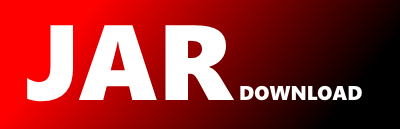
edu.berkeley.nlp.syntax.TreePath Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.syntax;
import java.util.List;
public class TreePath {
public enum Direction {
UP,
DOWN,
DOWN_LEFT,
DOWN_RIGHT;
}
public static class Transition {
private Tree fromNode;
private Tree toNode;
private Direction direction;
public Transition(Tree fromNode, Tree toNode, Direction direction) {
this.fromNode = fromNode;
this.toNode = toNode;
this.direction = direction;
}
public Tree getFromNode() {
return fromNode;
}
public Tree getToNode() {
return toNode;
}
public Direction getDirection() {
return direction;
}
}
private Tree startNode;
private Tree endNode;
private List> transitions;
public TreePath(List> transitions) {
if (transitions == null || transitions.size() == 0) {
throw new IllegalArgumentException("Cannot have empty transitions list");
}
this.transitions = transitions;
startNode = transitions.get(0).fromNode;
endNode = transitions.get(transitions.size()-1).toNode;
}
public Tree getStartNode() {
return startNode;
}
public Tree getEndNode() {
return endNode;
}
public List> getTransitions() {
return transitions;
}
private String cacheStr = null;
@Override
public String toString() {
if (cacheStr == null) {
StringBuilder sb = new StringBuilder();
sb.append("[ ");
for (Transition transition : transitions) {
sb.append(transition.fromNode.getLabel() + " " + transition.direction + " ");
}
sb.append(endNode.getLabel() + " ]");
cacheStr = sb.toString();
}
return cacheStr;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy