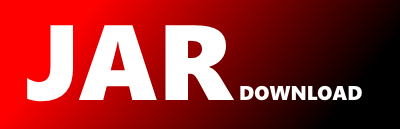
edu.berkeley.nlp.util.Iterables Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
/**
* Created by IntelliJ IDEA.
* User: aria42
* Date: Nov 15, 2008
*/
public class Iterables {
public static Iterable> zip(final Iterable s, final Iterable t) {
return new Iterable>() {
public Iterator> iterator() {
return Iterators.zip(s.iterator(),t.iterator());
}
};
}
public static Iterable concat(final Iterable...iterables) {
return new ConcatenationIterable(iterables);
}
public static int size(Iterable iterable) {
if (Collection.class.isInstance(iterable)) {
return ((Collection) iterable).size();
}
int count = 0;
for (T t : iterable) {
count++;
}
return count;
}
public static List fillList(Iterable iterable) {
return Iterators.fillList(iterable.iterator());
}
public static boolean isEmpty(Iterable iterable) {
return Iterables.size(iterable) == 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy