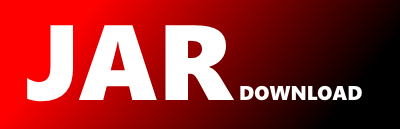
edu.berkeley.nlp.syntax.GrammaticalRole Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.syntax;
import edu.berkeley.nlp.util.IdentityHashSet;
import edu.berkeley.nlp.util.functional.FunctionalUtils;
import edu.berkeley.nlp.util.functional.Predicate;
import java.io.StringReader;
import java.util.List;
import java.util.Set;
/**
* User: aria42
* Date: Mar 24, 2009
*/
public enum GrammaticalRole {
SUBJECT, OBJECT, OTHER, NONE, NULL;
private static boolean isObject(TreePath treePath) {
for (TreePath.Transition transition : treePath.getTransitions()) {
if (transition.getDirection() != TreePath.Direction.UP) {
return false;
}
}
return true;
}
private static boolean isSubject(TreePath treePath) {
boolean hitS = false;
for (TreePath.Transition trans : treePath.getTransitions()) {
Tree toNode = trans.getToNode();
TreePath.Direction dir = trans.getDirection();
Tree dest = trans.getToNode();
if (dest.getLabel().startsWith("S")) {
hitS = true;
continue;
}
if (!hitS) {
if (dir != TreePath.Direction.UP) {
return false;
}
}
if (hitS) {
if (!(dir == TreePath.Direction.DOWN_RIGHT)) {
return false;
}
}
}
return hitS;
}
public static GrammaticalRole findRole(final Tree node, final Tree root)
{
Set> nodes = new IdentityHashSet>(root.getPostOrderTraversal());
if (!nodes.contains(node)) {
return GrammaticalRole.NONE;
}
GrammaticalRole curRole = GrammaticalRole.OTHER;
List> vpNodes = FunctionalUtils.filter(nodes,new Predicate>() {
public Boolean apply(Tree input) {
return input.isPhrasal() && input.getLabel().startsWith("VP");
}
});
TreePathFinder tpf = new TreePathFinder(root);
for (Tree vpNode : vpNodes) {
if (vpNode == node) continue;
TreePath tp = tpf.findPath(node,vpNode);
if (isSubject(tp)) {
return GrammaticalRole.SUBJECT;
}
if (isObject(tp)) {
curRole = GrammaticalRole.OBJECT;
}
}
return curRole;
}
public static void main(String[] args) {
String treeStr = "(ROOT (S (NP (DT The) (NN dog)) (VP (VBD ran) (NN home))))";
StringReader reader = new StringReader(treeStr);
Tree tree = new Trees.PennTreeReader(reader).next();
Tree subjNode = tree.getChildren().get(0).getChildren().get(0);
Tree objNode = tree.getChild(0).getChild(1).getChild(1);
System.out.println("subjNode is " + GrammaticalRole.findRole(subjNode,tree));
System.out.println("objNode is " + GrammaticalRole.findRole(objNode,tree));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy