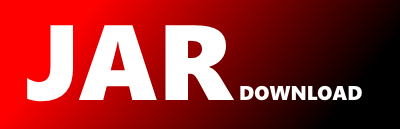
edu.berkeley.nlp.tokenizer.PTBLexer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
/**
*
*/
package edu.berkeley.nlp.tokenizer;
/* The following code was generated by JFlex 1.4_5 on 11/30/03 2:25 PM */
import java.util.*;
import java.io.*;
//import edu.stanford.nlp.trees.*;
/**
* This class is a scanner generated by
* JFlex 1.4_5
* on 11/30/03 2:25 PM from the specification file
* PTBLexer.flex
*/
public class PTBLexer {
/** This character denotes the end of file */
public static final int YYEOF = -1;
/** initial size of the lookahead buffer */
private static final int YY_BUFFERSIZE = 16384;
/** lexical states */
public static final int YYINITIAL = 0;
/**
* Translates characters to character classes
*/
private static final String yycmap_packed =
"\1\130\10\0\1\45\1\46\1\0\1\76\1\131\22\0\1\115\1\3"+
"\1\123\1\36\1\56\1\61\1\5\1\122\1\53\1\54\1\120\1\52"+
"\1\62\1\50\1\63\1\2\1\75\1\37\5\74\1\125\2\74\1\51"+
"\1\15\1\1\1\116\1\4\1\77\1\117\1\34\1\114\1\24\1\7"+
"\1\67\1\101\1\110\1\16\1\105\1\100\1\107\1\20\1\6\1\47"+
"\1\65\1\35\1\23\1\22\1\66\1\17\1\21\1\70\1\103\3\71"+
"\1\116\1\55\1\116\1\116\1\60\1\121\1\12\1\102\1\27\1\11"+
"\1\42\1\104\1\32\1\14\1\40\1\113\1\73\1\30\1\10\1\57"+
"\1\25\1\33\1\26\1\43\1\13\1\31\1\41\1\44\1\72\1\112"+
"\1\111\1\106\1\126\1\124\1\127\1\116\uff7e\0\1\64\2\0";
/**
* Translates characters to character classes
*/
private static final char [] yycmap = yy_unpack_cmap(yycmap_packed);
/**
* Translates DFA states to action switch labels.
*/
private static final int [] YY_ACTION = yy_unpack_YY_ACTION();
private static final String YY_ACTION_packed0 =
"\1\1\1\2\3\3\23\4\2\3\2\4\1\5\1\6"+
"\1\4\1\7\2\3\1\10\1\11\1\5\1\4\3\3"+
"\4\4\1\3\10\4\1\3\1\1\2\3\1\12\1\5"+
"\1\13\1\14\23\0\5\4\1\0\1\4\1\3\3\4"+
"\1\3\1\4\2\0\1\4\2\0\1\3\1\0\2\4"+
"\10\0\1\4\2\3\1\4\4\0\1\3\1\0\7\4"+
"\1\3\4\4\1\0\2\4\1\0\1\3\10\4\1\0"+
"\1\3\3\0\4\4\2\0\1\7\2\0\1\1\1\4"+
"\14\0\3\4\1\0\4\4\1\3\21\4\1\15\14\0"+
"\1\16\1\3\1\0\2\3\4\4\1\0\1\3\2\0"+
"\2\3\1\0\2\3\4\0\1\4\2\3\7\0\1\16"+
"\1\4\1\3\1\0\1\3\3\0\5\4\1\0\4\4"+
"\1\3\2\4\1\3\2\0\3\4\1\16\1\3\1\0"+
"\1\3\3\0\1\4\1\3\4\0\1\3\4\4\1\3"+
"\3\4\1\17\7\0\2\4\1\20\3\3\1\0\3\3"+
"\1\4\4\0\2\3\1\0\1\16\3\0\1\4\1\3"+
"\1\4\6\0\1\3\1\0\1\3\1\0\3\3\3\4"+
"\1\3\2\4\3\0\2\21\1\0\1\16\1\0\1\3"+
"\1\0\1\3\3\0\1\3\2\0\1\3\2\0\1\4"+
"\1\5\6\0\1\16\1\4\1\0\1\4\1\0\2\3"+
"\1\0\2\16\1\0\1\3\1\0\1\3\3\0\1\4"+
"\1\0\1\4\1\0\1\4\4\0\1\16\1\0\1\3"+
"\1\0\1\3\3\0\1\4\1\0\1\4\3\3\3\0"+
"\3\3\5\0\1\3\2\0\3\3\2\0\1\3\2\0"+
"\2\3\2\0\1\3\7\0";
private static int [] yy_unpack_YY_ACTION() {
int [] result = new int[448];
int offset = 0;
offset = yy_unpack_YY_ACTION(YY_ACTION_packed0, offset, result);
return result;
}
private static int yy_unpack_YY_ACTION(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/**
* Translates a state to a row index in the transition table
*/
private static final int [] yy_rowMap = yy_unpack_yy_rowMap();
private static final String yy_rowMap_packed0 =
"\0\0\0\132\0\264\0\132\0\u010e\0\u0168\0\u01c2\0\u021c"+
"\0\u0276\0\u02d0\0\u032a\0\u0384\0\u03de\0\u0438\0\u0492\0\u04ec"+
"\0\u0546\0\u05a0\0\u05fa\0\u0654\0\u06ae\0\u0708\0\u0762\0\u07bc"+
"\0\u0816\0\u0870\0\u08ca\0\u0924\0\u097e\0\132\0\u09d8\0\u0a32"+
"\0\u0a8c\0\u0ae6\0\u0b40\0\132\0\u0b9a\0\u0bf4\0\u0c4e\0\u0ca8"+
"\0\u0d02\0\u0d5c\0\u0db6\0\u0e10\0\u0e6a\0\u0ec4\0\u0f1e\0\u0f78"+
"\0\u0fd2\0\u102c\0\u1086\0\u10e0\0\u113a\0\u1194\0\u11ee\0\u1248"+
"\0\u12a2\0\u12fc\0\u1356\0\132\0\132\0\132\0\u13b0\0\u140a"+
"\0\u1464\0\u14be\0\u1518\0\u1572\0\u15cc\0\u1626\0\u1680\0\u16da"+
"\0\u1734\0\u178e\0\u17e8\0\u1842\0\u189c\0\u18f6\0\u1950\0\u19aa"+
"\0\u1a04\0\u1a5e\0\u1ab8\0\u1b12\0\u1b6c\0\u1bc6\0\u1c20\0\u1c7a"+
"\0\u1cd4\0\u1d2e\0\u1d88\0\u1de2\0\u1e3c\0\u1e96\0\u1ef0\0\u1f4a"+
"\0\u1fa4\0\u1ffe\0\u2058\0\u20b2\0\u210c\0\u2166\0\u21c0\0\u221a"+
"\0\u2274\0\u22ce\0\u2328\0\u20b2\0\u2382\0\u23dc\0\u2436\0\u2490"+
"\0\u24ea\0\u2544\0\u259e\0\u25f8\0\u2652\0\u26ac\0\u2706\0\u2760"+
"\0\u27ba\0\u2814\0\u286e\0\u28c8\0\u2922\0\u297c\0\u29d6\0\u2a30"+
"\0\u2a8a\0\u2ae4\0\u2b3e\0\u2b98\0\u2bf2\0\u2c4c\0\u2ca6\0\u2d00"+
"\0\u2d5a\0\u2db4\0\u2e0e\0\u2e68\0\u2ec2\0\u2f1c\0\u2f76\0\u2fd0"+
"\0\u302a\0\u3084\0\u30de\0\u3138\0\u3192\0\u0a8c\0\u31ec\0\u3246"+
"\0\u32a0\0\u32fa\0\u3354\0\u33ae\0\u3408\0\u3462\0\u34bc\0\u3516"+
"\0\u0b9a\0\u3570\0\u35ca\0\u3624\0\u367e\0\u36d8\0\u3732\0\u378c"+
"\0\u37e6\0\u3840\0\u389a\0\u38f4\0\u394e\0\u39a8\0\u3a02\0\u3a5c"+
"\0\u3ab6\0\u3b10\0\u3b6a\0\u3bc4\0\u3c1e\0\u3c78\0\u3cd2\0\u3d2c"+
"\0\u3d86\0\u3de0\0\u3e3a\0\u3e94\0\u3eee\0\u3f48\0\u3fa2\0\u3ffc"+
"\0\u4056\0\u40b0\0\u410a\0\u4164\0\u41be\0\u4218\0\u4272\0\u42cc"+
"\0\132\0\u4326\0\u4380\0\u43da\0\u4434\0\u448e\0\u44e8\0\u4542"+
"\0\u459c\0\u45f6\0\u4650\0\u46aa\0\u4704\0\u475e\0\u1c20\0\u47b8"+
"\0\u4812\0\u47b8\0\u486c\0\u48c6\0\u4920\0\u497a\0\u49d4\0\u4a2e"+
"\0\u4a88\0\u4ae2\0\u1ffe\0\u2328\0\u4b3c\0\132\0\u2058\0\u4b96"+
"\0\u4bf0\0\u4c4a\0\u4ca4\0\u4cfe\0\u4d58\0\u4db2\0\u4db2\0\u4d58"+
"\0\u4e0c\0\u4e66\0\u4ec0\0\u4f1a\0\u4f74\0\u4fce\0\u5028\0\u5082"+
"\0\u50dc\0\u5136\0\u5190\0\u51ea\0\u5244\0\u529e\0\u52f8\0\u5352"+
"\0\u53ac\0\u5406\0\u5460\0\u54ba\0\u5514\0\u556e\0\u55c8\0\u5622"+
"\0\u567c\0\u56d6\0\u210c\0\u5730\0\u578a\0\u57e4\0\u583e\0\u5898"+
"\0\u58f2\0\u594c\0\u59a6\0\u5a00\0\u5a5a\0\u5ab4\0\u5b0e\0\u5b68"+
"\0\u5bc2\0\u5c1c\0\u5c76\0\u5cd0\0\u5d2a\0\u5d84\0\u5dde\0\u5e38"+
"\0\u5e92\0\u5eec\0\u5f46\0\u5fa0\0\u5ffa\0\u6054\0\132\0\u60ae"+
"\0\u6108\0\u6162\0\u61bc\0\u6216\0\u6270\0\u62ca\0\u6324\0\u637e"+
"\0\132\0\u1c20\0\u63d8\0\u1ef0\0\u6432\0\u22ce\0\u648c\0\u64e6"+
"\0\u6540\0\u659a\0\u65f4\0\u664e\0\u66a8\0\u65f4\0\u664e\0\u6702"+
"\0\u675c\0\u67b6\0\u6810\0\u686a\0\u68c4\0\u691e\0\u6978\0\u69d2"+
"\0\u6a2c\0\u6a86\0\u6ae0\0\u6b3a\0\u6b94\0\u6702\0\u6bee\0\u69d2"+
"\0\u6c48\0\u6a86\0\u6ca2\0\u6cfc\0\u6d56\0\u6db0\0\u6e0a\0\u6e64"+
"\0\u6ebe\0\u6f18\0\u6f72\0\u6fcc\0\u7026\0\132\0\u578a\0\u7080"+
"\0\u70da\0\u7134\0\u7080\0\u718e\0\u71e8\0\u7242\0\u729c\0\u72f6"+
"\0\u7350\0\u73aa\0\u7404\0\u745e\0\u648c\0\u74b8\0\u7512\0\u1cd4"+
"\0\u756c\0\u75c6\0\u7620\0\u767a\0\u76d4\0\u772e\0\u62ca\0\u7788"+
"\0\u77e2\0\u783c\0\u7896\0\u659a\0\u66a8\0\u78f0\0\u794a\0\u79a4"+
"\0\u79fe\0\u7a58\0\u7ab2\0\u7b0c\0\u7b66\0\u7bc0\0\u7c1a\0\u7c74"+
"\0\u7cce\0\u7d28\0\u7d82\0\u7ddc\0\u7e36\0\u7e90\0\u7eea\0\u7f44"+
"\0\u7f9e\0\u7ff8\0\u8052\0\u80ac\0\u8106\0\u8160\0\u81ba\0\u8214"+
"\0\u826e\0\u82c8\0\u8322\0\u2058\0\u6432\0\u82c8\0\u837c\0\u83d6"+
"\0\u8430\0\u848a\0\u84e4\0\u853e\0\u8598\0\u85f2\0\u864c\0\u86a6"+
"\0\u8700\0\u49d4\0\u875a\0\u87b4\0\u880e\0\u8868\0\u88c2\0\u891c"+
"\0\u8976\0\u89d0\0\u8a2a\0\u8a84\0\u62ca\0\u4ae2\0\u8ade\0\u7350"+
"\0\u8a2a\0\u8b38\0\u8b92\0\u8bec\0\u8c46\0\u8ca0\0\u8cfa\0\u880e";
private static int [] yy_unpack_yy_rowMap() {
int [] result = new int[448];
int offset = 0;
offset = yy_unpack_yy_rowMap(yy_rowMap_packed0, offset, result);
return result;
}
private static int yy_unpack_yy_rowMap(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int high = ((int) packed.charAt(i++)) << 16;
result[j++] = high | packed.charAt(i++);
}
return j;
}
/**
* The transition table of the DFA
*/
private static final int [] yytrans = yy_unpack_yytrans();
private static final String yytrans_packed0 =
"\1\2\1\3\3\4\1\5\1\6\1\7\1\10\1\11"+
"\1\12\1\13\1\14\1\4\1\15\1\16\1\17\1\20"+
"\1\21\1\15\1\22\1\23\1\10\1\24\1\25\2\10"+
"\1\26\1\27\1\30\1\31\1\32\2\10\1\33\1\10"+
"\1\34\1\35\1\36\1\37\1\40\1\41\1\42\1\43"+
"\1\44\1\45\1\4\1\46\1\47\1\4\1\41\1\50"+
"\1\51\1\52\1\53\1\54\1\55\1\15\2\10\1\32"+
"\1\56\1\2\1\4\1\57\1\60\1\24\1\61\1\62"+
"\1\63\1\10\1\64\1\65\3\10\1\66\1\35\1\4"+
"\1\67\1\70\1\71\1\72\1\73\1\74\1\32\1\75"+
"\1\76\1\74\1\36\134\0\1\77\1\100\2\0\7\100"+
"\1\0\20\100\2\0\5\100\2\0\1\100\7\0\1\100"+
"\5\0\7\100\4\0\15\100\23\0\1\101\1\0\1\102"+
"\1\0\1\103\3\0\1\104\1\105\2\106\1\0\1\107"+
"\1\0\1\110\1\111\1\112\1\113\1\0\1\113\1\0"+
"\1\114\1\0\1\115\1\0\3\116\14\0\1\117\54\0"+
"\1\120\2\0\1\121\2\122\1\123\1\124\1\125\1\126"+
"\1\123\1\127\7\122\1\130\6\123\2\122\1\0\1\131"+
"\1\132\1\123\1\133\1\134\1\123\1\135\1\0\1\136"+
"\1\137\1\0\1\127\2\0\1\140\1\4\1\141\1\142"+
"\2\143\1\144\1\145\5\122\2\123\2\131\2\0\2\122"+
"\1\123\1\122\1\123\1\122\1\123\2\122\3\123\1\122"+
"\1\135\4\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\121\2\122\5\123\1\127\7\122\7\123\2\122\1\0"+
"\1\131\1\123\1\146\1\147\1\126\1\123\1\135\1\0"+
"\1\136\1\137\1\0\1\127\2\0\1\140\1\4\1\141"+
"\1\142\2\143\1\144\1\150\5\122\2\123\2\131\2\0"+
"\2\122\1\123\1\122\1\123\1\122\1\123\2\122\3\123"+
"\1\122\1\135\4\0\1\150\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\20\123\1\0\1\131\5\123"+
"\2\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\154\1\145\7\123\2\131\2\0\15\123\5\0"+
"\1\145\2\0\1\131\6\0\1\120\2\0\1\151\7\123"+
"\1\0\20\123\1\0\1\131\5\123\1\135\1\0\1\141"+
"\1\152\4\0\1\140\1\0\1\141\1\153\2\143\1\144"+
"\1\150\7\123\2\131\2\0\15\123\1\135\4\0\1\150"+
"\2\0\1\131\4\0\2\155\1\156\2\155\1\157\7\160"+
"\1\155\20\160\1\155\1\161\5\160\1\162\1\0\1\163"+
"\1\164\4\155\1\165\1\155\1\163\1\166\2\167\1\170"+
"\1\171\7\160\2\161\2\155\15\160\1\162\4\155\1\171"+
"\2\155\1\161\4\155\2\0\1\120\2\0\1\151\7\123"+
"\1\0\7\123\1\172\10\123\1\0\1\131\5\123\2\0"+
"\1\141\1\152\4\0\1\140\1\0\1\141\1\153\2\143"+
"\1\154\1\145\7\123\2\131\2\0\15\123\5\0\1\145"+
"\2\0\1\131\6\0\1\120\2\0\1\151\7\123\1\0"+
"\13\123\1\173\4\123\1\0\1\131\5\123\2\0\1\141"+
"\1\152\4\0\1\140\1\0\1\141\1\153\2\143\1\154"+
"\1\145\7\123\2\131\2\0\15\123\5\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\121\2\122\5\123\1\127"+
"\7\122\7\123\2\122\1\0\1\131\5\123\1\135\1\0"+
"\1\136\1\137\1\0\1\127\2\0\1\140\1\4\1\141"+
"\1\142\2\143\1\144\1\145\5\122\2\123\2\131\2\0"+
"\2\122\1\123\1\122\1\123\1\122\1\123\2\122\3\123"+
"\1\122\1\135\4\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\121\2\122\4\123\1\174\1\127\4\122\1\175"+
"\2\122\7\123\2\122\1\0\1\131\1\123\1\176\1\177"+
"\2\123\1\135\1\0\1\136\1\137\1\0\1\127\2\0"+
"\1\140\1\4\1\141\1\142\2\143\1\144\1\145\5\122"+
"\2\123\2\131\2\0\2\122\1\123\1\122\1\123\1\122"+
"\1\123\2\122\3\123\1\122\1\135\4\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\121\2\122\2\123\1\124"+
"\2\123\1\127\7\122\4\123\1\200\2\123\2\122\1\0"+
"\1\131\5\123\1\135\1\0\1\136\1\137\1\0\1\127"+
"\2\0\1\140\1\4\1\141\1\142\2\143\1\144\1\150"+
"\5\122\2\123\2\131\2\0\2\122\1\123\1\122\1\123"+
"\1\122\1\123\2\122\3\123\1\122\1\135\4\0\1\150"+
"\2\0\1\131\6\0\1\120\2\0\1\121\2\122\5\123"+
"\1\127\7\122\7\123\2\122\1\0\1\131\5\123\1\135"+
"\1\0\1\136\1\137\1\0\1\127\2\0\1\140\1\4"+
"\1\141\1\142\2\143\1\201\1\145\5\122\2\123\2\131"+
"\2\0\2\122\1\123\1\122\1\123\1\122\1\123\2\122"+
"\3\123\1\122\1\135\4\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\121\2\122\5\123\1\127\7\122\7\123"+
"\2\122\1\0\1\131\2\123\1\202\2\123\1\135\1\0"+
"\1\136\1\137\1\0\1\127\2\0\1\140\1\4\1\141"+
"\1\142\2\143\1\144\1\145\5\122\2\123\2\131\2\0"+
"\2\122\1\123\1\122\1\123\1\122\1\123\2\122\3\123"+
"\1\122\1\135\4\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\121\2\122\2\123\1\203\2\123\1\127\7\122"+
"\1\204\6\123\2\122\1\0\1\131\1\205\4\123\1\135"+
"\1\0\1\136\1\137\1\0\1\127\2\0\1\140\1\4"+
"\1\141\1\142\2\143\1\144\1\206\1\207\4\122\2\123"+
"\2\131\2\0\2\122\1\123\1\122\1\123\1\122\1\123"+
"\2\122\3\123\1\122\1\135\4\0\1\206\2\0\1\131"+
"\6\0\1\120\2\0\1\151\7\123\1\0\12\123\1\210"+
"\5\123\1\0\1\131\5\123\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\141\1\153\2\143\1\154\1\211\7\123"+
"\2\131\2\0\15\123\5\0\1\211\2\0\1\131\6\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\5\123\1\135\1\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\144\1\145\7\123\2\131\2\0"+
"\15\123\1\135\4\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\20\123\1\0\1\131\5\123"+
"\2\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\154\1\150\7\123\2\131\2\0\15\123\5\0"+
"\1\150\2\0\1\131\6\0\1\120\2\0\1\151\7\123"+
"\1\0\20\123\1\0\1\131\5\123\1\135\1\0\1\141"+
"\1\152\4\0\1\140\1\0\1\141\1\153\2\143\1\212"+
"\1\145\7\123\2\131\2\0\15\123\1\135\4\0\1\145"+
"\2\0\1\131\6\0\1\120\2\0\1\121\2\122\1\123"+
"\1\213\3\123\1\127\7\122\3\123\1\214\2\123\1\215"+
"\2\122\1\0\1\131\1\123\1\216\1\123\1\217\1\205"+
"\1\135\1\0\1\136\1\137\1\0\1\127\2\0\1\140"+
"\1\4\1\141\1\142\2\143\1\144\1\145\5\122\2\123"+
"\2\131\2\0\2\122\1\123\1\122\1\123\1\122\1\123"+
"\2\122\3\123\1\122\1\135\4\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\121\2\122\2\123\1\124\1\123"+
"\1\124\1\127\7\122\3\123\1\220\1\221\2\123\2\122"+
"\1\0\1\131\3\123\1\222\1\123\1\135\1\0\1\136"+
"\1\137\1\0\1\127\2\0\1\140\1\4\1\141\1\142"+
"\2\143\1\144\1\145\5\122\2\123\2\131\2\0\2\122"+
"\1\123\1\122\1\123\1\122\1\123\2\122\3\123\1\122"+
"\1\135\4\0\1\145\2\0\1\131\42\0\1\31\75\0"+
"\1\223\3\0\7\131\1\0\20\131\1\0\1\224\5\131"+
"\2\0\1\131\1\225\1\226\3\0\1\140\1\0\1\131"+
"\1\153\1\143\2\227\1\0\7\131\2\224\2\0\15\131"+
"\10\0\1\224\6\0\1\120\2\0\1\151\7\123\1\0"+
"\13\123\1\220\4\123\1\0\1\131\5\123\1\135\1\0"+
"\1\141\1\152\4\0\1\140\1\0\1\141\1\153\2\143"+
"\1\144\1\145\7\123\2\131\2\0\15\123\1\135\4\0"+
"\1\145\2\0\1\131\6\0\1\120\2\0\1\151\5\123"+
"\1\126\1\123\1\0\20\123\1\0\1\131\5\123\1\135"+
"\1\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\144\1\145\7\123\2\131\2\0\15\123\1\135"+
"\4\0\1\145\2\0\1\131\51\0\1\35\47\0\1\35"+
"\16\0\1\120\2\0\1\121\2\122\5\123\1\127\7\122"+
"\1\230\6\123\2\122\1\0\1\131\2\123\1\231\2\123"+
"\1\135\1\0\1\232\1\137\1\0\1\127\2\0\1\140"+
"\1\4\1\233\1\142\2\143\1\144\1\234\5\122\2\123"+
"\2\131\2\0\2\122\1\123\1\122\1\123\1\122\1\123"+
"\2\122\3\123\1\122\1\135\4\0\1\234\2\0\1\131"+
"\24\0\1\235\1\0\1\235\14\0\1\42\10\0\1\236"+
"\1\226\10\0\2\226\10\0\2\42\27\0\1\42\43\0"+
"\1\42\34\0\2\42\27\0\1\42\43\0\1\42\11\0"+
"\1\226\10\0\2\226\10\0\2\42\27\0\1\42\43\0"+
"\1\237\11\0\1\240\10\0\2\240\10\0\2\237\27\0"+
"\1\237\61\0\1\241\56\0\1\120\2\0\1\151\7\123"+
"\1\0\7\123\1\242\10\123\1\0\1\131\5\123\2\0"+
"\1\233\1\152\4\0\1\140\1\0\1\233\1\153\2\143"+
"\1\154\1\234\7\123\2\131\2\0\15\123\5\0\1\234"+
"\2\0\1\131\64\0\1\47\110\0\1\42\23\0\1\243"+
"\10\0\2\42\17\0\1\244\7\0\1\42\12\0\4\245"+
"\1\0\1\245\4\0\1\246\1\0\1\247\1\0\1\250"+
"\2\0\1\250\1\251\1\252\10\0\1\253\2\254\12\0"+
"\1\255\6\0\1\245\1\253\1\247\3\0\1\256\30\0"+
"\1\256\6\0\1\120\2\0\1\121\2\122\5\123\1\127"+
"\7\122\2\123\1\257\4\123\2\122\1\0\1\131\3\123"+
"\1\260\1\123\1\135\1\0\1\136\1\137\1\0\1\127"+
"\2\0\1\140\1\4\1\141\1\142\2\143\1\144\1\150"+
"\5\122\1\123\1\261\2\131\2\0\2\122\1\123\1\122"+
"\1\123\1\122\1\123\2\122\3\123\1\122\1\135\4\0"+
"\1\150\2\0\1\131\6\0\1\120\2\0\1\262\2\122"+
"\4\123\1\263\1\127\7\122\1\172\3\123\1\126\1\264"+
"\1\123\2\122\1\0\1\131\1\265\1\123\1\266\1\124"+
"\1\123\1\135\1\0\1\136\1\137\1\0\1\127\2\0"+
"\1\140\1\4\1\141\1\142\2\143\1\144\1\145\5\122"+
"\2\123\2\131\2\0\2\122\1\123\1\122\1\123\1\122"+
"\1\123\2\122\3\123\1\122\1\135\4\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\121\2\122\5\123\1\127"+
"\7\122\4\123\1\220\2\123\2\122\1\0\1\131\5\123"+
"\1\135\1\0\1\136\1\137\1\0\1\127\2\0\1\140"+
"\1\4\1\141\1\142\2\143\1\144\1\145\5\122\2\123"+
"\2\131\2\0\2\122\1\123\1\122\1\123\1\122\1\123"+
"\2\122\3\123\1\122\1\135\4\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\121\2\122\2\123\1\124\2\123"+
"\1\127\7\122\4\123\1\124\2\123\2\122\1\0\1\131"+
"\5\123\1\135\1\0\1\136\1\137\1\0\1\127\2\0"+
"\1\140\1\4\1\141\1\142\2\143\1\144\1\145\5\122"+
"\2\123\2\131\2\0\2\122\1\123\1\122\1\123\1\122"+
"\1\123\2\122\3\123\1\122\1\135\4\0\1\145\2\0"+
"\1\131\6\0\1\223\3\0\7\131\1\0\20\131\1\0"+
"\1\224\5\131\2\0\1\131\1\225\1\226\3\0\1\140"+
"\1\0\1\131\1\153\1\143\2\227\1\0\7\131\2\224"+
"\2\0\12\131\1\267\2\131\10\0\1\224\6\0\1\120"+
"\2\0\1\121\2\122\2\123\1\270\2\123\1\127\7\122"+
"\7\123\2\122\1\0\1\131\1\123\1\271\1\123\1\124"+
"\1\123\1\135\1\0\1\136\1\137\1\0\1\127\2\0"+
"\1\140\1\4\1\141\1\142\2\143\1\144\1\145\5\122"+
"\2\123\2\131\2\0\2\122\1\123\1\122\1\123\1\122"+
"\1\123\2\122\3\123\1\122\1\135\4\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\121\2\122\5\123\1\127"+
"\7\122\3\123\1\272\3\123\2\122\1\0\1\131\2\123"+
"\1\273\1\274\1\123\1\135\1\0\1\136\1\137\1\0"+
"\1\127\2\0\1\140\1\4\1\141\1\142\2\143\1\144"+
"\1\145\5\122\2\123\2\131\2\0\2\122\1\123\1\122"+
"\1\123\1\122\1\123\2\122\3\123\1\122\1\135\4\0"+
"\1\145\2\0\1\131\6\0\1\120\2\0\1\121\2\122"+
"\2\123\1\275\2\123\1\127\7\122\7\123\2\122\1\0"+
"\1\131\1\276\1\123\1\277\2\123\1\135\1\0\1\136"+
"\1\137\1\0\1\127\2\0\1\140\1\4\1\141\1\142"+
"\2\143\1\144\1\145\5\122\2\123\2\131\2\0\2\122"+
"\1\123\1\122\1\123\1\122\1\123\2\122\1\300\2\123"+
"\1\122\1\135\4\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\13\123\1\124\4\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\154\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\121\2\122\5\123\1\127\7\122\3\123\1\301\3\123"+
"\2\122\1\0\1\131\5\123\1\135\1\0\1\302\1\137"+
"\1\0\1\127\2\0\1\140\1\4\1\303\1\142\2\143"+
"\1\144\1\145\5\122\2\123\2\131\2\0\2\122\1\123"+
"\1\122\1\123\1\122\1\123\2\122\3\123\1\122\1\135"+
"\4\0\1\145\2\0\1\131\6\0\1\120\2\0\1\121"+
"\2\122\2\123\1\304\2\123\1\127\7\122\1\305\6\123"+
"\2\122\1\0\1\131\5\123\1\135\1\0\1\136\1\137"+
"\1\0\1\127\2\0\1\140\1\4\1\141\1\142\2\143"+
"\1\144\1\145\5\122\2\123\2\131\2\0\2\122\1\123"+
"\1\122\1\123\1\122\1\123\2\122\1\124\2\123\1\122"+
"\1\135\4\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\121\2\122\2\123\1\124\2\123\1\127\7\122\1\306"+
"\6\123\2\122\1\0\1\131\2\123\1\307\2\123\1\135"+
"\1\0\1\136\1\137\1\0\1\127\2\0\1\140\1\4"+
"\1\141\1\142\2\143\1\144\1\145\5\122\2\123\2\131"+
"\2\0\2\122\1\123\1\122\1\123\1\122\1\123\2\122"+
"\3\123\1\122\1\135\4\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\121\2\122\5\123\1\127\7\122\7\123"+
"\2\122\1\0\1\131\3\123\1\310\1\123\1\135\1\0"+
"\1\136\1\137\1\0\1\127\2\0\1\140\1\4\1\141"+
"\1\142\2\143\1\144\1\145\5\122\2\123\2\131\2\0"+
"\2\122\1\123\1\122\1\123\1\122\1\123\2\122\3\123"+
"\1\122\1\135\4\0\1\145\2\0\1\131\123\0\1\67"+
"\132\0\1\70\132\0\1\4\16\0\4\245\1\0\1\245"+
"\4\0\1\246\1\0\1\247\1\0\1\250\2\0\1\250"+
"\1\251\1\252\10\0\1\253\2\254\12\0\1\255\6\0"+
"\1\245\1\253\1\247\3\0\1\256\25\0\1\4\2\0"+
"\1\256\12\0\7\311\1\0\20\311\1\0\6\311\2\0"+
"\1\311\6\0\2\311\5\0\11\311\2\0\15\311\10\0"+
"\1\311\7\0\1\100\2\0\7\100\1\0\20\100\2\0"+
"\5\100\2\0\1\100\7\0\1\100\5\0\7\100\4\0"+
"\15\100\15\0\4\100\1\4\125\100\7\0\1\312\133\0"+
"\1\313\130\0\1\314\1\0\1\315\17\0\1\316\6\0"+
"\1\317\107\0\1\320\132\0\1\320\133\0\1\320\127\0"+
"\1\320\1\0\1\320\1\0\1\320\116\0\1\321\1\315"+
"\17\0\1\316\6\0\1\317\131\0\1\322\101\0\1\321"+
"\151\0\1\320\106\0\1\323\162\0\1\324\34\0\2\324"+
"\27\0\1\324\16\0\1\315\17\0\1\316\6\0\1\317"+
"\172\0\1\325\35\0\7\326\1\0\20\326\1\0\6\326"+
"\2\0\1\326\7\0\1\326\5\0\11\326\2\0\15\326"+
"\10\0\1\326\11\0\1\127\2\327\2\0\1\116\2\0"+
"\1\127\7\327\1\116\6\0\2\327\2\0\3\116\4\0"+
"\1\327\1\127\1\0\1\327\5\0\1\127\4\0\5\327"+
"\6\0\2\327\1\0\1\327\1\0\1\327\1\0\2\327"+
"\3\0\1\327\17\0\1\120\2\0\1\121\2\122\5\123"+
"\1\127\7\122\7\123\2\122\1\0\1\131\5\123\2\0"+
"\1\136\1\137\1\0\1\327\2\0\1\140\1\4\1\141"+
"\1\142\2\143\1\330\1\145\5\122\2\123\2\131\2\0"+
"\2\122\1\123\1\122\1\123\1\122\1\123\2\122\3\123"+
"\1\122\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\7\123\1\0\20\123\1\0\1\131\5\123\2\0"+
"\1\141\1\152\4\0\1\140\1\0\1\141\1\153\2\143"+
"\1\330\1\145\7\123\2\131\2\0\15\123\5\0\1\145"+
"\2\0\1\131\6\0\1\120\2\0\1\151\7\123\1\0"+
"\20\123\1\0\1\131\5\123\1\135\1\0\1\141\1\152"+
"\4\0\1\140\1\0\1\141\1\153\2\143\1\331\1\145"+
"\7\123\2\131\2\0\15\123\1\135\4\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\151\5\123\1\276\1\123"+
"\1\0\20\123\1\0\1\131\3\123\1\124\1\123\2\0"+
"\1\141\1\152\4\0\1\140\1\0\1\141\1\153\2\143"+
"\1\330\1\145\7\123\2\131\2\0\13\123\1\126\1\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\7\123\1\0\20\123\1\0\1\131\5\123\1\135\1\0"+
"\1\141\1\152\4\0\1\140\1\0\1\141\1\153\2\143"+
"\1\332\1\145\7\123\2\131\2\0\15\123\1\135\4\0"+
"\1\145\2\0\1\131\11\0\1\127\2\327\5\0\1\127"+
"\7\327\7\0\2\327\11\0\1\327\1\127\1\0\1\327"+
"\5\0\1\127\4\0\5\327\6\0\2\327\1\0\1\327"+
"\1\0\1\327\1\0\2\327\3\0\1\327\17\0\1\120"+
"\2\0\1\151\7\123\1\0\20\123\1\0\1\131\5\123"+
"\1\135\1\0\1\141\1\152\4\0\1\140\1\0\1\333"+
"\1\153\2\143\1\331\1\145\7\123\2\131\2\0\15\123"+
"\1\135\4\0\1\145\2\0\1\131\6\0\1\120\3\0"+
"\7\131\1\0\20\131\1\0\6\131\2\0\1\131\1\152"+
"\4\0\1\140\1\0\1\131\1\153\3\143\1\0\11\131"+
"\2\0\15\131\10\0\1\131\6\0\1\120\2\0\1\151"+
"\5\123\1\276\1\123\1\0\11\123\1\334\6\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\335\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\5\123\1\336\1\123\1\0\20\123\1\0\1\131"+
"\5\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\15\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\5\123\1\126\1\123\1\0\20\123\1\0\1\131\5\123"+
"\1\135\1\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\332\1\145\7\123\2\131\2\0\15\123"+
"\1\135\4\0\1\145\2\0\1\131\51\0\1\135\47\0"+
"\1\135\16\0\1\120\2\0\1\121\2\122\5\123\1\127"+
"\7\122\7\123\2\122\1\0\1\131\5\123\2\0\1\232"+
"\1\137\1\0\1\327\2\0\1\140\1\4\1\233\1\142"+
"\2\143\1\330\1\337\5\122\2\123\2\131\2\0\2\122"+
"\1\123\1\122\1\123\1\122\1\123\2\122\3\123\1\122"+
"\5\0\1\337\2\0\1\131\11\0\1\127\2\340\5\341"+
"\1\127\7\340\7\341\2\340\1\0\1\342\5\341\2\0"+
"\1\340\1\127\1\0\1\327\4\0\1\341\1\127\4\0"+
"\5\340\2\341\2\342\2\0\2\340\1\341\1\340\1\341"+
"\1\340\1\341\2\340\3\341\1\340\10\0\1\342\6\0"+
"\1\120\131\0\1\120\2\0\1\151\7\123\1\0\20\123"+
"\1\0\1\131\5\123\2\0\1\233\1\152\4\0\1\140"+
"\1\0\1\233\1\153\2\143\1\330\1\337\7\123\2\131"+
"\2\0\15\123\5\0\1\337\2\0\1\131\11\0\1\127"+
"\2\343\5\344\1\127\7\343\7\344\2\343\1\0\6\344"+
"\2\0\1\343\1\127\1\0\1\327\4\0\1\344\1\142"+
"\4\0\5\343\4\344\2\0\2\343\1\344\1\343\1\344"+
"\1\343\1\344\2\343\3\344\1\343\10\0\1\344\12\0"+
"\7\143\1\0\20\143\1\0\6\143\2\0\1\143\1\152"+
"\6\0\1\143\1\0\3\143\1\0\11\143\2\0\15\143"+
"\10\0\1\143\12\0\7\345\1\346\20\345\1\0\1\143"+
"\5\345\2\0\1\345\1\152\1\346\5\0\1\345\1\0"+
"\1\143\1\347\1\143\1\0\7\345\2\143\2\0\15\345"+
"\10\0\1\143\12\0\4\346\1\0\1\346\4\0\1\350"+
"\1\0\1\351\5\0\1\352\12\0\2\353\21\0\1\346"+
"\1\0\1\351\43\0\1\120\2\0\1\151\7\123\1\0"+
"\20\123\1\0\1\131\5\123\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\354\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\15\123\5\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\151\7\123\1\0\11\123\2\124\5\123"+
"\1\0\1\131\5\123\2\0\1\141\1\152\4\0\1\140"+
"\1\0\1\141\1\153\2\143\1\330\1\145\7\123\2\131"+
"\2\0\15\123\5\0\1\145\2\0\1\131\12\0\2\355"+
"\2\356\1\357\1\356\1\357\1\0\2\360\1\361\1\360"+
"\1\362\2\360\3\357\1\363\3\357\2\360\1\0\4\357"+
"\2\364\2\0\1\360\7\0\1\357\5\0\1\360\1\355"+
"\1\360\1\362\1\360\4\357\2\0\2\360\1\357\1\360"+
"\1\357\1\360\1\357\2\360\3\357\1\360\10\0\1\357"+
"\16\0\1\116\12\0\1\116\12\0\3\116\75\0\7\341"+
"\1\0\20\341\1\0\1\342\5\341\2\0\1\341\7\0"+
"\1\341\5\0\7\341\2\342\2\0\15\341\10\0\1\342"+
"\12\0\7\344\1\0\20\344\1\0\6\344\2\0\1\344"+
"\7\0\1\344\1\153\4\0\11\344\2\0\15\344\10\0"+
"\1\344\77\0\1\365\44\0\7\326\1\0\20\326\1\0"+
"\6\326\2\0\1\326\7\0\1\326\5\0\6\326\1\366"+
"\2\326\2\0\15\326\10\0\1\326\16\0\1\116\12\0"+
"\1\116\12\0\3\116\30\0\1\365\40\0\1\120\2\0"+
"\1\151\7\123\1\0\20\123\1\0\1\131\5\123\2\0"+
"\1\141\1\152\4\0\1\140\1\0\1\141\1\153\2\143"+
"\1\330\1\145\6\123\1\367\2\131\2\0\15\123\5\0"+
"\1\145\2\0\1\131\6\0\1\120\3\0\7\131\1\0"+
"\20\131\1\0\6\131\2\0\1\131\1\152\4\0\1\140"+
"\1\0\1\131\1\153\3\143\1\0\6\131\1\370\2\131"+
"\2\0\15\131\10\0\1\131\51\0\1\135\25\0\1\365"+
"\21\0\1\135\16\0\1\120\2\0\1\151\7\123\1\0"+
"\20\123\1\0\1\131\5\123\2\0\1\233\1\152\4\0"+
"\1\140\1\0\1\233\1\153\2\143\1\330\1\337\6\123"+
"\1\367\2\131\2\0\15\123\5\0\1\337\2\0\1\131"+
"\12\0\7\341\1\0\20\341\1\0\1\342\5\341\2\0"+
"\1\341\7\0\1\341\5\0\6\341\1\371\2\342\2\0"+
"\15\341\10\0\1\342\6\0\1\120\70\0\1\365\44\0"+
"\7\344\1\0\20\344\1\0\6\344\2\0\1\344\7\0"+
"\1\344\1\153\4\0\6\344\1\372\2\344\2\0\15\344"+
"\10\0\1\344\12\0\7\143\1\0\20\143\1\0\6\143"+
"\2\0\1\143\1\152\6\0\1\143\1\0\3\143\1\0"+
"\6\143\1\373\2\143\2\0\15\143\10\0\1\143\12\0"+
"\2\345\1\374\4\345\1\346\20\345\1\0\1\143\5\345"+
"\2\0\1\345\1\152\1\346\5\0\1\345\1\0\1\143"+
"\1\347\1\143\1\0\6\345\1\375\2\143\2\0\15\345"+
"\10\0\1\143\12\0\4\346\1\0\1\346\4\0\1\350"+
"\1\0\1\351\5\0\1\352\12\0\2\353\21\0\1\346"+
"\1\0\1\351\2\0\1\365\40\0\1\120\2\0\1\151"+
"\2\123\1\376\4\123\1\0\20\123\1\0\1\131\5\123"+
"\2\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\330\1\145\7\123\2\131\2\0\15\123\5\0"+
"\1\145\2\0\1\131\6\0\1\120\2\0\1\151\7\123"+
"\1\0\13\123\1\377\4\123\1\0\1\131\5\123\2\0"+
"\1\141\1\152\4\0\1\140\1\0\1\141\1\153\2\143"+
"\1\330\1\145\7\123\2\131\2\0\15\123\5\0\1\145"+
"\2\0\1\131\6\0\1\120\2\0\1\151\7\123\1\0"+
"\20\123\1\0\1\131\1\123\1\u0100\3\123\2\0\1\141"+
"\1\152\4\0\1\140\1\0\1\141\1\153\2\143\1\330"+
"\1\145\7\123\2\131\2\0\15\123\5\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\121\2\122\5\123\1\127"+
"\7\122\7\123\2\122\1\0\1\131\5\123\2\0\1\136"+
"\1\137\1\0\1\327\2\0\1\140\1\4\1\141\1\142"+
"\2\143\1\330\1\145\2\122\1\u0101\2\122\2\123\2\131"+
"\2\0\2\122\1\123\1\122\1\123\1\122\1\123\2\122"+
"\3\123\1\122\5\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\20\123\1\0\1\131\2\123"+
"\1\u0102\2\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\7\123\1\0\20\123\1\0\1\131\5\123\2\0"+
"\1\141\1\152\4\0\1\140\1\0\1\335\1\153\2\143"+
"\1\330\1\145\7\123\2\131\2\0\12\123\1\124\2\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\3\123\1\124\3\123\1\0\20\123\1\0\1\131\5\123"+
"\1\135\1\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\332\1\145\7\123\2\131\2\0\15\123"+
"\1\135\4\0\1\145\2\0\1\131\12\0\7\345\1\346"+
"\20\345\1\0\1\143\5\345\2\0\1\345\1\152\1\346"+
"\5\0\1\345\1\0\1\143\1\347\1\143\1\0\1\345"+
"\1\u0103\5\345\2\143\2\0\15\345\10\0\1\143\6\0"+
"\1\120\2\0\1\151\7\123\1\0\15\123\1\134\2\123"+
"\1\0\1\131\4\123\1\126\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\141\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\15\123\5\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\151\7\123\1\0\12\123\1\u0104\2\123"+
"\1\264\2\123\1\0\1\131\5\123\2\0\1\141\1\152"+
"\4\0\1\140\1\0\1\u0105\1\153\2\143\1\330\1\145"+
"\7\123\2\131\2\0\15\123\5\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\151\5\123\1\124\1\123\1\0"+
"\12\123\1\u0106\5\123\1\0\1\131\3\123\1\u0107\1\123"+
"\1\135\1\0\1\141\1\152\4\0\1\140\1\0\1\335"+
"\1\153\2\143\1\331\1\145\7\123\2\131\2\0\15\123"+
"\1\135\4\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\7\123\1\0\20\123\1\0\1\131\2\123\1\126"+
"\2\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\15\123"+
"\5\0\1\145\2\0\1\131\12\0\1\u0108\1\346\1\u0108"+
"\1\346\1\0\1\346\4\0\1\350\1\0\1\351\5\0"+
"\1\352\12\0\2\353\21\0\1\346\1\0\1\351\43\0"+
"\1\120\2\0\1\121\2\122\5\123\1\127\4\122\1\u0109"+
"\2\122\7\123\2\122\1\0\1\131\5\123\1\135\1\0"+
"\1\136\1\137\1\0\1\327\2\0\1\140\1\4\1\141"+
"\1\142\2\143\1\331\1\145\1\122\1\u010a\3\122\2\123"+
"\2\131\2\0\2\122\1\123\1\122\1\123\1\122\1\123"+
"\2\122\3\123\1\122\1\135\4\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\151\7\123\1\0\20\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\u010b\7\123\2\131\2\0"+
"\15\123\5\0\1\u010b\2\0\1\131\12\0\4\346\1\0"+
"\1\346\4\0\1\350\1\0\1\351\4\0\1\u010c\1\352"+
"\12\0\2\353\21\0\1\346\1\0\1\351\47\0\2\345"+
"\1\374\4\345\1\346\20\345\1\0\1\143\5\345\2\u010d"+
"\1\345\1\152\1\346\5\0\1\345\1\0\1\143\1\347"+
"\1\143\1\0\7\345\2\143\2\0\15\345\1\u010d\7\0"+
"\1\143\6\0\1\120\2\0\1\151\2\123\1\126\4\123"+
"\1\0\20\123\1\0\1\131\5\123\2\0\1\141\1\152"+
"\4\0\1\140\1\0\1\141\1\153\2\143\1\330\1\145"+
"\7\123\2\131\2\0\15\123\5\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\151\4\123\1\124\2\123\1\0"+
"\20\123\1\0\1\131\2\123\1\u010e\2\123\2\0\1\141"+
"\1\152\4\0\1\140\1\0\1\141\1\153\2\143\1\330"+
"\1\145\7\123\2\131\2\0\15\123\5\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\151\7\123\1\0\20\123"+
"\1\0\1\131\3\123\1\124\1\123\2\0\1\141\1\152"+
"\4\0\1\140\1\0\1\141\1\153\2\143\1\330\1\145"+
"\7\123\2\131\2\0\15\123\5\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\151\7\123\1\0\14\123\1\124"+
"\3\123\1\0\1\131\5\123\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\141\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\15\123\5\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\1\u010f\4\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\6\123\1\124\2\131"+
"\2\0\15\123\5\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\11\123\1\124\6\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\7\123\1\0\20\123\1\0\1\131\5\123\2\0"+
"\1\141\1\152\4\0\1\140\1\0\1\141\1\153\2\143"+
"\1\330\1\145\7\123\2\131\2\0\11\123\1\124\3\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\7\123\1\0\7\123\1\u0110\10\123\1\0\1\131\5\123"+
"\2\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\330\1\145\7\123\2\131\2\0\15\123\5\0"+
"\1\145\2\0\1\131\12\0\7\326\1\0\20\326\1\0"+
"\1\u0111\5\326\2\0\1\326\7\0\1\326\5\0\7\326"+
"\2\u0111\2\0\15\326\10\0\1\u0111\6\0\1\223\3\0"+
"\7\131\1\0\20\131\1\0\1\u0112\5\131\2\0\1\131"+
"\1\225\1\226\3\0\1\140\1\0\1\131\1\153\1\143"+
"\2\227\1\0\7\131\2\u0112\2\0\15\131\10\0\1\u0112"+
"\12\0\7\341\1\0\20\341\1\0\1\u0113\5\341\2\0"+
"\1\341\7\0\1\341\5\0\7\341\2\u0113\2\0\15\341"+
"\10\0\1\u0113\12\0\7\143\1\0\20\143\1\0\1\u0114"+
"\5\143\2\0\1\143\1\152\6\0\1\143\1\0\3\143"+
"\1\0\7\143\2\u0114\2\0\15\143\10\0\1\u0114\6\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\4\123\1\124\1\135\1\0\1\141\1\152\4\0\1\140"+
"\1\0\1\141\1\153\2\143\1\331\1\145\7\123\2\131"+
"\2\0\15\123\1\135\4\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\4\123\1\124\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\2\123\1\124\12\123\5\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\121\2\122\5\123\1\127\7\122\7\123"+
"\2\122\1\0\1\131\5\123\2\0\1\232\1\137\1\0"+
"\1\327\2\0\1\140\1\4\1\233\1\142\2\143\1\330"+
"\1\145\5\122\2\123\2\131\2\0\2\122\1\123\1\122"+
"\1\123\1\122\1\123\2\122\3\123\1\122\5\0\1\145"+
"\2\0\1\131\6\0\1\120\2\0\1\151\7\123\1\0"+
"\20\123\1\0\1\131\5\123\2\0\1\233\1\152\4\0"+
"\1\140\1\0\1\233\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\15\123\5\0\1\145\2\0\1\131\12\0"+
"\4\346\1\0\1\346\3\0\1\4\1\350\1\0\1\351"+
"\5\0\1\352\1\4\11\0\2\353\21\0\1\346\1\0"+
"\1\351\63\0\1\u0115\1\0\1\u0115\41\0\1\u0115\113\0"+
"\1\236\120\0\1\u0116\11\0\1\240\2\0\1\4\5\0"+
"\2\240\10\0\2\u0116\27\0\1\u0116\43\0\1\u0117\34\0"+
"\2\u0117\27\0\1\u0117\6\0\1\120\2\0\1\151\7\123"+
"\1\0\20\123\1\0\1\131\5\123\2\0\1\141\1\152"+
"\4\0\1\140\1\0\1\u0118\1\153\2\143\1\330\1\145"+
"\7\123\2\131\2\0\15\123\5\0\1\145\2\0\1\131"+
"\67\0\1\u0119\131\0\1\u011a\46\0\6\346\7\0\1\346"+
"\20\0\2\346\5\0\2\346\1\0\7\346\1\0\5\346"+
"\7\0\4\346\15\0\15\346\20\0\1\245\200\0\1\245"+
"\54\0\1\u011b\147\0\1\245\141\0\1\u011c\101\0\1\4"+
"\163\0\1\245\153\0\1\4\35\0\1\4\104\0\1\u011d"+
"\36\0\1\120\2\0\1\151\7\123\1\0\13\123\1\124"+
"\4\123\1\0\1\131\5\123\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\141\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\15\123\5\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\2\123\1\124\2\123\2\0\1\141\1\152\4\0\1\140"+
"\1\0\1\141\1\153\2\143\1\330\1\145\7\123\2\131"+
"\2\0\15\123\5\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\12\123\1\272\5\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\11\0\1\127\2\327"+
"\2\0\1\116\2\0\1\127\2\327\1\u011e\4\327\1\116"+
"\6\0\2\327\2\0\3\116\4\0\1\327\1\127\1\0"+
"\1\327\5\0\1\127\4\0\5\327\6\0\2\327\1\0"+
"\1\327\1\0\1\327\1\0\2\327\3\0\1\327\17\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\1\u011f\4\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\7\123\1\0\13\123\1\126\4\123\1\0\1\131"+
"\5\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\15\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\7\123\1\0\20\123\1\0\1\131\5\123\2\0\1\141"+
"\1\152\4\0\1\140\1\0\1\u0120\1\153\2\143\1\330"+
"\1\145\7\123\2\131\2\0\15\123\5\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\151\7\123\1\0\15\123"+
"\1\u0121\2\123\1\0\1\131\5\123\2\0\1\141\1\152"+
"\4\0\1\140\1\0\1\u0122\1\153\2\143\1\330\1\145"+
"\7\123\2\131\2\0\15\123\5\0\1\145\2\0\1\131"+
"\6\0\1\120\3\0\7\131\1\0\20\131\1\0\6\131"+
"\2\0\1\131\1\152\4\0\1\140\1\0\1\131\1\153"+
"\3\143\1\0\11\131\2\0\15\131\10\0\1\u0123\6\0"+
"\1\120\2\0\1\151\7\123\1\0\15\123\1\u0124\2\123"+
"\1\0\1\131\5\123\2\0\1\141\1\152\4\0\1\140"+
"\1\0\1\333\1\153\2\143\1\330\1\145\7\123\2\131"+
"\2\0\15\123\5\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\12\123\1\124\5\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\333\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\4\123\1\124\2\123\1\0\20\123\1\0\1\131"+
"\5\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\15\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\7\123\1\0\20\123\1\0\1\131\5\123\2\0\1\141"+
"\1\152\4\0\1\140\1\0\1\141\1\153\2\143\1\330"+
"\1\145\7\123\2\131\2\0\2\123\1\124\12\123\5\0"+
"\1\145\2\0\1\131\6\0\1\120\2\0\1\151\7\123"+
"\1\0\20\123\1\0\1\131\1\124\4\123\2\0\1\141"+
"\1\152\4\0\1\140\1\0\1\141\1\153\2\143\1\330"+
"\1\145\7\123\2\131\2\0\15\123\5\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\151\5\123\1\334\1\123"+
"\1\0\20\123\1\0\1\131\5\123\2\0\1\141\1\152"+
"\4\0\1\140\1\0\1\141\1\153\2\143\1\330\1\145"+
"\7\123\2\131\2\0\15\123\5\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\151\5\123\1\124\1\123\1\0"+
"\20\123\1\0\1\131\5\123\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\141\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\15\123\5\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\151\3\123\1\124\3\123\1\0\20\123"+
"\1\0\1\131\5\123\2\0\1\141\1\152\4\0\1\140"+
"\1\0\1\141\1\153\2\143\1\330\1\145\7\123\2\131"+
"\2\0\15\123\5\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\7\123\1\124\10\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\7\123\1\0\12\123\1\124\5\123\1\0\1\131"+
"\5\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\15\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\121"+
"\2\122\5\123\1\127\6\122\1\u010a\7\123\2\122\1\0"+
"\1\131\5\123\2\0\1\232\1\137\1\0\1\327\2\0"+
"\1\140\1\4\1\233\1\142\2\143\1\330\1\337\5\122"+
"\2\123\2\131\2\0\2\122\1\123\1\122\1\123\1\122"+
"\1\123\2\122\3\123\1\122\5\0\1\337\2\0\1\131"+
"\6\0\1\120\2\0\1\151\3\123\1\124\3\123\1\0"+
"\11\123\1\124\6\123\1\0\1\131\5\123\2\0\1\233"+
"\1\152\4\0\1\140\1\0\1\233\1\153\2\143\1\330"+
"\1\337\7\123\2\131\2\0\15\123\5\0\1\337\2\0"+
"\1\131\6\0\1\120\2\0\1\151\7\123\1\0\20\123"+
"\1\0\1\131\5\123\2\0\1\141\1\152\4\0\1\140"+
"\1\0\1\333\1\153\2\143\1\330\1\145\7\123\2\131"+
"\2\0\15\123\5\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\20\123\1\0\1\131\3\123"+
"\1\u0125\1\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\7\123\1\0\20\123\1\0\1\131\4\123\1\126"+
"\2\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\330\1\145\7\123\2\131\2\0\15\123\5\0"+
"\1\145\2\0\1\131\6\0\1\120\2\0\1\151\7\123"+
"\1\0\20\123\1\0\1\131\5\123\2\0\1\141\1\152"+
"\4\0\1\140\1\0\1\u0126\1\153\2\143\1\330\1\145"+
"\7\123\2\131\2\0\15\123\5\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\151\7\123\1\0\7\123\1\276"+
"\10\123\1\0\1\131\5\123\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\141\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\15\123\5\0\1\145\2\0\1\131\21\0"+
"\1\u0127\126\0\1\u0128\152\0\1\320\125\0\1\u0129\145\0"+
"\1\u012a\76\0\1\u012b\136\0\1\4\142\0\1\320\130\0"+
"\1\u012c\141\0\1\320\111\0\1\4\21\0\1\324\34\0"+
"\2\324\27\0\1\324\17\0\1\u012d\124\0\7\326\1\0"+
"\20\326\1\0\6\326\2\0\1\326\1\u012e\6\0\1\326"+
"\5\0\11\326\2\0\15\326\10\0\1\326\12\0\7\143"+
"\1\346\20\143\1\0\6\143\2\0\1\143\1\152\1\346"+
"\5\0\1\143\1\0\1\143\1\347\1\143\1\0\11\143"+
"\2\0\15\143\10\0\1\143\12\0\7\143\1\346\20\143"+
"\1\0\6\143\2\u010d\1\143\1\152\1\346\5\0\1\143"+
"\1\0\1\143\1\347\1\143\1\0\11\143\2\0\15\143"+
"\1\u010d\7\0\1\143\6\0\1\120\2\0\1\151\7\123"+
"\1\0\20\123\1\0\1\131\5\123\1\135\1\0\1\233"+
"\1\152\4\0\1\140\1\0\1\233\1\153\2\143\1\331"+
"\1\337\7\123\2\131\2\0\15\123\1\135\4\0\1\337"+
"\2\0\1\131\6\0\1\120\2\0\1\151\6\123\1\124"+
"\1\0\20\123\1\0\1\131\5\123\2\0\1\141\1\152"+
"\4\0\1\140\1\0\1\141\1\153\2\143\1\330\1\145"+
"\7\123\2\131\2\0\15\123\5\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\151\7\123\1\0\20\123\1\0"+
"\1\131\5\123\2\0\1\233\1\152\4\0\1\140\1\0"+
"\1\u012f\1\153\2\143\1\330\1\337\7\123\2\131\2\0"+
"\15\123\5\0\1\337\2\0\1\131\6\0\1\120\2\0"+
"\1\151\5\123\1\u0130\1\123\1\0\20\123\1\0\1\131"+
"\5\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\15\123"+
"\5\0\1\145\2\0\1\131\12\0\4\346\1\0\1\346"+
"\3\0\1\u0131\1\350\1\0\1\351\5\0\1\352\1\u0131"+
"\11\0\2\353\21\0\1\346\1\0\1\351\41\0\5\346"+
"\1\u0132\2\u0133\5\342\1\u0132\7\u0133\7\342\2\u0133\1\346"+
"\6\342\2\346\1\u0133\1\u0134\1\346\1\327\4\346\1\342"+
"\1\u0132\2\346\1\u0135\1\346\5\u0133\4\342\2\346\2\u0133"+
"\1\342\1\u0133\1\342\1\u0133\1\342\2\u0133\3\342\1\u0133"+
"\10\346\1\342\12\346\7\342\1\346\20\342\1\346\6\342"+
"\2\346\1\342\1\u0136\1\346\1\0\4\346\1\342\3\346"+
"\1\u0135\1\346\11\342\2\346\15\342\10\346\1\342\12\346"+
"\7\342\1\346\20\342\1\346\6\342\2\346\1\342\1\u0136"+
"\1\346\1\0\4\346\1\342\3\346\1\0\1\346\11\342"+
"\2\346\15\342\10\346\1\342\4\346\6\0\7\143\1\0"+
"\20\143\1\0\6\143\2\u0137\1\143\1\152\6\0\1\143"+
"\1\0\2\143\1\u0138\1\0\11\143\2\0\15\143\1\u0137"+
"\7\0\1\143\24\0\1\346\200\0\1\346\72\0\1\346"+
"\143\0\1\346\71\0\1\120\2\0\1\151\7\123\1\0"+
"\20\123\1\0\1\131\5\123\2\0\1\233\1\152\4\0"+
"\1\140\1\0\1\233\1\153\2\143\1\330\1\337\6\123"+
"\1\u0139\2\131\2\0\15\123\5\0\1\337\2\0\1\131"+
"\11\0\1\u013a\2\u013b\5\u013c\1\u013a\7\u013b\7\u013c\2\u013b"+
"\1\0\6\u013c\2\0\1\u013b\1\u013a\1\0\1\u013a\4\0"+
"\1\u013c\1\u013d\4\0\5\u013b\4\u013c\2\0\2\u013b\1\u013c"+
"\1\u013b\1\u013c\1\u013b\1\u013c\2\u013b\3\u013c\1\u013b\10\0"+
"\1\u013c\12\0\7\u013c\1\0\20\u013c\1\0\6\u013c\2\0"+
"\1\u013c\7\0\1\u013c\1\357\4\0\11\u013c\2\0\15\u013c"+
"\10\0\1\u013c\11\0\1\u013a\2\u013b\5\u013c\1\u013a\2\u013b"+
"\1\u013e\4\u013b\7\u013c\2\u013b\1\0\6\u013c\2\0\1\u013b"+
"\1\u013a\1\0\1\u013a\4\0\1\u013c\1\u013d\4\0\5\u013b"+
"\4\u013c\2\0\2\u013b\1\u013c\1\u013b\1\u013c\1\u013b\1\u013c"+
"\2\u013b\3\u013c\1\u013b\10\0\1\u013c\11\0\1\u013a\2\u013b"+
"\5\u013c\1\u013a\7\u013b\7\u013c\2\u013b\1\0\6\u013c\2\0"+
"\1\u013b\1\u013a\1\0\1\u013a\4\0\1\u013c\1\u013d\4\0"+
"\2\u013b\1\u013e\2\u013b\4\u013c\2\0\2\u013b\1\u013c\1\u013b"+
"\1\u013c\1\u013b\1\u013c\2\u013b\3\u013c\1\u013b\10\0\1\u013c"+
"\12\0\7\u013c\1\0\12\u013c\1\u013f\5\u013c\1\0\6\u013c"+
"\2\0\1\u013c\7\0\1\u013c\1\357\4\0\11\u013c\2\0"+
"\15\u013c\10\0\1\u013c\12\0\7\u013c\1\0\20\u013c\1\0"+
"\3\u013c\1\u013f\2\u013c\2\0\1\u013c\7\0\1\u013c\1\357"+
"\4\0\11\u013c\2\0\15\u013c\10\0\1\u013c\4\0\46\u0140"+
"\1\0\71\u0140\7\u0141\1\u0140\20\u0141\1\u0140\6\u0141\1\u0140"+
"\1\0\1\u0141\1\u0142\6\u0140\1\u0141\5\u0140\11\u0141\2\u0140"+
"\15\u0141\10\u0140\1\u0141\6\u0140\1\u0143\2\u0140\1\u0144\7\u0145"+
"\1\u0140\20\u0145\1\u0140\1\u0146\5\u0145\1\u0140\1\0\1\u0147"+
"\1\u0148\4\u0140\1\u0149\1\u0140\1\u0147\1\u014a\2\u014b\1\u014c"+
"\1\u014d\7\u0145\2\u0146\2\u0140\15\u0145\5\u0140\1\u014d\2\u0140"+
"\1\u0146\6\u0140\1\u0143\3\u0140\7\u0146\1\u0140\20\u0146\1\u0140"+
"\6\u0146\1\u0140\1\0\1\u0146\1\u0148\4\u0140\1\u0149\1\u0140"+
"\1\u0146\1\u014a\3\u014b\1\u0140\11\u0146\2\u0140\15\u0146\10\u0140"+
"\1\u0146\4\u0140\6\u014e\7\u014f\1\u014e\20\u014f\1\u014e\6\u014f"+
"\1\u014e\1\346\1\u014f\1\u0150\1\u014e\1\u0140\4\u014e\1\u014f"+
"\3\u014e\1\u0151\1\u014e\11\u014f\2\u014e\15\u014f\10\u014e\1\u014f"+
"\4\u014e\6\u0140\7\u0152\1\u0140\20\u0152\1\u0140\6\u0152\1\u0140"+
"\1\0\1\u0152\7\u0140\1\u0152\1\u014a\4\u0140\11\u0152\2\u0140"+
"\15\u0152\10\u0140\1\u0152\12\u0140\7\u014b\1\u0140\20\u014b\1\u0140"+
"\6\u014b\1\u0140\1\0\1\u014b\1\u0148\6\u0140\1\u014b\1\u0140"+
"\3\u014b\1\u0140\11\u014b\2\u0140\15\u014b\10\u0140\1\u014b\4\u0140"+
"\6\0\7\143\1\0\20\143\1\0\6\143\2\u0137\1\143"+
"\1\152\6\0\1\143\1\0\2\143\1\u0153\1\0\11\143"+
"\2\0\15\143\1\u0137\7\0\1\143\4\0\6\u0140\7\u014b"+
"\1\u0140\20\u014b\1\u0140\6\u014b\1\u0154\1\u0137\1\u014b\1\u0148"+
"\6\u0140\1\u014b\1\u0140\2\u014b\1\u0138\1\u0140\11\u014b\2\u0140"+
"\15\u014b\1\u0154\7\u0140\1\u014b\4\u0140\2\0\1\120\2\0"+
"\1\151\7\123\1\0\20\123\1\0\1\131\2\123\1\u0155"+
"\2\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\15\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\7\123\1\0\15\123\1\u0156\2\123\1\0\1\131\5\123"+
"\2\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\330\1\145\7\123\2\131\2\0\15\123\5\0"+
"\1\145\2\0\1\131\6\0\1\120\2\0\1\151\7\123"+
"\1\0\20\123\1\0\1\131\3\123\1\276\1\123\1\135"+
"\1\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\331\1\145\7\123\2\131\2\0\15\123\1\135"+
"\4\0\1\145\2\0\1\131\6\0\1\120\2\0\1\121"+
"\2\122\5\123\1\127\7\122\7\123\1\u0157\1\122\1\0"+
"\1\131\5\123\2\0\1\136\1\137\1\0\1\327\2\0"+
"\1\140\1\4\1\141\1\142\2\143\1\330\1\145\5\122"+
"\2\123\2\131\2\0\2\122\1\123\1\122\1\123\1\122"+
"\1\123\2\122\3\123\1\122\5\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\151\5\123\1\124\1\123\1\0"+
"\20\123\1\0\1\131\5\123\1\135\1\0\1\141\1\152"+
"\4\0\1\140\1\0\1\141\1\153\2\143\1\331\1\145"+
"\7\123\2\131\2\0\15\123\1\135\4\0\1\145\2\0"+
"\1\131\12\0\7\143\1\0\20\143\1\0\6\143\2\u0137"+
"\1\143\1\152\6\0\1\143\1\0\2\143\1\u0158\1\0"+
"\11\143\2\0\15\143\1\u0137\7\0\1\143\6\0\1\120"+
"\2\0\1\151\7\123\1\0\20\123\1\0\1\131\1\u0159"+
"\4\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\15\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\4\123\1\u015a\2\123\1\0\20\123\1\0\1\131\5\123"+
"\2\0\1\233\1\152\4\0\1\140\1\0\1\233\1\153"+
"\2\143\1\330\1\337\7\123\2\131\2\0\15\123\5\0"+
"\1\337\2\0\1\131\6\0\1\120\2\0\1\151\7\123"+
"\1\0\7\123\1\124\10\123\1\0\1\131\5\123\1\135"+
"\1\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\332\1\145\7\123\2\131\2\0\15\123\1\135"+
"\4\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\7\123\1\0\15\123\1\124\2\123\1\0\1\131\5\123"+
"\2\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\330\1\145\7\123\2\131\2\0\15\123\5\0"+
"\1\145\2\0\1\131\31\0\1\u015b\37\0\1\u015b\46\0"+
"\1\120\2\0\1\121\2\122\5\123\1\127\7\122\7\123"+
"\1\122\1\u010a\1\0\1\131\5\123\2\0\1\136\1\137"+
"\1\0\1\327\2\0\1\140\1\4\1\141\1\142\2\143"+
"\1\330\1\145\5\122\2\123\2\131\2\0\2\122\1\123"+
"\1\122\1\123\1\122\1\123\2\122\3\123\1\122\5\0"+
"\1\145\2\0\1\131\6\0\1\120\2\0\1\121\2\122"+
"\5\123\1\127\7\122\7\123\2\122\1\0\1\131\5\123"+
"\1\135\1\0\1\136\1\137\1\0\1\327\2\0\1\140"+
"\1\4\1\141\1\142\2\143\1\331\1\145\5\122\2\123"+
"\2\131\2\0\2\122\1\123\1\122\1\123\1\122\1\123"+
"\2\122\3\123\1\122\1\135\4\0\1\145\2\0\1\131"+
"\34\0\1\u015c\102\0\1\u015d\4\0\2\u015e\6\0\7\u015e"+
"\7\0\2\u015e\7\0\2\u015f\1\u015e\15\0\5\u015e\6\0"+
"\2\u015e\1\0\1\u015e\1\0\1\u015e\1\0\2\u015e\3\0"+
"\1\u015e\1\u015f\16\0\1\120\2\0\1\151\7\123\1\0"+
"\20\123\1\0\1\131\5\123\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\141\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\12\123\1\126\2\123\5\0\1\145\2\0"+
"\1\131\6\0\1\120\2\0\1\151\7\123\1\0\20\123"+
"\1\0\1\131\5\123\2\0\1\141\1\152\4\0\1\140"+
"\1\0\1\141\1\153\2\143\1\330\1\145\7\123\2\131"+
"\2\0\6\123\1\124\6\123\5\0\1\145\2\0\1\131"+
"\6\0\1\120\2\0\1\151\7\123\1\0\20\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\4\123\1\126\10\123\5\0\1\145\2\0\1\131\6\0"+
"\1\u0160\3\0\7\326\1\0\20\326\1\0\1\u0161\5\326"+
"\2\0\1\326\1\u0162\6\0\1\326\5\0\7\326\2\u0161"+
"\2\0\15\326\10\0\1\u0161\6\0\1\120\3\0\7\131"+
"\1\0\20\131\1\0\1\u0112\5\131\2\0\1\131\1\152"+
"\1\226\3\0\1\140\1\0\1\131\1\153\1\143\2\227"+
"\1\0\7\131\2\u0112\2\0\15\131\10\0\1\u0112\4\0"+
"\2\346\1\u0163\3\346\7\342\1\346\20\342\1\346\1\u0164"+
"\5\342\2\346\1\342\1\u0165\1\346\1\0\4\346\1\342"+
"\3\346\1\0\1\346\7\342\2\u0164\2\346\15\342\10\346"+
"\1\u0164\4\346\6\0\7\143\1\0\20\143\1\0\1\u0114"+
"\5\143\2\0\1\143\1\152\1\226\5\0\1\143\1\0"+
"\1\143\2\227\1\0\7\143\2\u0114\2\0\15\143\10\0"+
"\1\u0114\120\0\1\u0166\54\0\1\u0167\11\0\1\240\2\0"+
"\1\4\5\0\2\240\10\0\2\u0167\27\0\1\u0167\43\0"+
"\1\u0117\11\0\1\240\2\0\1\4\5\0\2\240\10\0"+
"\2\u0117\27\0\1\u0117\6\0\1\120\2\0\1\151\7\123"+
"\1\0\20\123\1\0\1\131\5\123\2\0\1\233\1\u0168"+
"\4\0\1\140\1\0\1\233\1\153\2\143\1\330\1\337"+
"\7\123\2\131\2\0\15\123\5\0\1\337\2\0\1\131"+
"\67\0\1\u0169\163\0\1\u016a\55\0\1\u016b\120\0\1\u016c"+
"\114\0\1\4\123\0\1\127\2\327\3\0\1\u016d\1\0"+
"\1\127\7\327\7\0\2\327\11\0\1\327\1\127\1\0"+
"\1\327\5\0\1\127\4\0\5\327\6\0\2\327\1\0"+
"\1\327\1\0\1\327\1\0\2\327\3\0\1\327\17\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\5\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\u016e\7\123\2\131\2\0\15\123"+
"\5\0\1\u016e\2\0\1\131\6\0\1\120\2\0\1\151"+
"\7\123\1\0\7\123\1\u016f\10\123\1\0\1\131\5\123"+
"\2\0\1\233\1\152\4\0\1\140\1\0\1\233\1\153"+
"\2\143\1\330\1\337\7\123\2\131\2\0\15\123\5\0"+
"\1\337\2\0\1\131\6\0\1\120\2\0\1\151\7\123"+
"\1\0\13\123\1\124\4\123\1\0\1\131\5\123\1\135"+
"\1\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\331\1\145\7\123\2\131\2\0\15\123\1\135"+
"\4\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\5\123\1\126\1\123\1\0\20\123\1\0\1\131\5\123"+
"\1\135\1\0\1\233\1\152\4\0\1\140\1\0\1\233"+
"\1\153\2\143\1\332\1\337\7\123\2\131\2\0\15\123"+
"\1\135\4\0\1\337\2\0\1\131\6\0\1\120\3\0"+
"\7\131\1\0\20\131\1\0\6\131\2\0\1\131\1\152"+
"\4\0\1\140\1\0\1\131\1\153\3\143\1\0\11\131"+
"\2\0\4\131\1\u0170\10\131\10\0\1\131\6\0\1\120"+
"\2\0\1\151\4\123\1\242\2\123\1\0\20\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\151\7\123\1\0\20\123\1\0\1\131\2\123\1\u0124"+
"\2\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\15\123"+
"\5\0\1\145\2\0\1\131\6\0\1\120\2\0\1\151"+
"\7\123\1\0\20\123\1\0\1\131\5\123\1\135\1\0"+
"\1\233\1\152\4\0\1\140\1\0\1\233\1\153\2\143"+
"\1\332\1\337\7\123\2\131\2\0\15\123\1\135\4\0"+
"\1\337\2\0\1\131\17\0\1\u0171\157\0\1\u0172\102\0"+
"\1\u0173\147\0\1\u0174\132\0\1\u0175\133\0\1\u0176\104\0"+
"\7\u0177\1\0\20\u0177\1\0\6\u0177\2\0\1\u0177\7\0"+
"\1\u0177\5\0\11\u0177\2\0\15\u0177\10\0\1\u0177\6\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\5\123\1\135\1\0\1\233\1\152\4\0\1\140\1\0"+
"\1\233\1\153\2\143\1\331\1\145\7\123\2\131\2\0"+
"\15\123\1\135\4\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\7\123\1\0\20\123\1\0\1\131\3\123"+
"\1\u0178\1\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\4\0\5\346\1\u0132"+
"\2\u0133\5\342\1\u0132\7\u0133\7\342\2\u0133\1\346\6\342"+
"\2\346\1\u0133\1\u0134\1\346\1\327\4\346\1\342\1\u0132"+
"\2\346\1\0\1\346\5\u0133\4\342\2\346\2\u0133\1\342"+
"\1\u0133\1\342\1\u0133\1\342\2\u0133\3\342\1\u0133\10\346"+
"\1\342\4\346\6\0\7\u0179\1\0\20\u0179\2\0\5\u0179"+
"\2\0\1\u0179\7\0\1\u0179\5\0\7\u0179\4\0\15\u0179"+
"\62\0\2\u0137\46\0\1\u0137\22\0\7\345\1\0\20\345"+
"\1\0\1\143\5\345\2\0\1\345\1\152\6\0\1\345"+
"\1\0\3\143\1\0\7\345\2\143\2\0\15\345\10\0"+
"\1\143\6\0\1\120\2\0\1\151\7\123\1\0\20\123"+
"\1\0\1\131\1\u017a\4\123\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\141\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\15\123\5\0\1\145\2\0\1\131\11\0"+
"\1\u013a\2\u017b\5\0\1\u013a\7\u017b\7\0\2\u017b\11\0"+
"\1\u017b\1\u013a\1\0\1\u017b\5\0\1\u013a\4\0\5\u017b"+
"\6\0\2\u017b\1\0\1\u017b\1\0\1\u017b\1\0\2\u017b"+
"\3\0\1\u017b\15\0\5\346\1\u017c\2\u013b\5\u013c\1\u017c"+
"\7\u013b\7\u013c\2\u013b\1\346\6\u013c\2\346\1\u013b\1\u017c"+
"\1\346\1\u017b\4\346\1\u013c\1\u017d\2\346\1\0\1\346"+
"\5\u013b\4\u013c\2\346\2\u013b\1\u013c\1\u013b\1\u013c\1\u013b"+
"\1\u013c\2\u013b\3\u013c\1\u013b\10\346\1\u013c\12\346\7\u013c"+
"\1\346\20\u013c\1\346\6\u013c\2\346\1\u013c\2\346\1\0"+
"\4\346\1\u013c\1\356\2\346\1\0\1\346\11\u013c\2\346"+
"\15\u013c\10\346\1\u013c\4\346\5\0\1\u013a\2\u013b\5\u013c"+
"\1\u013a\7\u013b\7\u013c\2\u013b\1\0\6\u013c\2\0\1\u013b"+
"\1\u013a\1\0\1\u017b\4\0\1\u013c\1\u013d\4\0\5\u013b"+
"\4\u013c\2\0\2\u013b\1\u013c\1\u013b\1\u013c\1\u013b\1\u013c"+
"\2\u013b\3\u013c\1\u013b\10\0\1\u013c\16\0\1\u017e\125\0"+
"\4\326\1\u017f\2\326\1\0\20\326\1\0\6\326\2\0"+
"\1\326\1\u012e\6\0\1\326\5\0\11\326\2\0\15\326"+
"\10\0\1\326\12\0\4\u0177\1\u0180\2\u0177\1\0\20\u0177"+
"\1\0\6\u0177\2\0\1\u0177\7\0\1\u0177\5\0\11\u0177"+
"\2\0\15\u0177\10\0\1\u0177\12\0\4\326\1\u017f\2\326"+
"\1\0\20\326\1\0\6\326\2\0\1\326\7\0\1\326"+
"\5\0\11\326\2\0\15\326\10\0\1\326\16\0\1\u0181"+
"\12\0\1\116\12\0\3\116\71\0\1\120\2\0\1\151"+
"\4\123\1\126\2\123\1\0\20\123\1\0\1\131\5\123"+
"\2\0\1\141\1\152\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\330\1\145\7\123\2\131\2\0\15\123\5\0"+
"\1\145\2\0\1\131\6\0\1\120\3\0\4\131\1\u0182"+
"\2\131\1\0\20\131\1\0\6\131\2\0\1\131\1\152"+
"\4\0\1\140\1\0\1\131\1\153\3\143\1\0\11\131"+
"\2\0\15\131\10\0\1\131\6\0\1\120\2\0\1\151"+
"\4\123\1\126\2\123\1\0\20\123\1\0\1\131\5\123"+
"\2\0\1\233\1\152\4\0\1\140\1\0\1\233\1\153"+
"\2\143\1\330\1\337\7\123\2\131\2\0\15\123\5\0"+
"\1\337\2\0\1\131\12\0\4\341\1\u0183\2\341\1\0"+
"\20\341\1\0\1\342\5\341\2\0\1\341\7\0\1\341"+
"\5\0\7\341\2\342\2\0\15\341\10\0\1\342\6\0"+
"\1\120\7\0\1\u017e\125\0\4\344\1\u0184\2\344\1\0"+
"\20\344\1\0\6\344\2\0\1\344\7\0\1\344\1\153"+
"\4\0\11\344\2\0\15\344\10\0\1\344\12\0\4\143"+
"\1\u0185\2\143\1\0\20\143\1\0\6\143\2\0\1\143"+
"\1\152\6\0\1\143\1\0\3\143\1\0\11\143\2\0"+
"\15\143\10\0\1\143\12\0\4\143\1\u0185\2\143\1\346"+
"\20\143\1\0\6\143\2\0\1\143\1\152\1\346\5\0"+
"\1\143\1\0\1\143\1\347\1\143\1\0\11\143\2\0"+
"\15\143\10\0\1\143\12\0\4\346\1\u017e\1\346\4\0"+
"\1\350\1\0\1\351\5\0\1\352\12\0\2\353\21\0"+
"\1\346\1\0\1\351\41\0\6\346\4\342\1\u0186\2\342"+
"\1\346\20\342\1\346\6\342\2\346\1\342\1\u0136\1\346"+
"\1\0\4\346\1\342\3\346\1\0\1\346\11\342\2\346"+
"\15\342\10\346\1\342\4\346\6\0\4\u0179\1\u0187\2\u0179"+
"\1\0\20\u0179\2\0\5\u0179\2\0\1\u0179\7\0\1\u0179"+
"\5\0\7\u0179\4\0\15\u0179\23\0\7\345\1\0\20\345"+
"\1\0\1\143\5\345\2\u010d\1\345\1\152\6\0\1\345"+
"\1\0\3\143\1\0\7\345\2\143\2\0\15\345\1\u010d"+
"\7\0\1\143\16\0\1\u017e\32\0\2\u0137\46\0\1\u0137"+
"\16\0\1\120\2\0\1\151\7\123\1\0\13\123\1\u0188"+
"\4\123\1\0\1\131\5\123\2\0\1\141\1\152\4\0"+
"\1\140\1\0\1\141\1\153\2\143\1\330\1\145\7\123"+
"\2\131\2\0\15\123\5\0\1\145\2\0\1\131\6\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\5\123\2\0\1\141\1\152\1\u0189\3\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\6\0\1\120\2\0"+
"\1\121\2\122\5\123\1\127\7\122\7\123\2\122\1\0"+
"\1\131\5\123\2\0\1\136\1\137\1\0\1\327\2\0"+
"\1\140\1\4\1\141\1\142\2\143\1\330\1\145\1\122"+
"\1\u018a\3\122\2\123\2\131\2\0\2\122\1\123\1\122"+
"\1\123\1\122\1\123\2\122\3\123\1\122\5\0\1\145"+
"\2\0\1\131\12\0\7\345\1\0\20\345\1\0\1\143"+
"\5\345\2\0\1\345\1\u018b\6\0\1\345\1\0\3\143"+
"\1\0\7\345\2\143\2\0\15\345\10\0\1\143\6\0"+
"\1\120\2\0\1\151\7\123\1\0\20\123\1\0\1\131"+
"\5\123\2\0\1\141\1\152\4\0\1\140\1\0\1\141"+
"\1\153\2\143\1\330\1\145\7\123\2\131\2\0\4\123"+
"\1\124\10\123\5\0\1\145\2\0\1\131\6\0\1\120"+
"\2\0\1\151\3\123\1\u018c\3\123\1\0\20\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\141\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\53\0\1\4\7\0"+
"\1\4\77\0\1\u018d\106\0\1\u018e\1\u018f\2\0\7\u018f"+
"\1\0\20\u018f\2\0\5\u018f\2\0\1\u018f\7\0\1\u018f"+
"\5\0\7\u018f\4\0\15\u018f\54\0\1\u0190\34\0\2\u0190"+
"\27\0\1\u0190\6\0\1\u0160\3\0\7\326\1\0\20\326"+
"\1\0\6\326\2\0\1\326\1\u0162\6\0\1\326\5\0"+
"\11\326\2\0\15\326\10\0\1\326\12\0\7\u0177\1\0"+
"\20\u0177\1\0\1\u0191\5\u0177\2\0\1\u0177\7\0\1\u0177"+
"\5\0\7\u0177\2\u0191\2\0\15\u0177\10\0\1\u0191\4\0"+
"\2\346\1\u0163\3\346\7\342\1\346\20\342\1\346\6\342"+
"\2\346\1\342\1\u0165\1\346\1\0\4\346\1\342\3\346"+
"\1\0\1\346\11\342\2\346\15\342\10\346\1\342\4\346"+
"\6\0\7\341\1\0\20\341\1\0\1\u0192\5\341\2\0"+
"\1\341\7\0\1\341\5\0\7\341\2\u0192\2\0\15\341"+
"\10\0\1\u0192\54\0\1\u016d\120\0\1\u0117\11\0\1\240"+
"\2\0\1\u0193\5\0\2\240\10\0\2\u0117\27\0\1\u0117"+
"\12\0\7\341\1\0\3\341\1\u0194\14\341\1\0\1\342"+
"\5\341\2\0\1\341\7\0\1\341\5\0\7\341\2\342"+
"\2\0\15\341\10\0\1\342\67\0\1\4\131\0\1\u0195"+
"\61\0\1\u0196\146\0\1\4\107\0\4\346\1\0\1\346"+
"\4\0\1\350\1\0\1\351\5\0\1\352\7\0\1\u0197"+
"\2\0\2\353\21\0\1\346\1\0\1\351\43\0\1\120"+
"\2\0\1\151\7\123\1\0\20\123\1\0\1\131\5\123"+
"\2\0\1\141\1\u0168\4\0\1\140\1\0\1\141\1\153"+
"\2\143\1\330\1\145\7\123\2\131\2\0\15\123\5\0"+
"\1\145\2\0\1\131\20\0\1\312\146\0\1\u0198\144\0"+
"\1\u0198\102\0\1\u0199\131\0\1\73\131\0\1\74\116\0"+
"\1\120\2\0\1\151\5\123\1\126\1\123\1\0\20\123"+
"\1\0\1\131\5\123\2\0\1\141\1\152\4\0\1\140"+
"\1\0\1\141\1\153\2\143\1\330\1\145\7\123\2\131"+
"\2\0\15\123\5\0\1\145\2\0\1\131\67\0\1\u019a"+
"\50\0\1\120\2\0\1\151\7\123\1\0\20\123\1\0"+
"\1\131\5\123\2\0\1\141\1\152\4\0\1\140\1\0"+
"\1\u019b\1\153\2\143\1\330\1\145\7\123\2\131\2\0"+
"\15\123\5\0\1\145\2\0\1\131\4\0\5\346\1\u017c"+
"\2\u017b\5\0\1\u017c\7\u017b\7\0\2\u017b\1\346\6\0"+
"\2\346\1\u017b\1\u017c\1\346\1\u017b\4\346\1\0\1\u017c"+
"\2\346\1\0\1\346\5\u017b\4\0\2\346\2\u017b\1\0"+
"\1\u017b\1\0\1\u017b\1\0\2\u017b\3\0\1\u017b\10\346"+
"\1\0\4\346\45\0\1\135\15\0\1\4\31\0\1\135"+
"\22\0\7\326\1\0\20\326\1\0\6\326\1\135\1\0"+
"\1\326\1\u012e\6\0\1\326\3\0\1\4\1\0\11\326"+
"\2\0\15\326\1\135\7\0\1\326\12\0\7\u0177\1\0"+
"\20\u0177\1\0\6\u0177\1\135\1\0\1\u0177\7\0\1\u0177"+
"\3\0\1\4\1\0\11\u0177\2\0\15\u0177\1\135\7\0"+
"\1\u0177\16\0\1\315\17\0\1\316\6\0\1\317\3\0"+
"\1\135\15\0\1\4\31\0\1\135\16\0\1\120\3\0"+
"\7\131\1\0\20\131\1\0\6\131\1\135\1\0\1\131"+
"\1\152\4\0\1\140\1\0\1\131\1\153\2\143\1\u019c"+
"\1\0\11\131\2\0\15\131\1\135\7\0\1\131\4\0"+
"\6\346\7\342\1\346\20\342\1\346\6\342\1\135\1\346"+
"\1\342\1\u0136\1\346\1\0\4\346\1\342\3\346\1\u019d"+
"\1\346\11\342\2\346\15\342\1\135\7\346\1\342\4\346"+
"\6\0\7\344\1\0\20\344\1\0\6\344\1\135\1\0"+
"\1\344\7\0\1\344\1\153\2\0\1\4\1\0\11\344"+
"\2\0\15\344\1\135\7\0\1\344\12\0\7\143\1\0"+
"\20\143\1\0\6\143\1\135\1\0\1\143\1\152\6\0"+
"\1\143\1\0\2\143\1\u019c\1\0\11\143\2\0\15\143"+
"\1\135\7\0\1\143\4\0\6\346\7\342\1\346\20\342"+
"\1\346\6\342\1\135\1\346\1\342\1\u0136\1\346\1\0"+
"\4\346\1\342\3\346\1\4\1\346\11\342\2\346\15\342"+
"\1\135\7\346\1\342\4\346\45\0\1\135\15\0\1\u019e"+
"\31\0\1\135\16\0\1\120\2\0\1\151\6\123\1\u0139"+
"\1\0\20\123\1\0\1\131\5\123\2\0\1\141\1\152"+
"\4\0\1\140\1\0\1\141\1\153\2\143\1\330\1\145"+
"\7\123\2\131\2\0\15\123\5\0\1\145\2\0\1\131"+
"\6\0\1\u019f\131\0\1\120\2\0\1\121\2\122\5\123"+
"\1\127\7\122\7\123\2\122\1\0\1\131\5\123\1\135"+
"\1\0\1\136\1\137\1\0\1\327\2\0\1\140\1\4"+
"\1\141\1\142\2\143\1\332\1\145\5\122\2\123\2\131"+
"\2\0\2\122\1\123\1\122\1\123\1\122\1\123\2\122"+
"\3\123\1\122\1\135\4\0\1\145\2\0\1\131\12\0"+
"\7\341\1\0\3\341\1\u01a0\14\341\1\0\1\342\5\341"+
"\2\0\1\341\7\0\1\341\5\0\7\341\2\342\2\0"+
"\15\341\10\0\1\342\6\0\1\120\2\0\1\151\4\123"+
"\1\u016f\2\123\1\0\20\123\1\0\1\131\5\123\2\0"+
"\1\141\1\152\4\0\1\140\1\0\1\141\1\153\2\143"+
"\1\330\1\145\7\123\2\131\2\0\15\123\5\0\1\145"+
"\2\0\1\131\33\0\1\u01a1\105\0\1\u018f\2\0\7\u018f"+
"\1\0\20\u018f\2\0\5\u018f\2\0\1\u018f\7\0\1\u018f"+
"\5\0\7\u018f\4\0\15\u018f\15\0\4\u018f\1\u015e\125\u018f"+
"\37\0\1\u01a2\34\0\2\u01a2\27\0\1\u01a2\12\0\7\u0177"+
"\1\0\20\u0177\1\0\1\u01a3\5\u0177\2\0\1\u0177\7\0"+
"\1\u0177\5\0\7\u0177\2\u01a3\2\0\15\u0177\10\0\1\u01a3"+
"\4\0\6\346\7\342\1\346\20\342\1\346\1\u01a4\5\342"+
"\2\346\1\342\1\u0136\1\346\1\0\4\346\1\342\3\346"+
"\1\0\1\346\7\342\2\u01a4\2\346\15\342\10\346\1\u01a4"+
"\4\346\37\0\1\u01a5\34\0\2\u01a5\17\0\1\u01a6\7\0"+
"\1\u01a5\4\0\6\346\7\342\1\346\20\342\1\346\6\342"+
"\2\346\1\342\1\u0136\1\346\1\0\4\346\1\342\3\346"+
"\1\u01a7\1\346\11\342\2\346\15\342\10\346\1\342\4\346"+
"\115\0\1\u01a8\56\0\1\4\120\0\1\u0196\142\0\1\u0174"+
"\74\0\1\151\7\u0199\1\0\20\u0199\2\0\5\u0199\2\0"+
"\1\u0199\7\0\1\u0199\3\0\1\u01a9\1\145\7\u0199\4\0"+
"\15\u0199\5\0\1\145\7\0\6\346\7\u0179\1\346\20\u0179"+
"\1\346\1\0\5\u0179\2\346\1\u0179\1\u0136\1\346\1\0"+
"\4\346\1\u0179\3\346\1\0\1\346\7\u0179\2\0\2\346"+
"\15\u0179\10\346\1\0\4\346\2\0\1\120\2\0\1\151"+
"\7\123\1\0\20\123\1\0\1\131\5\123\2\0\1\233"+
"\1\152\4\0\1\140\1\0\1\233\1\153\2\143\1\330"+
"\1\u01aa\7\123\2\131\2\0\15\123\5\0\1\u01aa\2\0"+
"\1\131\6\0\1\u01ab\127\0\6\346\7\342\1\346\20\342"+
"\1\346\6\342\2\346\1\342\1\u0136\1\346\1\0\4\346"+
"\1\342\3\346\1\u01ac\1\346\11\342\2\346\15\342\10\346"+
"\1\342\4\346\73\0\1\4\75\0\1\u01ad\34\0\2\u01ad"+
"\27\0\1\u01ad\12\0\7\u0177\1\0\20\u0177\1\0\1\u01ae"+
"\5\u0177\2\0\1\u0177\7\0\1\u0177\5\0\7\u0177\2\u01ae"+
"\2\0\15\u0177\10\0\1\u01ae\4\0\6\346\7\342\1\346"+
"\20\342\1\346\1\u01af\5\342\2\346\1\342\1\u0136\1\346"+
"\1\0\4\346\1\342\3\346\1\0\1\346\7\342\2\u01af"+
"\2\346\15\342\10\346\1\u01af\4\346\37\0\1\u01b0\34\0"+
"\2\u01b0\27\0\1\u01b0\43\0\1\u01a5\34\0\2\u01a5\27\0"+
"\1\u01a5\12\0\7\u0179\1\0\20\u0179\2\0\5\u0179\2\0"+
"\1\u0179\7\0\1\u0179\5\0\1\u0179\1\u01b1\5\u0179\4\0"+
"\15\u0179\100\0\1\u01b2\63\0\1\346\33\0\1\346\10\0"+
"\1\346\47\0\1\u01b3\1\0\2\u01b3\1\0\40\u01b3\2\0"+
"\4\u01b3\2\0\21\u01b3\1\0\16\u01b3\1\0\5\u01b3\2\0"+
"\4\u01b3\7\0\7\u0179\1\0\20\u0179\2\0\5\u0179\2\0"+
"\1\u0179\7\0\1\u0179\5\0\1\u0179\1\u01b4\5\u0179\4\0"+
"\7\u0179\1\u01b1\5\u0179\54\0\1\4\34\0\2\4\27\0"+
"\1\4\12\0\7\u0177\1\0\20\u0177\1\0\1\u01b5\5\u0177"+
"\2\0\1\u0177\7\0\1\u0177\5\0\7\u0177\2\u01b5\2\0"+
"\15\u0177\10\0\1\u01b5\4\0\6\346\7\342\1\346\20\342"+
"\1\346\1\u01b6\5\342\2\346\1\342\1\u0136\1\346\1\0"+
"\4\346\1\342\3\346\1\0\1\346\7\342\2\u01b6\2\346"+
"\15\342\10\346\1\u01b6\4\346\37\0\1\u01b7\34\0\2\u01b7"+
"\27\0\1\u01b7\51\0\2\u0137\14\0\1\u019e\31\0\1\u0137"+
"\131\0\1\u01b8\14\0\1\u01b9\1\0\1\u01b9\1\u01b3\1\0"+
"\40\u01b9\2\0\1\u01b9\1\u01b3\2\u01b9\2\0\5\u01b9\2\u01b3"+
"\12\u01b9\1\0\1\u01b3\15\u01b9\1\0\5\u01b9\2\0\1\u01b9"+
"\2\u01b3\1\u01b9\64\0\1\u01ba\116\0\1\u01bb\44\0\1\u01bb"+
"\14\0\6\346\7\u0179\1\346\20\u0179\1\346\1\0\5\u0179"+
"\2\346\1\u0179\1\u0136\1\346\1\0\4\346\1\u0179\3\346"+
"\1\0\1\346\1\u0179\1\u01bc\5\u0179\2\0\2\346\15\u0179"+
"\10\346\1\0\4\346\37\0\1\u01bd\34\0\2\u01bd\27\0"+
"\1\u01bd\67\0\1\u01be\105\0\1\u01bf\34\0\2\u01bf\27\0"+
"\1\u01bf\4\0\6\346\7\u0179\1\346\4\u0179\1\u01b1\13\u0179"+
"\1\346\1\0\5\u0179\2\346\1\u0179\1\u0136\1\346\1\0"+
"\4\346\1\u0179\3\346\1\0\1\346\7\u0179\2\0\2\346"+
"\15\u0179\10\346\1\0\4\346\37\0\1\u01c0\34\0\2\u01c0"+
"\27\0\1\u01c0\4\0";
private static int [] yy_unpack_yytrans() {
int [] result = new int[36180];
int offset = 0;
offset = yy_unpack_yytrans(yytrans_packed0, offset, result);
return result;
}
private static int yy_unpack_yytrans(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
value--;
do result[j++] = value; while (--count > 0);
}
return j;
}
/* error codes */
private static final int YY_UNKNOWN_ERROR = 0;
private static final int YY_NO_MATCH = 1;
private static final int YY_PUSHBACK_2BIG = 2;
/* error messages for the codes above */
private static final String YY_ERROR_MSG[] = {
"Unkown internal scanner error",
"Error: could not match input",
"Error: pushback value was too large"
};
/**
* YY_ATTRIBUTE[aState] contains the attributes of state aState
*/
private static final int [] YY_ATTRIBUTE = yy_unpack_YY_ATTRIBUTE();
private static final String YY_ATTRIBUTE_packed0 =
"\1\1\1\11\1\1\1\11\1\1\23\3\2\1\2\3"+
"\1\1\1\11\1\3\4\1\1\11\1\1\1\3\3\1"+
"\4\3\1\1\10\3\4\1\1\3\3\11\23\0\5\3"+
"\1\0\1\3\1\1\3\3\1\5\1\3\2\0\1\3"+
"\2\0\1\3\1\0\2\3\4\0\1\2\3\0\1\3"+
"\1\1\1\5\1\3\4\0\1\3\1\0\14\3\1\0"+
"\2\3\1\0\11\3\1\0\1\1\3\0\4\3\2\0"+
"\1\1\2\0\1\1\1\3\2\0\1\2\11\0\3\3"+
"\1\0\4\3\1\1\21\3\1\15\14\0\2\1\1\2"+
"\6\3\1\0\1\3\2\2\2\1\1\2\1\15\1\5"+
"\4\0\1\3\2\5\7\0\1\1\1\3\1\1\1\2"+
"\1\1\1\0\2\2\5\3\1\2\4\3\1\5\2\3"+
"\1\1\2\0\3\3\2\1\1\2\1\1\3\0\1\3"+
"\1\1\4\0\1\1\4\3\1\1\3\3\1\11\7\0"+
"\2\3\1\15\1\5\1\3\1\5\1\0\2\5\1\1"+
"\1\3\1\0\2\2\1\0\2\7\1\0\1\1\3\0"+
"\1\3\1\1\1\3\4\0\1\2\1\0\1\5\1\2"+
"\1\5\1\0\1\1\1\3\1\5\3\3\1\1\2\3"+
"\3\0\1\15\1\5\1\0\1\1\1\0\1\5\1\2"+
"\1\5\3\0\1\1\2\0\1\1\1\2\1\0\1\3"+
"\1\1\6\0\1\1\1\3\1\0\1\3\1\2\2\5"+
"\1\2\2\3\1\2\1\3\1\2\1\3\3\2\1\3"+
"\1\0\1\3\1\0\1\3\4\0\1\1\1\2\1\1"+
"\1\2\1\1\3\0\1\3\1\2\1\3\2\1\1\3"+
"\1\0\1\2\1\0\2\1\1\3\4\0\1\2\1\1"+
"\2\0\2\1\1\3\1\0\1\2\1\1\2\0\1\1"+
"\1\3\2\0\1\1\1\2\3\0\1\2\2\0";
private static int [] yy_unpack_YY_ATTRIBUTE() {
int [] result = new int[448];
int offset = 0;
offset = yy_unpack_YY_ATTRIBUTE(YY_ATTRIBUTE_packed0, offset, result);
return result;
}
private static int yy_unpack_YY_ATTRIBUTE(String packed, int offset, int [] result) {
int i = 0; /* index in packed string */
int j = offset; /* index in unpacked array */
int l = packed.length();
while (i < l) {
int count = packed.charAt(i++);
int value = packed.charAt(i++);
do result[j++] = value; while (--count > 0);
}
return j;
}
/** the input device */
private java.io.Reader yy_reader;
/** the current state of the DFA */
private int yy_state;
/** the current lexical state */
protected int yy_lexical_state = YYINITIAL;
/** this buffer contains the current text to be matched and is
the source of the yytext() string */
protected char yy_buffer[] = new char[YY_BUFFERSIZE];
/** the textposition at the last accepting state */
protected int yy_markedPos;
/** the textposition at the last state to be included in yytext */
protected int yy_pushbackPos;
/** the current text position in the buffer */
protected int yy_currentPos;
/** startRead marks the beginning of the yytext() string in the buffer */
protected int yy_startRead;
/** endRead marks the last character in the buffer, that has been read
from input */
protected int yy_endRead;
/** number of newlines encountered up to the start of the matched text */
protected int yyline;
/** the number of characters up to the start of the matched text */
protected int yychar;
/**
* the number of characters from the last newline up to the start of the
* matched text
*/
protected int yycolumn;
/**
* yy_atBOL == true <=> the scanner is currently at the beginning of a line
*/
protected boolean yy_atBOL = true;
/** yy_atEOF == true <=> the scanner is at the EOF */
protected boolean yy_atEOF;
/* user code: */
final static public String opendblquote = "``";
final static public String closedblquote = "''";
final static public String openparen = "-LRB-";
final static public String closeparen = "-RRB-";
final static public String openbrace = "-LCB-";
final static public String closebrace = "-RCB-";
public static final String ptbmdash = "--";
public static final String cr = "*CR*"; // js: for tokenizing carriage returns
/** This quotes a character with a backslash, but doesn't do it
* if the character is already preceded by a backslash
*/
static private String delimit (String s, char c) {
int i = s.indexOf(c);
while (i != -1) {
if (i == 0 || s.charAt(i - 1) != '\\') {
s = s.substring(0, i) + "\\" + s.substring(i);
i = s.indexOf(c, i + 2);
} else {
i = s.indexOf(c, i + 1);
}
}
return s;
}
/**
* Creates a new scanner
* There is also a java.io.InputStream version of this constructor.
*
* @param in the java.io.Reader to read input from.
*/
PTBLexer(java.io.Reader in) {
this.yy_reader = in;
}
/**
* Creates a new scanner.
* There is also java.io.Reader version of this constructor.
*
* @param in the java.io.Inputstream to read input from.
*/
PTBLexer(java.io.InputStream in) {
this(new java.io.InputStreamReader(in));
}
/**
* Unpacks the compressed character translation table.
*
* @param packed the packed character translation table
* @return the unpacked character translation table
*/
private static char [] yy_unpack_cmap(String packed) {
char [] map = new char[0x10000];
int i = 0; /* index in packed string */
int j = 0; /* index in unpacked array */
while (i < 198) {
int count = packed.charAt(i++);
char value = packed.charAt(i++);
do map[j++] = value; while (--count > 0);
}
return map;
}
/**
* Refills the input buffer.
*
* @return false
, iff there was new input.
*
* @exception IOException if any I/O-Error occurs
*/
private boolean yy_refill() throws java.io.IOException {
if (yy_reader==null) return true;
/* first: make room (if you can) */
if (yy_startRead > 0) {
System.arraycopy(yy_buffer, yy_startRead,
yy_buffer, 0,
yy_endRead-yy_startRead);
/* translate stored positions */
yy_endRead-= yy_startRead;
yy_currentPos-= yy_startRead;
yy_markedPos-= yy_startRead;
yy_pushbackPos-= yy_startRead;
yy_startRead = 0;
}
/* is the buffer big enough? */
if (yy_currentPos >= yy_buffer.length) {
/* if not: blow it up */
char newBuffer[] = new char[yy_currentPos*2];
System.arraycopy(yy_buffer, 0, newBuffer, 0, yy_buffer.length);
yy_buffer = newBuffer;
}
/* finally: fill the buffer with new input */
int numRead = yy_reader.read(yy_buffer, yy_endRead,
yy_buffer.length-yy_endRead);
if (numRead < 0) {
return true;
}
else {
yy_endRead+= numRead;
return false;
}
}
/**
* Closes the input stream.
*/
public final void yyclose() throws java.io.IOException {
yy_atEOF = true; /* indicate end of file */
yy_endRead = yy_startRead; /* invalidate buffer */
if (yy_reader != null)
yy_reader.close();
}
/**
* Resets the scanner to read from a new input stream.
* Does not close the old reader.
*
* All internal variables are reset, the old input stream
* cannot be reused (internal buffer is discarded and lost).
* Lexical state is set to YY_INITIAL.
*
* @param reader the new input stream
*/
public final void yyreset(java.io.Reader reader) throws java.io.IOException {
yy_reader = reader;
yy_atBOL = true;
yy_atEOF = false;
yy_endRead = yy_startRead = 0;
yy_currentPos = yy_markedPos = yy_pushbackPos = 0;
yyline = yychar = yycolumn = 0;
yy_lexical_state = YYINITIAL;
}
/**
* Returns the current lexical state.
*/
public final int yystate() {
return yy_lexical_state;
}
/**
* Enters a new lexical state
*
* @param newState the new lexical state
*/
public final void yybegin(int newState) {
yy_lexical_state = newState;
}
/**
* Returns the text matched by the current regular expression.
*/
public final String yytext() {
return new String( yy_buffer, yy_startRead, yy_markedPos-yy_startRead );
}
/**
* Returns the character at position pos from the
* matched text.
*
* It is equivalent to yytext().charAt(pos), but faster
*
* @param pos the position of the character to fetch.
* A value from 0 to yylength()-1.
*
* @return the character at position pos
*/
public final char yycharat(int pos) {
return yy_buffer[yy_startRead+pos];
}
/**
* Returns the length of the matched text region.
*/
public final int yylength() {
return yy_markedPos-yy_startRead;
}
/**
* Reports an error that occured while scanning.
*
* In a wellformed scanner (no or only correct usage of
* yypushback(int) and a match-all fallback rule) this method
* will only be called with things that "Can't Possibly Happen".
* If this method is called, something is seriously wrong
* (e.g. a JFlex bug producing a faulty scanner etc.).
*
* Usual syntax/scanner level error handling should be done
* in error fallback rules.
*
* @param errorCode the code of the errormessage to display
*/
private void yy_ScanError(int errorCode) {
String message;
try {
message = YY_ERROR_MSG[errorCode];
}
catch (ArrayIndexOutOfBoundsException e) {
message = YY_ERROR_MSG[YY_UNKNOWN_ERROR];
}
throw new Error(message);
}
/**
* Pushes the specified amount of characters back into the input stream.
*
* They will be read again by then next call of the scanning method
*
* @param number the number of characters to be read again.
* This number must not be greater than yylength()!
*/
public void yypushback(int number) {
if ( number > yylength() )
yy_ScanError(YY_PUSHBACK_2BIG);
yy_markedPos -= number;
}
/**
* Resumes scanning until the next regular expression is matched,
* the end of input is encountered or an I/O-Error occurs.
*
* @return the next token
* @exception IOException if any I/O-Error occurs
*/
public String next() throws java.io.IOException {
int yy_input;
int yy_action;
// cached fields:
int yy_currentPos_l;
int yy_markedPos_l;
int yy_endRead_l = yy_endRead;
char [] yy_buffer_l = yy_buffer;
char [] yycmap_l = yycmap;
int [] yytrans_l = yytrans;
int [] yy_rowMap_l = yy_rowMap;
int [] yy_attr_l = YY_ATTRIBUTE;
int yy_pushbackPos_l = yy_pushbackPos = -1;
boolean yy_was_pushback;
while (true) {
yy_markedPos_l = yy_markedPos;
yy_action = -1;
yy_currentPos_l = yy_currentPos = yy_startRead = yy_markedPos_l;
yy_state = yy_lexical_state;
yy_was_pushback = false;
yy_forAction: {
while (true) {
if (yy_currentPos_l < yy_endRead_l)
yy_input = yy_buffer_l[yy_currentPos_l++];
else if (yy_atEOF) {
yy_input = YYEOF;
break yy_forAction;
}
else {
// store back cached positions
yy_currentPos = yy_currentPos_l;
yy_markedPos = yy_markedPos_l;
yy_pushbackPos = yy_pushbackPos_l;
boolean eof = yy_refill();
// get translated positions and possibly new buffer
yy_currentPos_l = yy_currentPos;
yy_markedPos_l = yy_markedPos;
yy_buffer_l = yy_buffer;
yy_endRead_l = yy_endRead;
yy_pushbackPos_l = yy_pushbackPos;
if (eof) {
yy_input = YYEOF;
break yy_forAction;
}
else {
yy_input = yy_buffer_l[yy_currentPos_l++];
}
}
int yy_next = yytrans_l[ yy_rowMap_l[yy_state] + yycmap_l[yy_input] ];
if (yy_next == -1) break yy_forAction;
yy_state = yy_next;
int yy_attributes = yy_attr_l[yy_state];
if ( (yy_attributes & 2) == 2 )
yy_pushbackPos_l = yy_currentPos_l;
if ( (yy_attributes & 1) == 1 ) {
yy_was_pushback = (yy_attributes & 4) == 4;
yy_action = yy_state;
yy_markedPos_l = yy_currentPos_l;
if ( (yy_attributes & 8) == 8 ) break yy_forAction;
}
}
}
// store back cached position
yy_markedPos = yy_markedPos_l;
if (yy_was_pushback)
yy_markedPos = yy_pushbackPos_l;
switch (yy_action < 0 ? yy_action : YY_ACTION[yy_action]) {
case 10:
{ return closedblquote; }
case 18: break;
case 9:
{ return closeparen; }
case 19: break;
case 4:
{ return yytext(); }//String word = Americanize.americanize(yytext());
//return word; }
case 20: break;
case 13:
{ return opendblquote; }
case 21: break;
case 14:
{ return (delimit(yytext(), '/')); }
case 22: break;
case 5:
{ /* ignore */ }
case 23: break;
case 17:
{ String fred = yytext();
yypushback(1);
return fred; }
case 24: break;
case 11:
{ return openbrace; }
case 25: break;
case 3:
{ return yytext(); }
case 26: break;
case 7:
{ if (yylength() >= 3 && yylength() <= 4) {
return ptbmdash;
} else {
return yytext();
} }
case 27: break;
case 8:
{ return openparen; }
case 28: break;
case 2:
{ /*System.err.println(yytext());*/ }
case 29: break;
case 15:
{ return ptbmdash; }
case 30: break;
case 1:
{ return (delimit(yytext(), '*')); }
case 31: break;
case 6:
{ return(cr); }
case 32: break;
case 12:
{ return closebrace; }
case 33: break;
case 16:
{ yypushback(1); return yytext(); }
case 34: break;
default:
if (yy_input == YYEOF && yy_startRead == yy_currentPos) {
yy_atEOF = true;
{ return null; }
}
else {
yy_ScanError(YY_NO_MATCH);
}
}
}
}
/**
* Runs the scanner on input files.
*
* This is a standalone scanner, it will print any unmatched
* text to System.out unchanged.
*
* @param argv the command line, contains the filenames to run
* the scanner on.
*/
public static void main(String argv[]) {
if (argv.length == 0) {
System.out.println("Usage : java PTBLexer ");
}
else {
for (int i = 0; i < argv.length; i++) {
PTBLexer scanner = null;
try {
scanner = new PTBLexer( new java.io.FileReader(argv[i]) );
while ( !scanner.yy_atEOF ) scanner.next();
}
catch (java.io.FileNotFoundException e) {
System.out.println("File not found : \""+argv[i]+"\"");
}
catch (java.io.IOException e) {
System.out.println("IO error scanning file \""+argv[i]+"\"");
System.out.println(e);
}
catch (Exception e) {
System.out.println("Unexpected exception:");
e.printStackTrace();
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy