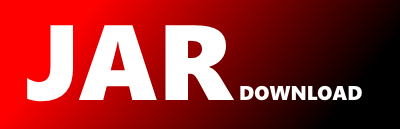
edu.berkeley.nlp.util.BufferedIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Queue;
public class BufferedIterator implements Iterator {
private Iterator it ;
private Queue buffer ;
private int numToBuffer ;
public BufferedIterator(Iterator it, int numToBuffer) {
this.it = it;
this.buffer = new LinkedList();
this.numToBuffer = numToBuffer;
refill();
}
public BufferedIterator(Iterator it) {
this(it,100);
}
public boolean hasNext() {
// TODO Auto-generated method stub
if (!buffer.isEmpty()) return false;
return it.hasNext();
}
public T next() {
// TODO Auto-generated method stub
if (buffer.isEmpty()) {
refill();
}
if (buffer.isEmpty()) {
throw new RuntimeException();
}
return buffer.remove();
}
private void refill( ) {
for (int i=0; i < numToBuffer; ++i) {
if (it.hasNext()) {
buffer.add(it.next());
}
}
}
public void remove() {
// TODO Auto-generated method stub
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy