\u0160\7\u0174"+
"\1\u02e0\1\u0174\1\u0160\1\u0177\3\u0174\3\u01da\15\u0174\1\u02e0"+
"\4\u0174\1\u0232\1\u0166\1\146\3\u01da\2\u0160\1\u0166\1\u0160"+
"\1\u0174\1\u0233\2\u0160\1\u0166\1\u014c\4\u0174\1\u0166\2\u0174"+
"\2\u0177\1\u01da\1\u0166\2\u0174\1\u0166\1\u0234\10\u0174\5\u0160"+
"\1\u01da\1\u0177\1\u0160\1\u01da\1\u0177\2\u0166\1\u0160\1\u0166"+
"\1\u01da\2\u0166\1\u01da\1\u0166\5\u0177\2\u0166\7\u0177\1\u02e1"+
"\1\u0177\1\u0166\4\u0177\3\u01da\15\u0177\1\u02e1\4\u0177\1\u0232"+
"\1\u0166\1\150\3\u01da\4\u0166\1\u0177\1\u0232\3\u0166\1\150"+
"\4\u0177\1\u0166\4\u0177\1\u01da\1\u0166\2\u0177\1\u0166\1\u0223"+
"\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166\1\u01da\1\u0177\3\u0166"+
"\60\0\1\5\112\0\1\u01d4\31\0\1\377\2\0\1\u02e2"+
"\11\0\2\377\7\0\2\u01d4\24\0\1\u01d4\2\0\1\u01d4"+
"\3\0\1\126\4\0\6\126\1\0\13\126\1\0\3\126"+
"\3\0\22\126\2\0\1\126\3\0\1\u02e3\1\126\1\0"+
"\5\126\1\0\1\127\4\126\1\0\2\126\4\0\2\126"+
"\1\0\16\126\2\0\1\126\4\0\2\126\4\0\6\126"+
"\1\0\10\126\1\u02e4\2\126\1\0\3\126\3\0\15\126"+
"\1\u02e4\4\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\6\126\1\0"+
"\3\126\1\73\7\126\1\0\3\126\3\0\10\126\1\73"+
"\11\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\4\126\1\u02e5\1\126"+
"\1\0\13\126\1\0\3\126\3\0\4\126\1\u02e5\15\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\2\126\4\0\6\126\1\0\1\126\1\u02e6"+
"\11\126\1\0\3\126\3\0\6\126\1\u02e6\13\126\2\0"+
"\1\126\3\0\2\126\1\0\5\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\1\126\77\0\1\u02e7\143\0\1\u02e8\44\0\1\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\11\152\1\146\1\160\3\152\3\0\1\u01a6\21\152\1\165"+
"\1\150\1\146\3\0\1\166\1\146\1\150\1\167\1\u01a6"+
"\1\170\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152"+
"\2\160\1\0\1\150\2\152\1\150\1\176\10\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\1\147\1\150\1\0\1\306\5\152\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\u01a7\21\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\u01a6\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\1\146\17\0\1\5\26\0\1\5\106\0\1\5"+
"\30\0\1\5\112\0\1\u02e9\30\0\1\u02e9\116\0\1\u02ea"+
"\26\0\1\u02ea\77\0\1\u02eb\1\0\1\u02eb\1\150\1\0"+
"\1\u02eb\5\u02ec\1\u02ed\1\u02eb\6\u02ec\1\u02ee\1\u02ef\1\u02ec"+
"\1\u02eb\2\u02ec\1\u02f0\1\u02ec\3\0\1\u02f1\13\u02ec\1\u02ee"+
"\1\u02ef\2\u02ec\1\u02f0\1\u02ec\1\u02f2\1\u02ed\1\u02eb\3\0"+
"\4\u02eb\1\u02f1\2\u02eb\1\u02ec\1\u016b\1\173\4\u02ec\1\u02eb"+
"\4\u02ec\1\0\1\150\2\u02ec\1\u02eb\1\u02f3\10\u02ec\5\u02eb"+
"\1\0\1\u02ec\1\u02eb\1\0\1\u02ec\2\150\1\u02eb\1\146"+
"\1\0\1\147\1\150\1\0\1\151\5\152\1\150\1\146"+
"\7\152\1\u02f4\1\152\1\146\1\160\3\152\3\0\1\164"+
"\14\152\1\u02f4\4\152\1\165\1\150\1\146\3\0\1\166"+
"\1\146\1\150\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150\2\152"+
"\1\150\1\176\10\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\7\152\1\u02f4\1\152\1\146\1\160"+
"\3\152\3\0\1\307\14\276\1\u02f5\4\276\1\165\1\150"+
"\1\301\3\0\1\166\1\146\1\302\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152"+
"\1\214\1\276\1\152\2\160\1\0\1\150\1\276\1\152"+
"\1\150\1\176\1\276\1\152\1\276\1\152\1\276\1\152"+
"\1\276\1\152\5\146\1\0\1\160\1\146\1\0\1\160"+
"\2\150\1\146\1\150\1\0\2\150\1\0\4\150\1\u02f6"+
"\21\150\3\0\4\150\1\u02f6\20\150\3\0\23\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\4\150\1\126"+
"\1\0\1\u02f7\2\0\6\126\1\0\13\126\1\0\3\126"+
"\3\0\22\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\5\126\1\u02f8"+
"\1\0\13\126\1\0\3\126\3\0\5\126\1\u02f8\14\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\2\126\4\0\6\126\1\0\2\126\1\u02f9"+
"\10\126\1\0\3\126\3\0\7\126\1\u02f9\12\126\2\0"+
"\1\126\3\0\2\126\1\0\5\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\2\126\4\0\6\126\1\u02fa\13\126\1\0\3\126"+
"\3\0\22\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\6\126\1\u02fb"+
"\13\126\1\0\3\126\3\0\22\126\2\0\1\126\3\0"+
"\2\126\1\0\5\126\1\0\1\127\4\126\1\0\2\126"+
"\4\0\2\126\1\0\16\126\2\0\1\126\4\0\2\126"+
"\4\0\6\126\1\0\13\126\1\0\2\126\1\u02f9\3\0"+
"\21\126\1\u02f9\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\6\126\1\121"+
"\13\126\1\0\3\126\3\0\22\126\2\0\1\126\3\0"+
"\2\126\1\0\5\126\1\0\1\127\4\126\1\0\2\126"+
"\4\0\2\126\1\0\16\126\2\0\1\126\4\0\2\126"+
"\4\0\6\126";
private static final String ZZ_TRANS_PACKED_1 =
"\1\u02fc\13\126\1\0\3\126\3\0\22\126\2\0\1\126"+
"\3\0\2\126\1\0\5\126\1\0\1\127\4\126\1\0"+
"\2\126\4\0\2\126\1\0\16\126\2\0\1\126\4\0"+
"\1\126\1\146\1\0\2\150\1\0\1\146\1\u02fd\4\146"+
"\1\150\13\146\1\150\3\146\3\0\1\146\1\u02fd\20\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\2\150\1\0\6\146\1\150\11\146\1\u02fd\1\146"+
"\1\150\3\146\3\0\16\146\1\u02fd\3\146\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\2\150"+
"\1\0\6\146\1\150\4\146\1\u02fd\6\146\1\150\3\146"+
"\3\0\11\146\1\u02fd\10\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\6\146"+
"\1\150\2\146\1\u02fd\10\146\1\150\3\146\3\0\7\146"+
"\1\u02fd\12\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\1\146\1\150\1\0\2\150\1\0\1\150\5\u0299"+
"\2\150\11\u0299\1\150\4\u0299\3\0\22\u0299\1\u02fe\2\150"+
"\3\0\4\150\1\u0299\5\150\4\u0299\1\150\4\u0299\1\0"+
"\1\150\2\u0299\1\150\1\266\10\u0299\5\150\1\0\1\u0299"+
"\1\150\1\0\1\u0299\4\150\1\0\1\u020b\1\150\1\0"+
"\1\150\5\u029a\2\150\11\u029a\2\150\3\u029a\3\0\22\u029a"+
"\1\u02ff\2\150\3\0\1\u020d\3\150\1\u029a\5\150\4\u029a"+
"\1\150\2\u029a\2\150\1\0\1\150\2\u029a\1\150\1\266"+
"\10\u029a\5\150\1\0\2\150\1\0\4\150\1\146\1\0"+
"\2\150\1\0\6\146\1\150\4\146\1\u0300\6\146\1\150"+
"\3\146\3\0\11\146\1\u0300\10\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\4\146\1\u0301\1\146\1\150\13\146\1\150\3\146\3\0"+
"\4\146\1\u0301\15\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\6\146\1\150"+
"\3\146\1\u0302\7\146\1\150\3\146\3\0\10\146\1\u0302"+
"\11\146\2\150\1\146\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150\2\146"+
"\1\150\1\176\15\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\2\150\1\0\3\146\1\u0303\2\146\1\150"+
"\13\146\1\150\3\146\3\0\3\146\1\u0303\16\146\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146"+
"\1\150\2\146\2\150\1\0\1\150\2\146\1\150\1\176"+
"\15\146\1\0\1\150\1\146\1\0\3\150\1\146\2\0"+
"\1\u0304\1\u0305\2\0\5\u0305\2\0\11\u0305\2\0\3\u0305"+
"\3\0\22\u0305\12\0\1\u0305\5\0\4\u0305\1\0\2\u0305"+
"\4\0\2\u0305\2\0\10\u0305\15\0\1\146\1\0\2\150"+
"\1\0\6\146\1\150\10\146\1\u0306\2\146\1\150\3\146"+
"\3\0\15\146\1\u0306\4\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\1\146\1\u0166\1\u01da\1\u021d\1\u0166"+
"\1\u01da\1\u0166\5\u0307\2\u0166\11\u0307\1\u0166\1\u015c\3\u0307"+
"\3\u01da\22\u0307\1\u0224\1\u0166\1\150\3\u01da\1\u0220\3\u0166"+
"\1\u0307\1\u0221\3\u0166\1\u0222\4\u0307\1\u0166\2\u0307\2\u015c"+
"\1\u01da\1\u0166\2\u0307\1\u0166\1\u0223\10\u0307\5\u0166\1\u01da"+
"\1\u015c\1\u0166\1\u01da\1\u015c\3\u0166\1\150\1\0\2\150"+
"\1\0\26\150\3\0\25\150\3\0\11\150\1\u0308\11\150"+
"\1\0\4\150\1\266\15\150\1\0\2\150\1\0\4\150"+
"\1\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u015c\2\u0166\11\u015c"+
"\1\u0166\4\u015c\3\u01da\22\u015c\1\u0224\1\u0166\1\150\3\u01da"+
"\4\u0166\1\u015c\1\u0221\3\u0166\1\u0222\4\u015c\1\u0166\4\u015c"+
"\1\u01da\1\u0166\2\u015c\1\u0166\1\u0223\10\u015c\5\u0166\1\u01da"+
"\1\u015c\1\u0166\1\u01da\1\u015c\3\u0166\1\146\1\0\1\u02f7"+
"\1\150\1\0\1\146\5\172\1\150\1\146\11\172\1\146"+
"\1\173\3\172\3\0\22\172\1\u0163\1\150\1\146\3\0"+
"\2\146\1\150\1\146\1\172\2\146\1\172\1\173\1\u0164"+
"\4\172\1\150\2\172\2\173\1\0\1\150\2\172\1\150"+
"\1\176\10\172\5\146\1\0\1\173\1\146\1\0\1\173"+
"\2\150\1\146\1\u016f\1\0\2\u0170\1\0\1\u016f\5\u0309"+
"\1\u0170\1\u016f\11\u0309\1\u016f\1\u0170\3\u0309\3\0\22\u0309"+
"\2\u0170\1\u016f\3\0\2\u016f\1\u0170\1\u016f\1\u0309\3\u016f"+
"\1\u0170\1\u022e\4\u0309\1\u0170\2\u0309\2\u0170\1\0\1\u0170"+
"\2\u0309\1\u0170\1\u016f\10\u0309\5\u016f\1\0\1\u0170\1\u016f"+
"\1\0\3\u0170\2\u016f\1\0\2\u0170\1\0\1\u016f\5\u0309"+
"\1\u0170\1\u016f\7\u0309\1\u030a\1\u0309\1\u016f\1\u0170\3\u0309"+
"\3\0\15\u0309\1\u030a\4\u0309\2\u0170\1\u016f\3\0\2\u016f"+
"\1\u0170\1\u016f\1\u0309\3\u016f\1\u0170\1\u022e\4\u0309\1\u0170"+
"\2\u0309\2\u0170\1\0\1\u0170\2\u0309\1\u0170\1\u016f\10\u0309"+
"\5\u016f\1\0\1\u0170\1\u016f\1\0\3\u0170\2\u016f\1\0"+
"\2\u0170\1\0\1\u016f\5\u0309\1\u0170\1\u016f\4\u0309\1\u030b"+
"\4\u0309\1\u016f\1\u0170\3\u0309\3\0\12\u0309\1\u030b\7\u0309"+
"\2\u0170\1\u016f\3\0\2\u016f\1\u0170\1\u016f\1\u0309\3\u016f"+
"\1\u0170\1\u022e\4\u0309\1\u0170\2\u0309\2\u0170\1\0\1\u0170"+
"\2\u0309\1\u0170\1\u016f\10\u0309\5\u016f\1\0\1\u0170\1\u016f"+
"\1\0\3\u0170\2\u016f\1\0\2\u0170\1\0\1\u016f\1\u0309"+
"\1\u030c\3\u0309\1\u0170\1\u016f\11\u0309\1\u016f\1\u0170\3\u0309"+
"\3\0\2\u0309\1\u030c\17\u0309\2\u0170\1\u016f\3\0\2\u016f"+
"\1\u0170\1\u016f\1\u0309\3\u016f\1\u0170\1\u022e\4\u0309\1\u0170"+
"\2\u0309\2\u0170\1\0\1\u0170\2\u0309\1\u0170\1\u016f\10\u0309"+
"\5\u016f\1\0\1\u0170\1\u016f\1\0\3\u0170\2\u016f\1\0"+
"\2\u0170\1\0\1\u016f\5\u0309\1\u0170\1\u016f\11\u0309\1\u016f"+
"\1\u0170\1\u0309\1\u030d\1\u0309\3\0\20\u0309\1\u030d\1\u0309"+
"\2\u0170\1\u016f\3\0\2\u016f\1\u0170\1\u016f\1\u0309\3\u016f"+
"\1\u0170\1\u022e\4\u0309\1\u0170\2\u0309\2\u0170\1\0\1\u0170"+
"\2\u0309\1\u0170\1\u016f\10\u0309\5\u016f\1\0\1\u0170\1\u016f"+
"\1\0\3\u0170\1\u016f\1\u0170\1\0\2\u0170\1\0\1\u0170"+
"\5\u030e\2\u0170\11\u030e\2\u0170\3\u030e\3\0\22\u030e\3\u0170"+
"\3\0\4\u0170\1\u030e\4\u0170\1\u022f\4\u030e\1\u0170\2\u030e"+
"\2\u0170\1\0\1\u0170\2\u030e\2\u0170\10\u030e\5\u0170\1\0"+
"\2\u0170\1\0\4\u0170\1\146\1\0\2\150\1\0\4\146"+
"\1\u030f\1\146\1\150\13\146\1\150\3\146\3\0\4\146"+
"\1\u030f\15\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\1\147\1\150\1\0\1\151\5\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\u0310"+
"\21\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\u0310\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\1\146\1\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u0177\2\u0166"+
"\6\u0177\1\u0311\2\u0177\1\u0166\4\u0177\3\u01da\14\u0177\1\u0311"+
"\5\u0177\1\u0232\1\u0166\1\150\3\u01da\4\u0166\1\u0177\1\u0232"+
"\3\u0166\1\150\4\u0177\1\u0166\4\u0177\1\u01da\1\u0166\2\u0177"+
"\1\u0166\1\u0223\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166\1\u01da"+
"\1\u0177\3\u0166\1\150\1\0\2\150\1\0\26\150\3\0"+
"\25\150\3\0\22\150\1\u0312\1\0\4\150\1\266\15\150"+
"\1\0\2\150\1\0\4\150\1\146\1\0\2\150\1\0"+
"\3\146\1\u020e\2\146\1\150\1\146\1\u0313\2\146\1\u0210"+
"\4\146\1\u0211\1\146\1\150\3\146\3\0\3\146\1\u020e"+
"\2\146\1\u0313\2\146\1\u0210\4\146\1\u0211\3\146\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146"+
"\1\150\2\146\2\150\1\0\1\150\2\146\1\150\1\176"+
"\15\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\6\146\1\150\2\146\1\u0314\10\146\1\150"+
"\3\146\3\0\7\146\1\u0314\12\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\1\146\1\150\1\0\2\150"+
"\1\0\11\150\1\u0315\14\150\3\0\7\150\1\u0315\15\150"+
"\3\0\23\150\1\0\4\150\1\266\15\150\1\0\2\150"+
"\1\0\4\150\1\146\1\0\1\147\1\150\1\0\1\151"+
"\4\152\1\u0231\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\164\4\152\1\u0231\14\152\1\165\1\150\1\146"+
"\3\0\1\166\1\146\1\150\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0"+
"\1\150\2\152\1\150\1\176\10\152\5\146\1\0\1\160"+
"\1\146\1\0\1\160\2\150\2\146\1\0\1\147\1\150"+
"\1\0\1\151\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\3\0\1\164\21\152\1\165\1\u02b7\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\1\146\1\150\1\0\1\u0316\1\150"+
"\1\0\26\150\3\0\25\150\3\0\23\150\1\0\4\150"+
"\1\266\15\150\1\0\2\150\1\0\5\150\1\0\2\150"+
"\1\0\1\150\5\u0225\2\150\3\u0225\1\u0317\5\u0225\1\150"+
"\1\u0226\3\u0225\3\0\11\u0225\1\u0317\10\u0225\3\150\3\0"+
"\4\150\1\u0225\5\150\4\u0225\1\150\2\u0225\2\u0226\1\0"+
"\1\150\2\u0225\1\150\1\266\10\u0225\5\150\1\0\1\u0226"+
"\1\150\1\0\1\u0226\3\150\1\146\1\0\2\150\1\0"+
"\4\146\1\u0318\1\146\1\150\13\146\1\150\3\146\3\0"+
"\4\146\1\u0318\15\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\1\147\1\150\1\0\1\151"+
"\2\152\1\271\2\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\3\0\1\164\2\152\1\271\16\152\1\165\1\150"+
"\1\146\3\0\1\166\1\146\1\150\1\167\1\164\1\170"+
"\1\171\1\172\1\173\1\u0150\4\152\1\214\2\152\2\160"+
"\1\0\1\150\2\152\1\150\1\176\10\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\1\147"+
"\1\150\1\0\1\151\5\152\1\150\1\146\1\152\1\u0319"+
"\7\152\1\146\1\160\3\152\3\0\1\164\6\152\1\u0319"+
"\12\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\164\1\170\1\171\1\172\1\173\1\u0150\4\152"+
"\1\214\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\1\146\1\150\1\0\2\150\1\0\1\150\1\u0166\1\u031a"+
"\1\150\1\u0166\5\150\1\u016c\1\150\1\u016d\10\150\1\u016d"+
"\3\0\1\150\1\u0166\1\u031a\1\150\1\u0166\3\150\1\u016c"+
"\1\150\1\u016d\6\150\1\u016d\3\150\3\0\23\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\4\150\1\146"+
"\1\0\2\150\1\0\4\146\1\u031b\1\146\1\150\13\146"+
"\1\150\3\146\3\0\4\146\1\u031b\15\146\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\2\150"+
"\1\0\6\146\1\150\10\146\1\u031c\2\146\1\150\3\146"+
"\3\0\15\146\1\u031c\4\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\6\146"+
"\1\150\7\146\1\u031d\3\146\1\150\3\146\3\0\14\146"+
"\1\u031d\5\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\1\146\1\150\1\0\2\150\1\0\16\150\1\u031e"+
"\7\150\3\0\14\150\1\u031e\10\150\3\0\23\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\5\150\1\0"+
"\2\150\1\0\1\150\5\u0299\2\150\11\u0299\1\150\1\u031f"+
"\3\u0299\3\0\22\u0299\1\u02fe\2\150\3\0\4\150\1\u0299"+
"\5\150\4\u0299\1\150\2\u0299\2\u031f\1\0\1\150\2\u0299"+
"\1\150\1\266\10\u0299\5\150\1\0\1\u031f\1\150\1\0"+
"\1\u031f\4\150\1\0\2\150\1\0\22\150\1\u0320\3\150"+
"\3\0\25\150\3\0\21\150\2\u0320\1\0\4\150\1\266"+
"\15\150\1\0\1\u0320\1\150\1\0\1\u0320\3\150\1\u0166"+
"\1\u01da\1\u0321\1\u0166\1\u01da\1\u0166\5\u015c\2\u0166\11\u015c"+
"\1\u0166\1\u02c4\3\u015c\3\u01da\22\u015c\1\u0224\1\u0166\1\150"+
"\3\u01da\1\u0253\3\u0166\1\u015c\1\u0221\3\u0166\1\150\4\u015c"+
"\1\u0166\2\u015c\2\u02c4\1\u01da\1\u0166\2\u015c\1\u0166\1\u0223"+
"\10\u015c\5\u0166\1\u01da\1\u02c4\1\u0166\1\u01da\1\u02c4\3\u0166"+
"\1\150\1\0\2\150\1\0\22\150\1\u0322\3\150\3\0"+
"\25\150\3\0\21\150\2\u0322\1\0\4\150\1\266\15\150"+
"\1\0\1\u0322\1\150\1\0\1\u0322\4\150\1\0\2\150"+
"\1\0\22\150\1\u0323\3\150\3\0\25\150\3\0\21\150"+
"\2\u0323\1\0\4\150\1\266\15\150\1\0\1\u0323\1\150"+
"\1\0\1\u0323\4\150\1\0\2\150\1\0\1\150\3\u02a4"+
"\1\u0324\1\u02a4\2\150\11\u02a4\2\150\3\u02a4\3\0\4\u02a4"+
"\1\u0324\15\u02a4\3\150\3\0\4\150\1\u02a4\5\150\4\u02a4"+
"\1\150\2\u02a4\2\150\1\0\1\150\2\u02a4\1\150\1\266"+
"\10\u02a4\5\150\1\0\2\150\1\0\4\150\1\146\1\0"+
"\2\150\1\0\6\146\1\u0325\13\146\1\150\3\146\3\0"+
"\22\146\2\150\1\146\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150\2\146"+
"\1\150\1\176\15\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\2\150\1\0\4\146\1\u0326\1\146\1\150"+
"\13\146\1\150\3\146\3\0\4\146\1\u0326\15\146\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146"+
"\1\150\2\146\2\150\1\0\1\150\2\146\1\150\1\176"+
"\15\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\1\146\1\u0257\4\146\1\150\13\146\1\150"+
"\3\146\3\0\1\146\1\u0257\20\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\1\u0259\1\u0257\4\146\1\150\13\146\1\150\3\146\3\0"+
"\1\u01a3\1\u025a\20\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\1\u0259\5\146\1\u0325\13\146\1\150\3\146"+
"\3\0\22\u01a3\2\150\1\301\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3"+
"\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\2\150"+
"\1\0\1\u0259\5\146\1\150\4\146\1\u0300\6\146\1\150"+
"\3\146\3\0\11\u01a3\1\u0327\10\u01a3\2\150\1\301\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3"+
"\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3"+
"\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\2\150\1\0\1\u0259\3\146\1\u0326\1\146"+
"\1\150\13\146\1\150\3\146\3\0\4\u01a3\1\u0328\15\u01a3"+
"\2\150\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\5\146\1\150\3\146\1\u0302\7\146\1\150\3\146\3\0"+
"\10\u01a3\1\u0329\11\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\1\u0259\2\146\1\u0303\2\146\1\150\13\146"+
"\1\150\3\146\3\0\3\u01a3\1\u032a\16\u01a3\2\150\1\301"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\1\u0259\3\146\1\u0301"+
"\1\146\1\150\13\146\1\150\3\146\3\0\4\u01a3\1\u032b"+
"\15\u01a3\2\150\1\301\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146"+
"\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\1\u0259\5\146\1\150\10\146\1\u0306\2\146\1\150\3\146"+
"\3\0\15\u01a3\1\u032c\4\u01a3\2\150\1\301\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146"+
"\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146"+
"\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\2\150\1\0\1\u0259\3\146\1\u030f\1\146\1\150"+
"\13\146\1\150\3\146\3\0\4\u01a3\1\u032d\15\u01a3\2\150"+
"\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3"+
"\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0"+
"\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\1\147\1\150\1\0\1\306"+
"\5\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\u032e\21\276\1\165\1\150\1\301\3\0\1\166\1\146"+
"\1\302\1\167\1\u0310\1\170\1\171\1\172\1\173\1\u0150"+
"\1\276\1\152\1\276\1\152\1\214\1\276\1\152\2\160"+
"\1\0\1\150\1\276\1\152\1\150\1\176\1\276\1\152"+
"\1\276\1\152\1\276\1\152\1\276\1\152\5\146\1\0"+
"\1\160\1\146\1\0\1\160\2\150\2\146\1\0\2\150"+
"\1\0\1\146\1\u0257\1\146\1\u020e\2\146\1\150\1\146"+
"\1\u0313\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146"+
"\3\0\1\146\1\u0257\1\146\1\u020e\2\146\1\u0313\2\146"+
"\1\u0210\4\146\1\u0211\3\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\1\u0257\1\146\1\u020e\2\146\1\150\1\146\1\u0313\2\146"+
"\1\u0210\4\146\1\u0211\1\146\1\150\3\146\3\0\1\u01a3"+
"\1\u025a\1\u01a3\1\u025b\2\u01a3\1\u032f\2\u01a3\1\u025d\4\u01a3"+
"\1\u025e\3\u01a3\2\150\1\301\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3"+
"\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\1\147"+
"\1\150\1\0\1\306\4\152\1\u0231\1\150\1\146\11\152"+
"\1\146\1\160\3\152\3\0\1\307\4\276\1\u0266\14\276"+
"\1\165\1\150\1\301\3\0\1\166\1\146\1\302\1\167"+
"\1\164\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152"+
"\1\276\1\152\1\214\1\276\1\152\2\160\1\0\1\150"+
"\1\276\1\152\1\150\1\176\1\276\1\152\1\276\1\152"+
"\1\276\1\152\1\276\1\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\5\152\1\150\1\146\11\152\1\146\1\160\3\152"+
"\3\0\1\307\21\276\1\165\1\u02b7\1\301\3\0\1\166"+
"\1\146\1\302\1\167\1\164\1\170\1\171\1\172\1\173"+
"\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276\1\152"+
"\2\160\1\0\1\150\1\276\1\152\1\150\1\176\1\276"+
"\1\152\1\276\1\152\1\276\1\152\1\276\1\152\5\146"+
"\1\0\1\160\1\146\1\0\1\160\2\150\2\146\1\0"+
"\2\150\1\0\1\u0259\3\146\1\u0318\1\146\1\150\13\146"+
"\1\150\3\146\3\0\4\u01a3\1\u0330\15\u01a3\2\150\1\301"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\1\147\1\150\1\0\1\306\5\152"+
"\1\150\1\146\1\152\1\u0319\7\152\1\146\1\160\3\152"+
"\3\0\1\307\6\276\1\u0331\12\276\1\165\1\150\1\301"+
"\3\0\1\166\1\146\1\302\1\167\1\164\1\170\1\171"+
"\1\172\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214"+
"\1\276\1\152\2\160\1\0\1\150\1\276\1\152\1\150"+
"\1\176\1\276\1\152\1\276\1\152\1\276\1\152\1\276"+
"\1\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\2\146\1\0\1\147\1\150\1\0\1\306\2\152\1\271"+
"\2\152\1\150\1\146\11\152\1\146\1\160\3\152\3\0"+
"\1\307\2\276\1\370\16\276\1\165\1\150\1\301\3\0"+
"\1\166\1\146\1\302\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\1\276\1\152\1\276\1\152\1\214\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\2\150\1\0\4\146\1\u0332\1\146\1\150\13\146"+
"\1\150\3\146\3\0\4\146\1\u0332\15\146\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\2\150"+
"\1\0\1\u0259\3\146\1\u0332\1\146\1\150\13\146\1\150"+
"\3\146\3\0\4\u01a3\1\u0333\15\u01a3\2\150\1\301\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3"+
"\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3"+
"\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150"+
"\2\146\1\0\2\150\1\0\1\u0259\5\146\1\150\10\146"+
"\1\u031c\2\146\1\150\3\146\3\0\15\u01a3\1\u0334\4\u01a3"+
"\2\150\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\1\146\1\u0160\1\u01da\2\u0166\1\u01da"+
"\1\u0160\5\u0174\1\u0166\1\u0160\6\u0174\1\u0335\2\u0174\1\u0160"+
"\1\u0177\3\u0174\3\u01da\14\u0174\1\u0335\5\u0174\1\u0232\1\u0166"+
"\1\146\3\u01da\2\u0160\1\u0166\1\u0160\1\u0174\1\u0233\2\u0160"+
"\1\u0166\1\u014c\4\u0174\1\u0166\2\u0174\2\u0177\1\u01da\1\u0166"+
"\2\u0174\1\u0166\1\u0234\10\u0174\5\u0160\1\u01da\1\u0177\1\u0160"+
"\1\u01da\1\u0177\2\u0166\1\u0160\1\u0166\1\u01da\2\u0166\1\u01da"+
"\1\u0166\5\u0177\2\u0166\6\u0177\1\u0336\2\u0177\1\u0166\4\u0177"+
"\3\u01da\14\u0177\1\u0336\5\u0177\1\u0232\1\u0166\1\150\3\u01da"+
"\4\u0166\1\u0177\1\u0232\3\u0166\1\150\4\u0177\1\u0166\4\u0177"+
"\1\u01da\1\u0166\2\u0177\1\u0166\1\u0223\10\u0177\5\u0166\1\u01da"+
"\1\u0177\1\u0166\1\u01da\1\u0177\3\u0166\27\0\1\u0337\35\0"+
"\1\u0338\21\0\2\u0337\24\0\1\u0337\2\0\1\u0337\3\0"+
"\1\126\4\0\6\126\1\0\13\126\1\0\3\126\3\0"+
"\22\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\14\126"+
"\1\u0339\1\126\2\0\1\126\4\0\2\126\4\0\4\126"+
"\1\u033a\1\126\1\0\13\126\1\0\3\126\3\0\4\126"+
"\1\u033a\15\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\6\126\1\0"+
"\13\126\1\0\1\126\1\73\1\126\3\0\20\126\1\73"+
"\1\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\2\126\4\0\6\126\1\0\10\126"+
"\1\u033b\2\126\1\0\3\126\3\0\15\126\1\u033b\4\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\1\126\65\0\1\u033c\107\0\1\5\24\0"+
"\1\5\111\0\1\u033d\26\0\1\u033d\70\0\1\u02eb\1\0"+
"\1\u02eb\1\150\1\0\26\u02eb\3\0\25\u02eb\3\0\10\u02eb"+
"\1\150\1\u033e\11\u02eb\1\0\1\150\3\u02eb\1\u02f3\15\u02eb"+
"\1\0\2\u02eb\1\0\1\u02eb\2\150\2\u02eb\1\0\1\u02eb"+
"\1\150\1\0\1\u02eb\5\u02ec\2\u02eb\11\u02ec\1\u02eb\4\u02ec"+
"\3\0\22\u02ec\1\u02f2\2\u02eb\3\0\4\u02eb\1\u02ec\2\u02eb"+
"\1\u02ec\1\173\1\u033f\4\u02ec\1\u02eb\4\u02ec\1\0\1\150"+
"\2\u02ec\1\u02eb\1\u02f3\10\u02ec\5\u02eb\1\0\1\u02ec\1\u02eb"+
"\1\0\1\u02ec\2\150\2\u02eb\1\0\1\u02eb\1\150\1\0"+
"\1\u02eb\5\u02ec\2\u02eb\7\u02ec\1\u0340\1\u02ec\1\u02eb\4\u02ec"+
"\3\0\15\u02ec\1\u0340\4\u02ec\1\u02f2\2\u02eb\3\0\4\u02eb"+
"\1\u02ec\2\u02eb\1\u02ec\1\173\1\u033f\4\u02ec\1\u02eb\4\u02ec"+
"\1\0\1\150\2\u02ec\1\u02eb\1\u02f3\10\u02ec\5\u02eb\1\0"+
"\1\u02ec\1\u02eb\1\0\1\u02ec\2\150\2\u02eb\1\0\1\u02eb"+
"\1\150\1\0\1\u02eb\5\u02ec\2\u02eb\4\u02ec\1\u0341\4\u02ec"+
"\1\u02eb\4\u02ec\3\0\12\u02ec\1\u0341\7\u02ec\1\u02f2\2\u02eb"+
"\3\0\4\u02eb\1\u02ec\2\u02eb\1\u02ec\1\173\1\u033f\4\u02ec"+
"\1\u02eb\4\u02ec\1\0\1\150\2\u02ec\1\u02eb\1\u02f3\10\u02ec"+
"\5\u02eb\1\0\1\u02ec\1\u02eb\1\0\1\u02ec\2\150\2\u02eb"+
"\1\0\1\u02eb\1\150\1\0\1\u02eb\1\u02ec\1\u0342\3\u02ec"+
"\2\u02eb\11\u02ec\1\u02eb\4\u02ec\3\0\2\u02ec\1\u0342\17\u02ec"+
"\1\u02f2\2\u02eb\3\0\4\u02eb\1\u02ec\2\u02eb\1\u02ec\1\173"+
"\1\u033f\4\u02ec\1\u02eb\4\u02ec\1\0\1\150\2\u02ec\1\u02eb"+
"\1\u02f3\10\u02ec\5\u02eb\1\0\1\u02ec\1\u02eb\1\0\1\u02ec"+
"\2\150\2\u02eb\1\0\1\u02eb\1\150\1\0\1\u02eb\5\u02ec"+
"\2\u02eb\11\u02ec\1\u02eb\2\u02ec\1\u0343\1\u02ec\3\0\20\u02ec"+
"\1\u0343\1\u02ec\1\u02f2\2\u02eb\3\0\4\u02eb\1\u02ec\2\u02eb"+
"\1\u02ec\1\173\1\u033f\4\u02ec\1\u02eb\4\u02ec\1\0\1\150"+
"\2\u02ec\1\u02eb\1\u02f3\10\u02ec\5\u02eb\1\0\1\u02ec\1\u02eb"+
"\1\0\1\u02ec\2\150\2\u02eb\1\0\1\u02eb\1\150\1\0"+
"\1\u02eb\5\u0344\2\u02eb\11\u0344\1\u02eb\1\u0345\3\u0344\3\0"+
"\22\u0344\3\u02eb\3\0\4\u02eb\1\u0344\3\u02eb\1\150\1\u033e"+
"\4\u0344\1\u02eb\2\u0344\2\u0345\1\0\1\150\2\u0344\1\u02eb"+
"\1\u02f3\10\u0344\5\u02eb\1\0\1\u0345\1\u02eb\1\0\1\u0345"+
"\2\150\1\u02eb\1\u0346\1\0\1\u0346\1\u0170\1\0\26\u0346"+
"\3\0\25\u0346\3\0\10\u0346\1\u0170\1\u033e\11\u0346\1\0"+
"\1\u0170\21\u0346\1\0\2\u0346\1\0\1\u0346\2\u0170\1\u0346"+
"\1\146\1\0\1\147\1\150\1\0\1\151\5\152\1\150"+
"\1\146\4\152\1\u0190\4\152\1\146\1\160\3\152\3\0"+
"\1\164\11\152\1\u0190\7\152\1\165\1\150\1\146\3\0"+
"\1\166\1\146\1\150\1\167\1\164\1\170\1\171\1\172"+
"\1\173\1\u0150\4\152\1\214\2\152\2\160\1\0\1\150"+
"\2\152\1\150\1\176\10\152\5\146\1\0\1\160\1\146"+
"\1\0\1\160\2\150\2\146\1\0\1\147\1\150\1\0"+
"\1\306\5\152\1\150\1\146\4\152\1\u0190\4\152\1\146"+
"\1\160\3\152\3\0\1\307\11\276\1\u01cb\7\276\1\165"+
"\1\150\1\301\3\0\1\166\1\146\1\302\1\167\1\164"+
"\1\170\1\171\1\172\1\173\1\u0150\1\276\1\152\1\276"+
"\1\152\1\214\1\276\1\152\2\160\1\0\1\150\1\276"+
"\1\152\1\150\1\176\1\276\1\152\1\276\1\152\1\276"+
"\1\152\1\276\1\152\5\146\1\0\1\160\1\146\1\0"+
"\1\160\2\150\1\146\1\150\1\0\2\150\1\0\5\150"+
"\1\302\20\150\3\0\5\150\1\302\17\150\3\0\23\150"+
"\1\0\4\150\1\266\15\150\1\0\2\150\1\0\4\150"+
"\1\u0347\1\0\2\u0347\1\0\26\u0347\3\0\25\u0347\3\0"+
"\23\u0347\1\0\22\u0347\1\0\2\u0347\1\0\4\u0347\1\126"+
"\4\0\6\126\1\u01ff\13\126\1\0\3\126\3\0\22\126"+
"\2\0\1\126\3\0\2\126\1\0\5\126\1\0\1\127"+
"\4\126\1\0\2\126\4\0\2\126\1\0\16\126\2\0"+
"\1\126\4\0\2\126\4\0\6\126\1\0\13\126\1\0"+
"\1\126\1\u0291\1\126\3\0\20\126\1\u0291\1\126\2\0"+
"\1\126\3\0\2\126\1\0\5\126\1\0\1\127\4\126"+
"\1\0\2\126\4\0\2\126\1\0\16\126\2\0\1\126"+
"\4\0\1\126\6\0\2\u0348\1\0\1\u0348\4\0\1\u0349"+
"\1\u034a\1\0\1\u034b\1\0\1\u034c\3\0\1\u010b\1\0"+
"\1\u034d\1\u034b\3\0\1\u034e\2\u0348\1\0\1\u0348\2\0"+
"\1\u0349\1\u034a\1\0\1\u034b\1\0\1\u034c\3\0\1\u034d"+
"\1\u034b\12\0\1\u034e\14\0\1\u010f\1\u010b\24\0\1\u010f"+
"\2\0\1\u010f\10\0\1\u034f\5\u02fb\2\0\11\u02fb\2\0"+
"\3\u02fb\3\0\22\u02fb\11\0\2\u02fb\1\0\1\u0104\2\0"+
"\1\u0350\4\u02fb\1\u0104\2\u02fb\4\0\2\u02fb\2\0\10\u02fb"+
"\15\0\1\146\1\0\1\u02f7\1\150\1\0\6\146\1\150"+
"\13\146\1\150\3\146\3\0\22\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\1\146\1\150\1\0\2\150"+
"\1\0\1\150\5\u0351\2\150\11\u0351\2\150\3\u0351\3\0"+
"\22\u0351\3\150\3\0\4\150\1\u0351\5\150\4\u0351\1\150"+
"\2\u0351\2\150\1\0\1\150\2\u0351\1\150\1\266\10\u0351"+
"\5\150\1\0\2\150\1\0\5\150\1\0\2\150\1\0"+
"\1\150\5\u0352\2\150\11\u0352\2\150\3\u0352\3\0\22\u0352"+
"\3\150\3\0\4\150\1\u0352\5\150\4\u0352\1\150\2\u0352"+
"\2\150\1\0\1\150\2\u0352\1\150\1\266\10\u0352\5\150"+
"\1\0\2\150\1\0\4\150\1\146\1\0\2\150\1\0"+
"\6\146\1\150\2\146\1\u0353\10\146\1\150\3\146\3\0"+
"\7\146\1\u0353\12\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\6\146\1\214"+
"\13\146\1\150\3\146\3\0\22\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\6\146\1\u0354\13\146\1\150\3\146\3\0\22\146\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146"+
"\1\150\2\146\2\150\1\0\1\150\2\146\1\150\1\176"+
"\15\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\6\146\1\150\13\146\1\150\2\146\1\u0353"+
"\3\0\21\146\1\u0353\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\1\146\3\0\1\u0305\2\0\5\u0305\2\0"+
"\11\u0305\2\0\3\u0305\3\0\22\u0305\12\0\1\u0305\5\0"+
"\4\u0305\1\0\2\u0305\4\0\2\u0305\2\0\10\u0305\15\0"+
"\4\u0305\1\u02a1\137\u0305\1\146\1\0\2\150\1\0\4\146"+
"\1\u0355\1\146\1\150\13\146\1\150\3\146\3\0\4\146"+
"\1\u0355\15\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\1\146\1\u0166\1\u01da\1\u021d\1\u0166\1\u01da\1\u0166"+
"\5\u0307\2\u0166\11\u0307\1\u0166\1\u015c\3\u0307\3\u01da\22\u0307"+
"\1\u0224\1\u0166\1\150\3\u01da\1\u0220\3\u0166\1\u0307\1\u0221"+
"\3\u0166\1\150\4\u0307\1\u0166\2\u0307\2\u015c\1\u01da\1\u0166"+
"\2\u0307\1\u0166\1\u0223\10\u0307\5\u0166\1\u01da\1\u015c\1\u0166"+
"\1\u01da\1\u015c\4\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u02a4"+
"\2\u0166\11\u02a4\1\u0166\1\150\3\u02a4\3\u01da\22\u02a4\1\u02a6"+
"\1\u0166\1\150\3\u01da\4\u0166\1\u02a4\4\u0166\1\150\4\u02a4"+
"\1\u0166\2\u02a4\2\150\1\u01da\1\u0166\2\u02a4\1\u0166\1\u0223"+
"\10\u02a4\5\u0166\1\u01da\1\150\1\u0166\1\u01da\1\150\3\u0166"+
"\1\u016f\1\0\2\u0170\1\0\1\u016f\5\u0356\1\u0170\1\u016f"+
"\11\u0356\1\u016f\1\u0170\3\u0356\3\0\22\u0356\2\u0170\1\u016f"+
"\3\0\2\u016f\1\u0170\1\u016f\1\u0356\3\u016f\1\u0170\1\u022e"+
"\4\u0356\1\u0170\2\u0356\2\u0170\1\0\1\u0170\2\u0356\1\u0170"+
"\1\u016f\10\u0356\5\u016f\1\0\1\u0170\1\u016f\1\0\3\u0170"+
"\2\u016f\1\0\2\u0170\1\0\1\u016f\1\u0357\4\u0356\1\u0170"+
"\1\u016f\11\u0356\1\u016f\1\u0170\3\u0356\3\0\1\u0356\1\u0357"+
"\20\u0356\2\u0170\1\u016f\3\0\2\u016f\1\u0170\1\u016f\1\u0356"+
"\3\u016f\1\u0170\1\u022e\4\u0356\1\u0170\2\u0356\2\u0170\1\0"+
"\1\u0170\2\u0356\1\u0170\1\u016f\10\u0356\5\u016f\1\0\1\u0170"+
"\1\u016f\1\0\3\u0170\2\u016f\1\0\2\u0170\1\0\1\u016f"+
"\5\u0356\1\u0170\1\u016f\10\u0356\1\u0357\1\u016f\1\u0170\3\u0356"+
"\3\0\16\u0356\1\u0357\3\u0356\2\u0170\1\u016f\3\0\2\u016f"+
"\1\u0170\1\u016f\1\u0356\3\u016f\1\u0170\1\u022e\4\u0356\1\u0170"+
"\2\u0356\2\u0170\1\0\1\u0170\2\u0356\1\u0170\1\u016f\10\u0356"+
"\5\u016f\1\0\1\u0170\1\u016f\1\0\3\u0170\2\u016f\1\0"+
"\2\u0170\1\0\1\u016f\5\u0356\1\u0170\1\u016f\3\u0356\1\u0357"+
"\5\u0356\1\u016f\1\u0170\3\u0356\3\0\11\u0356\1\u0357\10\u0356"+
"\2\u0170\1\u016f\3\0\2\u016f\1\u0170\1\u016f\1\u0356\3\u016f"+
"\1\u0170\1\u022e\4\u0356\1\u0170\2\u0356\2\u0170\1\0\1\u0170"+
"\2\u0356\1\u0170\1\u016f\10\u0356\5\u016f\1\0\1\u0170\1\u016f"+
"\1\0\3\u0170\2\u016f\1\0\2\u0170\1\0\1\u016f\5\u0356"+
"\1\u0170\1\u016f\1\u0356\1\u0357\7\u0356\1\u016f\1\u0170\3\u0356"+
"\3\0\7\u0356\1\u0357\12\u0356\2\u0170\1\u016f\3\0\2\u016f"+
"\1\u0170\1\u016f\1\u0356\3\u016f\1\u0170\1\u022e\4\u0356\1\u0170"+
"\2\u0356\2\u0170\1\0\1\u0170\2\u0356\1\u0170\1\u016f\10\u0356"+
"\5\u016f\1\0\1\u0170\1\u016f\1\0\3\u0170\1\u016f\1\u0170"+
"\1\0\2\u0170\1\0\1\u0170\5\u0358\2\u0170\11\u0358\2\u0170"+
"\3\u0358\3\0\22\u0358\3\u0170\3\0\4\u0170\1\u0358\4\u0170"+
"\1\u022f\4\u0358\1\u0170\2\u0358\2\u0170\1\0\1\u0170\2\u0358"+
"\2\u0170\10\u0358\5\u0170\1\0\2\u0170\1\0\4\u0170\1\146"+
"\1\0\2\150\1\0\6\146\1\u0359\13\146\1\150\3\146"+
"\3\0\22\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\1\147\1\150\1\0\1\u035a\5\152"+
"\1\150\1\146\11\152\1\146\1\160\3\152\3\0\1\303"+
"\21\152\1\165\1\150\1\146\3\0\1\166\1\146\1\150"+
"\1\167\1\303\1\170\1\u035b\1\172\1\173\1\u0150\4\152"+
"\1\u035c\2\152\2\160\1\0\1\150\2\152\1\150\1\176"+
"\10\152\5\146\1\0\1\160\1\146\1\0\1\160\2\150"+
"\1\146\1\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u0177\2\u0166"+
"\7\u0177\1\u035d\1\u0177\1\u0166\4\u0177\3\u01da\15\u0177\1\u035d"+
"\4\u0177\1\u0232\1\u0166\1\150\3\u01da\4\u0166\1\u0177\1\u0232"+
"\3\u0166\1\150\4\u0177\1\u0166\4\u0177\1\u01da\1\u0166\2\u0177"+
"\1\u0166\1\u0223\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166\1\u01da"+
"\1\u0177\3\u0166\1\150\1\0\2\150\1\0\26\150\3\0"+
"\25\150\3\0\22\150\1\302\1\0\4\150\1\266\15\150"+
"\1\0\2\150\1\0\4\150\1\146\1\0\2\150\1\0"+
"\6\146\1\150\10\146\1\u035e\2\146\1\150\3\146\3\0"+
"\15\146\1\u035e\4\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\6\146\1\150"+
"\13\146\1\150\1\146\1\u0238\1\146\3\0\20\146\1\u0238"+
"\1\146\2\150\1\146\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150\2\146"+
"\1\150\1\176\15\146\1\0\1\150\1\146\1\0\3\150"+
"\1\146\1\150\1\0\2\150\1\0\24\150\1\302\1\150"+
"\3\0\20\150\1\302\4\150\3\0\23\150\1\0\4\150"+
"\1\266\15\150\1\0\2\150\1\0\5\150\1\0\1\u02f7"+
"\1\150\1\0\26\150\3\0\25\150\3\0\23\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\4\150\1\u0166"+
"\1\u01da\2\u0166\1\u01da\1\u0166\5\u0226\2\u0166\11\u0226\1\u0166"+
"\4\u0226\3\u01da\22\u0226\1\u02a6\1\u0166\1\150\3\u01da\4\u0166"+
"\1\u0226\4\u0166\1\u035f\4\u0226\1\u0166\4\u0226\1\u01da\1\u0166"+
"\2\u0226\1\u0166\1\u0223\10\u0226\5\u0166\1\u01da\1\u0226\1\u0166"+
"\1\u01da\1\u0226\3\u0166\1\146\1\0\2\150\1\0\6\146"+
"\1\244\13\146\1\150\3\146\3\0\22\146\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\1\146\1\150\1\0"+
"\2\150\1\0\26\150\3\0\25\150\3\0\11\150\1\302"+
"\11\150\1\0\4\150\1\266\15\150\1\0\2\150\1\0"+
"\4\150\1\146\1\0\2\150\1\0\6\146\1\252\13\146"+
"\1\150\3\146\3\0\22\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\4\146"+
"\1\u0360\1\146\1\150\13\146\1\150\3\146\3\0\4\146"+
"\1\u0360\15\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\6\146\1\150\13\146"+
"\1\150\3\146\3\0\22\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\u0238\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\1\146\1\150\1\0\2\150\1\0"+
"\26\150\3\0\25\150\3\0\17\150\2\302\2\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\5\150\1\0"+
"\2\150\1\0\1\150\5\u0299\2\150\11\u0299\1\150\1\u0361"+
"\3\u0299\3\0\22\u0299\1\u02fe\2\150\3\0\4\150\1\u0299"+
"\5\150\4\u0299\1\150\2\u0299\2\u0361\1\0\1\150\2\u0299"+
"\1\150\1\266\10\u0299\5\150\1\0\1\u0361\1\150\1\0"+
"\1\u0361\4\150\1\0\2\150\1\0\22\150\1\u0362\3\150"+
"\3\0\25\150\3\0\21\150\2\u0362\1\0\4\150\1\266"+
"\15\150\1\0\1\u0362\1\150\1\0\1\u0362\4\150\1\0"+
"\2\150\1\0\22\150\1\u0363\3\150\3\0\25\150\3\0"+
"\21\150\2\u0363\1\0\4\150\1\266\15\150\1\0\1\u0363"+
"\1\150\1\0\1\u0363\4\150\1\0\2\150\1\0\26\150"+
"\3\u0227\25\150\2\0\1\u0227\11\150\1\u0364\11\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\5\150\1\0"+
"\2\150\1\0\26\150\3\0\22\u0365\3\150\3\0\12\150"+
"\1\u0365\1\150\1\u0365\2\150\1\u0365\3\150\1\0\1\150"+
"\1\u0365\2\150\1\266\1\u0365\1\150\1\u0365\1\150\1\u0365"+
"\1\150\1\u0365\6\150\1\0\2\150\1\0\4\150\1\146"+
"\1\0\2\150\1\0\6\146\1\u0366\13\146\1\150\3\146"+
"\3\0\22\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\1\u0259\5\146\1\150"+
"\2\146\1\u0353\10\146\1\150\3\146\3\0\7\u01a3\1\u0367"+
"\12\u01a3\2\150\1\301\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146"+
"\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\1\u0259\5\146\1\u0366\13\146\1\150\3\146\3\0\22\u01a3"+
"\2\150\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\5\146\1\u0354\13\146\1\150\3\146\3\0\22\u01a3\2\150"+
"\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3"+
"\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0"+
"\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259\5\146"+
"\1\150\13\146\1\150\2\146\1\u0353\3\0\21\u01a3\1\u0367"+
"\2\150\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\5\146\1\214\13\146\1\150\3\146\3\0\22\u01a3\2\150"+
"\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3"+
"\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0"+
"\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259\3\146"+
"\1\u0355\1\146\1\150\13\146\1\150\3\146\3\0\4\u01a3"+
"\1\u0368\15\u01a3\2\150\1\301\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3"+
"\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146"+
"\1\0\1\150\1\146\1\0\3\150\2\146\1\0\2\150"+
"\1\0\1\u0259\5\146\1\u0359\13\146\1\150\3\146\3\0"+
"\22\u01a3\2\150\1\301\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146"+
"\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\1\147\1\150"+
"\1\0\1\u0369\5\152\1\150\1\146\11\152\1\146\1\160"+
"\3\152\3\0\1\275\21\276\1\165\1\150\1\301\3\0"+
"\1\166\1\146\1\302\1\167\1\303\1\170\1\u035b\1\172"+
"\1\173\1\u0150\1\276\1\152\1\276\1\152\1\u035c\1\276"+
"\1\152\2\160\1\0\1\150\1\276\1\152\1\150\1\176"+
"\1\276\1\152\1\276\1\152\1\276\1\152\1\276\1\152"+
"\5\146\1\0\1\160\1\146\1\0\1\160\2\150\2\146"+
"\1\0\2\150\1\0\1\u0259\5\146\1\150\10\146\1\u035e"+
"\2\146\1\150\3\146\3\0\15\u01a3\1\u036a\4\u01a3\2\150"+
"\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3"+
"\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0"+
"\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259\5\146"+
"\1\244\13\146\1\150\3\146\3\0\22\u01a3\2\150\1\301"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3\1\146"+
"\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0\1\150"+
"\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\6\146\1\362\13\146"+
"\1\150\3\146\3\0\22\146\2\150\1\146\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150"+
"\1\0\1\150\2\146\1\150\1\176\15\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\5\146\1\362\13\146\1\150\3\146\3\0\22\u01a3\2\150"+
"\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3"+
"\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0"+
"\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259\3\146"+
"\1\u0360\1\146\1\150\13\146\1\150\3\146\3\0\4\u01a3"+
"\1\u036b\15\u01a3\2\150\1\301\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3"+
"\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146"+
"\1\0\1\150\1\146\1\0\3\150\1\146\1\u0160\1\u01da"+
"\2\u0166\1\u01da\1\u0160\5\u0174\1\u0166\1\u0160\11\u0174\1\u0160"+
"\1\u0177\3\u0174\3\u01da\22\u0174\1\u0232\1\u0166\1\146\3\u01da"+
"\2\u0160\1\u0166\1\u0160\1\u0174\1\u0233\2\u0160\1\u0166\1\u014c"+
"\4\u0174\1\u0166\2\u036c\2\u0177\1\u01da\1\u0166\2\u0174\1\u0166"+
"\1\u0234\10\u0174\5\u0160\1\u01da\1\u0177\1\u0160\1\u01da\1\u0177"+
"\2\u0166\1\u0160\1\u0166\1\u01da\2\u0166\1\u01da\1\u0166\5\u0177"+
"\2\u0166\11\u0177\1\u0166\4\u0177\3\u01da\22\u0177\1\u0232\1\u0166"+
"\1\150\3\u01da\4\u0166\1\u0177\1\u0232\3\u0166\1\150\4\u0177"+
"\1\u0166\2\u035d\2\u0177\1\u01da\1\u0166\2\u0177\1\u0166\1\u0223"+
"\10\u0177\5\u0166\1\u01da\1\u0177\1\u0166\1\u01da\1\u0177\3\u0166"+
"\27\0\1\u036d\57\0\2\u036d\24\0\1\u036d\2\0\1\u036d"+
"\32\0\1\u0337\57\0\2\u0337\24\0\1\u0337\2\0\1\u0337"+
"\3\0\1\126\4\0\6\126\1\u0104\13\126\1\0\3\126"+
"\3\0\22\126\2\0\1\126\3\0\2\126\1\0\5\126"+
"\1\0\1\127\4\126\1\0\2\126\4\0\2\126\1\0"+
"\16\126\2\0\1\126\4\0\2\126\4\0\4\126\1\u0147"+
"\1\126\1\0\13\126\1\0\3\126\3\0\4\126\1\u0147"+
"\15\126\2\0\1\126\3\0\2\126\1\0\5\126\1\0"+
"\1\127\4\126\1\0\2\126\4\0\2\126\1\0\16\126"+
"\2\0\1\126\4\0\1\126\77\0\1\u036e\55\0\1\u036f"+
"\30\0\1\u036f\101\0\1\u02eb\1\0\1\u02eb\1\150\1\0"+
"\1\u02eb\5\u0370\2\u02eb\11\u0370\2\u02eb\3\u0370\3\0\22\u0370"+
"\3\u02eb\3\0\4\u02eb\1\u0370\3\u02eb\2\150\4\u0370\1\u02eb"+
"\2\u0370\2\u02eb\1\0\1\150\2\u0370\1\u02eb\1\u02f3\10\u0370"+
"\5\u02eb\1\0\2\u02eb\1\0\1\u02eb\2\150\2\u02eb\1\0"+
"\1\u02eb\1\150\1\0\1\u02eb\5\u0371\2\u02eb\11\u0371\1\u02eb"+
"\1\u02ec\3\u0371\3\0\22\u0371\1\u02f2\2\u02eb\3\0\4\u02eb"+
"\1\u0371\2\u02eb\1\u02ec\2\173\4\u0371\1\u02eb\2\u0371\2\u02ec"+
"\1\0\1\150\2\u0371\1\u02eb\1\u02f3\10\u0371\5\u02eb\1\0"+
"\1\u02ec\1\u02eb\1\0\1\u02ec\2\150\2\u02eb\1\0\1\u02eb"+
"\1\150\1\0\1\u02eb\1\u0372\4\u02ec\2\u02eb\11\u02ec\1\u02eb"+
"\4\u02ec\3\0\1\u02ec\1\u0372\20\u02ec\1\u02f2\2\u02eb\3\0"+
"\4\u02eb\1\u02ec\2\u02eb\1\u02ec\1\173\1\u033f\4\u02ec\1\u02eb"+
"\4\u02ec\1\0\1\150\2\u02ec\1\u02eb\1\u02f3\10\u02ec\5\u02eb"+
"\1\0\1\u02ec\1\u02eb\1\0\1\u02ec\2\150\2\u02eb\1\0"+
"\1\u02eb\1\150\1\0\1\u02eb\5\u02ec\2\u02eb\10\u02ec\1\u0372"+
"\1\u02eb\4\u02ec\3\0\16\u02ec\1\u0372\3\u02ec\1\u02f2\2\u02eb"+
"\3\0\4\u02eb\1\u02ec\2\u02eb\1\u02ec\1\173\1\u033f\4\u02ec"+
"\1\u02eb\4\u02ec\1\0\1\150\2\u02ec\1\u02eb\1\u02f3\10\u02ec"+
"\5\u02eb\1\0\1\u02ec\1\u02eb\1\0\1\u02ec\2\150\2\u02eb"+
"\1\0\1\u02eb\1\150\1\0\1\u02eb\5\u02ec\2\u02eb\3\u02ec"+
"\1\u0372\5\u02ec\1\u02eb\4\u02ec\3\0\11\u02ec\1\u0372\10\u02ec"+
"\1\u02f2\2\u02eb\3\0\4\u02eb\1\u02ec\2\u02eb\1\u02ec\1\173"+
"\1\u033f\4\u02ec\1\u02eb\4\u02ec\1\0\1\150\2\u02ec\1\u02eb"+
"\1\u02f3\10\u02ec\5\u02eb\1\0\1\u02ec\1\u02eb\1\0\1\u02ec"+
"\2\150\2\u02eb\1\0\1\u02eb\1\150\1\0\1\u02eb\5\u02ec"+
"\2\u02eb\1\u02ec\1\u0372\7\u02ec\1\u02eb\4\u02ec\3\0\7\u02ec"+
"\1\u0372\12\u02ec\1\u02f2\2\u02eb\3\0\4\u02eb\1\u02ec\2\u02eb"+
"\1\u02ec\1\173\1\u033f\4\u02ec\1\u02eb\4\u02ec\1\0\1\150"+
"\2\u02ec\1\u02eb\1\u02f3\10\u02ec\5\u02eb\1\0\1\u02ec\1\u02eb"+
"\1\0\1\u02ec\2\150\1\u02eb\1\u02ed\1\u01da\1\u02ed\1\u0166"+
"\1\u01da\1\u02ed\5\u0345\2\u02ed\11\u0345\1\u02ed\4\u0345\3\u01da"+
"\22\u0345\1\u0373\1\u02ed\1\u02eb\3\u01da\4\u02ed\1\u0345\3\u02ed"+
"\1\u0166\1\u0374\4\u0345\1\u02ed\4\u0345\1\u01da\1\u0166\2\u0345"+
"\1\u02ed\1\u0375\10\u0345\5\u02ed\1\u01da\1\u0345\1\u02ed\1\u01da"+
"\1\u0345\2\u0166\2\u02ed\1\u01da\1\u02ed\1\u0166\1\u01da\1\u02ed"+
"\5\u0345\2\u02ed\11\u0345\1\u02ed\4\u0345\3\u01da\22\u0345\1\u0373"+
"\1\u02ed\1\u02eb\3\u01da\4\u02ed\1\u0345\3\u02ed\1\u0166\1\u033e"+
"\4\u0345\1\u02ed\4\u0345\1\u01da\1\u0166\2\u0345\1\u02ed\1\u0375"+
"\10\u0345\5\u02ed\1\u01da\1\u0345\1\u02ed\1\u01da\1\u0345\2\u0166"+
"\1\u02ed\1\u0346\1\0\1\u0346\1\u0170\1\0\26\u0346\3\0"+
"\25\u0346\3\0\10\u0346\1\u0170\1\u0376\11\u0346\1\0\1\u0170"+
"\21\u0346\1\0\2\u0346\1\0\1\u0346\2\u0170\1\u0346\1\u0377"+
"\1\0\1\u0377\1\u0347\1\0\26\u0377\3\0\22\u0377\1\u0347"+
"\2\u0377\3\0\10\u0377\2\u0347\11\u0377\1\0\1\u0347\21\u0377"+
"\1\0\2\u0377\1\0\1\u0377\2\u0347\1\u0377\30\0\1\u0378"+
"\24\0\1\u0378\105\0\1\u0348\26\0\1\u0348\126\0\1\u0348"+
"\24\0\1\u0348\75\0\1\u0379\30\0\1\u0379\110\0\1\5"+
"\30\0\1\5\111\0\1\u037a\66\0\1\5\7\0\1\5"+
"\47\0\1\u037b\7\0\1\u037c\3\0\1\u037c\3\0\2\u037c"+
"\7\0\1\u037b\5\0\1\u037c\3\0\1\u037c\1\0\2\u037c"+
"\100\0\1\u01da\45\0\1\u01da\14\0\1\u01da\45\0\1\150"+
"\1\0\2\150\1\0\1\150\5\u0351\2\150\11\u0351\2\150"+
"\3\u0351\3\0\22\u0351\1\u037d\2\150\3\0\4\150\1\u0351"+
"\5\150\4\u0351\1\150\2\u0351\2\150\1\0\1\150\2\u0351"+
"\1\150\1\266\10\u0351\5\150\1\0\2\150\1\0\5\150"+
"\1\0\1\u020b\1\150\1\0\1\150\5\u0352\2\150\11\u0352"+
"\2\150\3\u0352\3\0\22\u0352\3\150\3\0\1\u020d\3\150"+
"\1\u0352\5\150\4\u0352\1\150\2\u0352\2\150\1\0\1\150"+
"\2\u0352\1\150\1\266\10\u0352\5\150\1\0\2\150\1\0"+
"\4\150\1\146\1\0\2\150\1\0\6\146\1\150\13\146"+
"\1\150\1\146\1\u0302\1\146\3\0\20\146\1\u0302\1\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\1\146"+
"\1\150\1\0\2\150\1\0\1\u037e\5\u0354\2\150\11\u0354"+
"\2\150\3\u0354\3\0\22\u0354\3\150\3\0\3\150\2\u0354"+
"\1\150\1\214\2\150\1\u037f\4\u0354\1\214\2\u0354\2\150"+
"\1\0\1\150\2\u0354\1\150\1\266\10\u0354\5\150\1\0"+
"\2\150\1\0\4\150\1\146\1\0\2\150\1\0\6\146"+
"\1\u015a\13\146\1\150\3\146\3\0\22\146\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\1\146\1\u016f\1\0"+
"\2\u0170\1\0\1\u016f\5\u0380\1\u0170\1\u016f\11\u0380\1\u016f"+
"\1\u0170\3\u0380\3\0\22\u0380\2\u0170\1\u016f\3\0\2\u016f"+
"\1\u0170\1\u016f\1\u0380\3\u016f\1\u0170\1\u022e\4\u0380\1\u0170"+
"\2\u0380\2\u0170\1\0\1\u0170\2\u0380\1\u0170\1\u016f\10\u0380"+
"\5\u016f\1\0\1\u0170\1\u016f\1\0\3\u0170\2\u016f\1\0"+
"\1\u02f7\1\u0170\1\0\1\u016f\5\u0380\1\u0170\1\u016f\11\u0380"+
"\1\u016f\1\u0170\3\u0380\3\0\22\u0380\2\u0170\1\u016f\3\0"+
"\2\u016f\1\u0170\1\u016f\1\u0380\3\u016f\1\u0170\1\u022e\4\u0380"+
"\1\u0170\2\u0380\2\u0170\1\0\1\u0170\2\u0380\1\u0170\1\u016f"+
"\10\u0380\5\u016f\1\0\1\u0170\1\u016f\1\0\3\u0170\1\u016f"+
"\1\u0170\1\0\2\u0170\1\0\1\u0170\5\u0381\2\u0170\11\u0381"+
"\2\u0170\3\u0381\3\0\22\u0381\3\u0170\3\0\4\u0170\1\u0381"+
"\4\u0170\1\u022f\4\u0381\1\u0170\2\u0381\2\u0170\1\0\1\u0170"+
"\2\u0381\2\u0170\10\u0381\5\u0170\1\0\2\u0170\1\0\4\u0170"+
"\1\146\1\0\2\150\1\0\3\146\1\u0382\2\146\1\150"+
"\4\146\1\u014f\3\146\1\u014f\2\146\1\150\2\u014f\1\146"+
"\3\0\3\146\1\u0382\5\146\1\u014f\3\146\1\u014f\1\146"+
"\2\u014f\1\146\2\150\1\146\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0\1\150"+
"\2\146\1\150\1\176\15\146\1\0\1\150\1\146\1\0"+
"\3\150\2\146\1\0\2\150\1\0\4\146\1\u0383\1\146"+
"\1\150\13\146\1\150\3\146\3\0\4\146\1\u0383\15\146"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\4\146\1\150\2\146\2\150\1\0\1\150\2\146\1\150"+
"\1\176\15\146\1\0\1\150\1\146\1\0\3\150\1\146"+
"\1\150\1\0\2\150\1\0\1\150\3\u02a4\1\u0384\1\u02a4"+
"\2\150\11\u02a4\2\150\3\u02a4\3\0\4\u02a4\1\u0384\15\u02a4"+
"\3\150\3\0\4\150\1\u02a4\5\150\4\u02a4\1\150\2\u0324"+
"\2\150\1\0\1\150\2\u02a4\1\150\1\266\10\u02a4\5\150"+
"\1\0\2\150\1\0\4\150\1\146\1\0\2\150\1\0"+
"\6\146\1\u0118\13\146\1\150\3\146\3\0\22\146\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146"+
"\1\150\2\146\2\150\1\0\1\150\2\146\1\150\1\176"+
"\15\146\1\0\1\150\1\146\1\0\3\150\1\146\1\150"+
"\1\0\2\150\1\0\1\150\5\u0299\2\150\11\u0299\1\150"+
"\1\u0385\3\u0299\3\0\22\u0299\1\u02fe\2\150\3\0\4\150"+
"\1\u0299\5\150\4\u0299\1\150\2\u0299\2\u0385\1\0\1\150"+
"\2\u0299\1\150\1\266\10\u0299\5\150\1\0\1\u0385\1\150"+
"\1\0\1\u0385\4\150\1\0\2\150\1\0\22\150\1\302"+
"\3\150\3\0\25\150\3\0\21\150\2\302\1\0\4\150"+
"\1\266\15\150\1\0\1\302\1\150\1\0\1\302\4\150"+
"\1\0\2\150\1\0\22\150\1\u0386\3\150\3\0\25\150"+
"\3\0\21\150\2\u0386\1\0\4\150\1\266\15\150\1\0"+
"\1\u0386\1\150\1\0\1\u0386\4\150\1\0\2\150\1\0"+
"\1\u0387\25\150\3\0\22\u0365\2\150\1\u0325\3\0\12\150"+
"\1\u0365\1\150\1\u0365\2\150\1\u0365\3\150\1\0\1\150"+
"\1\u0365\2\150\1\266\1\u0365\1\150\1\u0365\1\150\1\u0365"+
"\1\150\1\u0365\6\150\1\0\2\150\1\0\5\150\1\0"+
"\2\150\1\0\1\150\2\u0166\1\150\1\u0166\4\150\1\u01a9"+
"\1\u016c\1\150\1\u016d\10\150\1\u016d\3\0\1\150\2\u0166"+
"\1\150\1\u0166\2\150\1\u01a9\1\u016c\1\150\1\u016d\6\150"+
"\1\u016d\3\150\3\0\23\150\1\0\4\150\1\266\15\150"+
"\1\0\2\150\1\0\4\150\1\146\1\0\2\150\1\0"+
"\1\u0259\5\146\1\150\13\146\1\150\1\146\1\u0302\1\146"+
"\3\0\20\u01a3\1\u0329\1\u01a3\2\150\1\301\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146"+
"\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146"+
"\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150\2\146"+
"\1\0\2\150\1\0\1\u0259\5\146\1\u015a\13\146\1\150"+
"\3\146\3\0\22\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\3\146\1\u0388\2\146\1\150\4\146\1\u014f"+
"\3\146\1\u014f\2\146\1\150\2\u014f\1\146\3\0\3\u01a3"+
"\1\u0389\5\u01a3\1\u01a5\3\u01a3\1\u01a5\1\u01a3\2\u01a5\1\u01a3"+
"\2\150\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259"+
"\3\146\1\u0383\1\146\1\150\13\146\1\150\3\146\3\0"+
"\4\u01a3\1\u038a\15\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\2\146\1\0"+
"\2\150\1\0\1\u0259\5\146\1\u0118\13\146\1\150\3\146"+
"\3\0\22\u01a3\2\150\1\301\3\0\2\146\1\150\5\146"+
"\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3"+
"\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146"+
"\1\0\1\150\1\146\1\0\3\150\1\146\27\0\1\u038b"+
"\57\0\2\u038b\24\0\1\u038b\2\0\1\u038b\70\0\1\u038c"+
"\71\0\1\5\130\0\1\u02eb\1\0\1\u02eb\1\150\1\0"+
"\1\u02eb\5\u038d\2\u02eb\11\u038d\2\u02eb\3\u038d\3\0\22\u038d"+
"\3\u02eb\3\0\4\u02eb\1\u038d\3\u02eb\1\150\1\u033e\4\u038d"+
"\1\u02eb\2\u038d\2\u02eb\1\0\1\150\2\u038d\1\u02eb\1\u02f3"+
"\10\u038d\5\u02eb\1\0\2\u02eb\1\0\1\u02eb\2\150\2\u02eb"+
"\1\0\1\u02eb\1\150\1\0\1\u02eb\5\u038e\2\u02eb\11\u038e"+
"\1\u02eb\1\u02ec\3\u038e\3\0\22\u038e\1\u02f2\2\u02eb\3\0"+
"\4\u02eb\1\u038e\2\u02eb\1\u02ec\1\173\1\u033f\4\u038e\1\u02eb"+
"\2\u038e\2\u02ec\1\0\1\150\2\u038e\1\u02eb\1\u02f3\10\u038e"+
"\5\u02eb\1\0\1\u02ec\1\u02eb\1\0\1\u02ec\2\150\2\u02eb"+
"\1\0\1\u02f7\1\150\1\0\1\u02eb\5\u02ec\2\u02eb\11\u02ec"+
"\1\u02eb\4\u02ec\3\0\22\u02ec\1\u02f2\2\u02eb\3\0\4\u02eb"+
"\1\u02ec\2\u02eb\1\u02ec\1\173\1\u033f\4\u02ec\1\u02eb\4\u02ec"+
"\1\0\1\150\2\u02ec\1\u02eb\1\u02f3\10\u02ec\5\u02eb\1\0"+
"\1\u02ec\1\u02eb\1\0\1\u02ec\2\150\2\u02eb\1\0\1\u02eb"+
"\1\150\1\0\1\u02eb\5\u038f\2\u02eb\11\u038f\2\u02eb\3\u038f"+
"\3\0\22\u038f\3\u02eb\3\0\4\u02eb\1\u038f\3\u02eb\2\150"+
"\4\u038f\1\u02eb\2\u038f\2\u02eb\1\0\1\150\2\u038f\1\u02eb"+
"\1\u02f3\10\u038f\5\u02eb\1\0\2\u02eb\1\0\1\u02eb\2\150"+
"\1\u02eb\1\u0346\1\0\1\u0346\1\u0170\1\0\1\u0346\5\u0390"+
"\2\u0346\11\u0390\2\u0346\3\u0390\3\0\22\u0390\3\u0346\3\0"+
"\4\u0346\1\u0390\3\u0346\1\u0170\1\150\4\u0390\1\u0346\2\u0390"+
"\2\u0346\1\0\1\u0170\2\u0390\2\u0346\10\u0390\5\u0346\1\0"+
"\2\u0346\1\0\1\u0346\2\u0170\1\u0346\10\0\1\u0391\4\0"+
"\1\u0392\2\0\1\u0393\4\0\1\u0394\13\0\1\u0391\2\0"+
"\1\u0392\2\0\1\u0393\4\0\1\u0394\77\0\1\u0391\7\0"+
"\1\u0393\4\0\1\u0394\13\0\1\u0391\5\0\1\u0393\4\0"+
"\1\u0394\67\0\1\150\1\0\2\150\1\0\1\150\5\u0395"+
"\2\150\11\u0395\2\150\3\u0395\3\0\22\u0395\3\150\3\0"+
"\4\150\1\u0395\5\150\4\u0395\1\150\2\u0395\2\150\1\0"+
"\1\150\2\u0395\1\150\1\266\10\u0395\5\150\1\0\2\150"+
"\1\0\5\150\1\0\2\150\1\0\3\150\1\u0396\7\150"+
"\1\u0397\3\150\1\u0397\3\150\2\u0397\1\150\3\0\3\150"+
"\1\u0396\5\150\1\u0397\3\150\1\u0397\1\150\2\u0397\4\150"+
"\3\0\23\150\1\0\4\150\1\266\15\150\1\0\2\150"+
"\1\0\5\150\1\0\2\150\1\0\6\150\1\u0166\17\150"+
"\3\0\23\150\1\u0166\1\150\3\0\10\150\1\u0166\12\150"+
"\1\0\4\150\1\266\15\150\1\0\2\150\1\0\4\150"+
"\1\146\1\0\2\150\1\0\3\146\1\u020e\2\146\1\150"+
"\1\146\1\u0398\2\146\1\u0210\4\146\1\u0211\1\146\1\150"+
"\3\146\3\0\3\146\1\u020e\2\146\1\u0398\2\146\1\u0210"+
"\4\146\1\u0211\3\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\6\146\1\u023b"+
"\13\146\1\150\3\146\3\0\22\146\2\150\1\146\3\0"+
"\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150\2\146"+
"\2\150\1\0\1\150\2\146\1\150\1\176\15\146\1\0"+
"\1\150\1\146\1\0\3\150\1\146\1\150\1\0\2\150"+
"\1\0\26\150\3\0\25\150\3\0\11\150\1\u0399\11\150"+
"\1\0\4\150\1\266\15\150\1\0\2\150\1\0\5\150"+
"\1\0\2\150\1\0\3\150\1\u039a\22\150\3\0\3\u0365"+
"\1\u039b\16\u0365\3\150\3\0\12\150\1\u0365\1\150\1\u0365"+
"\2\150\1\u0365\3\150\1\0\1\150\1\u0365\2\150\1\266"+
"\1\u0365\1\150\1\u0365\1\150\1\u0365\1\150\1\u0365\6\150"+
"\1\0\2\150\1\0\4\150\1\146\1\0\2\150\1\0"+
"\1\146\1\u0257\1\146\1\u020e\2\146\1\150\1\146\1\u0398"+
"\2\146\1\u0210\4\146\1\u0211\1\146\1\150\3\146\3\0"+
"\1\146\1\u0257\1\146\1\u020e\2\146\1\u0398\2\146\1\u0210"+
"\4\146\1\u0211\3\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\2\146\1\0\2\150\1\0\1\u0259\1\u0257"+
"\1\146\1\u020e\2\146\1\150\1\146\1\u0398\2\146\1\u0210"+
"\4\146\1\u0211\1\146\1\150\3\146\3\0\1\u01a3\1\u025a"+
"\1\u01a3\1\u025b\2\u01a3\1\u039c\2\u01a3\1\u025d\4\u01a3\1\u025e"+
"\3\u01a3\2\150\1\301\3\0\2\146\1\150\5\146\1\150"+
"\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146"+
"\2\150\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0"+
"\1\150\1\146\1\0\3\150\2\146\1\0\2\150\1\0"+
"\1\u0259\5\146\1\u023b\13\146\1\150\3\146\3\0\22\u01a3"+
"\2\150\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c"+
"\1\u01a3\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150"+
"\1\0\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146"+
"\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150"+
"\1\146\1\0\3\150\1\146\60\0\1\u039d\4\0\1\u039d"+
"\56\0\1\u02eb\1\0\1\u02f7\1\150\1\0\1\u02eb\5\u039e"+
"\2\u02eb\11\u039e\2\u02eb\3\u039e\3\0\22\u039e\3\u02eb\3\0"+
"\4\u02eb\1\u039e\3\u02eb\1\150\1\u033e\4\u039e\1\u02eb\2\u039e"+
"\2\u02eb\1\0\1\150\2\u039e\1\u02eb\1\u02f3\10\u039e\5\u02eb"+
"\1\0\2\u02eb\1\0\1\u02eb\2\150\2\u02eb\1\0\1\u02f7"+
"\1\150\1\0\1\u02eb\5\u039f\2\u02eb\11\u039f\1\u02eb\1\u02ec"+
"\3\u039f\3\0\22\u039f\1\u02f2\2\u02eb\3\0\4\u02eb\1\u039f"+
"\2\u02eb\1\u02ec\1\173\1\u033f\4\u039f\1\u02eb\2\u039f\2\u02ec"+
"\1\0\1\150\2\u039f\1\u02eb\1\u02f3\10\u039f\5\u02eb\1\0"+
"\1\u02ec\1\u02eb\1\0\1\u02ec\2\150\2\u02eb\1\0\1\u02eb"+
"\1\150\1\0\1\u02eb\5\u038d\2\u02eb\11\u038d\2\u02eb\3\u038d"+
"\3\0\22\u038d\3\u02eb\3\0\4\u02eb\1\u038d\3\u02eb\1\150"+
"\1\u03a0\4\u038d\1\u02eb\2\u038d\2\u02eb\1\0\1\150\2\u038d"+
"\1\u02eb\1\u02f3\10\u038d\5\u02eb\1\0\2\u02eb\1\0\1\u02eb"+
"\2\150\1\u02eb\1\u0346\1\0\1\u0346\1\u0170\1\0\1\u0346"+
"\5\u03a1\2\u0346\11\u03a1\2\u0346\3\u03a1\3\0\22\u03a1\3\u0346"+
"\3\0\4\u0346\1\u03a1\3\u0346\1\u0170\1\u0376\4\u03a1\1\u0346"+
"\2\u03a1\2\u0346\1\0\1\u0170\2\u03a1\2\u0346\10\u03a1\5\u0346"+
"\1\0\2\u0346\1\0\1\u0346\2\u0170\1\u0346\23\0\1\u03a2"+
"\26\0\1\u03a2\115\0\1\u03a3\26\0\1\u03a3\76\0\1\u03a4"+
"\30\0\1\u03a4\125\0\1\u03a5\26\0\1\u03a5\73\0\1\150"+
"\1\0\2\150\1\0\3\150\1\u03a6\4\150\1\u03a7\2\150"+
"\1\u03a8\4\150\1\u03a9\5\150\3\0\3\150\1\u03a6\2\150"+
"\1\u03a7\2\150\1\u03a8\4\150\1\u03a9\6\150\3\0\23\150"+
"\1\0\4\150\1\266\15\150\1\0\2\150\1\0\5\150"+
"\1\0\2\150\1\0\3\150\1\u03a6\7\150\1\u03a8\4\150"+
"\1\u03a9\5\150\3\0\3\150\1\u03a6\5\150\1\u03a8\4\150"+
"\1\u03a9\6\150\3\0\23\150\1\0\4\150\1\266\15\150"+
"\1\0\2\150\1\0\4\150\1\146\1\0\2\150\1\0"+
"\6\146\1\150\10\146\1\u03aa\2\146\1\150\3\146\3\0"+
"\15\146\1\u03aa\4\146\2\150\1\146\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\4\146\1\150\2\146\2\150\1\0"+
"\1\150\2\146\1\150\1\176\15\146\1\0\1\150\1\146"+
"\1\0\3\150\1\146\1\u0166\1\u01da\2\u0166\1\u01da\1\u0166"+
"\3\u02a4\1\u03ab\1\u02a4\2\u0166\11\u02a4\1\u0166\1\150\3\u02a4"+
"\3\u01da\4\u02a4\1\u03ab\15\u02a4\1\u02a6\1\u0166\1\150\3\u01da"+
"\4\u0166\1\u02a4\4\u0166\1\150\4\u02a4\1\u0166\2\u02a4\2\150"+
"\1\u01da\1\u0166\2\u02a4\1\u0166\1\u0223\10\u02a4\5\u0166\1\u01da"+
"\1\150\1\u0166\1\u01da\1\150\3\u0166\1\150\1\0\2\150"+
"\1\0\1\150\1\u03ac\24\150\3\0\1\150\1\u03ac\23\150"+
"\3\0\23\150\1\0\4\150\1\266\15\150\1\0\2\150"+
"\1\0\5\150\1\0\2\150\1\0\1\u0387\1\u03ac\24\150"+
"\3\0\1\u0365\1\u03ad\20\u0365\2\150\1\u0325\3\0\12\150"+
"\1\u0365\1\150\1\u0365\2\150\1\u0365\3\150\1\0\1\150"+
"\1\u0365\2\150\1\266\1\u0365\1\150\1\u0365\1\150\1\u0365"+
"\1\150\1\u0365\6\150\1\0\2\150\1\0\4\150\1\146"+
"\1\0\2\150\1\0\1\u0259\5\146\1\150\10\146\1\u03aa"+
"\2\146\1\150\3\146\3\0\15\u01a3\1\u03ae\4\u01a3\2\150"+
"\1\301\3\0\2\146\1\150\5\146\1\150\1\u014c\1\u01a3"+
"\1\146\1\u01a3\1\146\1\150\1\u01a3\1\146\2\150\1\0"+
"\1\150\1\u01a3\1\146\1\150\1\176\1\u01a3\1\146\1\u01a3"+
"\1\146\1\u01a3\1\146\1\u01a3\6\146\1\0\1\150\1\146"+
"\1\0\3\150\1\146\27\0\1\u03af\57\0\2\u03af\24\0"+
"\1\u03af\2\0\1\u03af\3\0\1\u02eb\1\0\1\u02f7\1\150"+
"\1\0\1\u02eb\5\u03b0\2\u02eb\11\u03b0\2\u02eb\3\u03b0\3\0"+
"\22\u03b0\3\u02eb\3\0\4\u02eb\1\u03b0\3\u02eb\1\150\1\u033e"+
"\4\u03b0\1\u02eb\2\u03b0\2\u02eb\1\0\1\150\2\u03b0\1\u02eb"+
"\1\u02f3\10\u03b0\5\u02eb\1\0\2\u02eb\1\0\1\u02eb\2\150"+
"\2\u02eb\1\0\1\u02f7\1\150\1\0\1\u02eb\5\u0372\2\u02eb"+
"\11\u0372\1\u02eb\1\u02ec\3\u0372\3\0\22\u0372\1\u02f2\2\u02eb"+
"\3\0\4\u02eb\1\u0372\2\u02eb\1\u02ec\1\173\1\u033f\4\u0372"+
"\1\u02eb\2\u0372\2\u02ec\1\0\1\150\2\u0372\1\u02eb\1\u02f3"+
"\10\u0372\5\u02eb\1\0\1\u02ec\1\u02eb\1\0\1\u02ec\2\150"+
"\1\u02eb\1\u02ed\1\u01da\1\u02ed\1\u0166\1\u01da\1\u02ed\5\u038f"+
"\2\u02ed\11\u038f\1\u02ed\1\u02eb\3\u038f\3\u01da\22\u038f\1\u0373"+
"\1\u02ed\1\u02eb\3\u01da\4\u02ed\1\u038f\3\u02ed\1\u0166\1\150"+
"\4\u038f\1\u02ed\2\u038f\2\u02eb\1\u01da\1\u0166\2\u038f\1\u02ed"+
"\1\u0375\10\u038f\5\u02ed\1\u01da\1\u02eb\1\u02ed\1\u01da\1\u02eb"+
"\2\u0166\1\u02ed\1\u0346\1\0\1\u02f7\1\u0170\1\0\1\u0346"+
"\5\u03b1\2\u0346\11\u03b1\2\u0346\3\u03b1\3\0\22\u03b1\3\u0346"+
"\3\0\4\u0346\1\u03b1\3\u0346\1\u0170\1\u0376\4\u03b1\1\u0346"+
"\2\u03b1\2\u0346\1\0\1\u0170\2\u03b1\2\u0346\10\u03b1\5\u0346"+
"\1\0\2\u0346\1\0\1\u0346\2\u0170\1\u0346\20\0\1\u03b2"+
"\26\0\1\u03b2\105\0\1\u03b3\30\0\1\u03b3\120\0\1\u03b4"+
"\26\0\1\u03b4\105\0\1\u03b5\30\0\1\u03b5\102\0\1\150"+
"\1\0\2\150\1\0\16\150\1\u03b6\7\150\3\0\14\150"+
"\1\u03b6\10\150\3\0\23\150\1\0\4\150\1\266\15\150"+
"\1\0\2\150\1\0\5\150\1\0\2\150\1\0\17\150"+
"\1\u03b7\6\150\3\0\15\150\1\u03b7\7\150\3\0\23\150"+
"\1\0\4\150\1\266\15\150\1\0\2\150\1\0\5\150"+
"\1\0\2\150\1\0\1\150\1\u03b8\24\150\3\0\1\150"+
"\1\u03b8\23\150\3\0\23\150\1\0\4\150\1\266\15\150"+
"\1\0\2\150\1\0\5\150\1\0\2\150\1\0\14\150"+
"\1\u03b9\11\150\3\0\12\150\1\u03b9\12\150\3\0\23\150"+
"\1\0\4\150\1\266\15\150\1\0\2\150\1\0\4\150"+
"\1\146\1\0\2\150\1\0\4\146\1\u03ba\1\146\1\150"+
"\13\146\1\150\3\146\3\0\4\146\1\u03ba\15\146\2\150"+
"\1\146\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146"+
"\1\150\2\146\2\150\1\0\1\150\2\146\1\150\1\176"+
"\15\146\1\0\1\150\1\146\1\0\3\150\1\146\1\150"+
"\1\0\2\150\1\0\26\150\3\0\25\150\3\0\11\150"+
"\1\u03bb\11\150\1\0\4\150\1\266\15\150\1\0\2\150"+
"\1\0\5\150\1\0\2\150\1\0\10\150\1\u03bc\15\150"+
"\3\0\6\150\1\u03bc\16\150\3\0\23\150\1\0\4\150"+
"\1\266\15\150\1\0\2\150\1\0\5\150\1\0\2\150"+
"\1\0\1\u0387\7\150\1\u03bc\15\150\3\0\6\u0365\1\u03bd"+
"\13\u0365\2\150\1\u0325\3\0\12\150\1\u0365\1\150\1\u0365"+
"\2\150\1\u0365\3\150\1\0\1\150\1\u0365\2\150\1\266"+
"\1\u0365\1\150\1\u0365\1\150\1\u0365\1\150\1\u0365\6\150"+
"\1\0\2\150\1\0\4\150\1\146\1\0\2\150\1\0"+
"\1\u0259\3\146\1\u03ba\1\146\1\150\13\146\1\150\3\146"+
"\3\0\4\u01a3\1\u03be\15\u01a3\2\150\1\301\3\0\2\146"+
"\1\150\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146"+
"\1\150\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146"+
"\1\150\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146"+
"\1\u01a3\6\146\1\0\1\150\1\146\1\0\3\150\1\146"+
"\27\0\1\u03bf\57\0\2\u03bf\24\0\1\u03bf\2\0\1\u03bf"+
"\3\0\1\u02eb\1\0\1\u02f7\1\150\1\0\26\u02eb\3\0"+
"\25\u02eb\3\0\10\u02eb\1\150\1\u033e\11\u02eb\1\0\1\150"+
"\3\u02eb\1\u02f3\15\u02eb\1\0\2\u02eb\1\0\1\u02eb\2\150"+
"\1\u02eb\1\u0346\1\0\1\u02f7\1\u0170\1\0\1\u0346\5\u03c0"+
"\2\u0346\11\u03c0\2\u0346\3\u03c0\3\0\22\u03c0\3\u0346\3\0"+
"\4\u0346\1\u03c0\3\u0346\1\u0170\1\u0376\4\u03c0\1\u0346\2\u03c0"+
"\2\u0346\1\0\1\u0170\2\u03c0\2\u0346\10\u03c0\5\u0346\1\0"+
"\2\u0346\1\0\1\u0346\2\u0170\1\u0346\16\0\1\u03c1\26\0"+
"\1\u03c1\111\0\1\u0104\143\0\1\u02fb\162\0\1\u03c1\24\0"+
"\1\u03c1\64\0\1\150\1\0\2\150\1\0\13\150\1\u03c2"+
"\12\150\3\0\11\150\1\u03c2\13\150\3\0\23\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\5\150\1\0"+
"\2\150\1\0\4\150\1\u03c3\21\150\3\0\4\150\1\u03c3"+
"\20\150\3\0\23\150\1\0\4\150\1\266\15\150\1\0"+
"\2\150\1\0\5\150\1\0\2\150\1\0\12\150\1\u03c4"+
"\13\150\3\0\10\150\1\u03c4\14\150\3\0\23\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\5\150\1\0"+
"\2\150\1\0\3\150\1\u03c5\22\150\3\0\3\150\1\u03c5"+
"\21\150\3\0\23\150\1\0\4\150\1\266\15\150\1\0"+
"\2\150\1\0\4\150\1\146\1\0\2\150\1\0\6\146"+
"\1\u035c\13\146\1\150\3\146\3\0\22\146\2\150\1\146"+
"\3\0\2\146\1\150\5\146\1\150\1\u014c\4\146\1\150"+
"\2\146\2\150\1\0\1\150\2\146\1\150\1\176\15\146"+
"\1\0\1\150\1\146\1\0\3\150\1\146\1\u0166\1\u01da"+
"\2\u0166\1\u01da\1\u0166\5\u02a4\2\u0166\4\u02a4\1\u0324\4\u02a4"+
"\1\u0166\1\150\3\u02a4\3\u01da\12\u02a4\1\u0324\7\u02a4\1\u02a6"+
"\1\u0166\1\150\3\u01da\4\u0166\1\u02a4\4\u0166\1\150\4\u02a4"+
"\1\u0166\2\u02a4\2\150\1\u01da\1\u0166\2\u02a4\1\u0166\1\u0223"+
"\10\u02a4\5\u0166\1\u01da\1\150\1\u0166\1\u01da\1\150\3\u0166"+
"\1\150\1\0\2\150\1\0\6\150\1\u0325\17\150\3\0"+
"\25\150\3\0\23\150\1\0\4\150\1\266\15\150\1\0"+
"\2\150\1\0\5\150\1\0\2\150\1\0\1\u0387\5\150"+
"\1\u0325\17\150\3\0\22\u0365\2\150\1\u0325\3\0\12\150"+
"\1\u0365\1\150\1\u0365\2\150\1\u0365\3\150\1\0\1\150"+
"\1\u0365\2\150\1\266\1\u0365\1\150\1\u0365\1\150\1\u0365"+
"\1\150\1\u0365\6\150\1\0\2\150\1\0\4\150\1\146"+
"\1\0\2\150\1\0\1\u0259\5\146\1\u035c\13\146\1\150"+
"\3\146\3\0\22\u01a3\2\150\1\301\3\0\2\146\1\150"+
"\5\146\1\150\1\u014c\1\u01a3\1\146\1\u01a3\1\146\1\150"+
"\1\u01a3\1\146\2\150\1\0\1\150\1\u01a3\1\146\1\150"+
"\1\176\1\u01a3\1\146\1\u01a3\1\146\1\u01a3\1\146\1\u01a3"+
"\6\146\1\0\1\150\1\146\1\0\3\150\1\146\27\0"+
"\1\u03c6\57\0\2\u03c6\24\0\1\u03c6\2\0\1\u03c6\3\0"+
"\1\u0346\1\0\1\u02f7\1\u0170\1\0\26\u0346\3\0\25\u0346"+
"\3\0\10\u0346\1\u0170\1\u0376\11\u0346\1\0\1\u0170\21\u0346"+
"\1\0\2\u0346\1\0\1\u0346\2\u0170\1\u0346\31\0\1\u03b4"+
"\24\0\1\u03b4\65\0\1\150\1\0\2\150\1\0\11\150"+
"\1\u03c7\14\150\3\0\7\150\1\u03c7\15\150\3\0\23\150"+
"\1\0\4\150\1\266\15\150\1\0\2\150\1\0\5\150"+
"\1\0\2\150\1\0\6\150\1\214\17\150\3\0\25\150"+
"\3\0\23\150\1\0\4\150\1\266\15\150\1\0\2\150"+
"\1\0\5\150\1\0\2\150\1\0\6\150\1\u0354\17\150"+
"\3\0\25\150\3\0\23\150\1\0\4\150\1\266\15\150"+
"\1\0\2\150\1\0\5\150\1\0\2\150\1\0\25\150"+
"\1\u03c7\3\0\21\150\1\u03c7\3\150\3\0\23\150\1\0"+
"\4\150\1\266\15\150\1\0\2\150\1\0\4\150\27\0"+
"\1\5\57\0\2\5\24\0\1\5\2\0\1\5\3\0"+
"\1\150\1\0\2\150\1\0\24\150\1\u03c4\1\150\3\0"+
"\20\150\1\u03c4\4\150\3\0\23\150\1\0\4\150\1\266"+
"\15\150\1\0\2\150\1\0\4\150";
private static int [] zzUnpackTrans() {
int [] result = new int[88500];
int offset = 0;
offset = zzUnpackTrans(ZZ_TRANS_PACKED_0, offset, result);
offset = zzUnpackTrans(ZZ_TRANS_PACKED_1, offset, result);
return result;
}
private static int zzUnpackTrans(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
value--;
do result[j++] = value; while (--count > 0);
}
return j;
}
/* error codes */
private static final int ZZ_UNKNOWN_ERROR = 0;
private static final int ZZ_NO_MATCH = 1;
private static final int ZZ_PUSHBACK_2BIG = 2;
/* error messages for the codes above */
private static final String ZZ_ERROR_MSG[] = {
"Unkown internal scanner error",
"Error: could not match input",
"Error: pushback value was too large"
};
/**
* ZZ_ATTRIBUTE[aState] contains the attributes of state aState
*/
private static final int [] ZZ_ATTRIBUTE = zzUnpackAttribute();
private static final String ZZ_ATTRIBUTE_PACKED_0 =
"\1\0\2\1\3\11\1\1\5\3\1\1\11\3\2\1"+
"\3\3\2\1\1\11\22\3\6\1\2\3\4\1\2\3"+
"\1\1\2\3\1\1\1\11\2\3\2\1\4\3\5\1"+
"\1\3\3\11\1\1\24\0\6\3\1\1\2\3\1\5"+
"\1\3\2\0\1\3\4\0\1\3\2\0\1\3\1\0"+
"\2\3\1\0\1\1\7\3\2\0\16\3\1\1\2\3"+
"\1\0\2\3\1\0\1\3\2\0\3\3\2\0\3\3"+
"\1\0\1\1\7\0\3\3\1\0\6\3\1\0\1\1"+
"\1\3\3\0\11\3\1\0\11\3\1\0\17\3\1\0"+
"\2\3\1\0\3\3\2\0\6\3\1\0\2\1\4\0"+
"\1\1\2\0\1\2\2\0\1\3\6\0\2\1\3\0"+
"\6\3\1\1\1\3\6\0\1\1\1\11\4\3\1\1"+
"\24\3\1\0\1\15\20\0\1\1\2\0\1\2\7\3"+
"\3\0\2\3\1\2\2\1\1\5\4\0\1\2\1\5"+
"\4\2\1\5\6\0\1\3\1\7\4\2\1\7\3\2"+
"\3\3\2\0\12\3\1\2\1\0\5\3\1\5\1\2"+
"\1\5\2\0\1\1\2\0\1\3\2\1\1\3\1\1"+
"\1\0\1\1\2\0\3\1\2\3\2\1\2\0\1\1"+
"\1\0\5\3\1\0\1\1\4\3\2\1\12\3\1\0"+
"\1\1\5\3\1\0\1\1\1\0\2\2\1\3\5\0"+
"\1\5\2\0\1\15\3\0\1\5\1\15\1\5\2\0"+
"\1\2\1\0\1\2\1\0\1\1\4\3\1\11\2\1"+
"\1\3\2\1\2\3\1\1\4\3\6\0\1\11\27\0"+
"\3\3\1\0\3\5\1\3\3\5\1\0\2\5\2\2"+
"\1\5\1\1\10\0\1\3\3\5\1\2\1\3\1\0"+
"\1\1\3\0\2\3\1\1\1\3\1\1\1\0\4\3"+
"\1\0\1\1\6\0\1\1\2\0\1\5\1\3\1\5"+
"\2\0\1\3\3\0\6\1\1\0\1\1\3\3\1\1"+
"\2\3\1\1\1\0\3\3\1\1\4\3\1\0\1\1"+
"\1\0\1\1\2\2\2\0\1\1\1\0\1\1\3\0"+
"\1\1\2\3\1\1\3\0\1\1\1\2\2\3\1\0"+
"\1\1\1\0\1\11\12\0\2\1\5\0\1\5\1\15"+
"\1\0\1\3\1\0\1\3\1\5\1\1\7\0\1\3"+
"\1\2\4\0\2\3\3\0\2\3\1\0\1\3\4\0"+
"\1\1\1\0\1\3\1\1\1\0\1\1\4\0\11\1"+
"\1\3\1\0\1\1\2\3\1\1\2\3\1\0\2\1"+
"\2\2\1\1\4\0\1\1\1\11\4\0\1\5\6\0"+
"\2\3\4\0\1\1\1\3\1\11\1\1\11\0\1\3"+
"\1\2\6\1\1\0\1\3\1\2\5\0\1\2\1\0"+
"\1\3\1\5\4\0\2\1\1\5\2\1\1\2\2\0"+
"\7\1\1\3\2\1\1\3\1\0\2\1\2\2\2\0"+
"\1\1\12\0\2\2\2\0\1\3\5\0\1\1\1\0"+
"\1\2\2\1\1\0\1\3\1\0\3\1\2\0\2\1"+
"\1\3\3\0\3\1\1\3\1\1\1\0\2\1\1\0"+
"\2\1\1\3\1\0\1\1\3\0\1\1\1\5\1\0"+
"\1\5\1\0\1\1\7\0\1\2\2\1\3\0\2\1"+
"\2\0\2\1\2\0\2\1\6\0\1\1\3\0\1\2"+
"\1\0\2\1\1\0\2\1\1\2\1\1\13\0\2\1"+
"\1\0\2\1\11\0\1\2\1\0\2\1\1\0\1\1"+
"\7\0";
private static int [] zzUnpackAttribute() {
int [] result = new int[967];
int offset = 0;
offset = zzUnpackAttribute(ZZ_ATTRIBUTE_PACKED_0, offset, result);
return result;
}
private static int zzUnpackAttribute(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/** the input device */
java.io.Reader zzReader;
/** the current state of the DFA */
int zzState;
/** the current lexical state */
int zzLexicalState = YYINITIAL;
/** this buffer contains the current text to be matched and is
the source of the yytext() string */
char zzBuffer[] = new char[ZZ_BUFFERSIZE];
/** the textposition at the last accepting state */
int zzMarkedPos;
/** the textposition at the last state to be included in yytext */
int zzPushbackPos;
/** the current text position in the buffer */
int zzCurrentPos;
/** startRead marks the beginning of the yytext() string in the buffer */
int zzStartRead;
/** endRead marks the last character in the buffer, that has been read
from input */
int zzEndRead;
/** number of newlines encountered up to the start of the matched text */
int yyline;
/** the number of characters up to the start of the matched text */
int yychar;
/**
* the number of characters from the last newline up to the start of the
* matched text
*/
int yycolumn;
/**
* zzAtBOL == true <=> the scanner is currently at the beginning of a line
*/
boolean zzAtBOL = true;
/** zzAtEOF == true <=> the scanner is at the EOF */
boolean zzAtEOF;
/* user code: */
/**
* Constructs a new PTBLexer with a custom LexedTokenFactory.
*
* @param r The Reader to tokenize text from
* @param tf The LexedTokenFactory that will be invoked to convert
* each substring extracted by the lexer into some kind of Object (
* such as a Word).
*/
public PTBLexer(Reader r, FeatureLabelTokenFactory tf, boolean tokenizeCRs) {
this(r, tf, tokenizeCRs, false);
}
/**
* Constructs a new PTBLexer which suppresses PTB token transforms
* (like -LRB-, -RRB-).
*
* @param suppressEscaping if true, suppress the PTB token transform and
* keep the original ones
*/
public PTBLexer(Reader r, FeatureLabelTokenFactory tf, boolean tokenizeCRs, boolean suppressEscaping) {
this(r);
this.invertable = false;
tokenFactory = tf;
this.tokenizeCRs = tokenizeCRs;
// System.err.println("DEBUG: suppressEscaping="+suppressEscaping);
this.suppressEscaping = suppressEscaping;
}
/**
* Constructs a new PTBLexer which produces FeatureLabels.
*
* @param r The Reader to tokenize text from
* @param invertable If the invertable flag is set to true then the
* produced FeatureLabels will contain fields for the text
* both before and after the current token (but after the
* previous token and before the next token) and the
* original version of this word, and the character offset
* for when the token begins and ends. In this case the tokenFactory
* necessarily vends FeatureLabels.
*/
public PTBLexer(Reader r, boolean invertable, boolean tokenizeCRs) {
this(r);
tokenFactory = new FeatureLabelTokenFactory();
this.invertable = invertable;
this.tokenizeCRs = tokenizeCRs;
// System.err.println("invertable2: "+this.invertable);
}
/*
* This has now been extended to cover the main Windows CP1252 characters,
* at either their correct Unicode codepoints, or in their invalid
* positions as 8 bit chars inside the iso-8859 control region.
*
* ellipses 85 0133 2026 8230
* single quote curly starting 91 0145 2018 8216
* single quote curly ending 92 0146 2019 8217
* double quote curly starting 93 0147 201C 8220
* double quote curly ending 94 0148 201D 8221
* en dash 96 0150 2013 8211
* em dash 97 0151 2014 8212
*/
public static final String opendblquote = "``";
public static final String closedblquote = "''";
public static final String openparen = "-LRB-";
public static final String closeparen = "-RRB-";
public static final String openbrace = "-LCB-";
public static final String closebrace = "-RCB-";
public static final String ptbmdash = "--";
public static final String ptbellipsis = "...";
/** For tokenizing carriage returns. (JS) */
public static final String cr = "*CR*";
/** This quotes a character with a backslash, but doesn't do it
* if the character is already preceded by a backslash.
*/
private static String delimit (String s, char c) {
int i = s.indexOf(c);
while (i != -1) {
if (i == 0 || s.charAt(i - 1) != '\\') {
s = s.substring(0, i) + "\\" + s.substring(i);
i = s.indexOf(c, i + 2);
} else {
i = s.indexOf(c, i + 1);
}
}
return s;
}
private static String normalizeCp1252(String in) {
String s1 = in;
// s1 = s1.replaceAll("[\u0085\u2026]", "..."); // replaced directly
// s1 = s1.replaceAll("[\u0096\u0097\u2013\u2014]", "--");
try {
s1 = s1.replaceAll("'", "'");
// s1 = s1.replaceAll(""", "\""); // replaced directly
s1 = s1.replaceAll("[\u0091\u2018]", "`");
s1 = s1.replaceAll("[\u0092\u2019]", "'");
s1 = s1.replaceAll("[\u0093\u201C]", "``");
s1 = s1.replaceAll("[\u0094\u201D]", "''");
s1 = s1.replaceAll("\u00BC", "1\\/4");
s1 = s1.replaceAll("\u00BD", "1\\/2");
s1 = s1.replaceAll("\u00BE", "3\\/4");
s1 = s1.replaceAll("\u00A2", "cents");
s1 = s1.replaceAll("\u00A3", "#");
s1 = s1.replaceAll("[\u0080\u20AC]", "$"); // Euro -- no good translation!
} catch (Exception e){
System.err.println("Exception in normalizeCp1252 caught. String: "+s1);
}
return s1;
}
private static String normalizeAmp(final String in) {
return in.replaceAll("(?i:&)", "&");
}
private FeatureLabelTokenFactory tokenFactory;
private boolean invertable;
private boolean tokenizeCRs;
private boolean suppressEscaping;
private FeatureLabel prevWord = new FeatureLabel();
private FeatureLabel getNext() {
return getNext(yytext(), yytext());
}
private FeatureLabel getNext(String txt, String current) {
if (!invertable) {
return tokenFactory.makeToken(txt, yychar, yylength());
}
FeatureLabel word = (FeatureLabel)tokenFactory.makeToken(txt, yychar, yylength());
word.put(AbstractMapLabel.CURRENT_KEY, current);
word.put(AbstractMapLabel.BEFORE_KEY, prevWord.get(AbstractMapLabel.AFTER_KEY));
prevWord = word;
return prevWord;
}
/**
* Creates a new scanner
* There is also a java.io.InputStream version of this constructor.
*
* @param in the java.io.Reader to read input from.
*/
PTBLexer(java.io.Reader in) {
this.zzReader = in;
tokenFactory = new FeatureLabelTokenFactory();
}
/**
* Creates a new scanner.
* There is also java.io.Reader version of this constructor.
*
* @param in the java.io.Inputstream to read input from.
*/
PTBLexer(java.io.InputStream in) {
this(new java.io.InputStreamReader(in));
}
/**
* Unpacks the compressed character translation table.
*
* @param packed the packed character translation table
* @return the unpacked character translation table
*/
private static char [] zzUnpackCMap(String packed) {
char [] map = new char[0x10000];
int i = 0; /* index in packed string */
int j = 0; /* index in unpacked array */
while (i < 256) {
int count = packed.charAt(i++);
char value = packed.charAt(i++);
do map[j++] = value; while (--count > 0);
}
return map;
}
/**
* Refills the input buffer.
*
* @return false
, iff there was new input.
*
* @exception java.io.IOException if any I/O-Error occurs
*/
private boolean zzRefill() throws java.io.IOException {
if (zzReader==null) return true;
/* first: make room (if you can) */
if (zzStartRead > 0) {
System.arraycopy(zzBuffer, zzStartRead,
zzBuffer, 0,
zzEndRead-zzStartRead);
/* translate stored positions */
zzEndRead-= zzStartRead;
zzCurrentPos-= zzStartRead;
zzMarkedPos-= zzStartRead;
zzPushbackPos-= zzStartRead;
zzStartRead = 0;
}
/* is the buffer big enough? */
if (zzCurrentPos >= zzBuffer.length) {
/* if not: blow it up */
char newBuffer[] = new char[zzCurrentPos*2];
System.arraycopy(zzBuffer, 0, newBuffer, 0, zzBuffer.length);
zzBuffer = newBuffer;
}
/* finally: fill the buffer with new input */
int numRead = zzReader.read(zzBuffer, zzEndRead,
zzBuffer.length-zzEndRead);
if (numRead < 0) {
return true;
}
else {
zzEndRead+= numRead;
return false;
}
}
/**
* Closes the input stream.
*/
public final void yyclose() throws java.io.IOException {
zzAtEOF = true; /* indicate end of file */
zzEndRead = zzStartRead; /* invalidate buffer */
if (zzReader != null)
zzReader.close();
}
/**
* Resets the scanner to read from a new input stream.
* Does not close the old reader.
*
* All internal variables are reset, the old input stream
* cannot be reused (internal buffer is discarded and lost).
* Lexical state is set to ZZ_INITIAL.
*
* @param reader the new input stream
*/
public final void yyreset(java.io.Reader reader) {
zzReader = reader;
zzAtBOL = true;
zzAtEOF = false;
zzEndRead = zzStartRead = 0;
zzCurrentPos = zzMarkedPos = zzPushbackPos = 0;
yyline = yychar = yycolumn = 0;
zzLexicalState = YYINITIAL;
}
/**
* Returns the current lexical state.
*/
public final int yystate() {
return zzLexicalState;
}
/**
* Enters a new lexical state
*
* @param newState the new lexical state
*/
public final void yybegin(int newState) {
zzLexicalState = newState;
}
/**
* Returns the text matched by the current regular expression.
*/
public final String yytext() {
return new String( zzBuffer, zzStartRead, zzMarkedPos-zzStartRead );
}
/**
* Returns the character at position pos from the
* matched text.
*
* It is equivalent to yytext().charAt(pos), but faster
*
* @param pos the position of the character to fetch.
* A value from 0 to yylength()-1.
*
* @return the character at position pos
*/
public final char yycharat(int pos) {
return zzBuffer[zzStartRead+pos];
}
/**
* Returns the length of the matched text region.
*/
public final int yylength() {
return zzMarkedPos-zzStartRead;
}
/**
* Reports an error that occured while scanning.
*
* In a wellformed scanner (no or only correct usage of
* yypushback(int) and a match-all fallback rule) this method
* will only be called with things that "Can't Possibly Happen".
* If this method is called, something is seriously wrong
* (e.g. a JFlex bug producing a faulty scanner etc.).
*
* Usual syntax/scanner level error handling should be done
* in error fallback rules.
*
* @param errorCode the code of the errormessage to display
*/
private void zzScanError(int errorCode) {
String message;
try {
message = ZZ_ERROR_MSG[errorCode];
}
catch (ArrayIndexOutOfBoundsException e) {
message = ZZ_ERROR_MSG[ZZ_UNKNOWN_ERROR];
}
throw new Error(message);
}
/**
* Pushes the specified amount of characters back into the input stream.
*
* They will be read again by then next call of the scanning method
*
* @param number the number of characters to be read again.
* This number must not be greater than yylength()!
*/
public void yypushback(int number) {
if ( number > yylength() )
zzScanError(ZZ_PUSHBACK_2BIG);
zzMarkedPos -= number;
}
/**
* Resumes scanning until the next regular expression is matched,
* the end of input is encountered or an I/O-Error occurs.
*
* @return the next token
* @exception java.io.IOException if any I/O-Error occurs
*/
public FeatureLabel next() throws java.io.IOException {
int zzInput;
int zzAction;
// cached fields:
int zzCurrentPosL;
int zzMarkedPosL;
int zzEndReadL = zzEndRead;
char [] zzBufferL = zzBuffer;
char [] zzCMapL = ZZ_CMAP;
int [] zzTransL = ZZ_TRANS;
int [] zzRowMapL = ZZ_ROWMAP;
int [] zzAttrL = ZZ_ATTRIBUTE;
int zzPushbackPosL = zzPushbackPos = -1;
boolean zzWasPushback;
while (true) {
zzMarkedPosL = zzMarkedPos;
yychar+= zzMarkedPosL-zzStartRead;
zzAction = -1;
zzCurrentPosL = zzCurrentPos = zzStartRead = zzMarkedPosL;
zzState = zzLexicalState;
zzWasPushback = false;
zzForAction: {
while (true) {
if (zzCurrentPosL < zzEndReadL)
zzInput = zzBufferL[zzCurrentPosL++];
else if (zzAtEOF) {
zzInput = YYEOF;
break zzForAction;
}
else {
// store back cached positions
zzCurrentPos = zzCurrentPosL;
zzMarkedPos = zzMarkedPosL;
zzPushbackPos = zzPushbackPosL;
boolean eof = zzRefill();
// get translated positions and possibly new buffer
zzCurrentPosL = zzCurrentPos;
zzMarkedPosL = zzMarkedPos;
zzBufferL = zzBuffer;
zzEndReadL = zzEndRead;
zzPushbackPosL = zzPushbackPos;
if (eof) {
zzInput = YYEOF;
break zzForAction;
}
else {
zzInput = zzBufferL[zzCurrentPosL++];
}
}
int zzNext = zzTransL[ zzRowMapL[zzState] + zzCMapL[zzInput] ];
if (zzNext == -1) break zzForAction;
zzState = zzNext;
int zzAttributes = zzAttrL[zzState];
if ( (zzAttributes & 2) == 2 )
zzPushbackPosL = zzCurrentPosL;
if ( (zzAttributes & 1) == 1 ) {
zzWasPushback = (zzAttributes & 4) == 4;
zzAction = zzState;
zzMarkedPosL = zzCurrentPosL;
if ( (zzAttributes & 8) == 8 ) break zzForAction;
}
}
}
// store back cached position
zzMarkedPos = zzMarkedPosL;
if (zzWasPushback)
zzMarkedPos = zzPushbackPosL;
switch (zzAction < 0 ? zzAction : ZZ_ACTION[zzAction]) {
case 22:
{ if (!suppressEscaping) {
return getNext(normalizeAmp(yytext()), yytext()); }
else {
return getNext();
}
}
case 28: break;
case 26:
{ String s = yytext();
yypushback(1); // return a period for next time
return getNext(s, yytext());
}
case 29: break;
case 12:
{ if (invertable) {
if (prevWord == null) {
prevWord = new FeatureLabel();
}
prevWord.appendAfter(yytext());
}
}
case 30: break;
case 8:
{ if (invertable) {
if (prevWord == null) {
prevWord = new FeatureLabel();
}
prevWord.appendAfter(yytext());
}
}
case 31: break;
case 2:
{ if (!suppressEscaping) {
return getNext(openparen, yytext()); }
else {
return getNext();
}
}
case 32: break;
case 4:
{ return getNext();
}
case 33: break;
case 14:
{ if (!suppressEscaping) {
return getNext(normalizeCp1252(yytext()), yytext()); }
else {
return getNext();
}
}
case 34: break;
case 17:
{ if (!suppressEscaping) {
return getNext(closedblquote, yytext()); }
else {
return getNext();
}
}
case 35: break;
case 6:
{ if (!suppressEscaping) {
String word = Americanize.americanize(yytext());
return getNext(word, yytext()); }
else {
return getNext();
}
}
case 36: break;
case 21:
{ if (!suppressEscaping) {
return getNext(delimit(yytext(), '/'), yytext()); }
else {
return getNext();
}
}
case 37: break;
case 18:
{ if (!suppressEscaping) {
return getNext(openbrace, yytext()); }
else {
return getNext();
}
}
case 38: break;
case 24:
{ yypushback(1); return getNext();
}
case 39: break;
case 11:
{ if (!suppressEscaping) {
return getNext(openparen, yytext()); }
else {
return getNext();
}
}
case 40: break;
case 20:
{ if (!suppressEscaping) {
return getNext(opendblquote, yytext()); }
else {
return getNext();
}
}
case 41: break;
case 7:
{ if (!suppressEscaping) {
return getNext(ptbmdash, yytext()); }
else {
return getNext();
}
}
case 42: break;
case 3:
{ if (!suppressEscaping) {
return getNext(delimit(yytext(), '/'), yytext()); }
else {
return getNext();
}
}
case 43: break;
case 19:
{ if (!suppressEscaping) {
return getNext(closebrace, yytext()); }
else {
return getNext();
}
}
case 44: break;
case 15:
{ if (!suppressEscaping) {
return getNext(ptbellipsis, yytext()); }
else {
return getNext();
}
}
case 45: break;
case 5:
{ if (!suppressEscaping) {
return getNext(closeparen, yytext()); }
else {
return getNext();
}
}
case 46: break;
case 10:
{ if (yylength() >= 3 && yylength() <= 4 && !suppressEscaping) {
return getNext(ptbmdash, yytext());
} else {
return getNext();
}
}
case 47: break;
case 27:
{ yypushback(3) ; return getNext();
}
case 48: break;
case 1:
{ String str = yytext();
if (invertable) { prevWord.appendAfter(str); }
int first = str.charAt(0);
System.err.println("Untokenizable: " + yytext() +
" (first char in decimal: " + first + ")");
}
case 49: break;
case 16:
{ if (!suppressEscaping) {
return getNext(delimit(yytext(), '*'), yytext()); }
else {
return getNext();
}
}
case 50: break;
case 9:
{ if (tokenizeCRs) {
return getNext(cr, yytext()); // js: for tokenizing carriage returns
} else if (invertable) {
prevWord.appendAfter(yytext());
}
}
case 51: break;
case 23:
{ /* invert quote - using trailing context didn't work.... */
String str = yytext();
yypushback(2);
if (!suppressEscaping) {
return getNext("`", str); }
else {
return getNext(str,str);
}
}
case 52: break;
case 25:
{ if (!suppressEscaping) {
return getNext("&", yytext()); }
else {
return getNext();
}
}
case 53: break;
case 13:
{ if (!suppressEscaping) {
return getNext(normalizeCp1252(yytext()), yytext()); }
else {
return getNext();
}
}
case 54: break;
default:
if (zzInput == YYEOF && zzStartRead == zzCurrentPos) {
zzAtEOF = true;
{
if (invertable) { prevWord.appendAfter(yytext()); }
return null;
}
}
else {
zzScanError(ZZ_NO_MATCH);
}
}
}
}
}