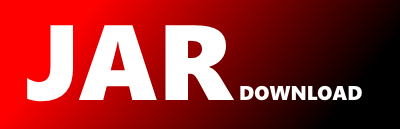
edu.berkeley.nlp.util.AbstractTMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
import java.io.*;
import java.util.*;
/**
* Just a dummy class.
* TODO: move common functionality here.
*/
public abstract class AbstractTMap implements Serializable {
protected static final long serialVersionUID = 42;
public static class Functionality implements Serializable {
public T[] createArray(int n) { return (T[])(new Object[n]); }
public T intern(T x) { return x; } // Override to get desired behavior, e.g., interning
}
public static class ObjectFunctionality extends Functionality
© 2015 - 2025 Weber Informatics LLC | Privacy Policy