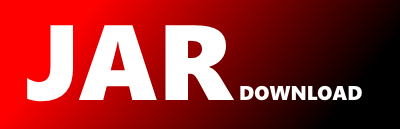
edu.berkeley.nlp.util.ConcatenationIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
import java.util.*;
/**
* Concatenates an iterator over iterators into one long iterator.
*
* @author Dan Klein
*/
public class ConcatenationIterator implements Iterator {
Iterator> sourceIterators;
Iterator currentIterator;
Iterator lastIteratorToReturn;
public boolean hasNext() {
if (currentIterator.hasNext())
return true;
return false;
}
public E next() {
if (currentIterator.hasNext()) {
E e = currentIterator.next();
lastIteratorToReturn = currentIterator;
advance();
return e;
}
throw new NoSuchElementException();
}
private void advance() {
while (! currentIterator.hasNext() && sourceIterators.hasNext()) {
currentIterator = sourceIterators.next();
}
}
public void remove() {
if (lastIteratorToReturn == null)
throw new IllegalStateException();
currentIterator.remove();
}
public ConcatenationIterator(Iterator> sourceIterators) {
this.sourceIterators = sourceIterators;
this.currentIterator = (new ArrayList()).iterator();
this.lastIteratorToReturn = null;
advance();
}
public ConcatenationIterator(Collection> iteratorCollection) {
this(iteratorCollection.iterator());
}
public static void main(String[] args) {
List list0 = Collections.emptyList();
List list1 = Arrays.asList("a b c d".split(" "));
List list2 = Arrays.asList("e f".split(" "));
List> iterators = new ArrayList>();
iterators.add(list1.iterator());
iterators.add(list0.iterator());
iterators.add(list2.iterator());
iterators.add(list0.iterator());
Iterator iterator = new ConcatenationIterator(iterators);
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy