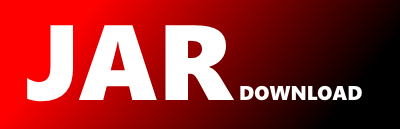
edu.berkeley.nlp.util.BoundedList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.List;
/**
* List which returns special boundary symbols hwen get() is called outside the
* range of the list.
*
* @author Dan Klein
*/
public class BoundedList extends AbstractList {
private E leftBoundary;
private E rightBoundary;
private List list;
/**
* Returns the object at the given index, provided the index is between 0
* (inclusive) and size() (exclusive). If the index is < 0, then a left
* boundary object is returned. If the index is >= size(), a right boundary
* object is returned. The default boundary objects are both null, unless
* other objects are specified on construction.
*/
@Override
public E get(int index) {
if (index < 0) return leftBoundary;
if (index >= list.size()) return rightBoundary;
return list.get(index);
}
@Override
public int size() {
return list.size();
}
public BoundedList(List list, E leftBoundary, E rightBoundary) {
this.list = list;
this.leftBoundary = leftBoundary;
this.rightBoundary = rightBoundary;
}
public BoundedList(List list, E boundary) {
this(list, boundary, boundary);
}
public BoundedList(List list) {
this(list, null);
}
@Override
public List subList(int fromIndex, int toIndex)
{
List retVal = new ArrayList(toIndex - fromIndex);
for (int i = fromIndex; i < toIndex; ++i)
{
retVal.add(get(i));
}
return retVal;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy