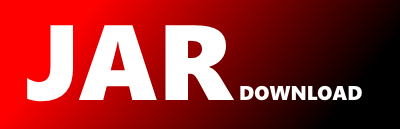
edu.berkeley.nlp.util.MapUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
import java.util.*;
public class MapUtils {
// One-level hash maps
public static boolean contains(Map map, S key) {
return map != null && map.containsKey(key);
}
public static T get(Map map, S key, T defaultVal) {
return map == null || !map.containsKey(key) ? defaultVal : map.get(key);
}
public static T getMut(Map map, S key, T defaultVal) {
if(!map.containsKey(key)) {
map.put(key, defaultVal); // Mutate
return defaultVal;
}
return map.get(key);
}
public static boolean putIfAbsent(Map map, S key, T val) {
if (map.containsKey(key)) return false;
map.put(key, val);
return true;
}
public static void set(Map map, S key, T val) {
map.put(key, val);
}
public static void incr(Map map, S key, int dVal) {
if(!map.containsKey(key)) map.put(key, dVal);
else map.put(key, map.get(key) + dVal);
}
public static void incr(Map map, S key) {
incr(map, key, 1);
}
public static void incr(Map map, S key, double dVal) {
if(!map.containsKey(key)) map.put(key, dVal);
else map.put(key, map.get(key) + dVal);
}
// Two-level hash maps
public static boolean contains(Map> map, S key1, T key2) {
if(map == null) return false;
Map m = map.get(key1);
return m != null && m.containsKey(key2);
}
public static U get(Map> map, S key1, T key2, U defaultVal) {
if(map == null || !map.containsKey(key1)) return defaultVal;
Map m = map.get(key1);
return m == null || !m.containsKey(key2) ? defaultVal : m.get(key2);
}
public static U getMut(Map> map, S key1, T key2, U defaultVal) {
Map m = map.get(key1);
if(m == null) {
map.put(key1, m = new HashMap());
m.put(key2, defaultVal);
return defaultVal;
}
else if(!m.containsKey(key2)) {
m.put(key2, defaultVal);
return defaultVal;
}
return m.get(key2);
}
public static void add(Map> map, S key1, T key2) {
Set s = map.get(key1);
if(s == null) map.put(key1, s = new HashSet());
s.add(key2);
}
public static void set(Map> map, S key1, T key2, U val) {
Map m = map.get(key1);
if(m == null) map.put(key1, m = new HashMap());
m.put(key2, val);
}
public static void incr(Map> map, S key1, T key2, int dVal) {
Map m = map.get(key1);
if(m == null) {
map.put(key1, m = new HashMap());
m.put(key2, dVal);
}
else if(!m.containsKey(key2))
m.put(key2, dVal);
else
m.put(key2, m.get(key2) + dVal);
}
public static void incr(Map> map, S key1, T key2) {
incr(map, key1, key2, 1);
}
public static void incr(Map> map, S key1, T key2, double dVal) {
Map m = map.get(key1);
if(m == null) {
map.put(key1, m = new HashMap());
m.put(key2, dVal);
}
else if(!m.containsKey(key2))
m.put(key2, dVal);
else
m.put(key2, m.get(key2) + dVal);
}
// Create a list if it doesn't exist
public static List getListMut(Map> map, S key) {
List list = map.get(key);
if(list == null)
map.put(key, list = new ArrayList());
return list;
}
// Hard operations
// Wrapper for operations on maps and sets
public static T getHard(Map map, S key) {
T value = map.get(key);
if(value == null) throw new RuntimeException("Doesn't contain key: " + key);
return value;
}
public static void putHard(Map map, S key, T value) {
if(map.containsKey(key)) throw new RuntimeException("Already contains key; " + key);
map.put(key, value);
}
public static T removeHard(Map map, S key) {
T value = map.remove(key);
if(value == null) throw new RuntimeException("Doesn't contain key");
return value;
}
public static void addHard(Set set, S key) {
if(set.contains(key)) throw new RuntimeException("Already contains key");
set.add(key);
}
public static void removeHard(Set set, S key) {
if(!set.remove(key)) throw new RuntimeException("Doesn't contain key");
}
// Print out the top k values a hash table sorted by descending value
// Should only take O(k \log n) time,
// but right now the implementation is slow
public static PriorityQueue toPriorityQueue(Map map) {
PriorityQueue pq = new PriorityQueue();
for(Map.Entry e : map.entrySet())
pq.add(e.getKey(), e.getValue());
return pq;
}
public static PriorityQueue toPriorityQueue(TDoubleMap map) {
PriorityQueue pq = new PriorityQueue();
for(TDoubleMap.Entry e : map)
pq.add(e.getKey(), e.getValue());
return pq;
}
public static String topNToString(TDoubleMap map, int numTop) {
return topNToString(toPriorityQueue(map), numTop);
}
public static String topNToString(Map map, int numTop) {
return topNToString(toPriorityQueue(map), numTop);
}
public static String topNToString(PriorityQueue pq, int numTop) {
StringBuilder sb = new StringBuilder();
sb.append('{');
for(Pair pair : getTopN(pq, numTop)) {
Object key = pair.getFirst();
double value = pair.getSecond();
sb.append(' ');
sb.append(key);
sb.append(':');
sb.append(Fmt.D(value));
}
if(pq.size() > numTop)
sb.append(" ...("+(pq.size()-numTop)+ " more)");
sb.append(" }");
return sb.toString();
}
// Return a list of the top n elements in the following structures
public static List> getTopN(Map map, int n) {
return getTopN(toPriorityQueue(map), n);
}
public static List> getTopN(TDoubleMap map, int n) {
return getTopN(toPriorityQueue(map), n);
}
public static List> getTopN(PriorityQueue pq, int n) {
List> list = new ArrayList>();
for(int i = 0; i < n && pq.hasNext(); i++) {
double priority = pq.getPriority();
T element = pq.next();
list.add(new Pair(element, priority));
}
return list;
}
public static Map compose(Map m1, Map m2, Map mapToFill) {
for (Map.Entry entry: m1.entrySet()) {
V val = m2.get(entry.getValue());
if (val != null)
mapToFill.put(entry.getKey(), val);
}
return mapToFill;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy